Why You Understand Solutions But Can’t Solve Coding Problems on Your Own
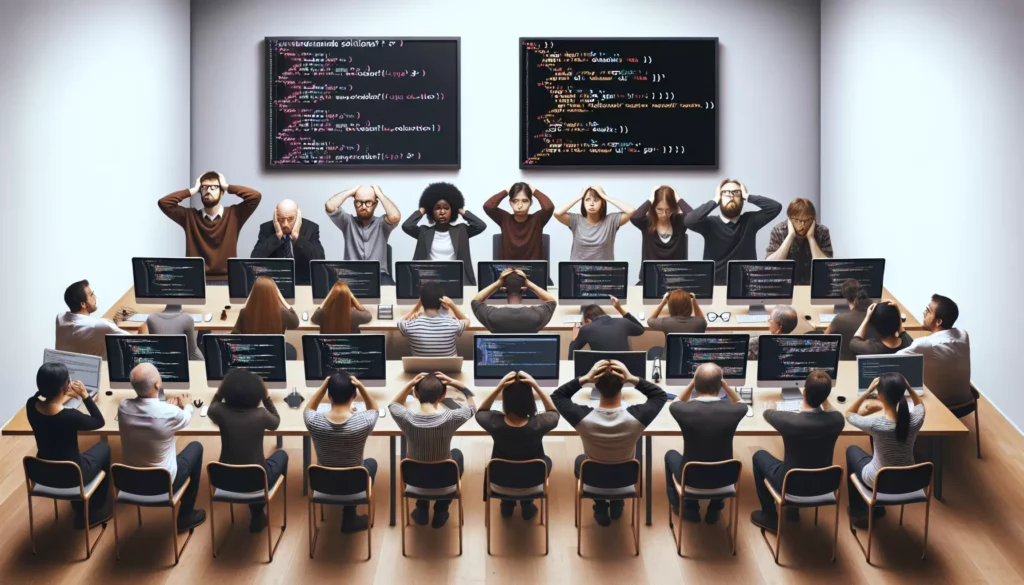
Have you ever experienced that frustrating moment when you look at a solution to a coding problem and think, “That makes perfect sense! Why couldn’t I come up with it myself?” You’re not alone. This phenomenon is incredibly common among programming learners at all levels, from beginners to those preparing for technical interviews at top tech companies.
In this comprehensive guide, we’ll explore the cognitive disconnect between understanding solutions and generating them independently. More importantly, we’ll provide actionable strategies to bridge this gap and transform your problem solving abilities.
The Understanding-Implementation Gap Explained
There’s a fundamental difference between recognizing a solution and creating one from scratch. This cognitive disparity has several root causes that affect programmers at all skill levels.
Recognition vs. Recall
When you read a solution, you’re engaging in recognition memory, which is significantly easier than recall memory required to generate a solution independently. Recognition involves identifying information when presented with it, while recall demands retrieving information without external cues.
Consider this analogy: most people can recognize hundreds of faces they’ve seen before, but would struggle to draw even a few from memory. The same principle applies to coding solutions.
The Illusion of Understanding
Reading a solution often creates an illusion of comprehension. You follow the logic step by step, nodding along, convinced you grasp the concept fully. However, true understanding requires the ability to reconstruct the solution pathway independently.
Research in cognitive psychology shows that passive learning (reading solutions) creates weaker neural connections than active learning (solving problems). When you merely read a solution, you’re not building the neural pathways necessary to retrieve that knowledge under pressure.
Different Cognitive Processes
Understanding solutions and creating them engage different parts of your brain:
- Understanding solutions primarily uses analytical thinking to evaluate existing logic.
- Creating solutions requires synthesis, creativity, and a more complex integration of knowledge.
It’s similar to the difference between appreciating a beautiful chess move and being able to discover it during a match. The latter requires pattern recognition, forward thinking, and creative application of principles.
Common Obstacles to Independent Problem Solving
Before we discuss solutions, let’s identify the specific barriers that might be holding you back from solving problems independently.
Knowledge Fragmentation
You may understand individual concepts but struggle to integrate them into a cohesive solution. For example, you might understand how hash tables work and how to implement breadth first search separately, but fail to see how they can be combined to solve a particular problem.
Starting Point Paralysis
Many programmers freeze when facing a blank editor. Without a clear starting point, the infinite possibilities can be overwhelming. This often manifests as:
- Staring at the problem description without writing any code
- Starting multiple approaches without committing to one
- Overanalyzing edge cases before implementing the core logic
Missing Problem-Solving Frameworks
Experienced programmers rely on mental frameworks to approach problems systematically. Without these frameworks, you might resort to random attempts or immediately look for hints.
Pressure and Time Constraints
The stress of timed coding challenges or technical interviews can significantly impair your problem solving abilities. Under pressure, your working memory capacity decreases, making it harder to hold multiple concepts in mind simultaneously.
Lack of Deliberate Practice
Many learners solve problems randomly without strategic focus on specific patterns or concepts. This scattershot approach leads to slower progress than deliberate, structured practice.
The Path from Understanding to Implementation
Now that we’ve identified the challenges, let’s explore practical strategies to bridge the gap between understanding solutions and creating them independently.
1. Implement Active Recall in Your Learning
Active recall is the practice of retrieving information from memory rather than simply reviewing it. Here’s how to apply this to coding:
- Solution replication: After understanding a solution, close it and try to reimplement it without looking back.
- Spaced repetition: Revisit problems you’ve solved after increasing intervals (1 day, 3 days, 1 week, etc.).
- Explain solutions verbally: Teaching requires deeper understanding than passive consumption.
Research shows that the effort of retrieving information strengthens neural pathways, making future recall easier.
2. Develop a Systematic Problem-Solving Approach
Having a structured method transforms vague problem descriptions into concrete steps:
- Understand the problem: Restate it in your own words and identify inputs, outputs, and constraints.
- Explore examples: Work through sample inputs manually to understand patterns.
- Break it down: Divide the problem into smaller, manageable subproblems.
- Plan your approach: Sketch the algorithm before coding.
- Implement: Write clean, readable code.
- Review and optimize: Look for edge cases and efficiency improvements.
Let’s apply this framework to a simple problem:
Given an array of integers, find two numbers that add up to a target value.
Understand: We need to find indices of two numbers in an array that sum to a target.
Examples:
Input: nums = [2,7,11,15], target = 9
Output: [0,1] (because nums[0] + nums[1] = 2 + 7 = 9)
Break it down:
- We need to check pairs of numbers
- For each number, we need to find if its complement (target – number) exists in the array
Plan: Use a hash map to store visited numbers and their indices. For each number, check if its complement exists in the hash map.
Implement:
function twoSum(nums, target) {
const map = new Map();
for (let i = 0; i < nums.length; i++) {
const complement = target - nums[i];
if (map.has(complement)) {
return [map.get(complement), i];
}
map.set(nums[i], i);
}
return null;
}
Review: This solution has O(n) time complexity and handles all valid inputs correctly.
3. Build Your Pattern Recognition Library
Experienced programmers recognize problem patterns that suggest particular solution approaches. Start building your own pattern library:
- Sliding window: For problems involving subarrays or substrings.
- Two pointers: For searching pairs in sorted arrays or linked list manipulation.
- Breadth-first/Depth-first search: For tree and graph traversal problems.
- Dynamic programming: For optimization problems with overlapping subproblems.
- Binary search: For efficient searching in sorted collections.
When studying solutions, categorize them by pattern rather than by problem. This helps you transfer knowledge to new problems.
4. Practice Incremental Coding
Instead of attempting to code the entire solution at once, build it incrementally:
- Start with a simplified version of the problem
- Implement a working solution for that version
- Gradually add complexity and handle edge cases
For example, when implementing a graph algorithm, first focus on correctly representing the graph, then implement basic traversal, and only then add the specific logic needed for the problem.
This approach provides small wins that build confidence and prevents feeling overwhelmed.
5. Use the Feynman Technique
Named after physicist Richard Feynman, this technique involves explaining concepts in simple terms to identify gaps in your understanding:
- Choose a concept or algorithm
- Explain it as if teaching a beginner
- Identify areas where your explanation falters
- Review and simplify
This process forces you to confront areas of shallow understanding and develop deeper comprehension.
Practical Exercises to Bridge the Gap
Here are specific exercises designed to strengthen your independent problem solving skills:
Solution Reconstruction
After understanding a solution:
- Close the solution
- Wait 30 minutes to clear your working memory
- Reimplement the solution without references
- Compare your implementation with the original
This exercise strengthens recall and helps identify specific concepts you need to review.
Constraint Modification
Take a problem you’ve solved and modify its constraints:
- What if the input could be much larger?
- What if we needed to optimize for space instead of time?
- What if the input structure changed slightly?
These variations force you to adapt your understanding rather than memorize specific solutions.
Algorithmic Journaling
Maintain a problem solving journal with entries for each problem:
- Initial thoughts and approaches
- Obstacles encountered
- Key insights that led to the solution
- Pattern categorization
Reviewing this journal periodically helps identify recurring challenges and track improvement.
Timed Mini-Challenges
Set a timer for 15-20 minutes and attempt to solve a focused, small-scale problem. This builds your ability to work under time constraints without the pressure of a full interview problem.
Pseudocode First
Before writing actual code, write detailed pseudocode for your solution. This separates algorithmic thinking from syntax concerns and helps clarify your approach.
Advanced Strategies for Technical Interview Preparation
If you’re preparing for technical interviews at major tech companies, these additional strategies can help bridge the understanding-implementation gap in high-pressure situations.
Mock Interviews with Verbalization
Practice explaining your thought process while coding. This simulates the interview environment and strengthens the connection between your understanding and implementation.
Schedule regular mock interviews with peers or using platforms that offer this service. Request specific feedback on areas where your implementation doesn’t match your verbal explanation.
Strategic Time Management
In interviews, allocate your time deliberately:
- 5-10% understanding the problem and asking clarifying questions
- 15-20% designing your approach
- 50-60% implementing the solution
- 15-20% testing and optimization
Practice this time allocation in your preparation to develop a rhythm that prevents getting stuck in any one phase.
Develop a Personal Algorithm Template Library
Create simplified templates for common algorithms that you can adapt to specific problems. These aren’t for memorization but for internalizing the structure of effective solutions.
For example, a BFS template might look like:
function bfs(startNode) {
const queue = [startNode];
const visited = new Set([startNode]);
while (queue.length > 0) {
const node = queue.shift();
// Process node here
for (const neighbor of getNeighbors(node)) {
if (!visited.has(neighbor)) {
visited.add(neighbor);
queue.push(neighbor);
}
}
}
}
Having this structure internalized makes it easier to apply BFS to specific problems.
Systematic Review of Failed Attempts
When you fail to solve a problem independently, conduct a thorough review:
- What specific insight was missing from your approach?
- At what point did your solution diverge from the correct path?
- What pattern or technique would have led you to the correct solution?
This targeted analysis is more valuable than simply studying the correct solution.
The Psychology of Problem Solving
Understanding the psychological aspects of problem solving can help you overcome mental barriers.
Embracing Productive Struggle
Research shows that effective learning happens during periods of productive struggle. When you’re stuck on a problem, your brain is forming new neural connections.
Set a “struggle timer” of 20-30 minutes before looking at hints. This builds your tolerance for the discomfort that accompanies deep problem solving.
Growth Mindset in Programming
Adopt a growth mindset about your programming abilities. View challenges as opportunities to develop your skills rather than tests of your fixed ability.
Track your progress over time to see how problems that once seemed impossible become manageable through consistent practice.
Managing Cognitive Load
Complex problem solving places heavy demands on your working memory. Reduce this cognitive load by:
- Taking clear notes during the problem solving process
- Drawing diagrams to visualize the problem
- Breaking the problem into smaller components
- Using consistent variable names and coding patterns
These techniques free up mental resources for the core algorithmic thinking.
Case Study: From Understanding to Implementation
Let’s walk through a complete case study of bridging the understanding-implementation gap for a classic problem.
Problem: Find the longest palindromic substring in a given string.
Initial Understanding Phase:
After reading the solution, you understand that the efficient approach involves:
- Expanding around potential centers of palindromes
- Considering both odd and even length palindromes
- Tracking the longest palindrome found
Bridging Activities:
- Manual simulation: Work through examples by hand, expanding around each potential center.
For the string “babad”:
- Center at ‘b’ (index 0): Just “b”
- Center at ‘a’ (index 1): Just “a”
- Center at ‘b’ (index 2): Expand to “bab”
- Center at ‘a’ (index 3): Just “a”
- Center at ‘d’ (index 4): Just “d”
- Center between indices 0-1: No palindrome
- Center between indices 1-2: “aba”
- And so on…
- Pseudocode development:
function longestPalindrome(s):
if s is empty, return ""
longestSoFar = first character of s
for each possible center position:
check odd length palindrome by expanding around center
check even length palindrome by expanding around center and next character
update longestSoFar if a longer palindrome is found
return longestSoFar
- Incremental implementation: First implement the helper function to expand around centers, then the main function.
Final Implementation:
function longestPalindrome(s) {
if (!s || s.length < 1) return "";
let start = 0;
let maxLength = 1;
function expandAroundCenter(left, right) {
while (left >= 0 && right < s.length && s[left] === s[right]) {
const currentLength = right - left + 1;
if (currentLength > maxLength) {
maxLength = currentLength;
start = left;
}
left--;
right++;
}
}
for (let i = 0; i < s.length; i++) {
// Odd length palindromes
expandAroundCenter(i, i);
// Even length palindromes
expandAroundCenter(i, i + 1);
}
return s.substring(start, start + maxLength);
}
Reinforcement: After implementing the solution, identify the key pattern (expanding around centers) and add it to your pattern library for substring problems.
Common Pitfalls and How to Avoid Them
As you work to bridge the understanding-implementation gap, watch out for these common pitfalls:
Solution Memorization
Pitfall: Attempting to memorize specific solutions rather than understanding principles.
Remedy: Focus on the reasoning behind solutions and the general approaches. Practice applying the same pattern to different problems.
Premature Optimization
Pitfall: Getting stuck trying to find the most efficient solution immediately.
Remedy: Start with a working solution, even if inefficient. You can optimize once you have something functional.
Tutorial Dependency
Pitfall: Relying too heavily on tutorials and walkthroughs.
Remedy: Use the “progressive hint” approach. Try solving problems independently, and only look at minimal hints when truly stuck.
Isolated Practice
Pitfall: Practicing in isolation without feedback or discussion.
Remedy: Join coding communities, participate in code reviews, and explain your solutions to others.
Inconsistent Practice
Pitfall: Sporadic, infrequent practice sessions.
Remedy: Establish a consistent schedule with shorter, regular sessions rather than occasional marathons.
Tools and Resources for Bridging the Gap
Several tools and platforms can help you bridge the understanding-implementation gap:
Interactive Learning Platforms
- AlgoCademy: Offers AI-powered assistance and step-by-step guidance for algorithmic problem solving.
- LeetCode: Provides a vast library of problems with difficulty ratings and company tags.
- HackerRank: Offers skill-based challenges and contests.
Visualization Tools
- Algorithm Visualizers: Tools that graphically demonstrate how algorithms work.
- Code Execution Visualizers: Step through code execution to understand the flow.
Books and Courses
- “Cracking the Coding Interview” by Gayle Laakmann McDowell
- “Algorithm Design Manual” by Steven Skiena
- Stanford’s Algorithms Specialization on Coursera
Community Resources
- Coding Discord servers for real-time discussion
- Reddit communities like r/learnprogramming and r/cscareerquestions
- Local coding meetups for in-person practice and networking
Conclusion: The Journey from Understanding to Mastery
Bridging the gap between understanding solutions and implementing them independently is a crucial step in your development as a programmer. It’s a journey that requires patience, deliberate practice, and strategic learning.
Remember these key takeaways:
- The understanding-implementation gap is a normal part of the learning process, not a reflection of your potential.
- Active recall and deliberate practice are more effective than passive consumption of solutions.
- Developing a systematic problem-solving approach provides structure when facing new challenges.
- Building a pattern recognition library helps you transfer knowledge between similar problems.
- Consistent, focused practice with proper feedback loops accelerates your progress.
By applying the strategies outlined in this guide, you’ll gradually close the gap between recognizing good solutions and creating them independently. This skill is invaluable not just for technical interviews, but for your entire programming career.
The next time you find yourself nodding along to a solution but wondering if you could have created it yourself, remember that with the right approach and consistent practice, you can develop this ability. The journey from understanding to implementation is challenging but ultimately rewarding, transforming you from a code reader into a true problem solver.