Why Your Brute Force Solutions Keep Getting Rejected by Interviewers
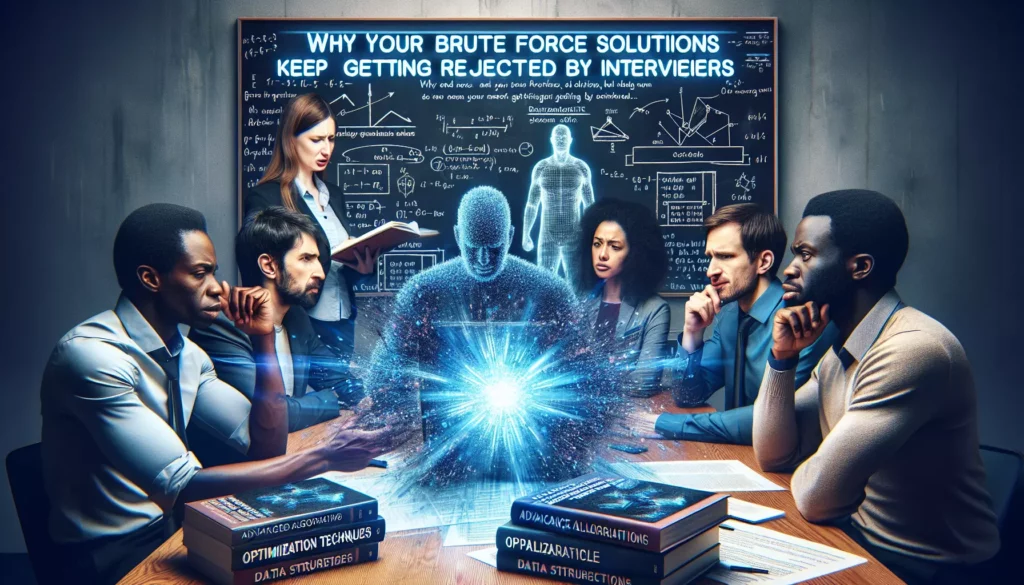
Technical interviews can be intimidating, especially when you’ve spent hours practicing problems only to have your solutions dismissed as “brute force” approaches. This common experience leaves many candidates feeling frustrated and confused about what interviewers are actually looking for.
In this comprehensive guide, we’ll explore why brute force solutions often get rejected, what interviewers are really evaluating, and how you can level up your problem-solving approach to impress even the most demanding technical interviewers at top companies.
Understanding Brute Force Solutions
Before diving into why interviewers reject brute force solutions, let’s clarify what “brute force” actually means in the context of coding interviews.
What Is a Brute Force Solution?
A brute force solution is an approach that directly applies computing power to try all possibilities without employing clever algorithmic techniques. It’s the most straightforward way to solve a problem, often relying on nested loops or checking every possible combination.
For example, if asked to find two numbers in an array that sum to a target value, a brute force solution might look like this:
function findTwoSum(nums, target) {
for (let i = 0; i < nums.length; i++) {
for (let j = i + 1; j < nums.length; j++) {
if (nums[i] + nums[j] === target) {
return [i, j];
}
}
}
return null;
}
This solution works by checking every possible pair of numbers in the array, resulting in O(n²) time complexity.
Why Do Developers Default to Brute Force?
There are several reasons why candidates often present brute force solutions:
- Simplicity: Brute force approaches are typically the first solutions that come to mind.
- Reliability: They almost always work correctly, even if inefficiently.
- Pressure: Under interview stress, it’s easier to fall back on straightforward approaches.
- Starting point: Many were taught to get a working solution first, then optimize.
Why Interviewers Reject Brute Force Solutions
While brute force solutions can correctly solve problems, they often fall short of what interviewers are looking for. Here’s why these solutions typically get rejected:
1. Poor Performance at Scale
The most obvious reason is performance. Brute force solutions often have suboptimal time and space complexity, making them impractical for large inputs. Companies like Google, Amazon, and Facebook deal with massive datasets where inefficient algorithms simply won’t work.
Consider this example of finding the maximum subarray sum:
// Brute force approach: O(n³) time complexity
function maxSubarraySumBruteForce(nums) {
let maxSum = -Infinity;
for (let i = 0; i < nums.length; i++) {
for (let j = i; j < nums.length; j++) {
let currentSum = 0;
for (let k = i; k <= j; k++) {
currentSum += nums[k];
}
maxSum = Math.max(maxSum, currentSum);
}
}
return maxSum;
}
This O(n³) solution would time out with even moderately sized arrays, while Kadane’s algorithm solves it in O(n) time:
// Optimal approach: O(n) time complexity
function maxSubarraySum(nums) {
let maxSoFar = nums[0];
let maxEndingHere = nums[0];
for (let i = 1; i < nums.length; i++) {
maxEndingHere = Math.max(nums[i], maxEndingHere + nums[i]);
maxSoFar = Math.max(maxSoFar, maxEndingHere);
}
return maxSoFar;
}
2. Demonstrates Limited Problem-Solving Skills
Interviewers use coding challenges as a proxy to assess your broader problem-solving abilities. Defaulting to brute force suggests you might:
- Lack familiarity with common algorithmic patterns
- Have limited exposure to optimization techniques
- Struggle to recognize when more elegant solutions exist
- Fail to apply appropriate data structures to simplify problems
These skills are crucial for day-to-day engineering work, where optimization and elegant solutions can save significant resources.
3. Indicates Potential Knowledge Gaps
When you present only a brute force solution, interviewers might infer gaps in your computer science fundamentals. They expect candidates to recognize common patterns and apply appropriate techniques like:
- Dynamic programming for optimization problems
- Hash tables for lookup optimization
- Two-pointer techniques for array manipulation
- Tree or graph traversal algorithms
- Divide and conquer approaches
4. Shows Lack of Consideration for Resource Constraints
In real-world software development, resources are limited. Memory, processing power, battery life, and network bandwidth all come with constraints. Engineers who default to brute force solutions without considering these limitations might create products that:
- Drain device batteries rapidly
- Consume excessive memory
- Respond slowly to user interactions
- Scale poorly as user bases grow
What Interviewers Are Really Looking For
Understanding what interviewers actually want will help you better prepare and approach coding challenges. Here’s what they’re typically evaluating:
1. Analytical Thinking Process
More than just the final solution, interviewers care about how you approach problems. They want to see:
- Problem clarification: Do you ask thoughtful questions to understand requirements?
- Systematic reasoning: Can you break down complex problems into manageable pieces?
- Pattern recognition: Do you identify when common algorithmic patterns apply?
- Edge case consideration: Do you proactively think about boundary conditions?
Articulating your thought process out loud gives interviewers insight into your analytical abilities.
2. Algorithmic Knowledge and Application
Interviewers expect you to recognize and apply appropriate algorithms and data structures:
- Time and space complexity awareness: Understanding the efficiency implications of your choices
- Data structure selection: Choosing the right tool for the job (hash maps, heaps, trees, etc.)
- Algorithm application: Applying established algorithms when appropriate
3. Optimization Mindset
The ability to iteratively improve solutions demonstrates valuable engineering skills:
- Identifying bottlenecks: Recognizing which parts of an algorithm are inefficient
- Trading space for time: Understanding when to use additional memory to improve runtime
- Preprocessing: Recognizing when upfront work can lead to faster subsequent operations
4. Code Quality and Readability
Even optimized solutions need to be maintainable:
- Clean variable naming: Using descriptive identifiers that convey purpose
- Modular design: Breaking complex logic into well-defined functions
- Comments and documentation: Adding explanations for non-obvious parts
- Testing approach: Demonstrating how you would verify correctness
Common Interview Problems and Their Optimal Solutions
Let’s examine some frequently asked interview problems, contrasting brute force approaches with more optimal solutions:
Problem 1: Two Sum
Problem: Given an array of integers and a target sum, find two numbers that add up to the target.
Brute Force (Often Rejected):
function twoSumBruteForce(nums, target) {
for (let i = 0; i < nums.length; i++) {
for (let j = i + 1; j < nums.length; j++) {
if (nums[i] + nums[j] === target) {
return [i, j];
}
}
}
return null;
}
// Time Complexity: O(n²)
// Space Complexity: O(1)
Optimal Solution (Preferred by Interviewers):
function twoSumOptimal(nums, target) {
const numMap = {};
for (let i = 0; i < nums.length; i++) {
const complement = target - nums[i];
if (complement in numMap) {
return [numMap[complement], i];
}
numMap[nums[i]] = i;
}
return null;
}
// Time Complexity: O(n)
// Space Complexity: O(n)
Why It’s Better: The optimal solution uses a hash map to store previously seen values, allowing for O(1) lookups of complements. This reduces the time complexity from O(n²) to O(n) at the cost of O(n) space, a worthwhile trade-off for most applications.
Problem 2: Detect a Cycle in a Linked List
Problem: Determine if a linked list contains a cycle.
Brute Force (Often Rejected):
function hasCycleBruteForce(head) {
const seenNodes = new Set();
let current = head;
while (current !== null) {
if (seenNodes.has(current)) {
return true;
}
seenNodes.add(current);
current = current.next;
}
return false;
}
// Time Complexity: O(n)
// Space Complexity: O(n)
Optimal Solution (Preferred by Interviewers):
function hasCycleOptimal(head) {
if (!head || !head.next) return false;
let slow = head;
let fast = head;
while (fast && fast.next) {
slow = slow.next;
fast = fast.next.next;
if (slow === fast) {
return true;
}
}
return false;
}
// Time Complexity: O(n)
// Space Complexity: O(1)
Why It’s Better: While both solutions have O(n) time complexity, the optimal solution uses Floyd’s Tortoise and Hare algorithm to achieve O(1) space complexity, making it more memory-efficient. This demonstrates knowledge of specialized algorithms for linked list problems.
Problem 3: Finding the kth Largest Element
Problem: Find the kth largest element in an unsorted array.
Brute Force (Often Rejected):
function findKthLargestBruteForce(nums, k) {
nums.sort((a, b) => b - a);
return nums[k - 1];
}
// Time Complexity: O(n log n)
// Space Complexity: O(1) or O(n) depending on the sorting algorithm
Optimal Solution (Preferred by Interviewers):
function findKthLargest(nums, k) {
return quickSelect(nums, 0, nums.length - 1, nums.length - k);
}
function quickSelect(nums, left, right, k) {
if (left === right) return nums[left];
const pivotIndex = partition(nums, left, right);
if (k === pivotIndex) {
return nums[k];
} else if (k < pivotIndex) {
return quickSelect(nums, left, pivotIndex - 1, k);
} else {
return quickSelect(nums, pivotIndex + 1, right, k);
}
}
function partition(nums, left, right) {
const pivot = nums[right];
let i = left;
for (let j = left; j < right; j++) {
if (nums[j] <= pivot) {
[nums[i], nums[j]] = [nums[j], nums[i]];
i++;
}
}
[nums[i], nums[right]] = [nums[right], nums[i]];
return i;
}
// Average Time Complexity: O(n)
// Worst-case Time Complexity: O(n²)
// Space Complexity: O(log n) due to recursion
Why It’s Better: The quickselect algorithm has an average time complexity of O(n), which is better than the O(n log n) complexity of sorting the entire array. This shows understanding of when to use specialized algorithms rather than generic approaches.
How to Improve Beyond Brute Force
If you find yourself consistently defaulting to brute force solutions, here are strategies to level up your problem-solving approach:
1. Master Common Algorithmic Patterns
Recognize and practice these fundamental patterns that appear across many problems:
- Two Pointers: For array/string problems involving pairs, subarrays, or sorting
- Sliding Window: For contiguous sequence problems like subarrays or substrings
- Binary Search: Not just for sorted arrays, but also for search space reduction
- BFS/DFS: For tree and graph traversal problems
- Dynamic Programming: For optimization problems with overlapping subproblems
- Greedy Algorithms: For local optimization problems
For each pattern, understand:
- When to apply it
- The template implementation
- Common variations and edge cases
2. Analyze Time and Space Complexity
Develop the habit of analyzing your solutions before presenting them:
- Identify the operations that dominate complexity
- Count nested loops and recursive calls
- Consider average and worst-case scenarios
- Evaluate memory usage for data structures
This analysis helps you recognize when your solution is suboptimal and prompts you to look for improvements.
3. Practice Optimization Techniques
Learn these common optimization approaches:
- Precomputation: Calculate and store values upfront to avoid repeated work
- Memoization: Cache results of expensive function calls
- Space-time tradeoffs: Use additional memory to reduce computation time
- Problem transformation: Reframe the problem in a way that makes it easier to solve
4. Use the Right Data Structures
Each data structure has strengths and weaknesses. Know when to use:
- Hash Tables: For O(1) lookups, finding duplicates, or counting occurrences
- Heaps: For priority queues, finding k largest/smallest elements
- Trees: For hierarchical data and range queries
- Tries: For prefix matching and word problems
- Graphs: For relationship and connection problems
- Stacks/Queues: For processing elements in specific orders
5. Develop a Systematic Approach to Problem Solving
Follow this framework for each interview problem:
- Understand the problem: Ask clarifying questions, confirm constraints, and discuss edge cases
- Explore examples: Walk through small test cases to understand patterns
- Break it down: Divide the problem into smaller, manageable subproblems
- Brainstorm approaches: Consider multiple algorithms before coding
- Start with brute force: Explain it briefly, analyze its complexity, then improve it
- Optimize incrementally: Identify bottlenecks and apply appropriate techniques
- Code with clarity: Write clean, readable code with meaningful variable names
- Test thoroughly: Check edge cases and validate correctness
- Analyze final complexity: Discuss time and space requirements of your solution
Presenting Brute Force in Interviews Strategically
While you should aim for optimal solutions, there are strategic ways to incorporate brute force into your interview process:
1. Use Brute Force as a Starting Point
It’s perfectly acceptable to begin with a brute force solution if you:
- Acknowledge its limitations: “Let me start with a straightforward approach, though I recognize it’s not optimal.”
- Analyze its complexity: “This solution would be O(n²) time and O(1) space.”
- Pivot quickly: “Now let’s see if we can improve this using a hash map…”
This demonstrates that you can both solve the problem and critically evaluate solutions.
2. Verbalize Your Optimization Process
Walk the interviewer through your thought process:
- Identify inefficiencies: “I notice we’re doing repeated lookups here, which is inefficient.”
- Consider alternatives: “We could use a hash map to reduce lookup time from O(n) to O(1).”
- Evaluate tradeoffs: “This would increase our space complexity to O(n), but significantly improve runtime.”
3. When Brute Force Might Be Acceptable
In some scenarios, a well-implemented brute force solution might be sufficient:
- When you can prove no better asymptotic solution exists
- For problems with very small input sizes where constants matter more than big O
- When the optimal solution is extremely complex and error-prone
However, always discuss these considerations explicitly rather than assuming brute force is adequate.
Real Interview Stories: Brute Force Rejections and Successes
Let’s examine some real (anonymized) experiences from candidates:
Case Study 1: The Two-Sum Rejection
“I implemented the two-sum problem using nested loops. The interviewer asked if I could do better, but I couldn’t think of anything on the spot. They seemed disappointed and moved on quickly. Later I realized a hash map would have been much more efficient.”
Lesson: Familiarize yourself with hash map optimizations for lookup problems. When an interviewer asks if you can do better, it’s a strong hint that a more optimal solution exists.
Case Study 2: The Incremental Improvement Success
“I started with a brute force solution for finding duplicate numbers, but immediately said, ‘This is O(n²), which isn’t ideal. Let me think about how we can improve it.’ I talked through using a hash set to track seen numbers, bringing it down to O(n) time. The interviewer seemed impressed with my process even though my first instinct wasn’t optimal.”
Lesson: Demonstrating awareness of limitations and actively working toward improvements can be just as valuable as immediately producing the optimal solution.
Case Study 3: The Algorithm Knowledge Gap
“I was asked to find the kth smallest element in an array. I sorted the array and returned the kth element. The interviewer asked if I knew about quickselect, which I didn’t. They spent the rest of the interview explaining it to me, but I didn’t get the job.”
Lesson: Familiarize yourself with specialized algorithms for common interview problems. While sorting works, knowing more efficient approaches demonstrates deeper algorithmic knowledge.
Conclusion: Beyond Brute Force
Brute force solutions keep getting rejected not because they’re incorrect, but because they fail to demonstrate the full range of skills interviewers are evaluating. Technical interviews assess not just your ability to solve problems, but how you approach optimization, your knowledge of algorithms and data structures, and your consideration of real-world constraints.
By understanding what interviewers are looking for and developing a systematic approach to problem-solving, you can move beyond brute force to create elegant, efficient solutions that showcase your true capabilities as a developer.
Remember that the journey from brute force to optimal solutions mirrors the growth path of a software engineer. Just as you wouldn’t ship a brute force algorithm in production code without considering its performance implications, approaching interview problems with the same optimization mindset demonstrates that you’re ready to contribute to real-world engineering challenges.
The next time you face a coding interview, embrace the opportunity to demonstrate not just that you can solve the problem, but that you can solve it well.
Practice Questions to Test Your Optimization Skills
To help you practice moving beyond brute force solutions, try these common interview problems:
- Find all pairs of numbers in an array that sum to a given target.
- Determine if a string has all unique characters.
- Find the longest substring without repeating characters.
- Merge two sorted arrays into one sorted array.
- Detect if two strings are anagrams of each other.
For each problem, first write the brute force solution, analyze its complexity, then challenge yourself to optimize it using the techniques discussed in this article.
With practice and the right approach, you’ll transform from a brute force coder to an algorithmic thinker who impresses even the toughest interviewers.