Why Your Code Has More Issues Than Your Relationships
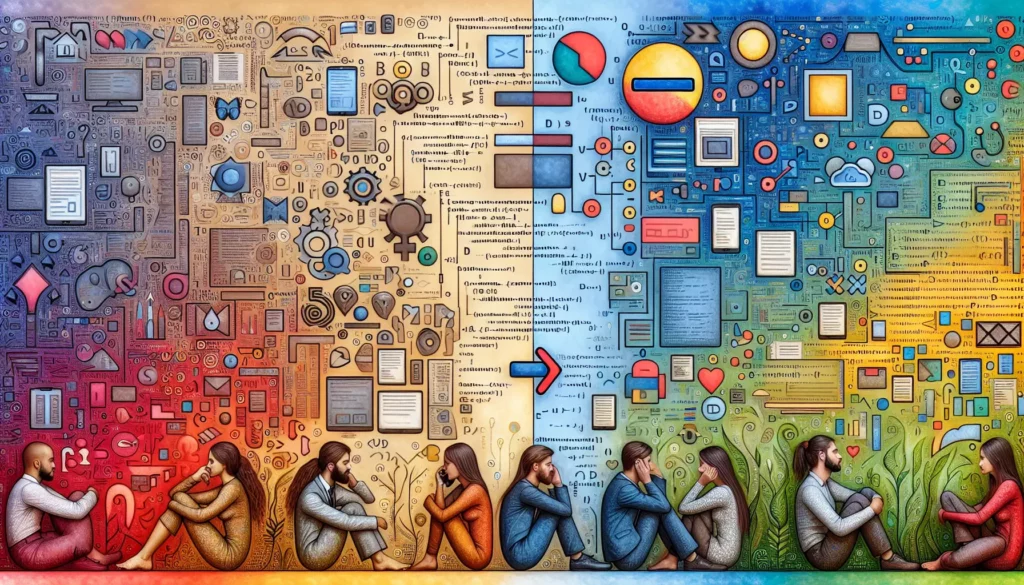
In the world of programming, it’s not uncommon to find yourself in a love-hate relationship with your code. You spend countless hours nurturing it, debugging it, and trying to make it perfect. Yet, somehow, it always seems to have more issues than your personal relationships. If you’ve ever found yourself wondering why your code is so problematic, you’re not alone. Let’s dive into the reasons why your code might be giving you more headaches than your significant other.
1. Complexity: The Root of All Evil
Just like relationships, code can become incredibly complex over time. As your project grows, so does the number of interconnected parts, dependencies, and potential points of failure. This complexity is often the root cause of many issues in your code.
Consider this: in a relationship, you typically deal with one person (or a few, if you’re into polyamory). In code, you’re juggling hundreds, thousands, or even millions of lines, each with its own quirks and potential for conflict.
How to Address Complexity
- Modularization: Break your code into smaller, manageable modules. This is like focusing on different aspects of a relationship one at a time.
- Clean Code Principles: Follow best practices like SOLID principles to keep your code organized and maintainable.
- Regular Refactoring: Don’t be afraid to revisit and improve your code. It’s like relationship counseling, but for your software.
2. Communication Breakdown: When Code Doesn’t Speak Your Language
In relationships, communication is key. The same goes for coding. However, unlike human languages that evolve over thousands of years, programming languages and frameworks change at a breakneck pace. This rapid evolution can lead to misunderstandings between you and your code.
Common Communication Issues in Code
- Outdated Documentation: When documentation doesn’t keep up with code changes, it’s like trying to understand your partner using an outdated dictionary.
- Inconsistent Naming Conventions: Imagine if your partner kept changing their name every day. That’s what inconsistent naming in code feels like.
- Lack of Comments: Code without comments is like a partner who never explains their actions. It leaves you constantly guessing.
Improving Code Communication
To enhance communication in your code:
- Write clear, concise comments that explain the ‘why’ behind your code, not just the ‘what’.
- Use meaningful variable and function names that describe their purpose.
- Keep your documentation up-to-date, treating it as an integral part of your codebase.
Here’s an example of how commenting can improve code readability:
// Bad example
function calc(a, b) {
return a * b + 100;
}
// Good example
/**
* Calculates the total cost including a fixed service fee.
* @param {number} price - The base price of the item.
* @param {number} quantity - The number of items purchased.
* @returns {number} The total cost including the service fee.
*/
function calculateTotalCost(price, quantity) {
const SERVICE_FEE = 100;
return price * quantity + SERVICE_FEE;
}
3. Trust Issues: Debugging and Testing
Trust is fundamental in any relationship, including the one you have with your code. When your code behaves unexpectedly or breaks without warning, it can feel like a betrayal. This is where debugging and testing come into play.
Why Your Code Might Be Untrustworthy
- Lack of Testing: Without proper testing, you’re essentially trusting your code blindly.
- Poor Error Handling: When your code doesn’t gracefully handle exceptions, it’s like a partner who overreacts to every small problem.
- Hidden Dependencies: Unexpected interactions between different parts of your code can lead to trust issues.
Building Trust with Your Code
To establish a more trustworthy relationship with your code:
- Implement comprehensive unit tests to catch issues early.
- Use integration and end-to-end tests to ensure different parts of your application work well together.
- Implement proper error handling and logging to understand what goes wrong and why.
Here’s an example of how you might implement a simple unit test:
// Function to test
function add(a, b) {
return a + b;
}
// Test suite
describe('add function', () => {
test('adds 1 + 2 to equal 3', () => {
expect(add(1, 2)).toBe(3);
});
test('adds -1 + 1 to equal 0', () => {
expect(add(-1, 1)).toBe(0);
});
test('adds 0.1 + 0.2 to be close to 0.3', () => {
expect(add(0.1, 0.2)).toBeCloseTo(0.3);
});
});
4. Commitment Issues: Version Control and Code Management
In relationships, commitment can be a touchy subject. In coding, commitment is literally part of the process, thanks to version control systems like Git. However, poor version control practices can lead to a whole host of issues.
Signs of Commitment Issues in Your Code
- Infrequent Commits: Like a partner who’s afraid to commit, not committing your code regularly can lead to big, messy conflicts.
- Poor Commit Messages: Vague or unhelpful commit messages are like trying to remember important dates in your relationship without writing them down.
- Lack of Branching Strategy: Working directly on the main branch is like making major life decisions without consulting your partner.
Improving Your Commitment to Code
To better manage your code and avoid commitment issues:
- Commit early and often, with clear and descriptive commit messages.
- Use feature branches for new developments and bug fixes.
- Implement a code review process to ensure quality before merging into the main branch.
Here’s an example of a good commit message structure:
feat: add user authentication functionality
- Implement JWT-based authentication
- Create login and registration endpoints
- Add password hashing using bcrypt
Closes #123
5. Emotional Baggage: Legacy Code and Technical Debt
Just as people carry emotional baggage from past relationships, codebases often carry the weight of legacy code and technical debt. This “baggage” can make it difficult to move forward and implement new features efficiently.
Signs of Emotional Baggage in Your Code
- Outdated Libraries and Frameworks: Using old, unsupported technologies is like holding onto mementos from past relationships.
- Spaghetti Code: Tangled, hard-to-follow code is like a complex web of unresolved past issues.
- Duplicate Code: Repeating the same mistakes (or code) over and over is a sign of not learning from the past.
Dealing with Your Code’s Emotional Baggage
To address legacy code and technical debt:
- Gradually refactor problematic areas of your codebase.
- Implement a strategy for updating dependencies regularly.
- Use design patterns and architectural principles to organize your code better.
Here’s an example of how you might refactor some legacy code:
// Old, problematic code
function getUserData(userId) {
// Directly accessing database
const db = new Database();
const userData = db.query(`SELECT * FROM users WHERE id = ${userId}`);
db.close();
return userData;
}
// Refactored code
class UserRepository {
constructor(database) {
this.db = database;
}
getUserById(userId) {
return this.db.query('SELECT * FROM users WHERE id = ?', [userId]);
}
}
function getUserData(userId, userRepository) {
return userRepository.getUserById(userId);
}
6. Unrealistic Expectations: Balancing Perfection and Pragmatism
In both relationships and coding, setting unrealistic expectations can lead to frustration and disappointment. Striving for perfect code can sometimes be counterproductive, leading to over-engineering and missed deadlines.
Signs of Unrealistic Expectations in Your Code
- Over-optimization: Spending excessive time optimizing code that doesn’t need it.
- Feature Creep: Continuously adding new features without considering the overall impact on the project.
- Perfectionism: Refusing to ship code until it’s “perfect,” which it never will be.
Finding Balance in Your Coding Practices
To manage expectations and find a healthy balance:
- Embrace the principle of “Good Enough” – focus on delivering working, maintainable code rather than perfect code.
- Use agile methodologies to break down large projects into manageable sprints.
- Regularly reassess project priorities and be willing to adjust your approach.
7. Lack of Growth: Continuous Learning and Improvement
Both relationships and codebases need continuous effort to grow and improve. Stagnation in your coding practices can lead to a buildup of issues over time.
Signs of Stagnation in Your Coding Practices
- Resistance to New Technologies: Refusing to learn new languages or frameworks.
- Ignoring Industry Trends: Not keeping up with best practices and emerging patterns.
- Lack of Code Reviews: Not seeking or providing feedback on code.
Fostering Growth in Your Coding Journey
To promote continuous improvement:
- Allocate time for learning new technologies and techniques.
- Participate in coding communities and open-source projects.
- Implement regular code reviews and pair programming sessions.
8. Neglect: The Silent Killer of Code Quality
Just as neglect can destroy a relationship, neglecting your code can lead to a gradual decline in quality and maintainability. This neglect often manifests in small ways that compound over time.
Signs of Code Neglect
- Ignored Warnings: Dismissing compiler or linter warnings without addressing them.
- Outdated Documentation: Failing to update documentation as the code evolves.
- Skipped Refactoring: Putting off necessary refactoring tasks in favor of quick fixes.
Giving Your Code the Attention It Deserves
To combat neglect and maintain code quality:
- Set up automated tools like linters and static code analyzers to catch issues early.
- Schedule regular “housekeeping” sessions to address technical debt and minor issues.
- Treat documentation as a first-class citizen, updating it alongside code changes.
Here’s an example of how you might set up a linter configuration (e.g., for ESLint in JavaScript):
{
"extends": "eslint:recommended",
"rules": {
"indent": ["error", 2],
"quotes": ["error", "single"],
"semi": ["error", "always"],
"no-unused-vars": "warn",
"no-console": "warn"
},
"env": {
"node": true,
"es6": true
}
}
9. Compatibility Issues: When Your Code Doesn’t Play Well with Others
In relationships, compatibility is crucial. The same applies to code. Your code needs to work well with other parts of the system, third-party libraries, and even different environments.
Common Compatibility Issues in Code
- Browser Inconsistencies: Code that works in one browser but breaks in another.
- Dependency Conflicts: When different parts of your application require incompatible versions of the same library.
- Platform-Specific Bugs: Issues that only occur on certain operating systems or devices.
Improving Code Compatibility
To enhance the compatibility of your code:
- Use cross-platform testing tools to catch browser-specific issues early.
- Implement a robust dependency management strategy.
- Write platform-agnostic code when possible, and clearly document any platform-specific features.
10. Lack of Boundaries: Coupling and Cohesion
In healthy relationships, maintaining proper boundaries is essential. In coding, this concept translates to managing coupling (the degree of interdependence between modules) and cohesion (the degree to which elements of a module belong together).
Signs of Poor Boundaries in Code
- High Coupling: Changes in one part of the code frequently require changes in many other parts.
- Low Cohesion: Modules or classes that try to do too many unrelated things.
- Global State Abuse: Overuse of global variables or singletons.
Establishing Healthy Boundaries in Your Code
To improve the structure and maintainability of your code:
- Apply the Single Responsibility Principle to keep classes and functions focused.
- Use dependency injection to manage object creation and lifetime.
- Implement clear interfaces between modules to minimize coupling.
Here’s an example of improving code boundaries:
// Poor boundaries
class UserManager {
constructor() {
this.database = new Database();
this.logger = new Logger();
this.emailService = new EmailService();
}
createUser(userData) {
// Create user in database
// Log the action
// Send welcome email
}
}
// Improved boundaries
class UserRepository {
constructor(database) {
this.database = database;
}
createUser(userData) {
// Create user in database
}
}
class UserService {
constructor(userRepository, logger, emailService) {
this.userRepository = userRepository;
this.logger = logger;
this.emailService = emailService;
}
createUser(userData) {
const user = this.userRepository.createUser(userData);
this.logger.log('User created', user);
this.emailService.sendWelcomeEmail(user.email);
return user;
}
}
Conclusion: Nurturing a Healthy Relationship with Your Code
Just like personal relationships, your relationship with your code requires attention, care, and continuous effort. By addressing these common issues – from managing complexity and improving communication to establishing trust and maintaining boundaries – you can create a codebase that’s not only functional but also a joy to work with.
Remember, perfect code doesn’t exist, just as perfect relationships don’t exist. The goal is to create code that’s maintainable, scalable, and resilient. This requires a balance of technical skills, best practices, and a mindset of continuous improvement.
As you continue your coding journey, treat your code with the same respect and care you would a valued partner. Communicate clearly, set realistic expectations, be willing to learn and grow, and don’t be afraid to seek help when needed. With time and effort, you’ll find that your code can be just as rewarding (and sometimes less complicated) than your personal relationships.
Happy coding, and may your commits be ever in your favor!