Why Your Code Runs Perfectly Until You Show It to Someone Else
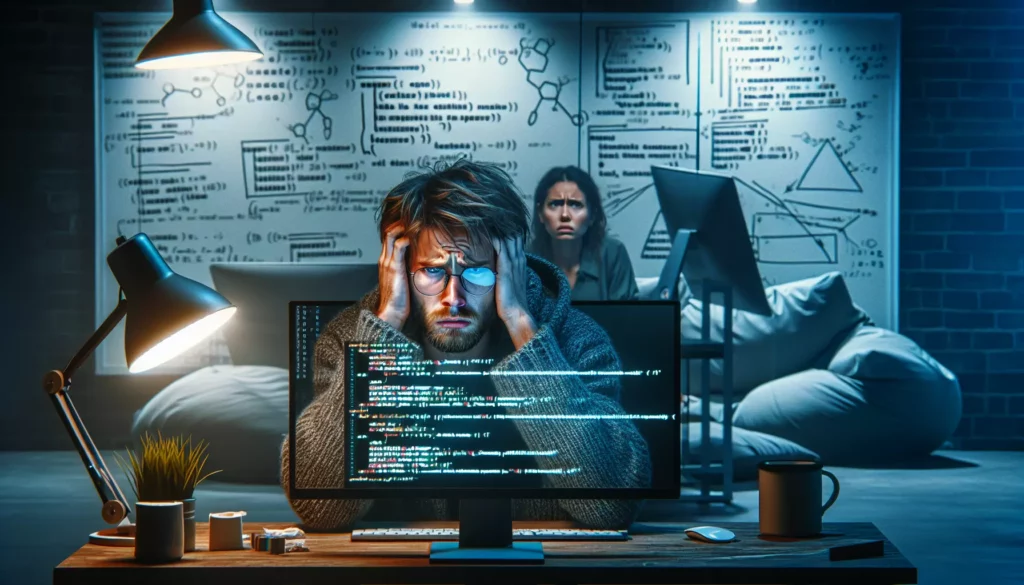
Have you ever experienced the frustration of writing code that works flawlessly on your machine, only to have it mysteriously fail when you demonstrate it to others? This phenomenon, often jokingly referred to as the “demo effect” or “Murphy’s Law of Programming,” is a common experience among developers of all skill levels. In this comprehensive guide, we’ll explore the reasons behind this perplexing occurrence and provide practical solutions to ensure your code performs consistently, regardless of the audience.
The Phenomenon Explained
Before we dive into the reasons and solutions, let’s take a moment to understand what exactly we’re dealing with. The scenario typically unfolds like this:
- You spend hours crafting and testing your code.
- Everything works perfectly on your local machine.
- You confidently prepare to showcase your work to colleagues, clients, or during a presentation.
- The moment you attempt to run your code in front of others, it inexplicably fails or behaves unexpectedly.
This situation can be embarrassing, frustrating, and even detrimental to your professional reputation. However, understanding the underlying causes can help you prevent such occurrences and become a more reliable developer.
Common Causes of the “Demo Effect”
1. Environment Differences
One of the most frequent culprits behind code behaving differently when shown to others is the variation in development environments. Your local machine’s setup may differ significantly from the environment where you’re demonstrating your code. These differences can include:
- Operating system versions
- Installed dependencies and their versions
- System configurations and settings
- Hardware specifications
For example, you might have developed your code on a Windows machine with Python 3.8, but the demonstration computer runs Linux with Python 3.6. Such discrepancies can lead to unexpected behavior or outright failures.
2. Hardcoded Paths and Dependencies
Another common issue arises from hardcoding file paths or relying on specific local configurations. When your code contains absolute paths or references to files and resources that exist only on your machine, it’s bound to fail when run on a different system.
Consider this Python example:
# Problematic code with hardcoded path
with open("C:\\Users\\YourName\\Documents\\data.txt", "r") as file:
data = file.read()
# Better approach using relative paths
import os
script_dir = os.path.dirname(os.path.abspath(__file__))
file_path = os.path.join(script_dir, "data.txt")
with open(file_path, "r") as file:
data = file.read()
3. Untracked or Uncommitted Changes
If you’re using version control systems like Git, it’s easy to forget about untracked files or uncommitted changes. These modifications might be crucial for your code to function correctly, but they won’t be present when you clone the repository on another machine or pull the latest changes.
4. Hidden Dependencies
Your development environment might have accumulated various dependencies over time that you’re not explicitly aware of. These could be system-wide installations, global npm packages, or Python modules installed in your global environment. When you run your code on a clean system, these hidden dependencies are missing, causing unexpected errors.
5. Race Conditions and Timing Issues
Sometimes, code that works perfectly on your machine might fail elsewhere due to subtle timing differences or race conditions. This is particularly common in asynchronous code, multi-threaded applications, or when dealing with network requests and database operations.
6. Cache and Persistent Data
Your local environment might have cached data or persistent storage that your code relies on without your explicit knowledge. When running the code on a fresh system, this data is absent, leading to different behavior or errors.
7. Different User Permissions
Code that runs with specific user permissions on your development machine might fail when executed under different user contexts. This is especially relevant for operations involving file system access, network connections, or system-level operations.
Strategies to Ensure Consistent Performance
Now that we’ve identified the common causes, let’s explore strategies to mitigate these issues and ensure your code performs consistently across different environments.
1. Use Virtual Environments
Virtual environments are a powerful tool for isolating project dependencies and ensuring consistency across different systems. They allow you to create a self-contained environment for each project, preventing conflicts between different projects’ requirements.
For Python projects, you can use tools like venv
or virtualenv
:
# Create a virtual environment
python -m venv myproject_env
# Activate the virtual environment
# On Windows:
myproject_env\Scripts\activate
# On Unix or MacOS:
source myproject_env/bin/activate
# Install project dependencies
pip install -r requirements.txt
For JavaScript projects, consider using nvm
(Node Version Manager) to manage Node.js versions and npm
or yarn
for package management:
# Use a specific Node.js version
nvm use 14.17.0
# Install project dependencies
npm install
2. Containerization with Docker
Docker takes environment isolation to the next level by containerizing your entire application and its dependencies. This ensures that your code runs in the same environment regardless of the host system. Here’s a basic example of a Dockerfile for a Python application:
# Use an official Python runtime as a parent image
FROM python:3.8-slim-buster
# Set the working directory in the container
WORKDIR /app
# Copy the current directory contents into the container at /app
COPY . /app
# Install any needed packages specified in requirements.txt
RUN pip install --no-cache-dir -r requirements.txt
# Make port 80 available to the world outside this container
EXPOSE 80
# Define environment variable
ENV NAME World
# Run app.py when the container launches
CMD ["python", "app.py"]
3. Use Configuration Files
Instead of hardcoding values or relying on environment-specific settings, use configuration files to manage different environments. This allows you to easily switch between development, staging, and production settings without changing your code.
For example, in a Python project, you might use a config.ini
file:
# config.ini
[DEFAULT]
DEBUG = False
DATABASE_URI = sqlite:///production.db
[DEVELOPMENT]
DEBUG = True
DATABASE_URI = sqlite:///development.db
[TESTING]
DATABASE_URI = sqlite:///test.db
Then, in your Python code:
import configparser
import os
config = configparser.ConfigParser()
config.read('config.ini')
# Use the appropriate configuration based on the environment
env = os.environ.get('ENVIRONMENT', 'DEVELOPMENT')
current_config = config[env]
debug_mode = current_config.getboolean('DEBUG')
db_uri = current_config['DATABASE_URI']
4. Implement Comprehensive Testing
Robust testing is crucial for catching environment-specific issues before they manifest during demonstrations. Implement a variety of tests, including:
- Unit tests for individual components
- Integration tests for interactions between different parts of your system
- End-to-end tests that simulate real-world usage scenarios
- Performance tests to identify potential bottlenecks
Here’s an example of a simple unit test in Python using the unittest
framework:
import unittest
from mymodule import add_numbers
class TestMathFunctions(unittest.TestCase):
def test_add_numbers(self):
self.assertEqual(add_numbers(2, 3), 5)
self.assertEqual(add_numbers(-1, 1), 0)
self.assertEqual(add_numbers(0, 0), 0)
if __name__ == '__main__':
unittest.main()
5. Use Relative Paths and Environment Variables
Instead of hardcoding absolute paths, use relative paths or environment variables to reference files and resources. This makes your code more portable across different systems.
Example using environment variables in Python:
import os
# Retrieve database connection string from environment variable
db_connection_string = os.environ.get('DATABASE_URL')
# Use a default value if the environment variable is not set
if db_connection_string is None:
db_connection_string = "sqlite:///default.db"
# Now use db_connection_string to connect to your database
6. Implement Proper Error Handling and Logging
Robust error handling and logging can help you quickly identify and diagnose issues that occur in different environments. Make sure to catch and handle exceptions appropriately, and implement a logging system that provides detailed information about the application’s state and any errors that occur.
Example of error handling and logging in Python:
import logging
# Configure logging
logging.basicConfig(level=logging.INFO, format='%(asctime)s - %(levelname)s - %(message)s')
def divide_numbers(a, b):
try:
result = a / b
logging.info(f"Successfully divided {a} by {b}. Result: {result}")
return result
except ZeroDivisionError:
logging.error(f"Attempted to divide {a} by zero")
raise ValueError("Cannot divide by zero")
except Exception as e:
logging.error(f"An unexpected error occurred: {str(e)}")
raise
# Usage
try:
divide_numbers(10, 2) # This will work
divide_numbers(10, 0) # This will raise an error
except ValueError as ve:
print(f"Caught an error: {ve}")
7. Use Version Control Effectively
Proper use of version control systems like Git can help prevent issues related to untracked files or uncommitted changes. Some best practices include:
- Regularly commit your changes and push them to a remote repository
- Use meaningful commit messages to track the evolution of your code
- Utilize branches for different features or experiments
- Include a comprehensive
.gitignore
file to avoid tracking unnecessary files
Example of a basic .gitignore
file for a Python project:
# Byte-compiled / optimized / DLL files
__pycache__/
*.py[cod]
*$py.class
# Virtual environment
venv/
env/
# IDE-specific files
.vscode/
.idea/
# Logs
*.log
# OS-specific files
.DS_Store
Thumbs.db
8. Document Dependencies and Setup Instructions
Maintain clear and up-to-date documentation of your project’s dependencies and setup instructions. This should include:
- Required software and tools
- Steps to set up the development environment
- Instructions for installing dependencies
- Configuration steps and environment variables
- Common issues and their solutions
A well-maintained README.md
file in your project repository can serve this purpose effectively.
Preparing for Demonstrations
Even with all these precautions in place, it’s essential to prepare thoroughly for code demonstrations. Here are some additional tips to ensure smooth presentations:
1. Rehearse in a Clean Environment
Before your demonstration, test your code in a fresh environment that closely mimics the conditions of your presentation setup. This could involve using a virtual machine, a separate physical machine, or a cloud-based development environment.
2. Have a Backup Plan
Always have a backup plan in case something goes wrong during the demonstration. This could include:
- Pre-recorded videos of your code running successfully
- Screenshots or step-by-step documentation of the expected output
- A prepared explanation of what the code should do, even if you can’t show it in action
3. Be Honest and Transparent
If something does go wrong during your demonstration, be honest about it. Explain what you think might be causing the issue and how you would typically debug or resolve it. This shows your problem-solving skills and adaptability, which are valuable traits in a developer.
4. Use Version Control Tags
Before important demonstrations, create a Git tag for the specific version of your code that you’ve tested and know works correctly. This allows you to easily revert to a known good state if needed:
# Create a tag for the demo version
git tag -a v1.0-demo -m "Version 1.0 for demonstration"
# Push the tag to the remote repository
git push origin v1.0-demo
# If needed, you can later checkout this specific version
git checkout v1.0-demo
Conclusion
The phenomenon of code working perfectly until you show it to someone else is a common experience in software development. By understanding the underlying causes and implementing the strategies discussed in this article, you can significantly reduce the likelihood of encountering unexpected issues during demonstrations.
Remember that consistent performance across different environments is a hallmark of well-designed, robust software. By adopting best practices such as using virtual environments, containerization, comprehensive testing, and effective version control, you’ll not only improve the reliability of your demonstrations but also enhance the overall quality and portability of your code.
Ultimately, the goal is to develop resilient, well-documented, and easily reproducible projects. This not only makes your life easier as a developer but also builds trust and credibility with your colleagues, clients, and the broader development community.
As you continue to grow in your programming journey, remember that every “failed” demonstration is an opportunity to learn and improve. Embrace these challenges, and you’ll emerge as a more skilled and confident developer, capable of creating software that performs consistently, regardless of who’s watching.