Why Your Code Reviews Aren’t Catching Important Issues
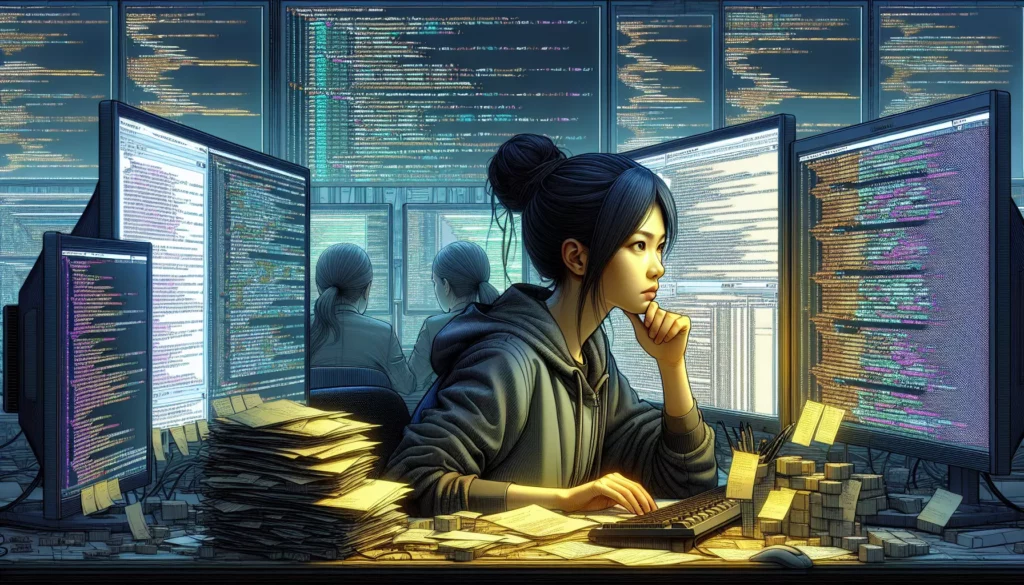
Code reviews are one of the most valuable practices in software development. When done correctly, they can significantly improve code quality, facilitate knowledge sharing, and catch bugs before they reach production. However, many teams find that despite regular code reviews, critical issues still slip through the cracks.
If your team is experiencing this problem, you’re not alone. In this article, we’ll explore why code reviews sometimes fail to catch important issues and provide actionable strategies to make your review process more effective.
Table of Contents
- Understanding the Limitations of Traditional Code Reviews
- Common Pitfalls in Code Review Processes
- Going Beyond Syntax: Reviewing for Logic and Design
- Tools and Techniques to Enhance Code Reviews
- Building a Healthy Code Review Culture
- Measuring Code Review Effectiveness
- Your Action Plan for Better Code Reviews
- Conclusion
Understanding the Limitations of Traditional Code Reviews
Before we dive into solutions, let’s understand why code reviews might not be catching all the issues they should.
The Human Factor
Code reviews are inherently human processes, which means they’re subject to human limitations:
- Attention fatigue: After reviewing code for extended periods, reviewers experience mental fatigue, making it easier to miss subtle issues.
- Cognitive bias: Reviewers might unconsciously pay more attention to familiar patterns or issues they’ve encountered before, overlooking novel problems.
- Time constraints: When under pressure to complete reviews quickly, thoroughness often suffers.
A study by SmartBear found that developers can effectively review only about 200-400 lines of code per hour. Beyond that, effectiveness dramatically decreases. Yet many organizations routinely ask developers to review much larger changesets.
Scope and Focus Issues
Many code reviews suffer from undefined or overly broad scope:
- Unclear objectives: Without specific goals, reviewers may focus on superficial issues like formatting instead of more critical concerns.
- Too much code at once: Large pull requests make comprehensive review practically impossible.
- Lack of context: Reviewers may not understand the full context of changes, especially in complex systems.
The Limitations of Static Analysis
While automated tools can catch certain types of issues, they have significant limitations:
- They can’t evaluate whether code actually solves the intended business problem
- They struggle with detecting logical errors that don’t violate syntax rules
- They can’t assess whether a solution is the most appropriate one for a given context
Common Pitfalls in Code Review Processes
Now that we understand the inherent challenges, let’s examine specific pitfalls that prevent code reviews from catching important issues.
Surface-Level Reviews
Many reviews focus exclusively on surface-level concerns:
- Style over substance: Excessive focus on formatting, variable naming, and other stylistic elements
- Missing the forest for the trees: Getting caught up in minor details while missing architectural flaws
- Ignoring non-functional requirements: Overlooking performance, security, and scalability considerations
This code snippet might pass a surface-level review despite containing a serious issue:
function getUserData(userId) {
// Looks clean and follows style guidelines
const userData = database.query(`SELECT * FROM users WHERE id = ${userId}`);
return userData;
}
The SQL injection vulnerability here could be missed if the reviewer is only checking style and syntax.
Rubber-Stamp Approvals
Sometimes code reviews become a formality rather than a genuine quality check:
- Approval without thorough review: Quickly approving code to meet deadlines or avoid confrontation
- Reciprocity bias: “You approved my code quickly, so I’ll do the same for you”
- Authority bias: Automatically approving code from senior developers without proper scrutiny
A GitHub study found that pull requests with review comments were 2.6 times more likely to be of higher quality than those without comments. This suggests that “rubber stamp” approvals often let issues slip through.
Siloed Knowledge
When knowledge is concentrated among a few team members:
- Single-reviewer dependency: Relying on one person who “knows that part of the codebase”
- Specialized knowledge gaps: Missing security, performance, or accessibility issues due to lack of expertise
- Incomplete historical context: New team members reviewing code without understanding its historical evolution
Inadequate Testing Context
Reviewing code without understanding how it’s tested:
- Test coverage blind spots: Approving code without verifying adequate test coverage
- Missing edge cases: Failing to consider how the code handles boundary conditions
- Integration assumptions: Reviewing components in isolation without considering system-wide effects
Going Beyond Syntax: Reviewing for Logic and Design
To catch more meaningful issues, code reviews need to go deeper than syntax and style.
Architectural and Design Review
Effective code reviews should evaluate architectural decisions:
- Design pattern appropriateness: Is this the right pattern for the problem?
- Component responsibilities: Does this code belong here, or is it violating separation of concerns?
- Interface design: Are the APIs intuitive, consistent, and following the principle of least surprise?
Consider this example:
class UserManager {
constructor(database) {
this.database = database;
}
async getUser(id) {
return await this.database.users.findById(id);
}
async updateEmail(id, newEmail) {
const user = await this.getUser(id);
user.email = newEmail;
await this.database.users.save(user);
// Send confirmation email
const mailer = new EmailService();
await mailer.sendConfirmation(user.email);
}
}
A syntax-focused review might miss that this class violates the Single Responsibility Principle by handling both data access and email notifications.
Business Logic Validation
Reviews should verify that code correctly implements business requirements:
- Requirement traceability: Does the code actually solve the business problem described in the ticket?
- Edge case handling: How does the code handle unexpected inputs or conditions?
- Business rule enforcement: Are all business rules correctly implemented?
Security and Performance Considerations
These critical non-functional requirements often get overlooked:
- Security vulnerabilities: Input validation, authentication, authorization, data protection
- Performance implications: Algorithmic complexity, resource usage, potential bottlenecks
- Concurrency issues: Race conditions, deadlocks, thread safety
For example, this code might functionally work but has serious performance issues:
function findDuplicates(array) {
const duplicates = [];
for (let i = 0; i < array.length; i++) {
for (let j = 0; j < array.length; j++) {
if (i !== j && array[i] === array[j] && !duplicates.includes(array[i])) {
duplicates.push(array[i]);
}
}
}
return duplicates;
}
The nested loops create O(n²) complexity, and the additional includes check makes it even worse. This could cause significant performance problems with large arrays.
Tools and Techniques to Enhance Code Reviews
Now that we understand what we're missing, let's explore tools and techniques to improve our code reviews.
Automated Code Analysis
Leverage automation to catch issues before human review:
- Static analysis tools: Tools like ESLint, SonarQube, or Checkstyle can automatically flag common issues
- Security scanning: Tools like Snyk, OWASP Dependency Check, or GitHub's CodeQL can identify security vulnerabilities
- Performance profiling: Automated benchmarks and performance tests can highlight potential bottlenecks
By automating detection of routine issues, human reviewers can focus their attention on more complex problems.
Structured Review Checklists
Checklists help ensure comprehensive reviews:
- Domain-specific checklists: Tailored to your application's specific concerns
- Role-based checklists: Different items for security reviews, performance reviews, etc.
- Customized by component: Frontend, backend, data access layers may have different concerns
A basic checklist might include:
- Does the code solve the stated problem?
- Is the solution unnecessarily complex?
- Are there adequate tests for new functionality and edge cases?
- Are there potential security vulnerabilities?
- Will this code perform well under expected load?
- Is error handling comprehensive and appropriate?
- Is the code maintainable and well-documented?
Multi-Stage Review Process
Different review stages can focus on different aspects:
- Design reviews: Conducted before implementation begins to validate approach
- Implementation reviews: Traditional code reviews focusing on the code itself
- Specialized reviews: Security, performance, or accessibility experts reviewing specific aspects
Pair Programming as Continuous Review
Pair programming offers real-time code review benefits:
- Immediate feedback: Issues caught as code is written
- Knowledge sharing: Natural transfer of domain and technical knowledge
- Reduced review overhead: Less formal review needed later
Studies show that while pair programming may initially slow development, it often results in higher quality code with fewer defects, potentially reducing overall development time when including debugging and rework.
Building a Healthy Code Review Culture
Tools and processes alone aren't enough. The culture around code reviews significantly impacts their effectiveness.
Creating Psychological Safety
Team members need to feel safe giving and receiving feedback:
- Separating code from identity: Critique the code, not the coder
- Encouraging questions: "I don't understand this approach" should be welcomed, not seen as criticism
- Leading by example: Senior team members should openly accept and act on feedback
Balancing Thoroughness with Pragmatism
Finding the right balance is crucial:
- Right-sizing reviews: Keep pull requests small and focused
- Time-boxing review sessions: Schedule dedicated review time rather than squeezing it between tasks
- Prioritizing feedback: Distinguish between "must fix" issues and "nice to have" suggestions
Knowledge Sharing Through Reviews
Reviews should be learning opportunities:
- Explaining the "why": When suggesting changes, explain the reasoning
- Referencing resources: Link to documentation, articles, or patterns that support suggestions
- Rotating reviewers: Ensure knowledge is spread throughout the team
Consider this feedback example:
Instead of:
"Use a Set here instead of an array."
Try:
"I suggest using a Set here instead of an array with includes() checks. This would improve the time complexity from O(n) to O(1) for duplicate checking, which could be significant for larger inputs. Here's a quick example of how it might look: [example code]"
The second approach not only suggests a change but explains why it matters and how to implement it, creating a learning opportunity.
Measuring Code Review Effectiveness
To improve code reviews, you need to measure their effectiveness.
Quantitative Metrics
Useful metrics to track include:
- Defect escape rate: How many bugs make it to production despite reviews?
- Review coverage: What percentage of code changes are reviewed?
- Review velocity: How long do reviews take to complete?
- Review size: How many lines of code per review?
Qualitative Assessment
Numbers don't tell the whole story:
- Peer feedback: Do team members find reviews helpful?
- Learning outcomes: Are developers improving based on review feedback?
- Issue diversity: Are reviews catching different types of issues or just the same ones?
Post-Incident Analysis
When issues do reach production:
- Root cause analysis: Could the issue have been caught in review? Why wasn't it?
- Process improvement: What changes to the review process could prevent similar issues?
- Knowledge sharing: Ensure lessons learned are communicated to the whole team
Your Action Plan for Better Code Reviews
Here's a practical step-by-step approach to improving your code review process:
Short-Term Improvements (Next Sprint)
- Establish clear guidelines: Document what makes a good review in your team
- Implement size limits: Set a maximum of 200-400 lines of code per review
- Create basic checklists: Start with a simple checklist of common issues to check
- Add automated tools: Integrate at least one static analysis tool into your CI pipeline
Medium-Term Improvements (Next Quarter)
- Implement review pairing: Assign two reviewers with complementary expertise
- Conduct review workshops: Practice reviewing code as a team to calibrate standards
- Refine metrics: Establish baseline measurements and improvement targets
- Create specialized checklists: Develop more detailed checklists for different types of code
Long-Term Improvements (Next Year)
- Implement multi-stage reviews: Separate design, implementation, and specialized reviews
- Build knowledge base: Document common issues and their solutions for team reference
- Continuous improvement: Regularly review and update your review process based on effectiveness
- Mentor review skills: Explicitly develop code review skills through mentoring and training
Sample Code Review Checklist
Here's a starter checklist you can adapt for your team:
Functionality
- Does the code solve the problem described in the ticket?
- Are all acceptance criteria met?
- Are edge cases handled appropriately?
- Is error handling comprehensive and user-friendly?
Code Quality
- Is the code DRY (Don't Repeat Yourself)?
- Does it follow SOLID principles where appropriate?
- Is the code reasonably simple or is there unnecessary complexity?
- Are functions and methods focused on a single responsibility?
Testing
- Are there appropriate unit tests for new functionality?
- Do tests cover edge cases and error conditions?
- Are tests readable and maintainable?
- Is there adequate integration testing?
Security
- Is user input properly validated and sanitized?
- Are authentication and authorization checks in place?
- Are sensitive data (passwords, tokens, PII) properly protected?
- Are there any potential injection vulnerabilities?
Performance
- Are there any potential performance bottlenecks?
- Is resource usage (memory, CPU, network, disk) appropriate?
- Are database queries optimized?
- Is the code likely to scale with expected growth?
Documentation
- Is the code sufficiently commented where needed?
- Are complex algorithms or business rules explained?
- Is API documentation complete?
- Are changes to existing behavior documented?
Conclusion
Code reviews are one of the most powerful tools for maintaining and improving code quality, but they're often not living up to their potential. By understanding the common pitfalls and implementing the strategies outlined in this article, you can transform your code review process from a superficial formality into a genuinely valuable practice.
Remember that effective code reviews go beyond catching bugs. They're opportunities for knowledge sharing, mentoring, and building a stronger engineering culture. The most successful teams view code reviews not as gatekeeping but as collaboration—a chance for the whole team to contribute to better solutions.
Start by addressing the most immediate issues in your current process. Perhaps your reviews are too large, or maybe they're focusing too much on style over substance. Even small improvements can yield significant benefits. Over time, continue refining your approach based on what works for your team and the specific challenges you face.
With thoughtful implementation of the techniques discussed here, you'll catch more important issues before they reach production, speed up knowledge sharing across your team, and ultimately deliver better software more efficiently.
What aspect of your code review process will you improve first?