Why Your Code Reviews Are Creating Team Conflicts (And How To Fix Them)
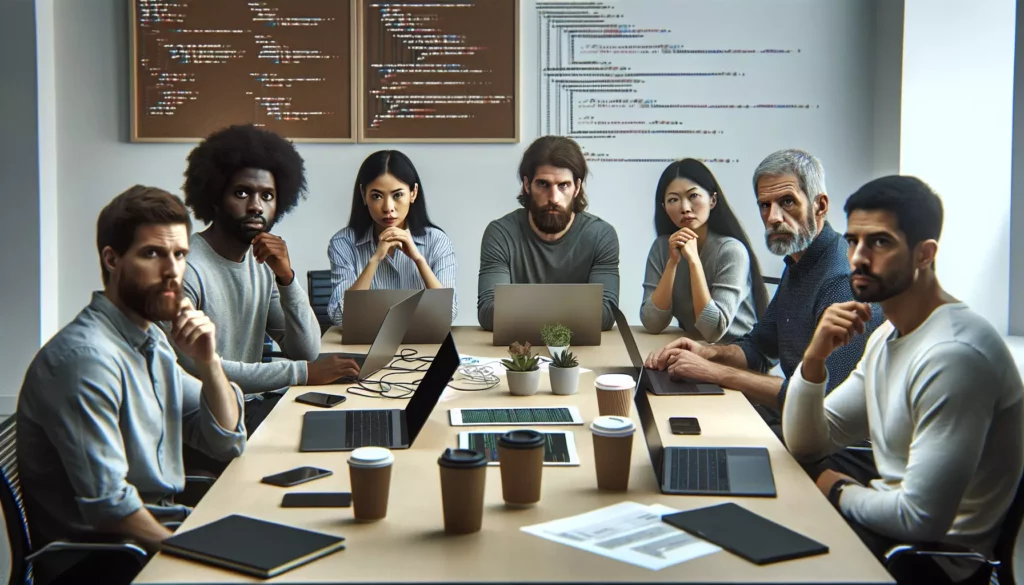
Code reviews are meant to improve code quality and foster knowledge sharing among developers. However, when implemented poorly, they can become a source of tension, frustration, and team conflict. If your team’s code review process is causing more problems than it solves, it might be time to reassess your approach.
In this comprehensive guide, we’ll explore why code reviews sometimes lead to team conflicts, identify common pitfalls in the review process, and provide actionable strategies to transform your code reviews into collaborative learning opportunities rather than battlegrounds for ego and criticism.
Table of Contents
- The Importance of Effective Code Reviews
- Signs Your Code Review Process Is Creating Conflict
- Common Causes of Conflict in Code Reviews
- Communication Problems in Code Reviews
- Power Dynamics and Their Impact
- Cultural Issues in Code Reviews
- Process Problems That Lead to Conflict
- Better Practices for Conflict Free Code Reviews
- Tools and Techniques to Improve Code Reviews
- Measuring Success in Your Code Review Process
- Conclusion: Building a Positive Code Review Culture
The Importance of Effective Code Reviews
Before diving into what’s going wrong, let’s remind ourselves why code reviews are worth getting right. When implemented effectively, code reviews offer numerous benefits:
- Quality assurance: They help catch bugs, security vulnerabilities, and performance issues early.
- Knowledge sharing: They spread understanding of the codebase across the team.
- Mentorship: Senior developers can guide juniors through best practices.
- Consistency: They ensure code adheres to team standards and conventions.
- Collective ownership: They foster a sense that the code belongs to the team, not individuals.
A study by SmartBear found that code reviews can identify up to 80% of defects in software. Microsoft research showed that well implemented code review processes can reduce development costs by 15-20% over the life of a project.
However, these benefits only materialize when code reviews are conducted in a constructive, respectful manner. When they become adversarial or overly critical, they can have the opposite effect.
Signs Your Code Review Process Is Creating Conflict
How do you know if your code review process is causing problems? Watch for these warning signs:
Emotional Responses to Reviews
If developers regularly become defensive, frustrated, or discouraged after code reviews, something’s wrong. You might notice:
- Developers taking feedback personally rather than professionally
- Emotional reactions during or after review sessions
- Reluctance to submit code for review
- Developers appearing deflated after reviews
Delays and Bottlenecks
Conflict often manifests as process slowdowns:
- Pull requests sitting unreviewed for days
- Developers avoiding reviewing certain team members’ code
- Long, drawn out back and forth discussions on pull requests
- Repeated rejection of code without clear path to resolution
Interpersonal Tension
Look for signs of deteriorating team dynamics:
- Formation of cliques or factions within the team
- Decreased collaboration outside of mandatory reviews
- Complaints about specific reviewers or submitters
- Passive aggressive comments in review threads
Declining Code Quality Despite Reviews
Paradoxically, when code reviews create conflict, code quality often suffers:
- Developers making minimal changes just to pass review
- Increasing technical debt despite review processes
- Same issues appearing repeatedly across the codebase
- Reviews becoming superficial or rubber stamp exercises
If you recognize several of these signs in your team, it’s likely that your code review process is contributing to conflict rather than collaboration.
Common Causes of Conflict in Code Reviews
Understanding the root causes of conflict in code reviews is the first step toward improvement. Here are the most common issues:
Overly Critical Feedback
Reviews that focus exclusively on what’s wrong, without acknowledging what’s right, can feel like personal attacks rather than constructive feedback. This is especially problematic when:
- Feedback is delivered in harsh or judgmental language
- Every minor issue is flagged, creating a sea of negative comments
- Positive aspects of the code are never mentioned
- The tone suggests incompetence rather than opportunity for improvement
Subjective Opinions Presented as Objective Facts
Code style and approach often have multiple valid solutions. Problems arise when reviewers present their preferences as the only correct way:
- “This is bad code” instead of “I find this approach difficult to understand”
- Insisting on changes without explaining the reasoning
- Rejecting code based on stylistic preferences not documented in team standards
- Demanding rewrites that don’t materially improve the code
Inconsistent Standards
Developers become frustrated when they perceive that different standards apply to different team members:
- Junior developers held to higher standards than seniors
- Some developers allowed to bypass review processes
- Shifting expectations from one review to the next
- Different reviewers enforcing contradictory standards
Lack of Context
Reviews conducted without understanding the constraints the developer was working under can lead to inappropriate feedback:
- Suggesting time consuming improvements when there’s a tight deadline
- Not considering legacy code constraints
- Ignoring business requirements that shaped implementation decisions
- Reviewing code in isolation without understanding the larger system
Communication Problems in Code Reviews
Even when the intent behind feedback is good, poor communication can create unnecessary conflict. Here are common communication issues:
Tone Problems in Written Feedback
Text based communication lacks the nuance of face to face interaction, making it easy for messages to be misinterpreted:
- Short, terse comments that seem dismissive
- Excessive use of imperatives (“change this,” “fix that”) without explanation
- Sarcasm or humor that falls flat in written form
- All caps or excessive punctuation that comes across as shouting
For example, a simple comment like “This won’t work” can be interpreted as “You don’t know what you’re doing” even if the reviewer just meant to highlight a specific edge case.
Lack of Clarity
Vague or ambiguous feedback creates frustration and wastes time:
- Comments like “This could be better” without explaining how
- Pointing out problems without suggesting solutions
- Using jargon or acronyms not familiar to all team members
- Providing contradictory feedback within the same review
Missing the “Why” Behind Feedback
Feedback that doesn’t explain reasoning prevents learning and often feels arbitrary:
- Requesting changes without explaining the benefit
- Not connecting feedback to broader principles or patterns
- Failing to distinguish between critical issues and minor suggestions
- Not providing context or references for best practices being cited
Example of Poor vs. Effective Communication
Poor Communication:
This function is a mess. Rewrite it using a more efficient approach.
Effective Communication:
I notice this function is using nested loops which gives it O(n²) complexity. For our dataset sizes, this might cause performance issues. Consider using a hash map approach which could reduce this to O(n) complexity. Here's an example of how that might look: [example or link]. What do you think?
The second approach explains the reasoning, suggests a specific alternative, provides resources, and invites dialogue rather than commanding a change.
Power Dynamics and Their Impact
Code reviews don’t happen in a vacuum. The organizational hierarchy and team dynamics significantly influence how reviews are conducted and received.
The Senior Junior Divide
When senior developers review junior developers’ code, several issues can arise:
- Juniors may feel intimidated and reluctant to defend their choices
- Seniors might forget what it’s like to be learning and set unrealistic expectations
- Knowledge gaps may be treated as character flaws rather than learning opportunities
- Juniors might perceive any criticism as a threat to their job security
Peer Competition
Developers at the same level sometimes use code reviews as a way to establish dominance:
- Nitpicking to demonstrate superior knowledge
- Protecting “territory” by scrutinizing changes to “their” code more harshly
- Using reviews to showcase their own expertise to management
- Competitive dynamics where finding issues is seen as “winning”
Management Involvement
When managers are directly involved in code reviews, additional pressures emerge:
- Developers feeling that every review is a performance evaluation
- Confusion between technical feedback and managerial direction
- Hesitation to question or discuss feedback from someone who controls promotions
- Reviews becoming about pleasing the manager rather than improving the code
Addressing Power Imbalances
To mitigate these issues:
- Rotate reviewers to avoid consistent power dynamics
- Encourage two way reviews where juniors also review seniors’ code
- Create explicit spaces for questions and learning in the review process
- Separate performance evaluation from the regular code review process
- Establish that everyone’s code, regardless of seniority, is subject to the same standards
Cultural Issues in Code Reviews
Team culture and broader organizational culture significantly impact how code reviews function. Cultural misalignments can be a major source of conflict.
Blame Culture vs. Learning Culture
In a blame culture:
- Bugs or issues are treated as personal failures
- Reviews focus on finding someone at fault
- Developers hide problems rather than addressing them openly
- Reviews become exercises in deflection and defensiveness
In a learning culture:
- Issues are treated as opportunities for team improvement
- Focus is on systems and processes, not individual blame
- Mistakes are openly discussed without shame
- Reviews are collaborative problem solving sessions
Perfectionism vs. Pragmatism
Cultural expectations around code quality can create tension:
- Some teams value theoretical perfection over shipping working code
- Others prioritize speed to market over maintainability
- Conflicts arise when team members have mismatched expectations
- Without explicit values, subjective judgments fill the void
Individualism vs. Collectivism
Different views of code ownership affect reviews:
- Individualistic cultures: “This is my code, and you’re criticizing me”
- Collective cultures: “This is our codebase, and we’re improving it together”
- Personal attachment to code makes feedback harder to receive
- Collective ownership facilitates more objective discussions
Building a Healthier Code Review Culture
To address cultural issues:
- Explicitly define and document team values around code quality and collaboration
- Lead by example, with senior team members demonstrating how to gracefully receive feedback
- Celebrate improvements and learning, not just catching issues
- Frame code reviews as a team activity improving a shared asset, not personal evaluation
- Recognize and discuss cultural differences openly, especially in diverse or distributed teams
Process Problems That Lead to Conflict
Even with good intentions and communication, poorly designed review processes can generate unnecessary friction.
Timing Issues
When in the development cycle reviews occur matters:
- Reviews of completed work are more likely to create defensive reactions
- Last minute reviews create pressure and deadline stress
- Long delays between submission and review break developer flow
- Unpredictable review schedules make planning difficult
Scope Problems
The size and focus of what’s being reviewed impacts effectiveness:
- Enormous pull requests overwhelm reviewers and lead to superficial reviews
- Tiny, fragmented reviews make it hard to see the big picture
- Unclear boundaries about what aspects should be reviewed create confusion
- Reviews that try to address too many concerns at once become unfocused
Lack of Clear Standards
Without established guidelines, reviews become subjective:
- Absence of documented coding standards leads to opinion based feedback
- No clear definition of what constitutes a “blocker” vs. a “suggestion”
- Inconsistent expectations about test coverage or documentation
- Ambiguity about which architectural patterns are preferred
Process Improvements
To address these issues:
- Implement early feedback mechanisms like pair programming or design reviews
- Set clear expectations for review turnaround time (e.g., within 24 hours)
- Limit pull request size to facilitate thorough reviews
- Create and document team standards for code quality, style, and architecture
- Distinguish between required changes and optional suggestions
- Use automated tools to handle style and formatting issues
For example, Google’s engineering practices suggest that code reviews should ideally be completed within one business day, and that changes should be small enough to be understood in about 30 minutes.
Better Practices for Conflict Free Code Reviews
Now that we’ve identified common problems, let’s explore specific practices that can transform your code review process from a source of conflict to a collaborative learning opportunity.
For Code Authors: Submitting Review Ready Code
As an author, you can set the stage for a positive review experience:
- Self review first: Review your own code before submitting it to catch obvious issues.
- Provide context: Include a clear description of what the code does, why it’s needed, and any constraints you were working under.
- Highlight areas of concern: If you’re uncertain about an approach, proactively ask for feedback on those specific parts.
- Keep changes focused: Submit smaller, logically cohesive changes rather than massive overhauls.
- Run automated checks: Ensure your code passes linting, formatting, and tests before asking humans to review it.
- Be responsive: Engage with reviewers promptly and constructively.
Example of a good pull request description:
## What
This PR implements the user authentication flow using OAuth2.
## Why
We need to support single sign-on for enterprise customers as specified in ticket AUTH-123.
## How
- Added OAuth2 client configuration
- Implemented token exchange and validation
- Added user profile retrieval from OAuth provider
- Created session management for authenticated users
## Testing
- Unit tests cover the token validation logic
- Integration tests for the full authentication flow
- Manually tested with Google and GitHub OAuth providers
## Notes
I'm particularly interested in feedback on the token refresh mechanism. I considered two approaches (detailed in comments).
For Reviewers: Providing Constructive Feedback
As a reviewer, your approach significantly impacts how feedback is received:
- Start with positives: Begin by acknowledging what’s good about the code.
- Ask questions rather than making demands: “Have you considered X?” instead of “You should do X.”
- Explain the reasoning: Connect feedback to specific principles or consequences.
- Prioritize issues: Distinguish between critical problems and minor suggestions.
- Offer solutions: When pointing out problems, suggest potential fixes.
- Be specific: Vague feedback is frustrating and unhelpful.
- Focus on the code, not the coder: “This function is complex” instead of “You wrote this function in a complex way.”
- Use a friendly tone: Remember that text can come across more harshly than intended.
Example of constructive feedback:
I really like how you've structured the authentication flow, especially the separation of concerns between token validation and user profile retrieval.
One thing I noticed is that the token refresh mechanism might encounter race conditions if multiple requests try to refresh simultaneously. Have you considered using a mutex or semaphore pattern here? Something like what's described in [link to pattern] might help prevent duplicate refresh calls.
The error handling in the OAuth client is comprehensive! One minor suggestion: consider consolidating the error types to make them more consistent with our other authentication modules.
For Teams: Establishing Healthy Review Practices
At the team level, establish processes that encourage collaboration:
- Create clear guidelines: Document what makes good code in your team’s context.
- Use a code review checklist: Ensure consistent, thorough reviews.
- Implement the “rule of three”: If a discussion goes back and forth more than twice in comments, switch to a face to face or video conversation.
- Rotate reviewers: Avoid always having the same people review each other’s code.
- Consider pair programming: For complex changes, pair programming can prevent many review issues.
- Set reasonable expectations: Define SLAs for review turnaround time.
- Recognize good reviews: Acknowledge and praise thoughtful, helpful reviews.
For Leaders: Setting the Tone
Team leaders and managers play a crucial role in shaping review culture:
- Model good behavior: Submit your own code for review and accept feedback gracefully.
- Create psychological safety: Ensure team members feel safe admitting mistakes and asking questions.
- Mediate conflicts: Step in constructively when review discussions become unproductive.
- Provide training: Offer guidance on both technical aspects and communication skills for reviews.
- Balance quality and velocity: Help the team find the right trade offs between perfectionism and pragmatism.
- Recognize that reviews take time: Allocate sufficient capacity for thorough reviews in sprint planning.
Tools and Techniques to Improve Code Reviews
The right tools and techniques can significantly reduce friction in the code review process.
Leveraging Automation
Automate what can be automated to focus human review on what matters:
- Linters and formatters: Tools like ESLint, Prettier, Black, or RuboCop can enforce style conventions automatically.
- Static analysis tools: SonarQube, CodeClimate, or language specific analyzers can identify potential bugs and code smells.
- Automated testing: Comprehensive test suites give reviewers confidence that the code works as expected.
- CI/CD integration: Automatically run checks and tests when code is submitted for review.
- Code coverage tools: Visualize which parts of the code are covered by tests.
Example GitHub Actions workflow for automated checks:
name: Code Quality Checks
on:
pull_request:
branches: [ main, develop ]
jobs:
lint:
runs-on: ubuntu-latest
steps:
- uses: actions/checkout@v2
- name: Set up Node.js
uses: actions/setup-node@v1
with:
node-version: '14'
- name: Install dependencies
run: npm ci
- name: Run linting
run: npm run lint
test:
runs-on: ubuntu-latest
steps:
- uses: actions/checkout@v2
- name: Set up Node.js
uses: actions/setup-node@v1
with:
node-version: '14'
- name: Install dependencies
run: npm ci
- name: Run tests
run: npm test
Code Review Tools and Features
Modern code review platforms offer features to improve the review experience:
- Inline comments: Precisely link feedback to specific lines of code.
- Review templates: Standardize review format and ensure comprehensive coverage.
- Draft pull requests: Get early feedback without triggering formal review processes.
- Suggested changes: Propose specific code modifications that can be directly applied.
- Review assignments: Clearly designate who is responsible for reviewing what.
- Status indicators: Show at a glance which reviews are pending, approved, or need changes.
Structured Review Techniques
Beyond tools, specific review methodologies can improve effectiveness:
- Two phase reviews: First review for high level design, second for implementation details.
- Checklist based reviews: Use standardized checklists to ensure consistent coverage.
- Time boxed reviews: Limit review sessions to maintain focus and prevent fatigue.
- Group reviews: For critical components, conduct team reviews where multiple perspectives are shared.
- Progressive reviews: Review code incrementally as it’s developed rather than all at once.
Example code review checklist:
## Functionality
- [ ] Code works as described in the requirements
- [ ] Edge cases are handled appropriately
- [ ] Error states are handled gracefully
## Security
- [ ] Input is validated and sanitized
- [ ] Authentication and authorization checks are in place
- [ ] Sensitive data is handled securely
## Performance
- [ ] Code performs efficiently with expected data volumes
- [ ] No unnecessary database queries or API calls
- [ ] Appropriate caching is implemented where beneficial
## Maintainability
- [ ] Code is well structured and follows project patterns
- [ ] Naming is clear and consistent
- [ ] Comments explain "why" not just "what"
## Testing
- [ ] Unit tests cover critical functionality
- [ ] Edge cases are tested
- [ ] Tests are clear and maintainable themselves
Measuring Success in Your Code Review Process
How do you know if your code review process is improving? Track these metrics and indicators:
Quantitative Metrics
Numbers can tell part of the story:
- Review turnaround time: How long does it take for code to get reviewed?
- Defect escape rate: How many bugs make it to production despite reviews?
- Review participation: What percentage of team members actively participate in reviews?
- Review size: Are pull requests staying within manageable sizes?
- Review frequency: Are reviews happening regularly or in bursts?
- Automation effectiveness: What percentage of issues are caught by automated tools vs. human reviewers?
Qualitative Indicators
The human side is equally important:
- Team satisfaction: Do team members find the review process valuable?
- Learning outcomes: Are developers gaining knowledge through reviews?
- Collaboration quality: Is the tone of review discussions constructive and positive?
- Psychological safety: Do team members feel comfortable having their code reviewed?
- Knowledge distribution: Is understanding of the codebase spreading across the team?
Gathering Feedback
Regularly assess and adjust your process:
- Conduct anonymous surveys about the review experience
- Include code review process in retrospectives
- Hold periodic review process reviews
- Watch for patterns in review comments and discussions
- Check in with team members individually about their review experiences
Continuous Improvement
Use feedback to refine your approach:
- Experiment with process changes based on team input
- Address recurring issues with targeted improvements
- Share successful review patterns across teams
- Periodically revisit and update review guidelines
- Celebrate improvements in review culture and outcomes
Conclusion: Building a Positive Code Review Culture
Code reviews don’t have to be battlegrounds. By addressing the common causes of conflict and implementing thoughtful processes, you can transform your team’s review culture from confrontational to collaborative.
Remember these key principles:
- Focus on the code, not the coder: Keep feedback objective and non personal.
- Communicate with empathy: Consider how your feedback will be received.
- Balance thoroughness with pragmatism: Perfect is the enemy of good.
- Leverage automation: Let tools handle the objective aspects so humans can focus on design and logic.
- Establish clear expectations: Document standards and processes to reduce subjectivity.
- Prioritize learning over finding fault: Frame reviews as collaborative learning opportunities.
- Continuously improve: Regularly assess and refine your review process.
Effective code reviews are an investment in your team’s future. They build a stronger codebase, develop more skilled engineers, and foster a culture of collaboration and continuous improvement. By addressing the conflicts that arise in your review process, you’re not just making reviews more pleasant—you’re building a foundation for long term team success.
Remember that changing a team’s review culture takes time and consistent effort. Start with small improvements, celebrate progress, and maintain focus on the ultimate goal: a team that learns and grows together through constructive collaboration.
What step will you take today to improve your team’s code review process?