Why Your Code Organization Isn’t Scaling With Project Size
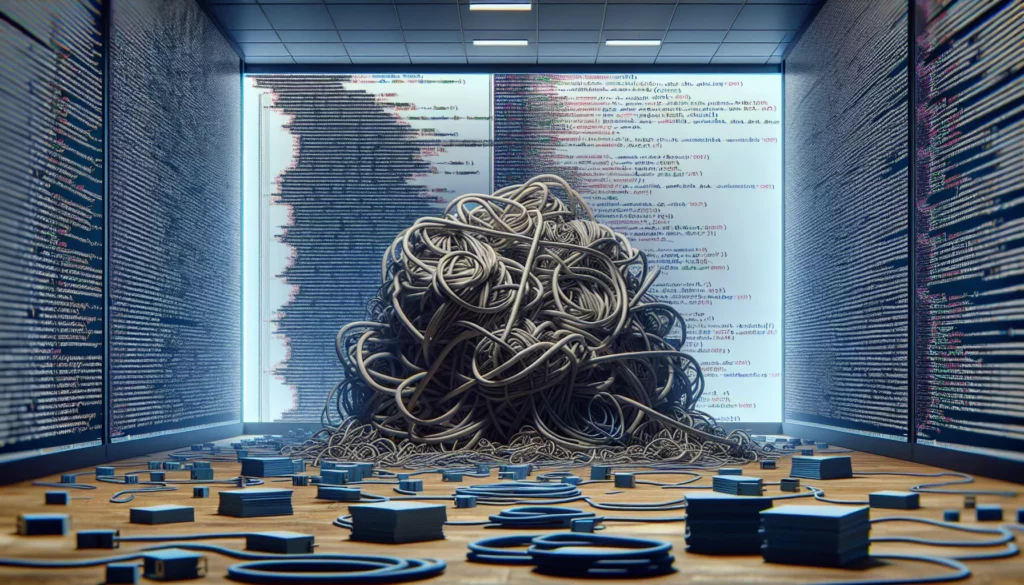
As software projects grow, many developers find themselves drowning in a sea of disorganized code. What started as a simple, manageable project gradually transforms into a complex labyrinth where finding and modifying code becomes increasingly difficult. If you’ve ever spent hours hunting down a bug in a massive codebase or struggled to add a seemingly simple feature, you’ve experienced the pain of poor code organization at scale.
In this comprehensive guide, we’ll explore why many codebases fail to scale effectively and provide practical strategies to organize your code for growth. Whether you’re a solo developer working on a side project or part of a large team building enterprise software, these principles will help you create maintainable, extensible codebases that can evolve with your needs.
Table of Contents
- Signs Your Code Organization Is Failing
- Common Mistakes in Code Organization
- Core Principles for Scalable Code Organization
- Architectural Patterns for Scalability
- The Power of Modularization
- Naming Conventions and Documentation
- Refactoring Strategies for Growing Codebases
- Testing as an Organizational Tool
- Tools and Technologies for Code Organization
- Team Practices for Maintaining Organization
- Case Studies: Before and After
- Conclusion: Building for the Future
Signs Your Code Organization Is Failing
Before we dive into solutions, let’s identify the warning signs that your code organization isn’t scaling well:
Increasing Bug Frequency
When changes in one part of your codebase unexpectedly break functionality in seemingly unrelated areas, it’s a clear indicator of poor separation of concerns and excessive coupling. As your project grows, these unexpected side effects become more common and harder to predict.
Developer Onboarding Takes Longer
New team members require weeks or months to become productive because understanding the codebase structure is too complex. They struggle to form a mental model of how components interact and where specific functionality resides.
Feature Development Slows Down
Tasks that used to take days now take weeks. Developers spend more time figuring out where and how to implement changes than actually writing code. The “fear factor” increases as developers become hesitant to modify existing code.
Code Duplication Proliferates
Developers create new implementations instead of reusing existing code because they can’t find or don’t understand existing functionality. This leads to multiple solutions for the same problems scattered throughout the codebase.
Test Coverage Decreases
As the codebase becomes more tangled, writing effective tests becomes increasingly difficult. Complex dependencies make unit testing challenging, and integration tests become brittle due to the many moving parts.
Common Mistakes in Code Organization
Most scalability issues stem from a few fundamental organizational mistakes:
Organizing by Technical Type Rather Than Domain
One of the most common mistakes is organizing code by technical type (controllers, models, services, etc.) rather than by domain or feature. This approach forces developers to jump between multiple directories to understand or modify a single feature.
For example, consider a typical MVC application structure:
/controllers
UserController.js
ProductController.js
OrderController.js
/models
User.js
Product.js
Order.js
/services
UserService.js
ProductService.js
OrderService.js
To understand the complete user management feature, a developer needs to look in three different directories. As the application grows, this problem compounds exponentially.
Monolithic File Structure
When files grow too large (often exceeding 1000 lines), they become difficult to navigate and understand. These “god objects” or “god files” typically handle too many responsibilities and violate the Single Responsibility Principle.
For example, a UserManager.js
file that handles authentication, profile management, preferences, permissions, and notifications will become unwieldy as each of these features expands.
Excessive Coupling Between Components
When components are tightly coupled, changes in one area cascade to others, creating a domino effect of modifications. This often happens when code directly references implementation details of other components instead of relying on well-defined interfaces.
Inconsistent Abstraction Levels
Mixing high-level business logic with low-level implementation details makes code harder to understand and maintain. When a file jumps between different levels of abstraction, it becomes difficult to extract a clear understanding of its purpose.
Premature Optimization for Flexibility
Overengineering for hypothetical future requirements often leads to unnecessarily complex code structures that are harder to understand and modify than simpler designs would have been.
Core Principles for Scalable Code Organization
To build codebases that scale effectively, follow these fundamental principles:
Single Responsibility Principle
Each module, class, or function should have one and only one reason to change. This principle, part of the SOLID design principles, encourages creating focused components that do one thing well.
For example, instead of a massive UserManager
class, you might have separate classes for UserAuthenticator
, ProfileManager
, PreferencesStore
, and PermissionsValidator
.
Encapsulation and Information Hiding
Components should expose well-defined interfaces while hiding their implementation details. This allows internal implementations to change without affecting consumers of the component.
Consider this JavaScript example:
// Poor encapsulation
const user = {
name: 'John',
email: 'john@example.com',
passwordHash: 'abc123hash'
};
// Better encapsulation
const user = (function() {
const privateData = {
name: 'John',
email: 'john@example.com',
passwordHash: 'abc123hash'
};
return {
getName: () => privateData.name,
getEmail: () => privateData.email,
authenticate: (password) => hashPassword(password) === privateData.passwordHash
};
})();
Dependency Inversion
High-level modules should not depend on low-level modules. Both should depend on abstractions. This principle facilitates testing and allows components to be replaced or modified with minimal impact on other parts of the system.
Composition Over Inheritance
Favor object composition over class inheritance for code reuse. Inheritance creates tight coupling between parent and child classes, while composition offers more flexibility and clearer separation of concerns.
Explicit Over Implicit
Make dependencies, behaviors, and intentions explicit in your code. Avoid “magic” that requires deep knowledge of the codebase to understand. Explicit code might be more verbose, but it’s easier to understand and maintain.
Architectural Patterns for Scalability
Several architectural patterns can help organize large codebases effectively:
Domain-Driven Design (DDD)
DDD organizes code around business domains and subdomain concepts rather than technical concerns. It emphasizes a ubiquitous language shared between developers and domain experts.
Key DDD concepts include:
- Bounded Contexts: Explicit boundaries between different parts of your domain model
- Entities and Value Objects: Different ways of modeling domain concepts
- Aggregates: Clusters of domain objects treated as a unit
- Repositories: Abstraction layer for data access
Clean Architecture / Hexagonal Architecture
These related architectural styles focus on separating concerns and making the business logic independent of external concerns like databases, UI, or third-party services.
The key principle is the dependency rule: source code dependencies should point only inward, toward higher-level policies. This creates a system where business rules don’t depend on UI or database details.
A typical clean architecture might have these layers:
- Entities: Enterprise-wide business rules
- Use Cases: Application-specific business rules
- Interface Adapters: Convert data between use cases and external formats
- Frameworks & Drivers: External frameworks, databases, UI, etc.
Microservices Architecture
For very large systems, microservices architecture breaks down the application into small, independently deployable services organized around business capabilities. Each service has its own codebase, allowing teams to work independently.
While microservices can help with organizational scaling, they introduce complexity in deployment, monitoring, and inter-service communication. They’re best suited for large organizations with multiple teams working on the same system.
Feature-Based Organization
Instead of organizing by technical layer, group code by feature or business capability. This approach makes it easier to locate all code related to a specific feature.
For example:
/features
/user-management
UserController.js
User.js
UserService.js
UserRepository.js
/product-catalog
ProductController.js
Product.js
ProductService.js
ProductRepository.js
/order-processing
OrderController.js
Order.js
OrderService.js
OrderRepository.js
This structure makes it immediately clear where to find all components related to user management, product catalogs, or order processing.
The Power of Modularization
Effective modularization is perhaps the most powerful technique for managing large codebases:
Module Boundaries
Well-defined modules have clear boundaries and communicate through explicit interfaces. They hide implementation details and maintain internal consistency.
When designing module boundaries, consider:
- Which functionality naturally belongs together?
- What changes together should stay together
- Which parts need to be reused separately?
Cohesion and Coupling
Aim for high cohesion (elements within a module are strongly related) and low coupling (minimal dependencies between modules). This makes individual modules easier to understand and modify without affecting other parts of the system.
Package by Feature vs. Package by Layer
Package by feature groups all elements of a feature together, while package by layer groups similar technical components. For most applications, packaging by feature leads to better organization at scale.
Practical Modularization Techniques
Here are some practical approaches to modularization:
- NPM/Yarn Workspaces: For JavaScript/TypeScript projects, workspaces allow managing multiple packages within a single repository
- Internal Libraries: Extract commonly used functionality into internal libraries with well-defined APIs
- Module Boundaries Enforcement: Use tools like ESLint with custom rules to enforce module boundaries
Here’s an example of a monorepo structure using workspaces:
/packages
/core
package.json
/src
index.ts
models.ts
/api
package.json
/src
index.ts
routes.ts
/ui
package.json
/src
index.tsx
components/
package.json
Naming Conventions and Documentation
Clear naming and documentation are crucial for codebase navigability:
Consistent Naming Patterns
Establish and follow consistent naming conventions for files, classes, functions, and variables. Good names should reveal intent and reduce the need for comments.
Some guidelines:
- Use meaningful, descriptive names that reveal purpose
- Be consistent with casing (camelCase, PascalCase, etc.)
- Use verbs for functions/methods and nouns for classes/variables
- Avoid abbreviations unless they’re universally understood
Self-Documenting Code
Write code that explains itself through clear structure and naming. Self-documenting code reduces the need for extensive comments and documentation.
Compare these two function implementations:
// Poorly self-documenting
function p(d, t) {
return d * (1 + t);
}
// Self-documenting
function calculatePriceWithTax(basePrice, taxRate) {
return basePrice * (1 + taxRate);
}
Documentation Strategies
Different types of documentation serve different purposes:
- API Documentation: Document public interfaces, parameters, return values, and exceptions
- Architecture Documentation: Explain the high-level structure, major components, and their relationships
- Decision Records: Document why certain design decisions were made
- Tutorials and Examples: Show how to use the code for common scenarios
Tools like JSDoc, TypeDoc, or Swagger can help generate documentation from code comments:
/**
* Calculates the final price including tax
*
* @param {number} basePrice - The price before tax
* @param {number} taxRate - The tax rate as a decimal (e.g., 0.07 for 7%)
* @returns {number} The final price including tax
*/
function calculatePriceWithTax(basePrice, taxRate) {
return basePrice * (1 + taxRate);
}
Refactoring Strategies for Growing Codebases
As codebases grow, regular refactoring becomes essential:
Incremental Refactoring
Large-scale refactoring is risky. Instead, use the “boy scout rule”: leave the code better than you found it. Make small improvements as you work on features or fix bugs.
Identifying Refactoring Targets
Look for these warning signs that indicate refactoring is needed:
- Code Smells: Duplicated code, long methods, large classes, etc.
- Hotspots: Files that change frequently or have many bugs
- High Cognitive Load: Code that’s difficult to understand at a glance
- Violated Principles: Code that breaks SOLID or other design principles
Common Refactoring Techniques
These refactoring patterns address common organizational issues:
- Extract Method/Class/Module: Break down large components into smaller, focused ones
- Move Method/Field: Relocate functionality to more appropriate classes
- Replace Conditional with Polymorphism: Use object-oriented patterns instead of complex conditionals
- Introduce Parameter Object: Group related parameters into a single object
- Replace Inheritance with Composition: Use composition for more flexible code organization
Safe Refactoring Practices
To refactor safely:
- Ensure good test coverage before starting
- Make small, incremental changes
- Commit frequently
- Run tests after each change
- Use automated refactoring tools when available
Testing as an Organizational Tool
Testing isn’t just for catching bugs; it’s also a powerful tool for code organization:
Test-Driven Development (TDD)
TDD forces you to think about interfaces and dependencies before implementation. By writing tests first, you naturally create more modular, loosely coupled code.
The TDD cycle:
- Write a failing test that defines the desired behavior
- Write the minimal code to pass the test
- Refactor the code while ensuring tests still pass
Testing Pyramid
Different types of tests enforce different organizational aspects:
- Unit Tests: Verify that individual components work correctly in isolation
- Integration Tests: Ensure that components work together correctly
- End-to-End Tests: Validate complete user workflows
A well-balanced testing pyramid (many unit tests, fewer integration tests, even fewer E2E tests) helps maintain organization at different levels of abstraction.
Tests as Documentation
Well-written tests serve as executable documentation, showing how components are intended to be used:
describe('UserService', () => {
describe('registerUser', () => {
it('should create a new user with hashed password', async () => {
// This test demonstrates how to use the UserService
const userService = new UserService(mockUserRepository);
const newUser = await userService.registerUser({
username: 'testuser',
email: 'test@example.com',
password: 'password123'
});
expect(newUser.username).toBe('testuser');
expect(newUser.email).toBe('test@example.com');
expect(newUser.password).not.toBe('password123'); // Password should be hashed
});
});
});
Tools and Technologies for Code Organization
Several tools can help maintain organization as codebases grow:
Static Analysis Tools
Tools like ESLint, SonarQube, or CodeClimate analyze code without running it to find potential issues:
- Enforce style guidelines and best practices
- Detect code smells and potential bugs
- Calculate metrics like complexity, coupling, and cohesion
- Identify duplicated code
Type Systems
Static typing with TypeScript, Flow, or similar tools provides several organizational benefits:
- Makes interfaces explicit
- Catches integration errors at compile time
- Provides better IDE support for navigation and refactoring
- Serves as inline documentation
Example of TypeScript interfaces defining clear boundaries:
interface User {
id: string;
username: string;
email: string;
}
interface UserRepository {
findById(id: string): Promise<User | null>;
findByEmail(email: string): Promise<User | null>;
create(user: Omit<User, 'id'>): Promise<User>;
update(id: string, data: Partial<User>): Promise<User>;
}
interface UserService {
getUserProfile(id: string): Promise<UserProfile>;
registerUser(data: RegistrationData): Promise<User>;
updateProfile(id: string, data: ProfileUpdateData): Promise<User>;
}
Dependency Management
Modern package managers and module systems help organize dependencies:
- NPM/Yarn workspaces for monorepos
- Semantic versioning for managing compatibility
- Lock files for dependency stability
- Module bundlers like Webpack or Rollup for code splitting
Visualization Tools
Tools that visualize code structure help identify organizational issues:
- Dependency graphs
- UML diagrams
- Architecture diagrams
- Heat maps showing complexity or change frequency
Team Practices for Maintaining Organization
Code organization is as much about people as it is about technology:
Code Reviews
Effective code reviews should evaluate organizational aspects:
- Is the code placed in the appropriate module?
- Does it follow established patterns and conventions?
- Are responsibilities clearly separated?
- Is the abstraction level appropriate?
Knowledge Sharing
Prevent knowledge silos through practices like:
- Pair programming
- Architecture decision records
- Internal tech talks
- Documentation sprints
- Code walkthroughs
Continuous Improvement
Regularly evaluate and improve your code organization:
- Schedule refactoring sprints
- Conduct architecture reviews
- Track technical debt
- Set measurable goals for code quality
Onboarding Processes
Design onboarding to reinforce good organizational practices:
- Provide architecture overviews
- Document code navigation strategies
- Assign small tasks across different areas of the codebase
- Pair new developers with experienced team members
Case Studies: Before and After
Let’s examine some real-world examples of codebase reorganization:
Case Study 1: Refactoring a Monolithic Web Application
Before: A web application organized by technical layers (controllers, models, services) had grown to over 200,000 lines of code. Developers spent 60% of their time understanding code rather than writing it. Features took weeks longer than estimated.
Approach:
- Reorganized code by domain/feature instead of technical layer
- Established clear module boundaries with explicit interfaces
- Created shared libraries for cross-cutting concerns
- Implemented a hexagonal architecture pattern
After: Developer productivity increased by 40%. Onboarding time for new developers decreased from months to weeks. Bug rates dropped by 30% as code became more predictable.
Case Study 2: Scaling a JavaScript Frontend
Before: A React application with 50+ components all in a flat directory structure. Component files averaged 500+ lines. State management was inconsistent, with some components using Redux, others using Context API, and some managing their own state.
Approach:
- Implemented Atomic Design methodology (atoms, molecules, organisms, templates, pages)
- Split large components into smaller, focused ones
- Standardized state management patterns
- Created a component library with Storybook documentation
After: Component reuse increased by 60%. New feature development time decreased by 35%. UI consistency improved across the application.
Case Study 3: Microservices Migration
Before: A monolithic backend application handling everything from authentication to payment processing. Changes required full redeployment, and teams blocked each other’s work.
Approach:
- Identified bounded contexts using Domain-Driven Design
- Incrementally extracted services, starting with the most independent ones
- Implemented API gateways and service discovery
- Established team ownership for each service
After: Teams could deploy independently. System resilience improved as failures were isolated to specific services. Scaling became more granular and cost-effective.
Conclusion: Building for the Future
Code organization isn’t just about aesthetics; it’s about creating systems that can grow and evolve over time. By following the principles and practices outlined in this guide, you can build codebases that scale with your project’s complexity.
Remember these key takeaways:
- Organize around business domains and features rather than technical layers
- Establish clear module boundaries with explicit interfaces
- Embrace the Single Responsibility Principle at all levels
- Use consistent naming and documentation to make code navigable
- Refactor continuously to prevent organizational debt
- Leverage testing to enforce good organization
- Use appropriate tools and technologies to support your organizational strategy
- Establish team practices that maintain organization over time
Effective code organization requires ongoing attention and discipline, but the investment pays dividends in developer productivity, system maintainability, and business agility. By addressing organizational issues early and consistently, you can prevent the all-too-common scenario where development grinds to a halt under the weight of its own complexity.
As your codebase grows, revisit your organizational strategies regularly. What works for a small project may not scale to a large one, and what works for one team may not work for another. Be willing to adapt your approach based on feedback and changing requirements.
With thoughtful organization, even the largest codebases can remain comprehensible, maintainable, and a joy to work with.