Why Your Code Review Comments Sound Harsh But Are Actually Helpful
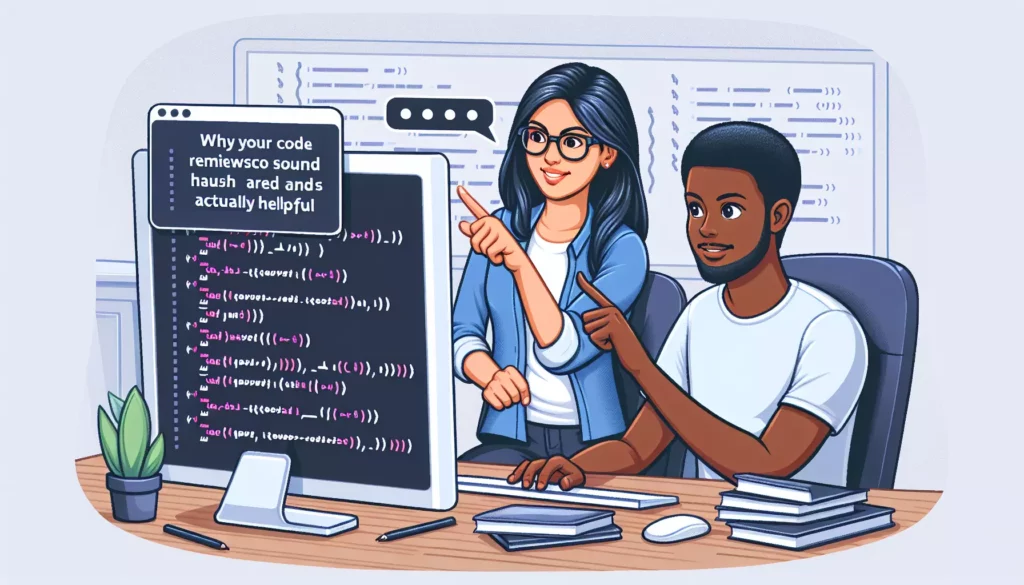
We’ve all been there. You submit your code for review, feeling proud of your solution, only to receive feedback that feels like a punch to the gut. Comments like “This code is inefficient,” “Your variable names are confusing,” or “This approach won’t scale” can sting, especially when you’ve poured hours into your work.
But here’s the truth: what feels harsh in the moment is often the feedback that helps you grow the most as a developer. Those seemingly brutal code review comments are actually gifts in disguise.
In this article, we’ll explore why code review comments might sound harsh, why that’s actually okay, and how to make the most of feedback that initially feels like criticism. Whether you’re a beginner programmer or an experienced developer, understanding the value behind direct feedback can transform your coding journey.
The Psychology Behind Receiving Code Review Feedback
Before diving into code reviews specifically, it’s worth understanding why feedback can feel so personal. When we write code, we’re not just producing functional text—we’re expressing our problem-solving abilities, our understanding of the domain, and even our personal style.
Why Code Feedback Feels Personal
Code is a creative product of our minds. We make countless decisions while coding: how to structure data, which algorithms to use, how to name variables, how to organize functions. Each decision reflects our current understanding and approach to the problem.
When someone critiques these decisions, it can feel like they’re critiquing our:
- Intelligence (“You didn’t think of this edge case?”)
- Knowledge (“This approach is inefficient”)
- Effort (“This looks rushed”)
- Care for quality (“Did you even test this?”)
This response is natural. Research in cognitive psychology shows that we tend to identify with our creative outputs. This phenomenon, sometimes called the IKEA effect, suggests we value things more when we’ve invested our own labor into creating them.
The Gap Between Intent and Perception
What complicates matters further is that written feedback lacks many contextual cues that soften criticism in face-to-face conversations. There’s no reassuring smile, no tone of voice indicating support, no immediate opportunity to ask clarifying questions.
A comment that the reviewer meant as a quick suggestion (“Consider using a hash map here for O(1) lookup”) might read to the recipient as a harsh judgment (“You should have known to use a hash map; this solution is amateur”).
This disconnect between intended meaning and perceived meaning explains why code reviews can feel more critical than they’re meant to be.
Common “Harsh” Comments and What They Actually Mean
Let’s examine some code review comments that often feel harsh but contain valuable insights:
“This code is inefficient”
How it feels: “You wasted resources and don’t understand performance considerations.”
What it likely means: “There’s a more optimal approach that would benefit the codebase.”
When a reviewer comments on efficiency, they’re highlighting an opportunity for improvement. They’re not suggesting you’re incompetent—they’re sharing knowledge about potential bottlenecks or scaling issues that might not be apparent now but could become problems later.
For example, consider this Python code for finding duplicates:
def find_duplicates(numbers):
duplicates = []
for i in range(len(numbers)):
for j in range(i+1, len(numbers)):
if numbers[i] == numbers[j] and numbers[i] not in duplicates:
duplicates.append(numbers[i])
return duplicates
A reviewer might comment: “This nested loop approach is inefficient. It’s O(n²) time complexity.”
Instead of taking this personally, recognize it as an opportunity to learn about a more efficient approach:
def find_duplicates(numbers):
seen = set()
duplicates = set()
for num in numbers:
if num in seen:
duplicates.add(num)
else:
seen.add(num)
return list(duplicates)
“Your variable names are confusing”
How it feels: “You can’t even name things properly. Basic stuff!”
What it likely means: “Clear naming would make this code more maintainable for everyone, including your future self.”
Naming is one of the hardest problems in computer science, as the saying goes. When a reviewer critiques your variable names, they’re advocating for code readability and maintainability—not attacking your creativity.
Consider this JavaScript snippet:
function calc(a, b, c, t) {
let res = 0;
if (t == 1) {
res = a + b + c;
} else if (t == 2) {
res = a * b * c;
}
return res;
}
A reviewer might comment: “These variable names are confusing. What do a, b, c, and t represent?”
A clearer version might be:
function calculate(firstNumber, secondNumber, thirdNumber, operationType) {
let result = 0;
if (operationType === 1) {
result = firstNumber + secondNumber + thirdNumber;
} else if (operationType === 2) {
result = firstNumber * secondNumber * thirdNumber;
}
return result;
}
“This won’t scale”
How it feels: “You didn’t think ahead. Your solution is short-sighted.”
What it likely means: “As our system grows, we might encounter issues with this approach. Let’s consider alternatives.”
Scalability concerns aren’t criticisms of your current solution but forward-thinking considerations. Experienced developers have often seen small design decisions create significant technical debt as systems grow.
“Did you test this?”
How it feels: “You’re careless and didn’t do your due diligence.”
What it likely means: “I noticed some edge cases that might cause issues. Let’s make sure we’ve covered all scenarios.”
This question usually comes up when a reviewer spots potential bugs or edge cases. Rather than an accusation of negligence, it’s an invitation to discuss test coverage and edge case handling.
Why Direct Feedback Matters in Programming
Now that we’ve reframed how to interpret seemingly harsh comments, let’s explore why direct, unvarnished feedback is particularly valuable in programming contexts.
Code Is Logical, Not Emotional
Unlike other creative fields where subjective aesthetic preferences might dominate feedback, code has objective qualities that can be evaluated. Does it work correctly? Is it efficient? Is it maintainable? Is it secure?
These aren’t matters of opinion—they’re measurable aspects of code quality. Direct feedback addresses these qualities without unnecessary sugar-coating.
The Cost of Poor Code Is High
In programming, the stakes are often high. Inefficient algorithms can waste computing resources and money. Security vulnerabilities can expose sensitive data. Unmaintainable code can slow down development for months or years.
Given these potential costs, direct feedback isn’t just helpful—it’s necessary. Would you prefer a reviewer to soften their language about a security vulnerability at the expense of clarity about the risk?
Learning Happens at the Edge of Comfort
Educational psychology tells us that learning happens most effectively when we’re challenged—when we’re pushed slightly beyond our comfort zone into what’s called the “zone of proximal development.”
Direct feedback that points out gaps in our knowledge or approach creates precisely this kind of productive discomfort. It highlights the space between what we know and what we could know, inviting growth.
Industry Standards Are High
Top tech companies maintain high standards for code quality, and for good reason. Systems at scale require thoughtful design, efficient implementation, and meticulous attention to detail.
Direct feedback in code reviews helps maintain these standards and prepares developers to work in environments where code quality matters intensely. This is particularly relevant for those aiming to work at major tech companies (often called FAANG).
How to Extract Maximum Value from “Harsh” Feedback
Now that we understand why direct feedback is valuable, let’s explore strategies for getting the most benefit from it:
Separate the Technical from the Personal
When you receive feedback that feels harsh, take a moment to separate the technical content from any perceived personal criticism. Ask yourself: “What is the technical insight here? What can I learn from this?”
For example, if someone comments, “This function is way too long and does too many things,” focus on the principle being highlighted (single responsibility) rather than any implied criticism of your coding style.
Ask Clarifying Questions
If feedback is unclear or seems unnecessarily critical, ask clarifying questions. This not only helps you understand the feedback better but also demonstrates your commitment to improvement.
Instead of defensively responding “What’s wrong with my approach?”, try “Could you explain more about why a different approach would be better? I’d like to understand the tradeoffs.”
Look for Patterns Across Reviews
One harsh comment might be an outlier; consistent feedback across multiple reviews likely highlights a genuine area for improvement. Look for patterns in the feedback you receive.
If multiple reviewers comment on your function length, variable naming, or test coverage, that’s valuable data about where to focus your development efforts.
Treat Feedback as a Learning Resource
Code reviews are essentially personalized learning materials. Each comment points to a concept, best practice, or technique you can research further.
When a reviewer suggests a different approach, don’t just make the change—understand why it’s better. Research the pattern or principle they’re referencing. This turns a momentary sting into a lasting lesson.
Remember: Everyone’s Code Gets Critiqued
Even the most senior developers receive critical feedback on their code. In fact, often the more experienced the developer, the more rigorous the review their code receives, because expectations are higher.
Understanding that critique is universal in programming can help depersonalize the experience and focus on the technical insights being offered.
How to Give Effective Code Review Feedback
If you’re on the giving end of code reviews, you can help ensure your feedback is received as helpful rather than harsh:
Be Specific and Actionable
Vague criticisms like “This code is messy” are more likely to feel personal than specific, actionable feedback like “Breaking this function into smaller, focused functions would improve readability.”
Compare these two comments:
Harsh and unhelpful: “This is inefficient.”
Direct but constructive: “Using a hash map here instead of nested loops would reduce the time complexity from O(n²) to O(n).”
Explain the “Why”
When suggesting changes, explain the reasoning behind your feedback. This helps the recipient understand the principle rather than just the specific instance.
For example: “I suggest using dependency injection here because it will make this code more testable and flexible when requirements change.”
Acknowledge the Positive
Pointing out what works well serves multiple purposes: it reinforces good practices, balances critical feedback, and shows you’re engaging thoughtfully with the code rather than just looking for flaws.
Try: “I like how you’ve structured the error handling here. For the validation logic, I suggest…”
Use Questions to Promote Thinking
Phrasing feedback as questions can invite the developer to think through alternatives rather than simply dictating changes.
“Have you considered using a factory pattern here?” feels more collaborative than “You should use a factory pattern here.”
Remember the Human
While code is logical, developers are human. Consider how your feedback might be received, especially if you’re reviewing code from someone more junior or new to the team.
This doesn’t mean avoiding direct feedback—it means delivering it with awareness and respect.
Case Study: Transforming “Harsh” Feedback into Growth
To illustrate how seemingly harsh feedback can lead to significant growth, let’s look at a realistic case study:
The Scenario
A junior developer, Alex, submits a pull request implementing a new feature that searches through user records. The code works but receives the following feedback from a senior developer:
- “This query will be extremely slow on large datasets. It’s loading all records into memory first.”
- “Variable names like ‘data’ and ‘result’ are too generic and make the code hard to follow.”
- “There’s no error handling here. What happens if the database connection fails?”
- “This approach won’t scale as our user base grows.”
Initial Reaction
Alex feels deflated. The feature works in testing, and they spent days on it. The feedback feels like an attack on their abilities.
The Transformation
Instead of taking the feedback personally, Alex decides to use it as a learning opportunity:
- Understanding efficiency: Alex researches database query optimization and learns about pagination, indexing, and query planning.
- Improving naming: Alex studies naming conventions and replaces generic names with specific ones that reflect the data’s purpose.
- Learning error handling: Alex implements proper error handling and learns about resilient system design.
- Thinking about scale: Alex discusses scalability with the senior developer and learns about design patterns that accommodate growth.
The Outcome
Six months later, Alex is giving similar feedback to new team members. The initially “harsh” review sparked a learning journey that significantly accelerated Alex’s development as a programmer.
Moreover, the revised feature has handled a 10x increase in user volume without issues—something the original implementation couldn’t have managed.
Common Patterns in Technical Feedback
As you receive more code reviews, you’ll notice that certain types of feedback appear repeatedly across the industry. Understanding these common patterns can help you anticipate and learn from them:
Performance Considerations
Feedback about performance typically focuses on:
- Time complexity (Big O notation)
- Memory usage
- Database query efficiency
- Network requests optimization
Example feedback: “This O(n²) sorting algorithm won’t perform well on large datasets. Consider using a built-in sort function that implements O(n log n) algorithms.”
Code Organization and Design
This category includes feedback about:
- Function and class responsibilities
- Code modularity
- Design patterns
- Separation of concerns
Example feedback: “This class is handling data access, business logic, and presentation. Consider splitting it according to the Single Responsibility Principle.”
Readability and Maintainability
Feedback about making code understandable focuses on:
- Naming conventions
- Comment quality
- Code formatting
- Function length
Example feedback: “This function is 200 lines long, making it difficult to understand. Breaking it into smaller, focused functions would improve readability.”
Error Handling and Edge Cases
This feedback addresses robustness:
- Input validation
- Exception handling
- Edge case consideration
- Graceful degradation
Example feedback: “What happens if the API returns an error? This code assumes the happy path but doesn’t handle failure scenarios.”
Testing
Test-related feedback includes:
- Test coverage
- Test quality
- Testability of the code
- Edge case testing
Example feedback: “This code is difficult to test because it has hidden dependencies. Injecting dependencies would make it more testable.”
Feedback in Different Programming Contexts
The nature of “harsh” feedback varies somewhat across different programming contexts:
Academic Settings
In computer science education, feedback often focuses on algorithmic correctness, theoretical understanding, and following assignment specifications. Comments might address inefficient algorithms or misunderstandings of core concepts.
While academic feedback can feel harsh (“Your algorithm is incorrect”), it’s designed to ensure you grasp fundamental principles that will serve as the foundation for your programming career.
Open Source Projects
Open source maintainers review countless contributions and often provide direct, concise feedback due to time constraints. Comments might seem terse (“This breaks existing functionality”) but reflect the need to maintain quality in community-built software.
The directness in open source reviews isn’t personal—it’s a product of the volunteer nature of the work and the need to maintain quality standards across diverse contributions.
Professional Settings
In professional environments, feedback often addresses business concerns alongside technical ones. Comments might focus on scalability, maintainability, or alignment with company standards.
Professional feedback that feels harsh (“This approach won’t scale to our user base”) often reflects real business constraints and experiences with similar challenges.
Technical Interviews
During coding interviews, especially at top tech companies, interviewers may provide direct feedback on your approach. This might feel particularly intense due to the high-stakes nature of interviews.
Interview feedback like “That’s not the optimal solution” is testing not just your technical skills but also how you respond to feedback—a crucial skill for any developer.
The Long-term Benefits of Embracing Direct Feedback
Developers who learn to appreciate and leverage direct feedback gain several significant advantages over their careers:
Accelerated Skill Development
Direct feedback highlights specific areas for improvement, allowing you to focus your learning efforts more effectively. Rather than plateauing, you continuously identify and address gaps in your knowledge and skills.
This targeted learning approach can compress years of gradual improvement into months of focused growth.
Increased Resilience
Regularly engaging with challenging feedback builds professional resilience. Over time, what once felt like harsh criticism begins to feel like valuable input.
This resilience extends beyond code reviews to other professional challenges, making you more adaptable throughout your career.
Stronger Collaboration Skills
Learning to process and respond constructively to direct feedback improves your ability to collaborate with diverse teams. You become known as someone who values improvement over ego—a highly desirable trait in collaborative environments.
Higher Quality Work
Perhaps most importantly, embracing feedback consistently leads to producing higher quality code. Each review cycle raises your personal standards and expands your understanding of what constitutes excellent software.
Over time, you’ll find yourself anticipating and addressing potential feedback before submitting your code, leading to increasingly sophisticated solutions.
Conclusion: Reframing “Harsh” as “Helpful”
The journey from feeling wounded by code review comments to appreciating their value is a transformative one that most successful developers undergo.
Remember these key insights:
- What feels like harsh feedback is often direct communication about objective code qualities
- The temporary discomfort of criticism is far outweighed by the long-term benefits of improvement
- Every developer, regardless of experience level, receives critical feedback throughout their career
- Learning to extract value from direct feedback is a career-accelerating skill
The next time you receive a code review comment that makes you wince, try this reframe: This isn’t an attack—it’s a personalized learning opportunity from someone who cared enough to provide detailed feedback.
By embracing rather than avoiding direct feedback, you position yourself for continuous growth in a field where learning never stops. The developers who advance most quickly are often those who actively seek out the most rigorous reviews they can get—because they understand that temporarily bruised feelings are a small price to pay for lasting improvement.
So the next time your code review feels harsh, take a deep breath, look for the learning opportunity, and remember: this feedback isn’t an obstacle to your success—it’s a crucial ingredient in it.