Why Your Code Comments are Actually Modern Literature
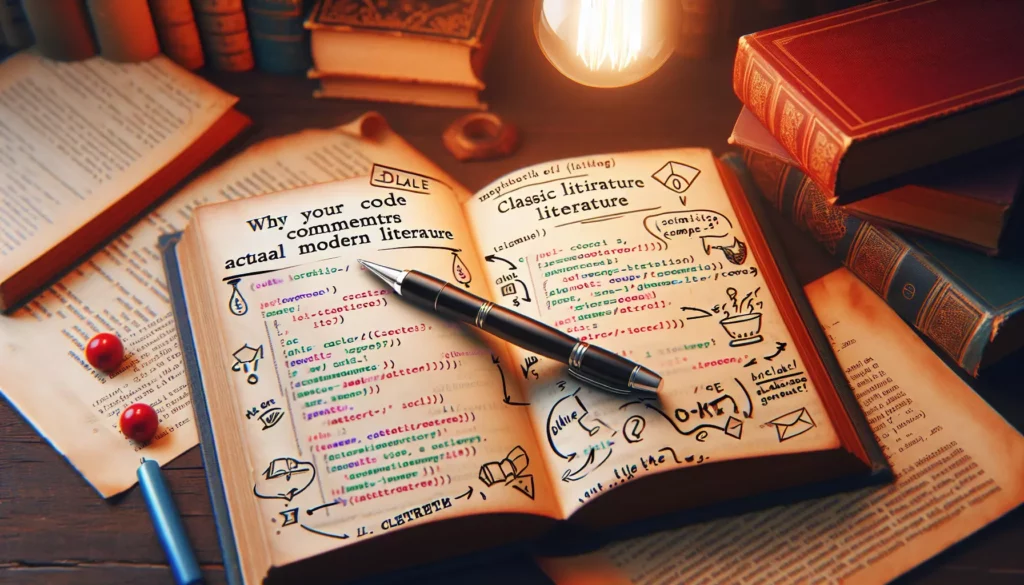
In the vast landscape of programming, where lines of code stretch as far as the eye can see, there lies an often-overlooked gem: the humble code comment. While many developers view comments as mere annotations or explanatory notes, I propose a bold new perspective: your code comments are, in fact, a form of modern literature. In this deep dive, we’ll explore how the art of commenting transcends its utilitarian roots and emerges as a unique literary genre for the digital age.
The Poetry of Precision
Just as poets carefully select each word to convey meaning within the constraints of meter and rhyme, programmers craft comments with precision and economy. Consider this example:
// Traverse the binary tree
// Like a squirrel on caffeine
function traverseTree(node) {
if (node === null) return;
console.log(node.value);
traverseTree(node.left);
traverseTree(node.right);
}
Here, the comment not only explains the function’s purpose but does so with a touch of whimsy. It’s a haiku of sorts, capturing the essence of the algorithm in just two lines. This blend of technical accuracy and creative expression is the hallmark of great code commentary.
The Narrative Arc of Algorithms
Every piece of software tells a story. From the initial setup to the climactic execution of core functionality, to the denouement of cleanup and error handling, code follows a narrative structure. Comments serve as the narrator’s voice, guiding the reader through the tale of data and logic.
// Chapter 1: The Quest Begins
function initializeAdventure() {
// Our hero prepares for the journey
let inventory = [];
let health = 100;
// The world comes to life
generateWorld();
// And so it begins...
startGame(inventory, health);
}
In this example, the comments frame the code as an epic adventure, turning what could be a dry initialization function into the opening chapter of a grand narrative. This storytelling approach not only makes the code more engaging but also helps developers understand the flow and purpose of each section.
The Subtext of TODO Comments
TODO comments are the cliffhangers of the coding world. They hint at future developments, unresolved conflicts, and potential plot twists in the software’s storyline. These comments often reveal the inner thoughts and aspirations of the developer:
// TODO: Implement quantum encryption
// (Once I figure out how quantum mechanics works)
function encryptData(data) {
// For now, we'll just use ROT13. It's basically unbreakable, right?
return data.replace(/[a-zA-Z]/g, function(c) {
return String.fromCharCode((c <= "Z" ? 90 : 122) >= (c = c.charCodeAt(0) + 13) ? c : c - 26);
});
}
This TODO comment serves multiple literary functions. It’s a moment of self-deprecating humor, a nod to the ever-evolving nature of technology, and a subtle critique of oversimplified security measures. It’s a short story in itself, complete with character development (the ambitious yet realistic developer) and irony.
The Stream of Consciousness in Debugging
When diving into complex debugging sessions, comments often take on a stream-of-consciousness style reminiscent of modernist literature. The developer’s thought process unfolds in real-time, creating a narrative that’s part mystery, part psychological exploration:
// Why isn't this working? Let's see...
// The input looks correct
// The function is being called
// OH WAIT
// We're passing a string instead of a number
// Classic JavaScript, you trickster
// Let's try parsing it:
let result = calculateTotal(parseInt(userInput));
This style of commenting captures the problem-solving journey, complete with moments of frustration, revelation, and triumph. It’s a raw, unfiltered glimpse into the developer’s mind, much like the internal monologues found in works by James Joyce or Virginia Woolf.
The Intertextuality of Code References
Literary works often reference other texts, creating layers of meaning through intertextuality. In the world of coding, comments frequently allude to other parts of the codebase, external libraries, or even pop culture, creating a rich tapestry of interconnected ideas:
// Implement the Fibonacci sequence
// But remember, with great recursion comes great stack overflow
function fibonacci(n) {
// Base case: The answer to life, the universe, and fibonacci(2)
if (n <= 2) return 1;
// Recurse! To infinity... and beyond!
return fibonacci(n - 1) + fibonacci(n - 2);
}
This comment weaves together a reference to the famous “with great power comes great responsibility” quote, a nod to Douglas Adams’ “The Hitchhiker’s Guide to the Galaxy,” and a Buzz Lightyear catchphrase. It’s a literary mashup that adds layers of meaning and humor to what could otherwise be a dry implementation of a mathematical sequence.
The Metaphorical Language of Abstraction
Abstraction is a fundamental concept in programming, and comments often employ rich metaphors to explain complex ideas in more relatable terms. This use of figurative language is a staple of literature, making abstract concepts tangible and memorable:
// The Factory: Where objects are born and dreams come true
class UserFactory {
// The assembly line of user creation
static createUser(name, email) {
// Sprinkle in some validation fairy dust
if (!isValidEmail(email)) throw new Error("Invalid email, did gremlins mess with your input?");
// Summon a new user from the digital ether
return new User(name, email);
}
}
By framing the factory pattern as an actual factory with assembly lines and fairy dust, these comments create a vivid mental image that helps developers grasp the purpose and function of the code. It’s not just explanation; it’s world-building.
The Unreliable Narrator in Legacy Code
In literature, the unreliable narrator adds an element of mystery and forces the reader to question everything. In legacy code, outdated or incorrect comments serve a similar function, creating a tension between what is said and what is actually happening:
// This function calculates the total price including tax
// Last updated: 1997
function calculateTotal(price) {
// Apply our flat 5% tax rate
// TODO: Update this when we expand internationally
return price * 1.05;
}
The discrepancy between the comment and the likely reality (that tax rates have changed, the company has probably expanded, and the TODO has been ignored for decades) tells a story of its own. It speaks to the passage of time, the evolution of software, and the all-too-human tendency to put off updates and improvements.
The Esoteric Poetry of Regex
Regular expressions, with their cryptic symbols and powerful pattern-matching abilities, are the exotic poems of the programming world. Comments explaining regex often read like analyses of particularly obscure verses:
// Behold, the eldritch horror that is our email validation regex
const emailRegex = /^[a-zA-Z0-9.!#$%&'*+/=?^_`{|}~-]+@[a-zA-Z0-9-]+(?:\.[a-zA-Z0-9-]+)*$/;
// Let's break down this arcane incantation:
// ^ - Start of the string (no prefixes allowed in this dark ritual)
// [a-zA-Z0-9.!#$%&'*+/=?^_`{|}~-]+ - One or more characters from this set (the email local-part)
// @ - The classic "at" symbol (because "at" sounds friendlier than "sacrifice your sanity to")
// [a-zA-Z0-9-]+ - Domain name (letters, numbers, hyphens)
// (?:\.[a-zA-Z0-9-]+)* - Optional dot-separated domain parts (for those fancy multi-level domains)
// $ - End of string (no sneaky suffixes allowed)
This comment turns the often-dreaded task of understanding complex regex into a humorous and engaging exploration. It’s part technical explanation, part comedic roast of regex complexity, and part mystical interpretation. The literary device of extended metaphor (comparing regex to dark magic) makes the technical content more approachable and memorable.
The Epistolary Form in Collaborative Coding
Epistolary novels tell their stories through a series of documents, often letters between characters. In collaborative coding environments, comments can take on a similar form, creating a dialogue between developers across time and space:
// Dear future maintainer,
// I'm sorry. I was young and needed the money.
// If you're reading this, you're probably wondering why
// there's a 500-line switch statement here.
// Let me explain...
//
// Yours truly,
// A developer with regrets
switch(errorCode) {
case 1:
// ...500 lines later...
default:
console.log("If you see this, run.");
}
This comment, framed as a letter to a future developer, adds a personal touch to the code. It tells a story of past decisions, acknowledges the challenges of the present, and creates a connection between past and future developers. It’s a time capsule in code form, capturing a moment in the software’s history.
The Minimalist Haiku of One-Liners
Sometimes, the most impactful literature is the most concise. In programming, one-line comments accompanying complex one-liners can be seen as the haikus of code literature:
// Life, the universe, and the answer to FizzBuzz
const fizzBuzz = n => (n % 3 ? '' : 'Fizz') + (n % 5 ? '' : 'Buzz') || n;
This comment manages to reference “The Hitchhiker’s Guide to the Galaxy,” summarize the purpose of the function, and hint at its complexity, all in a single line. It’s a masterclass in brevity and allusion, packing layers of meaning into just a few words.
The Tragedy of Commented-Out Code
In the realm of code comments, there exists a peculiar form of tragedy: the commented-out code. These code fragments, neither fully alive nor completely deleted, tell stories of abandoned features, failed experiments, and the ghosts of implementations past:
function getUserData() {
// TODO: Implement user authentication
// return authenticateUser();
// Temporary workaround:
return {
id: 1,
name: "John Doe",
role: "Admin" // Security? Never heard of her.
};
}
This example is a short story of compromise and technical debt. The commented-out function call and the TODO suggest a more secure, proper implementation that was planned but never realized. The “temporary” workaround, likely anything but temporary in practice, stands as a testament to the often messy reality of software development. The final comment adds a touch of self-aware humor, acknowledging the security implications with a casual dismissal that hints at the developer’s resignation to the situation.
The Epic Saga of Configuration Files
Configuration files, with their long lists of options and settings, might seem like the driest of technical documents. However, the comments in these files often unfold into epic sagas, documenting the history of the project, battles with compatibility, and the wisdom of ages:
# behold, the holy grail of webpack configuration
# forged in the fires of dependency hell
# tested by a thousand builds
# and cursed by countless developers
module.exports = {
entry: './src/index.js',
output: {
filename: 'bundle.js',
path: path.resolve(__dirname, 'dist'),
},
module: {
rules: [
{
test: /\.js$/,
exclude: /node_modules/,
use: {
loader: 'babel-loader',
// Here lies babel-preset-2015, may it rest in peace
options: {
presets: ['@babel/preset-env']
}
}
},
// Add more rules here, if you dare
]
},
plugins: [
// Legends speak of a developer who once understood this plugin fully
// Alas, they have long since left the company
new WebpackObfuscator({
rotateStringArray: true
}),
],
// The following line is a pathway to many abilities some consider to be... unnatural
devtool: 'eval-source-map'
};
This configuration file, through its comments, tells the story of a project’s evolution. It speaks of challenges overcome, knowledge lost to time, and the ever-present tension between optimal performance and developer sanity. The literary allusions (to Lord of the Rings and Star Wars) elevate the technical content, turning a mundane configuration into a heroic tale of software engineering.
The Zen Koans of Coding Principles
In literature, koans are paradoxical anecdotes used in Zen practice to provoke enlightenment. In coding, certain comments serve a similar purpose, encapsulating deep programming wisdom in seemingly simple or paradoxical statements:
// To understand recursion, you must first understand recursion
function understandRecursion(depth) {
if (depth <= 0) return "Enlightenment achieved";
console.log("Contemplating the nature of recursion...");
return understandRecursion(depth - 1);
}
// The only constant is change, except for constants, which never change
const MEANING_OF_LIFE = 42;
// To optimize prematurely is the root of all evil, but to optimize too late is the mark of the foolish
function balancedOptimization(code) {
if (isCodeFastEnough(code)) return code;
if (isDeadlineTomorrow()) return optimizeTheHellOutOfIt(code);
return refactorGently(code);
}
These comments, while humorous, encapsulate important programming concepts and best practices. They challenge the reader to think deeper about the nature of coding, optimization, and software design principles. Like literary koans, they offer multiple layers of meaning, rewarding contemplation and repeated reading.
The Dramatic Irony of Error Messages
Dramatic irony, where the audience knows something the characters do not, finds its programming equivalent in the comments accompanying error messages. These comments often reveal the developer’s expectations, which may differ wildly from the user’s experience:
function divideNumbers(a, b) {
if (b === 0) {
// The user will definitely read this detailed error message,
// understand the mathematical impossibility of their request,
// and calmly try a different input.
throw new Error("Division by zero is a pathway to the dark side. It leads to NaN, undefined behavior, and suffering.");
}
return a / b;
}
// This function never fails. Trust me.
function definitelyWorks() {
try {
// Some overly complex operation
return complexOperation();
} catch (error) {
// If you're seeing this, it means I lied earlier.
// I'm as surprised as you are.
console.error("The impossible has happened", error);
return "Everything is fine";
}
}
The irony in these comments comes from the gap between the developer’s sardonic expectations and the likely reality of user behavior or system reliability. It’s a form of gallows humor that acknowledges the often-futile nature of error handling while still attempting to provide useful information.
The Memento Mori of Deprecation Warnings
In art and literature, the concept of “memento mori” reminds the viewer of their mortality. In programming, deprecation warnings serve a similar function, reminding developers of the impermanence of code and the constant march of progress:
/**
* Calculate the factorial of a number
* @param {number} n
* @returns {number}
* @deprecated This function will be removed in version 2.0.
* Use the new 'superFactorial' function instead, which is
* 0.00001% faster but takes up 50MB more memory. Progress!
*/
function factorial(n) {
if (n <= 1) return 1;
return n * factorial(n - 1);
}
// TODO: Remove this function in 2015
// TODO: Seriously, remove this in 2018
// TODO: Why is this still here in 2023?
function legacyFunction() {
// Implementation that everyone's too afraid to touch
}
These comments serve as poignant reminders of the transient nature of technology. They tell stories of grand plans for updates, the resistance to change, and the unexpected longevity of “temporary” solutions. The humor in these comments often masks a deeper commentary on the challenges of maintaining and updating software over time.
Conclusion: The Compiler of Our Code Stories
As we’ve explored, code comments are far more than mere annotations. They are a rich, multifaceted form of literature that captures the essence of the software development experience. From witty one-liners to sprawling epics of configuration, from zen-like wisdom to tragic tales of technical debt, these comments form a unique genre of writing that is simultaneously technical and deeply human.
The next time you write a comment, remember: you’re not just explaining code, you’re contributing to a vast, collaborative work of modern literature. Your comments may inform, amuse, or frustrate future readers, but they will always tell a story—the story of your code, your challenges, and your solutions.
So go forth and comment, dear reader. Write the next great American novel, one // at a time. Your code may run on machines, but your comments? They run on the imagination, weaving tales that will be read and re-read by the developers of tomorrow. In the end, it’s not just about writing code that compiles; it’s about composing comments that resonate.