Why Your Code Abstractions Are Causing More Problems Than They Solve
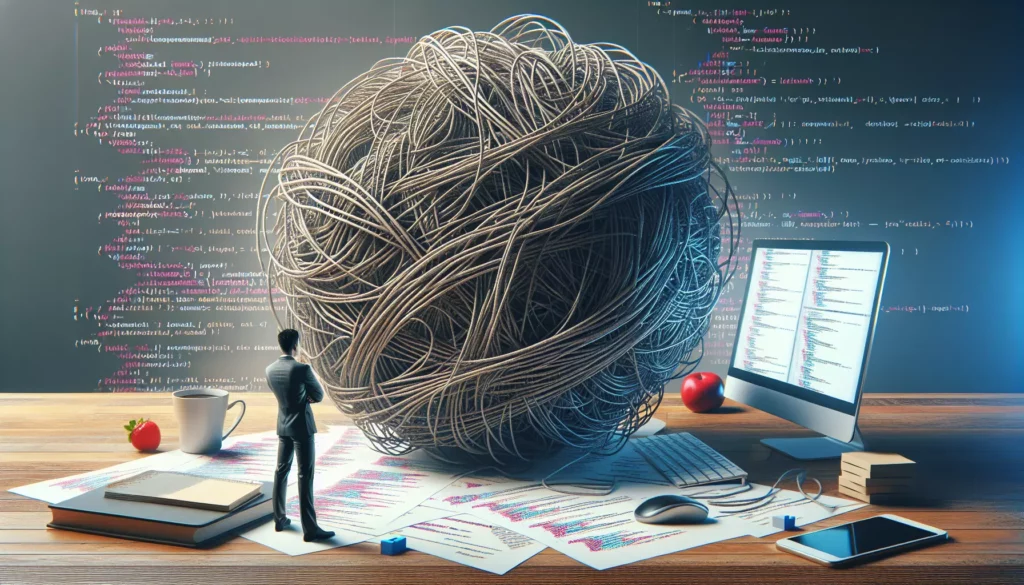
In the world of software development, abstraction is often treated as an unquestionable virtue. We’re taught early in our programming journey that good code is abstract code—that hiding implementation details behind clean interfaces makes our software more maintainable, reusable, and elegant.
But what if this conventional wisdom isn’t always right? What if, in our quest for the perfect abstraction, we’re actually making our codebases more complex, harder to understand, and ultimately less maintainable?
As developers grow in their careers, many come to realize that abstractions are a double-edged sword. Used wisely, they can indeed simplify complex systems. Used carelessly or prematurely, they can transform simple problems into architectural nightmares.
The Promise vs. Reality of Abstractions
Let’s start with what abstractions are supposed to do for us:
- Reduce complexity by hiding implementation details
- Promote code reuse
- Make code more maintainable
- Enable easier changes to the implementation
These are worthy goals. But in practice, many abstractions fail to deliver on these promises. Instead, they often:
- Add indirection that makes code harder to follow
- Create premature generalization for reuse cases that never materialize
- Increase the learning curve for new team members
- Make debugging more difficult
The Cost of Abstraction
Every abstraction comes with costs that are rarely discussed in programming textbooks:
1. Cognitive Load
Each layer of abstraction requires mental effort to understand. When debugging, developers must mentally “unfold” each abstraction to understand what’s actually happening. Consider this example:
// Without abstraction
if (user.age >= 18 && user.hasValidId) {
allowPurchase();
}
// With abstraction
if (user.isEligibleForPurchase()) {
allowPurchase();
}
The abstracted version looks cleaner, but when something goes wrong, you now have to look in multiple places to understand the logic. What exactly makes someone “eligible”? The definition might be simple now, but as business requirements evolve, that method could grow to include dozens of conditions spread across multiple classes.
2. Leaky Abstractions
Joel Spolsky famously pointed out that “all non-trivial abstractions, to some degree, are leaky.” This means that the underlying complexity you’re trying to hide will inevitably seep through your abstraction in unexpected ways.
Consider an ORM (Object-Relational Mapper) like Hibernate or Entity Framework. These tools abstract away SQL, but to use them effectively, you still need to understand SQL and database performance. When your application slows down due to N+1 query problems or inefficient joins, you’re forced to peek behind the abstraction anyway.
3. Premature Optimization for Reuse
Many abstractions are created in anticipation of future needs that never materialize. This is a form of premature optimization—you’re paying the cost of complexity up front for a benefit you may never need.
// What often happens in real projects
class DataProcessor {
process(data) {
// 100 lines of specific business logic for a single use case
}
}
// What developers often write "just in case"
class DataProcessorFactory {
createProcessor(type) {
switch(type) {
case 'TYPE_A': return new TypeAProcessor();
case 'TYPE_B': return new TypeBProcessor();
default: throw new Error('Unknown processor type');
}
}
}
class BaseProcessor {
preProcess() {}
process() { throw new Error('Must implement process'); }
postProcess() {}
}
class TypeAProcessor extends BaseProcessor {
process(data) {
// 100 lines of specific business logic
}
}
class TypeBProcessor extends BaseProcessor {
// Empty implementation because we "might need it later"
process(data) {
return data;
}
}
The second approach adds significant complexity with little immediate benefit. Worse, it forces all future development into this particular abstraction model, which may not be appropriate as requirements evolve.
The Abstraction Fallacy
There’s a common fallacy in software development that more abstraction always leads to better code. This belief stems from several misconceptions:
1. Confusing Simplicity with Familiarity
We often mistake familiar patterns for simplicity. When we see a design pattern we recognize, we think “this is simpler” because we don’t have to think about it as much. But for someone new to the codebase, each layer of indirection adds complexity.
For example, the Repository Pattern is often implemented reflexively in many enterprise applications:
// Using a repository pattern
class UserRepository {
getById(id) { /* database logic */ }
save(user) { /* database logic */ }
// many more methods
}
class UserService {
constructor(userRepository) {
this.userRepository = userRepository;
}
updateUserEmail(userId, newEmail) {
const user = this.userRepository.getById(userId);
user.email = newEmail;
this.userRepository.save(user);
return user;
}
}
This pattern is familiar to many developers, but it adds layers of code to traverse when reading and debugging. For simple CRUD operations, this abstraction might be overkill.
2. The DRY Principle Misapplied
Don’t Repeat Yourself (DRY) is a fundamental principle in programming. However, it’s often taken too far, leading developers to abstract code that shouldn’t be abstracted.
Consider two pieces of code that look similar but serve different business purposes. Combining them into a shared abstraction might seem like a DRY win, but it can create a coupling between unrelated features. When one feature needs to change, you risk breaking the other.
// Before: Two similar but separate functions
function calculateOrderDiscount(order) {
return order.total * (order.customer.isVIP ? 0.2 : 0.1);
}
function calculateShippingInsurance(package) {
return package.value * (package.isFragile ? 0.2 : 0.1);
}
// After: "DRY" but problematic abstraction
function calculatePercentageBased(value, condition, highRate = 0.2, lowRate = 0.1) {
return value * (condition ? highRate : lowRate);
}
// Usage
const orderDiscount = calculatePercentageBased(order.total, order.customer.isVIP);
const shippingInsurance = calculatePercentageBased(package.value, package.isFragile);
Now, if the business rules for order discounts change (maybe adding multiple tiers), you’ll have to modify a function that’s also used for shipping insurance calculations. This creates a coupling between unrelated business concepts.
3. Abstraction as Status Symbol
In some development cultures, complex abstractions are seen as a mark of engineering sophistication. This can lead to unnecessarily complex code written to impress peers rather than solve problems efficiently.
For example, implementing a complex event-driven architecture with message queues for a simple application that could be handled with direct method calls might demonstrate technical knowledge, but it adds significant complexity for little benefit.
Real-World Examples of Abstraction Gone Wrong
Let’s look at some common scenarios where abstractions cause more problems than they solve:
1. The Microservice Maze
Microservices are an architectural abstraction meant to improve scalability and team autonomy. However, many organizations have jumped on the microservice bandwagon without considering the costs:
- Distributed systems are inherently more complex than monoliths
- Network latency and failures become major concerns
- Debugging across service boundaries is challenging
- Data consistency becomes harder to maintain
For many applications, particularly those not operating at massive scale, a well-structured monolith would be simpler and more maintainable than a distributed microservice architecture.
2. The Framework Trap
Modern web frameworks like Angular, React, and Vue provide powerful abstractions for building user interfaces. However, they can also lead developers to over-engineer simple problems:
// Simple DOM manipulation without a framework
document.getElementById('counter').textContent = count.toString();
// The same task with a complex state management system
@Component({
selector: 'app-counter',
template: `<div>{{ count$ | async }}</div>`
})
export class CounterComponent {
count$ = this.store.select(state => state.counter.value);
constructor(private store: Store) {}
increment() {
this.store.dispatch(new IncrementAction());
}
}
For simple UI elements, the framework approach adds significant overhead in terms of code size, build complexity, and cognitive load.
3. The Generic Repository Horror
In data access layers, it’s common to see generic repository abstractions that try to handle all entity types through a single interface:
// Generic repository pattern
interface IRepository<T> {
getById(id: string): Promise<T>;
getAll(): Promise<T[]>;
add(entity: T): Promise<T>;
update(entity: T): Promise<T>;
delete(id: string): Promise<void>;
}
class UserRepository implements IRepository<User> {
// Implementation
}
class ProductRepository implements IRepository<Product> {
// Implementation
}
This approach seems elegant, but it often fails to accommodate the unique query needs of different entity types. As a result, developers end up creating additional methods or bypassing the abstraction entirely for complex queries, negating much of the benefit.
When Abstractions Are Worth It
Despite the problems discussed, abstractions are still essential tools in software development. The key is knowing when they’re worth the cost. Here are some scenarios where abstractions truly shine:
1. When the Domain Complexity Is High
In domains with inherently complex business rules, well-designed abstractions can make the code more understandable by aligning it with business concepts. Domain-Driven Design (DDD) provides patterns for creating these kinds of valuable abstractions.
For example, in a financial system, concepts like “Transaction,” “Account,” and “Ledger” might be complex enough to warrant careful abstraction that mirrors the business domain.
2. When the Technical Complexity Is Unavoidable
Some technical problems are inherently complex and benefit from abstraction. Concurrency, distributed systems, and security often fall into this category.
For instance, abstracting away the complexities of thread safety with a high-level concurrency primitive can prevent subtle bugs and make concurrent code more maintainable:
// Low-level concurrency (error-prone)
Lock lock = new ReentrantLock();
try {
lock.lock();
// Critical section
} finally {
lock.unlock();
}
// Higher-level abstraction
synchronizedBlock(() => {
// Critical section
});
3. When the Abstraction Has Proven Its Value
The best abstractions often emerge from concrete implementations rather than being designed up front. When you’ve written similar code multiple times and understand the variations and edge cases, you’re in a much better position to create an abstraction that truly adds value.
This follows the “Rule of Three” in programming: Write it once. Write it twice. Refactor the third time.
Finding the Right Balance
So how do we find the right balance with abstractions? Here are some practical guidelines:
1. Start Concrete, Then Abstract
Begin with concrete implementations that solve specific problems. Only abstract when you have multiple working examples and understand the commonalities and variations.
This approach follows the principle: “Make it work, make it right, make it fast (or abstract).”
2. Consider the Audience
Remember that code is read far more often than it’s written. Ask yourself: “Will this abstraction make the code easier to understand for someone new to the project?”
Sometimes, explicit code that states exactly what it does is more maintainable than cleverly abstracted code that requires knowledge of the abstraction to understand.
3. Measure the Tradeoffs
For each abstraction, consider:
- How much complexity does it add?
- How much duplication does it eliminate?
- How likely are the requirements to change?
- How much time will developers spend learning and navigating the abstraction?
If an abstraction adds more complexity than it removes, it’s probably not worth it.
4. Be Wary of Speculative Abstractions
Avoid creating abstractions for requirements that don’t exist yet. The YAGNI principle (You Aren’t Gonna Need It) is a valuable guideline here.
It’s often better to duplicate code temporarily than to create the wrong abstraction prematurely. Refactoring duplicated code is usually easier than refactoring an incorrect abstraction that has spread throughout your codebase.
Practical Strategies for Better Abstractions
If you decide an abstraction is warranted, here are strategies to make it more effective:
1. Keep Abstraction Layers Thin
Each layer of abstraction should add clear value. Avoid creating “pass-through” layers that simply delegate to another component without adding any real functionality:
// Unnecessary abstraction layer
class UserManager {
constructor(private userRepository: UserRepository) {}
getUser(id: string) {
return this.userRepository.getById(id); // Just passes through
}
saveUser(user: User) {
return this.userRepository.save(user); // Just passes through
}
}
2. Make Abstractions Transparent
Good abstractions can be understood without having to look at their implementation, but they should also be easy to inspect when needed. Provide clear documentation, meaningful error messages, and debugging tools.
For example, an HTTP client abstraction should provide a way to log the actual requests and responses for debugging purposes.
3. Design for Evolution
Requirements change over time. Design your abstractions to evolve without requiring massive rewrites:
- Use composition over inheritance where possible
- Consider the Strategy pattern for varying behaviors
- Make extension points explicit rather than assuming how the code will need to change
4. Test at the Right Level
Abstractions can make testing more challenging. Decide whether to test through the abstraction or test the components separately:
- Testing through abstractions verifies that they work correctly together
- Testing components separately allows more focused tests but may miss integration issues
A balanced approach often works best: unit test components individually and add integration tests that verify they work together through the abstraction.
Case Study: Simplifying Overengineered Code
Let’s look at a concrete example of simplifying an overengineered abstraction. Consider this notification system:
// Original overengineered version
interface NotificationStrategy {
send(message: string, recipient: string): Promise<void>;
}
class EmailNotificationStrategy implements NotificationStrategy {
async send(message: string, recipient: string): Promise<void> {
// Email sending logic
}
}
class SMSNotificationStrategy implements NotificationStrategy {
async send(message: string, recipient: string): Promise<void> {
// SMS sending logic
}
}
class PushNotificationStrategy implements NotificationStrategy {
async send(message: string, recipient: string): Promise<void> {
// Push notification logic
}
}
class NotificationFactory {
createStrategy(type: 'email' | 'sms' | 'push'): NotificationStrategy {
switch (type) {
case 'email': return new EmailNotificationStrategy();
case 'sms': return new SMSNotificationStrategy();
case 'push': return new PushNotificationStrategy();
}
}
}
class NotificationService {
private strategies: Map<string, NotificationStrategy> = new Map();
constructor(private factory: NotificationFactory) {}
registerStrategy(type: string, strategy: NotificationStrategy) {
this.strategies.set(type, strategy);
}
async notify(type: string, message: string, recipient: string): Promise<void> {
const strategy = this.strategies.get(type) || this.factory.createStrategy(type as any);
await strategy.send(message, recipient);
}
}
// Usage
const factory = new NotificationFactory();
const service = new NotificationService(factory);
await service.notify('email', 'Hello', 'user@example.com');
This implementation uses the Strategy and Factory patterns, but it’s overly complex for what it needs to do. Here’s a simplified version:
// Simplified version
const notifiers = {
email: async (message: string, recipient: string) => {
// Email sending logic
},
sms: async (message: string, recipient: string) => {
// SMS sending logic
},
push: async (message: string, recipient: string) => {
// Push notification logic
}
};
async function notify(type: 'email' | 'sms' | 'push', message: string, recipient: string) {
const notifier = notifiers[type];
if (!notifier) {
throw new Error(`Unknown notification type: ${type}`);
}
await notifier(message, recipient);
}
// Usage
await notify('email', 'Hello', 'user@example.com');
The simplified version is:
- More concise (fewer lines of code)
- Easier to understand at a glance
- Still extensible (you can add new notifiers to the object)
- More direct (fewer layers to navigate when debugging)
Yet it accomplishes the same task. This doesn’t mean the Strategy pattern is bad—it just wasn’t necessary for this particular problem.
Learning to Recognize Abstraction Smells
How can you tell when your abstractions are causing problems? Watch for these warning signs:
1. Abstraction Inversion
This happens when you need to bypass your abstraction to get something done. If you find yourself frequently working around an abstraction rather than through it, it might be solving the wrong problem.
2. Abstraction Confusion
When new team members consistently struggle to understand how to use your abstractions, it might indicate they’re too complex or poorly documented.
3. Abstraction Leakage
If users of your abstraction need to understand the implementation details to use it correctly, the abstraction is leaking and not providing its intended value.
4. Abstraction Overhead
When simple tasks require navigating through multiple layers of abstraction, the overhead might outweigh the benefits.
Conclusion: The Art of Practical Abstraction
Abstraction is neither good nor bad—it’s a tool that must be wielded with care. The best developers aren’t those who create the most elegant abstractions; they’re those who know when abstraction adds value and when it merely adds complexity.
Here are the key takeaways:
- Start with concrete implementations and abstract only when the pattern is clear
- Measure the cost of abstraction against its benefits
- Remember that the goal is maintainable code, not architectural purity
- Be willing to refactor or remove abstractions that aren’t providing value
- Value simplicity and readability over cleverness
By approaching abstraction with a pragmatic mindset, you can harness its power while avoiding its pitfalls. The next time you’re tempted to add another layer of abstraction to your code, ask yourself: “Is this making the system simpler or more complex?” Your future self—and your teammates—will thank you for your restraint.
Remember, the ultimate measure of good code isn’t how abstract or clever it is, but how well it solves the problem at hand while remaining maintainable over time. Sometimes, the most elegant solution is also the most direct.