Why Your Coding Speed Isn’t as Important as You Think
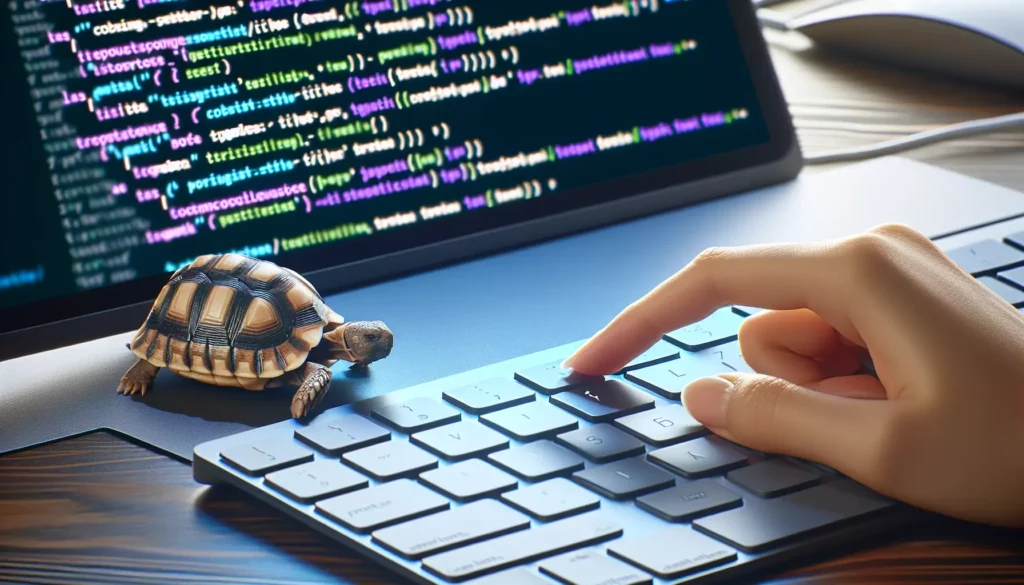
In the competitive world of software development, there’s a persistent myth that coding speed is the ultimate measure of a programmer’s worth. Many aspiring developers worry they’re not typing fast enough or solving problems quickly enough compared to their peers. Tech interviews that emphasize rapid problem-solving under time constraints only reinforce this anxiety.
But here’s the truth: your coding speed isn’t nearly as important as you might think.
While efficiency certainly matters, the obsession with raw typing speed or how quickly you can implement a solution often distracts from the qualities that truly make exceptional developers. In this comprehensive guide, we’ll explore why coding speed is overrated and what skills actually deserve your attention for long-term success.
The Myth of the Lightning-Fast Coder
The image of the “10x developer” furiously typing away, producing thousands of lines of code per day has become something of a legend in the tech industry. This archetype suggests that elite programmers are those who can implement solutions at breakneck speeds, outpacing their colleagues by orders of magnitude.
This myth is perpetuated by several factors:
- Timed coding interviews that reward quick solutions over thoughtful ones
- Hackathons where speed is essential to producing a working demo
- Productivity metrics that sometimes equate lines of code with output
- Competitive programming where the fastest correct solution wins
The reality, however, is far more nuanced. Professional software development is a marathon, not a sprint. Most experienced engineers and hiring managers understand that sustainable pace and quality trump raw speed in almost every real-world scenario.
Why Coding Speed Can Be Misleading
Speed Often Trades Off With Quality
When developers rush to implement solutions, they frequently make mistakes. These errors might include:
- Overlooking edge cases
- Introducing subtle bugs
- Creating security vulnerabilities
- Writing unmaintainable code
- Missing opportunities for optimization
The time saved by coding quickly is often lost many times over in debugging, refactoring, and fixing production issues. As the old programming adage goes: “Weeks of coding can save hours of planning.”
The Illusion of Productivity
Fast coding can create an illusion of productivity that doesn’t translate to actual value. Consider these scenarios:
- A developer rapidly implements the wrong solution to a problem
- A team quickly builds a feature that users don’t actually need
- A programmer writes verbose code that could be more elegantly expressed in fewer lines
In each case, speed led to wasted effort rather than meaningful progress. As software engineer and author Martin Fowler points out: “Any fool can write code that a computer can understand. Good programmers write code that humans can understand.”
The Complexity of Real-World Development
Professional software development involves much more than just writing code. A typical developer’s workflow includes:
- Understanding requirements
- Designing architecture
- Researching approaches
- Writing tests
- Reviewing code
- Documenting solutions
- Collaborating with team members
- Refactoring existing code
Coding speed represents only a fraction of this process. Being quick at typing or implementing algorithms doesn’t necessarily translate to being effective at these other crucial aspects of development.
What Actually Matters More Than Speed
Problem-Solving Ability
The heart of programming is problem-solving. The ability to break down complex problems, identify patterns, and develop effective solutions is far more valuable than typing speed. This includes:
- Analytical thinking: Dissecting problems into manageable components
- Pattern recognition: Identifying similarities to known problems
- Algorithmic thinking: Creating efficient approaches to solve problems
- Creativity: Finding novel solutions when standard approaches fail
A developer who takes time to deeply understand a problem before coding will often produce a better solution than someone who jumps immediately into implementation.
Code Quality and Maintainability
Code is read far more often than it’s written. High-quality, maintainable code pays dividends long after it’s written by:
- Making it easier for others to understand and modify
- Reducing bugs and unexpected behavior
- Simplifying future extensions and features
- Decreasing the cost of maintenance
Consider this example comparing two approaches to the same function:
Approach 1: Quick but confusing
function p(a,b,c){return a?b.map(i=>i.x<c?{...i,y:i.y+1}:i):b.filter(i=>i.x>c);}
Approach 2: Thoughtful and clear
function processItems(shouldUpdate, items, threshold) {
if (shouldUpdate) {
return items.map(item => {
if (item.x < threshold) {
return { ...item, y: item.y + 1 };
}
return item;
});
} else {
return items.filter(item => item.x > threshold);
}
}
The second approach takes slightly longer to write but saves enormous time for anyone who needs to understand or modify the code later.
Technical Depth and Understanding
Deep understanding of programming concepts, language features, and system architecture enables developers to make better decisions. This includes:
- Knowledge of language idioms and best practices
- Understanding of data structures and algorithms
- Familiarity with design patterns
- Awareness of performance implications
- Insight into system architecture
A developer with strong technical depth might take longer to implement a solution but will likely create one that’s more robust, efficient, and aligned with established patterns.
Communication and Collaboration
Software development is rarely a solo activity. The ability to effectively communicate and collaborate with others is often more important than individual coding speed. This includes:
- Clearly explaining technical concepts
- Providing constructive code reviews
- Documenting decisions and approaches
- Mentoring less experienced team members
- Working effectively across disciplines
Even the fastest coder will struggle in a professional environment if they can’t collaborate effectively with their team.
Real-World Perspectives on Coding Speed
To gain further insight, let’s consider what industry professionals and research tell us about coding speed versus other skills.
What Tech Leaders Say
Many influential figures in tech have weighed in on this topic:
“Make it work, make it right, make it fast—in that order.”
— Kent Beck, creator of Extreme Programming
This quote emphasizes that correctness should precede optimization for speed.
“Premature optimization is the root of all evil.”
— Donald Knuth, computer scientist
While Knuth was referring to performance optimization, the principle applies to the development process itself—rushing to code quickly before properly understanding the problem often leads to suboptimal solutions.
Research on Developer Productivity
Research on software development productivity consistently shows that raw coding speed is a poor predictor of overall effectiveness:
- A study from Microsoft Research found that the most productive developers were not necessarily those who committed the most code but those who helped others improve their work through collaborative practices like code reviews.
- Research published in “The Mythical Man-Month” by Fred Brooks indicates that adding developers to speed up a project often slows it down due to communication overhead, suggesting that individual coding speed is less important than effective collaboration.
- Studies on bug rates show that code written under time pressure typically contains significantly more defects, offsetting any gains from faster development.
When Coding Speed Does Matter
Despite the arguments above, there are specific contexts where coding speed does have legitimate value:
Competitive Programming
In competitive programming contests like Google Code Jam or the ACM International Collegiate Programming Contest, speed is explicitly part of the challenge. These competitions reward those who can quickly analyze problems and implement correct solutions.
However, it’s important to recognize that competitive programming is a specialized skill that emphasizes different abilities than day-to-day software development.
Technical Interviews
Many technical interviews, especially at large tech companies, include time-constrained coding challenges. Being able to solve problems efficiently under pressure can be advantageous in these settings.
That said, more companies are moving toward take-home projects or longer interview formats that better reflect real-world development practices.
Prototyping and Exploration
When building prototypes or exploring potential solutions, the ability to quickly implement and test ideas can be valuable. In these contexts, the goal is learning rather than producing production-ready code.
Emergency Situations
During production emergencies or critical bugs, the ability to quickly identify and fix issues can be crucial. However, even in these situations, careful analysis is typically more important than raw coding speed.
How to Improve What Really Matters
If raw coding speed isn’t the most important skill, what should developers focus on improving? Here are strategies for developing the capabilities that truly matter:
Strengthen Your Problem-Solving Skills
- Practice algorithmic thinking: Regularly solve diverse programming challenges, focusing on understanding the problem and developing a clear approach before coding.
- Learn multiple approaches: Study different algorithms and data structures to expand your problem-solving toolkit.
- Break down complex problems: Practice decomposing large problems into smaller, manageable components.
- Review and reflect: After solving a problem, consider whether there are more elegant or efficient solutions.
For example, when approaching a new problem, try using this systematic process:
- Understand the problem completely, including constraints and edge cases
- Devise a strategy before writing any code
- Consider multiple potential approaches
- Implement your solution clearly
- Test thoroughly, especially edge cases
- Reflect on what could be improved
Focus on Code Quality
- Study design patterns: Learn established patterns that solve common problems in elegant ways.
- Practice refactoring: Regularly revisit and improve existing code to make it clearer and more maintainable.
- Adopt test-driven development: Writing tests first encourages clearer thinking about requirements and design.
- Read high-quality code: Study well-maintained open-source projects to see examples of clean, readable code.
Consider this example of refactoring for clarity:
Before refactoring:
function calc(x, y, z) {
let r;
if (z == 1) {
r = x + y;
} else if (z == 2) {
r = x - y;
} else if (z == 3) {
r = x * y;
} else {
r = x / y;
}
return r;
}
After refactoring:
function calculate(firstNumber, secondNumber, operationType) {
const OPERATIONS = {
1: (a, b) => a + b, // Addition
2: (a, b) => a - b, // Subtraction
3: (a, b) => a * b, // Multiplication
4: (a, b) => a / b // Division
};
const operation = OPERATIONS[operationType] || OPERATIONS[4];
return operation(firstNumber, secondNumber);
}
Deepen Your Technical Knowledge
- Study language fundamentals: Thoroughly understand the languages you work with, including their idioms and best practices.
- Learn about system architecture: Understand how different components interact in large systems.
- Explore computer science concepts: Study topics like complexity analysis, memory management, and concurrency.
- Stay current with trends: Follow developments in your field to understand evolving best practices.
Enhance Communication and Collaboration
- Practice explaining technical concepts: Work on communicating complex ideas in clear, accessible ways.
- Contribute to open source: Participate in projects that require collaboration with diverse contributors.
- Give and receive code reviews: Thoughtful code reviews build both technical and communication skills.
- Document your work: Practice writing clear documentation that helps others understand your code and decisions.
Finding Balance: When to Optimize for Speed
While coding speed shouldn’t be your primary focus, there are ways to become more efficient without sacrificing quality:
Master Your Tools
Becoming proficient with your development environment can significantly improve efficiency:
- Learn keyboard shortcuts for your editor or IDE
- Use snippets and templates for common patterns
- Set up efficient workflows for tasks you perform frequently
- Automate repetitive tasks where possible
For example, in VS Code, mastering shortcuts like these can save time:
Ctrl+Shift+P
(Command Palette) for accessing commandsAlt+↑/↓
to move lines up or downCtrl+D
to select multiple occurrences of the same textF2
for symbol renaming
Develop Efficient Coding Habits
Certain practices can help you code more efficiently without compromising quality:
- Write tests early to catch issues before they become complex
- Use incremental development to validate approaches as you go
- Leverage existing libraries and frameworks appropriately
- Maintain a personal knowledge base of solutions to common problems
Continuous Learning and Practice
Regular practice leads to natural improvements in speed:
- Work on diverse projects to expand your experience
- Study patterns and idioms that enable efficient expression of common tasks
- Review your completed work to identify areas for improvement
- Learn from others through pair programming and code reviews
Reframing Technical Interviews
Many developers worry about coding speed primarily because of technical interviews. Here’s how to approach these situations:
Focus on Process Over Speed
In most quality interviews, interviewers care more about your problem-solving approach than raw speed:
- Communicate your thinking clearly as you work
- Ask clarifying questions to ensure you understand the problem
- Discuss tradeoffs between different approaches
- Explain your reasoning for design decisions
Good interviewers are typically more interested in your thought process than watching you race to a solution.
Practice Deliberately
If you’re preparing for interviews that do emphasize speed:
- Practice with timed exercises to build comfort with constraints
- Focus on common problem patterns that appear frequently in interviews
- Develop a systematic approach to problem-solving that you can apply consistently
- Review and learn from each practice session
Choose Environments That Value the Right Things
Consider whether companies that overemphasize coding speed align with your values:
- Research interview processes when applying to companies
- Ask about engineering culture during interviews
- Look for organizations that value thorough problem-solving and quality
The Long-Term View of Developer Success
When we look at what makes developers successful over entire careers, coding speed rarely appears as a determining factor. Instead, we see:
Adaptability
The ability to learn new technologies, approaches, and domains allows developers to remain relevant as the industry evolves. This adaptability often comes from strong fundamentals and a growth mindset rather than implementation speed.
Technical Judgment
Experienced developers are valued for their ability to make sound technical decisions, anticipate challenges, and choose appropriate solutions for specific contexts. This judgment develops through experience and reflection, not rapid coding.
Leadership and Influence
As developers progress in their careers, their impact increasingly comes from guiding others, shaping architecture, and influencing technical direction. These contributions depend on communication, vision, and collaborative skills rather than individual coding pace.
Problem Domain Expertise
Deep understanding of specific problem domains (finance, healthcare, e-commerce, etc.) often becomes more valuable than general programming speed. This expertise allows developers to create solutions that truly address user needs and business requirements.
Conclusion: Quality Trumps Speed
While coding speed has its place, it’s far from the most important skill for software developers. The most valuable developers are those who:
- Solve problems thoroughly and thoughtfully
- Write clean, maintainable code
- Communicate effectively with others
- Make sound technical decisions
- Continue learning and adapting
Rather than worrying about how quickly you can write code, focus on developing these fundamental capabilities. Over time, you’ll naturally become more efficient as your experience grows, but that efficiency will be built on a foundation of quality and understanding rather than rushed implementation.
Remember that software development is a marathon, not a sprint. The developers who thrive in the long run are those who consistently deliver value through thoughtful, well-crafted solutions—regardless of how quickly they type.
So the next time you feel anxious about your coding speed, take a deep breath and refocus on what truly matters: solving the right problems in the right way. Your future self—and your teammates—will thank you.