Why You Understand Programs but Can’t Design Them
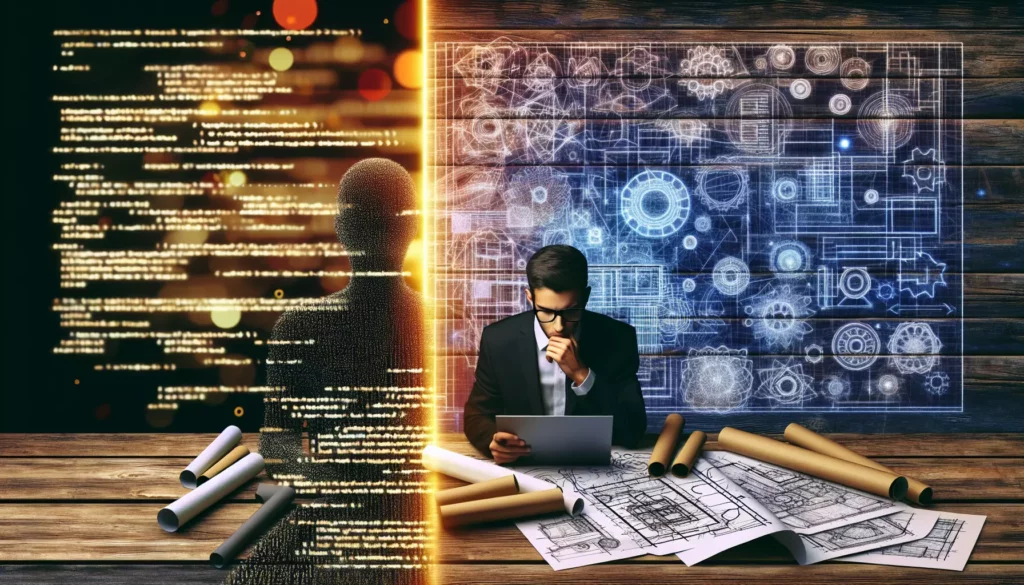
Have you ever found yourself nodding along to programming tutorials, completely understanding the code being explained, only to freeze when faced with a blank editor and a new problem? If so, you’re not alone. This phenomenon, where you can comprehend existing code but struggle to create your own solutions, is incredibly common among programming learners at all levels.
In this article, we’ll explore the gap between understanding and creation in programming, why it exists, and most importantly, how to bridge it. We’ll delve into the cognitive processes involved in both activities and provide actionable strategies to help you transform from a code reader to a code writer.
The Understanding-Creation Gap in Programming
Reading code and writing code engage different cognitive processes. When you read code, you’re following a predetermined path, recognizing patterns, and processing information that’s already structured. Your brain is in recognition mode, which is typically easier than generation mode.
Here’s why understanding existing programs is often easier than designing new ones:
1. Recognition vs. Creation
When you read code, your brain is performing pattern recognition—a task humans are naturally good at. You’re matching what you see against patterns you’ve learned before. This is fundamentally different from the creative process required to design a solution from scratch.
Consider this simple function:
function findMax(arr) {
let max = arr[0];
for (let i = 1; i < arr.length; i++) {
if (arr[i] > max) {
max = arr[i];
}
}
return max;
}
Reading this function, you can quickly understand it finds the maximum value in an array. The logic flows naturally, and each step builds on the previous one. But when asked to write a function to find the maximum value without reference, many beginners struggle with where to start.
2. The Illusion of Understanding
When reading code or following tutorials, we often experience what psychologists call the “illusion of comprehension.” We mistake familiarity with understanding. We think, “That makes sense!” but haven’t truly internalized the underlying principles or problem-solving strategies.
This is similar to watching someone play a musical instrument and thinking, “I could do that,” only to struggle when handed the instrument. Passive understanding doesn’t automatically translate to active skill.
3. Missing Context and Decision-Making
Existing code doesn’t show the thought process that led to its creation. You don’t see the false starts, the debugging, or the alternative approaches that were considered and rejected. You’re seeing the final product without witnessing the journey.
When designing programs, you need to make countless decisions: which data structures to use, how to organize your code, what edge cases to handle, and how to optimize for performance. These decisions aren’t apparent when reading finished code.
The Cognitive Science Behind Program Comprehension vs. Design
To better understand this gap, let’s explore the cognitive processes involved in both activities.
Understanding Programs: Bottom-Up and Top-Down Processing
When we read code, we use two main cognitive processes:
- Bottom-up processing: We examine individual lines and statements, understanding what each piece does.
- Top-down processing: We use our existing knowledge of programming patterns and concepts to form expectations about the code’s purpose and structure.
These processes work together efficiently when reading code, especially if the code follows familiar patterns and conventions.
Designing Programs: Working Memory and Cognitive Load
Program design, on the other hand, places significant demands on our working memory and executive functions:
- Working memory limitations: Humans can only hold about 4-7 items in working memory at once. When designing a program, you need to juggle the problem requirements, potential approaches, algorithm steps, language syntax, and more.
- Cognitive load: The mental effort required to solve problems and make decisions can be exhausting, leading to decision fatigue and “analysis paralysis.”
- Abstract thinking: Programming requires thinking in abstractions, which is a higher-order cognitive skill that develops with practice.
These cognitive demands explain why designing programs is inherently more challenging than understanding them. It’s not a reflection of your intelligence or potential as a programmer—it’s simply how our brains work.
Common Obstacles to Program Design
Let’s examine some specific challenges that make program design difficult, even for those who can read and understand code well.
1. Problem Framing Difficulties
Before writing code, you need to clearly understand and frame the problem. This involves:
- Identifying the inputs and outputs
- Breaking down complex problems into smaller, manageable parts
- Defining edge cases and constraints
Many programmers struggle with this initial step because it requires a different mindset than code comprehension. It’s about asking the right questions rather than finding answers.
2. Starting from a Blank Canvas
The blank editor screen can be intimidating. Without a starting point or template, many programmers feel overwhelmed by the possibilities and uncertain about where to begin.
This “blank page syndrome” isn’t unique to programming—writers, artists, and other creative professionals experience it too. It stems from the cognitive challenge of initiating a creative process without external structure.
3. Algorithmic Thinking Challenges
Designing efficient algorithms requires a particular way of thinking that doesn’t come naturally to most people. It involves:
- Breaking down problems into logical steps
- Identifying patterns and generalizing solutions
- Considering time and space complexity
- Finding edge cases and handling them appropriately
Even when you understand these concepts in theory, applying them to new problems is challenging.
4. Decision Paralysis
Programming offers many ways to solve a problem. Should you use a for loop or map()? Array or object? Recursion or iteration? These decisions can lead to analysis paralysis, where you spend more time deliberating than coding.
Consider this simple task: “Count the frequency of each character in a string.” You could approach this several ways:
// Approach 1: Using a for loop
function countCharacters(str) {
const charCount = {};
for (let i = 0; i < str.length; i++) {
const char = str[i];
charCount[char] = (charCount[char] || 0) + 1;
}
return charCount;
}
// Approach 2: Using reduce
function countCharacters(str) {
return [...str].reduce((acc, char) => {
acc[char] = (acc[char] || 0) + 1;
return acc;
}, {});
}
// Approach 3: Using forEach
function countCharacters(str) {
const charCount = {};
[...str].forEach(char => {
charCount[char] = (charCount[char] || 0) + 1;
});
return charCount;
}
Each approach is valid, but deciding which to use requires weighing factors like readability, performance, and personal preference.
5. Perfectionism and Fear of Failure
Many programmers are perfectionists who hesitate to write code unless they’re confident it’s the “right” or “best” solution. This perfectionism can be paralyzing, especially for beginners who haven’t yet developed confidence in their abilities.
The fear of writing “bad code” or making mistakes can prevent programmers from experimenting and learning through trial and error—a crucial part of skill development.
Bridging the Gap: How to Improve Program Design Skills
Now that we understand the challenges, let’s explore strategies to bridge the gap between understanding and creation.
1. Deliberate Practice: From Reading to Writing
The most effective way to improve your program design skills is through deliberate practice that gradually transitions from reading to writing:
- Read and understand existing code first
- Modify existing code to add features or fix bugs
- Write code with scaffolding (partial solutions or templates)
- Write code from scratch with clear requirements
- Write code from scratch with open-ended requirements
This progression helps you build confidence and skills incrementally, rather than jumping straight from reading to complex creation.
2. Develop a Systematic Problem-Solving Approach
Having a consistent approach to problem-solving can reduce cognitive load and provide structure to the design process:
- Understand the problem: Restate it in your own words. Identify inputs, outputs, and constraints.
- Break it down: Divide the problem into smaller, manageable sub-problems.
- Plan your approach: Sketch an algorithm or solution strategy before coding.
- Start with a simple implementation: Get a basic version working before optimizing.
- Test and refine: Check edge cases and improve your solution.
Following this framework gives you a roadmap for approaching new problems and reduces the feeling of starting from nothing.
3. Practice Algorithmic Thinking
Algorithmic thinking is a skill that improves with practice. Here are ways to develop it:
- Solve algorithm problems regularly: Platforms like LeetCode, HackerRank, and AlgoCademy offer structured problems that help build algorithmic thinking.
- Analyze different solutions: After solving a problem, study alternative approaches to understand the trade-offs.
- Think aloud: Verbalize your thought process while solving problems to make implicit thinking explicit.
- Learn common algorithm patterns: Familiarize yourself with patterns like two pointers, sliding window, and dynamic programming.
Here’s an example of how the same problem can be solved with different algorithmic approaches:
// Problem: Find if a string is a palindrome
// Approach 1: Compare characters from outside in
function isPalindrome(str) {
str = str.toLowerCase().replace(/[^a-z0-9]/g, '');
let left = 0;
let right = str.length - 1;
while (left < right) {
if (str[left] !== str[right]) {
return false;
}
left++;
right--;
}
return true;
}
// Approach 2: Reverse and compare
function isPalindrome(str) {
str = str.toLowerCase().replace(/[^a-z0-9]/g, '');
const reversed = [...str].reverse().join('');
return str === reversed;
}
Understanding both approaches helps you build a repertoire of techniques to apply to new problems.
4. Embrace Incremental Development and Prototyping
Instead of trying to design the perfect solution upfront, embrace an incremental approach:
- Start with a simplified version of the problem
- Write a working prototype, even if it’s inefficient
- Test and validate your approach
- Refine and expand your solution
This approach reduces the cognitive load of program design by breaking it into manageable steps. It also provides early feedback that can guide your development process.
5. Study the Design Process, Not Just the End Result
To truly learn program design, you need to study the process, not just the final code:
- Watch live coding sessions: Observe experienced programmers as they work through problems in real-time.
- Read code with comments explaining design decisions: Look for open-source projects with well-documented code and design rationales.
- Participate in code reviews: Giving and receiving feedback on code design helps develop critical thinking about program structure.
By understanding how experienced programmers approach problems, you can learn the thought processes behind effective program design.
6. Build a Mental Model Library
Experienced programmers have a rich library of mental models and patterns they can apply to new problems. Building your own library takes time, but you can accelerate the process:
- Study common design patterns: Familiarize yourself with established patterns like Singleton, Factory, Observer, etc.
- Learn data structures deeply: Understand when to use arrays, linked lists, trees, graphs, hash tables, and other structures.
- Analyze well-designed code: Study high-quality codebases to see how they structure solutions.
When you encounter a new problem, having these mental models allows you to think, “This is similar to X problem I’ve seen before,” which provides a starting point for your design.
Practical Exercises to Develop Program Design Skills
Let’s explore some specific exercises you can use to bridge the understanding-creation gap.
Exercise 1: Code Reading to Code Writing
- Read a function and explain what it does
- Close the function and try to rewrite it from memory
- Compare your version with the original
- Reflect on differences and understand why they exist
This exercise helps you transition from understanding to creation in a structured way.
Exercise 2: Incremental Complexity
Start with a simple problem and gradually add complexity:
- Write a function to sum an array of numbers
- Modify it to sum only even numbers
- Extend it to allow filtering by any condition
- Implement it using reduce instead of a for loop
This approach allows you to build confidence while gradually increasing the design challenges.
Exercise 3: Reverse Engineering
Given a function’s description and test cases (but not the implementation), write the function:
/*
Function: groupBy
Description: Groups array elements by a key generated from the callback function
Test cases:
groupBy([1, 2, 3, 4, 5], num => num % 2) should return { '0': [2, 4], '1': [1, 3, 5] }
groupBy(['apple', 'banana', 'orange'], fruit => fruit.length) should return { '5': ['apple'], '6': ['banana', 'orange'] }
*/
// Now write the implementation
This exercise simulates real-world programming where you have requirements and expected outputs but need to design the implementation yourself.
Exercise 4: Implementation Variations
Implement the same functionality in multiple ways:
- Using a for loop
- Using functional programming (map, filter, reduce)
- Using recursion
- Using object-oriented design
This exercise helps you see that there are multiple valid approaches to the same problem, reducing the paralysis that comes from seeking the “one right way.”
Exercise 5: Problem Decomposition Practice
Take a complex problem and break it down into sub-problems without writing any code. For example, if building a todo list application, you might decompose it into:
- Data structure to store todos
- Function to add a new todo
- Function to mark a todo as complete
- Function to filter todos by status
- Function to display todos in the UI
This exercise helps develop the crucial skill of breaking down problems before implementation.
The Role of Knowledge in Program Design
While practice is essential, knowledge also plays a crucial role in program design. Let’s explore the types of knowledge that support effective design skills.
1. Language Proficiency
Deep knowledge of your programming language allows you to:
- Express your ideas efficiently without getting stuck on syntax
- Use language features that simplify your code
- Write more idiomatic code that follows language conventions
For example, in JavaScript, understanding array methods like map, filter, and reduce can dramatically change how you approach data transformation problems:
// Without array methods
function getAdultNames(people) {
const adults = [];
for (let i = 0; i < people.length; i++) {
if (people[i].age >= 18) {
adults.push(people[i].name);
}
}
return adults;
}
// With array methods
function getAdultNames(people) {
return people
.filter(person => person.age >= 18)
.map(person => person.name);
}
The second approach is more declarative and often easier to design because it aligns with how we think about the problem: “filter the people to find adults, then get their names.”
2. Data Structures and Algorithms
Knowledge of data structures and algorithms provides templates for solving common problems:
- Need to track frequencies? Use a hash map.
- Need to process data in a specific order? Consider a queue or stack.
- Need to find the shortest path? Use breadth-first search or Dijkstra’s algorithm.
This knowledge gives you starting points for your designs and helps you make informed decisions about efficiency.
3. Design Patterns and Principles
Familiarity with design patterns and principles provides proven solutions to common design problems:
- Single Responsibility Principle: Each function or class should have one reason to change.
- DRY (Don’t Repeat Yourself): Avoid duplicating code by abstracting common functionality.
- Observer Pattern: A way to notify objects about changes to other objects.
These patterns and principles give you frameworks for structuring your code, reducing the cognitive load of design decisions.
4. Domain Knowledge
Understanding the domain you’re working in helps you design more effective solutions:
- You can anticipate requirements and edge cases
- You can use domain-specific terminology in your code
- You can make better trade-offs based on what matters in the domain
This is why experienced developers often emphasize the importance of learning the business domain, not just technical skills.
Psychological Aspects of Program Design
Finally, let’s address the psychological aspects that can impact your ability to design programs effectively.
1. Overcoming Perfectionism
Perfectionism can be a significant barrier to program design. To overcome it:
- Embrace “good enough” solutions: Perfect is the enemy of done.
- Use the “two-solution rule”: Implement a solution, then improve it, rather than trying to find the perfect solution immediately.
- Set time limits: Give yourself a fixed amount of time to design a solution before moving on.
Remember that even experienced programmers rarely write perfect code on the first try. Design is an iterative process.
2. Building Confidence
Confidence in your design abilities comes from:
- Celebrating small wins: Acknowledge when you successfully design and implement a solution, no matter how small.
- Keeping a code journal: Document your learning journey and progress over time.
- Seeking constructive feedback: Use code reviews to validate your approaches and identify areas for improvement.
As your confidence grows, you’ll find it easier to start new designs without second-guessing yourself.
3. Developing a Growth Mindset
A growth mindset—the belief that your abilities can be developed through dedication and hard work—is crucial for improving program design skills:
- View challenges as opportunities: Difficult design problems are chances to grow, not threats to your identity as a programmer.
- Focus on learning, not proving: Approach each design task as a learning experience rather than a test of your abilities.
- Embrace failure as feedback: When a design doesn’t work, see it as valuable information about what to try next.
With a growth mindset, you’ll be more willing to attempt challenging designs and learn from both successes and failures.
Conclusion: From Understanding to Creating
The gap between understanding programs and designing them is real, but it’s not insurmountable. By recognizing the different cognitive processes involved, developing systematic approaches to problem-solving, practicing deliberately, and building your knowledge base, you can bridge this gap and become proficient at program design.
Remember that program design is a skill that develops over time. Even experienced programmers face challenges when designing complex systems. The difference is that they’ve developed strategies and mental models that help them navigate the design process more effectively.
As you continue your programming journey, be patient with yourself. Celebrate your progress, learn from your mistakes, and keep practicing. With time and deliberate effort, you’ll find yourself not just understanding code, but confidently designing elegant solutions to complex problems.
The next time you face a blank editor, remember: you don’t need to create the perfect solution immediately. Start small, build incrementally, and trust the process. Your design skills will grow with each program you create.