Why Your Coding Habits are Actually Predicting Global Events
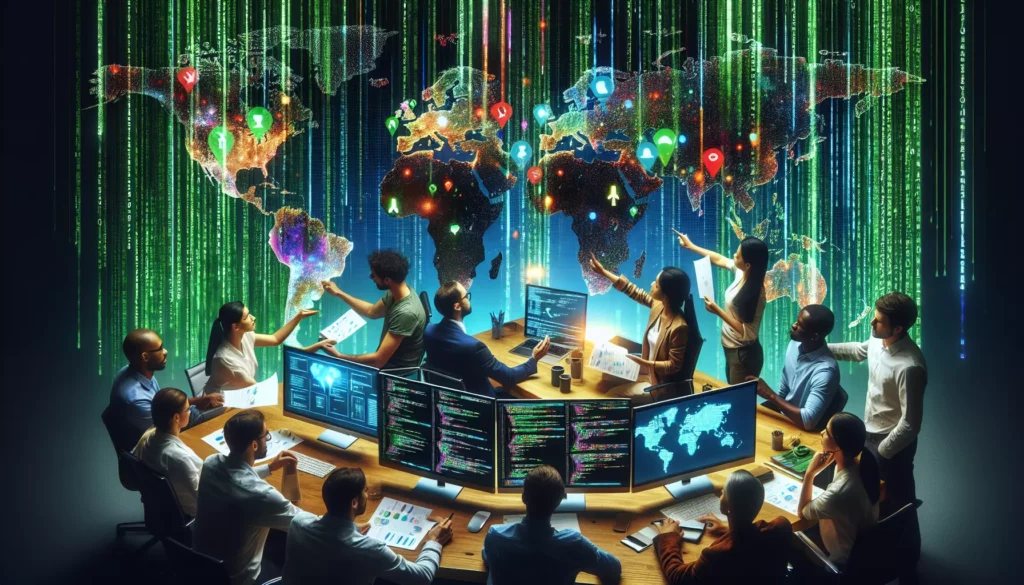
In the ever-evolving world of technology, it’s easy to get caught up in the minutiae of our daily coding tasks. We debug, we optimize, we refactor—all in the pursuit of creating more efficient and effective software. But what if I told you that your coding habits, those seemingly mundane practices you’ve developed over time, are actually part of a larger narrative? A narrative that’s subtly shaping and predicting global events. Intrigued? Let’s dive into this fascinating intersection of coding and world affairs.
The Butterfly Effect of Code
Before we delve into the specifics, it’s crucial to understand the concept of the butterfly effect in the context of coding. In chaos theory, the butterfly effect suggests that small changes in initial conditions can lead to large-scale and unpredictable consequences. Similarly, in the world of programming, a single line of code can have far-reaching implications that extend well beyond the confines of your IDE.
Consider this: when you’re working on a project, whether it’s a simple web application or a complex machine learning algorithm, you’re not just writing code. You’re contributing to a vast, interconnected ecosystem of digital information and processes that influence real-world outcomes.
The Global Language of Algorithms
As programmers, we often work with algorithms—step-by-step procedures for solving problems or performing tasks. These algorithms, particularly in the age of big data and artificial intelligence, have become a global language of sorts. They’re used to analyze market trends, predict weather patterns, and even influence political campaigns.
Your coding habits, the way you approach problem-solving, and the algorithms you favor are all part of this global algorithmic language. When you consistently choose one algorithm over another or optimize your code in a particular way, you’re inadvertently contributing to larger patterns that can be observed and analyzed on a global scale.
Case Study: The Stock Market Prediction
Let’s look at a concrete example. In 2008, a team of researchers from Indiana University found that they could predict movements in the stock market by analyzing Twitter sentiment. The algorithm they developed was based on natural language processing techniques that many programmers work with on a daily basis.
Now, imagine you’re a developer working on improving sentiment analysis algorithms. Your coding habits—how you preprocess text data, which machine learning models you prefer, how you handle edge cases—all contribute to the evolution of these predictive technologies. Your work, combined with that of thousands of other developers around the world, is shaping the tools that economists and financial analysts use to forecast market trends.
The Rise of Predictive Programming
One of the most intriguing aspects of modern software development is the concept of predictive programming. This isn’t about fortune-telling; it’s about creating systems that can anticipate future events based on current data and trends. Your coding habits play a crucial role in this predictive ecosystem.
Machine Learning and Global Predictions
Machine learning, a subset of artificial intelligence, is at the forefront of predictive programming. As a programmer, your approach to implementing machine learning algorithms can have a significant impact on their predictive capabilities. Let’s look at a simple example of a neural network implementation in Python:
import tensorflow as tf
from tensorflow import keras
model = keras.Sequential([
keras.layers.Dense(64, activation='relu', input_shape=(10,)),
keras.layers.Dense(64, activation='relu'),
keras.layers.Dense(1)
])
model.compile(optimizer='adam', loss='mean_squared_error')
# Train the model
model.fit(x_train, y_train, epochs=100, batch_size=32)
# Make predictions
predictions = model.predict(x_test)
In this code snippet, the choices you make—the number of layers, the activation functions, the optimizer—all influence the model’s ability to make accurate predictions. Now, scale this up to complex systems used for climate modeling, epidemic forecasting, or economic projections, and you begin to see how your coding habits can indirectly shape global predictions.
The Ethics of Predictive Code
With great power comes great responsibility, and the ability to predict global events through code is no exception. As developers, we must be acutely aware of the ethical implications of our work. Our coding habits don’t just affect the efficiency of our programs; they can potentially influence decision-making processes that impact millions of lives.
Bias in Algorithms
One of the most pressing concerns in predictive programming is the issue of bias. Our personal biases, often unconscious, can seep into the algorithms we create. For instance, if you’re developing a machine learning model to predict credit worthiness, your coding habits—such as how you handle missing data or which features you choose to include—can inadvertently perpetuate existing societal biases.
Consider this hypothetical code snippet for feature selection:
import pandas as pd
from sklearn.feature_selection import SelectKBest
from sklearn.feature_selection import f_classif
# Load the data
data = pd.read_csv('credit_data.csv')
# Separate features and target
X = data.drop('credit_score', axis=1)
y = data['credit_score']
# Select top 5 features
selector = SelectKBest(score_func=f_classif, k=5)
X_new = selector.fit_transform(X, y)
# Get the names of the selected features
selected_features = X.columns[selector.get_support(indices=True)].tolist()
print('Selected features:', selected_features)
In this example, the features you choose to include or exclude can have profound implications on the fairness of the resulting credit scoring system. If you habitually overlook certain types of data or rely too heavily on others, you may be contributing to a system that discriminates against certain groups of people.
The Globalization of Coding Practices
In today’s interconnected world, coding practices are no longer confined to geographical boundaries. The open-source movement, global coding bootcamps, and platforms like GitHub have created a melting pot of coding styles and practices. This globalization of coding habits is, in itself, a predictor of global trends.
The Rise of Coding Bootcamps
The proliferation of coding bootcamps around the world is a testament to the growing demand for programming skills. These intensive training programs often focus on practical, industry-relevant skills. The coding habits taught in these bootcamps can quickly spread across the globe, influencing how software is developed in various industries.
For example, if a particular design pattern or coding style gains popularity in Silicon Valley bootcamps, it’s likely to be adopted by developers worldwide. This can lead to a sort of “coding monoculture” that shapes how global software systems are built and maintained.
Open Source and Global Collaboration
The open-source movement has revolutionized how developers collaborate across borders. Your contributions to an open-source project can influence coding practices worldwide. Let’s say you create a popular JavaScript library for data visualization. Your coding habits—how you structure your code, the naming conventions you use, the way you handle edge cases—can become the de facto standard for developers around the world working on similar projects.
Here’s an example of how a simple utility function in an open-source library can spread globally:
// A utility function for formatting dates
export function formatDate(date, format = 'YYYY-MM-DD') {
const d = new Date(date);
const year = d.getFullYear();
const month = String(d.getMonth() + 1).padStart(2, '0');
const day = String(d.getDate()).padStart(2, '0');
return format
.replace('YYYY', year)
.replace('MM', month)
.replace('DD', day);
}
// Usage
console.log(formatDate(new Date(), 'DD/MM/YYYY')); // Outputs: 23/06/2023
If this function becomes widely adopted, it could influence how dates are formatted in applications worldwide, potentially affecting everything from financial reports to international communication systems.
The Impact of Coding Habits on Technological Trends
Your coding habits don’t just predict global events; they actively shape technological trends that have far-reaching consequences. The way you approach problem-solving, the tools you choose, and the coding paradigms you adopt all contribute to the direction of technological progress.
The Shift Towards Functional Programming
In recent years, there’s been a noticeable shift towards functional programming paradigms. This trend is not just a matter of personal preference; it reflects a broader move towards more predictable, testable, and scalable code. If you habitually write code in a functional style, you’re contributing to this global trend.
Consider this example of a functional approach to data processing:
const data = [1, 2, 3, 4, 5];
const processData = data =>
data
.filter(num => num % 2 === 0)
.map(num => num * 2)
.reduce((sum, num) => sum + num, 0);
console.log(processData(data)); // Outputs: 12
This functional approach to data processing is becoming increasingly common, influencing how large-scale data systems are designed and implemented. As more developers adopt these practices, we’re seeing a shift in how global data infrastructures are built and maintained.
The Rise of Microservices Architecture
Another trend that your coding habits might be influencing is the move towards microservices architecture. If you consistently write modular, loosely coupled code, you’re contributing to the broader trend of breaking down monolithic applications into smaller, more manageable services.
This architectural shift has profound implications for how global tech companies scale their operations and how startups approach building their products. It’s not just a technical decision; it affects business strategies, team structures, and even how companies compete in the global marketplace.
Coding Habits and Cybersecurity
In an era where cyber threats are a global concern, your coding habits play a crucial role in predicting and preventing security breaches. The way you handle user authentication, manage data, and implement encryption can have far-reaching consequences for global cybersecurity.
The Importance of Secure Coding Practices
Consider a simple example of password hashing:
import bcrypt
def hash_password(password):
salt = bcrypt.gensalt()
hashed = bcrypt.hashpw(password.encode('utf-8'), salt)
return hashed
def check_password(password, hashed):
return bcrypt.checkpw(password.encode('utf-8'), hashed)
# Usage
password = 'mysecretpassword'
hashed_password = hash_password(password)
# Checking the password
is_correct = check_password('mysecretpassword', hashed_password)
print('Password is correct:', is_correct)
By consistently implementing secure practices like proper password hashing, you’re contributing to a more robust global cybersecurity infrastructure. Your habits in this regard can influence how companies worldwide approach user data protection, potentially preventing large-scale data breaches that can have significant economic and political ramifications.
The Role of AI in Amplifying Coding Habits
Artificial Intelligence is not just a field that programmers work on; it’s increasingly becoming a tool that programmers work with. AI-powered coding assistants and code generation tools are becoming more prevalent, and they have the potential to amplify your coding habits on a global scale.
AI-Assisted Coding and Global Standardization
Consider tools like GitHub Copilot or OpenAI’s GPT models. These AI systems learn from vast repositories of code and can generate code snippets based on natural language prompts. Your coding habits, when fed into these systems, can influence the code suggestions provided to developers worldwide.
For instance, if you consistently use a particular design pattern or coding style, and this becomes part of the training data for an AI coding assistant, it could lead to that pattern being suggested to developers across the globe. This creates a feedback loop where individual coding habits can quickly become global standards.
The Environmental Impact of Coding Habits
It might seem far-fetched, but your coding habits can have a significant environmental impact, contributing to global climate trends. The efficiency of your code, particularly in large-scale applications, directly affects energy consumption and, consequently, carbon emissions.
Green Coding Practices
Consider the difference between an inefficient sorting algorithm and an optimized one when applied to large datasets:
def bubble_sort(arr):
n = len(arr)
for i in range(n):
for j in range(0, n-i-1):
if arr[j] > arr[j+1]:
arr[j], arr[j+1] = arr[j+1], arr[j]
return arr
def quick_sort(arr):
if len(arr) <= 1:
return arr
pivot = arr[len(arr) // 2]
left = [x for x in arr if x < pivot]
middle = [x for x in arr if x == pivot]
right = [x for x in arr if x > pivot]
return quick_sort(left) + middle + quick_sort(right)
# Usage
import random
import time
# Generate a large random array
arr = [random.randint(1, 1000) for _ in range(10000)]
# Time the bubble sort
start = time.time()
bubble_sort(arr.copy())
end = time.time()
print(f"Bubble sort took {end - start} seconds")
# Time the quick sort
start = time.time()
quick_sort(arr.copy())
end = time.time()
print(f"Quick sort took {end - start} seconds")
The choice between these two sorting algorithms can have a significant impact on energy consumption when scaled up to large systems processing vast amounts of data. By consistently choosing more efficient algorithms and writing optimized code, you’re contributing to reduced energy consumption on a global scale.
The Future of Coding and Global Predictions
As we look to the future, the relationship between coding habits and global events is likely to become even more pronounced. Emerging technologies like quantum computing and advanced AI systems will amplify the impact of individual coding decisions.
Quantum Computing and Global Predictions
Quantum computing has the potential to revolutionize how we approach complex computational problems. As quantum computers become more accessible, your coding habits in this new paradigm could have far-reaching effects on global prediction models.
For example, quantum algorithms could dramatically improve our ability to simulate complex systems, from financial markets to climate models. Your approach to quantum programming could influence how these simulations are constructed and interpreted, potentially reshaping global decision-making processes.
The Ethical Imperative
As the impact of our coding habits on global events becomes more apparent, there’s an increasing ethical imperative for developers to consider the broader implications of their work. This goes beyond just writing efficient code; it involves considering the societal, environmental, and economic impacts of the systems we create.
Platforms like AlgoCademy play a crucial role in this context. By focusing on algorithmic thinking and problem-solving skills, they’re not just teaching coding; they’re shaping the mindset of future developers who will be at the forefront of these global trends.
Conclusion: The Global Coder
In conclusion, your coding habits are far more than personal quirks or professional preferences. They are subtle yet powerful forces shaping the digital landscape and, by extension, the physical world. From influencing global economic models to contributing to cybersecurity trends, from shaping AI development to impacting environmental sustainability, your code is a thread in the complex tapestry of global events.
As we’ve explored, the butterfly effect of code is real and pervasive. A single line of code, a chosen algorithm, or a particular coding style can ripple out to affect systems and decisions on a global scale. This realization comes with both excitement and responsibility.
The next time you sit down to code, remember that you’re not just solving a local problem or building a single application. You’re participating in a global dialogue, contributing to worldwide trends, and potentially predicting—or even influencing—future events. Your coding habits matter, not just for your project or your team, but for the world at large.
As we move forward in this interconnected digital age, let’s code not just with efficiency and elegance in mind, but with a keen awareness of our global impact. Let’s strive to be ethical, thoughtful, and responsible global coders, using our skills and habits to shape a better, more predictable, and more equitable world.
After all, in the grand algorithm of global events, every variable counts—and you, the coder, are a crucial variable in this complex equation. So code on, with the knowledge that your habits are not just predicting global events, but actively shaping the future of our interconnected world.