Why Your Coding Playlist is Actually Controlling Your Algorithm Choices
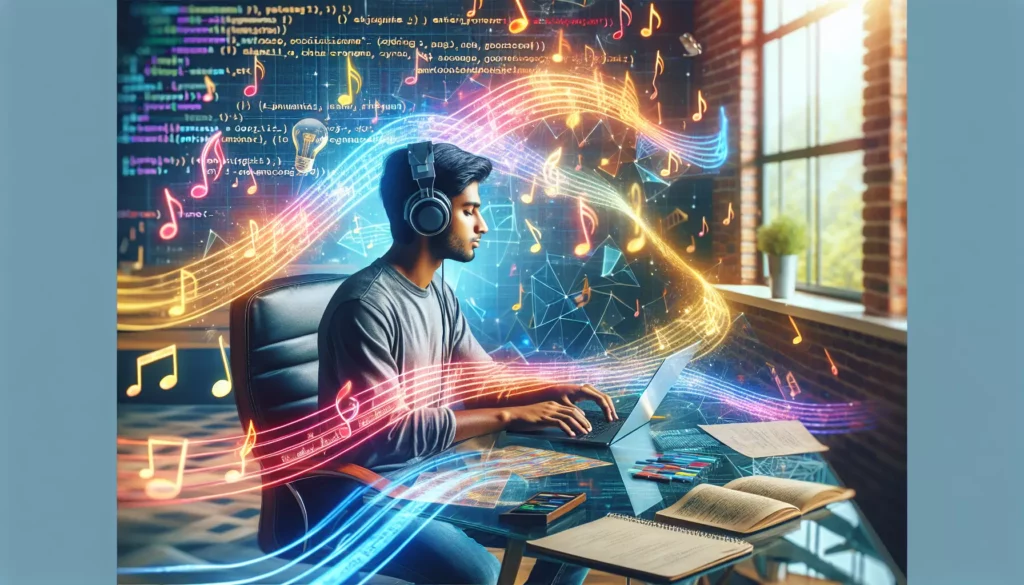
As a developer, you’ve probably experienced those marathon coding sessions where time seems to fly by, and your fingers dance across the keyboard in perfect harmony with your thoughts. For many of us, music is an integral part of this flow state, acting as a backdrop to our problem-solving and creative processes. But have you ever stopped to consider how your coding playlist might be influencing the very algorithms you choose to implement?
In this deep dive, we’ll explore the fascinating intersection of music and coding, uncovering how your favorite tunes might be subtly shaping your programming decisions. Whether you’re a seasoned software engineer or just starting your journey with AlgoCademy, understanding this connection could revolutionize your approach to both coding and curating the perfect work soundtrack.
The Science Behind Music and Cognitive Function
Before we delve into the specifics of how music affects our coding choices, it’s crucial to understand the broader impact of music on our cognitive functions. Numerous studies have shown that music can significantly influence our mood, focus, and even our problem-solving abilities.
1. The Mozart Effect and Beyond
You might have heard of the “Mozart Effect,” a phenomenon suggesting that listening to classical music, particularly Mozart, can temporarily boost spatial-temporal reasoning skills. While the original study’s claims have been debated, it sparked a wave of research into music’s effects on cognitive performance.
Recent studies have expanded on this concept, revealing that different types of music can affect various cognitive tasks in unique ways. For instance, ambient music has been found to improve abstract reasoning, while upbeat pop songs can enhance mood and energy levels.
2. Rhythm and Pattern Recognition
One of the most intriguing connections between music and coding lies in the realm of pattern recognition. Both music and programming are fundamentally about patterns – in music, it’s rhythms and melodies; in coding, it’s algorithms and data structures.
Research has shown that musicians often excel in pattern recognition tasks, a skill that’s equally crucial in programming. This suggests that exposure to music, especially while coding, might enhance our ability to recognize and implement efficient algorithmic patterns.
How Your Playlist Influences Algorithm Selection
Now that we’ve established the cognitive link between music and coding, let’s explore how your specific playlist choices might be influencing your algorithm selections.
1. Tempo and Time Complexity
The tempo of the music you listen to while coding could be subtly influencing your perception of time complexity in algorithms. Here’s how:
- Fast-paced music: When listening to high-tempo tracks, you might find yourself gravitating towards more efficient algorithms with lower time complexities. The quick beats could subconsciously push you to seek faster solutions, leading you to favor algorithms like Quick Sort (O(n log n)) over simpler but slower options like Bubble Sort (O(n²)).
- Slower, more relaxed tunes: On the other hand, mellower music might make you more tolerant of algorithms with higher time complexities. You might be more inclined to implement a depth-first search in a situation where a breadth-first approach could be more efficient, simply because the relaxed atmosphere doesn’t create a sense of urgency.
Consider this code snippet for Quick Sort, which you might be more likely to implement when listening to up-tempo music:
def quick_sort(arr):
if len(arr) <= 1:
return arr
pivot = arr[len(arr) // 2]
left = [x for x in arr if x < pivot]
middle = [x for x in arr if x == pivot]
right = [x for x in arr if x > pivot]
return quick_sort(left) + middle + quick_sort(right)
2. Genre and Problem-Solving Approaches
Different music genres can evoke various emotional states and cognitive patterns, potentially influencing your problem-solving approach:
- Classical music: Often associated with improved spatial-temporal reasoning, classical music might lead you to favor more structured and mathematically elegant algorithms. You might find yourself implementing a dynamic programming solution to the Fibonacci sequence problem instead of a simple recursive approach.
- Electronic or techno: The repetitive, pattern-based nature of electronic music could enhance your ability to recognize recurring subproblems, making you more likely to implement memoization or dynamic programming techniques.
- Jazz: Known for its improvisational nature, jazz might encourage more creative, out-of-the-box thinking. This could lead you to explore novel algorithmic approaches or unconventional data structures for solving problems.
Here’s an example of a dynamic programming solution for the Fibonacci sequence, which you might be more inclined to implement while listening to classical music:
def fibonacci(n):
if n <= 1:
return n
fib = [0] * (n + 1)
fib[1] = 1
for i in range(2, n + 1):
fib[i] = fib[i-1] + fib[i-2]
return fib[n]
3. Lyrics vs. Instrumental: Impact on Concentration and Code Complexity
The presence or absence of lyrics in your music can significantly affect your coding process:
- Instrumental music: Without the distraction of lyrics, you might find it easier to focus on complex algorithmic problems. This could lead you to tackle more challenging algorithms or implement more sophisticated data structures, such as balanced binary search trees or advanced graph algorithms.
- Music with lyrics: While some developers find lyrics distracting, others use them as a form of active noise cancellation for background chatter. However, processing lyrics while coding might reduce your available cognitive resources, potentially leading you to choose simpler, more straightforward algorithmic solutions.
Consider this implementation of a balanced binary search tree (AVL tree), which you might be more likely to tackle when listening to instrumental music:
class Node:
def __init__(self, key):
self.key = key
self.left = None
self.right = None
self.height = 1
class AVLTree:
def __init__(self):
self.root = None
def height(self, node):
if not node:
return 0
return node.height
def balance(self, node):
if not node:
return 0
return self.height(node.left) - self.height(node.right)
def update_height(self, node):
if not node:
return
node.height = 1 + max(self.height(node.left), self.height(node.right))
def right_rotate(self, y):
x = y.left
T2 = x.right
x.right = y
y.left = T2
self.update_height(y)
self.update_height(x)
return x
def left_rotate(self, x):
y = x.right
T2 = y.left
y.left = x
x.right = T2
self.update_height(x)
self.update_height(y)
return y
def insert(self, root, key):
if not root:
return Node(key)
if key < root.key:
root.left = self.insert(root.left, key)
else:
root.right = self.insert(root.right, key)
self.update_height(root)
balance = self.balance(root)
if balance > 1 and key < root.left.key:
return self.right_rotate(root)
if balance < -1 and key > root.right.key:
return self.left_rotate(root)
if balance > 1 and key > root.left.key:
root.left = self.left_rotate(root.left)
return self.right_rotate(root)
if balance < -1 and key < root.right.key:
root.right = self.right_rotate(root.right)
return self.left_rotate(root)
return root
def insert_key(self, key):
self.root = self.insert(self.root, key)
The Role of Music in Different Coding Phases
As you progress through different stages of the development process, you might find that your musical needs change. Let’s explore how different types of music can be beneficial during various coding phases:
1. Problem Analysis and Algorithm Design
During the initial stages of tackling a coding problem, when you’re analyzing requirements and designing your algorithm, you might benefit from:
- Ambient or nature sounds: These can help create a calm, focused environment conducive to deep thinking and problem analysis.
- Classical music: The structured nature of classical compositions can aid in organizing your thoughts and approaching problem-solving systematically.
For example, when designing a complex algorithm like A* pathfinding, you might find that ambient music helps you visualize the problem space more clearly:
import heapq
def heuristic(a, b):
return abs(b[0] - a[0]) + abs(b[1] - a[1])
def a_star(graph, start, goal):
open_set = []
heapq.heappush(open_set, (0, start))
came_from = {}
g_score = {start: 0}
f_score = {start: heuristic(start, goal)}
while open_set:
current = heapq.heappop(open_set)[1]
if current == goal:
path = []
while current in came_from:
path.append(current)
current = came_from[current]
path.append(start)
return path[::-1]
for neighbor in graph[current]:
tentative_g_score = g_score[current] + 1
if neighbor not in g_score or tentative_g_score < g_score[neighbor]:
came_from[neighbor] = current
g_score[neighbor] = tentative_g_score
f_score[neighbor] = g_score[neighbor] + heuristic(neighbor, goal)
heapq.heappush(open_set, (f_score[neighbor], neighbor))
return None
2. Implementation and Coding
When you’re in the thick of coding, translating your algorithm design into actual code, you might prefer:
- Electronic or techno music: The steady beat can help maintain a consistent coding rhythm, potentially increasing your productivity.
- Video game soundtracks: Designed to enhance focus without being distracting, these can be excellent for maintaining concentration during long coding sessions.
During this phase, you might find yourself implementing complex data structures more efficiently, like this trie implementation:
class TrieNode:
def __init__(self):
self.children = {}
self.is_end_of_word = False
class Trie:
def __init__(self):
self.root = TrieNode()
def insert(self, word):
node = self.root
for char in word:
if char not in node.children:
node.children[char] = TrieNode()
node = node.children[char]
node.is_end_of_word = True
def search(self, word):
node = self.root
for char in word:
if char not in node.children:
return False
node = node.children[char]
return node.is_end_of_word
def starts_with(self, prefix):
node = self.root
for char in prefix:
if char not in node.children:
return False
node = node.children[char]
return True
3. Debugging and Testing
When you’re hunting down bugs or running tests, you might benefit from:
- Jazz or blues: The improvisational nature of these genres can help you think creatively when troubleshooting unexpected issues.
- Instrumental versions of familiar songs: These can provide a comforting background that doesn’t distract from the detailed work of debugging.
During debugging, you might find yourself implementing more robust error handling and logging, like in this example:
import logging
logging.basicConfig(level=logging.DEBUG, format='%(asctime)s - %(levelname)s - %(message)s')
def divide(a, b):
try:
result = a / b
logging.info(f"Successfully divided {a} by {b}. Result: {result}")
return result
except ZeroDivisionError:
logging.error(f"Attempted to divide {a} by zero")
raise
except TypeError:
logging.error(f"Invalid types for division: {type(a)} and {type(b)}")
raise
try:
divide(10, 2)
divide(10, 0)
divide('10', 2)
except Exception as e:
logging.exception("An error occurred during division")
Optimizing Your Coding Playlist
Now that we understand the potential impact of music on our coding choices, how can we optimize our playlists to enhance our programming performance? Here are some strategies:
1. Match Music to Task Complexity
Consider creating different playlists for various levels of task complexity:
- Simple tasks: Upbeat, energetic music to maintain motivation and energy levels.
- Complex problem-solving: Instrumental, ambient, or classical music to enhance focus and cognitive performance.
- Repetitive tasks: Rhythmic, electronic music to help maintain a steady work pace.
2. Experiment with Binaural Beats
Binaural beats are a form of sound wave therapy that some believe can enhance concentration and cognitive performance. While scientific evidence is mixed, many developers report improved focus when listening to binaural beats, especially during challenging coding tasks.
3. Create Language-Specific Playlists
Interestingly, some developers find that certain music genres align well with specific programming languages:
- Python: Jazz or blues, mirroring the language’s flexibility and expressiveness.
- Java: Classical music, reflecting the language’s structure and complexity.
- JavaScript: Electronic or indie rock, capturing the language’s dynamic and versatile nature.
4. Use Music as a Productivity Timer
Create playlists of a specific duration to implement a version of the Pomodoro Technique. For example, a 25-minute playlist for focused work, followed by a 5-minute break with different music.
The Ethical Considerations of Music-Influenced Coding
As we explore the influence of music on our coding choices, it’s important to consider the ethical implications:
1. Bias in Algorithm Selection
If our music choices consistently lead us to favor certain types of algorithms, we might inadvertently introduce bias into our software. For example, always opting for the fastest algorithm might lead to increased energy consumption, which could have environmental implications at scale.
2. Workplace Considerations
In a team setting, personal music choices could lead to inconsistencies in coding styles and algorithm selections across the team. It’s important to balance personal productivity preferences with team cohesion and code consistency.
3. Cognitive Diversity
While optimizing our personal coding environment is important, it’s equally crucial to expose ourselves to diverse problem-solving approaches. Varying our music choices or occasionally coding in silence can help maintain cognitive flexibility.
Conclusion: Harmonizing Your Code and Playlist
The relationship between your coding playlist and algorithm choices is a fascinating intersection of cognitive science, music theory, and computer science. By understanding this connection, you can potentially optimize your coding environment to enhance your problem-solving skills, improve your algorithm selections, and boost your overall productivity.
Remember, the perfect coding soundtrack is highly personal. What works for one developer might be distracting for another. The key is to experiment with different genres, tempos, and styles to find what resonates with your coding rhythm.
As you continue your journey in software development, whether you’re just starting out with AlgoCademy or preparing for technical interviews with top tech companies, consider how your musical choices might be influencing your code. By harmonizing your playlist with your programming goals, you might just unlock new levels of coding proficiency and creativity.
So the next time you sit down to tackle a challenging algorithm or dive into a complex coding problem, take a moment to consider not just what you’re coding, but what you’re listening to. Your perfect algorithm solution might be just one playlist away!