Why Your Code Formatting Tools Aren’t Improving Readability
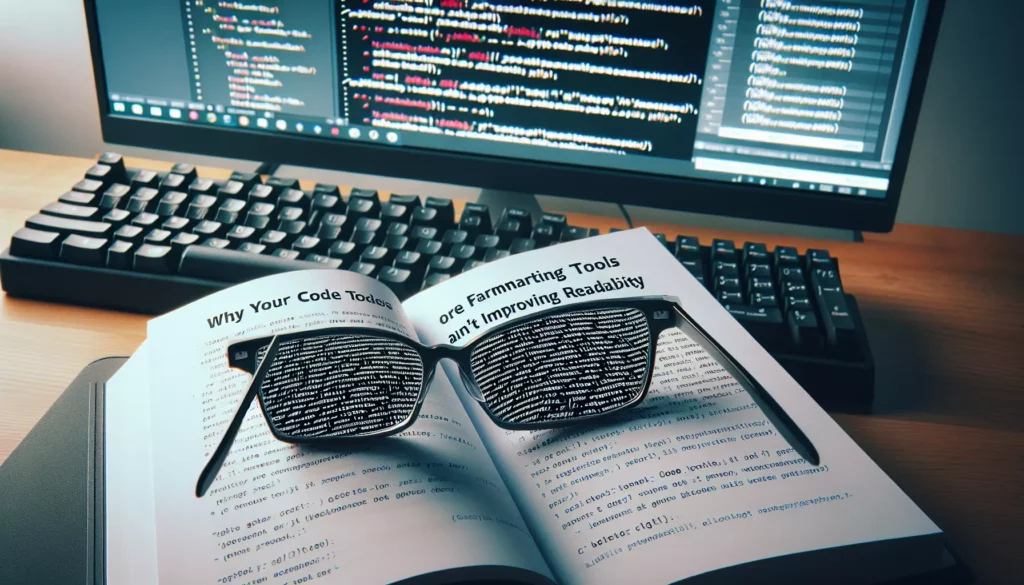
Code readability is a cornerstone of efficient programming. We’ve all heard the adage that code is read more often than it’s written. Yet despite the proliferation of code formatting tools promising to make our code more readable, many developers still struggle with comprehension when reviewing code.
So why aren’t your code formatting tools delivering on their promise of improved readability? In this comprehensive guide, we’ll explore the limitations of automated formatting tools, what truly makes code readable, and how to move beyond superficial fixes to create genuinely maintainable code.
Table of Contents
- Understanding Code Readability
- The Limitations of Automated Formatting Tools
- Beyond Formatting: What Actually Makes Code Readable
- The Cognitive Aspects of Code Comprehension
- Balancing Automation and Human Judgment
- Practical Strategies for Truly Readable Code
- Team Approaches to Code Readability
- The Future of Code Readability Tools
- Conclusion
Understanding Code Readability
Before diving into why formatting tools might be falling short, let’s establish what we mean by “readable code.” Code readability encompasses several dimensions:
- Visual clarity: Consistent indentation, spacing, and line breaks
- Cognitive load: How much mental effort is required to understand the code
- Semantic clarity: How well the code communicates its purpose and intent
- Navigability: How easily developers can find what they’re looking for
- Consistency: Following predictable patterns and conventions
Most formatting tools focus predominantly on visual clarity, which is only one piece of the readability puzzle. This narrow focus explains why even perfectly formatted code can still be difficult to understand.
Consider this perfectly formatted but difficult-to-understand example:
function processData(data) {
const x = data.map(i => i.v);
const y = x.filter(v => v > 0);
const z = y.reduce((a, b) => a + b, 0);
return z / y.length;
}
The formatting is consistent, but the variable names and structure don’t communicate the code’s purpose. Compare it to:
function calculateAverageOfPositiveValues(dataPoints) {
const allValues = dataPoints.map(point => point.value);
const positiveValues = allValues.filter(value => value > 0);
const sumOfPositiveValues = positiveValues.reduce((sum, value) => sum + value, 0);
return sumOfPositiveValues / positiveValues.length;
}
Same functionality, similar formatting, but vastly different readability.
The Limitations of Automated Formatting Tools
Popular code formatting tools like Prettier, ESLint, Black, or gofmt have transformed how teams maintain consistent code style. However, they have inherent limitations:
1. They Focus on Syntax, Not Semantics
Formatting tools can ensure your braces align and your indentation is consistent, but they can’t understand what your code is trying to accomplish. They can’t tell you that your variable name x
should be userAccountBalance
or that your function is doing too many things at once.
Consider this formatting-compliant code:
function f(a, b, c) {
let x = 0;
if (a) {
x = b + c;
} else {
x = b - c;
}
return x;
}
A formatter will ensure the braces and indentation are consistent, but it won’t suggest renaming the function to calculateResult
or the variables to more descriptive names.
2. They Can’t Assess Logical Structure
Code formatters don’t evaluate whether your code’s organizational structure makes sense. They won’t tell you when:
- A function is too long or does too many things
- A class has too many responsibilities
- Your conditional logic is unnecessarily complex
- Your code would benefit from being split into smaller functions
For example, a formatter will happily format a 500-line function with nested if statements 10 levels deep. It might look consistent, but it won’t be readable.
3. They Can Sometimes Make Readability Worse
In some cases, strict formatting rules can actually reduce readability by breaking up logical groupings or forcing unnatural line breaks. Consider this example of a complex SQL query being built:
// Before formatting
const query =
"SELECT users.name, users.email, " +
"COUNT(orders.id) AS order_count, " +
"SUM(orders.amount) AS total_spent " +
"FROM users " +
"LEFT JOIN orders ON users.id = orders.user_id " +
"WHERE users.status = 'active' " +
"GROUP BY users.id " +
"HAVING total_spent > 1000 " +
"ORDER BY total_spent DESC";
// After automated formatting
const query = "SELECT users.name, users.email, " + "COUNT(orders.id) AS order_count, " + "SUM(orders.amount) AS total_spent " + "FROM users " + "LEFT JOIN orders ON users.id = orders.user_id " + "WHERE users.status = 'active' " + "GROUP BY users.id " + "HAVING total_spent > 1000 " + "ORDER BY total_spent DESC";
The formatter has made the code technically “correct” but has eliminated the visual structure that made the SQL query components easy to identify.
4. They Don’t Account for Context
Formatting tools apply the same rules universally, without understanding the specific context of the code. Sometimes, deviating from standard formatting can actually enhance readability in specific situations.
For instance, some mathematical or tabular data might be more readable with specific alignment:
// Human-formatted for clarity
const matrix = [
[1.0, 0.0, 0.0],
[0.0, 1.0, 0.0],
[0.0, 0.0, 1.0]
];
// After formatting tool
const matrix = [
[1.0, 0.0, 0.0],
[0.0, 1.0, 0.0],
[0.0, 0.0, 1.0]
];
In this case, the formatting tool preserved the readability, but in more complex examples, the tool might rearrange the spacing in ways that make the matrix structure less visually apparent.
Beyond Formatting: What Actually Makes Code Readable
To truly improve code readability, we need to look beyond what automated formatters can provide. Here are the aspects of readability that require human judgment:
1. Meaningful Naming
Perhaps nothing impacts readability more than how we name things. Good names serve as documentation and make code self-explanatory:
// Poor naming
function calc(arr) {
let res = 0;
for (let i = 0; i < arr.length; i++) {
res += arr[i].val;
}
return res;
}
// Better naming
function calculateTotalRevenue(transactions) {
let totalRevenue = 0;
for (let i = 0; i < transactions.length; i++) {
totalRevenue += transactions[i].amount;
}
return totalRevenue;
}
No formatting tool can make these naming improvements for you. They require understanding the code’s purpose and context.
2. Appropriate Abstraction
Breaking complex operations into well-named functions with single responsibilities dramatically improves readability:
// Without appropriate abstraction
function processUserData(users) {
// 100 lines of complex code mixing multiple concerns
}
// With appropriate abstraction
function processUserData(users) {
const validUsers = filterActiveUsers(users);
const enrichedUsers = addUserMetadata(validUsers);
const sortedUsers = sortByPriority(enrichedUsers);
return generateUserReports(sortedUsers);
}
function filterActiveUsers(users) {
// 20 focused lines
}
function addUserMetadata(users) {
// 25 focused lines
}
// And so on...
The second approach is far more readable because it separates concerns and creates a clear narrative of what the code is doing.
3. Consistent Patterns and Idioms
Using consistent patterns throughout your codebase reduces the cognitive load of understanding new code. When developers recognize familiar patterns, they can focus on the unique aspects of the code rather than figuring out basic structure:
// Consistent pattern for API request handlers
async function getUserHandler(req, res) {
try {
const user = await userService.getUser(req.params.id);
return res.json({ success: true, data: user });
} catch (error) {
return handleApiError(error, res);
}
}
async function createUserHandler(req, res) {
try {
const user = await userService.createUser(req.body);
return res.json({ success: true, data: user });
} catch (error) {
return handleApiError(error, res);
}
}
Once a developer understands this pattern, every new handler they encounter will be immediately familiar.
4. Strategic Comments
While code should be as self-explanatory as possible, strategic comments can significantly enhance readability by explaining “why” rather than “what”:
// Don't do this
// Increment i
i++;
// Do this
// We're using a custom increment to handle the special case of overflow
// when operating in legacy compatibility mode
i = customIncrement(i);
Comments are most valuable when they explain non-obvious design decisions, business rules, or complex algorithms.
The Cognitive Aspects of Code Comprehension
Understanding how humans process and comprehend code can help us write more readable software. Research in program comprehension highlights several key findings:
1. Chunking and Mental Models
Humans understand code by building mental models and “chunking” related information. When code is structured to support these natural cognitive processes, it becomes more readable.
For example, grouping related operations together:
// Hard to chunk mentally
const name = user.name;
const products = getProducts();
const email = user.email;
const filteredProducts = filterProductsByPreference(products, user.preferences);
const phone = user.phone;
const sortedProducts = sortProductsByRelevance(filteredProducts);
// Easier to chunk mentally
// User information
const name = user.name;
const email = user.email;
const phone = user.phone;
// Product processing
const products = getProducts();
const filteredProducts = filterProductsByPreference(products, user.preferences);
const sortedProducts = sortProductsByRelevance(filteredProducts);
The second example supports natural chunking by grouping related operations, making it easier to comprehend.
2. Progressive Disclosure
Code is most readable when it reveals details progressively, starting with high-level concepts and allowing the reader to dive deeper as needed. This is why well-structured code with appropriate abstraction is easier to understand:
function processPayment(order) {
validateOrder(order);
calculateTotals(order);
chargeCustomer(order);
sendReceipt(order);
}
// Readers can stop here for a high-level understanding,
// or continue to implementation details if needed
function validateOrder(order) {
// Implementation details...
}
This structure allows readers to understand the high-level flow before diving into details.
3. Cognitive Load and Working Memory
Humans have limited working memory capacity. Code that requires tracking many variables, conditions, or nested levels simultaneously is inherently harder to understand.
Consider this hard-to-follow code:
function processData(data) {
let result = [];
for (let i = 0; i < data.length; i++) {
if (data[i].type === 'A' || data[i].type === 'B') {
if (data[i].value > 10 && (!data[i].isProcessed || data[i].priority === 'high')) {
if (checkAvailability(data[i].id) && data[i].region !== 'excluded') {
result.push(transformItem(data[i]));
}
}
}
}
return result;
}
Versus this more manageable version:
function processData(data) {
return data
.filter(isEligibleType)
.filter(meetsValueThreshold)
.filter(isAvailableInRegion)
.map(transformItem);
}
function isEligibleType(item) {
return item.type === 'A' || item.type === 'B';
}
function meetsValueThreshold(item) {
return item.value > 10 && (!item.isProcessed || item.priority === 'high');
}
function isAvailableInRegion(item) {
return checkAvailability(item.id) && item.region !== 'excluded';
}
The second example reduces cognitive load by breaking complex conditions into named functions and using a pipeline pattern that’s easier to follow.
Balancing Automation and Human Judgment
The key to truly readable code lies in finding the right balance between automated formatting tools and human judgment. Here’s how to strike that balance:
1. Use Formatters as a Foundation, Not a Complete Solution
Automated formatters provide a solid baseline for visual consistency. Use them to handle the mechanical aspects of formatting so your team can focus on higher-level readability concerns:
- Adopt a standard formatter for your project
- Configure it to match your team’s preferences
- Integrate it into your build process
- But recognize its limitations
2. Supplement with Linting Rules for Semantic Checks
While basic formatters focus on whitespace and syntax, linters can enforce some semantic rules:
- Variable naming conventions
- Function complexity limits
- Prohibited patterns
- Dead code detection
Tools like ESLint for JavaScript, Pylint for Python, or RuboCop for Ruby can help catch some semantic issues that basic formatters miss.
3. Establish Team Guidelines for What Tools Can’t Check
Create explicit team guidelines for aspects of readability that tools can’t verify:
- Naming conventions with examples
- Code organization principles
- Documentation requirements
- Abstraction principles (when to create new functions, classes, etc.)
These human-enforced guidelines are crucial for aspects of readability that require judgment and context.
4. Use Code Reviews to Enforce Readability
Code reviews provide the human judgment layer that automated tools lack. Train your team to evaluate code not just for correctness but for readability:
- “Would a new team member understand this?”
- “Will this be clear six months from now?”
- “Could this be structured more clearly?”
- “Do the names clearly convey intent?”
The best teams view readability feedback as valuable rather than nitpicking.
Practical Strategies for Truly Readable Code
Beyond understanding the limitations of formatting tools, here are practical strategies to create genuinely readable code:
1. Write Code for the Reader, Not the Computer
Computers will execute any syntactically correct code, regardless of how it’s structured. The true audience for your code is other developers (including your future self).
Before committing code, ask yourself: “If someone who’s never seen this project before reads this code, will they understand what it does and why?”
2. Tell a Story with Your Code
Well-written code reads like a story, with a clear beginning, middle, and end. Each function should have a single, clear purpose that’s evident from its name and structure.
async function processOrderCheckout(cart, user) {
// Beginning: Validation and preparation
validateCartItems(cart);
const shippingAddress = getShippingAddress(user);
// Middle: Core business logic
const order = createOrderFromCart(cart, user);
const paymentResult = await processPayment(order, user.paymentMethods);
// End: Finalization and side effects
if (paymentResult.success) {
sendOrderConfirmation(order, user.email);
updateInventory(cart.items);
clearUserCart(user.id);
}
return { order, paymentResult };
}
This code tells a clear story of the checkout process from beginning to end.
3. Optimize for the Common Case
Structure your code to make the most common path through it the most readable. Edge cases and error handling are important, but they shouldn’t obscure the primary purpose of the code.
// Hard to follow the main flow
function processOrder(order) {
if (!order) {
throw new Error('Order is required');
}
if (!order.items || order.items.length === 0) {
throw new Error('Order must have items');
}
if (!order.customerId) {
throw new Error('Order must have a customer ID');
}
// The actual business logic is buried under validation
const total = calculateTotal(order.items);
applyDiscounts(order, total);
chargeCustomer(order.customerId, total);
// More business logic...
}
// Main flow is clear, validation is separated
function processOrder(order) {
validateOrder(order);
const total = calculateTotal(order.items);
applyDiscounts(order, total);
chargeCustomer(order.customerId, total);
// More business logic...
}
function validateOrder(order) {
if (!order) {
throw new Error('Order is required');
}
if (!order.items || order.items.length === 0) {
throw new Error('Order must have items');
}
if (!order.customerId) {
throw new Error('Order must have a customer ID');
}
}
The second example makes the main flow more apparent by separating validation concerns.
4. Use Consistent Patterns
Consistency is a powerful tool for readability. When patterns are consistent, developers can focus on what the code does rather than how it’s structured.
For example, if you’re working with React components, adopt a consistent structure:
// A consistent component pattern
function UserProfile({ user }) {
// 1. Hooks
const [isEditing, setIsEditing] = useState(false);
// 2. Derived state
const fullName = `${user.firstName} ${user.lastName}`;
// 3. Event handlers
function handleEditClick() {
setIsEditing(true);
}
// 4. Render helpers
function renderActionButtons() {
return (
<div className="actions">
{isEditing ? (
<SaveButton onClick={handleSave} />
) : (
<EditButton onClick={handleEditClick} />
)}
</div>
);
}
// 5. Main render
return (
<div className="user-profile">
<h2>{fullName}</h2>
<div className="user-details">
{/* Details here */}
</div>
{renderActionButtons()}
</div>
);
}
When all components follow this pattern, developers immediately know where to look for specific aspects of the component.
5. Refactor for Readability
Readability isn’t just for new code. Regularly refactoring existing code to improve readability pays dividends in maintenance costs:
- Schedule regular refactoring sessions
- Improve readability when fixing bugs
- Apply the “boy scout rule”: leave code cleaner than you found it
Even small improvements accumulate over time to create more maintainable codebases.
Team Approaches to Code Readability
Readability is a team effort. Here’s how to build a team culture that values and enforces readable code:
1. Create a Readability Guide
Develop a team-specific readability guide that goes beyond basic formatting rules. Include:
- Naming conventions with examples
- Code organization principles
- Documentation expectations
- Examples of before/after refactoring for readability
This guide should evolve with your team and codebase.
2. Conduct Readability-Focused Code Reviews
Train team members to evaluate code specifically for readability during reviews. Questions to consider:
- Is the purpose of this code immediately clear?
- Are names descriptive and consistent?
- Is the code structured in a way that tells a clear story?
- Are complex operations broken down appropriately?
- Would a new team member understand this code?
3. Pair on Readability Improvements
Pair programming sessions specifically focused on improving readability can be highly effective:
- One developer explains the code to another
- Points of confusion indicate readability issues
- Both developers collaborate on improvements
This approach leverages the fact that explaining code to others is a powerful way to identify readability issues.
4. Measure and Reward Readability
Consider making readability a measurable and rewarded aspect of code quality:
- Track readability feedback in code reviews
- Recognize developers who consistently write readable code
- Include readability in performance evaluations
What gets measured and rewarded gets done.
The Future of Code Readability Tools
While current formatting tools have limitations, the future looks promising for more advanced readability assistance:
1. AI-Assisted Semantic Analysis
Machine learning models like those powering GitHub Copilot are beginning to understand code semantics, not just syntax. Future tools might:
- Suggest more descriptive variable names based on usage
- Identify functions that could be split for clarity
- Recommend structural improvements based on best practices
2. Cognitive Complexity Analysis
Beyond simple metrics like cyclomatic complexity, future tools might evaluate how difficult code is for humans to understand:
- Identifying code that requires tracking too many concepts simultaneously
- Suggesting restructuring to reduce cognitive load
- Visualizing complexity in ways that highlight problematic areas
3. Context-Aware Formatting
Future formatters might be smart enough to adapt their rules based on context:
- Preserving tabular alignment when it enhances readability
- Applying different formatting rules to different code patterns
- Learning from a team’s preferences and patterns
4. Interactive Readability Tools
Rather than just applying fixed rules, future tools might work interactively with developers:
- Suggesting alternative ways to structure code
- Providing readability scores with specific improvement suggestions
- Allowing developers to explore different organization options
Conclusion
Code formatting tools provide a valuable foundation for consistent code style, but they address only the surface-level aspects of readability. True readability requires human judgment, semantic understanding, and a focus on how humans comprehend code.
To create genuinely readable code:
- Use formatting tools for what they’re good at: enforcing consistent visual style
- Focus on meaningful naming, appropriate abstraction, and clear structure
- Consider the cognitive aspects of how humans understand code
- Build team practices that value and enforce readability
- Recognize that readability is an ongoing process, not a one-time fix
Remember that the goal of readable code isn’t just aesthetic satisfaction—it’s reduced bugs, faster onboarding, more efficient maintenance, and ultimately, a more productive and happier development team.
By moving beyond superficial formatting to focus on what truly makes code comprehensible to humans, you’ll create codebases that remain maintainable and extendable for years to come.
What readability practices have you found most effective in your team? How do you balance automated tools with human judgment? Share your experiences and continue the conversation about creating truly readable code.