Why Your Clean Code Principles Are Making Maintenance Harder
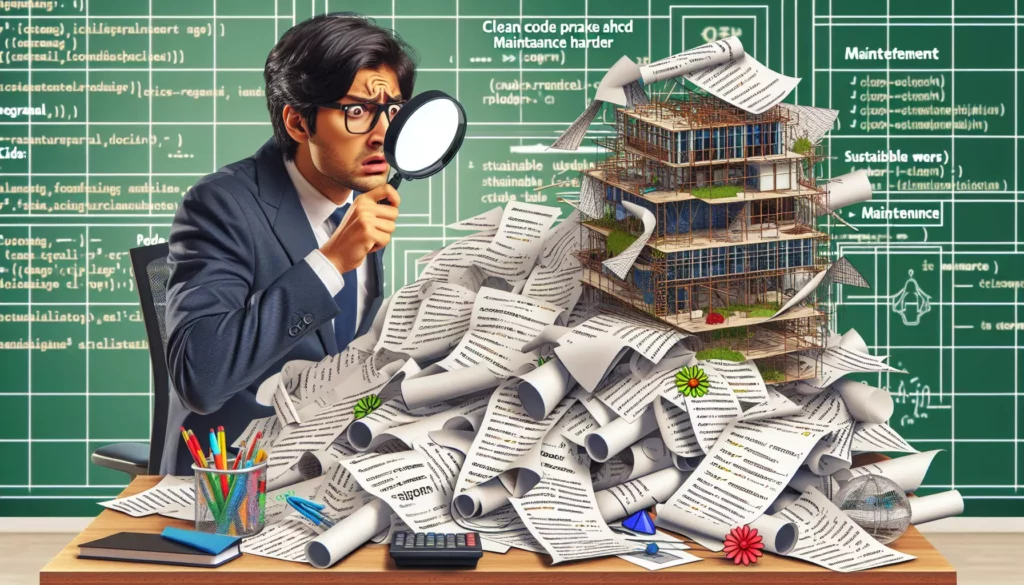
Clean code has been the gospel of software development for decades. We’ve all been taught that writing clean, elegant code is the path to maintainable software. But what if some of these sacred principles are actually making our codebases harder to maintain?
In this post, we’ll challenge conventional wisdom and explore how some clean code practices, when applied dogmatically, can lead to maintenance nightmares. We’ll look at real examples where “clean” code creates more problems than it solves and offer practical alternatives that prioritize long term maintainability over theoretical purity.
The Clean Code Paradox
Before we dive into specifics, let’s acknowledge an uncomfortable truth: software development is full of tradeoffs. What makes code easier to write initially might make it harder to change later. What makes code more elegant might make it more difficult to debug. And what makes code “clean” according to established principles might actually make it harder to maintain.
Robert C. Martin’s influential book “Clean Code” codified many principles that developers still follow religiously today. While many of these principles are valuable, their dogmatic application can lead to problems.
The clean code paradox is simple: code optimized for readability and elegance isn’t always optimized for maintainability and evolution.
The DRY Principle: When Abstraction Creates Coupling
Don’t Repeat Yourself (DRY) is perhaps the most fundamental clean code principle. We’re taught that duplication is the root of all evil in software. But overzealous application of DRY can create maintenance headaches through inappropriate coupling.
The Problem with Premature Abstraction
When we see similar code in multiple places, our instinct is to extract it into a shared function or class. But premature abstraction based on syntactic similarity rather than semantic similarity can create dangerous coupling.
Consider this example of “clean” code following DRY principles:
// A shared utility function to format data
function formatData(data, type) {
if (type === 'user') {
return {
id: data.userId,
name: `${data.firstName} ${data.lastName}`,
email: data.email
};
} else if (type === 'product') {
return {
id: data.productId,
name: data.productName,
price: `$${data.price.toFixed(2)}`
};
}
}
// Used in multiple places
const formattedUser = formatData(userData, 'user');
const formattedProduct = formatData(productData, 'product');
This looks clean, but what happens when requirements change? If the product team needs to change how products are formatted, but not users, we have a problem. Any change to this shared function could break unrelated code.
A More Maintainable Approach
Sometimes, strategic duplication is more maintainable than the wrong abstraction. Consider this alternative:
// Separate functions with clear responsibilities
function formatUserData(userData) {
return {
id: userData.userId,
name: `${userData.firstName} ${userData.lastName}`,
email: userData.email
};
}
function formatProductData(productData) {
return {
id: productData.productId,
name: productData.productName,
price: `$${productData.price.toFixed(2)}`
};
}
// Used in their respective contexts
const formattedUser = formatUserData(userData);
const formattedProduct = formatProductData(productData);
This code contains some duplication, but it’s more maintainable because:
- Changes to user formatting won’t affect product formatting
- Each function clearly communicates its purpose
- Functions can evolve independently as requirements change
As Sandi Metz famously said: “Duplication is far cheaper than the wrong abstraction.” When we extract code prematurely, we often create abstractions based on our current understanding, which may prove incorrect as the system evolves.
Small Functions: When Fragmentation Obscures Intent
Clean code advocates for small, single purpose functions. The ideal function should be only a few lines long and do exactly one thing. This principle can improve readability for individual functions, but it can also create a fragmented codebase that’s hard to follow.
The Problem with Function Fragmentation
When we break code into many tiny functions, we force readers to mentally jump between different parts of the codebase to understand the overall flow. This creates cognitive overhead and can make the code harder to reason about holistically.
Consider this “clean” approach with small functions:
function processOrder(order) {
validateOrder(order);
const pricing = calculatePricing(order);
applyDiscounts(pricing, order.discountCodes);
const finalOrder = prepareOrderForSubmission(order, pricing);
submitOrderToPaymentProcessor(finalOrder);
sendConfirmationEmail(order.customerEmail, finalOrder);
}
function validateOrder(order) {
// 5 lines of validation logic
}
function calculatePricing(order) {
// 5 lines of pricing calculation
}
// ... more small functions
This looks clean at first glance, but to understand what processOrder
actually does, a developer needs to jump between six different functions, which might be located in different parts of the file or even different files.
A More Maintainable Approach
Sometimes a slightly longer function with clear internal structure provides better maintainability because it keeps related logic together:
function processOrder(order) {
// Validation
if (!order.items || order.items.length === 0) {
throw new Error('Order must contain items');
}
if (!order.customerEmail) {
throw new Error('Customer email is required');
}
// Pricing calculation
let subtotal = 0;
for (const item of order.items) {
subtotal += item.price * item.quantity;
}
const tax = subtotal * 0.08;
const pricing = { subtotal, tax, total: subtotal + tax };
// Apply discounts
if (order.discountCodes) {
for (const code of order.discountCodes) {
if (code === 'SUMMER10') {
pricing.total *= 0.9; // 10% off
}
}
}
// Prepare and submit order
const finalOrder = {
...order,
pricing,
timestamp: new Date()
};
paymentAPI.submitOrder(finalOrder);
// Send confirmation
emailService.send({
to: order.customerEmail,
subject: 'Order Confirmation',
body: `Your order total is $${pricing.total.toFixed(2)}`
});
}
This function is longer but has several advantages for maintenance:
- The entire order processing flow is visible in one place
- A developer can understand the complete process without jumping around
- The internal structure with comments provides clear organization
- Debugging is easier when you can see the whole process
The key insight is that “small functions” should be a guideline, not a rule. The appropriate function length depends on what makes the code most maintainable in your specific context.
Excessive Abstraction: When Flexibility Becomes a Burden
Clean code often emphasizes abstraction and flexibility. We’re taught to build layers of abstraction that allow for future changes and extensions. But excessive abstraction can make code harder to understand and maintain.
The Problem with Abstraction Layers
Each layer of abstraction adds complexity and indirection. When we build highly flexible systems with multiple layers of abstraction, we often end up with code that’s harder to trace, debug, and modify.
Consider this “clean” and flexible approach:
// A flexible, abstracted data processing pipeline
class DataProcessor {
constructor(strategies = {}) {
this.inputStrategy = strategies.input || new DefaultInputStrategy();
this.transformStrategy = strategies.transform || new DefaultTransformStrategy();
this.outputStrategy = strategies.output || new DefaultOutputStrategy();
this.errorHandler = strategies.errorHandler || new DefaultErrorHandler();
}
process(data) {
try {
const input = this.inputStrategy.parse(data);
const transformed = this.transformStrategy.transform(input);
return this.outputStrategy.format(transformed);
} catch (error) {
return this.errorHandler.handle(error, data);
}
}
}
// Usage
const processor = new DataProcessor({
transform: new SpecializedTransformStrategy()
});
const result = processor.process(rawData);
This design is flexible and follows clean code principles like dependency injection and the strategy pattern. But it introduces significant complexity, even for simple data processing tasks.
A More Maintainable Approach
For many applications, a more direct approach with less abstraction is more maintainable:
// A straightforward data processing function
function processData(data) {
try {
// Parse input
const parsed = JSON.parse(data);
// Transform data
const transformed = parsed.map(item => ({
id: item.id,
name: item.name.toUpperCase(),
value: item.value * 1.1 // Apply 10% increase
}));
// Format output
return {
items: transformed,
count: transformed.length,
processed: new Date()
};
} catch (error) {
console.error('Failed to process data:', error);
return { error: error.message };
}
}
// Usage
const result = processData(rawData);
This approach has several advantages for maintenance:
- The entire data flow is visible and traceable
- There are fewer moving parts to understand and debug
- Changes can be made directly where needed
- The code is more predictable with fewer layers of indirection
The key insight is that abstraction should serve a clear purpose. Add abstraction when it solves actual problems you’re facing, not in anticipation of theoretical future flexibility needs.
Interface Segregation: When Too Many Interfaces Complicate the Codebase
The Interface Segregation Principle (ISP) suggests that “clients should not be forced to depend on interfaces they do not use.” This often leads to creating many small, specific interfaces. While this can be valuable, it can also lead to interface explosion and needless complexity.
The Problem with Interface Proliferation
When we create many small interfaces, we can end up with a fragmented API that’s harder to discover and use. Each new interface adds cognitive overhead and another piece developers need to learn.
Consider this “clean” approach with many segregated interfaces:
interface UserReader {
getUser(id: string): User;
findUsers(criteria: SearchCriteria): User[];
}
interface UserWriter {
createUser(user: UserInput): User;
updateUser(id: string, data: Partial<UserInput>): User;
}
interface UserDeleter {
deleteUser(id: string): void;
deactivateUser(id: string): User;
}
interface UserAuthenticator {
authenticateUser(email: string, password: string): AuthResult;
generateResetToken(email: string): string;
}
// Implementation
class UserService implements UserReader, UserWriter, UserDeleter, UserAuthenticator {
// Many methods implementing all interfaces
}
This follows ISP but creates a fragmented API that’s harder to discover and use. Developers need to know which interface to use for each operation.
A More Maintainable Approach
For many applications, a cohesive, well documented API is more maintainable than many small interfaces:
/**
* Handles all user-related operations including:
* - Reading and searching for users
* - Creating and updating user data
* - User deletion and deactivation
* - Authentication and password management
*/
class UserService {
// User retrieval
getUser(id: string): User { /* ... */ }
findUsers(criteria: SearchCriteria): User[] { /* ... */ }
// User management
createUser(user: UserInput): User { /* ... */ }
updateUser(id: string, data: Partial<UserInput>): User { /* ... */ }
deleteUser(id: string): void { /* ... */ }
deactivateUser(id: string): User { /* ... */ }
// Authentication
authenticateUser(email: string, password: string): AuthResult { /* ... */ }
generateResetToken(email: string): string { /* ... */ }
}
This approach has several advantages for maintenance:
- The API is more discoverable with related operations grouped together
- Developers have a clear entry point for all user-related functionality
- Documentation can describe the cohesive service rather than fragmented interfaces
- The code is more approachable for new team members
The key insight is that interfaces should serve the needs of actual clients. Create separate interfaces when different clients have distinctly different needs, not just to satisfy a theoretical principle.
Deep Class Hierarchies: When Inheritance Creates Rigidity
Object oriented programming encourages inheritance as a way to reuse code and model relationships. Clean code principles often lead to class hierarchies that model domain concepts. But deep inheritance hierarchies can create rigid, hard to maintain code.
The Problem with Deep Inheritance
Inheritance creates tight coupling between parent and child classes. When a class inherits from another, it becomes dependent on the parent’s implementation details. This can make changes difficult and lead to the fragile base class problem.
Consider this “clean” approach with a deep class hierarchy:
class Vehicle {
constructor(make, model) {
this.make = make;
this.model = model;
}
startEngine() {
console.log('Engine started');
}
stopEngine() {
console.log('Engine stopped');
}
}
class Car extends Vehicle {
constructor(make, model, doors) {
super(make, model);
this.doors = doors;
}
drive() {
console.log('Car driving');
}
}
class ElectricCar extends Car {
constructor(make, model, doors, batteryCapacity) {
super(make, model, doors);
this.batteryCapacity = batteryCapacity;
}
startEngine() {
console.log('Electric motor activated');
}
chargeBattery() {
console.log('Charging battery');
}
}
class TeslaCar extends ElectricCar {
constructor(model, doors, batteryCapacity, autopilotVersion) {
super('Tesla', model, doors, batteryCapacity);
this.autopilotVersion = autopilotVersion;
}
enableAutopilot() {
console.log(`Enabling autopilot v${this.autopilotVersion}`);
}
}
This hierarchy looks clean, but it creates rigid coupling. If we need to change how startEngine()
works in the base class, it could affect all descendants in unexpected ways.
A More Maintainable Approach
Composition often provides more flexibility and maintainability than deep inheritance:
// Engine types implemented as strategies
class GasEngine {
start() {
console.log('Gas engine started');
}
stop() {
console.log('Gas engine stopped');
}
}
class ElectricMotor {
start() {
console.log('Electric motor activated');
}
stop() {
console.log('Electric motor deactivated');
}
}
// Feature modules implemented as composable objects
class Autopilot {
constructor(version) {
this.version = version;
}
enable() {
console.log(`Enabling autopilot v${this.version}`);
}
}
// Vehicle using composition
class Vehicle {
constructor(make, model, engine) {
this.make = make;
this.model = model;
this.engine = engine;
this.features = [];
}
addFeature(feature) {
this.features.push(feature);
}
startEngine() {
this.engine.start();
}
stopEngine() {
this.engine.stop();
}
}
// Usage
const teslaModel3 = new Vehicle('Tesla', 'Model 3', new ElectricMotor());
teslaModel3.addFeature(new Autopilot('3.0'));
teslaModel3.startEngine(); // "Electric motor activated"
teslaModel3.features[0].enable(); // "Enabling autopilot v3.0"
This composition-based approach has several advantages for maintenance:
- Components can be changed independently without affecting others
- New combinations of features can be created without modifying existing code
- The code is more flexible and adaptable to requirement changes
- Testing is easier with smaller, more focused components
The key insight is that inheritance should be used sparingly, primarily for genuine “is-a” relationships where behavior is truly shared. Composition provides more flexibility for most code reuse needs.
Perfect Naming: When Consistency Trumps Precision
Clean code emphasizes the importance of precise, descriptive names. We’re taught that names should perfectly describe what a function or variable does. While good naming is important, obsessing over perfect names can sometimes harm maintainability.
The Problem with Naming Perfectionism
When we insist on perfect names, we often end up with excessively long names or frequent renaming as understanding evolves. This can make code verbose and create unnecessary churn in version control.
Consider this “clean” approach with extremely precise names:
function calculateAdjustedMonthlyPremiumBasedOnRiskFactorsAndCoverageOptions(
baseMonthlyPremium,
customerRiskProfile,
selectedCoverageOptions
) {
// Premium calculation logic
}
const eligibleNonSmokingCustomersWithGoodDrivingRecordAndMultiPolicyDiscount =
customers.filter(/* complex filtering logic */);
class ComprehensiveCustomerHealthAndFinancialRiskAssessmentStrategy {
// Risk assessment logic
}
These names are precise but create readability and maintainability problems. They make code more verbose and harder to work with.
A More Maintainable Approach
Names should be clear and consistent, but they don’t need to capture every nuance:
function calculatePremium(base, riskProfile, coverageOptions) {
// Premium calculation logic
}
const eligibleCustomers = customers.filter(/* complex filtering logic */);
class RiskAssessmentStrategy {
// Risk assessment logic
}
This approach has several advantages for maintenance:
- Code is more concise and readable
- Names are stable and less likely to change as understanding evolves
- Function signatures are more manageable
- The mental overhead of working with the code is reduced
The key insight is that names should be good enough to be clear in context, not perfect in isolation. Use documentation, types, and consistent patterns to provide additional context when needed.
Finding Balance: Practical Clean Code for Maintainability
Clean code principles remain valuable, but they must be applied with judgment rather than dogmatism. Here are practical guidelines for writing code that’s both clean and maintainable:
1. Apply DRY Selectively
Don’t repeat yourself, but don’t be afraid of strategic duplication when it improves maintainability. Ask:
- Will these similar code sections evolve together or separately?
- Is the duplication accidental (same code) or essential (similar but conceptually different code)?
- Would abstracting this code create coupling between unrelated concerns?
2. Right Size Your Functions
Functions should be focused, but not necessarily tiny. Consider:
- Does breaking this into smaller functions improve or harm understanding of the overall flow?
- Would keeping related logic together make debugging easier?
- Is the cognitive overhead of jumping between functions worth the benefits?
3. Add Abstraction When It Solves Problems
Abstraction should serve a purpose, not just follow a pattern. Ask:
- Does this abstraction solve an actual problem we’re facing now?
- Is the flexibility worth the additional complexity?
- Will this abstraction be easier or harder to work with for most developers?
4. Create Interfaces That Serve Users
Design interfaces based on how they’ll be used, not just to satisfy principles:
- Do different clients actually need different subsets of this functionality?
- Would a cohesive API be more discoverable and usable?
- Does this interface division reflect real usage patterns?
5. Prefer Composition Over Inheritance
Use inheritance sparingly and intentionally:
- Is this truly an “is-a” relationship with shared behavior?
- Would composition provide more flexibility for future changes?
- How deep is the inheritance hierarchy and is that complexity justified?
6. Name for Clarity and Consistency
Names should be clear but don’t need to be perfect:
- Is this name clear in the context where it’s used?
- Does it follow consistent patterns in the codebase?
- Is it concise enough to be readable in actual code?
Learning from Real World Examples
Some of the most maintainable codebases in the industry don’t rigidly follow all clean code principles. Here are examples of successful projects that prioritize maintainability:
Go Standard Library
The Go standard library often uses longer functions with clear internal structure rather than breaking everything into tiny functions. This makes the code flow more obvious and easier to follow.
SQLite
SQLite is known for its reliability and maintainability. It uses strategic duplication in some places rather than creating complex abstractions, making the code more direct and understandable.
React
React uses composition as its primary abstraction mechanism rather than deep inheritance hierarchies. This has proven more flexible and maintainable as the library has evolved.
Conclusion: Pragmatic Clean Code
Clean code principles are valuable, but they must serve the ultimate goal of creating maintainable software. Dogmatic application of these principles without considering their impact on maintainability can be counterproductive.
The most maintainable code often finds a balance between cleanliness and pragmatism:
- It’s clean enough to be understood and reasoned about
- It’s direct enough to be traced and debugged
- It’s flexible enough to accommodate change
- It’s stable enough to not require constant refactoring
As you develop your coding style, remember that principles are tools, not rules. The best developers know when to apply clean code principles strictly and when a more pragmatic approach better serves the needs of the project and team.
The next time you find yourself reflexively applying a clean code principle, pause and ask: “Will this actually make the code more maintainable in the long run?” Sometimes the cleanest solution isn’t the most maintainable one.
What clean code principles have you found helpful or harmful in your projects? How do you balance cleanliness with pragmatism? Share your experiences in the comments below.