Why Your Coding Skills Look Good on Paper But Not in Technical Interviews
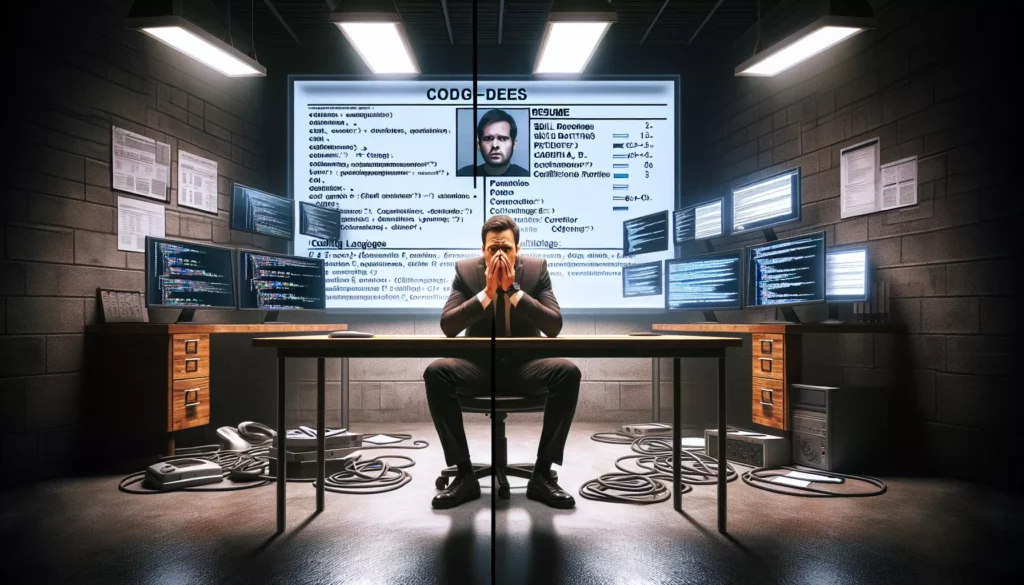
Technical interviews can be a source of frustration for many programmers. You might have years of experience, an impressive portfolio, and excellent coding skills that look stellar on paper, yet when it comes to whiteboard coding or live technical interviews, you find yourself struggling. This disconnect between your actual abilities and your interview performance is more common than you might think.
In this comprehensive guide, we will explore why your coding skills might not translate well in interview settings, identify the key differences between real world programming and technical interviews, and provide actionable strategies to bridge this gap. By understanding the unique challenges of technical interviews, you can better prepare yourself to showcase your true capabilities.
The Disconnect Between Practical Coding and Interview Performance
Many experienced developers find themselves in a perplexing situation: they excel in their day to day work but falter during technical interviews. This paradox stems from several key differences between real world programming environments and the artificial constraints of interview settings.
Real World Programming vs. Interview Coding
Real World Programming | Technical Interview Coding |
---|---|
Access to documentation, Stack Overflow, and other resources | Limited or no access to external resources |
Time to think through problems thoroughly | High pressure, time constraints |
Collaborative environment | Solo performance under observation |
Focus on practical solutions and maintainability | Focus on algorithmic efficiency and optimization |
Iterative development and testing | Expected to produce correct solutions quickly |
The “Resume Paradox”
The “Resume Paradox” occurs when your resume accurately reflects your professional accomplishments and skills, but interview performance tells a different story. This discrepancy can be jarring and discouraging, leading many qualified candidates to question their abilities.
Consider this common scenario: You have successfully built complex systems, contributed to significant projects, and solved challenging problems in your professional career. Your resume showcases these achievements eloquently. Yet, when asked to solve a seemingly simple algorithm problem on a whiteboard, you freeze up or struggle to articulate your thoughts clearly.
This doesn’t mean your resume is misleading or that you’re not as skilled as you claim. Rather, it highlights that technical interviews often test a specific subset of skills in an artificial environment that may not accurately reflect your ability to succeed in an actual job.
Why Technical Interviews Are Different From Real Programming
To bridge the gap between your paper qualifications and interview performance, it’s crucial to understand exactly what makes technical interviews so different from everyday coding tasks.
The Artificial Environment
Technical interviews create artificial constraints that rarely exist in professional settings:
- Time pressure: You typically have 30 60 minutes to solve problems that you might normally take hours to think through.
- Public performance: Having to code while others watch and evaluate you creates psychological pressure.
- Limited resources: No Google, no documentation, and often no IDE with helpful features like autocomplete or syntax checking.
- Whiteboard coding: Writing code without the ability to run it requires a different mental process than typical development.
Different Skill Emphasis
Technical interviews often emphasize skills that may not be central to your daily work:
- Algorithm recall: Remembering specific algorithms and data structures that you might rarely use in your actual job.
- Big O analysis: Detailed discussion of time and space complexity that may exceed what’s necessary in many programming roles.
- Problem solving under pressure: The ability to think clearly while being observed and evaluated.
- Communication while coding: Explaining your thought process while simultaneously solving a problem.
The Psychological Component
Perhaps the most significant difference is psychological. Technical interviews create a high stakes environment that triggers anxiety and stress responses in many candidates. This “interview anxiety” can impair cognitive function, making it difficult to access knowledge and skills you definitely possess.
A 2019 study published in the Journal of Occupational Health Psychology found that interview anxiety can reduce performance by up to 30% in technical assessments, regardless of actual skill level. This means that even if you’re in the top percentile of programmers, anxiety alone could make you appear average during an interview.
Common Interview Challenges That Trip Up Experienced Developers
Understanding the specific challenges that cause even skilled developers to underperform can help you prepare more effectively. Here are the most common stumbling blocks:
Algorithm Blind Spots
Many developers work for years without needing to implement certain algorithms from scratch. Common blind spots include:
- Graph algorithms (BFS, DFS, Dijkstra’s)
- Dynamic programming approaches
- Advanced tree traversals
- Specialized sorting algorithms
For example, you might be proficient at building complex systems but haven’t had to implement a breadth first search algorithm since college. When asked to do so in an interview, you might struggle despite being an otherwise excellent programmer.
Analysis Paralysis
The pressure to find the optimal solution immediately can lead to analysis paralysis. In real world coding, you might start with a working solution and iteratively improve it. In interviews, candidates often feel they need the perfect approach right away, causing them to freeze up instead of making progress.
Consider this common interview scenario:
Interviewer: “Given an array of integers, find the pair with the largest sum.”
Your thought process: “This seems simple. I could just use two loops to check all pairs… but wait, that’s O(n²). There must be a more efficient solution. Maybe sorting first? But that’s O(n log n). Is there an O(n) approach? What if I…”
While you’re overanalyzing, time is ticking away, and you haven’t written a single line of code. Meanwhile, starting with the brute force approach and then optimizing might have been a perfectly acceptable strategy.
Communication Challenges
Technical interviews don’t just test your coding abilities; they also evaluate how well you communicate your thought process. Many developers work independently or communicate asynchronously through comments and documentation. The expectation to verbalize your thinking in real time can be challenging.
Failing to articulate your approach clearly can make even correct solutions appear weak. Conversely, clear communication can make up for minor technical stumbles.
Edge Case Oversights
In production environments, you might rely on testing frameworks, user feedback, and iterative development to catch edge cases. In interviews, you’re expected to identify and handle edge cases proactively:
- Empty inputs
- Extremely large inputs
- Negative numbers or other unexpected values
- Duplicate elements
- Boundary conditions
Missing these considerations can give the impression that you lack thoroughness, even if your core algorithm is correct.
Time Management Issues
Many candidates spend too much time perfecting one part of the solution, leaving insufficient time for the complete implementation. Others rush through the problem without taking time to understand it fully, leading to fundamental misunderstandings.
The ability to pace yourself appropriately is a skill that must be developed specifically for interviews, as it’s quite different from the time management needed for day to day development work.
The Skills Gap: What Interview Coding Requires That Daily Work Doesn’t
Technical interviews often test a specific set of skills that may atrophy if not regularly used in your daily work. Understanding this skills gap can help you identify areas for focused preparation.
Computer Science Fundamentals
While modern frameworks and libraries abstract away many low level details, interviews often probe your understanding of fundamental concepts:
- Data structures: Detailed knowledge of arrays, linked lists, trees, graphs, hash tables, and their operations.
- Algorithm design: Familiarity with common paradigms like divide and conquer, greedy algorithms, and dynamic programming.
- Space and time complexity analysis: The ability to analyze and optimize solutions based on Big O notation.
- Memory management: Understanding how variables are stored and accessed, especially in languages with manual memory management.
Many developers can build successful applications without frequent recourse to these fundamentals, creating a potential gap when interviews focus heavily on them.
Coding Without Tools
Modern development environments provide numerous tools that enhance productivity:
- Syntax highlighting and error checking
- Autocomplete and intelligent code suggestions
- Integrated debugging tools
- Package managers and libraries
When these tools are removed in an interview setting, you’re forced to rely on raw coding skills that might be rusty. For example, manually tracking array indices or managing pointer arithmetic without the safety net of IDE warnings can be challenging if you’re not in the habit of doing so.
Rapid Problem Decomposition
In professional settings, you might spend hours or days thinking through a complex problem before writing any code. Interviews compress this process dramatically, requiring you to:
- Quickly understand problem requirements
- Identify key constraints and edge cases
- Formulate a workable approach in minutes
- Explain your reasoning while implementing your solution
This accelerated problem solving process demands mental agility that differs from the more methodical approach common in professional development.
Verbalization of Technical Concepts
Explaining your code and thought process clearly is a skill that requires practice. Many developers work in environments where deep technical discussions happen infrequently or are documented rather than verbalized.
Technical interviews test your ability to:
- Articulate complex concepts in simple terms
- Justify design decisions on the spot
- Respond to questions and feedback in real time
- Maintain clarity under pressure
This communication dimension adds another layer of complexity beyond pure coding ability.
How to Bridge the Gap: Strategies for Interview Success
Now that we understand the challenges, let’s explore practical strategies to bridge the gap between your actual skills and your interview performance.
Systematic Interview Preparation
Effective preparation requires a structured approach rather than random problem solving:
- Build a knowledge foundation: Review computer science fundamentals, focusing on data structures, algorithms, and complexity analysis.
- Develop a problem solving framework: Create a consistent approach to tackling interview questions (more on this below).
- Practice deliberately: Work through problems that target specific weaknesses rather than just solving what’s comfortable.
- Simulate interview conditions: Practice with time constraints, without IDE assistance, and while explaining your thought process aloud.
Consistency is key. Short, regular practice sessions (30 60 minutes daily) are more effective than occasional marathon sessions.
Develop a Problem Solving Framework
Having a systematic approach to interview questions can reduce anxiety and improve performance. Here’s a framework you can adapt:
- Understand the problem (2 3 minutes)
- Restate the problem in your own words
- Ask clarifying questions about constraints and expected output
- Work through a simple example to confirm understanding
- Explore approaches (3 5 minutes)
- Consider multiple possible solutions
- Discuss tradeoffs between approaches
- Select a promising approach to implement
- Plan your solution (2 3 minutes)
- Outline the algorithm steps before coding
- Identify helper functions or key components
- Consider edge cases and how to handle them
- Implement the solution (10 20 minutes)
- Write clean, readable code
- Narrate your thought process as you code
- Focus on correctness first, then optimization
- Test and refine (5 7 minutes)
- Walk through your solution with example inputs
- Check edge cases
- Analyze time and space complexity
- Suggest optimizations if time permits
This framework ensures you don’t skip crucial steps in the problem solving process and helps you manage time effectively.
Example: Applying the Framework
Let’s see how this framework might apply to a common interview question:
“Given an array of integers, find the two numbers that add up to a specific target.”
1. Understand the problem:
“So I need to find two distinct elements in the array whose sum equals a given target value. For example, if the array is [2, 7, 11, 15] and the target is 9, the answer would be [0, 1] because 2 + 7 = 9. Can I assume the array has at least two elements? Is there exactly one solution, or could there be multiple valid pairs? Should I return the indices or the values themselves?”
2. Explore approaches:
“I see a few possible approaches:
– A brute force solution would check every pair with nested loops. This would be O(n²) time and O(1) space.
– Another approach would be to sort the array first and then use two pointers. This would be O(n log n) time and O(1) space if we can modify the input array.
– We could also use a hash map to store elements we’ve seen, which would give us O(n) time and O(n) space.
Let me go with the hash map approach since it’s more efficient.”
3. Plan your solution:
“I’ll create a hash map to store elements I’ve seen so far. For each element in the array, I’ll calculate what value I need to find (target – current element). If that value exists in my hash map, I’ve found a solution. If not, I’ll add the current element to the hash map and continue. I need to handle edge cases like an empty array or no solution found.”
4. Implement the solution:
function twoSum(nums, target) {
const seen = {};
for (let i = 0; i < nums.length; i++) {
const complement = target - nums[i];
if (complement in seen) {
return [seen[complement], i];
}
seen[nums[i]] = i;
}
return null; // No solution found
}
5. Test and refine:
“Let me trace through this with the example [2, 7, 11, 15] and target 9:
– i=0: nums[0]=2, complement=7, seen={}, 7 not in seen, add seen[2]=0
– i=1: nums[1]=7, complement=2, seen={2:0}, 2 is in seen, return [0,1]
This solution has O(n) time complexity because we’re iterating through the array once, and O(n) space complexity for the hash map. Edge cases: if the array is empty, the for loop won’t execute and we’ll return null. If there’s no solution, we’ll check all elements and return null at the end.”
Mock Interviews and Peer Practice
While solo practice is valuable, nothing replaces the experience of actual interview conditions. Consider these options:
- Peer programming sessions: Find a coding buddy and take turns interviewing each other.
- Mock interview platforms: Services like Pramp, interviewing.io, or AlgoCademy offer structured practice with real people.
- Recording yourself: Practice explaining problems aloud while recording yourself, then review to identify areas for improvement in your communication.
These practices help acclimate you to the social pressure of being observed while coding, which is often the biggest source of interview anxiety.
Developing Mental Resilience
Technical preparation alone isn’t enough; mental preparation is equally important:
- Normalize struggle: Recognize that it’s normal to struggle with interview problems. Even experienced developers rarely solve problems perfectly on the first try.
- Practice recovery: Deliberately put yourself in challenging situations during practice and work on recovering from setbacks or mental blocks.
- Mindfulness techniques: Learn breathing exercises or quick meditation techniques to center yourself when anxiety rises during interviews.
- Positive self talk: Replace negative thoughts (“I’m bombing this interview”) with constructive ones (“This is challenging, but I can work through it step by step”).
Remember that interviewers are often more interested in your problem solving process than in whether you get the perfect answer immediately.
The Role of Computer Science Fundamentals
Many developers, especially self taught programmers or those who’ve worked primarily with high level frameworks, may have gaps in their computer science fundamentals. Addressing these gaps is crucial for interview success.
Essential Data Structures to Master
Certain data structures appear frequently in technical interviews. Understanding their properties, operations, and implementation details can give you a significant advantage:
- Arrays and Strings: Slicing, in place operations, and string manipulation techniques.
- Hash Tables: Implementation details, collision resolution, and common applications.
- Linked Lists: Singly and doubly linked lists, detecting cycles, and reversal algorithms.
- Stacks and Queues: Implementation approaches and typical use cases.
- Trees: Binary trees, binary search trees, balanced trees, and traversal methods.
- Graphs: Representation methods, traversal algorithms, and pathfinding approaches.
- Heaps: Implementation, heapify operations, and priority queue applications.
For each structure, you should understand:
- Time and space complexity of common operations
- Implementation details (at least conceptually)
- Typical problem patterns where they’re useful
- Common pitfalls and edge cases
Algorithm Patterns Worth Studying
Rather than trying to memorize hundreds of specific algorithms, focus on understanding common patterns that can be applied to multiple problems:
- Two Pointers: Used for array problems, especially with sorted arrays.
- Sliding Window: Efficient for substring or subarray problems.
- Binary Search: Not just for sorted arrays, but as a general technique for reducing search space.
- Breadth First Search: For level by level exploration of trees and graphs.
- Depth First Search: For exploring paths and backtracking problems.
- Dynamic Programming: For optimization problems with overlapping subproblems.
- Greedy Algorithms: For making locally optimal choices to find global optima.
- Divide and Conquer: For breaking problems into smaller, manageable subproblems.
Understanding these patterns allows you to recognize which approach might be suitable for a new problem you encounter.
Resources for Strengthening Fundamentals
If you need to strengthen your computer science fundamentals, consider these resources:
- Books: “Cracking the Coding Interview” by Gayle Laakmann McDowell, “The Algorithm Design Manual” by Steven Skiena.
- Online Courses: MIT OpenCourseWare’s “Introduction to Algorithms,” Stanford’s algorithms courses on Coursera.
- Interactive Platforms: AlgoCademy, LeetCode, HackerRank, and similar platforms for practice problems.
- Visualization Tools: Websites like VisuAlgo that help you visualize how algorithms and data structures work.
Remember that building a strong foundation takes time. Focus on understanding concepts deeply rather than memorizing solutions to specific problems.
Practical Coding Interview Strategies
Beyond technical knowledge, certain practical strategies can significantly improve your interview performance.
Start With Brute Force
Many candidates make the mistake of trying to jump immediately to the optimal solution. This approach often leads to analysis paralysis or incomplete answers. Instead:
- Begin by acknowledging the simplest solution, even if it’s inefficient.
- Implement this solution if time permits or if you’re struggling to see a more optimal approach.
- Use the brute force solution as a stepping stone to identify optimization opportunities.
This approach demonstrates that you can at least solve the problem, while giving you a foundation to build upon. Interviewers often value this incremental problem solving approach more than an immediate leap to the optimal solution.
Talk Through Your Thought Process
Silence during an interview creates uncertainty about what you’re thinking. Verbalizing your thought process has several benefits:
- It demonstrates your reasoning abilities even if you’re struggling with implementation.
- It gives the interviewer opportunities to provide hints if you’re heading in the wrong direction.
- It shows your communication skills, which are highly valued in collaborative development environments.
Practice “thinking aloud” during your preparation so it becomes natural during actual interviews.
Manage Edge Cases Systematically
Addressing edge cases demonstrates thoroughness and attention to detail. Develop a systematic approach:
- Before coding, identify potential edge cases (empty inputs, single elements, negative values, etc.)
- After implementing your core solution, explicitly check each edge case
- Consider adding comments or assertions to document your handling of edge cases
This systematic approach prevents oversights and shows that you write robust code, not just code that works for the happy path.
Optimize Only When Necessary
Premature optimization can lead to unnecessarily complex solutions or incomplete implementations. Follow this hierarchy:
- First, make it work (correctness)
- Then, make it clear (readability)
- Finally, make it fast (optimization)
If time is running short, prioritize a complete, correct solution over an optimized but incomplete one. You can always discuss potential optimizations even if you don’t have time to implement them.
Common Pitfalls to Avoid
Even well prepared candidates can fall into certain traps during technical interviews. Being aware of these pitfalls can help you avoid them.
Diving Into Code Too Quickly
In their eagerness to demonstrate coding skills, many candidates start writing code before fully understanding the problem or considering different approaches. This often leads to false starts, backtracking, and wasted time.
Better approach: Spend the first few minutes clarifying the problem and discussing potential solutions before writing any code. This investment pays off in more efficient implementation.
Ignoring Interviewer Hints
Interviewers often provide subtle hints when they see you struggling. These hints might come in the form of questions (“Have you considered using a different data structure?”) or observations about your current approach.
Better approach: Listen carefully to interviewer feedback and treat it as valuable guidance rather than criticism. Be willing to pivot based on their suggestions.
Overcomplicating Solutions
Some candidates try to impress interviewers with unnecessarily complex or “clever” solutions. This approach often backfires, leading to bugs, incomplete implementations, or solutions that are difficult to explain.
Better approach: Aim for clarity and correctness first. A well implemented, straightforward solution is more impressive than a partially implemented complex one.
Freezing When Stuck
When faced with a challenging problem or a bug they can’t immediately fix, some candidates freeze up or fall silent, creating awkward pauses that amplify anxiety.
Better approach: Develop strategies for when you get stuck:
- Try a different example to gain new insights
- Break the problem into smaller parts
- Revisit your assumptions about the problem
- Verbalize what you know and what you’re trying to figure out
The ability to work through blocks methodically is itself a valuable skill that interviewers look for.
Neglecting Code Quality
Under time pressure, it’s tempting to focus solely on getting a working solution without regard for code quality. However, messy, inconsistent, or poorly structured code can create a negative impression even if your algorithm is correct.
Better approach: Maintain basic code quality standards even in interviews:
- Use meaningful variable names
- Structure your code with appropriate functions and clear organization
- Follow consistent formatting and style
- Add brief comments for complex sections
These practices demonstrate professionalism and attention to detail.
From Interview to Real World: The Transfer of Skills
While we’ve focused on adapting your real world skills to interview settings, it’s worth noting that interview preparation can also enhance your practical programming abilities.
How Interview Prep Improves Daily Coding
The skills developed during interview preparation can translate to tangible benefits in your day to day work:
- Algorithm awareness: Recognizing when standard algorithms can simplify complex problems in your projects.
- Performance sensitivity: Greater awareness of efficiency and optimization opportunities in production code.
- Problem decomposition: Improved ability to break down complex tasks into manageable components.
- Technical communication: Enhanced ability to explain your approach to colleagues and stakeholders.
Many developers report that dedicated interview preparation made them better programmers overall, not just better at interviews.
Building Transferable Problem Solving Skills
To maximize the transfer of skills between interview preparation and practical work:
- Relate interview problems to real world scenarios you’ve encountered
- Practice explaining the practical applications of algorithms you study
- Look for opportunities to apply optimized approaches in your daily work
- Share your interview preparation insights with colleagues through knowledge sharing sessions
This bidirectional skill transfer creates a virtuous cycle where interview preparation and practical experience reinforce each other.
Conclusion: Bridging the Gap Between Paper and Performance
The disconnect between your coding skills on paper and your interview performance is not a reflection of your abilities as a developer. Rather, it highlights the specialized nature of technical interviews and the unique skills they demand.
By understanding the specific challenges of interview settings, systematically preparing for these challenges, and developing both technical knowledge and interview specific strategies, you can bridge this gap and demonstrate your true capabilities during the interview process.
Remember that interview skills, like any other skills, improve with deliberate practice. Each interview experience, whether successful or not, provides valuable data to refine your approach. With time and consistent effort, you can develop the ability to showcase your coding abilities effectively, ensuring that your interview performance matches the impressive skills displayed on your resume.
The journey from looking good on paper to performing well in interviews is challenging but achievable. By applying the strategies outlined in this guide, you can approach your next technical interview with greater confidence and a higher probability of success.