Why Your Algorithm Knowledge Isn’t Helping With Practical Problems
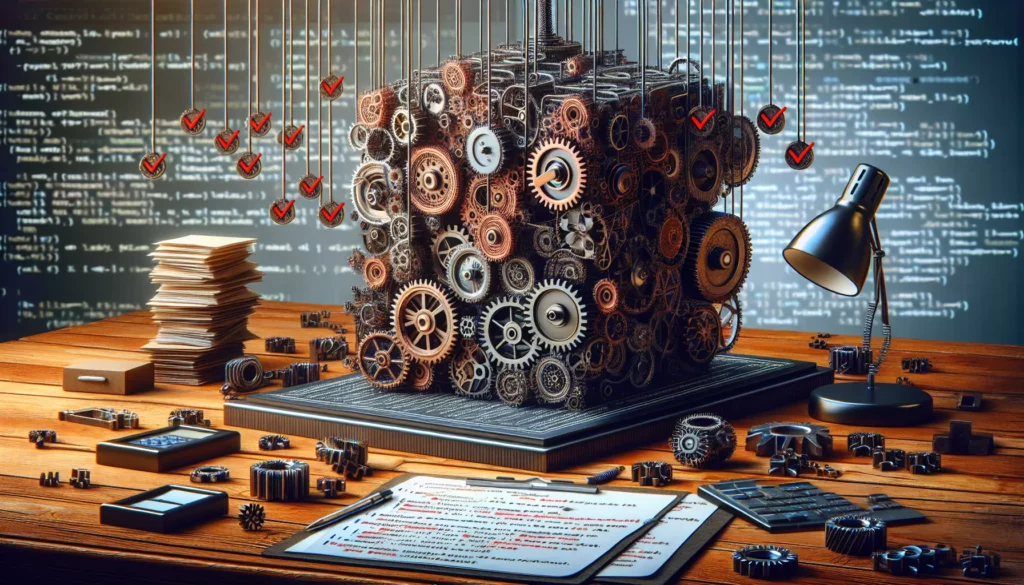
Have you ever spent countless hours mastering complex algorithms only to find yourself stumped when faced with real world coding challenges? You’re not alone. Many programmers experience a disconnect between their theoretical algorithm knowledge and their ability to solve practical problems. This phenomenon is more common than you might think, and it’s something we at AlgoCademy have observed across thousands of learners.
In this comprehensive guide, we’ll explore why your algorithm knowledge might not be translating to practical problem solving success, and more importantly, how to bridge that gap effectively.
The Algorithm Knowledge Paradox
Let’s start with a scenario many developers find familiar: You’ve diligently studied Big O notation, mastered sorting algorithms, memorized graph traversal techniques, and can recite the differences between dynamic programming and greedy algorithms. Yet, when faced with building a feature for a real application or fixing a bug in production code, you feel stuck.
This disconnect between theoretical knowledge and practical application creates what we call the “Algorithm Knowledge Paradox” — the phenomenon where increasing theoretical knowledge doesn’t proportionally improve practical problem solving abilities.
The Fundamental Disconnect
To understand this paradox, we must first recognize the key differences between algorithm exercises and real world programming:
- Well Defined vs. Ambiguous Problems: Algorithm problems typically have clear inputs, outputs, and constraints. Real world problems often start with unclear requirements and evolving specifications.
- Isolated vs. Integrated Solutions: Algorithm exercises focus on singular, isolated functions. Practical programming involves integrating solutions into complex systems with existing code and dependencies.
- Performance Optimization vs. Maintainability: While algorithm challenges emphasize optimal runtime and space complexity, real world coding often prioritizes readability, maintainability, and extensibility.
- Academic Elegance vs. Practical Compromise: Textbook solutions favor elegance and theoretical purity, while practical coding often requires pragmatic compromises.
7 Reasons Your Algorithm Knowledge Isn’t Translating to Practical Success
Let’s dive deeper into the specific reasons why strong algorithm knowledge doesn’t automatically make you effective at solving real world programming challenges:
1. Context Switching Challenges
Algorithm problems exist in a vacuum. You’re given a clean slate with precisely defined inputs and expected outputs. Real programming tasks require understanding existing codebases, organizational constraints, and business requirements that may not be immediately apparent.
Consider this example: Implementing a search function in an algorithm exercise might involve coding a binary search algorithm from scratch. In a real application, you’d need to consider:
- How the search integrates with existing database queries
- Performance implications across different data volumes
- User experience considerations like search result ranking
- Error handling for edge cases
- Internationalization and localization concerns
This context switching requires a different mental model than pure algorithm problem solving.
2. Overemphasis on Optimization
Algorithm courses and competitive programming naturally emphasize optimization — finding the solution with the best time and space complexity. While understanding computational complexity is valuable, this focus can create a mindset that optimization is always the primary goal.
In practical development, premature optimization can be counterproductive. As Donald Knuth famously stated, “Premature optimization is the root of all evil.” Real world programming often values:
- Code readability and maintainability
- Fast development cycles
- Ease of debugging and testing
- Team collaboration and knowledge sharing
A slightly less efficient algorithm that’s easier to understand and maintain is often the better choice in production environments.
3. Missing System Design Skills
Algorithm knowledge focuses on solving individual problems efficiently, but practical programming requires designing systems that solve collections of problems cohesively. Many developers with strong algorithm skills struggle with:
- Breaking down large problems into manageable components
- Designing clear interfaces between system parts
- Managing state and data flow across an application
- Anticipating future requirements and building extensible solutions
System design requires a different mental model than algorithm problem solving — one that emphasizes relationships between components rather than optimizing individual functions.
4. Limited Exposure to Real Codebases
Many algorithm focused learning paths don’t provide enough exposure to large, realistic codebases. Working effectively in production environments requires:
- Reading and understanding code written by others
- Navigating complex project structures
- Understanding architectural patterns and their implications
- Recognizing common patterns and antipatterns in code organization
This skill develops primarily through experience working with real world projects rather than solving standalone algorithm problems.
5. Neglecting Practical Tools and Technologies
Algorithm knowledge exists somewhat independently of specific programming tools and technologies. In contrast, practical problem solving requires proficiency with:
- Version control systems like Git
- Debugging tools and techniques
- Testing frameworks and methodologies
- Build systems and deployment pipelines
- Frameworks and libraries specific to your domain
These practical skills aren’t typically emphasized in algorithm focused education but are essential for day to day programming tasks.
6. The Communication Gap
Algorithm problems are typically solved individually, with minimal communication requirements. Real world programming is inherently collaborative, requiring:
- Clearly explaining technical concepts to team members
- Documenting code and design decisions
- Gathering and clarifying requirements from stakeholders
- Providing and receiving code reviews
- Justifying technical decisions to non technical team members
These soft skills are rarely developed through algorithm practice alone but are crucial for practical success.
7. Rigid Problem Solving Approaches
Algorithm education often teaches categorical approaches to problem solving: “This is a dynamic programming problem,” or “Use a greedy algorithm here.” This categorization is useful for algorithm challenges but can create rigid thinking patterns.
Real world problems rarely fit neatly into these categories and often require blending multiple approaches or creating novel solutions tailored to specific contexts.
Bridging the Gap: From Algorithm Knowledge to Practical Problem Solving
Now that we’ve identified the key disconnects, let’s explore strategies to bridge the gap between theoretical algorithm knowledge and practical problem solving skills:
1. Build Real Projects
Nothing beats hands on experience with real projects. Instead of only solving isolated algorithm problems, challenge yourself to build complete applications that:
- Solve practical problems you or others face
- Require integration of multiple components
- Need ongoing maintenance and feature additions
- Involve real users with real feedback
These projects will force you to confront the messy realities of practical programming that algorithm exercises don’t cover.
2. Study System Design
Complement your algorithm knowledge with dedicated study of system design principles. This includes:
- Component based architecture
- API design patterns
- Database schema design
- Scalability considerations
- Reliability and fault tolerance patterns
Resources like system design interview books, architecture pattern guides, and case studies of real system designs can help develop this crucial skill set.
3. Read and Analyze Production Code
Actively reading well written code is one of the most underrated learning activities. Explore open source projects in your area of interest and:
- Study how they organize complex functionality
- Notice patterns in error handling and edge case management
- Observe how they balance optimization with readability
- Review their testing strategies
This exposure to real world code will help you develop intuition for practical problem solving approaches.
4. Practice Incremental Development
Algorithm problems encourage finding the complete solution before implementation. Real world development works better with incremental approaches:
- Start with a minimal viable solution
- Add automated tests to verify behavior
- Refactor for clarity and maintainability
- Optimize only when necessary and with measured results
This incremental approach builds the muscle memory for practical development workflows.
5. Collaborate with Others
Seek opportunities to collaborate with other developers through:
- Open source contributions
- Pair programming sessions
- Code reviews (both giving and receiving)
- Team projects
These collaborative experiences develop the communication and teamwork skills essential for real world programming success.
6. Apply Algorithms in Context
When you learn new algorithms, challenge yourself to identify where and how they might apply in real applications. For example:
- Where might breadth first search be useful in a web application?
- How could dynamic programming optimize a real feature you’re building?
- What practical problems could benefit from graph algorithms?
This contextual thinking helps bridge theoretical knowledge with practical application.
7. Develop a Practical Toolbox
Invest time in learning practical development tools:
- Debugging techniques specific to your language/environment
- Profiling tools to identify real (not theoretical) bottlenecks
- Testing frameworks and methodologies
- Version control workflows
Proficiency with these tools dramatically increases your effectiveness in solving real world problems.
Case Study: Transforming a Theoretical Approach into a Practical Solution
Let’s examine a concrete example that illustrates the gap between algorithm knowledge and practical problem solving.
The Problem: Building a Product Recommendation Feature
Imagine you’re tasked with building a product recommendation feature for an e-commerce website. Users should see personalized product recommendations based on their browsing and purchase history.
The Algorithm Approach
A developer with strong algorithm knowledge might immediately think about:
- Collaborative filtering algorithms
- K-nearest neighbors for finding similar users
- Matrix factorization techniques
- Optimal data structures for fast similarity lookups
They might start by implementing a sophisticated recommendation algorithm from scratch:
def calculate_user_similarity(user1_history, user2_history):
# Implement cosine similarity calculation
common_items = set(user1_history.keys()) & set(user2_history.keys())
if not common_items:
return 0.0
sum_of_products = sum(user1_history[item] * user2_history[item] for item in common_items)
user1_magnitude = math.sqrt(sum(value ** 2 for value in user1_history.values()))
user2_magnitude = math.sqrt(sum(value ** 2 for value in user2_history.values()))
return sum_of_products / (user1_magnitude * user2_magnitude)
def recommend_products(current_user, all_users, product_database):
# Find similar users using KNN
similarities = [(other_user, calculate_user_similarity(current_user.history, other_user.history))
for other_user in all_users if other_user != current_user]
similar_users = sorted(similarities, key=lambda x: x[1], reverse=True)[:5]
# Collect products from similar users that current user hasn't seen
recommendations = {}
for user, similarity in similar_users:
for product in user.history:
if product not in current_user.history:
recommendations[product] = recommendations.get(product, 0) + similarity
# Return top recommendations
return sorted(recommendations.items(), key=lambda x: x[1], reverse=True)[:10]
While this approach demonstrates strong algorithm knowledge, it might face several practical challenges:
- Scaling issues with large user bases
- Cold start problems for new users
- Integration complexity with existing systems
- Maintenance overhead for custom algorithm implementation
- Difficulty measuring and improving recommendation quality
The Practical Approach
A developer skilled in practical problem solving might take a different approach:
- Start simple: Begin with a basic “frequently bought together” recommendation based on purchase co-occurrence, which covers a common use case without complex algorithms.
- Leverage existing tools: Research recommendation system libraries or services that have already solved common challenges rather than building from scratch.
- Consider the full system: Design how recommendations integrate with the product catalog, user profiles, and frontend display.
- Plan for measurement: Implement tracking to measure recommendation effectiveness through click-through rates and conversion.
- Build incrementally: Start with a minimum viable implementation, test with real users, and iterate based on results.
Their implementation approach might look more like:
// First iteration: Simple "frequently bought together" implementation
function getProductRecommendations(productId) {
// Query the database for products frequently purchased with this one
return db.query(`
SELECT p.id, p.name, p.price, p.image_url, COUNT(*) as purchase_count
FROM order_items oi1
JOIN order_items oi2 ON oi1.order_id = oi2.order_id AND oi1.product_id != oi2.product_id
JOIN products p ON oi2.product_id = p.id
WHERE oi1.product_id = ?
GROUP BY p.id
ORDER BY purchase_count DESC
LIMIT 5
`, [productId]);
}
// Set up tracking to measure effectiveness
function trackRecommendationClick(recommendationId, productId, position) {
analytics.track('recommendation_click', {
recommendation_id: recommendationId,
product_id: productId,
position: position,
timestamp: new Date()
});
}
This practical approach:
- Delivers value quickly with a simpler solution
- Leverages existing database capabilities
- Establishes measurement systems for future improvements
- Can be easily extended as needs evolve
- Avoids premature optimization and complexity
As the feature proves valuable, they might gradually incorporate more sophisticated algorithms, potentially using existing libraries or services rather than building everything from scratch.
How AlgoCademy Bridges the Theory Practice Gap
At AlgoCademy, we’ve designed our learning approach specifically to address the disconnect between algorithm knowledge and practical problem solving abilities. Here’s how our methodology bridges this gap:
Contextual Learning
Rather than teaching algorithms in isolation, we present them in the context of real world applications. For each algorithm or data structure, we explore:
- Practical use cases where it applies
- How it integrates into larger systems
- When simpler alternatives might be preferable
- Common implementation patterns in production code
This contextual approach helps learners develop intuition for when and how to apply theoretical knowledge.
Project Based Learning Paths
Our curriculum incorporates complete project implementations that require:
- Applying algorithms within realistic constraints
- Making design decisions that balance competing priorities
- Writing maintainable, well tested code
- Refactoring and improving existing implementations
These projects bridge the gap between isolated exercises and real world development.
Code Reading and Analysis
We emphasize the skill of reading and understanding code written by others through:
- Guided walkthroughs of well designed codebases
- Exercises that require modifying existing code
- Comparative analysis of different solution approaches
- Exposure to idiomatic patterns in various languages
This develops the critical skill of working effectively with existing code.
Incremental Development Practices
Our interactive coding exercises encourage proper development practices:
- Starting with test cases to understand requirements
- Building solutions incrementally
- Refactoring for readability and maintainability
- Considering edge cases and error handling
These practices build habits that transfer directly to professional development.
Community and Collaboration
Learning to code effectively is a social activity. Our platform facilitates:
- Peer code reviews and feedback
- Discussion of alternative approaches
- Collaborative problem solving
- Exposure to different perspectives and solutions
These collaborative elements develop the communication skills essential for real world success.
Practical Exercises to Bridge the Gap
To help you start bridging the gap between algorithm knowledge and practical problem solving, here are five exercises you can try:
Exercise 1: Refactor an Algorithm Implementation
Find a basic algorithm implementation (sorting, searching, etc.) and refactor it for:
- Better readability and documentation
- Robust error handling
- Configurability through parameters
- Testability with unit tests
This exercise helps you transition from “working code” to “production ready code.”
Exercise 2: Integrate an Algorithm into a Real Application
Take an algorithm you’ve studied (like a pathfinding algorithm) and integrate it into a simple web or mobile application. Focus on:
- Creating a usable interface around the algorithm
- Handling user input and validation
- Visualizing the algorithm’s operation
- Making the implementation maintainable
This exercise develops the skill of applying algorithms in practical contexts.
Exercise 3: Solve a Problem with Multiple Approaches
Select a problem (like text search) and implement multiple solutions:
- A simple, straightforward approach
- An optimized algorithm approach
- An approach using existing libraries or tools
Then compare them on dimensions beyond just performance: readability, maintainability, extensibility, and development time. This builds judgment about solution selection in real contexts.
Exercise 4: Reverse Engineer from Requirements
Start with a product description rather than a technical problem statement:
“Create a feature that allows users to see which friends have birthdays in the upcoming month and send them scheduled birthday messages.”
Work backwards to:
- Identify the core data structures and algorithms needed
- Design the components and their interactions
- Plan the implementation in incremental steps
- Consider practical concerns like privacy and error cases
This exercise develops the skill of translating requirements into technical solutions.
Exercise 5: Improve an Existing Codebase
Find an open source project with room for improvement and contribute by:
- Fixing bugs or addressing issues
- Adding tests for existing functionality
- Refactoring for better performance or readability
- Adding a small feature or enhancement
This develops the critical skill of working with existing code rather than starting from scratch.
Conclusion: Balancing Theory and Practice
The disconnect between algorithm knowledge and practical problem solving ability isn’t due to a flaw in either approach — it’s about the need for balance and integration between them.
Strong algorithm knowledge provides a foundation of problem solving tools and performance understanding that can be invaluable. Practical skills provide the context and techniques to apply that knowledge effectively in real world situations.
The most effective developers aren’t those who excel exclusively at either theoretical algorithms or practical coding — they’re those who can bridge between these worlds, applying theoretical knowledge in practical contexts while using practical experience to inform their theoretical understanding.
At AlgoCademy, we’re committed to developing both sides of this equation, creating learners who not only understand algorithms deeply but can apply them effectively to solve real world problems. We believe this integrated approach is the key to developing truly exceptional programmers who can tackle the complex challenges of modern software development.
Remember that bridging this gap is an ongoing journey rather than a destination. Each project you build, each system you design, and each problem you solve helps strengthen the connection between your theoretical knowledge and practical abilities.
What strategies have you found helpful in applying algorithm knowledge to practical problems? Share your experiences and continue the conversation as we all work to become more effective problem solvers.