Why Your Authentication System Has Security Holes
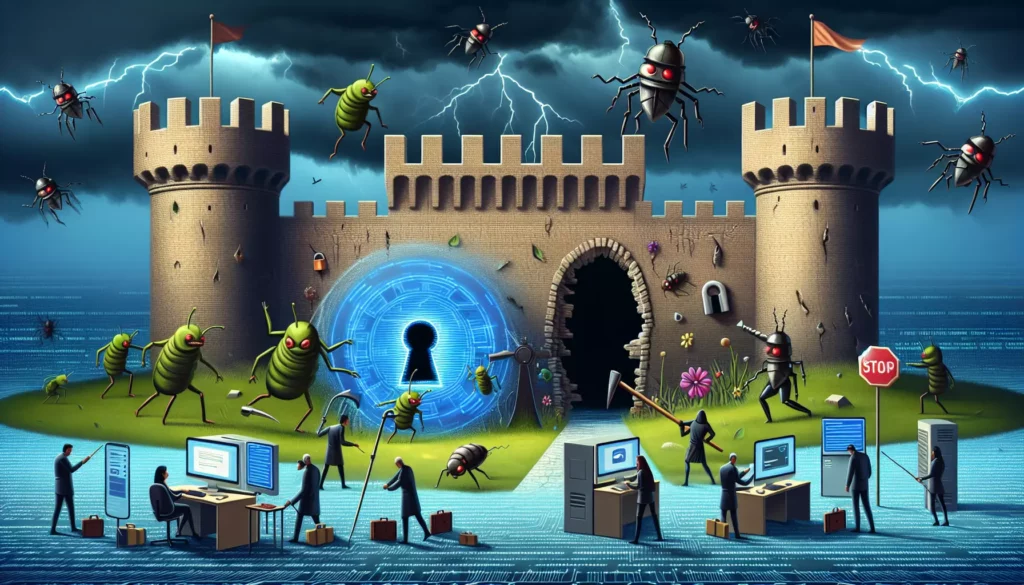
Authentication is the cornerstone of digital security, serving as the first line of defense against unauthorized access. Yet, many authentication systems contain critical vulnerabilities that can compromise user data and system integrity. In this comprehensive guide, we’ll explore common authentication security holes, their implications, and practical solutions to strengthen your authentication mechanisms.
Table of Contents
- Understanding Authentication: Beyond Username and Password
- Common Authentication Vulnerabilities
- Password Storage and Management Pitfalls
- Session Management Vulnerabilities
- Implementation Errors That Create Security Holes
- Social Engineering and Human Factor Vulnerabilities
- Multi-Factor Authentication: Not a Silver Bullet
- Authentication Security Best Practices
- Code Examples: Secure vs. Vulnerable Authentication
- The Future of Authentication
- Conclusion
Understanding Authentication: Beyond Username and Password
Authentication is the process of verifying that users are who they claim to be. While most people understand this as entering a username and password, authentication encompasses much more:
- Knowledge factors: Something the user knows (passwords, PINs, security questions)
- Possession factors: Something the user has (security tokens, mobile devices, smart cards)
- Inherence factors: Something the user is (biometrics like fingerprints, facial recognition)
- Location factors: Where the user is accessing from (geolocation)
- Behavioral factors: How the user interacts with the system (typing patterns, navigation habits)
A robust authentication system often combines multiple factors, creating layers of security that are harder to breach. However, each authentication method comes with its own set of potential vulnerabilities that attackers can exploit.
Common Authentication Vulnerabilities
Brute Force Attacks
Brute force attacks involve systematic attempts to guess authentication credentials. These attacks remain prevalent because they’re conceptually simple and can be effective against systems without proper protections.
Common vulnerabilities include:
- No account lockout policies after multiple failed attempts
- No rate limiting on login attempts
- No CAPTCHA or similar mechanisms to prevent automated attacks
- No monitoring systems to detect unusual login patterns
Real-world impact: In 2019, a brute force attack against Dunkin’ Donuts’ loyalty program allowed attackers to take over customer accounts and steal stored value, affecting thousands of customers.
Credential Stuffing
Credential stuffing leverages the common practice of password reuse across multiple sites. Attackers use leaked credentials from one breach to attempt access to other services.
Why it works:
- Studies show 65% of people reuse passwords across multiple sites
- Over 15 billion credentials have been exposed in data breaches
- Automated tools make testing millions of username/password combinations efficient
Real-world impact: In 2020, Nintendo reported that over 300,000 accounts were compromised through credential stuffing, giving attackers access to payment information and personal details.
Man-in-the-Middle (MITM) Attacks
MITM attacks occur when an attacker intercepts communication between a user and the authentication system, allowing them to steal credentials or session tokens.
Common vulnerabilities include:
- Lack of HTTPS implementation for login pages
- Improper certificate validation
- Using HTTP for parts of an authenticated session
- Insecure Wi-Fi networks that allow packet sniffing
Even in 2023, some applications still transmit authentication credentials over unencrypted channels or implement HTTPS incorrectly, creating opportunities for interception.
Password Storage and Management Pitfalls
Insecure Password Storage
The way passwords are stored can make or break your security posture. Common mistakes include:
- Plaintext storage: Storing passwords without any encryption
- Weak hashing algorithms: Using outdated algorithms like MD5 or SHA-1
- Hashing without salting: Making passwords vulnerable to rainbow table attacks
- Using the same salt for all passwords: Reducing the effectiveness of the salt
Consider the 2012 LinkedIn breach where 6.5 million unsalted SHA-1 password hashes were leaked. Despite SHA-1 being considered secure at the time, many passwords were quickly cracked due to the lack of salting.
Code Example: Insecure vs. Secure Password Storage
Insecure password storage (PHP):
// INSECURE: Direct MD5 hashing without salt
function storePassword($username, $password) {
$hashedPassword = md5($password); // Weak, fast, and unsalted hash
// Store in database
$query = "INSERT INTO users (username, password) VALUES ('$username', '$hashedPassword')";
// Execute query...
}
Secure password storage (PHP):
// SECURE: Using PHP's password_hash function (bcrypt by default)
function storePassword($username, $password) {
// Automatically generates a strong salt and uses a strong algorithm
$hashedPassword = password_hash($password, PASSWORD_DEFAULT, ['cost' => 12]);
// Store in database using prepared statements
$stmt = $pdo->prepare("INSERT INTO users (username, password) VALUES (?, ?)");
$stmt->execute([$username, $hashedPassword]);
}
Password Policy Problems
Many organizations implement counterproductive password policies that actually weaken security:
- Excessive complexity requirements that lead users to create predictable patterns
- Frequent mandatory changes that result in minor variations of previous passwords
- Short maximum length limits that prevent the use of strong passphrases
- Character type restrictions that limit password strength and frustrate users
The NIST Special Publication 800-63B now recommends against many traditional password policies, suggesting instead:
- Longer minimum lengths (8+ characters)
- Checking passwords against lists of compromised credentials
- Allowing all printable ASCII characters and spaces
- Only requiring password changes when there’s evidence of compromise
Session Management Vulnerabilities
Even with secure authentication, poor session management can create critical security holes.
Insecure Session Tokens
Session tokens are the keys to authenticated sessions. Common vulnerabilities include:
- Predictable token generation: Using algorithms that produce guessable tokens
- Insufficient entropy: Not using enough random data in token generation
- Exposed tokens: Sending tokens in URLs where they can be leaked via referrer headers
- Insecure storage: Storing tokens in insecure cookies or localStorage
A properly secured session token should be:
- Generated using cryptographically secure random number generators
- At least 128 bits of entropy
- Stored in HttpOnly, Secure cookies with appropriate SameSite attributes
- Transmitted only over encrypted connections
Code Example: Secure Session Token Generation
// Node.js secure session token generation
const crypto = require('crypto');
function generateSecureToken(length = 32) {
return crypto.randomBytes(length).toString('hex');
}
// Usage
const sessionToken = generateSecureToken();
// Set cookie with proper attributes
res.cookie('sessionId', sessionToken, {
httpOnly: true, // Prevents JavaScript access
secure: true, // Only sent over HTTPS
sameSite: 'strict', // Prevents CSRF
maxAge: 3600000 // 1 hour expiration
});
Insufficient Session Expiration
Sessions that remain valid for too long increase the risk of session hijacking. Issues include:
- No absolute timeout (sessions that never expire)
- No idle timeout (sessions that remain active despite inactivity)
- No mechanism to invalidate all sessions after password changes
- No way to track or limit concurrent sessions
Balancing security with user experience requires thoughtful session timeout policies:
- Short timeouts for sensitive operations (financial transactions, admin functions)
- Reasonable idle timeouts based on application risk profile
- Clear user notification about session status
- Re-authentication for critical actions
Cross-Site Request Forgery (CSRF)
CSRF attacks trick authenticated users into executing unwanted actions. Authentication systems are vulnerable when they:
- Rely solely on cookies for authentication without additional verification
- Don’t implement anti-CSRF tokens for state-changing operations
- Use predictable or reusable anti-CSRF tokens
- Don’t validate the origin of requests
Modern protections include:
- Implementing SameSite cookie attributes
- Using synchronized token patterns
- Checking Origin and Referer headers
- Requiring user interaction for sensitive operations
Implementation Errors That Create Security Holes
SQL Injection in Authentication
SQL injection remains one of the most dangerous vulnerabilities, especially in authentication contexts where it can bypass login requirements entirely.
Consider this vulnerable login code:
// VULNERABLE to SQL injection
function login($username, $password) {
$query = "SELECT * FROM users WHERE username='$username' AND password='$password'";
$result = mysqli_query($connection, $query);
if(mysqli_num_rows($result) > 0) {
// Login successful
return true;
}
return false;
}
An attacker could input admin' --
as the username and any password, resulting in the query:
SELECT * FROM users WHERE username='admin' --' AND password='anything'
The --
comments out the password check, granting access with just a known username.
Secure implementation using prepared statements:
// SECURE against SQL injection
function login($username, $password) {
$stmt = $connection->prepare("SELECT * FROM users WHERE username = ?");
$stmt->bind_param("s", $username);
$stmt->execute();
$result = $stmt->get_result();
if($row = $result->fetch_assoc()) {
// Verify password using secure comparison
if(password_verify($password, $row['password'])) {
return true;
}
}
return false;
}
Insecure Direct Object References
Authentication systems often use identifiers that, if exposed, allow attackers to access unauthorized resources.
Example vulnerability:
// VULNERABLE: User ID directly exposed in URL
// https://example.com/account?id=1234
function getUserData(request) {
$userId = request.getParameter("id");
return database.query("SELECT * FROM users WHERE id = " + userId);
}
An attacker could simply change the ID parameter to access other users’ data.
Secure implementation:
// SECURE: Using session to store authenticated user's ID
function getUserData(request, session) {
// Only get the authenticated user's own data
$userId = session.getAuthenticatedUserId();
if (!userId) {
return unauthorized();
}
return database.query("SELECT * FROM users WHERE id = ?", [userId]);
}
Race Conditions in Authentication Logic
Race conditions occur when the timing of operations affects the correctness of the program. In authentication contexts, they can lead to security bypasses.
A common example is in account lockout mechanisms:
// VULNERABLE to race condition
function checkLoginAttempts($username) {
$attempts = getLoginAttempts($username);
if($attempts >= 5) {
lockAccount($username);
return false;
}
// Attacker can make multiple parallel requests before this executes
incrementLoginAttempts($username);
return true;
}
Secure implementation using atomic operations:
// SECURE against race conditions
function checkLoginAttempts($username) {
// Atomic increment and check in a single database operation
$attempts = atomicIncrementAndGetLoginAttempts($username);
if($attempts > 5) {
lockAccount($username);
return false;
}
return true;
}
Social Engineering and Human Factor Vulnerabilities
Phishing Vulnerabilities
Even the most technically secure authentication system can be compromised through phishing. Authentication systems are vulnerable when they:
- Lack visual indicators that help users identify legitimate login pages
- Don’t implement anti-phishing measures like site-specific images
- Use generic email templates that are easy to imitate
- Don’t educate users about phishing techniques
Tech giants like Google and Microsoft have significantly reduced successful phishing attacks by implementing hardware security keys, which verify the authenticity of the login site cryptographically.
Social Engineering in Account Recovery
Account recovery processes often create backdoors into otherwise secure authentication systems:
- Weak security questions with answers that can be researched or guessed
- SMS-based recovery vulnerable to SIM swapping attacks
- Email-based recovery that creates a single point of failure
- Customer support agents who can be manipulated into resetting accounts
The infamous 2016 hack of John Podesta’s email began with a simple phishing email that appeared to come from Google, demonstrating how even high-profile targets can fall victim to these attacks.
Insider Threats
Authentication systems often overlook threats from within:
- Administrators with excessive access to authentication data
- Lack of audit trails for credential management actions
- Shared admin accounts without individual accountability
- No separation of duties for critical authentication functions
Mitigation strategies include:
- Implementing the principle of least privilege
- Creating comprehensive audit logs
- Requiring multi-person authorization for critical actions
- Regular review of access privileges
Multi-Factor Authentication: Not a Silver Bullet
While multi-factor authentication (MFA) significantly improves security, it’s not immune to vulnerabilities:
SMS and Email-Based MFA Weaknesses
- SIM swapping attacks: Attackers convince mobile carriers to transfer a victim’s phone number to a new SIM
- SS7 network vulnerabilities: Allow interception of SMS messages
- Email account compromises: If email is used as a factor, compromising email defeats MFA
- Delay problems: SMS delivery delays can frustrate users and lead to fallback authentication
In 2019, Twitter CEO Jack Dorsey’s account was compromised through a SIM swapping attack, highlighting that even tech leaders can fall victim to these attacks.
TOTP Application Vulnerabilities
Time-based One-Time Password (TOTP) apps like Google Authenticator improve upon SMS, but still have weaknesses:
- Device loss/theft exposes all stored TOTP seeds
- Malware on devices can steal TOTP seeds or intercept generated codes
- Backup processes for TOTP seeds often create security vulnerabilities
- Weak implementation of the TOTP algorithm (time drift issues, insufficient entropy)
A secure TOTP implementation should:
- Use cryptographically secure random number generators for seed creation
- Implement proper time synchronization
- Use appropriate OTP length (at least 6 digits)
- Have secure backup and recovery processes
Push Notification MFA Bypass
Push notification-based MFA (like Duo Push or Microsoft Authenticator) can be vulnerable to:
- MFA fatigue attacks: Bombarding users with authentication requests until they accidentally approve
- Social engineering: Convincing users to approve push notifications
- Lost/stolen devices: Physical access to the authentication device
- Man-in-the-middle attacks on the push notification channel
In 2022, Uber suffered a major breach where an attacker used MFA fatigue to convince an employee to accept an authentication request, granting access to critical internal systems.
Authentication Security Best Practices
Secure Password Management
- Use modern hashing algorithms designed for passwords (bcrypt, Argon2, PBKDF2)
- Implement proper salting practices with unique salts per password
- Set appropriate work factors that balance security and performance
- Check passwords against known breached password lists during registration and changes
- Encourage password managers to help users create and store strong, unique passwords
- Implement NIST-compliant password policies that focus on length over complexity
Robust MFA Implementation
- Offer multiple MFA options to accommodate different user needs
- Prioritize stronger factors like security keys (FIDO2/WebAuthn)
- Implement anti-automation measures for MFA to prevent brute force attacks
- Create secure MFA recovery processes that don’t create backdoors
- Consider risk-based MFA that adapts to user behavior and context
Secure Session Management
- Generate cryptographically secure session identifiers with sufficient entropy
- Implement proper cookie attributes (HttpOnly, Secure, SameSite)
- Create appropriate session timeout policies based on sensitivity
- Invalidate sessions properly on logout and password changes
- Implement mechanisms to detect and prevent session hijacking
Defense in Depth
- Implement rate limiting on authentication attempts
- Use CAPTCHAs or similar challenges to prevent automated attacks
- Create robust logging and monitoring for authentication events
- Implement account lockout policies that balance security and usability
- Use threat intelligence to block known malicious IP addresses
Code Examples: Secure vs. Vulnerable Authentication
Secure Authentication Flow (Node.js/Express)
const express = require('express');
const bcrypt = require('bcrypt');
const crypto = require('crypto');
const rateLimit = require('express-rate-limit');
const app = express();
// Rate limiting middleware
const loginLimiter = rateLimit({
windowMs: 15 * 60 * 1000, // 15 minutes
max: 5, // 5 attempts per window
message: 'Too many login attempts, please try again after 15 minutes'
});
// Secure user registration
app.post('/register', async (req, res) => {
try {
const { username, password, email } = req.body;
// Check password strength
if (password.length < 12) {
return res.status(400).json({ error: 'Password must be at least 12 characters' });
}
// Check against common passwords
if (isCommonPassword(password)) {
return res.status(400).json({ error: 'This password is commonly used and vulnerable' });
}
// Generate salt and hash
const salt = await bcrypt.genSalt(12);
const hashedPassword = await bcrypt.hash(password, salt);
// Store user in database with prepared statements
// db.query('INSERT INTO users (username, password, email) VALUES (?, ?, ?)',
// [username, hashedPassword, email]);
res.status(201).json({ message: 'User registered successfully' });
} catch (error) {
res.status(500).json({ error: 'Registration failed' });
}
});
// Secure login with rate limiting
app.post('/login', loginLimiter, async (req, res) => {
try {
const { username, password } = req.body;
// Get user from database (using prepared statements)
// const user = await db.query('SELECT * FROM users WHERE username = ?', [username]);
const user = { id: 1, username: 'test', password: '$2b$12$K3JNm1rVUC7ZH/zfBTUbO.9CvGRUyXbgUOWGbdHBFiaSYnpIThXOi' }; // Demo
if (!user) {
// Use constant time comparison to prevent timing attacks
await bcrypt.compare(password, '$2b$12$K3JNm1rVUC7ZH/zfBTUbO.9CvGRUyXbgUOWGbdHBFiaSYnpIThXOi');
return res.status(401).json({ error: 'Invalid credentials' });
}
// Verify password
const passwordValid = await bcrypt.compare(password, user.password);
if (!passwordValid) {
return res.status(401).json({ error: 'Invalid credentials' });
}
// Generate secure session token
const sessionToken = crypto.randomBytes(64).toString('hex');
// Store session in database with expiration
// db.query('INSERT INTO sessions (user_id, token, expires_at) VALUES (?, ?, ?)',
// [user.id, sessionToken, new Date(Date.now() + 3600000)]);
// Set secure cookie
res.cookie('session', sessionToken, {
httpOnly: true,
secure: true,
sameSite: 'strict',
maxAge: 3600000 // 1 hour
});
res.json({ message: 'Login successful' });
} catch (error) {
res.status(500).json({ error: 'Login failed' });
}
});
// Helper function to check common passwords
function isCommonPassword(password) {
const commonPasswords = ['password123', '123456789', 'qwerty123'];
return commonPasswords.includes(password);
}
app.listen(3000, () => console.log('Server running on port 3000'));
Implementing WebAuthn/FIDO2 (Modern Authentication)
// Client-side WebAuthn registration (simplified)
async function registerNewCredential(username) {
// Request challenge from server
const response = await fetch('/webauthn/generate-registration-options', {
method: 'POST',
headers: { 'Content-Type': 'application/json' },
body: JSON.stringify({ username })
});
const options = await response.json();
// Convert base64 challenge to ArrayBuffer
options.challenge = base64urlToArrayBuffer(options.challenge);
// Create credentials with browser's API
const credential = await navigator.credentials.create({
publicKey: options
});
// Prepare credential data for server
const credentialData = {
id: credential.id,
rawId: arrayBufferToBase64url(credential.rawId),
response: {
clientDataJSON: arrayBufferToBase64url(credential.response.clientDataJSON),
attestationObject: arrayBufferToBase64url(credential.response.attestationObject)
},
type: credential.type
};
// Send to server for verification
await fetch('/webauthn/verify-registration', {
method: 'POST',
headers: { 'Content-Type': 'application/json' },
body: JSON.stringify({ credential: credentialData })
});
return credential;
}
// Client-side WebAuthn authentication (simplified)
async function authenticateWithCredential(username) {
// Request challenge from server
const response = await fetch('/webauthn/generate-authentication-options', {
method: 'POST',
headers: { 'Content-Type': 'application/json' },
body: JSON.stringify({ username })
});
const options = await response.json();
// Convert base64 challenge to ArrayBuffer
options.challenge = base64urlToArrayBuffer(options.challenge);
// Allow credentials array needs conversion
if (options.allowCredentials) {
options.allowCredentials = options.allowCredentials.map(credential => {
return {
id: base64urlToArrayBuffer(credential.id),
type: credential.type,
transports: credential.transports
};
});
}
// Get credentials with browser's API
const credential = await navigator.credentials.get({
publicKey: options
});
// Prepare assertion for server verification
const assertionData = {
id: credential.id,
rawId: arrayBufferToBase64url(credential.rawId),
response: {
clientDataJSON: arrayBufferToBase64url(credential.response.clientDataJSON),
authenticatorData: arrayBufferToBase64url(credential.response.authenticatorData),
signature: arrayBufferToBase64url(credential.response.signature),
userHandle: credential.response.userHandle ?
arrayBufferToBase64url(credential.response.userHandle) : null
},
type: credential.type
};
// Send to server for verification
await fetch('/webauthn/verify-authentication', {
method: 'POST',
headers: { 'Content-Type': 'application/json' },
body: JSON.stringify({ credential: assertionData })
});
return credential;
}
// Helper functions for ArrayBuffer/Base64URL conversion
function arrayBufferToBase64url(buffer) {
const bytes = new Uint8Array(buffer);
let str = '';
for (const byte of bytes) {
str += String.fromCharCode(byte);
}
return btoa(str).replace(/\+/g, '-').replace(/\//g, '_').replace(/=+$/, '');
}
function base64urlToArrayBuffer(base64url) {
const base64 = base64url.replace(/-/g, '+').replace(/_/g, '/');
const binStr = atob(base64);
const bytes = new Uint8Array(binStr.length);
for (let i = 0; i < binStr.length; i++) {
bytes[i] = binStr.charCodeAt(i);
}
return bytes.buffer;
}
The Future of Authentication
Passwordless Authentication
The industry is moving toward eliminating passwords entirely:
- FIDO2/WebAuthn: Open standards for passwordless authentication
- Passkeys: Apple, Google, and Microsoft's implementation of FIDO standards
- Biometric authentication: Increasingly accurate and secure
- Magic links and one-time codes: Simplifying user experience
These methods aim to eliminate the fundamental weakness of passwords: the need for users to remember them.
Continuous Authentication
Moving beyond point-in-time authentication to continuous verification:
- Behavioral biometrics: Analyzing typing patterns, mouse movements, and app interactions
- Device fingerprinting: Recognizing user devices through unique characteristics
- Contextual analysis: Evaluating location, time, and network information
- AI-based anomaly detection: Identifying unusual patterns that may indicate account takeover
These approaches provide security without constant user