Why Your Code Splitting Isn’t Improving Performance
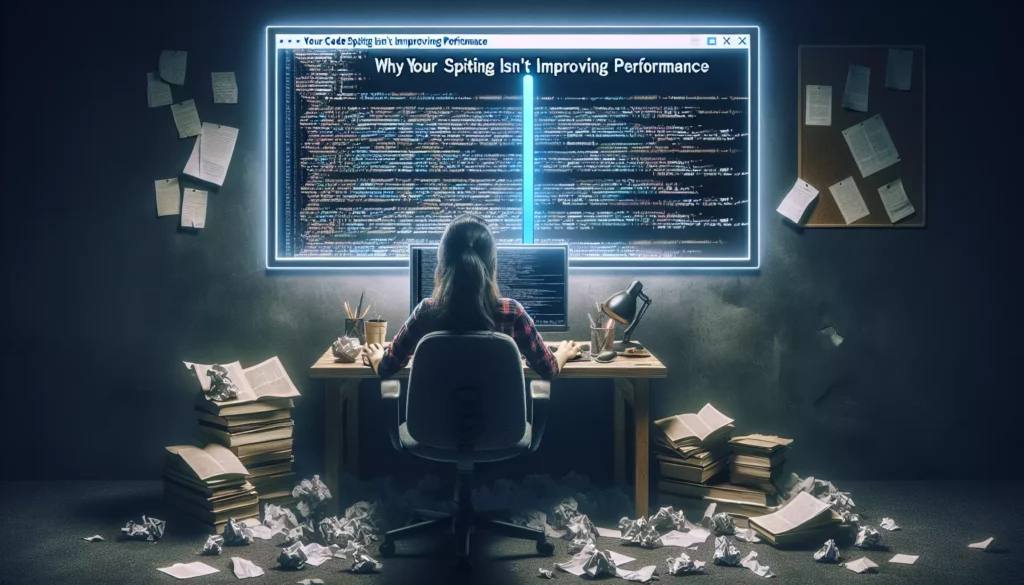
Code splitting is often touted as a silver bullet for web performance optimization. Break up your JavaScript bundle, load only what you need, and watch your application speed soar, right? Yet many developers find themselves disappointed when their carefully implemented code splitting strategy yields minimal performance improvements or, worse, degrades user experience.
If you’ve implemented code splitting but aren’t seeing the performance gains you expected, you’re not alone. In this comprehensive guide, we’ll explore why your code splitting efforts might be falling short and how to fix these issues to achieve real performance benefits.
Understanding Code Splitting: The Promise vs. Reality
Before diving into the problems, let’s briefly recap what code splitting is supposed to accomplish.
The Promise of Code Splitting
Code splitting is a technique that breaks your application bundle into smaller chunks, allowing you to:
- Load only the code necessary for the current view
- Reduce initial load time by deferring non-critical code
- Improve Time to Interactive (TTI) metrics
- Reduce main thread blocking during page load
When implemented correctly, code splitting can dramatically improve perceived performance, especially on slower connections and less powerful devices.
The Reality Many Developers Face
Despite following best practices, many developers encounter these common scenarios:
- Performance metrics show minimal improvement or even regression
- Users experience new loading delays during navigation
- Lighthouse scores remain unchanged despite significant effort
- The application feels slower in real-world usage
Let’s explore why these disconnects happen and how to address them.
Common Reason #1: Improper Chunking Strategy
One of the most common issues with code splitting implementations is poor chunking strategy. This manifests in several ways:
Too Many Small Chunks
While it might seem logical to split your code into many small chunks for maximum granularity, this approach can backfire due to network overhead. Each chunk requires a separate HTTP request, and the browser can only make a limited number of parallel requests.
Consider this React example where every component is lazily loaded:
// Anti-pattern: Too many small chunks
const Header = React.lazy(() => import('./Header'));
const Sidebar = React.lazy(() => import('./Sidebar'));
const Footer = React.lazy(() => import('./Footer'));
const ProfileCard = React.lazy(() => import('./ProfileCard'));
const UserAvatar = React.lazy(() => import('./UserAvatar'));
// ... and so on for dozens of small components
This creates a waterfall of requests that can actually slow down your application, especially on slower networks where request overhead is significant.
Chunks That Don’t Align With User Flows
If your chunks don’t align with how users actually navigate through your application, you may be loading code too late, creating jarring loading experiences.
The Solution: Strategic Chunking
Instead of arbitrarily splitting your code, develop a chunking strategy based on:
- Route-based splitting: Split at the route level first, which naturally aligns with user navigation patterns
- Feature-based splitting: Group related components and utilities into feature bundles
- Critical path optimization: Ensure above-the-fold content loads quickly without dependencies on unnecessary code
Here’s a more balanced approach:
// Better approach: Route-based chunks with feature grouping
const Dashboard = React.lazy(() => import('./routes/Dashboard'));
const UserProfile = React.lazy(() => import('./routes/UserProfile'));
const Settings = React.lazy(() => import('./routes/Settings'));
// Only split very large features within routes
const DataVisualization = React.lazy(() => import('./features/DataVisualization'));
Common Reason #2: Missing or Poor Preloading Strategy
Code splitting without a proper preloading strategy often leads to poor perceived performance. When users navigate to a new section, they encounter loading spinners or blank screens while chunks download.
The Problem: Reactive Loading
Many code splitting implementations only trigger chunk loading when a component is about to render. This reactive approach means users always experience a loading delay, even if it’s brief.
// Problematic approach: Loading only when needed
function App() {
return (
<Suspense fallback={<LoadingSpinner />}>
{isSettingsPage && <Settings />}
</Suspense>
);
}
The Solution: Predictive Preloading
Implement a predictive preloading strategy that anticipates user actions:
- Route preloading: Preload chunks for likely next routes
- Interaction-based preloading: Load chunks when users hover over links or buttons
- Idle-time loading: Use browser idle time to preload chunks
Here’s how you might implement these strategies:
// Route-based preloading with React Router
const Dashboard = React.lazy(() => import('./routes/Dashboard'));
// Preload on hover
function NavigationLink({ to, children }) {
const prefetchChunk = () => {
const chunk = import(`./routes${to}`);
};
return (
<Link
to={to}
onMouseEnter={prefetchChunk}
onTouchStart={prefetchChunk}
>
{children}
</Link>
);
}
// Idle-time preloading
if ('requestIdleCallback' in window) {
requestIdleCallback(() => {
// Preload likely next chunks
import('./routes/FrequentlyAccessedRoute');
});
}
Modern frameworks often provide utilities for this. For example, Next.js offers the prefetch
prop on its Link
component, and Gatsby has similar functionality.
Common Reason #3: Shared Dependencies Aren’t Optimized
Another common pitfall is failing to properly handle shared dependencies across chunks.
The Problem: Duplicate Code Across Chunks
Without proper configuration, the same libraries or utility functions can be included in multiple chunks, increasing the total download size.
For example, if both your Dashboard
and Settings
chunks use the same charting library, users might download that library twice.
The Solution: Extract Common Dependencies
Configure your bundler to extract common dependencies into shared chunks that can be cached and reused:
For webpack, you can use the SplitChunksPlugin
:
// webpack.config.js
module.exports = {
// ...
optimization: {
splitChunks: {
chunks: 'all',
cacheGroups: {
vendors: {
test: /[\\/]node_modules[\\/]/,
name: 'vendors',
chunks: 'all',
priority: 20
},
common: {
name: 'common',
minChunks: 2,
chunks: 'all',
priority: 10,
reuseExistingChunk: true,
enforce: true
}
}
}
}
};
This configuration extracts:
- Third-party libraries into a ‘vendors’ chunk
- Common code used across multiple chunks into a ‘common’ chunk
This prevents duplication and improves caching efficiency.
Common Reason #4: Poor Loading State Management
Even with optimal chunking and preloading, users will occasionally encounter loading states. How you handle these states dramatically affects perceived performance.
The Problem: Jarring or Empty Loading States
Common issues include:
- Blank screens while chunks load
- Generic spinners that don’t provide context
- Layout shifts when chunks finally load
- Flickering loading indicators for fast loads
// Problematic approach: Generic loading state
function App() {
return (
<Suspense fallback={<div>Loading...</div>}>
<LazyComponent />
</Suspense>
);
}
The Solution: Sophisticated Loading Strategies
Implement more sophisticated loading strategies:
- Skeleton screens that match the layout of the loading content
- Delayed loading indicators to prevent flickering for fast loads
- Content placeholders that maintain layout stability
- Progressive loading where partial content is shown as it becomes available
// Better approach: Skeleton screens with delayed indicators
function App() {
return (
<Suspense
fallback={
<DelayedFallback
minDisplayTime={500}
delay={200}
>
<SkeletonScreen layout="dashboard" />
</DelayedFallback>
}
>
<Dashboard />
</Suspense>
);
}
// Component that delays showing fallback for fast loads
// and ensures minimum display time to prevent flickering
function DelayedFallback({ children, delay, minDisplayTime }) {
const [show, setShow] = useState(false);
const startTime = useRef(0);
useEffect(() => {
const timer = setTimeout(() => {
startTime.current = Date.now();
setShow(true);
}, delay);
return () => {
const elapsed = Date.now() - startTime.current;
const remainingTime = minDisplayTime - elapsed;
if (remainingTime > 0 && startTime.current !== 0) {
// Keep showing the loader for the minimum time
setTimeout(() => {}, remainingTime);
}
clearTimeout(timer);
};
}, [delay, minDisplayTime]);
return show ? children : null;
}
Common Reason #5: Bundle Analysis Blind Spots
Many developers implement code splitting without proper analysis of their bundle composition, leading to suboptimal splitting decisions.
The Problem: Flying Blind
Without visibility into what’s actually in your bundles, you might:
- Split small components that don’t warrant their own chunks
- Miss large libraries that should be split
- Overlook duplicate code across chunks
- Make optimization decisions based on assumptions rather than data
The Solution: Bundle Analysis and Monitoring
Use bundle analysis tools to make data-driven decisions:
- Webpack Bundle Analyzer: Visualize the content of your webpack bundles
- Import Cost: See the size impact of imports directly in your editor
- Performance budgets: Set size limits for your chunks and get warnings when they’re exceeded
- Runtime monitoring: Track real-user metrics to see how your code splitting affects actual users
Setting up Webpack Bundle Analyzer:
// Install the plugin
// npm install --save-dev webpack-bundle-analyzer
// webpack.config.js
const { BundleAnalyzerPlugin } = require('webpack-bundle-analyzer');
module.exports = {
// ...
plugins: [
new BundleAnalyzerPlugin({
analyzerMode: process.env.ANALYZE === 'true' ? 'server' : 'disabled',
generateStatsFile: true,
statsFilename: 'stats.json',
})
]
};
Then run your build with analysis enabled:
ANALYZE=true npm run build
This will open a visual representation of your bundle composition, helping you identify optimization opportunities.
Common Reason #6: Network Considerations Ignored
Code splitting that works well in development or on fast connections may perform poorly under real-world network conditions.
The Problem: Optimizing for Ideal Conditions
Many code splitting strategies ignore:
- High latency connections where multiple requests are costly
- Bandwidth limitations where total bundle size matters more than splitting
- Connection reliability issues where requests may fail
- Cold vs. warm cache scenarios
The Solution: Network-Aware Code Splitting
Adapt your code splitting strategy to different network conditions:
- Connection-aware loading: Adjust your strategy based on the user’s connection
- Retry mechanisms: Handle chunk loading failures gracefully
- Prioritize critical chunks: Ensure the most important code loads first
- Test on realistic networks: Use throttling to simulate various connection types
// Connection-aware code splitting
function App() {
const [loadStrategy, setLoadStrategy] = useState('default');
useEffect(() => {
// Check connection type if available
if ('connection' in navigator) {
const connection = navigator.connection;
if (connection.saveData) {
// User has requested data saving mode
setLoadStrategy('minimal');
} else if (connection.effectiveType === '4g') {
// Fast connection, can be more aggressive with preloading
setLoadStrategy('aggressive');
} else if (connection.effectiveType === '3g' || connection.effectiveType === '2g') {
// Slower connection, be more conservative
setLoadStrategy('conservative');
}
// Listen for connection changes
connection.addEventListener('change', updateLoadStrategy);
return () => connection.removeEventListener('change', updateLoadStrategy);
}
}, []);
// Different loading components based on connection
const LoadingProvider = useMemo(() => {
switch (loadStrategy) {
case 'aggressive':
return AggressiveLoadingProvider;
case 'conservative':
return ConservativeLoadingProvider;
case 'minimal':
return MinimalLoadingProvider;
default:
return DefaultLoadingProvider;
}
}, [loadStrategy]);
return (
<LoadingProvider>
<Routes />
</LoadingProvider>
);
}
Common Reason #7: Framework-Specific Pitfalls
Different frameworks have their own approaches to code splitting, and misunderstanding these can lead to suboptimal implementations.
React-Specific Issues
Common React code splitting issues include:
- Overusing
React.lazy()
for small components - Not properly handling Suspense boundaries
- Inefficient context usage across split points
// Problematic React approach
// Too many small lazy components without proper boundaries
const Button = React.lazy(() => import('./Button'));
const Icon = React.lazy(() => import('./Icon'));
const Input = React.lazy(() => import('./Input'));
function Form() {
// This creates multiple suspense boundaries and waterfalls
return (
<div>
<Suspense fallback={<div>Loading button...</div>}>
<Button />
</Suspense>
<Suspense fallback={<div>Loading input...</div>}>
<Input />
</Suspense>
</div>
);
}
Better approach:
// Group related components in logical chunks
const FormElements = React.lazy(() => import('./FormElements'));
function Form() {
return (
<Suspense fallback={<FormSkeleton />}>
<FormElements />
</Suspense>
);
}
Vue-Specific Issues
In Vue, common pitfalls include:
- Misusing async components for small, frequently used components
- Not leveraging webpack chunks properly with dynamic imports
- Inefficient component registration patterns
// Problematic Vue approach
// Vue 3 example
const routes = [
{
path: '/dashboard',
// No explicit chunk name, may lead to unpredictable chunk names
component: () => import('./views/Dashboard.vue')
},
{
path: '/settings',
component: () => import('./views/Settings.vue')
}
]
Better approach:
// Better Vue approach with named chunks
const routes = [
{
path: '/dashboard',
component: () => import(/* webpackChunkName: "dashboard" */ './views/Dashboard.vue'),
// Preload related chunks
beforeEnter(to, from, next) {
// Preload related views that might be accessed from dashboard
import(/* webpackChunkName: "dashboard-analytics" */ './views/DashboardAnalytics.vue');
next();
}
},
{
path: '/settings',
component: () => import(/* webpackChunkName: "settings" */ './views/Settings.vue')
}
]
Angular-Specific Issues
Angular provides built-in routing-based code splitting, but issues can arise with:
- Improper module organization
- Not using preloading strategies
- Forgetting to optimize shared services
// Problematic Angular approach
// app-routing.module.ts
const routes: Routes = [
{ path: 'dashboard', loadChildren: () => import('./dashboard/dashboard.module').then(m => m.DashboardModule) },
{ path: 'settings', loadChildren: () => import('./settings/settings.module').then(m => m.SettingsModule) }
// No preloading strategy specified
];
Better approach:
// Better Angular approach with preloading
// app-routing.module.ts
const routes: Routes = [
{
path: 'dashboard',
loadChildren: () => import('./dashboard/dashboard.module').then(m => m.DashboardModule),
data: { preload: true } // Custom preloading flag
},
{
path: 'settings',
loadChildren: () => import('./settings/settings.module').then(m => m.SettingsModule)
}
];
@NgModule({
imports: [RouterModule.forRoot(routes, {
preloadingStrategy: CustomPreloadingStrategy
})],
exports: [RouterModule]
})
export class AppRoutingModule { }
// custom-preloading-strategy.ts
@Injectable({ providedIn: 'root' })
export class CustomPreloadingStrategy implements PreloadingStrategy {
preload(route: Route, load: () => Observable<any>): Observable<any> {
return route.data && route.data.preload ? load() : EMPTY;
}
}
Common Reason #8: Inefficient Cache Utilization
Code splitting can interfere with effective caching if not implemented with caching in mind.
The Problem: Cache Invalidation Issues
Common caching issues with code splitting include:
- Chunks that change frequently, reducing cache effectiveness
- Chunk naming strategies that don’t leverage long-term caching
- Inefficient cache invalidation when only small parts change
The Solution: Cache-Optimized Chunking
Implement cache-aware code splitting:
- Content hashing: Include content hashes in chunk filenames
- Stable chunking: Ensure chunk boundaries don’t change unnecessarily
- Vendor separation: Keep rarely changing vendor code in separate chunks
- Runtime/framework separation: Extract framework code that changes less frequently
Webpack configuration for optimal caching:
// webpack.config.js
module.exports = {
output: {
filename: '[name].[contenthash].js',
chunkFilename: '[name].[contenthash].chunk.js'
},
optimization: {
moduleIds: 'deterministic', // Keep module ids stable when vendor modules don't change
runtimeChunk: 'single', // Extract webpack runtime
splitChunks: {
cacheGroups: {
vendor: {
test: /[\\/]node_modules[\\/]/,
name: 'vendors',
chunks: 'all',
},
// Separate frequently updated libraries to prevent invalidating the entire vendor chunk
reactDom: {
test: /[\\/]node_modules[\\/](react-dom)[\\/]/,
name: 'react-dom',
chunks: 'all',
priority: 30, // Higher priority than vendor
}
}
}
}
};
Common Reason #9: Server-Side Rendering Complications
Code splitting can be particularly challenging when combined with server-side rendering (SSR).
The Problem: Client/Server Mismatches
Common SSR-related code splitting issues include:
- Hydration errors when client and server render different content
- Waterfall requests delaying interactivity after initial render
- Duplicate data fetching between server and client
- Complex state management across split points
The Solution: SSR-Aware Code Splitting
Adapt your code splitting strategy for SSR:
- Dynamic imports in useEffect: Load non-critical components after hydration
- Critical CSS extraction: Include only necessary styles in initial render
- State serialization: Properly transfer state from server to client
- Hybrid approaches: Consider approaches like partial hydration or islands architecture
Here’s an example using Next.js:
// pages/index.js
import dynamic from 'next/dynamic'
import { useEffect, useState } from 'react'
// Critical components loaded during SSR
import CriticalHeader from '../components/CriticalHeader'
import MainContent from '../components/MainContent'
// Non-critical components loaded client-side only
const ChatWidget = dynamic(() => import('../components/ChatWidget'), {
ssr: false,
loading: () => <div className="chat-placeholder" />
})
const HeavyAnalytics = dynamic(() => import('../components/HeavyAnalytics'))
export default function Home({ initialData }) {
const [showAnalytics, setShowAnalytics] = useState(false)
// Load analytics component only when needed
useEffect(() => {
const timer = setTimeout(() => {
// Load analytics after core page is interactive
setShowAnalytics(true)
}, 3000)
return () => clearTimeout(timer)
}, [])
return (
<div>
<CriticalHeader />
<MainContent data={initialData} />
{/* Chat widget loads client-side only */}
<ChatWidget />
{/* Analytics loads after delay */}
{showAnalytics && <HeavyAnalytics />}
</div>
)
}
export async function getServerSideProps() {
// Fetch only critical data for initial render
const initialData = await fetchCriticalData()
return {
props: { initialData }
}
}
Common Reason #10: Monitoring and Measurement Gaps
Finally, many code splitting efforts fail because developers don’t properly measure their impact or monitor performance over time.
The Problem: Flying Blind After Deployment
Without proper monitoring:
- You can’t tell if code splitting is actually helping real users
- Regression issues may go unnoticed
- You lack data to make further optimization decisions
- Success becomes subjective rather than data-driven
The Solution: Comprehensive Performance Monitoring
Implement robust performance monitoring:
- Real User Monitoring (RUM): Track actual user experiences
- Core Web Vitals tracking: Monitor LCP, FID, CLS metrics
- Chunk loading analytics: Track which chunks load, when, and how long they take
- Error tracking: Monitor for chunk loading failures
- A/B testing: Compare different code splitting strategies
Implementation example with web-vitals:
// performance-monitoring.js
import { getCLS, getFID, getLCP } from 'web-vitals';
function sendToAnalytics({ name, delta, id }) {
// Send metrics to your analytics service
const analyticsData = {
metric: name,
value: delta,
id: id,
page: window.location.pathname,
// Add user connection info if available
connection: navigator.connection ?
navigator.connection.effectiveType : 'unknown'
};
console.log('Analytics:', analyticsData);
// In production, send to your analytics service
// window.gtag('event', 'web_vitals', analyticsData);
}
// Track chunk loading performance
if ('performance' in window && 'getEntriesByType' in performance) {
// Create a performance observer
const observer = new PerformanceObserver((list) => {
list.getEntries().forEach((entry) => {
// Filter for JS chunk loads
if (entry.initiatorType === 'script' && entry.name.includes('chunk')) {
const chunkData = {
chunkUrl: entry.name,
loadTime: entry.duration,
size: entry.transferSize,
timestamp: entry.startTime
};
console.log('Chunk loaded:', chunkData);
// Send to analytics in production
}
});
});
// Observe resource timing entries
observer.observe({ entryTypes: ['resource'] });
}
// Initialize Core Web Vitals monitoring
export function initPerformanceMonitoring() {
getCLS(sendToAnalytics);
getFID(sendToAnalytics);
getLCP(sendToAnalytics);
}
Putting It All Together: A Comprehensive Code Splitting Strategy
To truly benefit from code splitting, you need a comprehensive strategy that addresses all the issues we’ve discussed. Here’s what an effective approach looks like:
1. Analyze Before You Split
- Use bundle analyzers to understand your current bundle composition
- Identify the largest libraries and components
- Map user flows to understand which code is needed when
2. Develop a Strategic Chunking Plan
- Start with route-based splitting as a foundation
- Identify large features that warrant their own chunks
- Extract common dependencies into shared chunks
- Document your chunking strategy for team alignment
3. Implement Sophisticated Loading Techniques
- Use predictive preloading for likely navigation paths
- Implement interaction-based loading (hover, focus)
- Create meaningful loading states that match the expected content
- Adapt loading strategies based on network conditions
4. Optimize for Caching
- Use content hashing for efficient cache invalidation
- Separate stable vendor code from frequently changing application code
- Extract the runtime into its own chunk
5. Measure and Iterate
- Implement comprehensive performance monitoring
- Track real-user metrics to gauge actual impact
- A/B test different splitting strategies
- Continuously refine your approach based on data
Conclusion
Code splitting is a powerful technique for improving web application performance, but it requires careful implementation and ongoing refinement to deliver its promised benefits. By understanding and addressing the common pitfalls we’ve explored, you can transform your code splitting strategy from a source of frustration into a significant performance enhancer.
Remember that performance optimization is always a balancing act. The goal isn’t to split your code into the smallest possible chunks, but rather to create an optimal loading strategy that delivers the best user experience across different devices, networks, and usage patterns.
By taking a holistic, data-driven