Why You Understand the Problem But Can’t Structure the Solution
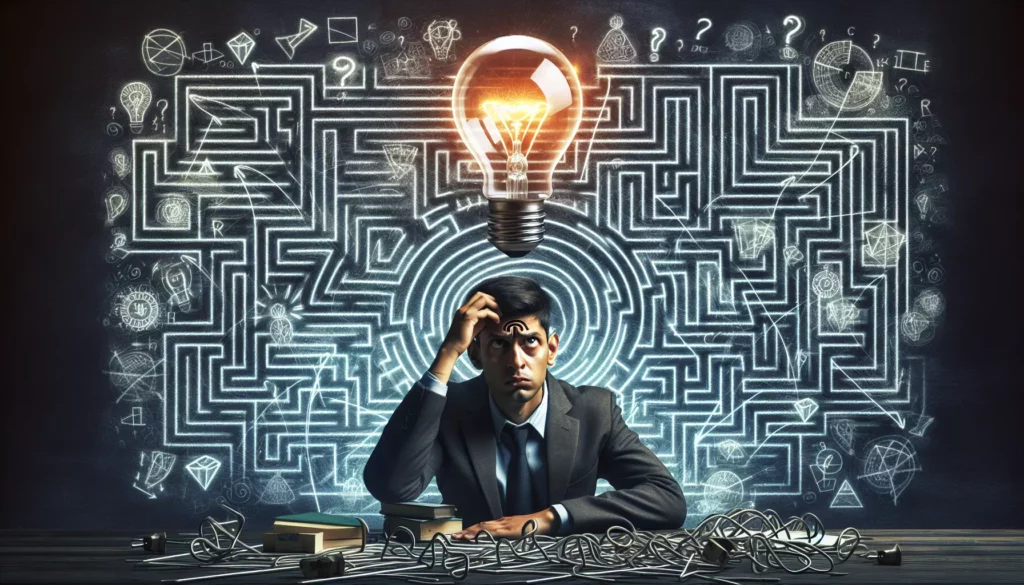
Have you ever found yourself staring at a coding problem, understanding exactly what it’s asking, yet unable to formulate a working solution? This frustrating disconnect between comprehension and implementation affects programmers at all levels, from beginners to seasoned professionals.
At AlgoCademy, we’ve observed thousands of learners face this precise challenge. They can explain the problem perfectly, but when it comes to structuring an algorithmic solution, they hit a mental wall. This article explores why this happens and provides actionable strategies to bridge this gap in your programming journey.
The Comprehension-Implementation Gap: A Common Programming Struggle
Understanding a problem and implementing its solution are two distinct cognitive processes. The first involves analytical thinking, while the second requires synthetic thinking. Let’s break down why these two abilities don’t always align.
Why Problem Understanding Doesn’t Guarantee Solution Implementation
When you read a problem statement, your brain processes the information to form a conceptual understanding. You identify the inputs, expected outputs, and constraints. This analytical process is different from the creative, constructive process of building a solution.
Consider this scenario: You’re asked to find all pairs in an array that sum to a specific target. You understand what’s being asked—find pairs of numbers that add up to a target value. Yet, translating this understanding into code requires a different set of skills.
The Four Stages of Problem Solving Proficiency
- Unconscious Incompetence: You don’t know what you don’t know. You might think a problem is simple because you don’t understand its complexities.
- Conscious Incompetence: You understand the problem but recognize you lack the skills to solve it. This is the stage we’re discussing—where comprehension exists without implementation ability.
- Conscious Competence: You can solve the problem with deliberate effort and thought.
- Unconscious Competence: You can solve similar problems almost automatically, without extensive deliberation.
Most programmers struggling with the comprehension-implementation gap are in the second stage. The good news? This recognition is a crucial step toward improvement.
Common Reasons Behind the Solution Structuring Struggle
Let’s explore the specific factors that contribute to difficulty in structuring solutions, even when the problem is well understood.
1. Lack of Algorithm Design Patterns Knowledge
Just as design patterns exist in software engineering, algorithm design patterns provide templates for solving common computational problems. Without knowledge of these patterns, you might understand a problem but lack the mental framework to approach its solution.
For example, when faced with a problem requiring finding the shortest path in a graph, knowing about Dijkstra’s algorithm provides an immediate structure for your solution. Without this knowledge, you might understand the goal but struggle to formulate an efficient approach.
2. Limited Problem Decomposition Skills
Complex problems require breaking them down into manageable sub-problems. This skill doesn’t automatically come with problem comprehension.
Consider sorting a large dataset efficiently. Understanding that you need the data in order is straightforward, but breaking this down into a divide-and-conquer approach (like merge sort) requires decomposition skills that go beyond problem comprehension.
// Example of problem decomposition in merge sort
function mergeSort(arr) {
// Base case
if (arr.length <= 1) return arr;
// Decomposition: Split the problem
const mid = Math.floor(arr.length / 2);
const left = mergeSort(arr.slice(0, mid));
const right = mergeSort(arr.slice(mid));
// Solve sub-problems and combine
return merge(left, right);
}
3. Weak Data Structure Intuition
Choosing the right data structure is often half the battle in algorithm design. You might understand what needs to be accomplished but lack intuition about which data structure would make the solution elegant and efficient.
For instance, recognizing that a hash map can transform an O(n²) lookup problem into an O(n) solution comes with experience and knowledge that extends beyond problem comprehension.
4. Difficulty Translating High-Level Ideas to Code
Sometimes, you have a high-level approach in mind but struggle to express it in code. This translation gap can be particularly frustrating because you feel so close to the solution.
A common example is understanding that you need to use recursion for a tree traversal problem but struggling with the exact implementation of the recursive function.
5. Overthinking and Perfectionism
Paradoxically, a deep understanding of a problem can sometimes lead to overthinking. You might be aware of edge cases, optimization opportunities, and alternative approaches, causing decision paralysis.
This often manifests as starting multiple solution approaches without completing any of them, or getting stuck trying to find the “perfect” solution instead of a working one.
Bridging the Gap: From Understanding to Implementation
Now that we’ve identified the common barriers, let’s explore strategies to overcome them and strengthen your ability to structure solutions.
Learn Algorithm Design Patterns
Just as learning vocabulary helps you express complex thoughts in a language, familiarizing yourself with common algorithm patterns provides building blocks for solutions.
Key patterns to master include:
- Two Pointers: For problems involving sorted arrays or linked lists
- Sliding Window: For problems involving contiguous sequences
- Binary Search: For efficiently finding elements in sorted collections
- Breadth-First Search (BFS): For level-by-level exploration of data structures
- Depth-First Search (DFS): For exploring as far as possible along branches
- Dynamic Programming: For optimization problems with overlapping subproblems
- Greedy Algorithms: For making locally optimal choices
Let’s see how recognizing a pattern can immediately provide structure to your solution:
// Two pointers pattern for finding pairs that sum to target
function findPairsWithSum(arr, target) {
arr.sort((a, b) => a - b); // Sort the array
let left = 0;
let right = arr.length - 1;
const result = [];
while (left < right) {
const sum = arr[left] + arr[right];
if (sum === target) {
result.push([arr[left], arr[right]]);
left++;
right--;
} else if (sum < target) {
left++;
} else {
right--;
}
}
return result;
}
Practice Decomposing Problems
Improve your ability to break down complex problems by explicitly practicing this skill:
- Start by identifying the main goal of the problem
- List the major steps or components needed to achieve this goal
- For each component, determine if it needs further breakdown
- Continue until you have manageable, implementable pieces
For example, if tackling a problem to find the kth largest element in an array:
- Main goal: Find the kth largest element
- Possible approaches:
- Sort the array and return the kth element from the end (decomposed into sorting + indexing)
- Use a min-heap of size k (decomposed into heap construction + processing elements)
- Use quickselect algorithm (decomposed into partitioning + recursive selection)
- Choose one approach and break it down further
Build Your Data Structure Intuition
Develop a mental model of when to use each data structure by understanding their strengths and weaknesses:
- Arrays/Lists: Sequential access, constant-time indexing
- Hash Maps: Fast lookups, insertions, and deletions
- Stacks: Last-in, first-out operations, tracking state in recursive algorithms
- Queues: First-in, first-out operations, breadth-first traversals
- Trees: Hierarchical relationships, searching, and sorting
- Graphs: Complex relationships and network problems
- Heaps: Priority queues, finding min/max elements efficiently
Practice matching problems to data structures:
// Problem: Find if two strings are anagrams
// Intuition: Use a hash map to count character frequencies
function areAnagrams(s1, s2) {
if (s1.length !== s2.length) return false;
const charCount = {};
// Count characters in first string
for (const char of s1) {
charCount[char] = (charCount[char] || 0) + 1;
}
// Decrement counts for second string
for (const char of s2) {
if (!charCount[char]) return false;
charCount[char]--;
}
// Verify all counts are zero
return Object.values(charCount).every(count => count === 0);
}
Practice Translating Ideas to Code
Improve your ability to express algorithmic ideas in code through deliberate practice:
- Start with pseudocode to bridge the gap between concept and implementation
- Build a library of common code snippets for frequent operations
- Practice implementing the same algorithm in different ways
For example, here’s how you might translate the concept of binary search into pseudocode first, then code:
// Pseudocode for binary search
// 1. Set left pointer to start of array and right pointer to end
// 2. While left <= right:
// a. Calculate mid point
// b. If target == array[mid], return mid
// c. If target < array[mid], move right pointer to mid-1
// d. If target > array[mid], move left pointer to mid+1
// 3. Return -1 if target not found
// Implementation in JavaScript
function binarySearch(arr, target) {
let left = 0;
let right = arr.length - 1;
while (left <= right) {
const mid = Math.floor((left + right) / 2);
if (arr[mid] === target) {
return mid;
} else if (arr[mid] < target) {
left = mid + 1;
} else {
right = mid - 1;
}
}
return -1; // Target not found
}
Adopt an Incremental Approach
Combat overthinking and perfectionism by adopting an incremental approach to solution development:
- Start with a naive, brute-force solution to ensure you understand the problem
- Get a working solution before optimizing
- Refine your solution step by step
- Use test cases to validate each iteration
This approach prevents decision paralysis and builds confidence through small wins.
Practical Exercises to Strengthen Your Solution Structuring Skills
Theory alone isn’t enough to bridge the comprehension-implementation gap. Here are practical exercises to strengthen your solution structuring muscles:
Exercise 1: Reverse-Engineer Solutions
Take existing solutions to problems and work backward to understand the thought process:
- Study a solution to a problem you understand
- Identify the key algorithm design patterns used
- Note how the problem was decomposed
- Observe the data structures chosen and why
- Try to recreate the solution without looking at the original
This exercise helps you internalize solution patterns and approaches.
Exercise 2: Verbalize Your Thought Process
Practice explaining your approach before and during coding:
- Read a problem statement
- Before writing any code, verbalize or write down your approach
- Identify the algorithm pattern you’ll use
- Explain your data structure choices
- Outline the steps of your solution
This exercise strengthens the connection between understanding and implementation by forcing you to articulate the bridge between them.
Exercise 3: Time-Boxed Problem Solving
Improve your ability to quickly structure solutions:
- Set a timer for 5-10 minutes
- Read a problem and sketch a high-level solution approach
- Focus on structure, not implementation details
- When time is up, evaluate your approach
This exercise develops your ability to quickly identify solution structures without getting bogged down in details.
Exercise 4: Implement Multiple Approaches
Expand your solution repertoire:
- Solve the same problem using different approaches
- Implement both iterative and recursive solutions
- Try different data structures for the same problem
- Compare the trade-offs between approaches
This exercise builds flexibility in your thinking and expands your solution toolbox.
Case Study: From Understanding to Implementation
Let’s walk through a complete example of bridging the comprehension-implementation gap for a common interview problem.
Problem: Group Anagrams
Given an array of strings, group anagrams together. An anagram is a word formed by rearranging the letters of another word.
Step 1: Ensure Problem Understanding
Input: [“eat”, “tea”, “tan”, “ate”, “nat”, “bat”]
Output: [[“eat”,”tea”,”ate”],[“tan”,”nat”],[“bat”]]
We need to group strings that are anagrams of each other. Anagrams have the same letters but in different orders.
Step 2: Identify the Algorithm Pattern
This is a grouping problem. We need to determine which strings are anagrams of each other and collect them. A hash map would be useful for grouping related items.
Step 3: Choose Appropriate Data Structures
We need:
- A hash map to group anagrams
- A way to identify if two strings are anagrams
Step 4: Decompose the Problem
- Define a way to identify anagrams (key generation)
- Iterate through the input strings
- For each string, generate its anagram key
- Group strings by their anagram key
- Return the grouped results
Step 5: Translate to Pseudocode
// Create an empty hash map for grouping
// For each string in the input array:
// Generate an anagram key (sorted characters or character count)
// If the key doesn't exist in the hash map, create a new group
// Add the current string to its anagram group
// Return all the groups as an array
Step 6: Implement the Solution
function groupAnagrams(strs) {
const groups = {};
for (const str of strs) {
// Generate anagram key by sorting the characters
const key = str.split('').sort().join('');
// Create group if it doesn't exist
if (!groups[key]) {
groups[key] = [];
}
// Add string to its group
groups[key].push(str);
}
// Return all groups
return Object.values(groups);
}
Step 7: Test and Refine
Let’s trace through the example:
- “eat” → key: “aet” → create group [“eat”]
- “tea” → key: “aet” → add to group [“eat”, “tea”]
- “tan” → key: “ant” → create group [“tan”]
- “ate” → key: “aet” → add to group [“eat”, “tea”, “ate”]
- “nat” → key: “ant” → add to group [“tan”, “nat”]
- “bat” → key: “abt” → create group [“bat”]
Final result: [[“eat”,”tea”,”ate”],[“tan”,”nat”],[“bat”]]
This matches our expected output, confirming our solution works!
Alternative Approach
We could also use character counts instead of sorting for the anagram key:
function groupAnagrams(strs) {
const groups = {};
for (const str of strs) {
// Generate anagram key using character counts
const counts = Array(26).fill(0);
for (const char of str) {
const index = char.charCodeAt(0) - 'a'.charCodeAt(0);
counts[index]++;
}
// Join counts with a separator that can't appear in the input
const key = counts.join('#');
if (!groups[key]) {
groups[key] = [];
}
groups[key].push(str);
}
return Object.values(groups);
}
This approach avoids the O(n log n) cost of sorting each string, potentially improving performance for long strings.
Common Pitfalls to Avoid When Structuring Solutions
Even as you work to bridge the comprehension-implementation gap, watch out for these common pitfalls:
1. Jumping to Implementation Too Quickly
Resist the urge to start coding immediately. Without proper planning, you may head down an inefficient path that’s hard to correct later.
Instead: Spend time on problem analysis and solution design first. Sketch your approach before typing a single line of code.
2. Overlooking Edge Cases
It’s easy to focus on the happy path and forget about edge cases, especially when you’re struggling with implementation.
Instead: Systematically identify edge cases as part of your solution structure. Consider empty inputs, extreme values, and special conditions.
3. Overcomplicating the Solution
Sometimes we reach for complex data structures or algorithms when simpler approaches would work.
Instead: Start with the simplest solution that could work. Add complexity only when necessary for performance or other requirements.
4. Getting Stuck in Analysis Paralysis
Overthinking can prevent you from making progress, especially when multiple approaches seem viable.
Instead: Choose one approach, implement it, and refine. Movement generates momentum.
5. Not Testing Incrementally
Writing a complete solution before testing any part of it makes debugging much harder.
Instead: Test small components of your solution as you build them. This provides faster feedback and builds confidence.
How AlgoCademy Helps Bridge the Comprehension-Implementation Gap
At AlgoCademy, we’ve designed our platform specifically to help learners overcome the challenge of translating problem understanding into working solutions.
Structured Learning Paths
Our curriculum systematically builds your algorithm design and problem-solving skills, introducing patterns and techniques in a progressive sequence that reinforces comprehension and implementation connections.
Pattern-Based Problem Organization
We organize problems by algorithm patterns, helping you recognize which approaches apply to different problem types. This pattern recognition is crucial for bridging the gap between understanding and implementation.
Interactive Problem Solving
Our platform provides immediate feedback as you work through problems, helping you identify gaps in your implementation approach and correct them in real-time.
AI-Powered Guidance
Our AI assistant helps break down complex problems into manageable steps, suggesting solution structures when you’re stuck without giving away complete solutions.
Visualization Tools
We provide visualization tools that help you see how algorithms work, strengthening the connection between conceptual understanding and code implementation.
Conclusion: Building the Bridge Between Understanding and Implementation
The gap between understanding a problem and implementing its solution is a common challenge, but it’s not insurmountable. By recognizing this disconnect and deliberately practicing the skills that bridge it, you can transform from someone who merely comprehends problems to someone who confidently implements solutions.
Remember that this is a journey. Each problem you tackle strengthens the neural pathways that connect analytical understanding to synthetic implementation. The strategies and exercises in this article provide a roadmap for this journey, but consistent practice is the vehicle that will carry you to your destination.
At AlgoCademy, we’re committed to supporting you at every step of this journey. Our platform, resources, and community are designed to help you not just understand problems, but master the art of structuring elegant, efficient solutions.
The next time you find yourself understanding a problem but struggling to structure its solution, remember: this gap is normal, it’s temporary, and with the right approach, you can bridge it. Happy coding!