Why You Understand Loops But Can’t Design Program Flow
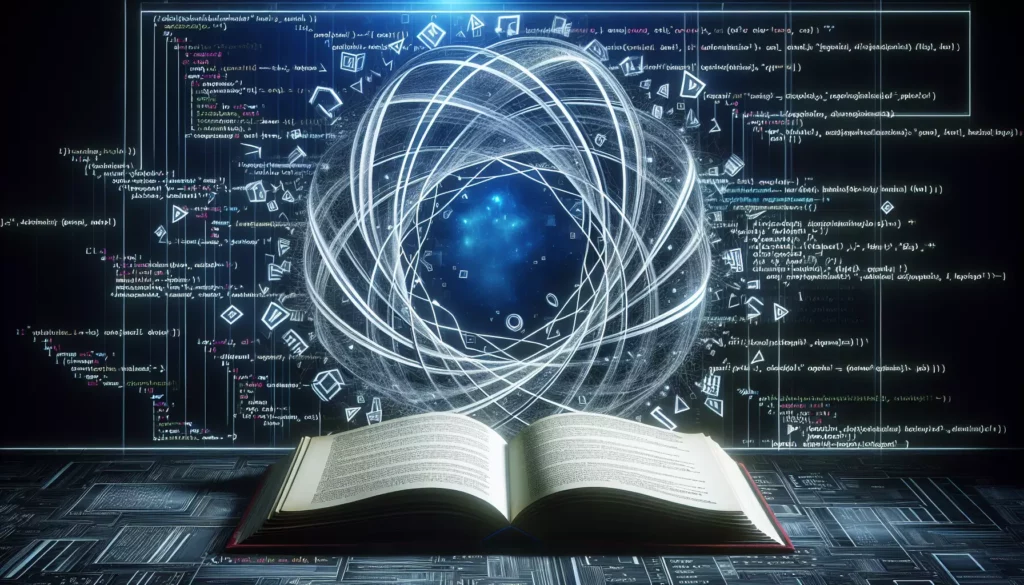
If you’ve ever found yourself confidently writing a for
loop but then struggling to design the overall flow of a program, you’re not alone. This common disconnect affects many programmers, from beginners to those with several years of experience. Understanding why this happens and how to bridge this gap is crucial for your development as a programmer.
The Disconnect Between Syntax Knowledge and Program Design
Many coding learners experience a peculiar phenomenon: they can write individual programming constructs correctly but struggle to piece them together into a coherent, working program. Let’s explore why this happens and how to overcome it.
The Building Blocks vs. The Blueprint
Learning to code often begins with mastering the fundamental building blocks: variables, loops, conditionals, and functions. These are the equivalent of learning individual words and grammar rules when studying a new language. You might be able to construct perfect sentences, but that doesn’t mean you can write a compelling essay.
Program flow design, on the other hand, is about creating the blueprint. It requires understanding how all these building blocks work together to solve a problem efficiently. This is more akin to storytelling or architecture than vocabulary memorization.
The Cognitive Load Difference
Consider the cognitive demands of writing a for
loop versus designing a program:
- Writing a loop: Requires understanding one concept with a specific syntax pattern
- Designing program flow: Requires holding multiple components in your mind simultaneously, understanding their interactions, and planning for various scenarios
The latter is clearly more demanding on your working memory and involves higher-order thinking skills like analysis, synthesis, and evaluation.
Why Learning Syntax Is Easier Than Learning Design
There are several reasons why most programmers find syntax easier to grasp than program design:
Clear Right and Wrong Answers
Syntax has definitive right and wrong answers. A loop either works or produces an error. This binary feedback makes learning syntax straightforward. You know immediately if you’ve mastered the concept.
Program design, however, exists on a spectrum. A program might work but be inefficient, hard to maintain, or difficult to extend. The feedback is nuanced and sometimes delayed.
Abundant Resources and Examples
Syntax is well-documented. Every programming language has comprehensive documentation, tutorials, and examples for basic constructs. You can find the correct syntax for a Python for
loop with a quick search.
Resources on program design are more abstract, diverse, and sometimes contradictory. They require interpretation and judgment to apply to your specific context.
Immediate Application
You can practice syntax immediately after learning it. Write a loop, run it, see the output. The learning cycle is tight and reinforcing.
Practicing program design requires working on larger projects and may take days or weeks to see if your approach was effective. This extended feedback loop makes learning slower and more challenging.
The Mental Models Gap
At the core of this disconnect is often an underdeveloped mental model of how programs actually work. Let’s explore this crucial concept:
What Are Programming Mental Models?
A mental model is an explanation of how something works in your mind. In programming, it’s your understanding of how data flows through a program, how different components interact, and how changes in one area affect others.
Experienced programmers have rich, detailed mental models that allow them to “run the program” in their heads before writing any code. This ability to simulate execution mentally is fundamental to good program design.
The Stages of Mental Model Development
Mental models develop through stages:
- Fragmented understanding: You know individual pieces but not how they connect
- Connected understanding: You can see relationships between components
- Systemic understanding: You can visualize the entire system and predict effects of changes
- Creative application: You can design new systems based on your mental models
Many programmers get stuck at stage 1 or 2, which explains why they can write loops but struggle with program design.
The Trace Table Technique
One effective way to build your mental model is to practice “tracing” code execution manually. Create a table with columns for each variable and step through the code line by line, updating variable values as you go.
For example, tracing this simple loop:
sum = 0
for i in range(1, 5):
sum = sum + i
print(sum)
Would produce this trace table:
Line | i | sum | Output |
---|---|---|---|
1 | undefined | 0 | |
2-3 (first iteration) | 1 | 1 | 1 |
2-3 (second iteration) | 2 | 3 | 3 |
2-3 (third iteration) | 3 | 6 | 6 |
2-3 (fourth iteration) | 4 | 10 | 10 |
This exercise forces you to think about program flow and builds your ability to mentally simulate execution.
Common Program Flow Design Pitfalls
Even programmers who understand syntax well often fall into these design traps:
The “Start Coding Immediately” Trap
Many programmers start writing code before they fully understand the problem or have a clear plan. This leads to a chaotic program structure that’s difficult to debug or extend.
Solution: Resist the urge to code immediately. Spend time understanding the problem, sketching a solution, and planning your approach. This investment pays dividends in reduced debugging time and more maintainable code.
The “Monolithic Function” Syndrome
This occurs when programmers create massive functions that try to do everything instead of breaking the problem down into smaller, focused components.
Solution: Practice identifying distinct responsibilities in your code and extracting them into separate functions. Follow the Single Responsibility Principle: each function should do one thing and do it well.
The “State Confusion” Problem
Many program flow issues stem from poorly managed state. Variables are modified in unexpected ways, leading to difficult-to-track bugs.
Solution: Be intentional about state management. Minimize global variables, clearly document state changes, and consider using immutable data structures where appropriate.
The “Happy Path Only” Mindset
Focusing only on the expected flow of a program without considering edge cases and error conditions leads to fragile code.
Solution: Always ask “What could go wrong here?” and design your program to handle those scenarios gracefully. Consider input validation, error handling, and recovery mechanisms.
Bridging the Gap: From Loops to Program Design
Now that we understand the problem, let’s explore practical strategies to improve your program design skills:
Start with Pseudocode
Before writing actual code, sketch your program’s logic in pseudocode. This allows you to focus on the flow without getting distracted by syntax details.
Example pseudocode for a simple contact management program:
INITIALIZE empty contacts list
FUNCTION display_menu:
PRINT menu options
GET user choice
RETURN user choice
FUNCTION add_contact:
GET contact name
GET contact phone
CREATE new contact record
ADD to contacts list
PRINT confirmation message
FUNCTION main:
WHILE program is running:
choice = display_menu()
IF choice is "add":
add_contact()
ELSE IF choice is "view":
view_contacts()
ELSE IF choice is "exit":
BREAK loop
ELSE:
PRINT invalid choice message
This approach helps you think through the program flow before getting into implementation details.
Visualize with Flowcharts and Diagrams
Visual representations can make program flow clearer. Tools like draw.io, Lucidchart, or even pen and paper can help you map out your program’s logic.
Start with high-level flowcharts showing the main components and their interactions, then drill down into more detailed flows for complex parts.
Practice Breaking Down Problems
Improve your ability to decompose large problems into smaller, manageable pieces:
- Identify the major components or steps needed to solve the problem
- For each component, determine what inputs it needs and what outputs it produces
- Define the interfaces between components clearly
- Implement and test each component independently
- Integrate the components step by step, testing as you go
This divide-and-conquer approach makes complex program design more manageable.
Study Well-Designed Programs
Reading well-structured code is one of the best ways to improve your program design skills. Look for open-source projects known for good design, and study how they organize their code.
Pay attention to:
- How the code is structured into files and modules
- How functions and classes are named and organized
- How data flows through the program
- How the program handles errors and edge cases
Try to understand not just what the code does, but why it’s structured that way.
Design Patterns: Templates for Program Flow
Design patterns are reusable solutions to common programming problems. Learning a few key patterns can dramatically improve your ability to design program flow.
The MVC Pattern for Structured Applications
The Model-View-Controller (MVC) pattern separates your application into three interconnected components:
- Model: Manages data and business logic
- View: Handles display and user interface
- Controller: Coordinates between model and view, handling user input
This separation makes your program easier to understand, test, and modify.
Example of MVC structure for a simple to-do application:
class TodoItem: # Model
def __init__(self, description, completed=False):
self.description = description
self.completed = completed
def mark_completed(self):
self.completed = True
class TodoView: # View
def display_items(self, items):
for i, item in enumerate(items):
status = "✓" if item.completed else " "
print(f"{i+1}. [{status}] {item.description}")
def get_command(self):
return input("Enter command (add/complete/quit): ")
def get_new_item_description(self):
return input("Enter new item: ")
class TodoController: # Controller
def __init__(self):
self.items = []
self.view = TodoView()
def run(self):
while True:
self.view.display_items(self.items)
command = self.view.get_command()
if command == "add":
description = self.view.get_new_item_description()
self.items.append(TodoItem(description))
elif command == "complete":
# Implementation left as exercise
pass
elif command == "quit":
break
else:
print("Unknown command")
The Repository Pattern for Data Management
The Repository pattern provides a clean separation between your data storage logic and the rest of your application. This pattern is especially useful for applications that interact with databases or external APIs.
A simple repository implementation:
class UserRepository:
def __init__(self, database_connection):
self.db = database_connection
def get_by_id(self, user_id):
# Query database for user with given ID
return self.db.execute("SELECT * FROM users WHERE id = ?", [user_id])
def save(self, user):
# Save user to database
if user.id:
# Update existing user
self.db.execute(
"UPDATE users SET name = ?, email = ? WHERE id = ?",
[user.name, user.email, user.id]
)
else:
# Insert new user
user.id = self.db.execute(
"INSERT INTO users (name, email) VALUES (?, ?)",
[user.name, user.email]
)
return user
This pattern allows you to change your data storage mechanism without affecting the rest of your application.
The Observer Pattern for Event-Driven Programs
The Observer pattern is useful for programs where certain events trigger actions in multiple places. It allows for loose coupling between components.
A simple implementation of the Observer pattern:
class Subject:
def __init__(self):
self._observers = []
def attach(self, observer):
if observer not in self._observers:
self._observers.append(observer)
def detach(self, observer):
try:
self._observers.remove(observer)
except ValueError:
pass
def notify(self, *args, **kwargs):
for observer in self._observers:
observer.update(self, *args, **kwargs)
class DataSource(Subject):
def __init__(self):
super().__init__()
self._data = None
@property
def data(self):
return self._data
@data.setter
def data(self, value):
self._data = value
self.notify()
class DataDisplay:
def update(self, subject, *args, **kwargs):
print(f"Data has changed to: {subject.data}")
# Usage
data_source = DataSource()
display1 = DataDisplay()
display2 = DataDisplay()
data_source.attach(display1)
data_source.attach(display2)
data_source.data = 10 # Both displays will be updated
This pattern is especially useful for user interfaces, where changes in data need to be reflected in multiple UI components.
From Theory to Practice: A Structured Approach to Program Design
Let’s put everything together into a practical, step-by-step approach for designing program flow:
Step 1: Understand the Problem Completely
Before writing any code, make sure you thoroughly understand what problem you’re solving:
- What are the inputs and outputs?
- What are the constraints and edge cases?
- What are the performance requirements?
- What are the user’s expectations?
Write these down and refer to them throughout the design process.
Step 2: Break Down the Problem
Decompose the problem into smaller, manageable components:
- Identify the major functions or modules needed
- Define the responsibilities of each component
- Determine how components will interact
Use diagrams or lists to visualize this breakdown.
Step 3: Choose Appropriate Data Structures
Select data structures that effectively represent your problem domain:
- What data will your program manipulate?
- What operations will be performed on this data?
- Which data structures best support these operations?
The right data structures can make your program flow much cleaner and more efficient.
Step 4: Sketch the High-Level Algorithm
Create a high-level plan for how your program will work:
- Use pseudocode or flowcharts
- Focus on the main flow without getting into implementation details
- Identify potential bottlenecks or complex parts
This step helps you see the big picture before diving into code.
Step 5: Design Interfaces Between Components
Clearly define how your components will communicate:
- What inputs does each component need?
- What outputs does it produce?
- How will error conditions be communicated?
Well-designed interfaces make components easier to test and integrate.
Step 6: Implement Components Incrementally
Build your program piece by piece:
- Start with the core functionality
- Implement one component at a time
- Test each component thoroughly before moving on
- Integrate components gradually, testing as you go
This incremental approach helps manage complexity and catch issues early.
Step 7: Refine and Refactor
Continuously improve your design:
- Look for duplicated code or complex sections
- Refactor to improve clarity and maintainability
- Add error handling and edge case management
- Optimize performance where needed
Remember that good design is an iterative process.
Case Study: Redesigning a Simple Program
Let’s apply these principles to transform a poorly designed program into a well-structured one.
The Original Program: A Weather Data Analyzer
Here’s a program that reads weather data from a file and performs some analysis:
def analyze_weather():
# Open file and read data
f = open("weather_data.csv", "r")
lines = f.readlines()
f.close()
# Process data
temperatures = []
humidity_values = []
for i in range(1, len(lines)): # Skip header
data = lines[i].strip().split(",")
temperatures.append(float(data[1]))
humidity_values.append(float(data[2]))
# Calculate statistics
avg_temp = sum(temperatures) / len(temperatures)
max_temp = max(temperatures)
min_temp = min(temperatures)
avg_humidity = sum(humidity_values) / len(humidity_values)
# Print results
print(f"Average temperature: {avg_temp:.2f}°C")
print(f"Maximum temperature: {max_temp:.2f}°C")
print(f"Minimum temperature: {min_temp:.2f}°C")
print(f"Average humidity: {avg_humidity:.2f}%")
# Find days above 30°C
hot_days = 0
for temp in temperatures:
if temp > 30:
hot_days += 1
print(f"Number of hot days (>30°C): {hot_days}")
# Run the analysis
analyze_weather()
This code has several issues:
- It does everything in one function
- It doesn’t handle errors
- It mixes data loading, processing, and presentation
- It’s hard to reuse or extend
The Redesigned Program
Let’s redesign this program using the principles we’ve discussed:
class WeatherData:
def __init__(self, date, temperature, humidity):
self.date = date
self.temperature = temperature
self.humidity = humidity
class WeatherDataRepository:
@staticmethod
def load_from_csv(file_path):
weather_data = []
try:
with open(file_path, "r") as file:
lines = file.readlines()
# Skip header
for i in range(1, len(lines)):
try:
data = lines[i].strip().split(",")
date = data[0]
temperature = float(data[1])
humidity = float(data[2])
weather_data.append(WeatherData(date, temperature, humidity))
except (IndexError, ValueError) as e:
print(f"Error processing line {i+1}: {e}")
continue
except FileNotFoundError:
print(f"Error: File {file_path} not found")
except Exception as e:
print(f"Error loading data: {e}")
return weather_data
class WeatherAnalyzer:
def __init__(self, weather_data):
self.weather_data = weather_data
def get_temperature_statistics(self):
if not self.weather_data:
return None, None, None
temperatures = [data.temperature for data in self.weather_data]
avg_temp = sum(temperatures) / len(temperatures)
max_temp = max(temperatures)
min_temp = min(temperatures)
return avg_temp, max_temp, min_temp
def get_average_humidity(self):
if not self.weather_data:
return None
humidity_values = [data.humidity for data in self.weather_data]
return sum(humidity_values) / len(humidity_values)
def count_days_above_temperature(self, threshold):
return sum(1 for data in self.weather_data if data.temperature > threshold)
class WeatherReporter:
@staticmethod
def print_analysis_report(analyzer):
avg_temp, max_temp, min_temp = analyzer.get_temperature_statistics()
if avg_temp is None:
print("No data available for analysis")
return
avg_humidity = analyzer.get_average_humidity()
hot_days = analyzer.count_days_above_temperature(30)
print(f"Weather Analysis Report")
print(f"----------------------")
print(f"Average temperature: {avg_temp:.2f}°C")
print(f"Maximum temperature: {max_temp:.2f}°C")
print(f"Minimum temperature: {min_temp:.2f}°C")
print(f"Average humidity: {avg_humidity:.2f}%")
print(f"Number of hot days (>30°C): {hot_days}")
def main():
# Load data
data = WeatherDataRepository.load_from_csv("weather_data.csv")
if not data:
print("No data available. Exiting.")
return
# Analyze data
analyzer = WeatherAnalyzer(data)
# Report results
WeatherReporter.print_analysis_report(analyzer)
if __name__ == "__main__":
main()
The redesigned program has several improvements:
- Separation of concerns: Data loading, analysis, and reporting are separated
- Error handling: Various error conditions are handled gracefully
- Reusability: Components can be used independently
- Extensibility: New analyses or data sources can be added easily
- Readability: Each component has a clear, focused responsibility
Moving Beyond the Basics: Advanced Program Flow Concepts
As you grow more comfortable with basic program design, consider these advanced concepts:
State Management Strategies
Managing state effectively is crucial for complex applications:
- Immutable data structures: Using immutable objects can make your program flow more predictable
- State machines: Formalizing state transitions helps manage complex workflows
- Centralized state management: Patterns like Redux in web development provide a single source of truth
Asynchronous Programming
Modern applications often need to handle operations that don’t complete immediately:
- Callbacks: Functions passed as arguments to be executed after an operation completes
- Promises/Futures: Objects representing the eventual result of an asynchronous operation
- Async/Await: Syntactic sugar that makes asynchronous code look synchronous
Understanding these patterns is essential for designing responsive applications.
Event-Driven Architecture
Many modern applications use events to coordinate between components:
- Event emitters: Components that broadcast events to interested listeners
- Message queues: Systems for passing messages between components, possibly across different services
- Reactive programming: A paradigm focused on data streams and the propagation of changes
These approaches can lead to more loosely coupled and scalable systems.
Conclusion: Bridging the Gap
The journey from understanding loops to mastering program design is challenging but rewarding. It requires moving beyond syntax to develop a deep understanding of how programs work and how components interact.
Remember these key takeaways:
- Program design is a different skill set from syntax knowledge
- Developing strong mental models is essential for effective design
- Break problems down into manageable components
- Plan before coding, using pseudocode and diagrams
- Study well-designed code to learn patterns and practices
- Practice incrementally, building from simple to complex designs
With deliberate practice and patience, you can bridge the gap between understanding loops and designing elegant, effective programs. The skills you develop will serve you throughout your programming career, making you more effective at solving complex problems and creating maintainable software.
Remember that program design is as much an art as it is a science. There’s rarely a single “right” design, but there are designs that are clearer, more maintainable, and more adaptable than others. Developing your design intuition takes time, but the investment pays off in programs that are easier to understand, maintain, and extend.