Why Your Code Smells are Actually a Form of Self-Expression
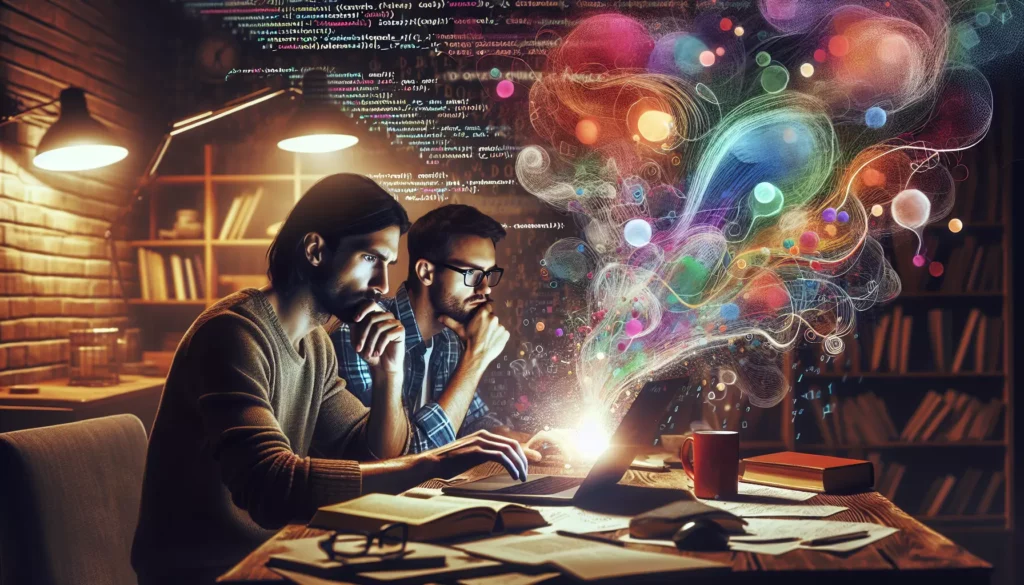
In the world of software development, we often hear about “code smells” – those telltale signs that something might be amiss in our codebase. But what if we told you that these so-called smells are not just indicators of potential issues, but also a unique form of self-expression? Today, we’re going to embark on a journey to explore this unconventional perspective, examining how our coding quirks and habits can be seen as a reflection of our personality and creativity.
Understanding Code Smells
Before we dive into the realm of self-expression, let’s refresh our understanding of code smells. In software development, code smells are surface indications that usually correspond to deeper problems in the system. They are not bugs or errors in the traditional sense, but rather indicators that the code might benefit from refactoring.
Some common code smells include:
- Duplicate Code
- Long Method
- Large Class
- Long Parameter List
- Divergent Change
- Shotgun Surgery
- Feature Envy
- Data Clumps
- Primitive Obsession
- Switch Statements
Traditionally, these smells are viewed as problems to be solved. But what if we looked at them from a different angle?
The Art of Coding
Programming, at its core, is a creative process. Just as painters use brushes and colors to create their masterpieces, programmers use languages and algorithms to build software. And just like any form of art, coding allows for personal style and expression.
Consider this: When you look at a piece of code, can you sometimes tell who wrote it without looking at the commit history? That’s because each programmer has their own unique style, their own way of approaching problems, and their own quirks that manifest in their code.
The Signature of Duplicate Code
Let’s start with one of the most common code smells: duplicate code. While it’s generally considered a bad practice, duplicate code can sometimes be a signature of a programmer who values consistency and predictability. They might prefer to see similar operations performed in a similar way throughout the codebase, even if it means repeating some code.
For example, consider this Python code:
def process_apples(apples):
for apple in apples:
clean(apple)
cut(apple)
package(apple)
def process_oranges(oranges):
for orange in oranges:
clean(orange)
cut(orange)
package(orange)
While this could be refactored to remove duplication, the programmer might argue that keeping the processes separate makes the code more readable and easier to modify if the processes need to diverge in the future.
The Poetry of Long Methods
Long methods are often frowned upon in the programming world. However, they can be seen as a form of narrative programming – telling a story through code. A programmer who writes long methods might be someone who thinks in terms of complete processes rather than small, discrete steps.
Consider this JavaScript example:
function prepareDinner() {
let ingredients = gatherIngredients();
let choppedVegetables = chopVegetables(ingredients.vegetables);
let marinatedMeat = marinateMeat(ingredients.meat);
let cookedMeat = cookMeat(marinatedMeat);
let cookedVegetables = cookVegetables(choppedVegetables);
let sauce = prepareSauce(ingredients.spices);
let meal = assembleMeal(cookedMeat, cookedVegetables, sauce);
return serveMeal(meal);
}
While this method could be broken down into smaller functions, keeping it as one long method allows the reader to follow the entire process of preparing dinner from start to finish in one place.
The Symphony of Large Classes
Large classes are often seen as a violation of the Single Responsibility Principle. However, they can also be viewed as a comprehensive approach to object-oriented programming. A programmer who writes large classes might be someone who sees objects as complex entities with many related behaviors.
Here’s a simplified example in Java:
public class SuperHero {
private String name;
private String[] powers;
private String weakness;
private String archEnemy;
public SuperHero(String name, String[] powers, String weakness, String archEnemy) {
// Constructor implementation
}
public void fight() {
// Fighting implementation
}
public void usePower(String power) {
// Power usage implementation
}
public void saveCity() {
// City saving implementation
}
public void defeatVillain(String villain) {
// Villain defeating implementation
}
// Many more methods...
}
While this class could be split into multiple smaller classes, keeping everything related to a superhero in one place might make sense to some programmers, especially if they view a superhero as a single, complex entity.
The Personal Touch in Code Smells
Now that we’ve reframed some common code smells as potential forms of expression, let’s dive deeper into how our coding habits can reflect our personalities and thought processes.
The Perfectionist’s Primitive Obsession
Primitive obsession is the use of primitive data types to represent domain ideas. While it’s often considered a code smell, it can also be a sign of a perfectionist programmer who wants full control over data representation.
Consider this C# example:
public class User {
public string FirstName { get; set; }
public string LastName { get; set; }
public int Age { get; set; }
public string Email { get; set; }
public string PhoneNumber { get; set; }
}
A perfectionist programmer might prefer this approach over using custom types for each field, as it gives them precise control over the data and its validation.
The Storyteller’s Comments
While not typically considered a code smell, excessive comments can be seen as one. However, they can also be a sign of a programmer who sees their code as a story to be told, complete with annotations and explanations.
Here’s an example in Python:
# The mystical process of brewing coffee
def brew_coffee():
# First, we summon the sacred beans
beans = select_coffee_beans()
# We then perform the ritual grinding
ground_beans = grind(beans)
# The water must be heated to the perfect temperature
water = heat_water(temperature=95) # 95°C, the ideal brewing temperature
# Now, we combine the elements in the sacred vessel
brew = mix(ground_beans, water)
# We wait patiently for the alchemical process to complete
wait(minutes=4)
# Finally, we separate the elixir from the spent grounds
return filter(brew)
While some might argue this is over-commented, for the storyteller programmer, these comments are an integral part of the code, explaining not just what the code does, but the thought process behind it.
The Architect’s Feature Envy
Feature envy occurs when a method in one class uses more features of another class than its own. While often considered problematic, it can also be a sign of a programmer who thinks in terms of services and interactions rather than encapsulated objects.
Consider this Ruby example:
class Order
def calculate_total(cart)
total = 0
cart.items.each do |item|
total += item.price * item.quantity
total -= item.discount if item.on_sale?
end
total += cart.shipping_cost
total *= (1 + cart.tax_rate)
total
end
end
While this method could be moved to the Cart class, keeping it in the Order class might make sense to a programmer who sees the Order as the central entity that coordinates between various other objects.
Embracing Your Coding Style
Now that we’ve explored how code smells can be viewed as forms of self-expression, it’s important to remember that this perspective doesn’t negate the value of clean code principles. Instead, it offers an alternative way of understanding our coding habits and those of others.
The Balance of Expression and Best Practices
While it’s fun to think of code smells as a form of self-expression, it’s crucial to strike a balance between personal style and widely accepted best practices. After all, code is not just for self-expression – it’s also a tool for solving problems and building software that others will use and maintain.
Here are some ways to embrace your coding style while still adhering to best practices:
- Understand the rules before you break them: Learn and internalize coding best practices. Only then can you make informed decisions about when and how to deviate from them.
- Document your intentions: If you choose to write code in a way that might be considered unconventional, add comments explaining your reasoning. This helps others understand your thought process.
- Be consistent: If you have a particular style or approach, apply it consistently throughout your codebase. Consistency makes code more predictable and easier to understand.
- Be open to feedback: Your unique coding style should not come at the expense of code readability or maintainability. Be open to feedback from code reviews and be willing to adapt your style when necessary.
- Refactor regularly: Even if you have reasons for writing code in a certain way, be open to refactoring it later if circumstances change or if you find a better approach.
Learning from Different Styles
One of the great things about viewing code smells as a form of self-expression is that it opens up opportunities for learning and growth. By examining and understanding different coding styles, we can expand our own coding repertoire and become more versatile programmers.
Here are some ways to learn from different coding styles:
- Read other people’s code: Contribute to open-source projects or review your colleagues’ code. Pay attention to how they structure their code and solve problems.
- Pair programming: Working closely with another programmer allows you to see their thought process in real-time and learn from their approach.
- Try different paradigms: If you’re used to object-oriented programming, try functional programming. If you typically use statically-typed languages, experiment with dynamically-typed ones.
- Attend coding meetups or conferences: These events expose you to a wide variety of coding styles and approaches from different programmers.
- Experiment with different styles: Don’t be afraid to try out different coding styles in your personal projects. This experimentation can help you find what works best for you and expand your coding toolkit.
Conclusion: Celebrating Diversity in Code
As we wrap up our exploration of code smells as a form of self-expression, it’s important to remember that diversity in coding styles, just like diversity in any field, can lead to innovation and creative problem-solving. While it’s crucial to adhere to best practices and write maintainable code, there’s also value in recognizing and appreciating the unique approaches that different programmers bring to the table.
Your code smells, quirks, and habits are not just potential issues to be fixed – they’re part of what makes you a unique programmer. They reflect your thought processes, your problem-solving approach, and your creativity. By understanding and embracing these aspects of your coding style, you can become a more self-aware and versatile programmer.
Remember, the goal is not to justify bad coding practices, but to recognize that there’s often more than one way to solve a problem in programming. By viewing code smells through the lens of self-expression, we open ourselves up to new perspectives and approaches that can ultimately make us better programmers.
So the next time you encounter a piece of code that doesn’t quite follow the textbook approach, take a moment to consider the story it might be telling. You might just learn something new about programming – and about the diverse ways we express ourselves through code.