Why Your Code Works But You Can’t Explain Why: The Knowledge Gap in Programming
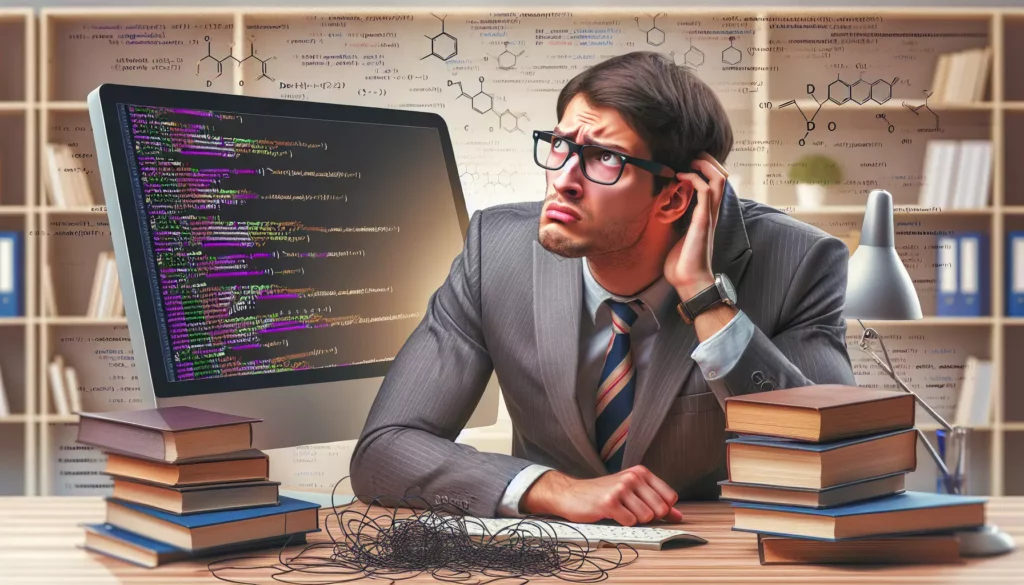
Have you ever written a piece of code that miraculously works, but when someone asks you to explain why, you find yourself at a loss for words? If so, you’re not alone. This phenomenon is so common in the programming world that it has earned its own nickname: “programming by coincidence.”
In this comprehensive guide, we’ll explore the reasons behind this knowledge gap, why it happens to both beginners and experienced developers, and most importantly, how to bridge this gap to become a more confident and competent programmer.
The “It Works, But I Don’t Know Why” Syndrome
Let’s start with a scenario most programmers have experienced: You’re working on a challenging problem. After numerous attempts, your code finally runs without errors and produces the correct output. There’s a moment of triumph! But then, a colleague or instructor asks, “Can you explain how your solution works?” Suddenly, that triumph transforms into uncertainty.
This situation highlights a fundamental truth about programming: making code work and understanding why it works are two different skills.
Why This Happens to Everyone
This phenomenon isn’t limited to beginners. Even senior developers occasionally find themselves in situations where their code works through what feels like magic rather than methodical understanding. Here’s why this happens across all experience levels:
- Trial and Error Approach: When facing challenging problems, many developers resort to trying different approaches until something works, without fully grasping the underlying principles.
- Copy-Paste Programming: With abundant resources like Stack Overflow, it’s tempting to borrow code snippets without fully understanding their mechanics.
- Deadline Pressure: When time is limited, the priority becomes getting functional code rather than fully comprehending every aspect of the solution.
- Complexity Overload: Modern programming involves numerous layers of abstraction, making it difficult to understand every component thoroughly.
The Dangers of Not Understanding Your Code
While getting code to work might seem like the ultimate goal, not understanding why it works can lead to several problems:
1. Debugging Nightmares
When code that you don’t fully understand stops working, debugging becomes exponentially more difficult. Without comprehension of the underlying mechanisms, you’re essentially searching for a needle in a haystack without knowing what a needle looks like.
Consider this JavaScript example:
function processArray(arr) {
return arr.filter(Boolean);
}
console.log(processArray([0, 1, false, 2, "", 3])); // Outputs: [1, 2, 3]
If you don’t understand that Boolean
as a function converts values to their boolean equivalent (and that 0
, false
, and ""
are falsy in JavaScript), you might be surprised by the output and struggle to modify this code for different requirements.
2. Maintenance Challenges
Code often needs to be maintained and updated. If you or your team doesn’t understand why certain code works, making changes becomes risky. What seems like a minor adjustment could break the entire system.
3. Limited Growth
Relying on coincidental programming limits your growth as a developer. True mastery comes from understanding, not just doing.
4. Technical Debt
Code that works but isn’t understood creates technical debt. Eventually, someone will need to decipher it, potentially requiring more time than if it had been properly understood from the beginning.
Common Scenarios Where Understanding Lags Behind Implementation
Let’s examine some specific situations where developers often find their code working without fully understanding why:
Recursive Functions
Recursion is a concept many programmers struggle to grasp intuitively. Consider this classic factorial function:
function factorial(n) {
if (n <= 1) return 1;
return n * factorial(n - 1);
}
console.log(factorial(5)); // Outputs: 120
Many developers can write this code and verify it works for calculating factorials, but tracing through the execution stack and understanding exactly how the recursive calls resolve requires a deeper level of comprehension.
Advanced Regular Expressions
Regular expressions often fall into the category of “write once, pray it works forever.” Consider this example for validating an email address:
const emailRegex = /^[a-zA-Z0-9._%+-]+@[a-zA-Z0-9.-]+\.[a-zA-Z]{2,}$/;
console.log(emailRegex.test("user@example.com")); // true
Many developers use regular expressions that they’ve found online without fully understanding each component and metacharacter.
Framework Magic
Modern frameworks abstract away many complexities, which is helpful for productivity but can hinder understanding. For instance, a React component might work correctly, but the developer might not fully grasp the component lifecycle or state management principles.
function Counter() {
const [count, setCount] = React.useState(0);
return (
<div>
<p>You clicked {count} times</p>
<button onClick={() => setCount(count + 1)}>
Click me
</button>
</div>
);
}
This simple counter works, but understanding React’s rendering process, hook rules, and state batching requires deeper knowledge.
Complex Algorithms
Developers often implement algorithms they don’t fully understand, especially when solving complex problems like dynamic programming or graph traversal.
function fibonacci(n, memo = {}) {
if (n in memo) return memo[n];
if (n <= 2) return 1;
memo[n] = fibonacci(n - 1, memo) + fibonacci(n - 2, memo);
return memo[n];
}
console.log(fibonacci(50)); // Works efficiently with memoization
A developer might implement this memoized Fibonacci function after seeing similar patterns, without fully understanding why memoization prevents exponential time complexity.
The Root Causes of the Understanding Gap
To address this issue, we need to identify its root causes:
1. Learning Style Mismatches
Programming education often focuses on syntax and implementation rather than conceptual understanding. This approach can work for some learners but leaves others with knowledge gaps.
2. Abstraction Layers
Modern programming involves numerous abstraction layers. From high-level languages that hide memory management to frameworks that abstract DOM manipulation, these layers enhance productivity but can obscure understanding.
3. Result-Oriented Culture
The programming culture often values working code over deep understanding. This prioritization can discourage the time-consuming process of building comprehensive knowledge.
4. Cognitive Load
Programming requires juggling multiple concepts simultaneously. This cognitive load can make it difficult to process the “why” behind code when you’re focused on making it work.
How to Bridge the Knowledge Gap
Now for the actionable part: how can you ensure you not only write working code but also understand why it works? Here are strategies to bridge that knowledge gap:
1. Embrace the “Explain It Like I’m Five” Technique
One of the most effective ways to test your understanding is to explain your code in simple terms. If you can’t explain a concept in simple language, you probably don’t understand it well enough.
Try this exercise: Take a piece of code you’ve written and explain each line as if you’re teaching someone with no programming experience. Where do you struggle? Those areas highlight your knowledge gaps.
2. Trace Code Execution Manually
Instead of relying solely on the computer to execute your code, trace through it manually with specific input values. This process forces you to think about what happens at each step.
For example, let’s trace the factorial function with n=3:
- Call factorial(3)
- 3 > 1, so return 3 * factorial(2)
- Call factorial(2)
- 2 > 1, so return 2 * factorial(1)
- Call factorial(1)
- 1 <= 1, so return 1
- Now factorial(2) completes: return 2 * 1 = 2
- Finally, factorial(3) completes: return 3 * 2 = 6
This step-by-step tracing builds a mental model of execution flow.
3. Implement From First Principles
Instead of copying solutions, try implementing algorithms and data structures from scratch based on their conceptual descriptions. This approach forces you to understand the underlying principles.
For instance, rather than copying a sorting algorithm, read about how bubble sort works conceptually, then implement it yourself:
function bubbleSort(arr) {
const n = arr.length;
let swapped;
do {
swapped = false;
for (let i = 0; i < n - 1; i++) {
if (arr[i] > arr[i + 1]) {
// Swap elements
[arr[i], arr[i + 1]] = [arr[i + 1], arr[i]];
swapped = true;
}
}
} while (swapped);
return arr;
}
When you implement it yourself, you’re forced to think about the logic behind each step.
4. Break It to Understand It
Once your code works, experiment by intentionally breaking it. Change parts of the code and predict what will happen. Then run it to see if your prediction was correct. This approach helps identify which parts of your code are essential and why.
5. Use Visualization Tools
Visualization tools can help bridge the gap between abstract code and concrete behavior. Tools like Python Tutor allow you to visualize code execution step-by-step.
For algorithms and data structures, websites like VisuAlgo provide interactive visualizations that can enhance understanding.
6. Read Documentation and Source Code
When using libraries or frameworks, don’t just use their APIs. Read the documentation to understand the design principles and, when possible, explore the source code.
For example, if you’re using a sorting method in a language’s standard library, look up its implementation to understand the algorithm it uses.
7. Teach Others
Teaching is one of the most effective ways to solidify understanding. When you teach, you identify gaps in your knowledge and are forced to fill them.
Consider starting a blog, contributing to forums like Stack Overflow, or mentoring less experienced programmers. The questions others ask will highlight aspects you haven’t fully considered.
Practical Examples: From Confusion to Clarity
Let’s look at some common examples where code might work but understanding lags, along with approaches to build deeper comprehension:
Example 1: Array Methods in JavaScript
A developer might use map
, filter
, and reduce
effectively without fully understanding their mechanics:
const numbers = [1, 2, 3, 4, 5];
// Double each number
const doubled = numbers.map(num => num * 2);
// Get only even numbers
const evens = numbers.filter(num => num % 2 === 0);
// Sum all numbers
const sum = numbers.reduce((total, num) => total + num, 0);
Path to Understanding: Implement these methods yourself to understand their inner workings:
// Custom map implementation
Array.prototype.myMap = function(callback) {
const result = [];
for (let i = 0; i < this.length; i++) {
result.push(callback(this[i], i, this));
}
return result;
};
// Test it
console.log(numbers.myMap(num => num * 2));
By implementing these methods yourself, you gain insight into how they iterate over arrays and apply callback functions.
Example 2: Asynchronous JavaScript
Promises and async/await can be particularly confusing:
async function fetchUserData(userId) {
try {
const response = await fetch(`https://api.example.com/users/${userId}`);
const data = await response.json();
return data;
} catch (error) {
console.error('Error fetching user data:', error);
return null;
}
}
Path to Understanding: Break down the async code into its Promise-based equivalent:
function fetchUserDataWithPromises(userId) {
return fetch(`https://api.example.com/users/${userId}`)
.then(response => response.json())
.then(data => data)
.catch(error => {
console.error('Error fetching user data:', error);
return null;
});
}
Then, trace the execution flow with specific focus on the event loop and how promises resolve.
Example 3: CSS Flexbox
Many developers use Flexbox successfully without fully understanding its principles:
.container {
display: flex;
justify-content: space-between;
align-items: center;
}
.item {
flex: 1;
}
Path to Understanding: Create a mental model of the flex container and items. Visualize the main axis and cross axis. Experiment by changing one property at a time and observing the results. Use tools like Flexbox Froggy to build intuition through practice.
Advanced Understanding: Beyond Making It Work
As you progress in your programming journey, aim to move beyond just making code work to understanding it at multiple levels:
1. Syntactic Understanding
This is the most basic level: knowing what the code syntax means and how to write it correctly.
2. Semantic Understanding
This involves understanding what the code does and how it achieves its purpose.
3. Algorithmic Understanding
At this level, you comprehend the efficiency and performance characteristics of your code, including time and space complexity.
4. Architectural Understanding
This highest level involves seeing how your code fits into the larger system and understanding the design patterns and principles at play.
True mastery involves all four levels. When you’re stuck at “it works but I don’t know why,” you typically have syntactic understanding but lack one or more of the higher levels.
Common Misconceptions About Code Understanding
Let’s address some misconceptions that can hinder the journey toward deeper understanding:
Misconception 1: “I need to understand everything before I can write code”
Reality: Learning to code is an iterative process. You’ll never understand everything, and that’s okay. Start with what you know, and build understanding as you go.
Misconception 2: “If it works, understanding doesn’t matter”
Reality: Working code is just the beginning. Understanding enables you to debug, optimize, and extend your code effectively.
Misconception 3: “I’m not smart enough to understand complex concepts”
Reality: Understanding comes from persistence and effective learning strategies, not innate intelligence. Complex concepts become simpler when broken down properly.
Misconception 4: “Experienced programmers always understand their code fully”
Reality: Even senior developers have knowledge gaps. The difference is they know how to identify and address these gaps effectively.
Building a Deeper Understanding Culture
Beyond individual practices, we can foster environments that value understanding:
In Educational Settings
- Emphasize conceptual understanding alongside syntax
- Encourage questions about why code works, not just how to make it work
- Incorporate visualization and hands-on experimentation
- Assess understanding through explanation, not just working code
In Professional Settings
- Allocate time for learning and exploration
- Conduct code reviews that focus on understanding, not just correctness
- Document the “why” behind code decisions, not just implementation details
- Create a safe environment where admitting knowledge gaps is encouraged
Conclusion: The Journey from “It Works” to “I Understand Why”
The gap between making code work and understanding why it works is a natural part of the programming journey. Rather than being discouraged by this gap, embrace it as an opportunity for growth.
Remember that understanding is not binary but exists on a spectrum. Each time you take a working piece of code and investigate why it works, you’re building deeper knowledge that will serve you throughout your programming career.
The next time you find yourself with code that works mysteriously, don’t just celebrate and move on. Take it as an invitation to explore, learn, and truly understand the magic behind your creation. This curiosity and commitment to understanding will transform you from someone who can write code into someone who truly masters the art of programming.
In the words of Richard Feynman: “What I cannot create, I do not understand.” Perhaps we should add: “What works but I cannot explain, I do not yet fully understand.”
So embrace the journey from code that simply works to code that you comprehensively understand. Your future self will thank you when debugging, optimizing, or extending that code months or years down the line.