Why You Understand Example Solutions But Can’t Adapt Them
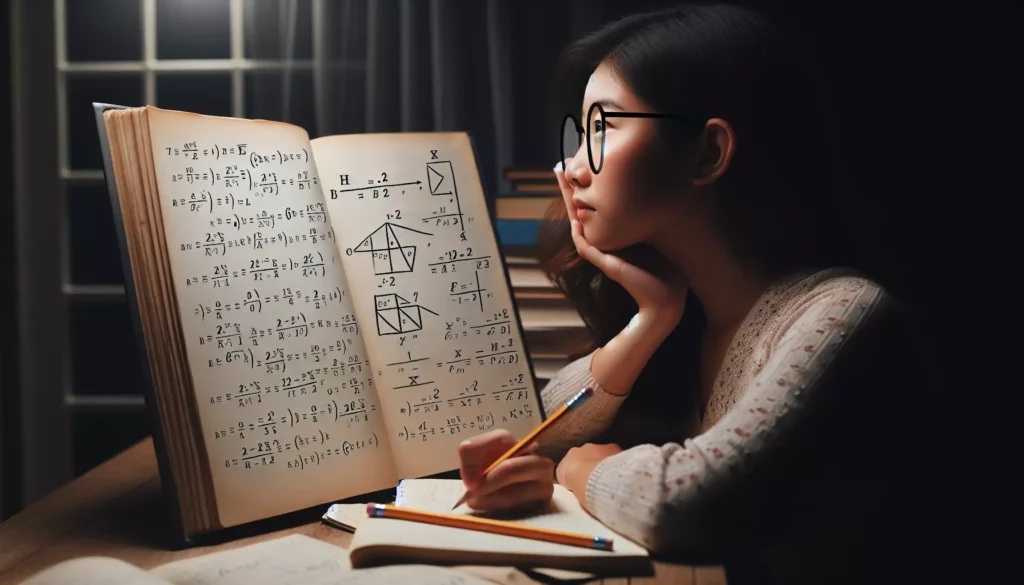
Have you ever found yourself nodding along to a coding tutorial, completely understanding the solution presented, only to freeze when faced with a slightly different problem on your own? This phenomenon, where comprehension doesn’t translate to application, is incredibly common among programming learners at all levels.
In this article, we’ll explore why many learners struggle to bridge the gap between understanding example solutions and creating their own, and provide practical strategies to overcome this challenge.
The Illusion of Understanding
When we read through a well-explained solution to a coding problem, our brains often trick us into believing we’ve mastered the concept. This is what cognitive scientists call the “illusion of understanding” or “knowledge illusion.”
Passive vs. Active Learning
Reading or watching someone solve a problem is passive learning. Your brain processes information without having to generate solutions independently. This requires significantly less cognitive effort than active learning, where you must retrieve knowledge and apply it to new situations.
Consider this analogy: watching someone play piano beautifully might make you feel like you understand how to play, but sitting at the keyboard yourself quickly reveals the gap between understanding and ability.
Recognition vs. Recall
When reviewing example solutions, you’re engaging in recognition—seeing familiar patterns and nodding along. But when solving problems independently, you need recall—retrieving concepts from memory without prompts.
Research shows recognition is far easier than recall. This explains why you might read a solution and think, “That makes perfect sense; I could have done that,” but struggle to produce similar solutions when starting from scratch.
Common Scenarios Where This Happens
Algorithm Challenges
You review a solution for finding the maximum subarray sum using Kadane’s algorithm. The explanation makes perfect sense:
def max_subarray(nums):
current_sum = max_sum = nums[0]
for num in nums[1:]:
current_sum = max(num, current_sum + num)
max_sum = max(max_sum, current_sum)
return max_sum
But when faced with a variation like “find the maximum product subarray,” you struggle to adapt the approach.
Data Structure Implementation
You understand how a binary search tree works when reading the implementation, but creating your own methods for traversing or modifying the tree proves challenging.
Framework Usage
Following a React tutorial seems straightforward, but building your own component with different requirements leaves you searching for examples that match your exact needs.
The Root Causes
1. Mental Models Gap
Expert programmers have developed robust mental models—conceptual frameworks that help them understand how programming concepts connect and interact. Beginners often have fragmented mental models with missing connections.
When you understand an example solution, you’re temporarily borrowing the author’s mental model. Without a strong mental model of your own, adaptation becomes difficult.
2. Pattern Recognition Limitations
Programming involves recognizing patterns and applying appropriate solutions. Experts have cataloged hundreds or thousands of patterns through experience.
As a learner, your pattern library is still developing. You might recognize a pattern when it’s pointed out but lack the experience to identify it independently in new contexts.
3. Working Memory Constraints
Our working memory—the mental workspace where we manipulate information—is limited. When learning new programming concepts, these limitations become apparent.
Example solutions offload this cognitive burden by presenting information in an organized manner. When solving problems independently, you must juggle multiple concepts simultaneously, which can overwhelm working memory.
4. Contextual Knowledge Gaps
Example solutions often contain implicit knowledge the author assumes you possess. These assumptions might include:
- Language-specific idioms and best practices
- Performance considerations
- Edge cases and error handling
- System design principles
Without this contextual knowledge, adapting solutions becomes challenging.
The Transfer Problem in Learning to Code
What we’re discussing is fundamentally a transfer problem—the ability to apply knowledge learned in one context to a new situation. Transfer is the holy grail of education, yet it’s notoriously difficult to achieve.
Near vs. Far Transfer
Educational psychologists distinguish between near transfer (applying knowledge to similar contexts) and far transfer (applying knowledge to dissimilar contexts).
Most coding education inadvertently focuses on near transfer—solving problems very similar to examples. But real-world programming requires far transfer, where you apply concepts in novel ways across different domains.
Surface vs. Deep Structure
Novices tend to focus on surface features of problems (the specific variables, language syntax, or problem domain), while experts recognize the deep structure (the underlying patterns and principles).
This focus on surface features makes it difficult to see how a solution from one context applies to a seemingly different problem with the same underlying structure.
Practical Strategies to Bridge the Gap
Now that we understand the problem, let’s explore practical strategies to improve your ability to adapt solutions and solve problems independently.
1. Implement Active Recall Practices
Instead of passively reading solutions, actively engage with the material:
- Close the book/tutorial: After reading a solution, close the resource and try to reimplement it from memory.
- Explain the solution: Verbalize or write out the solution in your own words, explaining why each step is necessary.
- Teach someone else: The “Feynman Technique” involves teaching concepts to others, which forces you to clarify your understanding.
2. Practice Deliberate Variations
After understanding an example solution, deliberately create variations:
- Change constraints: What if the input is sorted? What if we need to optimize for space instead of time?
- Reverse the problem: If the original problem finds a maximum, modify it to find a minimum.
- Combine problems: Take two problems you’ve solved and create a new problem that requires elements of both solutions.
For example, after learning how to find the maximum subarray sum, try these variations:
- Find the maximum subarray product
- Find the subarray with the smallest sum
- Find the subarray with sum closest to a target value
3. Develop Your Mental Models
Strengthen your conceptual understanding:
- Create visual representations: Draw diagrams of how data structures work or how algorithms process information.
- Build concept maps: Connect related programming concepts to see how they interact.
- Identify core principles: For each solution you study, identify the fundamental principles it employs (e.g., divide and conquer, dynamic programming, greedy approach).
4. Analyze Multiple Solutions
For any problem, study multiple approaches:
- Compare solutions: Analyze the tradeoffs between different approaches to the same problem.
- Identify patterns across solutions: Look for common techniques used across different problems.
- Study solution evolution: Start with a naive solution and incrementally improve it, understanding why each improvement helps.
5. Implement Interleaved Practice
Instead of practicing similar problems in blocks (blocked practice), mix different types of problems (interleaved practice):
- Create mixed problem sets: Practice different problem types in the same session.
- Revisit older problems: Return to previously solved problems after working on different concepts.
- Don’t categorize problems: When possible, practice problems without knowing which technique they require.
Research shows interleaved practice improves long-term retention and transfer ability, even though it feels more difficult in the moment.
Real-World Example: Adapting Binary Search
Let’s walk through a concrete example of how to practice adaptation using binary search.
The Standard Implementation
Most programmers learn binary search as a method to find a target value in a sorted array:
def binary_search(arr, target):
left, right = 0, len(arr) - 1
while left <= right:
mid = (left + right) // 2
if arr[mid] == target:
return mid
elif arr[mid] < target:
left = mid + 1
else:
right = mid - 1
return -1
Adaptation Process
To improve your ability to adapt this algorithm, try these modifications:
- Find first occurrence: Modify the algorithm to find the first occurrence of the target in a sorted array with duplicates.
- Search in rotated array: Adapt the algorithm to find a target in a sorted array that has been rotated.
- Find closest element: Modify the algorithm to find the element closest to a target value, not necessarily equal to it.
- Apply to different context: Use binary search to find the square root of a number to a certain precision.
First Occurrence Implementation
Here’s how you might adapt binary search to find the first occurrence:
def first_occurrence(arr, target):
left, right = 0, len(arr) - 1
result = -1
while left <= right:
mid = (left + right) // 2
if arr[mid] == target:
result = mid # Save this as a potential result
right = mid - 1 # But continue searching to the left
elif arr[mid] < target:
left = mid + 1
else:
right = mid - 1
return result
By working through these adaptations, you’ll develop a deeper understanding of the binary search algorithm and strengthen your ability to apply it in novel contexts.
The Importance of Productive Struggle
Research in learning science highlights the value of “productive struggle”—the process of grappling with concepts just beyond your current understanding. This struggle, while uncomfortable, is essential for deep learning.
Embracing Difficulty
When you struggle to adapt a solution, you’re engaging in productive learning. This difficulty is not a sign of failure but an indication that you’re pushing your boundaries.
Studies show that information learned with effort is better retained than information acquired easily. This phenomenon, known as the “desirable difficulty effect,” explains why passive reading feels easier but yields poorer results than active problem-solving.
Building Resilience
Regular exposure to the discomfort of not immediately knowing how to solve a problem builds intellectual resilience. This quality is essential for professional programmers, who regularly encounter novel problems without clear solutions.
Setting Appropriate Challenges
Productive struggle requires problems at the right level of difficulty—challenging enough to require effort but not so difficult that they lead to frustration and abandonment.
A good rule of thumb is the “85% rule”: aim for tasks where you can achieve about 85% success with effort. This balance maximizes learning while maintaining motivation.
Metacognitive Approaches
Metacognition—thinking about your thinking—plays a crucial role in developing adaptation skills.
Self-Questioning Techniques
When studying example solutions, ask yourself:
- “What problem is this solution addressing?”
- “What are the key insights that make this solution work?”
- “What constraints or assumptions does this solution depend on?”
- “How would I modify this if the constraints changed?”
- “What other problems could I solve using this approach?”
Reflection Practices
After attempting to solve a problem:
- Journal your approach: Document your thought process, including dead ends.
- Compare with example solutions: Analyze the differences between your approach and the example.
- Identify knowledge gaps: Note concepts or techniques you need to study further.
Progress Monitoring
Track your development over time:
- Keep a problem-solving log: Record problems you’ve solved and techniques used.
- Periodically revisit old problems: Test your retention and see how your approaches evolve.
- Note recurring challenges: Identify patterns in the types of problems that consistently challenge you.
Building a Systematic Problem-Solving Approach
Developing a systematic approach to problem-solving can bridge the gap between understanding examples and creating solutions.
The Four-Step Framework
- Understand: Thoroughly comprehend the problem before attempting a solution.
- Identify inputs, outputs, and constraints
- Work through simple examples manually
- Look for edge cases and special conditions
- Plan: Develop a solution strategy before coding.
- Break down the problem into subproblems
- Select appropriate data structures and algorithms
- Sketch the solution approach
- Implement: Translate your plan into code.
- Write clean, well-organized code
- Focus on correctness before optimization
- Comment on complex sections
- Review: Evaluate and refine your solution.
- Test with various inputs, including edge cases
- Analyze time and space complexity
- Consider alternative approaches
Applying this framework consistently helps develop the mental habits necessary for independent problem-solving.
Template Method
For common algorithm types, develop templates that you can adapt to specific problems:
- Recursive backtracking template
- Dynamic programming template
- Graph traversal template
- Sliding window template
For example, a basic backtracking template might look like:
def backtrack(current_state, choices, result):
# Base case: if we've reached a solution
if is_solution(current_state):
result.append(current_state.copy())
return
# Try each available choice
for choice in choices:
if is_valid(current_state, choice):
# Make the choice
apply_choice(current_state, choice)
# Recurse with the new state
backtrack(current_state, choices, result)
# Undo the choice (backtrack)
undo_choice(current_state, choice)
By understanding this template deeply, you can adapt it to solve a wide range of problems, from generating permutations to solving Sudoku puzzles.
The Role of Deliberate Practice
Not all practice is equally effective. Deliberate practice—focused, structured practice with feedback—is key to developing adaptation skills.
Components of Deliberate Practice
- Clear objectives: Define specific skills you want to improve.
- Focused attention: Eliminate distractions and concentrate fully on the task.
- Immediate feedback: Obtain rapid feedback on your solutions.
- Reflection: Analyze what worked, what didn’t, and why.
- Repetition with refinement: Practice similar problems with incremental challenges.
Creating a Deliberate Practice Routine
Establish a consistent practice schedule:
- Daily small problems: Solve one or two small problems daily.
- Weekly medium problems: Tackle more complex problems weekly.
- Monthly project challenges: Build small projects that integrate multiple concepts.
Leveraging Spaced Repetition
Spaced repetition—reviewing material at increasing intervals—enhances long-term retention:
- Review concepts 1 day after learning
- Review again 3 days later
- Then 7 days, 14 days, etc.
Several applications and systems can help implement spaced repetition for programming concepts.
Social Learning Strategies
Learning with others can significantly enhance your ability to adapt solutions.
Pair Programming
Pair programming—where two programmers work together on one computer—provides immediate feedback and exposes you to different problem-solving approaches.
Studies show that while pair programming might initially seem less efficient, it often produces higher-quality code and enhances learning, particularly for complex problems.
Code Reviews
Participating in code reviews, both as a reviewer and reviewee, develops critical evaluation skills:
- As a reviewer: You practice identifying alternative approaches and evaluating solution quality.
- As a reviewee: You receive feedback on your solutions and exposure to different perspectives.
Study Groups
Regular participation in programming study groups provides:
- Accountability: External motivation to practice consistently
- Diverse perspectives: Exposure to different solution approaches
- Teaching opportunities: Chances to solidify your understanding by explaining to others
Real-World Application Through Projects
Projects bridge the gap between isolated exercises and real-world programming.
Project-Based Learning Benefits
- Context integration: Projects require combining multiple concepts in meaningful ways.
- Authentic constraints: Real projects introduce practical constraints absent in isolated exercises.
- Intrinsic motivation: Building something useful provides stronger motivation than solving abstract problems.
Progressive Project Approach
Start with guided projects where you follow tutorials but make deliberate modifications:
- Follow the tutorial exactly
- Follow again with small modifications
- Extend the project with new features
- Build a similar project from scratch
This progression gradually develops your adaptation skills while providing necessary scaffolding.
Project Ideas for Different Levels
Beginner:
- Todo list application with filtering and sorting features
- Weather app that displays forecasts for multiple locations
- Simple calculator with history functionality
Intermediate:
- Social media dashboard that integrates multiple APIs
- E-commerce product page with filtering and cart functionality
- Data visualization tool for CSV files
Advanced:
- Collaborative document editor with real-time updates
- Personal finance tracker with budget analysis
- Algorithm visualizer for common algorithms
Leveraging Modern Learning Tools
Modern tools can accelerate the development of adaptation skills.
Interactive Coding Platforms
Platforms like AlgoCademy, LeetCode, and HackerRank provide:
- Structured problem sets: Organized by difficulty and topic
- Immediate feedback: Automated testing of your solutions
- Community solutions: Access to multiple approaches after solving
AI-Assisted Learning
AI tools can support the adaptation process:
- Code explanation: Tools that explain complex code in simple terms
- Solution comparison: AI that highlights differences between approaches
- Guided problem-solving: Systems that provide progressive hints rather than complete solutions
Version Control as a Learning Tool
Git and other version control systems can enhance learning:
- Solution evolution tracking: Commit different solution approaches to track your thinking
- Branching for alternatives: Create branches for different solution strategies
- Historical review: Review how your solutions have evolved over time
Conclusion: From Understanding to Mastery
The gap between understanding example solutions and creating your own is a natural part of the learning process. By recognizing this challenge and implementing the strategies outlined in this article, you can systematically develop your ability to adapt and create solutions independently.
Remember that this transition from passive comprehension to active creation is not just about accumulating knowledge—it’s about developing the mental models, problem-solving frameworks, and practical experience that allow you to apply that knowledge flexibly in new contexts.
The journey from understanding to mastery is challenging but achievable with deliberate practice, metacognitive awareness, and consistent effort. Each time you struggle to adapt a solution, you’re not failing—you’re developing the neural pathways that will eventually make such adaptation second nature.
Embrace the productive struggle, practice systematically, and trust the process. The ability to not just understand but to adapt and create solutions independently is what transforms a coding student into a true programmer.