Why Your Caching Strategy Is Causing Data Consistency Issues
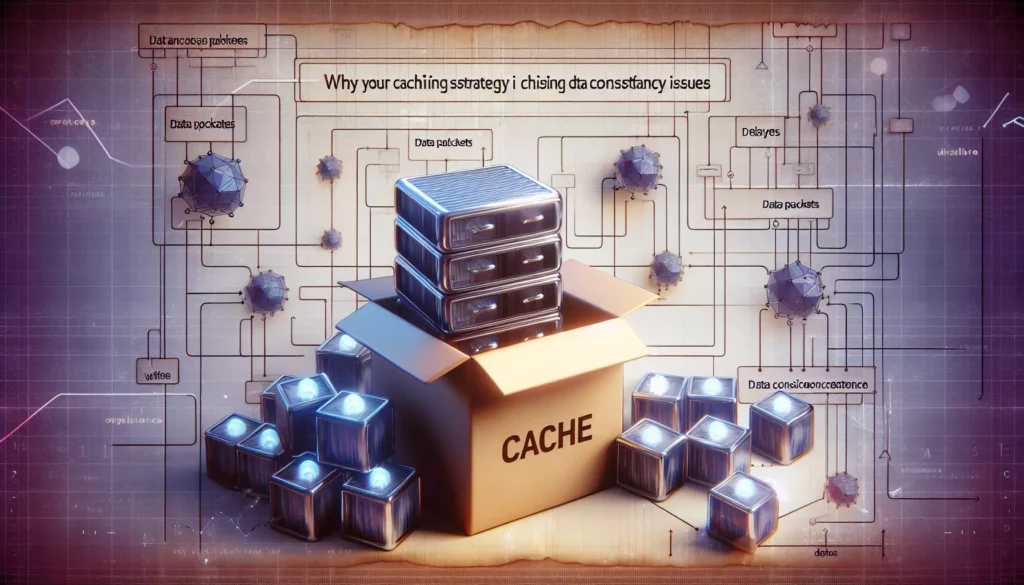
In the world of software development, caching is often introduced as a performance optimization technique. And indeed, when implemented correctly, caching can dramatically improve application response times, reduce database load, and enhance user experience. However, what many developers discover the hard way is that caching introduces a fundamental challenge: data consistency.
If you’ve noticed strange behaviors in your application where data seems outdated, inconsistent across different parts of your system, or mysteriously “flickering” between old and new values, your caching strategy might be the culprit. This comprehensive guide will help you understand why caching can lead to data consistency issues and provide practical solutions to address these challenges.
Table of Contents
- Understanding Caching: The Double-Edged Sword
- Common Data Consistency Issues in Cached Systems
- How to Identify Caching-Related Consistency Problems
- Cache Invalidation Strategies
- Consistency Patterns and Solutions
- Distributed Caching Challenges
- Testing Strategies for Cached Systems
- Monitoring and Observability for Cache Health
- Real-World Examples and Case Studies
- Conclusion: Building Reliable Cached Systems
Understanding Caching: The Double-Edged Sword
At its core, caching is simple: store a copy of data in a location that’s faster to access than the original source. However, this simplicity belies the complexity that arises when the original data changes.
The Fundamental Tradeoff
When we cache data, we’re making an explicit tradeoff between consistency and performance. The CAP theorem tells us that in distributed systems, we can have at most two of the following three properties:
- Consistency: All clients see the same data at the same time
- Availability: The system continues to function even when components fail
- Partition tolerance: The system continues to operate despite network failures
Since network partitions are a reality we must deal with, the real choice becomes one between consistency and availability. Caching typically pushes us toward availability at the expense of consistency.
Types of Caching
Different caching approaches present different consistency challenges:
- In-memory caching: Fast but typically limited to a single application instance
- Distributed caching: Shared across application instances but introduces network latency
- Database query caching: Reduces database load but can become stale
- HTTP caching: Improves web performance but has limited control mechanisms
- CDN caching: Excellent for static assets but challenging for dynamic content
Each of these approaches creates its own set of consistency considerations. An in-memory cache in a single-server application might have simpler consistency requirements than a globally distributed system using CDNs and multiple caching layers.
Common Data Consistency Issues in Cached Systems
Let’s explore the most common consistency issues that arise in cached systems:
Stale Data
This is the most obvious issue: a cache contains outdated information that no longer reflects the source of truth. For example, a product price is updated in the database, but users still see the old price because it’s cached.
// Example of potential stale data issue
function getProductPrice(productId) {
// Check if price is in cache
const cachedPrice = cache.get(`product:${productId}:price`);
if (cachedPrice) {
return cachedPrice; // This could be stale!
}
// If not in cache, get from database
const price = database.getProductPrice(productId);
// Store in cache for 1 hour
cache.set(`product:${productId}:price`, price, 3600);
return price;
}
In this example, if the price changes in the database, it won’t be reflected in the application until the cache expires or is manually invalidated.
Cache Stampede (Thundering Herd)
When a frequently accessed cache key expires, multiple concurrent requests might attempt to rebuild the cache simultaneously, potentially overwhelming the backend system.
// Vulnerable to cache stampede
async function getUserProfile(userId) {
const cacheKey = `user:${userId}:profile`;
// Check cache first
const cachedProfile = await cache.get(cacheKey);
if (cachedProfile) return JSON.parse(cachedProfile);
// Cache miss - fetch from database
// If 100 requests hit this simultaneously, we'll make 100 identical DB queries!
const profile = await database.getUserProfile(userId);
// Store in cache
await cache.set(cacheKey, JSON.stringify(profile), 300);
return profile;
}
Write-Behind Inconsistency
In write-behind caching patterns, where updates are first made to the cache and asynchronously written to the database, failures in the background write process can lead to data loss or inconsistency.
// Write-behind caching with potential consistency issues
async function updateUserPreferences(userId, preferences) {
const cacheKey = `user:${userId}:preferences`;
// Update cache immediately
await cache.set(cacheKey, JSON.stringify(preferences));
// Schedule background update to database
backgroundQueue.push({
task: 'updateUserPreferences',
data: { userId, preferences }
});
return { success: true }; // Returns before DB is updated!
}
If the background task fails, the cache and database will contain different data.
Read-After-Write Inconsistency
Users expect that after they update data, they’ll see their changes reflected immediately. With caching, this isn’t always guaranteed.
// Example of read-after-write inconsistency
async function updateUserProfile(userId, profileData) {
// Update in database
await database.updateUserProfile(userId, profileData);
// User profile is cached, but we don't update the cache!
// The next read will return stale data
return { success: true };
}
async function getUserProfile(userId) {
const cacheKey = `user:${userId}:profile`;
// Check cache first
const cachedProfile = await cache.get(cacheKey);
if (cachedProfile) return JSON.parse(cachedProfile);
// Cache miss - fetch from database
const profile = await database.getUserProfile(userId);
// Store in cache
await cache.set(cacheKey, JSON.stringify(profile), 3600);
return profile;
}
After calling updateUserProfile
, the user would still see their old profile data if they immediately refresh the page.
Cache Coherence in Distributed Systems
In systems with multiple application servers, each with its own local cache, updates made on one server may not be reflected in the caches of other servers.
// Server A
app.post('/update-status', (req, res) => {
const { userId, newStatus } = req.body;
// Update in database
database.updateUserStatus(userId, newStatus);
// Update local cache for Server A
localCache.set(`user:${userId}:status`, newStatus);
res.json({ success: true });
});
// Server B - still has old status in its local cache!
app.get('/user-status/:userId', (req, res) => {
const { userId } = req.params;
// Check local cache first
const cachedStatus = localCache.get(`user:${userId}:status`);
if (cachedStatus) {
return res.json({ status: cachedStatus }); // This is stale!
}
// Otherwise fetch from database
const status = database.getUserStatus(userId);
localCache.set(`user:${userId}:status`, status);
res.json({ status });
});
How to Identify Caching-Related Consistency Problems
Before you can fix cache consistency issues, you need to identify them. Here are some signs and methods to diagnose caching problems:
Common Symptoms
- Users reporting they don’t see their own updates
- Data appearing to “flicker” between old and new values
- Inconsistent results when calling the same API multiple times
- Data discrepancies between different parts of your application
- Issues that “fix themselves” after a certain period
Diagnostic Techniques
Add Cache Headers to Responses
For HTTP-based caching, include cache-related headers in your responses to help with debugging:
// Express.js example
app.get('/api/product/:id', (req, res) => {
const { id } = req.params;
const product = getProduct(id);
// Add cache debugging headers
res.set('X-Cache', cache.has(id) ? 'HIT' : 'MISS');
res.set('X-Cache-Expires', new Date(Date.now() + cacheTTL * 1000).toISOString());
res.json(product);
});
Implement Cache Logging
Add detailed logging around cache operations:
function getCachedData(key) {
const startTime = Date.now();
const value = cache.get(key);
const duration = Date.now() - startTime;
if (value) {
logger.debug({
message: 'Cache hit',
key,
duration,
valueSize: JSON.stringify(value).length
});
return value;
}
logger.debug({
message: 'Cache miss',
key,
duration
});
// Fetch and cache the data...
}
Implement Version Tagging
Add version information to your cached data:
function cacheUserData(userId, userData) {
const wrappedData = {
data: userData,
version: userData.version || Date.now(),
cachedAt: new Date().toISOString()
};
cache.set(`user:${userId}`, JSON.stringify(wrappedData));
}
This makes it easier to identify when you’re dealing with stale data.
Cache Invalidation Strategies
The famous quote “There are only two hard things in Computer Science: cache invalidation and naming things” exists for a reason. Let’s explore different cache invalidation strategies:
Time-Based Expiration
The simplest approach is to set a Time-To-Live (TTL) for cached items:
// Set cache with a 5-minute TTL
cache.set('user:1234', userData, 300);
Pros:
- Simple to implement
- Works well for data that changes predictably
- No additional logic needed for invalidation
Cons:
- Data can be stale for up to the TTL duration
- Hard to find the right TTL value (too short = cache ineffective, too long = stale data)
- Can’t handle immediate invalidation needs
Write-Through Caching
Update the cache whenever you update the underlying data:
async function updateUserProfile(userId, profileData) {
// Update in database
await database.updateUserProfile(userId, profileData);
// Update in cache
const cacheKey = `user:${userId}:profile`;
await cache.set(cacheKey, JSON.stringify(profileData));
return { success: true };
}
Pros:
- Cache is always up-to-date
- Solves read-after-write inconsistency
- Conceptually simple
Cons:
- Requires updating cache on every write operation
- Doesn’t handle distributed caching well without additional mechanisms
- Can increase write latency
Cache-Aside (Lazy Loading)
Load data into the cache only when it’s requested, and invalidate the cache when data changes:
async function getUserProfile(userId) {
const cacheKey = `user:${userId}:profile`;
// Check cache first
const cachedProfile = await cache.get(cacheKey);
if (cachedProfile) return JSON.parse(cachedProfile);
// Cache miss - fetch from database
const profile = await database.getUserProfile(userId);
// Store in cache
await cache.set(cacheKey, JSON.stringify(profile), 3600);
return profile;
}
async function updateUserProfile(userId, profileData) {
// Update in database
await database.updateUserProfile(userId, profileData);
// Invalidate cache
const cacheKey = `user:${userId}:profile`;
await cache.delete(cacheKey);
return { success: true };
}
Pros:
- Only caches data that’s actually requested
- Simple invalidation on write
- Works well for read-heavy workloads
Cons:
- First request after invalidation is slow
- Can lead to cache stampedes
- Requires careful tracking of which keys to invalidate
Event-Based Invalidation
Use events or message queues to notify all application instances when data changes:
// When data changes
async function updateProduct(productId, productData) {
// Update in database
await database.updateProduct(productId, productData);
// Publish event
await messageQueue.publish('product-updated', {
productId,
timestamp: Date.now()
});
return { success: true };
}
// In each application instance
messageQueue.subscribe('product-updated', (message) => {
const { productId } = message;
// Invalidate local cache
cache.delete(`product:${productId}`);
console.log(`Cache invalidated for product ${productId}`);
});
Pros:
- Works well in distributed environments
- Can provide near-real-time invalidation
- Decouples cache invalidation from write operations
Cons:
- More complex infrastructure required
- Potential for missed events if the message system fails
- Can introduce additional latency
Consistency Patterns and Solutions
Let’s explore some patterns that can help maintain data consistency in cached systems:
The Stale-While-Revalidate Pattern
This pattern serves stale content while fetching fresh content in the background:
async function getData(key) {
const cached = await cache.get(key);
if (cached) {
const { data, timestamp } = JSON.parse(cached);
const isStale = Date.now() - timestamp > STALE_THRESHOLD;
if (isStale) {
// Return stale data but refresh in background
refreshDataInBackground(key);
}
return data; // Return potentially stale data immediately
}
// Cache miss - fetch fresh data
return await fetchAndCacheData(key);
}
async function refreshDataInBackground(key) {
try {
// Fetch fresh data
const freshData = await fetchFromSource(key);
// Update cache
await cache.set(key, JSON.stringify({
data: freshData,
timestamp: Date.now()
}));
} catch (error) {
logger.error(`Background refresh failed for ${key}`, error);
}
}
This pattern provides a good balance between performance and freshness.
Two-Phase Commit for Cache Updates
For critical operations where consistency is paramount:
async function updateCriticalData(key, newValue) {
// Phase 1: Prepare
const transactionId = generateUniqueId();
await cache.set(`transaction:${transactionId}`, JSON.stringify({
key,
newValue,
status: 'pending'
}));
try {
// Phase 2: Commit to database
await database.update(key, newValue);
// Phase 3: Update cache and mark transaction complete
await Promise.all([
cache.set(key, JSON.stringify(newValue)),
cache.set(`transaction:${transactionId}`, JSON.stringify({
key,
newValue,
status: 'committed'
}))
]);
return { success: true };
} catch (error) {
// Mark transaction as failed
await cache.set(`transaction:${transactionId}`, JSON.stringify({
key,
newValue,
status: 'failed',
error: error.message
}));
throw error;
}
}
This approach is more complex but provides stronger consistency guarantees for critical operations.
Cache Versioning
Instead of invalidating cache entries, update a version identifier:
// Initialize or increment version
async function incrementResourceVersion(resourceType) {
const versionKey = `version:${resourceType}`;
const currentVersion = await cache.get(versionKey) || 0;
const newVersion = parseInt(currentVersion) + 1;
await cache.set(versionKey, newVersion);
return newVersion;
}
// When fetching data, include the version in the cache key
async function getResource(resourceType, resourceId) {
const versionKey = `version:${resourceType}`;
const version = await cache.get(versionKey) || 1;
const cacheKey = `${resourceType}:${resourceId}:v${version}`;
const cached = await cache.get(cacheKey);
if (cached) return JSON.parse(cached);
// Cache miss - fetch from database
const resource = await database.getResource(resourceType, resourceId);
// Cache with version
await cache.set(cacheKey, JSON.stringify(resource));
return resource;
}
// When updating resources, increment the version
async function updateResource(resourceType, resourceId, data) {
// Update in database
await database.updateResource(resourceType, resourceId, data);
// Increment version instead of invalidating specific keys
await incrementResourceVersion(resourceType);
return { success: true };
}
This pattern works well for resources that are frequently updated and where fine-grained invalidation is difficult.
Distributed Caching Challenges
Distributed caching introduces additional complexity:
Cache Coherence
In a distributed system, ensuring all cache instances have consistent data is challenging. Solutions include:
Centralized Cache
Using a service like Redis or Memcached as a shared cache:
// All application instances use the same Redis cache
const redis = require('redis');
const client = redis.createClient({
host: 'central-redis-server',
port: 6379
});
async function getData(key) {
return new Promise((resolve, reject) => {
client.get(key, (err, result) => {
if (err) return reject(err);
resolve(result ? JSON.parse(result) : null);
});
});
}
Publish/Subscribe for Invalidation
Using a pub/sub mechanism to coordinate cache invalidation:
// Setup Redis pub/sub
const subscriber = redis.createClient(redisConfig);
const publisher = redis.createClient(redisConfig);
// Subscribe to cache invalidation events
subscriber.subscribe('cache-invalidation');
subscriber.on('message', (channel, message) => {
if (channel === 'cache-invalidation') {
const { key } = JSON.parse(message);
localCache.delete(key); // Invalidate local cache
console.log(`Invalidated cache key: ${key}`);
}
});
// When data changes, publish invalidation event
async function invalidateCache(key) {
await publisher.publish('cache-invalidation', JSON.stringify({ key }));
}
Partial Failures
In distributed systems, some cache nodes might be unreachable. Strategies include:
- Circuit Breakers: Prevent cascading failures when cache services are down
- Fallbacks: Gracefully degrade to database queries when cache is unavailable
- Bulkheads: Isolate cache failures from affecting the entire system
async function getCachedData(key) {
try {
// Try to get from cache with timeout
const cachedData = await Promise.race([
cache.get(key),
new Promise((_, reject) =>
setTimeout(() => reject(new Error('Cache timeout')), 100)
)
]);
if (cachedData) return JSON.parse(cachedData);
} catch (error) {
// Log cache failure but continue
logger.warn(`Cache failure: ${error.message}`);
metrics.increment('cache.failures');
}
// Fallback to database
return await database.getData(key);
}
Testing Strategies for Cached Systems
Testing caching logic is crucial for preventing consistency issues:
Unit Testing Cache Logic
// Jest example testing cache-aside pattern
test('should return cached data when available', async () => {
// Mock cache
const mockCache = {
get: jest.fn().mockResolvedValue(JSON.stringify({ name: 'Cached User' })),
set: jest.fn()
};
// Mock database
const mockDb = {
getUserProfile: jest.fn()
};
const userService = new UserService(mockCache, mockDb);
const result = await userService.getUserProfile('user123');
expect(result).toEqual({ name: 'Cached User' });
expect(mockCache.get).toHaveBeenCalledWith('user:user123:profile');
expect(mockDb.getUserProfile).not.toHaveBeenCalled();
});
test('should fetch from database on cache miss', async () => {
// Mock cache miss
const mockCache = {
get: jest.fn().mockResolvedValue(null),
set: jest.fn()
};
// Mock database
const mockDb = {
getUserProfile: jest.fn().mockResolvedValue({ name: 'Database User' })
};
const userService = new UserService(mockCache, mockDb);
const result = await userService.getUserProfile('user123');
expect(result).toEqual({ name: 'Database User' });
expect(mockCache.get).toHaveBeenCalledWith('user:user123:profile');
expect(mockDb.getUserProfile).toHaveBeenCalledWith('user123');
expect(mockCache.set).toHaveBeenCalled();
});
Integration Testing
Test the full caching flow with a real or containerized cache:
// Integration test with real Redis
describe('User profile caching integration', () => {
let redisClient;
let userService;
beforeAll(async () => {
redisClient = new Redis({
host: 'localhost',
port: 6379
});
userService = new UserService(
new RedisCache(redisClient),
new UserDatabase()
);
});
afterAll(async () => {
await redisClient.quit();
});
beforeEach(async () => {
await redisClient.flushall();
});
test('should cache user profile after first request', async () => {
// First request should hit database
const profile1 = await userService.getUserProfile('test-user');
// Verify profile is now in cache
const cachedData = await redisClient.get('user:test-user:profile');
expect(cachedData).not.toBeNull();
expect(JSON.parse(cachedData)).toEqual(profile1);
// Second request should use cache
const startTime = Date.now();
const profile2 = await userService.getUserProfile('test-user');
const duration = Date.now() - startTime;
expect(profile2).toEqual(profile1);
expect(duration).toBeLessThan(10); // Should be very fast
});
});
Chaos Testing
Simulate cache failures and network partitions to ensure system resilience:
test('should handle cache failure gracefully', async () => {
// Mock a failing cache
const mockCache = {
get: jest.fn().mockRejectedValue(new Error('Connection refused')),
set: jest.fn().mockRejectedValue(new Error('Connection refused'))
};
const mockDb = {
getUserProfile: jest.fn().mockResolvedValue({ name: 'Fallback User' })
};
const userService = new UserService(mockCache, mockDb);
// System should fall back to database
const result = await userService.getUserProfile('user123');
expect(result).toEqual({ name: 'Fallback User' });
expect(mockDb.getUserProfile).toHaveBeenCalledWith('user123');
});
Monitoring and Observability for Cache Health
Proper monitoring is essential for detecting and diagnosing cache-related issues:
Key Metrics to Monitor
- Cache Hit Rate: Percentage of requests served from cache
- Cache Latency: Time taken for cache operations
- Cache Size: Memory usage of the cache
- Cache Evictions: Number of items removed due to memory pressure
- Cache Errors: Failed cache operations
// Example middleware for HTTP cache monitoring
function cacheMetricsMiddleware(req, res, next) {
const startTime = Date.now();
// Store original cache methods to wrap them
const originalGet = cache.get;
// Wrap cache.get to collect metrics
cache.get = async function(key) {
try {
const result = await originalGet.call(cache, key);
const duration = Date.now() - startTime;
if (result) {
metrics.increment('cache.hits');
metrics.timing('cache.hit.duration', duration);
} else {
metrics.increment('cache.misses');
}
return result;
} catch (error) {
metrics.increment('cache.errors');
throw error;
}
};
next();
// Restore original method after request
res.on('finish', () => {
cache.get = originalGet;
});
}
Logging for Cache Operations
Implement structured logging for cache operations:
class CacheLogger {
constructor(cache, logger) {
this.cache = cache;
this.logger = logger;
}
async get(key) {
const start = Date.now();
try {
const result = await this.cache.get(key);
const duration = Date.now() - start;
this.logger.debug({
operation: 'cache.get',
key,
hit: !!result,
duration
});
return result;
} catch (error) {
this.logger.error({
operation: 'cache.get',
key,
error: error.message,
stack: error.stack
});
throw error;
}
}
// Similar wrappers for set, delete, etc.
}
Distributed Tracing
Implement distributed tracing to understand how caching affects request flows:
async function getUserData(userId, tracingContext) {
const span = tracer.startSpan('getUserData', {
childOf: tracingContext
});
try {
span.setTag('userId', userId);
const cacheSpan = tracer.startSpan('cache.get', { childOf: span });
const cachedData = await cache.get(`user:${userId}`);
cacheSpan.setTag('cache.hit', !!cachedData);
cacheSpan.finish();
if (cachedData) {
span.setTag('data_source', 'cache');
span.finish();
return JSON.parse(cachedData);
}
const dbSpan = tracer.startSpan('database.query', { childOf: span });
const userData = await database.getUserById(userId);
dbSpan.finish();