Why Your Code Optimization Is Premature (And What To Focus On Instead)
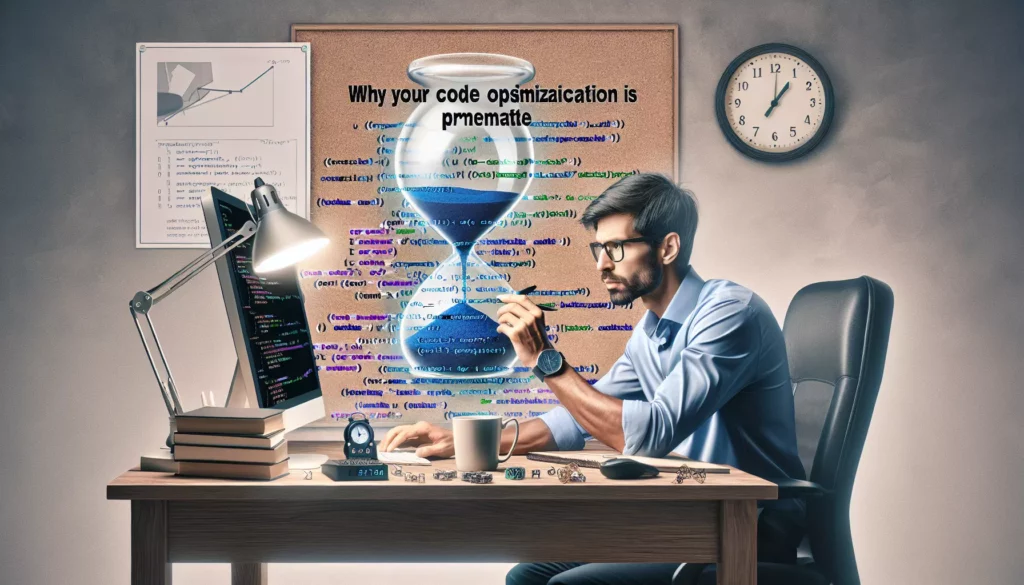
In the world of software development, there’s a famous quote by Donald Knuth that goes: “Premature optimization is the root of all evil.” Yet, many programmers, especially those new to the field, find themselves spending countless hours tweaking their code for performance before they’ve even confirmed there’s a performance problem to solve.
This obsession with optimization can lead to complex, hard-to-maintain code that might not even address the actual bottlenecks in your application. In this comprehensive guide, we’ll explore why premature optimization can be counterproductive, when you should actually optimize, and what you should focus on instead to become a more effective programmer.
What Is Premature Optimization?
Premature optimization refers to the practice of optimizing code for performance before you have evidence that optimization is necessary. It often involves making code more complex or less readable in pursuit of theoretical performance gains that may be negligible or unnecessary in practice.
Here’s a classic example: A developer might replace a simple, readable loop with a complex, manually unrolled version because they believe it will be faster, without measuring whether the performance difference matters in the context of their application.
The Complete Knuth Quote
While many developers know the shortened version of Knuth’s quote, the full statement provides important context:
“We should forget about small efficiencies, say about 97% of the time: premature optimization is the root of all evil. Yet we should not pass up our opportunities in that critical 3%.”
This nuance is crucial. Knuth isn’t suggesting we should never optimize; rather, he’s advocating for a measured approach where we focus our optimization efforts on the small percentage of code that will actually make a difference.
The Real Costs of Premature Optimization
When you optimize prematurely, you’re not just wasting time. You’re actively introducing several problems into your development process:
1. Increased Code Complexity
Optimized code is often more complex than its straightforward counterpart. This complexity makes the code harder to read, understand, and maintain—not just for others, but for your future self as well.
Consider this example of a simple function to find the maximum value in an array:
// Simple, readable approach
function findMax(array) {
let max = array[0];
for (let i = 1; i < array.length; i++) {
if (array[i] > max) {
max = array[i];
}
}
return max;
}
Now, imagine someone “optimizes” this with a divide-and-conquer approach:
// "Optimized" but more complex approach
function findMax(array) {
return findMaxRecursive(array, 0, array.length - 1);
}
function findMaxRecursive(array, start, end) {
if (start === end) return array[start];
const mid = Math.floor((start + end) / 2);
const leftMax = findMaxRecursive(array, start, mid);
const rightMax = findMaxRecursive(array, mid + 1, end);
return leftMax > rightMax ? leftMax : rightMax;
}
Is the second approach faster? Perhaps for very large arrays. But for most practical purposes, the performance difference would be negligible, while the complexity has increased substantially.
2. Extended Development Time
Time spent on premature optimization is time not spent on delivering features, fixing bugs, or improving the user experience. This opportunity cost can be substantial, especially in fast-moving projects or startups where time to market is critical.
3. Introducing Bugs
More complex code means more opportunities for bugs to creep in. What’s worse, these bugs might be subtle and hard to detect, especially if they’re related to edge cases in your optimization logic.
4. Hindering Future Changes
Heavily optimized code often makes assumptions about how it will be used. These assumptions can make the code brittle and resistant to change when requirements evolve—as they inevitably do.
When Optimization Actually Makes Sense
Despite the warnings against premature optimization, there are legitimate scenarios where optimization is not just beneficial but necessary:
1. When You Have Performance Requirements
Some applications have explicit performance requirements. For example, real-time systems, games, or high-frequency trading platforms often need to complete operations within strict time constraints. In these cases, optimization isn’t premature—it’s part of meeting the requirements.
2. When You Have Evidence of a Performance Problem
If users are complaining about slow response times, or if your monitoring shows that certain operations are taking too long, that’s a clear signal that optimization might be needed. But even then, you should measure first to identify the actual bottlenecks.
3. When You’re Working in a Performance-Critical Section
Some parts of your code will be executed much more frequently than others. Core algorithms, inner loops, or frequently called utility functions might be worth optimizing, even proactively, if you can demonstrate that they represent a significant portion of your application’s execution time.
The Right Approach to Optimization
If you’ve determined that optimization is indeed necessary, here’s a systematic approach to follow:
1. Measure First
Before making any changes, establish a baseline. Use profiling tools to identify where your application is spending most of its time. Modern development environments provide excellent profiling capabilities:
- Chrome DevTools Performance tab for JavaScript
- Visual Studio’s Performance Profiler
- Java’s VisualVM
- Python’s cProfile
Remember the adage: “Measure twice, cut once.” In optimization, it might be more like “Measure ten times, optimize once.”
2. Focus on Algorithms and Data Structures
The biggest performance gains usually come from algorithmic improvements, not micro-optimizations. Changing from an O(n²) algorithm to an O(n log n) algorithm will yield far greater benefits than tweaking an already efficient algorithm.
Consider this example of finding duplicate values in an array:
// O(n²) approach
function findDuplicates(array) {
const duplicates = [];
for (let i = 0; i < array.length; i++) {
for (let j = i + 1; j < array.length; j++) {
if (array[i] === array[j] && !duplicates.includes(array[i])) {
duplicates.push(array[i]);
}
}
}
return duplicates;
}
A better approach using a different data structure:
// O(n) approach
function findDuplicates(array) {
const seen = new Set();
const duplicates = new Set();
for (const item of array) {
if (seen.has(item)) {
duplicates.add(item);
} else {
seen.add(item);
}
}
return [...duplicates];
}
The second approach is not just marginally faster—it’s in an entirely different complexity class, which means it will perform vastly better for large inputs.
3. Optimize the Critical Path
After measuring, you’ll likely find that a small portion of your code accounts for a large percentage of execution time. This is often referred to as the 80/20 rule or Pareto principle: 80% of the time is spent in 20% of the code.
Focus your optimization efforts on this critical path. Optimizing code that’s rarely executed or already fast enough will yield minimal benefits.
4. Make One Change at a Time
When optimizing, change one thing at a time and measure the impact. This methodical approach helps you understand which changes are actually beneficial and which might be detrimental.
5. Consider the Trade-offs
Always weigh the benefits of optimization against the costs. Will the performance gain justify the increased complexity, development time, and potential for bugs? Sometimes, a slightly slower but more maintainable solution is the better choice.
What to Focus On Instead of Premature Optimization
If not premature optimization, what should developers focus on to create high-quality software? Here are some priorities that often yield better returns:
1. Code Clarity and Maintainability
Clear, maintainable code is easier to debug, extend, and optimize when necessary. Prioritize writing code that clearly expresses its intent and is easy for others (and your future self) to understand.
Consider these two implementations of a function to check if a string is a palindrome:
// Less readable
function p(s) {
return s === s.split("").reverse().join("");
}
Versus:
// More readable
function isPalindrome(text) {
const reversed = text.split("").reverse().join("");
return text === reversed;
}
The second version takes a few more lines, but it’s immediately clear what the function does and how it works.
2. Correctness
Before optimizing for speed, ensure your code is correct. A fast but incorrect solution is worse than a slow but correct one. Invest in comprehensive testing to verify that your code behaves as expected in all scenarios.
3. Good Architecture and Design
A well-designed system is easier to optimize when needed. Focus on creating a clean architecture with clear separation of concerns, appropriate abstractions, and minimal coupling between components.
For example, separating your business logic from your data access and presentation layers makes it easier to optimize each layer independently when necessary.
4. User Experience
Often, perceived performance matters more than actual performance. Techniques like progressive loading, providing feedback during long operations, and optimizing the critical rendering path can make your application feel faster to users, even if the underlying operations take the same amount of time.
5. Scalability
Rather than optimizing for the current load, design your system to scale. This might involve horizontal scaling (adding more servers), caching strategies, or asynchronous processing—approaches that can accommodate growing demand without requiring constant code optimization.
Common Optimization Myths and Misconceptions
Let’s debunk some common myths about optimization that often lead developers astray:
Myth 1: “Faster Code Is Always Better”
Reality: Code that’s marginally faster but significantly more complex or harder to maintain is often not worth the trade-off. The small performance gain might be imperceptible to users but could cost you dearly in development time and bug fixes.
Myth 2: “I Know What’s Slow Without Measuring”
Reality: Intuition about performance bottlenecks is notoriously unreliable, even for experienced developers. Modern compilers, interpreters, and runtime environments perform sophisticated optimizations that can make seemingly inefficient code run quickly and vice versa.
Myth 3: “More Memory Usage Is Always Bad”
Reality: Sometimes, using more memory can significantly improve performance by avoiding recomputation or reducing disk access. The trade-off between memory usage and speed should be evaluated based on your specific constraints and requirements.
Myth 4: “Optimization Is a One-Time Task”
Reality: Performance optimization is an ongoing process, not a one-time task. As your application evolves, new bottlenecks may emerge, and what was once a performance hotspot might become less significant.
Real-World Case Studies
Let’s examine some real-world examples of premature optimization gone wrong and effective optimization done right:
Case Study 1: The Over-Engineered Shopping Cart
A development team was building an e-commerce platform and decided to optimize their shopping cart implementation from the start. They used complex data structures and algorithms to ensure that cart operations would be lightning-fast, even with thousands of items.
The result? A shopping cart system that was indeed fast but also buggy and difficult to modify. When they needed to add features like saved carts or wishlist integration, the complex implementation made changes challenging. Moreover, their analytics showed that the average customer had only 3-5 items in their cart, making the optimizations unnecessary.
The lesson: They should have started with a simple implementation, measured actual usage patterns, and then optimized only if needed.
Case Study 2: The Database Query Transformation
A web application was experiencing slow page loads. The development team assumed the problem was inefficient JavaScript and spent weeks refactoring their front-end code for performance.
When they finally measured, they discovered the real bottleneck: a single database query that was retrieving far more data than needed and performing expensive joins. By optimizing just this query—adding appropriate indexes and fetching only the required fields—they achieved a 10x performance improvement with minimal code changes.
The lesson: Measure before optimizing to identify the actual bottlenecks.
Practical Tips for Balancing Performance and Maintainability
Here are some practical guidelines to help you strike the right balance between performance and code quality:
1. Write Clean Code First
Start by writing clear, correct, and maintainable code. This provides a solid foundation that you can optimize later if necessary.
2. Establish Performance Budgets
Define acceptable performance metrics for your application, such as maximum page load time or response time for critical operations. These budgets give you objective criteria for when optimization is necessary.
3. Implement Performance Monitoring
Set up monitoring to track your application’s performance in production. Tools like New Relic, Datadog, or even simple application logs can help you identify when performance degrades and which operations are causing problems.
4. Document Your Optimizations
When you do optimize code, document why and how you did it. This context helps future developers understand the reasoning behind complex code and make informed decisions about further changes.
// Optimized version of the matrix multiplication algorithm
// This uses tiling to improve cache locality and reduce memory access times
// Benchmarks showed a 40% performance improvement for matrices larger than 1000x1000
function multiplyMatricesFast(a, b) {
// Implementation details...
}
5. Use Abstractions to Hide Complexity
When you do need to write complex, optimized code, encapsulate it behind clean interfaces. This allows you to change the implementation details without affecting the rest of your codebase.
6. Consider Different Optimization Strategies
Sometimes, the best optimization doesn’t involve changing your code at all. Consider strategies like:
- Caching frequently accessed data
- Using content delivery networks (CDNs) for static assets
- Implementing load balancing for better resource utilization
- Leveraging database query optimizations
Preparing for Technical Interviews: The Optimization Perspective
If you’re preparing for technical interviews, especially at major tech companies, understanding optimization is crucial. However, the approach differs from day-to-day development:
In Interviews, Algorithm Efficiency Matters
During coding interviews, you’re often expected to provide the most efficient solution possible. This isn’t premature optimization; it’s demonstrating your knowledge of algorithms and data structures.
Balance Explanation with Implementation
In an interview, explain your thought process: start with a simple solution, analyze its efficiency, and then improve it. This shows that you understand both practical programming and theoretical computer science.
For example, if asked to find the kth largest element in an array:
// First, mention the simple approach
function findKthLargest(nums, k) {
// Sort the array in descending order
nums.sort((a, b) => b - a);
// Return the kth element (0-indexed)
return nums[k - 1];
}
// Time complexity: O(n log n) due to sorting
Then, explain a more efficient approach:
// Then, propose a more efficient solution using a min-heap
function findKthLargest(nums, k) {
// Using a priority queue / heap would give us O(n log k) time complexity
// In JavaScript, we could implement this with a min-heap
// Pseudocode:
// 1. Create a min-heap of size k
// 2. For each element in the array:
// a. If the heap size is less than k, add the element
// b. Else if the element is larger than the smallest in the heap,
// remove the smallest and add the new element
// 3. The root of the heap is the kth largest element
// (Implementation details would follow)
}
// Time complexity: O(n log k)
Know When to Optimize in Real Systems
Be prepared to discuss how you would approach optimization in a real-world scenario. Interviewers often want to see that you understand the balance between theoretical efficiency and practical considerations.
Conclusion: The Art of Knowing When to Optimize
Optimization is a powerful tool in a developer’s arsenal, but like any tool, it must be used at the right time and in the right way. Premature optimization can lead to complex, bug-prone code that solves problems you don’t actually have.
Instead of optimizing by default, adopt a measured approach:
- Start with clear, correct, maintainable code
- Establish performance requirements
- Measure to identify actual bottlenecks
- Optimize the critical few areas that will make a real difference
- Validate your optimizations with further measurement
Remember Knuth’s complete wisdom: premature optimization is problematic, but don’t miss the opportunities in that critical 3% of your code where optimization truly matters.
By focusing on writing good code first and optimizing selectively based on evidence, you’ll create software that’s not just fast, but also reliable, maintainable, and adaptable to changing requirements—qualities that are often more valuable than raw speed in the long run.
The true art of optimization isn’t knowing how to make code faster; it’s knowing when to make code faster, and when to leave well enough alone.