Why Your Automated Tests Aren’t Preventing Bugs
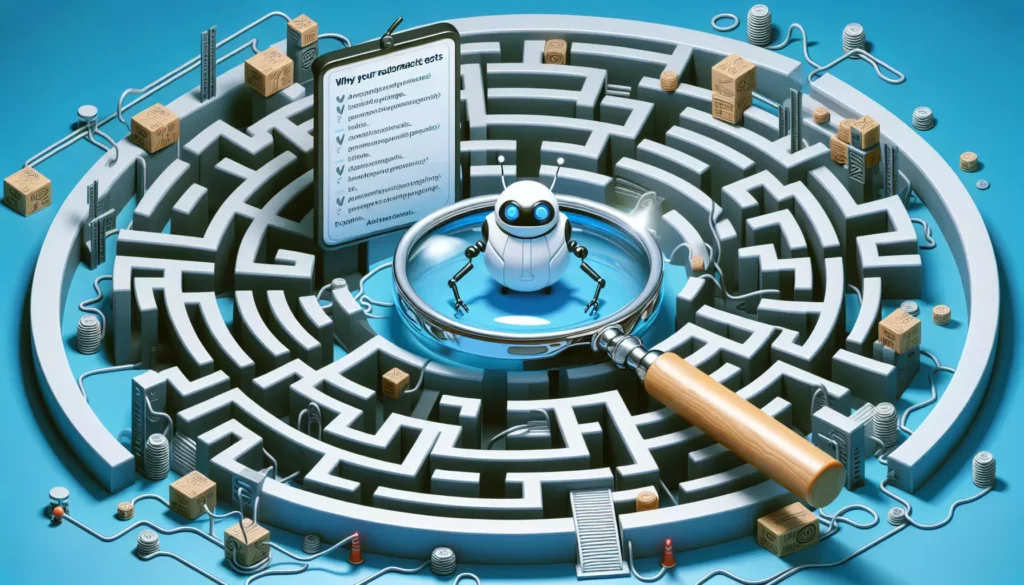
Automated testing is often touted as the silver bullet for software quality. Teams invest significant resources into building test suites with the expectation that bugs will be caught before they reach production. Yet, despite high test coverage and sophisticated testing frameworks, bugs continue to slip through. If you’ve ever wondered why your automated tests aren’t catching all the bugs, you’re not alone.
In this comprehensive guide, we’ll explore the common pitfalls in automated testing strategies and provide actionable solutions to make your testing more effective. Whether you’re preparing for technical interviews at top tech companies or working to improve your development practices, understanding these concepts will help you build more reliable software.
Table of Contents
- Understanding the Problem: Why Tests Fail to Catch Bugs
- Test Coverage Misconceptions
- Types of Bugs That Automated Tests Often Miss
- Common Test Design Issues
- Improving Test Effectiveness
- Beyond Unit Testing: A Comprehensive Testing Strategy
- Case Studies: Learning from Testing Failures
- Tools and Frameworks for Better Testing
- Building a Testing Culture
- Conclusion
Understanding the Problem: Why Tests Fail to Catch Bugs
Before diving into solutions, let’s understand why automated tests sometimes fail to catch bugs. This isn’t about poorly written tests (though that’s certainly a factor); it’s about fundamental limitations and misconceptions about what testing can accomplish.
The Fallacy of Complete Testing
One of the most pervasive myths in software development is the idea that we can test everything. Computer scientist Edsger W. Dijkstra famously noted that “Testing shows the presence, not the absence of bugs.” This fundamental truth highlights an important limitation: tests can only verify the specific scenarios they’re designed to check.
Consider a simple function that adds two numbers:
function add(a, b) {
return a + b;
}
To test this exhaustively would require checking every possible combination of inputs—an infinite set. Even with boundary testing and equivalence partitioning, we’re making assumptions about how the function behaves.
The Oracle Problem
For a test to be effective, we need to know what the correct outcome should be. This is known as the “test oracle problem.” In many real-world scenarios, determining the expected outcome is complex:
- For algorithmic problems, we might need to implement the solution twice (once in the production code, once in the test) to verify correctness
- For systems with emergent behavior, predicting all outcomes may be theoretically impossible
- For user interfaces, determining “correctness” often involves subjective human judgment
The Pesticide Paradox
Software testing expert Boris Beizer described the “pesticide paradox”: just as insects develop resistance to pesticides, software systems tend to develop “resistance” to tests. When we repeatedly run the same tests, they eventually stop finding new bugs because:
- Developers learn to avoid the specific mistakes that tests catch
- The codebase evolves to pass existing tests without necessarily becoming more robust
- New types of bugs emerge that existing tests weren’t designed to detect
Test Coverage Misconceptions
Many teams focus heavily on code coverage metrics, aiming for high percentages as proof of quality. However, coverage can be deeply misleading.
The 100% Coverage Myth
Even 100% code coverage doesn’t guarantee bug-free code. Consider this example:
function divideIfPositive(a, b) {
if (a > 0 && b > 0) {
return a / b;
}
return null;
}
// Test with 100% code coverage
test('divides positive numbers', () => {
expect(divideIfPositive(10, 2)).toBe(5);
});
test('returns null for negative inputs', () => {
expect(divideIfPositive(-1, 5)).toBeNull();
});
These tests achieve 100% code coverage but miss a critical bug: division by zero when b
is 0. The coverage metric doesn’t tell us about the quality of our test cases, only that each line executed at least once.
Path Coverage vs. Line Coverage
Line coverage (the most commonly used metric) only tells us if each line executed, not whether all possible paths through the code were tested. Consider this function:
function complexCondition(a, b, c) {
if ((a && b) || c) {
return 'condition met';
}
return 'condition not met';
}
This simple function has four possible paths:
- a=true, b=true (c doesn’t matter)
- a=true, b=false, c=true
- a=false, b=true, c=true (actually, this is redundant with #1)
- a=false, b=false, c=false
A single test case could hit 100% line coverage while testing only one path.
Meaningful Coverage
More important than raw coverage numbers is meaningful coverage: testing the right things. This includes:
- Edge cases and boundary conditions
- Error handling paths
- Business-critical functionality
- Complex algorithmic logic
Quality over quantity is the key principle here. Five well-designed tests may be more effective than 50 superficial ones.
Types of Bugs That Automated Tests Often Miss
Understanding the categories of bugs that frequently evade detection can help us design better testing strategies.
Integration Bugs
Unit tests excel at verifying that individual components work correctly in isolation but often miss issues that arise when components interact. Common integration bugs include:
- Data format mismatches between components
- Timing and race conditions
- Resource contention issues
- Conflicting assumptions about shared state
For example, component A might expect dates in ISO format, while component B provides them in Unix timestamp format. Each component works correctly according to its unit tests, but they fail when connected.
Environment-Specific Bugs
Tests typically run in controlled environments that may differ significantly from production:
- Different operating systems or browser versions
- Network latency and reliability differences
- Database scaling issues
- Cloud provider implementation details
A classic example is code that works in development but fails in production due to different file path conventions between Windows and Linux.
State and Order Dependency Bugs
Many tests assume a clean slate for each test case, but real-world usage involves complex state transitions:
- Tests that pass individually may fail when run together
- Bugs that only appear after specific sequences of operations
- Memory leaks and resource exhaustion that accumulate over time
Consider a shopping cart implementation. Individual tests for “add item” and “remove item” might pass, but using them together in certain sequences might reveal bugs like incorrect total calculations.
Concurrency and Race Conditions
Concurrency issues are notoriously difficult to test because they often depend on precise timing:
// A seemingly harmless counter implementation
let counter = 0;
function incrementCounter() {
const current = counter;
// Imagine some delay here (e.g., network call)
counter = current + 1;
}
// If two threads call this simultaneously, we might lose an increment
Standard unit tests will almost never catch this issue because they run sequentially, and even concurrent tests may not hit the exact timing needed to expose the bug.
User Experience and Usability Bugs
Automated tests typically verify functional correctness but miss usability issues:
- Confusing UI elements
- Performance perceived as slow by users
- Accessibility problems
- Mobile-specific interaction issues
These issues often require human evaluation or specialized testing approaches.
Common Test Design Issues
Even when we target the right types of bugs, poor test design can undermine effectiveness.
Brittle Tests
Brittle tests break frequently due to minor, non-functional changes in the code:
// Brittle test
test('user profile displays correctly', () => {
const wrapper = mount(<UserProfile />);
expect(wrapper.find('div.profile-container h2.username').text()).toBe('John Doe');
});
This test is tightly coupled to implementation details like CSS class names and DOM structure. When designers change the markup, the test breaks even though the functionality remains correct.
Test Doubles Gone Wrong
Mocks, stubs, and other test doubles are powerful tools but can lead to false confidence:
test('fetches user data', async () => {
// Mock API response
api.getUser = jest.fn().mockResolvedValue({ name: 'John', age: 30 });
const user = await UserService.fetchUser(123);
expect(user.name).toBe('John');
});
This test verifies that our code correctly processes the API response, but it doesn’t verify that we’re making the correct API call or handling API errors properly. If the API contract changes, our tests will continue to pass while production fails.
Testing Implementation Details
Tests should verify behavior, not implementation. Consider:
class ShoppingCart {
constructor() {
this.items = [];
}
addItem(item) {
this.items.push(item);
}
getItemCount() {
return this.items.length;
}
}
// Implementation detail test
test('addItem adds to internal items array', () => {
const cart = new ShoppingCart();
cart.addItem({ id: 1, name: 'Product' });
expect(cart.items.length).toBe(1);
});
// Behavior test
test('getItemCount returns correct count after adding item', () => {
const cart = new ShoppingCart();
cart.addItem({ id: 1, name: 'Product' });
expect(cart.getItemCount()).toBe(1);
});
The first test breaks encapsulation by accessing the private items
array. If we later refactor to use a different data structure, the test will fail even though the behavior is unchanged. The second test focuses on the observable behavior and will continue to pass through refactoring.
Overlooking Test Maintenance
As codebases evolve, tests require maintenance. Common issues include:
- Outdated tests that no longer reflect current requirements
- Redundant tests that slow down the test suite without adding value
- Missing tests for new functionality
Test maintenance should be an integral part of the development process, not an afterthought.
Improving Test Effectiveness
Now that we understand the problems, let’s explore strategies to make automated tests more effective at catching bugs.
Test-Driven Development (TDD)
TDD isn’t just about writing tests first; it’s a design methodology that leads to more testable code:
- Write a failing test that defines the desired behavior
- Implement the simplest code that makes the test pass
- Refactor to improve design while keeping tests green
This approach ensures that code is designed to be testable from the start and that tests verify behavior rather than implementation details.
Property-Based Testing
Rather than specifying individual test cases, property-based testing defines properties that should hold true for all inputs:
// Traditional example-based test
test('reverse reverses an array', () => {
expect(reverse([1, 2, 3])).toEqual([3, 2, 1]);
});
// Property-based test
testProp('reverse twice returns original array',
[gen.array(gen.int)],
(arr) => {
expect(reverse(reverse(arr))).toEqual(arr);
}
);
Property-based testing can explore a much wider range of inputs than manually specified examples, potentially uncovering edge cases you wouldn’t think to test.
Mutation Testing
Mutation testing evaluates the quality of your tests by introducing bugs (mutations) into your code and checking if tests catch them:
// Original code
function isPositive(num) {
return num > 0;
}
// Mutation 1: Change > to >=
function isPositive(num) {
return num >= 0;
}
// Mutation 2: Change > to <
function isPositive(num) {
return num < 0;
}
If your tests pass despite these mutations, they’re not sensitive enough to detect these changes in behavior. Tools like Stryker and PITest automate this process.
Fuzz Testing
Fuzz testing involves providing random, unexpected, or malformed inputs to find crashes and vulnerabilities:
function processUserInput(input) {
// Production code that parses and processes input
}
// Fuzz testing
for (let i = 0; i < 10000; i++) {
const randomInput = generateRandomInput();
try {
processUserInput(randomInput);
} catch (error) {
console.log(`Found bug with input: ${randomInput}`);
console.log(error);
}
}
This approach is particularly valuable for finding security vulnerabilities and robustness issues.
Test Prioritization
Not all tests are equally valuable. Prioritize testing efforts based on:
- Risk: Focus on code with the highest potential impact if bugs occur
- Complexity: Complex algorithms are more likely to contain bugs
- Change frequency: Code that changes often needs more testing
- Bug history: Areas with previous bugs tend to have more bugs
This doesn’t mean ignoring low-priority areas, but allocating testing resources proportionally to risk.
Beyond Unit Testing: A Comprehensive Testing Strategy
Unit tests alone cannot catch all bugs. A comprehensive strategy includes multiple testing types.
Integration Testing
Integration tests verify that components work together correctly:
// Integration test for user registration flow
test('user registration end-to-end', async () => {
// Test that database, authentication service, and email service
// all work together correctly
const user = await userService.register({
email: 'test@example.com',
password: 'password123'
});
// Verify user was created in database
const dbUser = await db.findUserByEmail('test@example.com');
expect(dbUser).not.toBeNull();
// Verify welcome email was sent
expect(emailService.sentEmails).toContainEqual({
to: 'test@example.com',
subject: 'Welcome to Our Service'
});
});
These tests are more complex to set up but catch issues that unit tests miss.
End-to-End Testing
E2E tests simulate real user interactions across the entire application:
// E2E test with Cypress
describe('Shopping cart', () => {
it('allows adding products and checking out', () => {
cy.visit('/products');
cy.contains('Product A').click();
cy.contains('Add to Cart').click();
cy.visit('/cart');
cy.contains('Product A').should('be.visible');
cy.contains('Checkout').click();
cy.url().should('include', '/checkout');
// Fill out checkout form and complete purchase
});
});
These tests are slower and more brittle than unit tests but provide confidence that the system works as a whole.
Contract Testing
Contract tests verify that services adhere to their API contracts:
// Consumer-driven contract test
pact
.given('a user exists')
.uponReceiving('a request for user details')
.withRequest({
method: 'GET',
path: '/api/users/123'
})
.willRespondWith({
status: 200,
headers: { 'Content-Type': 'application/json' },
body: {
id: 123,
name: Matchers.string('John Doe'),
email: Matchers.email()
}
});
Contract testing is particularly valuable in microservices architectures where services evolve independently.
Performance Testing
Performance tests verify that the system meets performance requirements:
- Load testing: How does the system handle expected load?
- Stress testing: At what point does the system break?
- Endurance testing: How does the system perform over time?
- Spike testing: How does the system handle sudden increases in load?
Tools like JMeter, Gatling, and k6 can automate these tests.
Chaos Engineering
Chaos engineering involves deliberately introducing failures to verify system resilience:
- Network partitions
- Service failures
- Resource exhaustion
- Clock skew
Netflix’s Chaos Monkey is a famous example of this approach, randomly terminating instances to ensure the system can handle failures gracefully.
Case Studies: Learning from Testing Failures
Real-world examples provide valuable insights into testing challenges.
The Mars Climate Orbiter Disaster
In 1999, NASA lost the $125 million Mars Climate Orbiter due to a unit conversion error. One team used metric units while another used imperial units. Despite extensive testing, this integration issue wasn’t caught because:
- Teams tested their components in isolation
- Tests verified that calculations worked correctly within each system’s assumptions
- Integration tests didn’t verify the correctness of the data exchange, only that data was exchanged
The lesson: Test not just that components communicate, but that they communicate correctly.
Knight Capital’s $440 Million Bug
In 2012, Knight Capital lost $440 million in 45 minutes due to a software deployment error. The issue involved:
- Reusing a flag in a configuration file for a new purpose
- Deploying new code to 7 of 8 servers, creating inconsistent behavior
- No automated verification of the deployment process
The lesson: Test deployment processes and configuration changes, not just application code.
Therac-25 Radiation Therapy Machine
The Therac-25 radiation therapy machine was involved in at least six accidents between 1985 and 1987, delivering lethal radiation doses to patients. The issues included:
- Race conditions that only occurred with very specific timing of operator actions
- Overreliance on software controls without hardware backups
- Reuse of code from previous models without understanding its assumptions
The lesson: Test for race conditions and edge cases, especially in safety-critical systems.
Tools and Frameworks for Better Testing
The right tools can significantly improve testing effectiveness.
Testing Frameworks
Different frameworks excel at different types of testing:
- Unit testing: Jest, JUnit, NUnit, pytest
- Integration testing: Testcontainers, Spring Test
- E2E testing: Cypress, Playwright, Selenium
- API testing: Postman, REST Assured, Pact
- Performance testing: JMeter, k6, Gatling
Choose frameworks that match your technology stack and testing needs.
Test Generators and Property Testing Tools
Tools for generating test cases can find edge cases you might miss:
- fast-check (JavaScript)
- jqwik (Java)
- Hypothesis (Python)
- QuickCheck (Haskell, with ports to many languages)
Static Analysis Tools
Static analysis can find bugs without executing code:
- ESLint/TSLint (JavaScript/TypeScript)
- SonarQube (multi-language)
- FindBugs/SpotBugs (Java)
- Pylint (Python)
These tools catch issues like potential null pointer exceptions, resource leaks, and security vulnerabilities.
Code Coverage Tools
While coverage isn’t everything, it helps identify untested areas:
- Istanbul/NYC (JavaScript)
- JaCoCo (Java)
- Coverage.py (Python)
- Coverlet (.NET)
Use these tools to identify gaps in your testing, not as the sole measure of quality.
Continuous Integration (CI) Systems
CI systems automate test execution and reporting:
- GitHub Actions
- Jenkins
- CircleCI
- Travis CI
Configure these to run different types of tests at appropriate stages of development.
Building a Testing Culture
Tools and techniques are important, but culture is the foundation of effective testing.
Making Testing a Shared Responsibility
Testing isn’t just for QA teams; it’s everyone’s responsibility:
- Developers should write and maintain tests for their code
- Product managers should define acceptance criteria that can be tested
- DevOps engineers should ensure testability of infrastructure
- QA specialists should focus on exploratory testing and test strategy
This shared ownership improves both the quality and relevance of tests.
Test Reviews
Just as we review code, we should review tests:
- Are tests testing the right things?
- Are edge cases covered?
- Are tests maintainable?
- Do tests provide meaningful feedback when they fail?
Test reviews catch issues that individual developers might miss.
Learning from Failures
When bugs slip through testing, treat it as a learning opportunity:
- Conduct blameless postmortems
- Add regression tests for each discovered bug
- Update testing strategies based on patterns of missed bugs
This continuous improvement cycle is essential for effective testing.
Measuring the Right Things
The metrics we track influence behavior. Focus on meaningful metrics:
- Escaped defects (bugs found in production)
- Test effectiveness (percentage of introduced bugs caught by tests)
- Mean time to detect issues
- Test maintenance cost
Avoid overemphasizing metrics like raw test count or coverage percentage.
Conclusion
Automated testing is a powerful tool for improving software quality, but it’s not a silver bullet. By understanding the limitations of testing and implementing a comprehensive strategy that goes beyond simple unit tests, you can significantly reduce the number of bugs that reach production.
Remember these key principles:
- No single type of testing can catch all bugs; use a diverse testing strategy
- Focus on testing behavior rather than implementation details
- Prioritize testing based on risk and complexity
- Build a culture where quality is everyone’s responsibility
- Learn from failures and continuously improve your testing approach
By applying these principles, you’ll not only catch more bugs before they reach users but also build more maintainable, robust software systems. And if you’re preparing for technical interviews at top tech companies, demonstrating this deep understanding of testing principles will set you apart as a developer who cares about quality.
What testing challenges is your team facing? Start a conversation about how you might apply these principles to address them, and remember that effective testing is a journey of continuous improvement.