Why Your Code Comments Aren’t Helping Other Developers
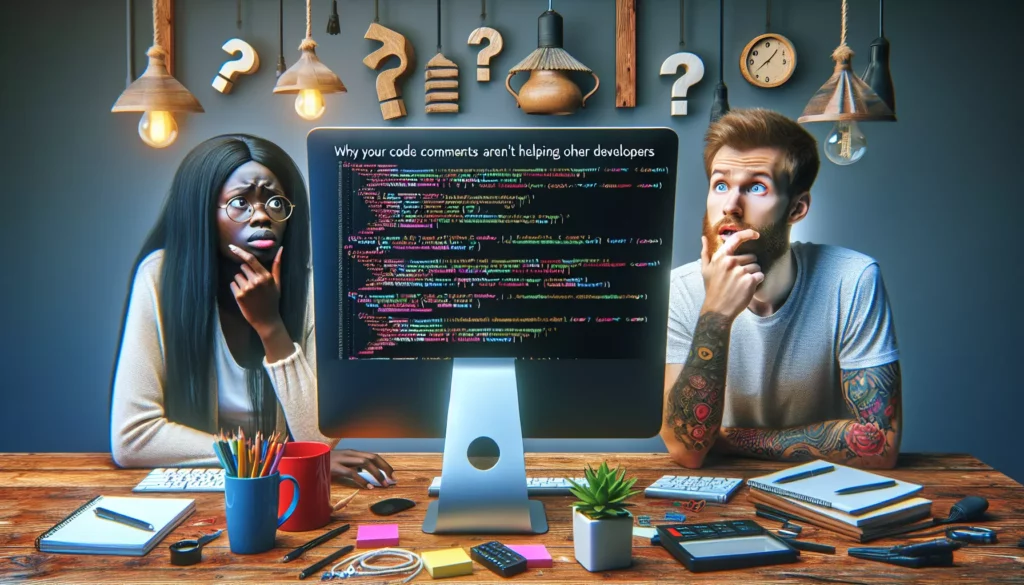
If you’ve ever inherited a codebase from another developer, you know the feeling: diving into unfamiliar code, trying to understand what’s happening, and suddenly encountering comments that leave you more confused than before. Despite our best intentions, many of the comments we write fail to serve their purpose—helping other developers understand our code.
In this article, we’ll explore why many code comments fall short, how to identify unhelpful commenting patterns, and most importantly, how to write comments that genuinely improve code comprehension for your team and future developers (including your future self).
The False Promise of “Self-Documenting Code”
Before diving into comment issues, let’s address a common misconception: the myth of “self-documenting code.” Some developers argue that with clean code practices, meaningful variable names, and clear function signatures, comments become unnecessary.
While clean code certainly reduces the need for explanatory comments, it’s rarely sufficient on its own. Even the most elegant code can’t explain:
- Why a particular algorithm or approach was chosen over alternatives
- Business context or domain-specific reasoning
- Workarounds for external system limitations
- Historical decisions that shaped the implementation
- Non-obvious edge cases the code handles
Good comments complement good code—they don’t compete with it. The goal isn’t to eliminate comments but to make them meaningful and valuable.
The Most Common Unhelpful Comment Types
Let’s examine the types of comments that frequently fail to help other developers:
1. The “Captain Obvious” Comment
These comments restate what the code clearly shows, adding no additional information:
// Increment counter
counter++;
// Check if user exists
if (user != null) {
// ...
}
These comments create noise without adding value. They can be particularly frustrating in codebases where meaningful comments are mixed with obvious ones, training developers to ignore all comments.
2. The Outdated Comment
One of the most dangerous comment types is the outdated comment—one that no longer reflects what the code actually does:
// Returns user's full name
public String getUserIdentifier() {
return user.getEmail(); // Code changed, but comment wasn't updated
}
These comments actively mislead developers and can cause significant confusion. They violate the principle of “Don’t Repeat Yourself” (DRY) by creating multiple sources of truth that easily fall out of sync.
3. The Cryptic Abbreviation
Comments filled with unexplained abbreviations or domain-specific jargon can be impenetrable:
// Apply FISC reg for KYC proc
validateCustomerIdentity();
// Impl NBT algo from 2018 RFC
calculateOptimalPath();
Unless all team members are familiar with these abbreviations, such comments create barriers to understanding rather than removing them.
4. The Comment That Tries Too Hard
Some developers attempt to be clever or humorous in their comments:
// I'm sorry, Dave, I'm afraid I can't do that
if (!userHasPermission()) {
throw new AccessDeniedException();
}
// Here be dragons!!!
public void processLegacyData() {
// ...
}
While occasionally amusing, these comments often fail to communicate useful information and can seem unprofessional in a team environment.
5. The Commented-Out Code
Perhaps the most common form of comment clutter is commented-out code:
// We used to do it this way
// for (int i = 0; i < users.size(); i++) {
// User user = users.get(i);
// processUser(user);
// }
// Now we do it this way
users.forEach(this::processUser);
This pattern creates several problems:
- It’s unclear why the code was commented out
- No one knows if it’s safe to delete
- It clutters the codebase with potentially outdated approaches
- Version control already preserves the history, making this redundant
6. The Apologetic Comment
Comments that acknowledge poor code without fixing it:
// Sorry, this is a bit of a hack but it works
// TODO: Clean this up when we have time
public void processData() {
// 50 lines of convoluted code...
}
These comments signal technical debt but rarely lead to improvements. They often serve more as emotional release for the original developer than as helpful information for others.
Why Bad Comments Persist
Understanding why developers continue to write unhelpful comments can help us address the root causes:
Misunderstanding the Purpose of Comments
Many developers see comments primarily as a way to explain what the code does, rather than why it does it. This fundamental misunderstanding leads to redundant comments that restate the code.
Institutional Requirements
Some organizations mandate comments for all functions or code blocks, leading to perfunctory comments written to satisfy a requirement rather than to communicate valuable information.
Commenting as an Afterthought
When comments are added after code is written (especially long after), they tend to be less thoughtful and insightful than those written alongside the code as part of the problem-solving process.
Fear of Deleting Information
Developers often hesitate to remove commented-out code or outdated comments for fear of losing valuable information, not realizing that version control systems already preserve this history.
The True Purpose of Comments
Before we can write better comments, we need to understand what makes a comment valuable. Good comments serve specific purposes:
1. Explaining the “Why” Not the “What”
The code itself tells you what it does. Comments should explain why:
// Using a timeout of 5 seconds because the external API
// occasionally stalls on large payloads
client.setTimeout(5000);
2. Providing Context and Background
Comments can provide essential context that isn’t apparent from the code:
// This calculation follows the tax regulations from
// IRS Publication 590-B (2022), Appendix A
double requiredDistribution = calculateRMD(age, accountBalance);
3. Warning About Non-Obvious Consequences
Good comments alert others to potential pitfalls:
// This operation locks the database connection
// and should not be called from the UI thread
void performBulkUpdate() {
// ...
}
4. Explaining Complex Algorithms
For complex algorithms, comments can provide a high-level overview that helps developers understand the approach before diving into details:
/**
* Implements Dijkstra's algorithm to find shortest paths.
* 1. Initialize distances for all nodes to infinity
* 2. Set distance to start node as 0
* 3. While unvisited nodes remain:
* a. Select node with smallest distance
* b. Update distances to all adjacent nodes
* c. Mark current node as visited
*/
public Map<Node, Integer> findShortestPaths(Node start) {
// Implementation follows...
}
Writing Comments That Actually Help
Now that we understand what makes comments valuable, let’s explore practical strategies for writing helpful comments:
Focus on Intent and Reasoning
The most valuable comments explain the developer’s intent and reasoning:
// Using exponential backoff to prevent overwhelming the service
// during recovery from failure
public void retryOperation() {
int delay = INITIAL_DELAY;
while (attempts < MAX_ATTEMPTS) {
try {
performOperation();
return;
} catch (ServiceException e) {
Thread.sleep(delay);
delay *= 2; // Exponential increase
attempts++;
}
}
}
Document Constraints and Assumptions
Make implicit constraints explicit:
/**
* Processes the customer order.
*
* @param order Must not be null and must have at least one item
* @throws IllegalArgumentException if order total exceeds $10,000
* (requires manager approval)
*/
public void processOrder(Order order) {
// Implementation...
}
Explain Business Rules and Domain Knowledge
Domain-specific logic often benefits from explanatory comments:
// Premium customers (tier > 2) are exempt from daily
// transaction limits as per policy updated Jan 2023
if (customer.getTier() <= 2 && dailyTransactions > DAILY_LIMIT) {
throw new LimitExceededException();
}
Use Comments to Guide Future Maintenance
Well-placed comments can help future developers understand how to properly maintain the code:
// The order of these operations is critical:
// 1. Lock the record
// 2. Validate the changes
// 3. Apply the updates
// 4. Release the lock
// Changing this sequence may result in data corruption
Make Strategic Use of TODO Comments
TODO comments can be valuable when they are specific and actionable:
// TODO: Replace this O(n²) algorithm with the O(n log n)
// approach described in ticket PERF-123 once the
// dependency on legacy sorting is removed
Use Standard Documentation Formats
For public APIs and libraries, use standardized documentation formats like JavaDoc, JSDoc, or docstrings:
/**
* Calculates the optimal route between two points.
*
* @param start The starting location coordinates
* @param end The destination coordinates
* @param preferences User preferences affecting route selection
* @return A Route object containing waypoints and estimated time
* @throws NoRouteFoundException if no valid route exists
*/
public Route calculateRoute(Point start, Point end,
RoutePreferences preferences) {
// Implementation...
}
Comments in Different Programming Paradigms
The nature of helpful comments varies across programming paradigms:
Object-Oriented Programming
In OOP, comments should focus on:
- Class responsibilities and relationships
- Design patterns being applied
- Inheritance and interface contract details
- Invariants that must be maintained
/**
* Implements the Observer pattern to notify registered listeners
* when inventory levels change.
*
* This class is not thread-safe and should only be accessed
* from the UI thread.
*/
public class InventoryManager implements Subject {
// Implementation...
}
Functional Programming
In functional programming, valuable comments often explain:
- Function composition patterns
- Side effect boundaries
- Recursion strategies
- Higher-order function usage
// This fold operation accumulates a running total while
// preserving the original collection structure
const processedData = data.reduce((acc, item) => {
// Pure function with no side effects
return {
...acc,
total: acc.total + item.value,
items: [...acc.items, transform(item)]
};
}, { total: 0, items: [] });
Procedural Programming
In procedural code, focus on:
- Data flow between functions
- State mutations and their scope
- Procedure sequencing requirements
// This function modifies the global application state
// and should only be called after initializeSystem()
void configureSettings(const Settings* settings) {
// Implementation...
}
Comments for Different Audiences
Consider who will be reading your comments:
For Team Members
Focus on business context, recent changes, and cross-functional impacts:
// This validation was added after the security audit in March
// It addresses vulnerability CVE-2023-1234 by preventing
// path traversal attacks
For Your Future Self
Document complex decisions and reasoning that might not be obvious when you revisit the code months later:
// Chose to use a custom implementation rather than the library
// because our data structure requires O(1) lookup and O(1) insertion,
// which the standard library couldn't provide without compromises
For API Consumers
Focus on usage patterns, requirements, and limitations:
/**
* Returns weather forecast data for the specified location.
*
* Rate limited to 100 requests per minute per API key.
* Coordinates should be in decimal degrees (e.g., 37.7749, -122.4194).
*
* @param latitude Decimal latitude between -90 and 90
* @param longitude Decimal longitude between -180 and 180
* @return Forecast object containing hourly and daily predictions
*/
When to Remove Comments
Just as important as writing good comments is removing unhelpful ones:
Remove Comments When Refactoring Makes Them Unnecessary
If you can refactor code to make its purpose self-evident, the comment may become redundant:
Before:
// Check if user is eligible for discount
if (user.getOrderCount() > 5 && user.getTotalSpend() > 500
&& !user.hasActiveDiscount()) {
applyDiscount(user);
}
After:
if (isEligibleForDiscount(user)) {
applyDiscount(user);
}
private boolean isEligibleForDiscount(User user) {
return user.getOrderCount() > 5
&& user.getTotalSpend() > 500
&& !user.hasActiveDiscount();
}
Delete Commented-Out Code
Trust your version control system to preserve history. If code is no longer needed, remove it completely rather than commenting it out.
Consolidate Redundant Comments
If you find multiple comments saying similar things, consider consolidating them into a single, more comprehensive comment.
Tools and Practices for Better Comments
Several tools and team practices can help improve comment quality:
Comment Linters
Tools like ESLint (JavaScript), PyLint (Python), and Checkstyle (Java) can enforce comment conventions and flag potentially problematic patterns.
Documentation Generators
Tools that generate documentation from code comments (like JavaDoc, JSDoc, Sphinx) encourage structured, comprehensive comments for public APIs.
Code Review Focus
Make comment quality a specific focus area in code reviews. Ask questions like:
- Do these comments explain why, not just what?
- Is there any domain knowledge that should be captured in comments?
- Are there any comments that have become outdated or redundant?
Comment Refactoring
Just as code benefits from periodic refactoring, so do comments. Schedule occasional sessions to review and improve comments throughout the codebase.
Real-World Examples: Before and After
Let’s look at some real-world examples of comment transformations:
Example 1: From Obvious to Insightful
Before:
// Loop through users
for (User user : users) {
// Process each user
processUser(user);
}
After:
// Processing users sequentially rather than in parallel
// to avoid overwhelming the authentication service
// See incident report INC-2023-07-15 for details
for (User user : users) {
processUser(user);
}
Example 2: From Cryptic to Clear
Before:
// Apply DT proc
calculateValue(user, 0.15);
After:
// Apply 15% discount for first-time customers
// (Marketing promotion "Welcome2023")
calculateValue(user, FIRST_TIME_CUSTOMER_DISCOUNT_RATE);
Example 3: From Apologetic to Actionable
Before:
// This is kind of a mess, sorry!
// TODO: Fix this someday
function handleUserData(data) {
// 50 lines of convoluted code...
}
After:
// Technical debt: This function handles three distinct responsibilities:
// 1. Data validation
// 2. User record updating
// 3. Notification dispatching
// TODO: Refactor into separate functions (JIRA: REFACTOR-123)
function handleUserData(data) {
// Same implementation, but with clear sections and additional
// explanatory comments for complex parts
}
Learning From Open Source
Open source projects often provide excellent examples of comment practices:
Linux Kernel
The Linux kernel maintains high comment quality with detailed explanations of complex algorithms, hardware interactions, and historical context. For example, from the scheduler code:
/*
* Scheduler load-balancing algorithm:
*
* The basic concept is to average the load across all CPUs, so that no
* CPU has more than the average. The imbalance is the amount of load that
* should be moved to bring a CPU to this average.
*
* Balancing within a CPU group is more urgent than balancing across groups.
*
* The load-balance algorithm is as follows:
*
* 1) Iterate from highest to lowest priority group
* a) For each CPU in that group:
* i) Calculate the CPU's load
* ii) If the load exceeds the average load of the group by a
* configurable amount, mark the CPU as overloaded
* iii) Look for a recipient CPU in the same group that is not
* overloaded
* iv) If a suitable recipient was found, move tasks to balance
* the load
* ...
*/
React
React’s codebase uses comments to explain complex state management and rendering optimizations:
// This is a bit of a hack.
// Rather than force render an update by clearing all pending updates
// (which might include updates from other inputs), we can clear only
// the pending update for this input.
// This is useful when, e.g., the user has typed a character, then
// clicked outside of the input box without hitting "enter" or "tab".
// In this case, we want to revert to the previous value.
// See https://github.com/facebook/react/issues/8188
PostgreSQL
PostgreSQL’s codebase extensively documents both implementation details and the underlying database theory:
/*
* Bloom filter is a probabilistic data structure that tests membership of
* an element in a set. A bloom filter parameter is the acceptable false
* positive rate. The implementation details of a bloom filter (number of
* bits and number of hash functions to use) can be derived from the maximum
* number of elements that can be inserted and the acceptable false positive
* rate.
*
* The bloom filter implementation in Postgres uses a variant of bloom
* filter called blocked bloom filter, which has better CPU cache
* efficiency. A blocked bloom filter divides the bloom filter into
* equal-sized blocks, where each block is of the size of one CPU cache
* line.
*/
Cultural Aspects of Code Comments
The quality of comments often reflects team culture:
Encouraging Knowledge Sharing
Comments should be seen as a knowledge-sharing tool. Teams that value knowledge transfer typically write more thorough, contextual comments.
Code Ownership vs. Collective Responsibility
Teams practicing collective code ownership tend to write more detailed comments, recognizing that any team member might need to understand and modify the code.
Comment-Driven Development
Some teams practice a form of “comment-driven development,” writing high-level comments before implementing code. This approach can lead to more thoughtful design and better documentation.
// First, we'll validate the input parameters
// Then we'll check if the user has sufficient permissions
// Next, we'll apply the business rules for order processing
// Finally, we'll record the transaction and return the result
// Now let's implement each step...
Conclusion: Towards a Comment Culture That Works
Effective code comments don’t happen by accident—they’re the result of intentional practices and a team culture that values clear communication. By focusing on the “why” behind code rather than the “what,” we can create comments that genuinely help other developers navigate and maintain our code.
Remember these key principles:
- Good comments explain why, not what
- Comments should provide context that code alone cannot express
- Keep comments updated when code changes
- Remove comments that no longer add value
- Use comments to share domain knowledge and design decisions
By improving our commenting practices, we’re not just making code easier to understand—we’re building a more collaborative, knowledge-sharing development culture that benefits everyone on the team.
The next time you write a comment, ask yourself: “Will this genuinely help another developer understand my code better than the code alone?” If the answer is yes, you’re on the right track.
Further Reading
- “Clean Code: A Handbook of Agile Software Craftsmanship” by Robert C. Martin
- “Code Complete” by Steve McConnell
- “The Art of Readable Code” by Dustin Boswell and Trevor Foucher
- “Write the Docs” community guidelines (writethedocs.org)