Why Your Cat is Better at Solving Algorithm Problems Than You
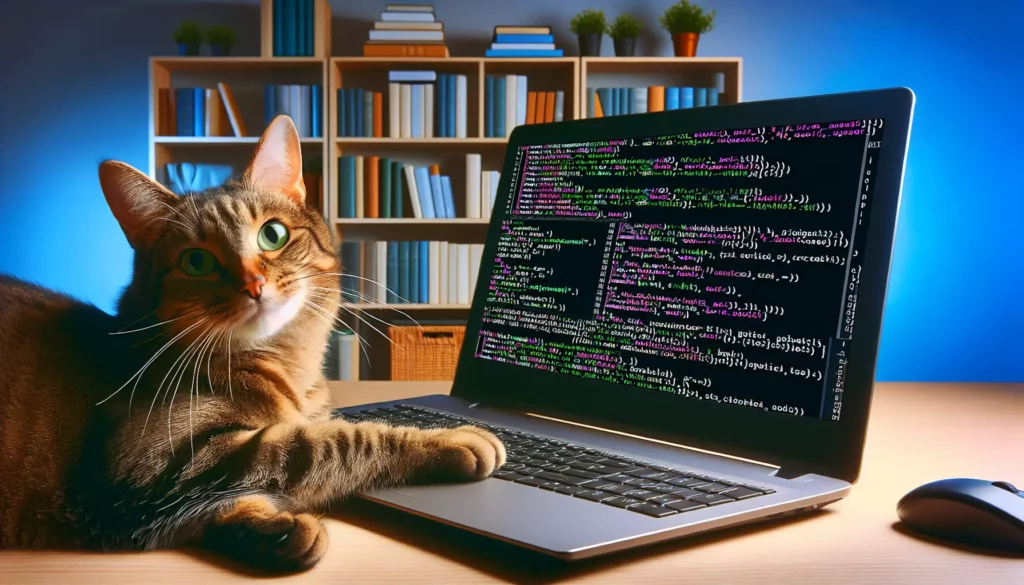
In the world of coding and algorithm problems, we often find ourselves scratching our heads, staring at the screen for hours, and wondering why that pesky bug won’t go away. But what if I told you that your furry feline friend might actually be better at solving these complex problems than you are? It may sound absurd, but hear me out. In this article, we’ll explore the surprising ways in which cats exhibit problem-solving skills that parallel those needed in algorithmic thinking and coding. We’ll also look at how understanding these feline traits can help us become better programmers and problem solvers ourselves.
The Feline Approach to Problem-Solving
Cats are known for their independent nature and curious personalities. These traits, as it turns out, are quite beneficial when it comes to tackling complex problems. Let’s break down some of the key characteristics that make cats natural-born algorithm solvers:
1. Patience and Persistence
Have you ever watched a cat stalk a toy or wait patiently by a mouse hole? Cats are masters of patience, willing to wait for extended periods to achieve their goals. This patience translates well to solving algorithm problems, where rushing can often lead to mistakes and oversights.
In coding, patience is a virtue. When faced with a challenging algorithm, it’s essential to take your time, analyze the problem thoroughly, and consider multiple approaches before diving into implementation. Just as a cat waits for the perfect moment to pounce, a good programmer knows when to strike with the right solution.
2. Keen Observation Skills
Cats are incredibly observant creatures. They notice the slightest movements and changes in their environment. This acute awareness is a valuable skill in algorithm problem-solving, where attention to detail can make all the difference.
When working on coding challenges, it’s crucial to observe patterns, identify edge cases, and spot potential optimizations. By honing our observational skills like a cat, we can become more adept at recognizing the nuances of a problem and devising efficient solutions.
3. Adaptability and Quick Learning
Cats are known for their ability to adapt to new situations quickly. Whether it’s figuring out how to open a door or navigating a new environment, cats demonstrate remarkable adaptability. This trait is invaluable in the ever-changing world of programming and algorithms.
In the field of computer science, new technologies and approaches emerge constantly. Being able to adapt quickly, learn new concepts, and apply them to solve problems is essential for success. Like a cat learning to use a new toy, programmers must be ready to embrace new tools and techniques to tackle algorithmic challenges effectively.
4. Breaking Problems into Smaller Parts
Have you ever seen a cat try to catch a toy that’s just out of reach? They often approach the problem step by step: first, they may try jumping, then climbing, and finally, they might enlist the help of nearby objects to reach their goal. This instinctive ability to break down a complex task into smaller, manageable steps is a cornerstone of algorithmic thinking.
In programming, we often use a divide-and-conquer approach to solve complex problems. By breaking down a large algorithm into smaller subproblems, we can tackle each part individually and then combine the solutions. This method is exemplified in algorithms like merge sort or in dynamic programming techniques.
Feline-Inspired Strategies for Better Algorithm Problem-Solving
Now that we’ve established how cats naturally embody some key traits of good problem-solvers, let’s explore how we can apply these feline-inspired strategies to improve our own algorithmic thinking and coding skills.
1. The “Cat Nap” Technique
Cats are famous for their frequent naps, and there’s wisdom in this habit when it comes to problem-solving. When you’re stuck on a difficult algorithm problem, sometimes the best approach is to step away and take a break. This “cat nap” technique allows your brain to process information subconsciously and often leads to fresh insights when you return to the problem.
Try this: Set a timer for 20-30 minutes of focused work on a problem. If you haven’t made significant progress by the end of this time, take a short break. Go for a walk, meditate, or even take a quick power nap. When you return, you may find that your mind has cleared and new solutions present themselves.
2. The “Curious Cat” Approach
Cats are naturally curious, always exploring their surroundings and investigating new objects. Apply this curiosity to your algorithm problem-solving by exploring different approaches and technologies. Don’t be afraid to try unconventional methods or learn about new algorithms that might be applicable to your problem.
For example, if you’re working on a graph problem, don’t limit yourself to the algorithms you already know. Take some time to research and experiment with different graph traversal techniques or optimizations. This curiosity can lead to more efficient solutions and a broader understanding of algorithmic concepts.
3. The “Feline Focus” Method
When a cat is focused on a target, whether it’s a toy or a real prey, their concentration is unparalleled. They block out all distractions and devote their full attention to the task at hand. Emulate this intense focus when tackling algorithm problems.
Create a distraction-free environment for your coding sessions. Turn off notifications, find a quiet space, and immerse yourself fully in the problem. This level of concentration can help you notice subtle details and patterns that you might otherwise miss.
4. The “Nine Lives” Mindset
Cats are often said to have nine lives due to their resilience and ability to survive challenging situations. Adopt this mindset when facing difficult algorithm problems. Don’t be discouraged by initial failures or setbacks. Instead, view each attempt as a learning opportunity and a chance to refine your approach.
Keep multiple solutions in mind and be willing to pivot if one approach isn’t working. Like a cat that tries different strategies to catch a elusive mouse, be persistent and flexible in your problem-solving efforts.
Practical Examples: Feline Problem-Solving in Action
Let’s look at some common algorithm problems and how we can apply our feline-inspired strategies to solve them more effectively.
Example 1: The “Mouse in the Maze” Problem (Graph Traversal)
Imagine a maze represented as a graph, where we need to find the shortest path from start to finish. This is a classic problem that can be solved using breadth-first search (BFS) or depth-first search (DFS) algorithms.
Feline Approach:
- Observation: Like a cat surveying its territory, start by carefully examining the structure of the maze.
- Breaking it down: Identify key decision points in the maze, similar to how a cat might plan its route through a complex environment.
- Persistence: Implement your chosen algorithm (BFS or DFS) and patiently explore all possible paths, just as a cat would methodically search every nook and cranny.
- Adaptability: If one approach proves inefficient, be ready to switch to an alternative algorithm or optimization technique.
Here’s a simple implementation of BFS for maze solving in Python:
from collections import deque
def solve_maze(maze, start, end):
rows, cols = len(maze), len(maze[0])
queue = deque([(start, [])])
visited = set([start])
while queue:
(x, y), path = queue.popleft()
if (x, y) == end:
return path + [(x, y)]
for dx, dy in [(0, 1), (1, 0), (0, -1), (-1, 0)]:
nx, ny = x + dx, y + dy
if 0 <= nx < rows and 0 <= ny < cols and maze[nx][ny] == 0 and (nx, ny) not in visited:
queue.append(((nx, ny), path + [(x, y)]))
visited.add((nx, ny))
return None # No path found
# Example usage
maze = [
[0, 0, 0, 0],
[1, 1, 0, 1],
[0, 0, 0, 0],
[0, 1, 1, 0]
]
start = (0, 0)
end = (3, 3)
path = solve_maze(maze, start, end)
print(f"Path found: {path}")
Example 2: The “Catching the Red Dot” Problem (Dynamic Programming)
Consider the classic dynamic programming problem of finding the maximum sum path in a grid. This can be likened to a cat trying to catch a red laser dot, always moving to the position that offers the highest reward.
Feline Approach:
- Breaking it down: Divide the grid into smaller subproblems, much like how a cat might break down the challenge of catching the dot into a series of individual pounces.
- Observation: Carefully consider the value of each move, just as a cat calculates each jump.
- Adaptability: Be ready to update your strategy based on new information, similar to how a cat adjusts its approach as the red dot moves.
- Patience: Build your solution step by step, starting from the simplest case and gradually increasing complexity.
Here’s a Python implementation of this dynamic programming solution:
def max_sum_path(grid):
if not grid:
return 0
rows, cols = len(grid), len(grid[0])
dp = [[0] * cols for _ in range(rows)]
# Initialize the first cell
dp[0][0] = grid[0][0]
# Initialize first row
for j in range(1, cols):
dp[0][j] = dp[0][j-1] + grid[0][j]
# Initialize first column
for i in range(1, rows):
dp[i][0] = dp[i-1][0] + grid[i][0]
# Fill the dp table
for i in range(1, rows):
for j in range(1, cols):
dp[i][j] = max(dp[i-1][j], dp[i][j-1]) + grid[i][j]
return dp[rows-1][cols-1]
# Example usage
grid = [
[1, 3, 1],
[1, 5, 1],
[4, 2, 1]
]
max_sum = max_sum_path(grid)
print(f"Maximum sum path: {max_sum}")
Leveraging AI: Your Digital Feline Companion
While we can learn a lot from our feline friends, we also have powerful digital tools at our disposal. Artificial Intelligence (AI) can serve as a complementary “digital cat” in our algorithm problem-solving toolkit. Platforms like AlgoCademy leverage AI to provide personalized guidance and support in tackling complex coding challenges.
How AI Enhances Feline-Inspired Problem-Solving
- Pattern Recognition: Just as cats are adept at recognizing patterns in their environment, AI excels at identifying patterns in code and suggesting optimizations.
- Tireless Assistance: While even the most persistent cat needs to sleep, AI can provide 24/7 support, offering hints and explanations whenever you need them.
- Adaptive Learning: AI systems can adapt to your learning style and pace, much like how cats adjust their behavior based on their environment.
- Vast Knowledge Base: AI can quickly access and apply a vast array of algorithmic knowledge, complementing your own understanding and problem-solving skills.
Integrating AI into Your Problem-Solving Routine
Here are some ways to effectively use AI-powered tools like those offered by AlgoCademy in your algorithm problem-solving process:
- Use AI for Initial Brainstorming: When faced with a new problem, consult AI for potential approaches and algorithms that might be applicable.
- Leverage AI for Code Review: After implementing your solution, use AI to review your code for potential optimizations or bug fixes.
- Learn from AI Explanations: When stuck, ask the AI to explain concepts or provide step-by-step breakdowns of complex algorithms.
- Practice with AI-Generated Problems: Use AI to generate custom practice problems that target your specific areas for improvement.
Conclusion: Embracing Your Inner Coding Cat
While it’s true that your cat isn’t literally solving complex algorithms (at least, not that we know of!), there’s a lot we can learn from their approach to problem-solving. By embracing patience, keen observation, adaptability, and the ability to break down complex problems, we can significantly improve our algorithm-solving skills.
Remember, the next time you’re stuck on a challenging coding problem, take a moment to observe your feline friend. Their focused yet relaxed approach to tackling obstacles might just inspire your next breakthrough. And don’t forget to leverage the power of AI tools like those provided by AlgoCademy to complement your newfound feline-inspired problem-solving techniques.
So, channel your inner coding cat, stay curious, be persistent, and don’t be afraid to take a “cat nap” when you need to reset your mind. With practice and the right mindset, you might just find yourself becoming as adept at solving algorithm problems as your cat is at catching that elusive red dot.
Happy coding, and may your algorithmic adventures be as graceful and successful as a cat’s perfect landing!