Why Your API Design Is Not Future Proof
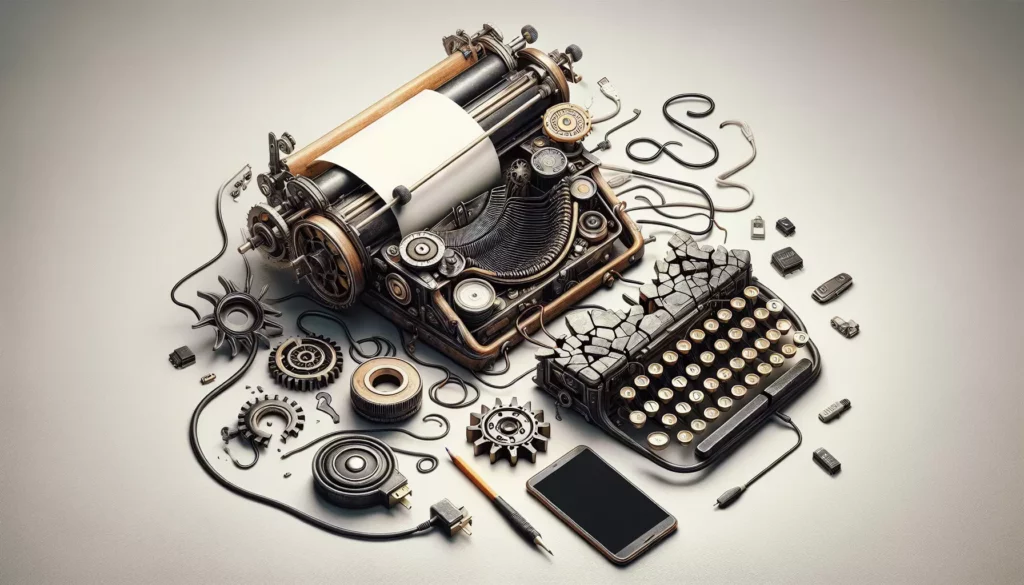
In the rapidly evolving world of software development, creating APIs that stand the test of time is a significant challenge. Many developers find themselves trapped in cycles of constant refactoring, versioning nightmares, and frustrated clients when their APIs fail to adapt to changing requirements. This comprehensive guide explores the common pitfalls in API design that compromise future proofing and provides actionable strategies to build more resilient interfaces.
Understanding the Importance of Future Proof API Design
APIs (Application Programming Interfaces) serve as the critical connectors in modern software ecosystems. They enable different systems to communicate and share data, functioning as contracts between services. When these contracts are poorly designed, the consequences can be far reaching and costly.
The Real Cost of Fragile API Design
Consider these sobering statistics:
- Companies spend up to 80% of their development resources maintaining existing systems rather than building new features
- API rewrites can cost organizations millions in development hours and lost opportunities
- According to industry surveys, over 60% of developers report significant challenges with API versioning and backward compatibility
The technical debt accumulated from shortsighted API design compounds over time, creating increasingly complex problems that become progressively more difficult to solve. Let’s examine the most common reasons APIs fail to stand the test of time.
Common Pitfalls That Undermine API Longevity
1. Exposing Implementation Details
One of the most prevalent mistakes in API design is leaking implementation details into the public interface. When internal structures and logic become part of your API contract, you severely limit your ability to evolve the underlying implementation.
Consider this problematic REST API endpoint:
GET /api/users/getUsersByMongoDBObjectId/507f1f77bcf86cd799439011
The issue? The endpoint directly exposes the underlying database technology (MongoDB) and its specific ID format. If the organization later decides to migrate to a different database system, they’ll face significant challenges maintaining compatibility.
A better approach would be:
GET /api/users/507f1f77bcf86cd799439011
This endpoint abstracts away the implementation details, providing flexibility to change the underlying technology without breaking the API contract.
2. Inadequate Versioning Strategy
Many APIs begin without a clear versioning strategy, making it difficult to introduce changes while maintaining backward compatibility. Without versioning, even minor modifications can break existing client integrations.
Common versioning approaches include:
- URL Path Versioning:
/api/v1/users
- Query Parameter Versioning:
/api/users?version=1
- Header Versioning: Using custom headers like
Accept-Version: 1.0
- Content Negotiation: Using the Accept header, e.g.,
Accept: application/vnd.company.api.v1+json
Each approach has tradeoffs, but the absence of any versioning strategy virtually guarantees future compatibility problems.
3. Tight Coupling to Specific Use Cases
APIs designed around specific client needs or current use cases often struggle to adapt when new requirements emerge. This problem manifests in endpoint designs that are too specific or data models that reflect a single application’s perspective.
Consider this overly specific endpoint:
GET /api/dashboard/topSellingProductsForMobileApp
This design couples the API to a particular client (mobile app) and a specific use case (dashboard display). A more adaptable approach would be:
GET /api/products/stats?metric=sales&order=desc&limit=10
This design allows any client to retrieve product sales statistics with flexible parameters, accommodating a wider range of current and future use cases.
4. Ignoring Backward Compatibility
Breaking changes in APIs create significant friction for consumers. Each change that requires clients to update their integration represents a potential point of failure and adoption barrier.
Common backward compatibility violations include:
- Removing fields from response payloads
- Changing field types or formats
- Altering URL structures
- Modifying authentication mechanisms without transition periods
Maintaining backward compatibility requires discipline and foresight, but pays dividends in client satisfaction and reduced support burden.
5. Insufficient Documentation and Examples
Even well designed APIs can fail if developers cannot understand how to use them correctly. Poor documentation leads to misuse, workarounds, and dependencies on undocumented behaviors that complicate future changes.
Modern API documentation should include:
- Clear descriptions of all endpoints, parameters, and response structures
- Interactive examples (like Swagger/OpenAPI interfaces)
- Code samples in multiple languages
- Use case examples and best practices
- Detailed error information and troubleshooting guidance
Documentation is not merely a reference but a critical component of the API’s usability and longevity.
Strategies for Building Future Proof APIs
Now that we’ve identified common pitfalls, let’s explore practical strategies to create APIs that can evolve gracefully over time.
1. Embrace the API First Approach
The API first approach inverts the traditional development process by designing the API contract before implementing the underlying functionality. This methodology forces teams to thoroughly consider the interface from the consumer’s perspective.
Key principles of API first design include:
- Designing for consumption, not implementation
- Creating machine readable API specifications (like OpenAPI)
- Validating designs with stakeholders before implementation
- Using the API specification as a contract between teams
By defining clear boundaries upfront, API first design reduces the likelihood of leaking implementation details or creating tightly coupled interfaces.
2. Implement Robust Versioning
While opinions differ on the best versioning approach, having a consistent strategy is non negotiable for future proof APIs. Whatever method you choose, consider these principles:
- Make versioning explicit and consistent across all endpoints
- Maintain support for previous versions with clear deprecation policies
- Document migration paths between versions
- Consider semantic versioning (MAJOR.MINOR.PATCH) principles even for REST APIs
A practical approach is to use URL path versioning for major changes (/v1/
, /v2/
) while handling minor changes through backward compatible extensions.
3. Design for Extensibility
Extensible APIs can accommodate new features without breaking existing functionality. Several patterns support this goal:
The Envelope Pattern
Wrap response data in a container object that can evolve:
{
"data": {
"id": 123,
"name": "Product XYZ"
},
"meta": {
"version": "1.2",
"deprecation": null
}
}
This pattern allows for adding metadata fields without affecting the core payload structure that clients depend on.
The Optional Fields Pattern
Make new fields optional and provide sensible defaults:
// Original v1 request
{
"name": "John Doe",
"email": "john@example.com"
}
// Extended v1 request with optional fields
{
"name": "John Doe",
"email": "john@example.com",
"preferences": {
"theme": "dark",
"notifications": true
}
}
By making new fields optional, existing clients can continue using the API without modification.
The Query Parameter Expansion Pattern
Use query parameters to control response detail levels:
GET /api/products/123 # Basic information
GET /api/products/123?expand=reviews # Include reviews
GET /api/products/123?expand=reviews,related,inventory
This pattern allows clients to request exactly the data they need while enabling the API to evolve with new expandable fields.
4. Implement Hypermedia Controls (HATEOAS)
Hypermedia as the Engine of Application State (HATEOAS) is a constraint of REST architecture that decouples clients from specific URL structures. With HATEOAS, responses include links that guide clients to available actions:
{
"id": 123,
"status": "pending",
"links": {
"self": "/orders/123",
"approve": "/orders/123/approve",
"cancel": "/orders/123/cancel",
"customer": "/customers/456"
}
}
This approach allows server side URL structures to evolve without breaking clients, as they follow the provided links rather than constructing URLs themselves.
5. Use Appropriate HTTP Methods and Status Codes
RESTful APIs should leverage the semantics of HTTP methods and status codes to create predictable interfaces:
- GET for retrieving resources (safe, idempotent)
- POST for creating resources or complex operations
- PUT for replacing resources (idempotent)
- PATCH for partial updates (should be idempotent)
- DELETE for removing resources (idempotent)
Similarly, use standard HTTP status codes consistently:
- 2xx for successful operations
- 4xx for client errors
- 5xx for server errors
Following these conventions creates intuitive interfaces that align with developer expectations and web standards.
6. Implement Comprehensive Pagination
As data sets grow, pagination becomes essential. Future proof pagination should be flexible and include metadata:
{
"data": [
{ "id": 1, "name": "Product A" },
{ "id": 2, "name": "Product B" }
],
"pagination": {
"total_items": 1432,
"total_pages": 72,
"current_page": 1,
"page_size": 20,
"links": {
"first": "/api/products?page=1&size=20",
"next": "/api/products?page=2&size=20",
"last": "/api/products?page=72&size=20"
}
}
}
This approach provides clients with the information needed to navigate large collections efficiently and adapts to growing data volumes.
Advanced Techniques for API Longevity
Beyond the fundamental strategies, several advanced techniques can further enhance API durability.
1. API Gateway Pattern
An API gateway serves as an intermediary between clients and your backend services, providing several benefits for future proofing:
- Decoupling client interfaces from backend implementations
- Supporting multiple API versions simultaneously
- Implementing cross cutting concerns like authentication, rate limiting, and logging
- Transforming requests and responses to maintain compatibility
Popular API gateway technologies include Amazon API Gateway, Kong, and Apigee.
2. Contract Testing
Contract testing verifies that API providers and consumers adhere to agreed upon interfaces. Tools like Pact enable automated testing of API contracts:
// Example Pact consumer test
const userServiceClient = new UserServiceClient();
const userPact = pact({
consumer: 'MyFrontend',
provider: 'UserService'
});
describe('User API', () => {
beforeAll(() => userPact.setup());
afterAll(() => userPact.finalize());
test('get user by ID', async () => {
await userPact.addInteraction({
state: 'a user with ID 123 exists',
uponReceiving: 'a request for user 123',
withRequest: {
method: 'GET',
path: '/api/users/123'
},
willRespondWith: {
status: 200,
body: {
id: 123,
name: Matchers.string('John Doe'),
email: Matchers.regex(/^[a-zA-Z0-9._%+-]+@[a-zA-Z0-9.-]+\.[a-zA-Z]{2,}$/, 'john@example.com')
}
}
});
const user = await userServiceClient.getUserById(123);
expect(user.id).toBe(123);
});
});
Contract testing catches compatibility issues early and documents the expected behavior of API interactions.
3. Feature Toggles for API Capabilities
Feature toggles (or feature flags) allow you to introduce new API capabilities incrementally:
// Server side implementation with feature toggle
function handleUserRequest(req, res) {
const user = getUserById(req.params.id);
const response = {
id: user.id,
name: user.name,
email: user.email
};
// New fields behind feature toggle
if (featureFlags.isEnabled('enhanced_user_data')) {
response.preferences = user.preferences;
response.activity = getUserActivity(user.id);
}
res.json(response);
}
This approach allows you to test new API features with select clients before making them generally available.
4. GraphQL for Flexible Data Requirements
GraphQL addresses many API evolution challenges by allowing clients to specify exactly what data they need:
// GraphQL query
query {
user(id: "123") {
id
name
email
# Client only requests fields it needs
}
}
This flexibility enables API providers to add new fields without breaking existing clients, as they only receive the data they explicitly request.
5. Comprehensive Monitoring and Usage Analytics
Understanding how clients use your API informs evolution decisions. Implement monitoring that captures:
- Endpoint popularity and traffic patterns
- Error rates and common failure modes
- Performance metrics and bottlenecks
- Client diversity (browsers, mobile apps, server integrations)
- Version adoption and deprecation progress
This data helps prioritize improvements and assess the impact of potential changes.
Case Studies: API Evolution Success Stories
Stripe API: A Model of Backward Compatibility
Stripe’s payment processing API is renowned for its commitment to backward compatibility. Key strategies include:
- API versioning via request headers
- Explicit version pinning for each account
- Gradual migration paths with clear documentation
- Expansion parameters for controlling response detail
This approach has allowed Stripe to evolve its API significantly while maintaining compatibility with integrations dating back to its early days.
Twilio: Versioning in the URL Path
Twilio uses URL path versioning (/2010-04-01/Accounts
) with date based versioning that clearly communicates the API’s age. Their approach includes:
- Long term support for all API versions
- Comprehensive migration guides between versions
- Consistent patterns across all API resources
- Helper libraries that abstract version differences
This strategy provides stability for developers while allowing Twilio to introduce major improvements in newer versions.
Creating Your API Evolution Strategy
Based on the principles and patterns discussed, here’s a framework for developing your own API evolution strategy:
1. Establish Clear Governance
- Define roles and responsibilities for API design decisions
- Create review processes for API changes
- Document compatibility requirements and exceptions
- Establish deprecation policies and timelines
2. Document Design Standards
- Create API style guides for consistency
- Define naming conventions and patterns
- Specify error handling approaches
- Establish data format standards
3. Implement Technical Safeguards
- Automate compatibility testing in CI/CD pipelines
- Create linting rules for API specifications
- Monitor for breaking changes in pull requests
- Implement automated documentation generation
4. Communicate Effectively with Consumers
- Maintain a developer portal with comprehensive documentation
- Provide change logs and migration guides
- Establish deprecation notification channels
- Create feedback mechanisms for API consumers
Tools for Building Future Proof APIs
Several tools can support your future proofing efforts:
API Design and Documentation
- Swagger/OpenAPI: Industry standard for API specification
- Postman: API development environment with documentation capabilities
- Stoplight: Visual API design with built in style guide enforcement
- Redoc: Documentation generator for OpenAPI specifications
API Testing and Validation
- Pact: Contract testing framework
- Dredd: OpenAPI specification validator
- REST Assured: Java DSL for API testing
- Karate: API test automation combining API test automation with UI automation
API Management and Gateways
- Kong: Open source API gateway
- Apigee: Google Cloud’s API management platform
- Amazon API Gateway: AWS managed API service
- Azure API Management: Microsoft’s API management solution
Common Questions About API Future Proofing
How long should we support older API versions?
The support timeline depends on several factors:
- Client update cycles (mobile apps typically have slower adoption)
- Integration complexity (more complex integrations need longer migration periods)
- Business criticality (mission critical APIs warrant longer support)
As a general guideline, major versions should be supported for at least 12 months after deprecation notice, with business critical APIs potentially requiring 24+ months.
Should we use GraphQL instead of REST for better future proofing?
GraphQL offers significant advantages for evolving APIs, particularly for data intensive applications with diverse client needs. However, it introduces its own complexity and may not be suitable for all use cases. Consider GraphQL when:
- Clients need flexible data requirements
- Your API serves multiple client types with different needs
- Performance optimization through reduced data transfer is important
Many organizations successfully combine REST and GraphQL APIs in their ecosystems.
How do we handle urgent security fixes in stable APIs?
Security issues present special challenges for API compatibility. Best practices include:
- Implement changes in a backward compatible way when possible
- Provide clear security advisories with specific migration steps
- Offer emergency support for critical integrations
- Consider maintaining parallel endpoints temporarily (secure and legacy) with appropriate warnings
Document your security exception process in your API governance policy.
Conclusion: Building APIs That Endure
Creating future proof APIs requires a combination of technical discipline, strategic foresight, and effective communication. By avoiding common pitfalls like exposing implementation details, neglecting versioning, and breaking backward compatibility, you can build interfaces that evolve gracefully with changing requirements.
Remember these key principles:
- Design for the long term, not just immediate needs
- Hide implementation details behind stable abstractions
- Implement consistent versioning from the start
- Build extensibility into your data structures
- Communicate clearly with API consumers about changes and migrations
With these practices in place, your APIs can become valuable, enduring assets rather than maintenance burdens. The investment in thoughtful API design pays dividends through reduced technical debt, improved developer experience, and greater business agility.
By approaching API development with future proofing in mind, you create the foundation for sustainable digital ecosystems that can adapt to tomorrow’s challenges while preserving today’s investments.