Why You Understand Code But Can’t Write It From Scratch
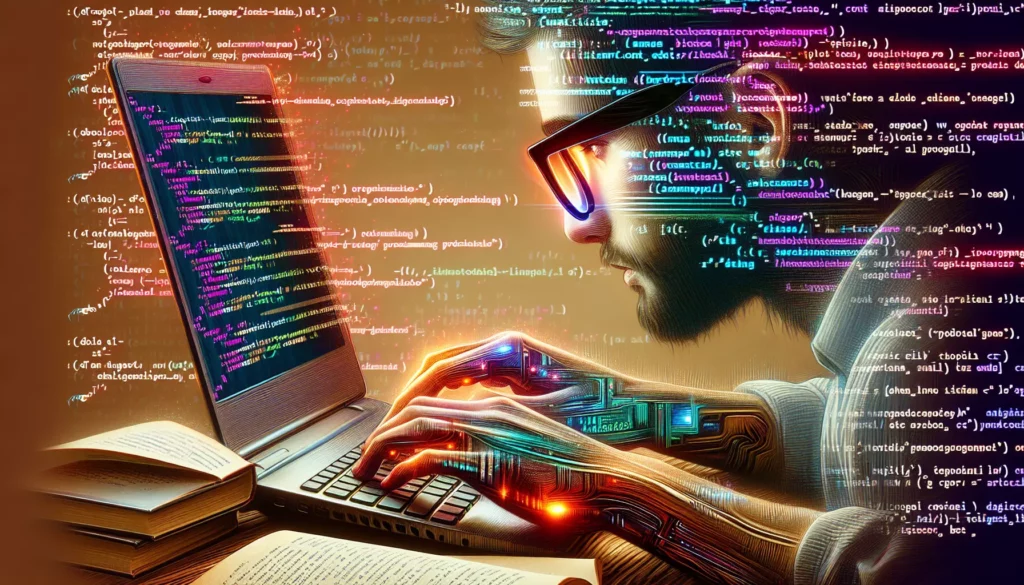
If you’ve ever found yourself nodding along while reading code, thinking “yes, I understand what’s happening here,” but then freeze up when faced with a blank editor and a new programming task, you’re not alone. This phenomenon is incredibly common, even among experienced developers. The gap between code comprehension and code creation is real, and it affects programmers at all levels.
In this comprehensive guide, we’ll explore why understanding code is often easier than writing it from scratch, the cognitive processes behind this discrepancy, and practical strategies to bridge this gap in your programming journey.
The Recognition vs. Recall Phenomenon
At the heart of this issue is a fundamental cognitive distinction: recognition versus recall. This distinction helps explain why many programmers find themselves stuck when attempting to create new code despite understanding existing code perfectly well.
Recognition: The Easier Path
When you read code, you’re engaging in recognition memory. Your brain is processing information that’s presented to you, and you’re determining whether it makes sense based on your existing knowledge. This process is relatively passive and requires less cognitive effort.
Recognition is similar to how you might recognize a face in a crowd without necessarily being able to describe that face in detail to someone else. In programming terms, it’s like looking at a sorting algorithm and thinking, “Yes, that’s a bubble sort,” without necessarily being able to implement it yourself.
Recall: The Challenging Task
Writing code from scratch, however, demands recall memory. You must actively retrieve information from your memory without external cues. This process requires you to:
- Retrieve syntax rules correctly
- Remember appropriate methods and functions
- Structure logic coherently
- Anticipate edge cases
- Organize your thoughts into executable code
Recall is inherently more difficult than recognition. It’s the difference between being able to select the correct answer from multiple choices versus having to produce the answer without any options provided.
The Knowledge Illusion in Programming
Another factor contributing to this discrepancy is what psychologists call the “knowledge illusion” or the “illusion of explanatory depth.” This refers to our tendency to believe we understand something more thoroughly than we actually do.
Passive vs. Active Understanding
When reading code, we often experience a sense of fluency or familiarity that can be misleading. We might think, “This makes perfect sense,” but this understanding is often passive. We’re following a path that someone else has created.
Writing code, on the other hand, requires active understanding. You need to forge your own path, make countless decisions, and solve problems without a predefined roadmap. This active process reveals gaps in knowledge that weren’t apparent during passive reading.
The Complexity of Generative Tasks
Creating something from nothing is fundamentally more complex than evaluating something that already exists. When writing code from scratch, you must:
- Define the problem clearly
- Break it down into manageable components
- Choose appropriate data structures and algorithms
- Implement a solution using correct syntax
- Test and debug your creation
This generative process requires a deeper level of understanding than simply reading and comprehending existing code.
Working Memory Limitations
Human working memory is remarkably limited. Research suggests that we can typically hold only about 4-7 items in our working memory at once. This limitation significantly impacts our ability to write code.
Cognitive Load in Programming
When writing code from scratch, you’re juggling multiple concerns simultaneously:
- The overall structure of your program
- The specific algorithm or function you’re implementing
- Syntax requirements of your programming language
- Variable names and their purposes
- Potential edge cases
- How this code will interact with other parts of your program
This high cognitive load can quickly overwhelm your working memory, leading to that frustrating feeling of “I know I know this, but I can’t seem to put it together.”
Reading vs. Writing: Cognitive Demands
When reading code, many of these concerns are already addressed for you. The structure exists, the variables are named, and the syntax is (presumably) correct. You can focus on understanding the logic without having to create it yourself.
This difference in cognitive demands explains why you might understand a complex algorithm when reading it, but struggle to implement even a simpler version when starting from scratch.
The Role of Pattern Recognition
Experienced programmers develop a mental library of patterns and solutions over time. This pattern recognition is a crucial aspect of programming expertise.
Pattern Matching When Reading
When reading code, you’re often recognizing patterns you’ve seen before. You might think, “This looks like a standard authentication system” or “This is implementing the Observer pattern.” This recognition happens quickly and often subconsciously.
Your brain is efficiently matching what you’re seeing against templates or patterns you’ve encountered previously, making comprehension relatively effortless.
Pattern Generation When Writing
Writing code requires you to generate these patterns from memory. You need to:
- Recall the appropriate pattern for your current problem
- Adapt it to your specific context
- Implement it correctly in your chosen programming language
This generative process demands a deeper level of familiarity with patterns than the recognition process does. It’s the difference between recognizing a song when you hear it versus being able to play that song on an instrument.
The Context Problem
Code never exists in isolation. When you’re reading existing code, you benefit from the context it provides. When writing from scratch, you must create this context yourself.
Contextual Clues in Existing Code
Existing code provides numerous contextual clues that aid understanding:
- File organization and structure
- Variable and function names
- Comments and documentation
- Import statements showing dependencies
- Test cases demonstrating expected behavior
These contextual elements scaffold your understanding, making it easier to follow the logic and purpose of the code.
Creating Context from Nothing
When writing code from scratch, you must establish this context yourself. You need to make decisions about:
- How to structure your solution
- What to name your variables and functions
- Which dependencies to include
- How to document your code
- How to test your implementation
This additional layer of decision-making adds to the complexity of writing code compared to reading it.
Practical Strategies to Bridge the Gap
Now that we understand why writing code is more challenging than reading it, let’s explore practical strategies to strengthen your code creation skills.
Deliberate Practice: Beyond Passive Learning
The most effective way to improve your ability to write code from scratch is through deliberate practice.
Code Implementation Exercises
Challenge yourself to implement solutions to problems without looking at examples first. Start with simple problems and gradually increase complexity. Resources like:
- LeetCode, HackerRank, and CodeWars for algorithm practice
- Project Euler for mathematically oriented programming challenges
- Exercism.io for language-specific exercises with mentorship
These platforms provide structured problems that force you to write code from scratch.
Reimplement from Memory
After studying a piece of code or a solution to a problem, close the reference and try to reimplement it from memory. This practice strengthens your recall abilities and helps build your mental model of how the code works.
For example, if you’ve just learned about implementing a binary search tree, study an implementation, then close it and try to code it yourself without referring back to the original. Compare your solution afterward to identify gaps in your understanding.
Scaffolding Your Learning
Use scaffolding techniques to bridge the gap between understanding and creation.
Start with Templates
Begin with code templates or skeletons that provide structure, then fill in the details. Over time, reduce your reliance on templates as you become more comfortable creating from scratch.
For example, if you’re learning web development with React, you might start with a component template:
import React from 'react';
function MyComponent(props) {
// Your logic here
return (
<div>
{/* Your JSX here */}
</div>
);
}
export default MyComponent;
Then gradually move to creating components without referring to templates.
Incremental Complexity
Build solutions incrementally, starting with the simplest version that works, then adding complexity. This approach reduces cognitive load by allowing you to focus on one aspect at a time.
For instance, when implementing a sorting algorithm, you might:
- Start with a basic implementation that handles the main case
- Add error checking for edge cases
- Optimize for performance
- Enhance readability and documentation
Building Your Mental Models
Strong mental models of programming concepts make it easier to write code from scratch.
Visualization Techniques
Practice visualizing how code executes. Draw diagrams, use visualization tools, or manually trace through code execution to strengthen your mental models.
For example, when learning recursion, trace through recursive functions step by step, drawing each stack frame and tracking variable values. This visualization helps solidify your understanding of how recursion works.
Conceptual Mapping
Create concept maps or cheat sheets that connect related programming concepts. This helps you see the bigger picture and recall solutions more effectively when writing code.
For instance, you might create a concept map for JavaScript array methods, showing how methods like map
, filter
, reduce
, and forEach
relate to each other and when to use each one.
Chunking and Pattern Building
Develop your ability to recognize and use common programming patterns.
Pattern Catalogs
Study and practice implementing common design patterns and algorithmic patterns. Create your own “pattern catalog” with examples of patterns you’ve implemented.
For example, familiarize yourself with common patterns like:
- Creational patterns (Factory, Singleton, Builder)
- Structural patterns (Adapter, Decorator, Composite)
- Behavioral patterns (Observer, Strategy, Command)
- Algorithmic patterns (Divide and Conquer, Dynamic Programming, Greedy Algorithms)
Code Snippets Library
Maintain a personal library of code snippets for common tasks. Review these regularly to reinforce your memory of how to implement them.
Your library might include snippets for:
- File operations
- API requests
- Authentication flows
- Data validation routines
- Common UI components
Metacognitive Strategies
Develop awareness of your own thinking processes when programming.
Verbalization
Practice explaining your code and thought process out loud, even when coding alone. This “rubber duck debugging” approach forces you to articulate your thinking, which strengthens your understanding and reveals gaps in your knowledge.
For example, as you write a function, verbalize:
- “I’m creating this function to parse JSON data from an API response.”
- “I need to check if the response is valid before parsing.”
- “If there’s an error, I’ll return a default object.”
Reflective Practice
After completing a programming task, reflect on your process. What was difficult? What came easily? What would you do differently next time? This reflection helps you identify patterns in your thinking and areas for improvement.
Keep a programming journal where you document:
- Challenges you faced
- How you overcame them
- New concepts or techniques you learned
- Questions for further exploration
The Experience Factor: Time and Exposure
It’s important to acknowledge that bridging the gap between understanding and writing code takes time and consistent exposure to different codebases and problems.
The 10,000-Hour Perspective
While the 10,000-hour rule of expertise has been debated, there’s truth to the idea that mastery requires significant practice. Writing code from scratch gets easier with experience as you:
- Build a larger mental library of patterns and solutions
- Develop stronger neural pathways for recalling programming concepts
- Automate lower-level thinking, freeing up working memory for higher-level problems
Be patient with yourself and recognize that this gap is normal, especially for newer programmers.
Diverse Exposure
Expose yourself to diverse programming challenges and codebases. Read and study code written by experienced developers in different domains. This diversity builds a richer mental model of programming patterns and solutions.
Some ways to gain diverse exposure:
- Contribute to open-source projects
- Review pull requests from colleagues
- Study implementations of popular libraries
- Tackle problems in different domains (web, data science, systems programming)
Psychological Barriers and How to Overcome Them
Sometimes the gap between understanding and writing code is widened by psychological factors.
Perfectionism and the Blank Editor
The blank editor syndrome, similar to writer’s block, can paralyze programmers. The fear of writing imperfect code prevents many developers from starting at all.
Strategies to Overcome Perfectionism:
- Embrace the “ugly first draft” approach: Give yourself permission to write messy, imperfect code that you can refine later.
- Time-box your work: Set a timer for 25 minutes and focus on making progress, not perfection.
- Use pseudocode: Start by writing plain-language steps before converting to actual code.
Impostor Syndrome
Many programmers struggle with impostor syndrome—the feeling that they’re not “real programmers” because they can’t write perfect code from scratch.
Combating Impostor Syndrome:
- Normalize the struggle: Recognize that even senior developers Google syntax and reference documentation regularly.
- Document your growth: Keep a record of problems you’ve solved to remind yourself of your progress.
- Join communities: Connect with other programmers to share experiences and realize your challenges are common.
Tools and Techniques for Bridging the Gap
Modern development environments provide tools that can help bridge the gap between understanding and writing code.
Leveraging IDE Features
Integrated Development Environments (IDEs) offer features that reduce the cognitive load of writing code from scratch:
- Code completion: Suggests methods, properties, and variables as you type
- Snippets: Pre-written code templates you can insert with a shortcut
- Refactoring tools: Help restructure code without changing its behavior
- Linters and formatters: Automatically catch errors and enforce style conventions
Learning to use these tools effectively can significantly reduce the friction of writing code from scratch.
Documentation and Reference Materials
Develop efficient strategies for using documentation and references:
- Create cheat sheets for languages and libraries you use frequently
- Bookmark official documentation for quick access
- Use interactive documentation like Jupyter notebooks for experimenting with code snippets
- Reference implementation examples from trusted sources
Remember that using references is not cheating—it’s part of professional programming practice.
From Understanding to Creating: A Progressive Approach
Let’s look at a concrete example of how to progress from understanding to creating code using a progressive approach.
Example: Implementing a Binary Search Algorithm
Stage 1: Study and Understand
Begin by studying an implementation of binary search:
function binarySearch(arr, target) {
let left = 0;
let right = arr.length - 1;
while (left <= right) {
const mid = Math.floor((left + right) / 2);
if (arr[mid] === target) {
return mid;
} else if (arr[mid] < target) {
left = mid + 1;
} else {
right = mid - 1;
}
}
return -1;
}
Make sure you understand:
- Why we track left and right boundaries
- How the middle point is calculated
- The logic for updating boundaries
- The termination condition
Stage 2: Modify Existing Code
Practice by modifying the existing code. For example, adapt it to find the index of the first occurrence of a value in an array that might contain duplicates:
function findFirstOccurrence(arr, target) {
let left = 0;
let right = arr.length - 1;
let result = -1;
while (left <= right) {
const mid = Math.floor((left + right) / 2);
if (arr[mid] === target) {
result = mid;
right = mid - 1; // Continue searching to the left
} else if (arr[mid] < target) {
left = mid + 1;
} else {
right = mid - 1;
}
}
return result;
}
Stage 3: Implement from Memory
Close all references and implement binary search from memory. Don’t worry about getting it perfect—focus on the core logic:
function myBinarySearch(array, target) {
let start = 0;
let end = array.length - 1;
while (start <= end) {
let middle = Math.floor((start + end) / 2);
if (array[middle] === target) {
return middle;
}
if (array[middle] < target) {
start = middle + 1;
} else {
end = middle - 1;
}
}
return -1;
}
Stage 4: Create a Variant from Scratch
Now create a variant without reference, such as a binary search that works on a rotated sorted array:
function searchRotatedArray(nums, target) {
let left = 0;
let right = nums.length - 1;
while (left <= right) {
const mid = Math.floor((left + right) / 2);
if (nums[mid] === target) {
return mid;
}
// Check which half is sorted
if (nums[left] <= nums[mid]) {
// Left half is sorted
if (nums[left] <= target && target < nums[mid]) {
// Target is in the left sorted half
right = mid - 1;
} else {
// Target is in the right half
left = mid + 1;
}
} else {
// Right half is sorted
if (nums[mid] < target && target <= nums[right]) {
// Target is in the right sorted half
left = mid + 1;
} else {
// Target is in the left half
right = mid - 1;
}
}
}
return -1;
}
This progressive approach builds your confidence and ability to create increasingly complex implementations from scratch.
The Reality of Professional Programming
It’s important to understand that even professional programmers don’t typically write complex code from scratch without references or assistance.
The Myth of the Solo Genius Programmer
The image of the programmer who codes complex systems entirely from memory is largely a myth. In reality, professional development involves:
- Referencing documentation and API guides
- Looking up syntax and examples
- Searching for solutions to specific problems
- Reusing and adapting existing code
- Collaborating with others
The goal isn’t to memorize everything but to develop the ability to solve problems effectively using available resources.
The Value of Understanding vs. Memorization
What truly matters in programming is not memorization but understanding. When you deeply understand a concept, you can:
- Recognize when and how to apply it
- Adapt it to new situations
- Debug issues when they arise
- Explain it to others
Focus on building this deep understanding rather than perfect recall. The ability to write code from scratch will improve naturally as your understanding deepens.
Conclusion: Embracing the Journey from Understanding to Creation
The gap between understanding code and writing it from scratch is a natural part of the programming journey. It reflects fundamental cognitive processes like recognition versus recall, working memory limitations, and the complexity of generative tasks.
Rather than being discouraged by this gap, embrace it as an opportunity for growth. Use the strategies outlined in this article to progressively build your code creation skills:
- Engage in deliberate practice
- Use scaffolding techniques
- Build strong mental models
- Develop pattern recognition
- Apply metacognitive strategies
Remember that even experienced programmers face this challenge. The difference is that they’ve developed strategies to bridge the gap and have accumulated a larger library of patterns and solutions through years of practice.
With consistent effort and the right approach, you’ll gradually narrow the gap between understanding and creating code. You’ll find yourself more confidently facing that blank editor, armed with the skills and strategies to transform your understanding into working code.
The journey from code reader to code creator is challenging but immensely rewarding. Each step you take builds not just your programming skills but your problem-solving abilities more broadly. Embrace the process, celebrate your progress, and keep coding!