Why You Understand Functions But Can’t Build Full Applications
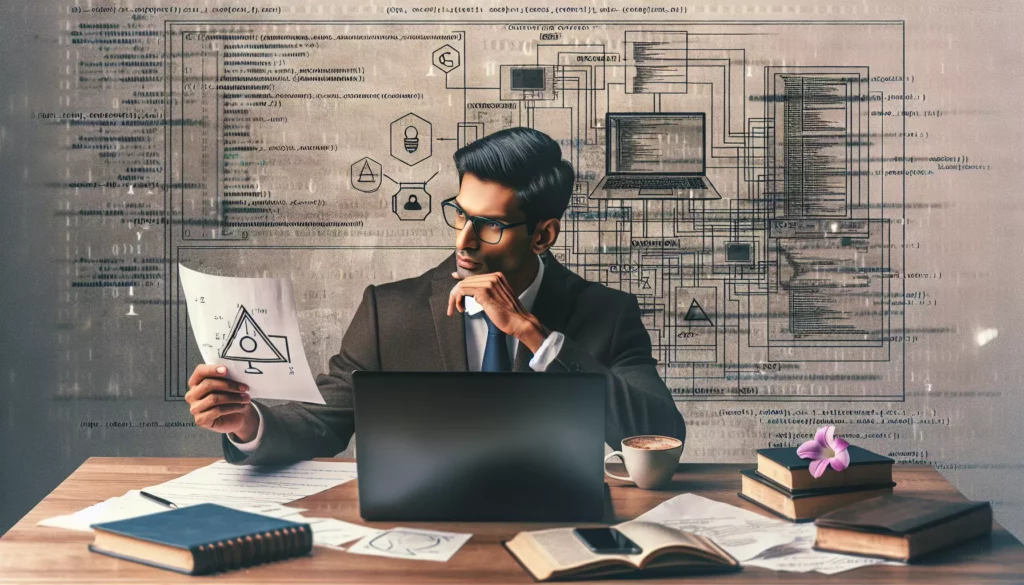
Many coding learners face a common frustration: they understand individual programming concepts like functions, loops, and conditionals, but struggle when trying to build complete applications. You might find yourself nodding along during tutorials, confidently writing small code snippets, yet feeling completely lost when staring at a blank file wondering how to create a full project from scratch.
This disconnect between understanding components and building complete systems is normal, but it’s also a significant hurdle in your programming journey. In this article, we’ll explore why this gap exists and provide actionable strategies to bridge it.
The Function-to-Application Gap: Why It Exists
Before diving into solutions, let’s understand why this gap between understanding functions and building applications is so common.
1. Tutorial Syndrome
When learning to code, many of us fall into what’s called “tutorial syndrome” – the false sense of mastery that comes from following guided examples. You watch a tutorial, type along with the instructor, and everything makes perfect sense. But when you try to create something on your own, you freeze.
This happens because following a tutorial engages your recognition skills rather than your recall abilities. Recognition (identifying information when presented with it) is much easier than recall (retrieving information from memory without prompts). When building an application from scratch, you need recall, which is a more difficult cognitive skill.
2. Missing the Forest for the Trees
Programming education often focuses on teaching individual concepts in isolation. You learn about variables, then functions, then objects, and so on. This approach makes sense for introducing concepts gradually, but it can leave you without an understanding of how these pieces fit together in a cohesive application.
It’s like learning all the parts of a car engine separately without ever seeing how they work together to power a vehicle.
3. Architecture and Design Skills Gap
Building applications requires more than just coding knowledge – it demands architectural thinking and design skills that are rarely taught explicitly. These include:
- How to structure code across multiple files
- How to manage application state
- How to handle user interactions and events
- How to design interfaces between components
- How to make systems extensible and maintainable
These skills are often acquired through experience rather than direct instruction, creating a catch-22 for beginners.
4. The Complexity Jump
The cognitive load required to manage a complete application is exponentially higher than what’s needed to understand a single function. In an application, you’re juggling multiple concerns simultaneously:
- User interface considerations
- Data management
- Error handling
- Performance optimization
- Security concerns
- External integrations
This sudden increase in complexity can be overwhelming, even if each individual component makes sense on its own.
Bridging the Gap: From Functions to Full Applications
Now that we understand the challenges, let’s explore strategies to overcome them and develop the skills needed to build complete applications.
1. Start with Tiny Applications, Not Just Functions
Instead of practicing isolated functions, challenge yourself to build tiny but complete applications. These don’t need to be complex or impressive – the goal is to get comfortable with the full development cycle.
Here are some ideas for small applications to practice with:
- A command-line calculator
- A simple to-do list manager
- A basic weather information fetcher using an API
- A timer or stopwatch application
Even these simple projects will force you to think about program structure, user interaction, and data flow – all crucial skills for larger applications.
2. Study Application Architecture
Take time to learn about common architectural patterns and how they organize code. Understanding these patterns gives you mental models to follow when building your own applications.
Some foundational patterns to study include:
- MVC (Model-View-Controller): Separates application logic into three interconnected components
- Component-based architecture: Used in frameworks like React and Vue
- Repository pattern: Abstracts data access logic
- Service-oriented architecture: Organizes functionality into distinct services
Don’t just read about these patterns – implement simple versions to understand how they work in practice.
3. Reverse Engineer Existing Applications
One of the most effective ways to learn application development is to study existing codebases. Find open-source projects similar to what you want to build and analyze their structure.
When examining these projects, ask yourself:
- How is the code organized into files and directories?
- How do different components communicate with each other?
- How is state managed throughout the application?
- What design patterns are being used?
This approach gives you practical examples of how experienced developers tackle application architecture.
4. Use the “Expand and Refactor” Method
Start with a simple working program, then gradually expand it while periodically refactoring to maintain clean code. This incremental approach prevents you from getting overwhelmed by complexity.
For example, if building a to-do application:
- Start with just the ability to add items to a list
- Add the ability to mark items as complete
- Refactor to improve code organization
- Add the ability to delete items
- Refactor again
- Add persistence to save items between sessions
This gradual expansion allows you to maintain a working application at each step while slowly increasing its capabilities.
5. Learn to Plan Before Coding
Professional developers rarely start coding immediately. They plan their application structure, often using diagrams and documentation to map out components and their interactions.
Before writing code, spend time on:
- Identifying the core features and requirements
- Sketching the user interface (if applicable)
- Mapping out data models and their relationships
- Defining the main components and their responsibilities
- Planning the communication between components
This planning phase helps you see the big picture before getting lost in implementation details.
Practical Examples: From Function to Application
Let’s walk through some concrete examples to illustrate the difference between understanding functions and building applications.
Example 1: Calculator Function vs. Calculator Application
First, here’s a simple calculator function that many beginners could write:
function calculate(a, b, operation) {
switch(operation) {
case "add":
return a + b;
case "subtract":
return a - b;
case "multiply":
return a * b;
case "divide":
if (b === 0) return "Error: Division by zero";
return a / b;
default:
return "Invalid operation";
}
}
// Usage
console.log(calculate(5, 3, "add")); // 8
This function works fine, but it’s far from a calculator application. A complete calculator application would need:
- A user interface (whether command-line or graphical)
- Input validation and error handling
- A way to display results
- Memory functions (like storing previous calculations)
- A main program loop or event handlers
Here’s a simplified example of what a command-line calculator application might look like:
// calculator.js
const readline = require('readline');
class Calculator {
constructor() {
this.memory = 0;
this.history = [];
}
calculate(a, b, operation) {
let result;
switch(operation) {
case "add":
result = a + b;
break;
case "subtract":
result = a - b;
break;
case "multiply":
result = a * b;
break;
case "divide":
if (b === 0) throw new Error("Division by zero");
result = a / b;
break;
default:
throw new Error("Invalid operation");
}
this.history.push({ a, b, operation, result });
return result;
}
storeInMemory(value) {
this.memory = value;
}
getMemory() {
return this.memory;
}
getHistory() {
return this.history;
}
}
class CalculatorApp {
constructor() {
this.calculator = new Calculator();
this.rl = readline.createInterface({
input: process.stdin,
output: process.stdout
});
}
start() {
console.log("Welcome to Calculator App!");
this.showMenu();
}
showMenu() {
console.log("\nOptions:");
console.log("1. Perform calculation");
console.log("2. View history");
console.log("3. Exit");
this.rl.question("Enter your choice: ", (choice) => {
switch(choice) {
case "1":
this.performCalculation();
break;
case "2":
this.showHistory();
break;
case "3":
this.exit();
break;
default:
console.log("Invalid choice. Please try again.");
this.showMenu();
}
});
}
performCalculation() {
this.rl.question("Enter first number: ", (a) => {
this.rl.question("Enter second number: ", (b) => {
this.rl.question("Enter operation (add, subtract, multiply, divide): ", (operation) => {
try {
const result = this.calculator.calculate(parseFloat(a), parseFloat(b), operation);
console.log(`Result: ${result}`);
} catch (error) {
console.log(`Error: ${error.message}`);
}
this.showMenu();
});
});
});
}
showHistory() {
const history = this.calculator.getHistory();
if (history.length === 0) {
console.log("No calculations performed yet.");
} else {
console.log("\nCalculation History:");
history.forEach((entry, index) => {
console.log(`${index + 1}. ${entry.a} ${entry.operation} ${entry.b} = ${entry.result}`);
});
}
this.showMenu();
}
exit() {
console.log("Thank you for using Calculator App!");
this.rl.close();
}
}
// Run the application
const app = new CalculatorApp();
app.start();
Notice the significant increase in complexity! This application includes:
- A class structure to organize code
- State management (history and memory)
- User interface handling
- Input validation and error handling
- Program flow control
Example 2: Todo List Function vs. Todo List Application
Here’s a simple function to manage a todo list:
function manageTodo(todos, action, todoText, todoId) {
switch(action) {
case "add":
return [...todos, { id: Date.now(), text: todoText, completed: false }];
case "toggle":
return todos.map(todo =>
todo.id === todoId ? { ...todo, completed: !todo.completed } : todo
);
case "delete":
return todos.filter(todo => todo.id !== todoId);
default:
return todos;
}
}
// Usage
let myTodos = [];
myTodos = manageTodo(myTodos, "add", "Learn JavaScript");
console.log(myTodos);
This function handles the core logic, but a complete todo list application would need much more:
// todoApp.js
const fs = require('fs').promises;
const path = require('path');
const readline = require('readline');
class TodoModel {
constructor() {
this.todos = [];
this.dataFile = path.join(__dirname, 'todos.json');
}
async load() {
try {
const data = await fs.readFile(this.dataFile, 'utf8');
this.todos = JSON.parse(data);
} catch (error) {
// File doesn't exist or is invalid, start with empty array
this.todos = [];
}
}
async save() {
await fs.writeFile(this.dataFile, JSON.stringify(this.todos, null, 2), 'utf8');
}
addTodo(text) {
const newTodo = {
id: Date.now(),
text,
completed: false,
createdAt: new Date().toISOString()
};
this.todos.push(newTodo);
return newTodo;
}
toggleTodo(id) {
const todo = this.todos.find(todo => todo.id === id);
if (todo) {
todo.completed = !todo.completed;
return true;
}
return false;
}
deleteTodo(id) {
const initialLength = this.todos.length;
this.todos = this.todos.filter(todo => todo.id !== id);
return this.todos.length !== initialLength;
}
getAllTodos() {
return this.todos;
}
getCompletedTodos() {
return this.todos.filter(todo => todo.completed);
}
getPendingTodos() {
return this.todos.filter(todo => !todo.completed);
}
}
class TodoView {
constructor() {
this.rl = readline.createInterface({
input: process.stdin,
output: process.stdout
});
}
showMenu() {
console.log("\n=== TODO APP ===");
console.log("1. View all todos");
console.log("2. View pending todos");
console.log("3. View completed todos");
console.log("4. Add new todo");
console.log("5. Mark todo as completed/pending");
console.log("6. Delete todo");
console.log("7. Exit");
}
askForOption() {
return new Promise(resolve => {
this.rl.question("\nSelect an option: ", answer => {
resolve(answer.trim());
});
});
}
askForText() {
return new Promise(resolve => {
this.rl.question("Enter todo text: ", answer => {
resolve(answer.trim());
});
});
}
askForId() {
return new Promise(resolve => {
this.rl.question("Enter todo ID: ", answer => {
resolve(parseInt(answer.trim()));
});
});
}
displayTodos(todos) {
if (todos.length === 0) {
console.log("\nNo todos found.");
return;
}
console.log("\nYour Todos:");
todos.forEach(todo => {
const status = todo.completed ? "[x]" : "[ ]";
console.log(`${todo.id}: ${status} ${todo.text}`);
});
}
displayMessage(message) {
console.log(`\n${message}`);
}
close() {
this.rl.close();
}
}
class TodoController {
constructor(model, view) {
this.model = model;
this.view = view;
this.running = false;
}
async start() {
await this.model.load();
this.running = true;
this.runLoop();
}
async runLoop() {
while (this.running) {
this.view.showMenu();
const option = await this.view.askForOption();
switch(option) {
case '1':
this.view.displayTodos(this.model.getAllTodos());
break;
case '2':
this.view.displayTodos(this.model.getPendingTodos());
break;
case '3':
this.view.displayTodos(this.model.getCompletedTodos());
break;
case '4':
await this.addTodo();
break;
case '5':
await this.toggleTodo();
break;
case '6':
await this.deleteTodo();
break;
case '7':
this.exit();
break;
default:
this.view.displayMessage("Invalid option. Please try again.");
}
}
}
async addTodo() {
const text = await this.view.askForText();
if (text) {
this.model.addTodo(text);
await this.model.save();
this.view.displayMessage("Todo added successfully!");
} else {
this.view.displayMessage("Todo cannot be empty.");
}
}
async toggleTodo() {
this.view.displayTodos(this.model.getAllTodos());
const id = await this.view.askForId();
if (this.model.toggleTodo(id)) {
await this.model.save();
this.view.displayMessage("Todo status updated!");
} else {
this.view.displayMessage("Todo not found.");
}
}
async deleteTodo() {
this.view.displayTodos(this.model.getAllTodos());
const id = await this.view.askForId();
if (this.model.deleteTodo(id)) {
await this.model.save();
this.view.displayMessage("Todo deleted successfully!");
} else {
this.view.displayMessage("Todo not found.");
}
}
exit() {
this.view.displayMessage("Thank you for using Todo App!");
this.running = false;
this.view.close();
}
}
// Run the application
async function main() {
const model = new TodoModel();
const view = new TodoView();
const controller = new TodoController(model, view);
await controller.start();
}
main().catch(error => {
console.error("An error occurred:", error);
});
This complete application demonstrates:
- The MVC (Model-View-Controller) architecture pattern
- Persistence using file storage
- User interface management
- Error handling
- Advanced state management
- Asynchronous operations
Common Roadblocks and How to Overcome Them
As you work to bridge the gap between understanding functions and building applications, you’ll likely encounter several common roadblocks. Here’s how to recognize and overcome them:
1. Analysis Paralysis
Symptom: You spend excessive time planning without writing any code, overwhelmed by decisions about architecture, libraries, and design patterns.
Solution: Embrace the “minimum viable product” approach. Start with the simplest version that works, even if it’s not perfect. You can always refactor later. Set a time limit for your planning phase, then force yourself to start coding.
2. Dependency Hell
Symptom: You get lost in a maze of libraries, frameworks, and tools, spending more time configuring your environment than writing application code.
Solution: For learning projects, minimize dependencies. Start with vanilla programming languages before adding frameworks. When you do use libraries, choose established options with good documentation and strong community support.
3. Scope Creep
Symptom: Your project keeps growing in complexity as you think of new features to add, making it impossible to complete.
Solution: Write down your core requirements before starting. Create a separate “nice-to-have” list for additional features. Commit to building the core functionality first, then adding extras only after the basics work well.
4. Integration Challenges
Symptom: Individual components work fine in isolation, but break when you try to connect them.
Solution: Design clear interfaces between components from the start. Use integration tests to verify that components work together. Build your application incrementally, testing integration at each step rather than at the end.
5. State Management Confusion
Symptom: Your application behaves unpredictably as data changes throughout program execution.
Solution: Centralize your state management. Depending on the application type, this might mean using a state management library, a central store pattern, or simply being disciplined about how state is passed between components. Draw diagrams of your data flow to visualize how state changes propagate.
Learning Pathways: Structured Approaches to Building Application Skills
To systematically build your application development skills, consider these learning pathways:
Pathway 1: The Project-Based Approach
Build a series of increasingly complex applications, learning new concepts as needed:
- Command-line utility (e.g., a file organizer or note-taking app)
- Simple web application with basic CRUD operations
- Multi-page application with authentication and database integration
- Full-stack application with advanced features like real-time updates
This approach teaches you to build complete systems while progressively introducing new concepts.
Pathway 2: The Architecture-First Approach
Focus on understanding application structure before building complex features:
- Study common architectural patterns (MVC, MVVM, etc.)
- Implement simple applications using different patterns
- Analyze the strengths and weaknesses of each approach
- Build more complex applications using the most appropriate architecture
This approach gives you a strong foundation in software design principles.
Pathway 3: The Clone-and-Extend Approach
Learn by recreating existing applications and then extending them:
- Clone a simple open-source application
- Analyze its structure and architecture
- Add new features to the cloned application
- Refactor and improve the codebase
This approach gives you practical experience with real-world codebases while providing a scaffolding to build upon.
Resources for Mastering Application Development
Here are some resources specifically focused on bridging the gap between understanding functions and building applications:
Books
- “Clean Architecture” by Robert C. Martin – Provides principles for organizing code in large applications
- “Design Patterns: Elements of Reusable Object-Oriented Software” by Gamma, Helm, Johnson, and Vlissides – Classic reference on software design patterns
- “Head First Design Patterns” by Eric Freeman and Elisabeth Robson – More accessible introduction to design patterns
- “Software Engineering at Google” by Titus Winters, Tom Manshreck, and Hyrum Wright – Insights into building and maintaining large-scale applications
Online Courses
- Full Stack Open – Free course on modern web application development
- App Academy Open – Free full-stack curriculum
- The Odin Project – Project-based web development curriculum
- CS50’s Web Programming with Python and JavaScript – Harvard’s course on web application development
YouTube Channels
- Traversy Media – Project-based tutorials on web development
- The Net Ninja – Step-by-step application building tutorials
- Coding Garden – Live coding sessions building complete applications
- ArjanCodes – Focus on software design and architecture
GitHub Repositories
- Build Your Own X – Tutorials for building various applications from scratch
- Project Based Learning – Programming tutorials through project-based learning
- App Ideas Collection – A collection of application ideas for different skill levels
Conclusion: The Path Forward
The gap between understanding functions and building applications is real, but it’s not insurmountable. By recognizing this challenge and taking a structured approach to developing your architectural thinking and system design skills, you can bridge this gap.
Remember that application development is a skill that improves with practice. Each application you build, no matter how simple, teaches you valuable lessons about structure, state management, and integration that will serve you in more complex projects.
Start small, be patient with yourself, and focus on completing projects rather than making them perfect. Over time, you’ll develop an intuitive understanding of how to structure applications effectively, and the process will become more natural.
The journey from writing functions to building applications is a significant step in your programming career, marking the transition from a coding beginner to a software developer. Embrace the challenges along the way, as they’re essential to your growth as a programmer.
Happy coding!