Why Your “Clean Architecture” Is Making Things More Complicated
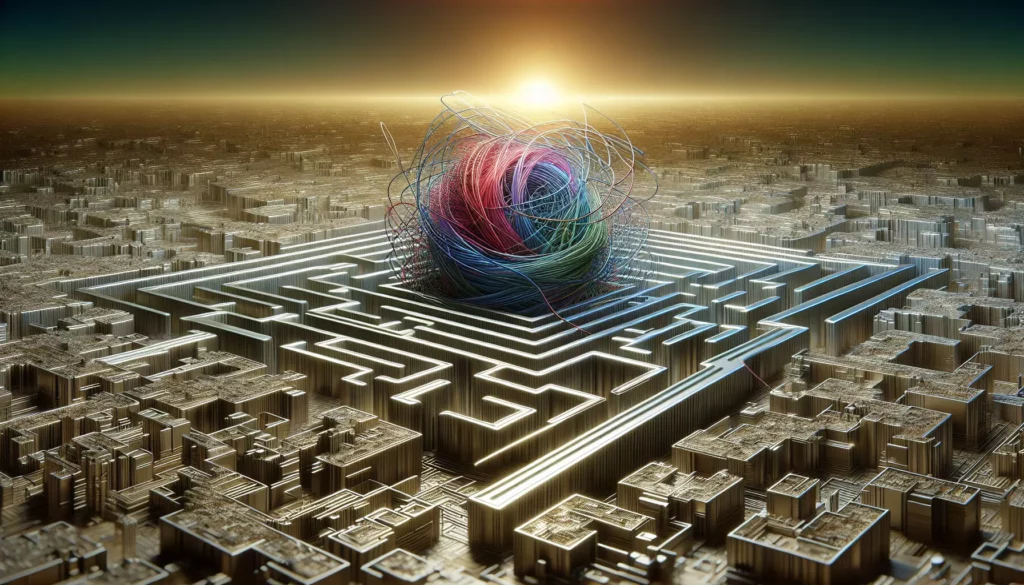
Clean architecture has become something of a Holy Grail in software development circles. Developers proudly proclaim their adherence to clean architecture principles, showing off elaborate diagrams with concentric circles, dependency arrows, and carefully segregated layers. But here’s a controversial take: what if your pursuit of clean architecture is actually making your codebase more complex, harder to maintain, and less intuitive for new team members?
In this deep dive, we’ll explore how overzealous application of clean architecture principles can lead to unnecessary complexity, examine real-world examples where simpler approaches might work better, and provide practical guidance on finding the right balance for your projects.
The Promise vs. Reality of Clean Architecture
First proposed by Robert C. Martin (Uncle Bob), clean architecture promised a way to create software systems that are:
- Independent of frameworks
- Testable
- Independent of the UI
- Independent of the database
- Independent of any external agency
These are admirable goals. Who wouldn’t want a system that’s easy to test and where business logic isn’t tightly coupled to external dependencies?
The reality, however, is that many teams implement “clean architecture” in ways that create more problems than they solve.
The Symptoms of Architecture Overengineering
Let’s look at some telltale signs that your clean architecture implementation might be making things worse:
- File count explosion: Simple features require modifying 10+ files across multiple directories
- Abstraction confusion: New team members struggle to follow the flow of data and control
- Boilerplate overload: You spend more time writing interfaces, factories, and adapters than actual business logic
- Test complexity: Tests require elaborate mocking and setup that mirrors the complexity of the architecture itself
- Development slowdown: Simple features take days instead of hours because of architectural overhead
If these symptoms sound familiar, you might be suffering from architecture overengineering.
The File Count Explosion Problem
One of the most common issues in over-architected systems is the sheer number of files required to implement even simple features. Let’s look at a typical example of implementing a user registration feature in an over-architected system versus a more pragmatic approach.
The “Clean” Approach
In a system rigidly following clean architecture principles, adding a simple user registration feature might require:
- UserRegistrationRequest (DTO)
- UserRegistrationResponse (DTO)
- UserRegistrationController
- IUserRegistrationUseCase (Interface)
- UserRegistrationUseCase (Implementation)
- IUserRepository (Interface)
- UserRepositoryImpl
- User (Domain Entity)
- UserMapper (to convert between domain and data layers)
- UserRegistrationValidator
- UserRegistrationPresenter
That’s 11 files for a single feature! And we haven’t even included tests yet.
A More Pragmatic Approach
In contrast, a more pragmatic approach might involve:
- UserController (handles registration and other user operations)
- UserService (contains business logic)
- UserRepository (data access)
- User (model)
Four files, and the functionality is just as testable if implemented correctly.
The clean architecture approach creates a significant increase in cognitive load. Developers need to jump between multiple files to understand the flow, making it harder to reason about the code.
When Abstraction Becomes Obfuscation
Abstraction is a powerful tool in software development, but too much of it can obscure rather than clarify.
The Interface Overload Problem
Consider this common pattern in “clean” codebases:
// Interface
public interface IUserRepository {
User findById(String id);
void save(User user);
void delete(String id);
}
// Implementation
public class UserRepositoryImpl implements IUserRepository {
private final DatabaseClient dbClient;
public UserRepositoryImpl(DatabaseClient dbClient) {
this.dbClient = dbClient;
}
@Override
public User findById(String id) {
return dbClient.query("SELECT * FROM users WHERE id = ?", id).mapToUser();
}
@Override
public void save(User user) {
dbClient.execute("INSERT INTO users VALUES (?, ?, ?)",
user.getId(), user.getName(), user.getEmail());
}
@Override
public void delete(String id) {
dbClient.execute("DELETE FROM users WHERE id = ?", id);
}
}
What does this interface actually buy us? In many cases, especially in smaller applications or stable teams, very little. The implementation is the only one that will ever exist, yet we’ve added an extra file and layer of indirection.
When Interfaces Make Sense
Interfaces are valuable when:
- You genuinely have multiple implementations (e.g., a real repository and a mock for testing)
- You’re building a library or framework that others will implement
- The system is so large that different teams work on different parts and need stable contracts
But in many applications, especially at early stages, these conditions don’t apply. Adding interfaces prematurely is a form of speculative generality—an antipattern where you build flexibility that you don’t actually need yet.
The Real-World Cost of Overengineering
The pursuit of architectural purity comes with real costs:
Development Velocity
When every feature requires creating and wiring up numerous components across multiple layers, development slows down. What could be a quick two-hour task becomes a day-long exercise in architectural compliance.
Onboarding Difficulty
New team members face a steeper learning curve when they have to understand not just the business domain but also a complex architectural framework with multiple layers of abstraction.
Maintenance Burden
More files and more abstraction layers mean more places where bugs can hide and more code that needs to be maintained over time.
Testing Complexity
While clean architecture promises better testability, overly complex architectures often require elaborate test setups with extensive mocking.
Real-World Example: A Simple CRUD API
Let’s examine a concrete example of how architectural overengineering can complicate a simple CRUD API for managing blog posts.
The Over-Engineered Version
In an over-engineered system, here’s what the structure might look like:
src/
├── domain/
│ ├── entities/
│ │ └── Post.java
│ ├── repositories/
│ │ └── IPostRepository.java
│ └── usecases/
│ ├── CreatePostUseCase.java
│ ├── GetPostUseCase.java
│ ├── UpdatePostUseCase.java
│ └── DeletePostUseCase.java
├── application/
│ ├── services/
│ │ └── PostService.java
│ └── dtos/
│ ├── PostRequestDTO.java
│ └── PostResponseDTO.java
├── infrastructure/
│ ├── repositories/
│ │ └── PostRepositoryImpl.java
│ └── config/
│ └── DatabaseConfig.java
└── presentation/
├── controllers/
│ └── PostController.java
├── presenters/
│ └── PostPresenter.java
└── validators/
└── PostValidator.java
To implement a simple “create post” operation, the flow might go:
- Controller receives PostRequestDTO
- Validator validates the request
- Controller calls the CreatePostUseCase
- UseCase transforms DTO to domain entity
- UseCase calls repository
- Repository saves entity
- UseCase returns result
- Presenter formats the result
- Controller returns the formatted response
That’s a lot of hops for a simple operation!
A More Pragmatic Version
A more pragmatic approach might look like:
src/
├── models/
│ └── Post.java
├── repositories/
│ └── PostRepository.java
├── services/
│ └── PostService.java
└── controllers/
└── PostController.java
The flow becomes:
- Controller receives request
- Controller calls service
- Service validates and processes
- Service calls repository
- Repository saves entity
- Controller returns response
This simpler approach is still testable, maintainable, and separates concerns without introducing excessive abstraction.
Finding the Right Balance
The key is finding the right balance between architectural purity and practical considerations. Here are some principles to guide you:
Start Simple, Evolve as Needed
Begin with a simpler architecture and introduce additional layers of abstraction only when they solve actual problems you’re facing. This approach aligns with the YAGNI principle (You Aren’t Gonna Need It).
Consider Team Size and Experience
Smaller teams or teams with varying experience levels often benefit from simpler architectures with fewer abstractions. Complex architectures work better with larger, more experienced teams where the overhead can be justified by the coordination benefits.
Match Architecture to Project Lifespan
A prototype or MVP probably doesn’t need the same architectural rigor as a system expected to be maintained for a decade. Be honest about your project’s expected lifespan and design accordingly.
Focus on Boundaries, Not Layers
Instead of obsessing over perfect layering, focus on identifying the natural boundaries in your system. Where does one subsystem end and another begin? What parts might genuinely need to change independently?
Practical Guidelines for Sustainable Architecture
Let’s look at some practical guidelines for creating architectures that are clean enough without being overengineered:
The Rule of Three
Don’t abstract until you have at least three concrete instances that would benefit from the abstraction. This applies to interfaces, base classes, and other abstraction mechanisms.
For example, don’t create an IRepository
interface until you have at least three different implementations that would use it.
Colocate Related Code
Instead of strictly separating code by architectural layer (which often means jumping between many files to understand a feature), consider organizing code by feature or domain concept.
For example, rather than:
src/
├── controllers/
│ ├── UserController.java
│ └── ProductController.java
├── services/
│ ├── UserService.java
│ └── ProductService.java
└── repositories/
├── UserRepository.java
└── ProductRepository.java
Consider:
src/
├── user/
│ ├── UserController.java
│ ├── UserService.java
│ └── UserRepository.java
└── product/
├── ProductController.java
├── ProductService.java
└── ProductRepository.java
This makes it easier to understand all the components related to a single feature.
Prefer Composition Over Inheritance
Inheritance hierarchies often become rigid and fragile over time. Prefer composition patterns that allow for more flexibility as requirements change.
Use Functional Approaches Where Appropriate
Modern programming languages offer functional programming features that can often replace complex object hierarchies with simpler, more composable code. Functions are easier to test, reason about, and compose than complex object networks.
Case Study: Refactoring an Over-Engineered System
Let’s look at a case study of refactoring an over-engineered system to something more maintainable. Consider a web application for managing coding tutorials (similar to what AlgoCademy might use internally).
The Original Over-Engineered Design
The original system had:
- 5 layers of abstraction
- Interfaces for everything, even with single implementations
- DTOs at every layer boundary
- Complex dependency injection configuration
- 30+ files to implement CRUD operations for tutorials
Adding a simple field to a tutorial required changes in 7+ files and took hours.
The Refactored Approach
The refactored system:
- Reduced to 3 logical layers (API, service, data)
- Eliminated interfaces with only one implementation
- Used the same model objects across layers where possible
- Organized code by feature rather than by layer
- Reduced to 12 files for the same functionality
After refactoring, adding a field took 15 minutes and required changes to just 3 files.
The Outcome
The simplified architecture resulted in:
- 40% faster development of new features
- New team members becoming productive in days instead of weeks
- Fewer bugs due to reduced complexity
- Smaller, more focused tests
- Better code review processes
The team still maintained good separation of concerns and testability but without the excessive overhead of the original design.
When Clean Architecture Makes Sense
Despite the criticisms, there are situations where a more formal clean architecture approach makes sense:
Large Enterprise Systems
Systems with dozens of developers across multiple teams benefit from the clear boundaries and contracts that clean architecture provides.
Systems with Multiple Frontends or Backends
When your business logic needs to support multiple UI frameworks (web, mobile, desktop) or multiple data sources, the separation provided by clean architecture is valuable.
Long-Lived Systems
Systems expected to live for many years and undergo multiple technology migrations benefit from the technology independence that clean architecture enables.
Regulated Environments
In regulated industries where audit trails and clear separation of responsibilities are required, clean architecture can help demonstrate compliance.
Practical Examples for Coding Education Platforms
Since we’re talking about AlgoCademy, let’s look at some specific examples of how these principles might apply to a coding education platform.
Tutorial Management
A typical tutorial management feature might include:
// A pragmatic approach for Tutorial management
// TutorialController.java
@RestController
@RequestMapping("/tutorials")
public class TutorialController {
private final TutorialService tutorialService;
public TutorialController(TutorialService tutorialService) {
this.tutorialService = tutorialService;
}
@GetMapping("/{id}")
public Tutorial getTutorial(@PathVariable String id) {
return tutorialService.findById(id);
}
@PostMapping
public Tutorial createTutorial(@RequestBody Tutorial tutorial) {
return tutorialService.create(tutorial);
}
// Other endpoints...
}
// TutorialService.java
@Service
public class TutorialService {
private final TutorialRepository repository;
public TutorialService(TutorialRepository repository) {
this.repository = repository;
}
public Tutorial findById(String id) {
return repository.findById(id)
.orElseThrow(() -> new NotFoundException("Tutorial not found"));
}
public Tutorial create(Tutorial tutorial) {
// Validation logic
if (tutorial.getTitle() == null || tutorial.getTitle().isEmpty()) {
throw new ValidationException("Title is required");
}
// Business logic
tutorial.setCreatedAt(LocalDateTime.now());
return repository.save(tutorial);
}
// Other methods...
}
// TutorialRepository.java
public interface TutorialRepository extends JpaRepository<Tutorial, String> {
List<Tutorial> findByDifficultyLevel(String level);
}
// Tutorial.java
@Entity
public class Tutorial {
@Id
private String id;
private String title;
private String content;
private String difficultyLevel;
private LocalDateTime createdAt;
// Getters and setters...
}
This approach is clean, maintainable, and extensible without introducing unnecessary abstractions.
Code Execution Service
For a service that executes user code (a core feature for a platform like AlgoCademy), you might genuinely need more abstraction to handle different languages and execution environments:
// CodeExecutionController.java
@RestController
@RequestMapping("/execute")
public class CodeExecutionController {
private final CodeExecutionService executionService;
@PostMapping
public ExecutionResult executeCode(@RequestBody ExecutionRequest request) {
return executionService.execute(request);
}
}
// CodeExecutionService.java
@Service
public class CodeExecutionService {
private final Map<String, CodeExecutor> executors;
public CodeExecutionService(List<CodeExecutor> executorList) {
this.executors = executorList.stream()
.collect(Collectors.toMap(CodeExecutor::getLanguage, Function.identity()));
}
public ExecutionResult execute(ExecutionRequest request) {
String language = request.getLanguage();
CodeExecutor executor = executors.get(language);
if (executor == null) {
throw new UnsupportedLanguageException(language);
}
return executor.execute(request.getCode(), request.getInputs());
}
}
// CodeExecutor.java (interface)
public interface CodeExecutor {
String getLanguage();
ExecutionResult execute(String code, List<String> inputs);
}
// JavaCodeExecutor.java
@Component
public class JavaCodeExecutor implements CodeExecutor {
@Override
public String getLanguage() {
return "java";
}
@Override
public ExecutionResult execute(String code, List<String> inputs) {
// Implementation for executing Java code
}
}
// PythonCodeExecutor.java
@Component
public class PythonCodeExecutor implements CodeExecutor {
@Override
public String getLanguage() {
return "python";
}
@Override
public ExecutionResult execute(String code, List<String> inputs) {
// Implementation for executing Python code
}
}
Here, the interface abstraction makes sense because we genuinely have multiple implementations (one per programming language). The architecture is more complex, but appropriately so given the requirements.
Evolving Your Architecture
Architecture isn’t static—it should evolve with your application. Here’s how to evolve your architecture sensibly:
Listen to the Pain
Let actual development pain guide your architectural decisions. If you’re spending too much time wiring components together, your architecture might be too complex. If you’re constantly fighting with tightly coupled code, you might need more structure.
Refactor Incrementally
Don’t try to rewrite everything at once. Identify the most painful parts of your codebase and refactor those first. Use the strangler fig pattern to gradually replace overly complex components.
Measure the Impact
Keep track of how architectural changes affect development velocity, bug rates, and team satisfaction. Use these metrics to guide further refinements.
Conclusion: Pragmatic Architecture Over Purity
Clean architecture offers valuable principles, but dogmatic adherence to architectural purity often leads to unnecessary complexity. Instead of focusing on having the “cleanest” architecture, aim for the most appropriate architecture for your specific context.
Remember these key takeaways:
- Start simple and add complexity only when needed
- Organize code by feature rather than by layer when possible
- Use interfaces and abstractions judiciously, not automatically
- Let the specific needs of your project guide architectural decisions
- Be willing to evolve your architecture as your application grows
The best architecture is one that enables your team to work effectively and deliver value to users—not one that looks perfect in a diagram or strictly adheres to a specific pattern.
For a coding education platform like AlgoCademy, focusing on pragmatic architecture means you can spend more time improving the learning experience and less time managing architectural overhead. Your users care about great tutorials and effective learning tools, not whether your codebase has the perfect layering.
What are your experiences with clean architecture? Have you found yourself drowning in abstractions, or has a more formal architecture saved your project? Share your thoughts and experiences in the comments!