Why Your Code Analysis Tools Aren’t Preventing Bad Practices
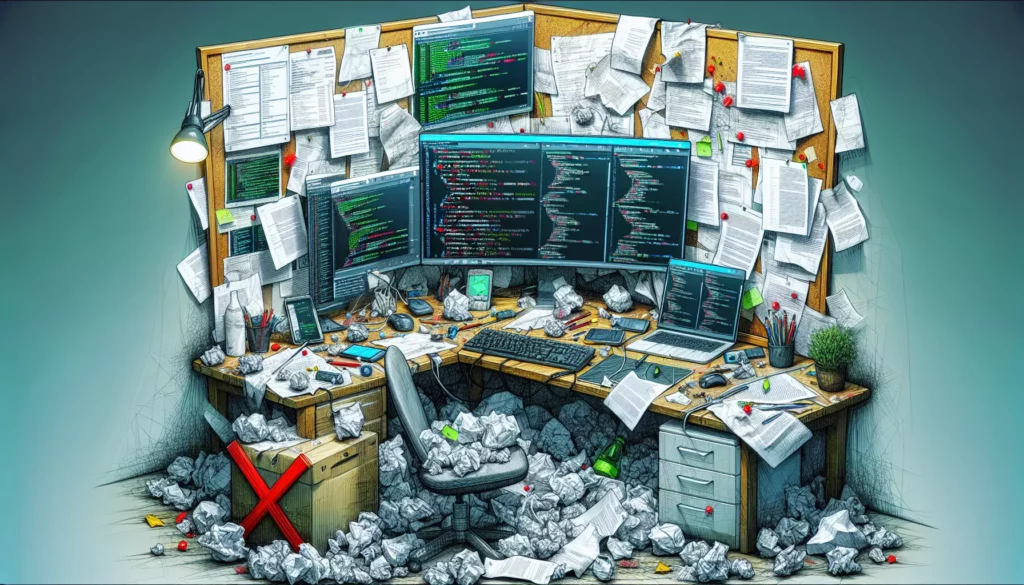
In the evolving landscape of software development, code analysis tools have become indispensable for maintaining code quality and catching potential issues before they make it to production. Yet, despite the sophisticated array of linters, static analyzers, and automated testing frameworks at our disposal, bad coding practices continue to persist in codebases across the industry.
This disconnect raises an important question: If our tools are so powerful, why aren’t they effectively preventing bad coding practices?
In this comprehensive guide, we’ll explore the limitations of code analysis tools, understand why they sometimes fail to catch problematic code, and learn strategies to enhance their effectiveness in your development workflow.
The Promise of Code Analysis Tools
Before diving into their shortcomings, let’s acknowledge what code analysis tools are designed to accomplish:
- Identify syntax errors and potential runtime bugs
- Enforce coding standards and style guidelines
- Detect security vulnerabilities and code smells
- Measure code quality metrics like complexity and maintainability
- Suggest optimizations and improvements
These tools have undoubtedly improved code quality across the industry. From simple linters like ESLint and Pylint to comprehensive static analysis platforms like SonarQube and sophisticated AI-powered assistants like GitHub Copilot, developers have never had more help available.
Yet the persistence of problematic code suggests these tools aren’t the complete solution many hoped they would be.
The Gap Between Theory and Practice
1. Tools Can Only Catch What They’re Programmed to Find
Code analysis tools operate based on predefined rules and patterns. They excel at identifying known issues but struggle with novel problems or context-specific bad practices.
Consider this JavaScript example:
// This will pass most linters without issue
function processData(data) {
if (data) {
// Hundreds of lines of complex logic
return result;
}
// Missing else clause with error handling
}
Many linters would miss the fact that this function has no error handling for the case when data is falsy. The code is syntactically correct and follows basic structure rules, but it may represent a logical error in your specific application context.
2. False Positives Lead to “Alert Fatigue”
When tools raise too many warnings or flag issues that developers consider unimportant, “alert fatigue” sets in. This phenomenon leads developers to:
- Ignore warnings entirely
- Add blanket suppressions or exceptions
- Disable certain rules project-wide
For example, in a React codebase:
// eslint-disable-next-line react-hooks/exhaustive-deps
useEffect(() => {
// Complex logic that intentionally doesn't include all dependencies
}, [dependency1]);
While sometimes these suppressions are justified, they often become a habit, undermining the tool’s effectiveness. Over time, important warnings get lost in the noise.
3. Focus on Syntax Over Semantics
Most code analysis tools excel at catching syntactic issues but struggle with semantic problems. They can tell you if your code is structured correctly but not necessarily if it does what it’s supposed to do.
Consider this Python example:
def calculate_average(numbers):
total = 0
for num in numbers:
total += num
return total / len(numbers) # Will crash on empty list
Syntactically, this function is fine. Most linters won’t flag it. But semantically, it has a critical flaw: it will raise a division by zero error if the input list is empty. This type of logical error requires a deeper understanding of the code’s purpose and expected inputs.
The Human Element: Why Developers Bypass Tools
1. Deadline Pressure
When facing tight deadlines, developers often make pragmatic choices that prioritize shipping over perfection. This might include:
- Ignoring non-critical warnings
- Adding “TODO” comments instead of fixing issues immediately
- Taking shortcuts that satisfy the tool’s requirements without addressing the underlying problem
The classic example is the “I’ll fix it later” comment:
// TODO: Refactor this. It's a quick fix for now because of the deadline
function quickAndDirtyFix() {
// Code that gets the job done but isn't ideal
}
2. Conflicting Priorities
Different stakeholders often have different priorities:
- Product managers want features shipped quickly
- Security teams want thorough vulnerability checks
- QA teams want comprehensive test coverage
- Developers want maintainable, elegant code
These competing interests create situations where bypassing tool recommendations seems like the rational choice to satisfy immediate business needs.
3. Tool Configuration Complexity
Many analysis tools require extensive configuration to be effective. When the setup process is too complex or time-consuming, teams may:
- Use default configurations that don’t match their project’s needs
- Configure tools too leniently to avoid disruption
- Skip certain types of analysis entirely
For example, setting up a comprehensive TypeScript configuration with strict type checking can be challenging:
{
"compilerOptions": {
"strict": true,
"noImplicitAny": true,
"strictNullChecks": true,
"strictFunctionTypes": true,
"strictBindCallApply": true,
"strictPropertyInitialization": true,
"noImplicitThis": true,
"alwaysStrict": true,
// Many more options...
}
}
Teams often opt for less strict configurations to avoid the initial pain of fixing all the issues that would be flagged by a more rigorous setup.
Technical Limitations of Analysis Tools
1. Static Analysis Constraints
Static analysis tools examine code without executing it, which inherently limits their capability to detect runtime issues. They make educated guesses about program behavior but can’t account for all possible execution paths or data values.
Consider this Java example:
public String getUserData(String userId) {
User user = userRepository.findById(userId);
return user.getName(); // Potential NullPointerException
}
A static analyzer might miss that this could throw a NullPointerException if the user isn’t found, especially if the repository’s interface doesn’t clearly indicate that it might return null.
2. Dynamic Language Challenges
Dynamically typed languages like JavaScript and Python present particular challenges for analysis tools. Without explicit type information, tools must make assumptions that can lead to both missed issues and false positives.
function processValue(value) {
return value.toString().trim();
}
// This could fail at runtime if value is null or undefined
// but many linters won't catch this without additional type hints
3. Distributed System Complexity
Modern applications often span multiple services, languages, and platforms. Code analysis tools typically focus on one codebase or language at a time, missing issues that arise from the interaction between components.
For example, a REST API might expect a specific format that isn’t enforced by the client code:
// Client code (JavaScript)
fetch('/api/users', {
method: 'POST',
body: JSON.stringify({ userName: user.name }) // Using camelCase
})
// Server code (Python)
@app.route('/api/users', methods=['POST'])
def create_user():
data = request.get_json()
# Expects snake_case: user_name, not userName
user_name = data.get('user_name')
# Potential bug due to naming convention mismatch
No single code analyzer would catch this cross-service inconsistency.
Common Bad Practices That Slip Through
Let’s examine some specific categories of bad practices that frequently evade detection by automated tools:
1. Poor Abstraction and Design
Tools struggle to identify high-level design issues like:
- Inappropriate abstractions
- Violation of SOLID principles
- Inconsistent levels of abstraction within a module
For example, a class that violates the Single Responsibility Principle:
class UserManager {
public void createUser(User user) { /* ... */ }
public void deleteUser(String userId) { /* ... */ }
public void sendEmail(String userId, String message) { /* ... */ }
public void generateReport(String format) { /* ... */ }
public void updateDatabaseSchema() { /* ... */ }
}
This class clearly does too many unrelated things, but most tools won’t flag this as an issue because it’s syntactically valid.
2. Implicit Knowledge Requirements
Code that requires domain knowledge or context not available to static analyzers often passes checks despite being problematic:
// Magic numbers with domain significance
if (status == 7) { // 7 means "processing" in this domain
startProcessing();
}
// Or undocumented requirements
function calculateTax(amount) {
// Assumes amount is already in cents, not dollars
return amount * 0.07;
}
3. Concurrency and Timing Issues
Race conditions, deadlocks, and other concurrency problems are notoriously difficult for static analyzers to detect:
// Potential race condition
public void updateCounter() {
int current = counter; // Read
current++; // Modify
counter = current; // Write
}
In a multi-threaded environment, this could lead to lost updates, but many static analyzers won’t flag it without specific concurrency-focused rules.
4. Maintainability Issues
Code that’s difficult to maintain but technically correct often passes analysis:
- Overly complex methods that are hard to understand
- Inconsistent naming conventions within semantic domains
- Copy-pasted code with minor variations
// Technically works but is a maintainability nightmare
function processTransaction(t) {
let a = t.a;
let r;
if (a > 1000) {
if (t.c == 'USD') {
r = a * 0.05;
if (t.d) {
r = r - (r * 0.1);
}
} else if (t.c == 'EUR') {
r = a * 0.04;
if (t.d && a > 5000) {
r = r - (r * 0.15);
}
}
// Many more nested conditions...
}
return r;
}
Bridging the Gap: Making Tools More Effective
While no tool can fully eliminate bad coding practices, several strategies can help bridge the gap between tool capabilities and real-world needs:
1. Customize and Contextualize
Generic rules often lead to generic results. Customize your analysis tools to match your project’s specific requirements:
- Create custom rules that enforce domain-specific best practices
- Adjust severity levels based on your team’s priorities
- Develop project-specific plugins for your linters
For example, a custom ESLint rule might enforce API-specific patterns:
// Custom ESLint rule to ensure proper error handling in API calls
{
"rules": {
"custom/api-error-handling": "error"
}
}
2. Integrate Analysis into the Development Workflow
Analysis tools are most effective when they provide immediate feedback:
- Configure IDE integrations for real-time feedback
- Set up pre-commit hooks to prevent problematic code from being committed
- Make CI/CD pipelines fail on critical issues
A sample pre-commit hook configuration:
# .pre-commit-config.yaml
repos:
- repo: https://github.com/pre-commit/pre-commit-hooks
rev: v4.4.0
hooks:
- id: trailing-whitespace
- id: end-of-file-fixer
- repo: https://github.com/pycqa/flake8
rev: 6.0.0
hooks:
- id: flake8
additional_dependencies: [flake8-docstrings]
3. Combine Multiple Analysis Approaches
Different types of analysis complement each other:
- Static analysis for syntax and known patterns
- Dynamic analysis through comprehensive testing
- Runtime monitoring to catch issues in production
- Manual code reviews for high-level concerns
A comprehensive approach might include:
# Static analysis with ESLint
npm run lint
# Unit tests with Jest
npm run test
# Integration tests with Cypress
npm run test:integration
# Property-based testing with fast-check
npm run test:properties
# Manual code review checklist
# - Does this code meet our design principles?
# - Is the abstraction level appropriate?
# - Are there hidden assumptions?
4. Educate and Build Culture
Tools are only as effective as the people using them:
- Invest in developer education about common pitfalls
- Explain the reasoning behind analysis rules
- Celebrate improvements in code quality
- Build a culture where quality is valued alongside speed
Consider implementing regular “code quality” workshops where team members can discuss specific examples from your codebase and how to improve them.
Advanced Strategies for Comprehensive Code Quality
1. Architectural Fitness Functions
Implement automated tests that verify your codebase adheres to architectural principles:
- Dependency direction checks
- Module boundary enforcement
- Layer isolation verification
Tools like ArchUnit for Java or dependency-cruiser for JavaScript can help:
// Using ArchUnit in Java to enforce layering
@Test
public void servicesShouldNotDependOnControllers() {
JavaClasses importedClasses = new ClassFileImporter().importPackages("com.myapp");
ArchRule rule = classes()
.that().resideInAPackage("..service..")
.should().notDependOnClassesThat().resideInAPackage("..controller..");
rule.check(importedClasses);
}
2. Property-Based Testing
Traditional unit tests check specific examples, but property-based testing verifies that code behaves correctly for all possible inputs within defined constraints.
This can catch edge cases that static analysis misses:
// Using fast-check in JavaScript
test('String reversal works for all strings', () => {
fc.assert(
fc.property(fc.string(), (s) => {
expect(reverse(reverse(s))).toBe(s);
})
);
});
3. Runtime Assertion and Monitoring
Complement static analysis with runtime checks:
- Design by Contract (preconditions, postconditions)
- Invariant checking
- Production monitoring for unexpected behaviors
For example, using contracts in Python with the icontract library:
from icontract import require, ensure
@require(lambda x: x >= 0)
@ensure(lambda result: result >= 0)
def square_root(x):
return x ** 0.5
Case Study: Transforming Tool Usage at Scale
Let’s examine how a hypothetical company improved their code analysis approach:
Initial State
- Multiple teams using different linters with default configurations
- Inconsistent enforcement of rules
- High rate of suppressions and overrides
- Quality issues still making it to production
The Transformation Process
- Analysis and Baseline: They began by analyzing their existing codebase to understand common issues and establish a quality baseline.
- Standardization: They created a company-wide set of analysis tools and configurations, with room for team-specific additions.
- Integration: Analysis was integrated at multiple points: IDE, pre-commit, CI/CD, and even post-deployment monitoring.
- Education: They invested in workshops to explain not just how to use the tools but why each rule matters.
- Incremental Improvement: Rather than fixing everything at once, they prioritized critical issues and gradually increased strictness.
Results
- 70% reduction in production bugs related to code quality
- Faster onboarding of new developers
- More consistent codebase across teams
- Improved developer satisfaction as fewer “surprise” issues emerged
The Future of Code Analysis
The field of code analysis continues to evolve rapidly, with several promising trends:
1. AI-Powered Analysis
Machine learning models trained on vast codebases can detect subtle patterns and potential issues that rule-based systems miss:
- Identifying unusual code structures that correlate with bugs
- Suggesting context-aware improvements
- Learning from an organization’s specific patterns and mistakes
2. Semantic Understanding
Next-generation tools are moving beyond syntax to understand code semantics:
- Analyzing data flow and control flow
- Reasoning about program behavior
- Understanding developer intent
3. Cross-System Analysis
As systems become more distributed, tools that can analyze interactions between components will become essential:
- API contract verification
- Cross-service data flow analysis
- Distributed transaction integrity
Conclusion: A Balanced Approach
Code analysis tools are powerful allies in the quest for high-quality software, but they’re not silver bullets. Their effectiveness depends on how they’re configured, integrated, and complemented by other practices.
The most successful approaches to code quality recognize that tools are just one part of a broader strategy that includes:
- Thoughtful tool selection and configuration
- Developer education and skill building
- Strong technical leadership and mentoring
- A culture that values quality alongside delivery speed
- Multiple layers of quality assurance, from static analysis to runtime monitoring
By understanding the limitations of your tools and implementing strategies to address them, you can significantly reduce the bad practices that slip through and build more reliable, maintainable software.
Remember, the goal isn’t perfect code—it’s code that effectively solves problems while being understandable, maintainable, and reliable. The right combination of tools and practices can help you achieve that balance, even if no single tool can get you there alone.
FAQ: Common Questions About Code Analysis Tools
How do I choose the right code analysis tools for my project?
Consider these factors:
- The programming languages and frameworks you’re using
- Your team’s experience level and familiarity with tools
- The types of issues you most want to prevent
- Integration capabilities with your existing workflow
- Community support and rule sets available
How strict should we make our analysis tool configuration?
Start with moderate strictness and gradually increase it. Too strict too soon can lead to frustration and rule-bypassing; too lenient provides little value. Find the balance where the tool catches important issues without becoming a hindrance to productivity.
How do we handle legacy code that doesn’t meet our current standards?
Consider these approaches:
- Use baseline features to only enforce rules on new or modified code
- Gradually refactor critical components to meet standards
- Document known issues and create a prioritized plan to address them
- Use different rule sets for legacy and new code
What metrics should we track to measure the effectiveness of our code analysis strategy?
Consider tracking:
- Reduction in production bugs over time
- Percentage of code covered by analysis
- Rule bypass/suppression rate
- Time spent fixing issues during development vs. after deployment
- Developer satisfaction with the tools and process
By continually refining your approach to code analysis, you can maximize its benefits while minimizing the friction it introduces to your development process.