Why You Struggle to Think Abstractly About Programming Problems
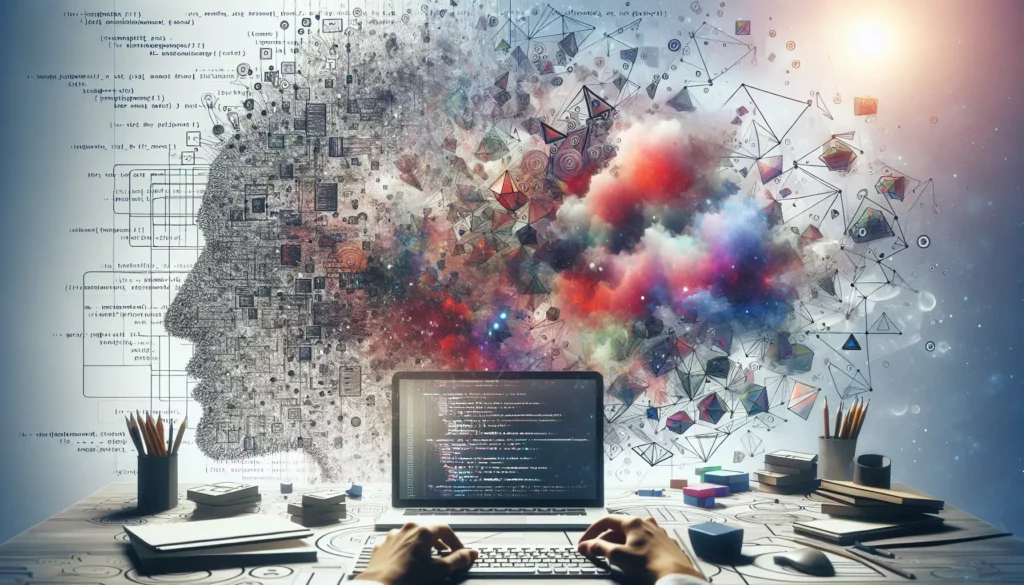
Have you ever sat down to solve a coding problem and felt completely stuck? You read the problem statement multiple times, but translating it into code seems impossible. If this sounds familiar, you might be struggling with abstract thinking — a critical skill for programming success.
Abstract thinking in programming is the ability to identify patterns, recognize underlying principles, and conceptualize solutions without getting lost in implementation details. It’s what separates programmers who can solve novel problems from those who can only follow tutorials.
In this comprehensive guide, we’ll explore why abstract thinking is challenging, how it impacts your programming journey, and practical strategies to improve this essential skill.
Understanding Abstract Thinking in Programming
Abstract thinking is the mental process of considering concepts, principles, and relationships rather than focusing solely on concrete details. In programming, it manifests in several ways:
- Recognizing that different problems share the same underlying structure
- Identifying appropriate data structures and algorithms for a given problem
- Breaking complex problems into manageable components
- Creating mental models that represent system behavior
- Thinking in terms of patterns rather than specific implementations
Consider the difference between these two approaches to a problem:
Concrete Thinking Example:
“I need to write code that adds all the numbers in this array. I’ll create a variable called ‘sum’ and set it to zero. Then I’ll loop through each number in the array and add it to ‘sum’. Finally, I’ll return ‘sum’.”
Abstract Thinking Example:
“This problem requires an accumulation pattern. I need to apply a reduction operation across a collection of values to produce a single result.”
The abstract thinker recognizes the pattern and can apply it to many similar problems, while the concrete thinker focuses on the specific implementation steps for this particular case.
Common Barriers to Abstract Thinking
Before we can improve abstract thinking, we need to understand what holds us back. Here are the most common barriers programmers face:
1. Lack of Foundational Knowledge
Without a solid understanding of programming fundamentals, it’s difficult to recognize patterns and abstractions. If you’re still struggling with basic syntax or concepts, your cognitive resources are consumed by these details rather than higher-level thinking.
For example, if you don’t fully understand how arrays work, you’ll have trouble seeing how array manipulation problems relate to each other.
2. Limited Exposure to Problem Patterns
Many programming problems follow established patterns. If you haven’t encountered these patterns before, you might approach each problem as entirely new rather than recognizing familiar structures.
Consider these common algorithmic patterns:
- Two-pointer technique
- Sliding window
- Breadth-first search
- Depth-first search
- Dynamic programming
- Divide and conquer
Experienced programmers immediately recognize when a problem fits one of these patterns. Beginners often miss these connections.
3. Fixation on Implementation Details
Getting caught up in “how” before understanding “what” and “why” limits abstract thinking. When you immediately jump to coding without fully grasping the problem, you miss opportunities to see the bigger picture.
This is common when tackling complex problems. Instead of stepping back to understand the overall structure, beginners often dive into coding specific parts without a cohesive strategy.
4. Cognitive Load and Mental Fatigue
Abstract thinking requires significant mental energy. When your brain is tired or overloaded, it naturally reverts to more concrete thinking patterns.
This explains why you might struggle with abstract thinking during lengthy coding sessions or when working on particularly challenging problems.
5. Educational Background
Traditional education often emphasizes memorization and following procedures rather than abstract reasoning. If your educational experience didn’t nurture abstract thinking, you may find it challenging in programming contexts.
Computer science education that focuses on implementation without teaching underlying principles can contribute to this issue.
The Impact of Abstract Thinking Challenges
Struggling with abstract thinking affects your programming journey in several significant ways:
1. Difficulty Solving New Problems
When you can’t think abstractly, each new problem feels overwhelming. You lack the mental frameworks to connect it to problems you’ve solved before, making your learning curve steeper than necessary.
This is particularly evident in technical interviews, where abstract thinking is essential for solving unfamiliar problems under time pressure.
2. Inefficient Learning
Without abstract thinking, you might learn specific solutions without understanding the underlying principles. This leads to a collection of disconnected techniques rather than a coherent body of knowledge.
For example, you might memorize how to implement a specific sorting algorithm without understanding the general concept of sorting algorithms and when to apply different approaches.
3. Code Quality Issues
Abstract thinking is crucial for writing clean, maintainable code. Without it, you’re more likely to create solutions that work for specific cases but lack flexibility and scalability.
This manifests in code duplication, overly complex functions, and designs that don’t accommodate changing requirements.
4. Limited Career Progression
As you advance in your programming career, abstract thinking becomes increasingly important. Senior roles require the ability to design systems, architect solutions, and make high-level decisions.
Engineers who struggle with abstract thinking often hit a ceiling in their career progression, finding it difficult to move beyond implementation-focused roles.
Practical Strategies to Improve Abstract Thinking
Now for the good news: abstract thinking is a skill that can be developed with practice. Here are effective strategies to enhance your abstract thinking abilities:
1. Study Algorithmic Patterns
Familiarize yourself with common algorithmic patterns and practice recognizing them in different problems. Resources like “Grokking the Coding Interview” organize problems by pattern, helping you build this recognition skill.
For example, study how the two-pointer technique applies to problems like:
- Finding pairs in a sorted array that sum to a target
- Detecting palindromes
- Merging sorted arrays
- Removing duplicates from sorted arrays
By seeing how the same pattern applies across different contexts, you’ll develop the ability to abstract away implementation details and focus on the underlying approach.
2. Practice Problem Decomposition
Regularly break down complex problems into smaller, more manageable components. This process forces you to think about the problem structure rather than immediately jumping to code.
Consider this approach:
- Identify the inputs and expected outputs
- Break the problem into logical steps or subproblems
- Determine the relationships between these components
- Solve each component independently
- Combine the solutions
This systematic decomposition builds your ability to see structure in complexity.
3. Implement Abstract Data Types
Build your own implementations of common abstract data types like stacks, queues, linked lists, and trees. This exercise helps you separate the conceptual properties of these structures from their specific implementations.
For instance, when implementing a stack, focus on understanding the LIFO (Last In, First Out) principle rather than just the mechanics of the code.
class Stack:
def __init__(self):
self.items = []
def push(self, item):
self.items.append(item)
def pop(self):
if not self.is_empty():
return self.items.pop()
def is_empty(self):
return len(self.items) == 0
def peek(self):
if not self.is_empty():
return self.items[-1]
Understanding that a stack is fundamentally about tracking the most recently added item, regardless of how it’s implemented, is an exercise in abstract thinking.
4. Use Visual Representations
Visualizing problems can bridge the gap between concrete and abstract thinking. Draw diagrams, use flowcharts, or create visual models to represent problem structures.
Tools like:
- Pen and paper for quick sketches
- Whiteboards for larger problems
- Visualization websites like VisuAlgo
- Flowchart software
These visual aids help you see patterns and relationships that might not be obvious when looking at code or problem descriptions.
5. Practice Algorithm Design Before Coding
Before writing any code, practice describing your algorithm in plain language or pseudocode. This forces you to think about the solution approach rather than implementation details.
For example, when solving a binary search problem:
// Abstract algorithm design
1. Set left pointer to start of array
2. Set right pointer to end of array
3. While left pointer is less than or equal to right pointer:
a. Calculate middle index
b. If target equals middle element, return middle index
c. If target greater than middle element, move left pointer to middle + 1
d. If target less than middle element, move right pointer to middle - 1
4. If we exit the loop, target was not found, return -1
This approach emphasizes the logical structure of the solution before getting caught up in language-specific syntax.
6. Study Design Patterns
Familiarize yourself with software design patterns, which are abstract solutions to common design problems. Understanding patterns like Factory, Observer, or Strategy helps you think about code organization at a higher level.
For example, the Strategy pattern allows you to define a family of algorithms, encapsulate each one, and make them interchangeable:
// Strategy interface
interface SortingStrategy {
void sort(int[] array);
}
// Concrete strategies
class QuickSort implements SortingStrategy {
public void sort(int[] array) {
// QuickSort implementation
}
}
class MergeSort implements SortingStrategy {
public void sort(int[] array) {
// MergeSort implementation
}
}
// Context
class Sorter {
private SortingStrategy strategy;
public void setStrategy(SortingStrategy strategy) {
this.strategy = strategy;
}
public void performSort(int[] array) {
strategy.sort(array);
}
}
This pattern helps you think abstractly about behavior encapsulation and interchangeability rather than specific sorting implementations.
7. Teach Concepts to Others
Explaining programming concepts to others forces you to articulate abstract ideas clearly. Whether through formal teaching, blog posts, or simply helping a colleague, teaching deepens your own understanding.
The process of simplifying complex ideas for others requires you to identify the essential principles and patterns, which strengthens your abstract thinking.
8. Review and Refactor Your Code
Regularly revisit your code with the specific goal of identifying patterns and abstractions. Ask yourself:
- Where am I repeating similar logic?
- Can I create a more general function to handle these cases?
- What’s the underlying principle behind this implementation?
- How might this solution apply to other problems?
This reflective practice builds your ability to see beyond specific implementations to the abstract patterns they represent.
Real-World Examples: Concrete vs. Abstract Thinking
Let’s examine some examples that illustrate the difference between concrete and abstract thinking in programming:
Example 1: Finding the Maximum Value
Concrete Approach:
function findMax(numbers) {
let max = numbers[0];
for (let i = 1; i < numbers.length; i++) {
if (numbers[i] > max) {
max = numbers[i];
}
}
return max;
}
Abstract Approach:
Recognizing this as a “reduction” pattern where we process a collection to produce a single value by repeatedly applying an operation (in this case, comparing and taking the maximum).
function findMax(numbers) {
return numbers.reduce((max, current) => Math.max(max, current), numbers[0]);
}
The abstract thinker sees beyond the specific implementation to the general pattern of reduction, which can be applied to many similar problems (finding minimum, sum, product, etc.).
Example 2: Checking for Palindromes
Concrete Approach:
function isPalindrome(str) {
str = str.toLowerCase().replace(/[^a-z0-9]/g, '');
let reversed = '';
for (let i = str.length - 1; i >= 0; i--) {
reversed += str[i];
}
return str === reversed;
}
Abstract Approach:
Recognizing this as a problem about symmetry and using the two-pointer technique to check corresponding positions from both ends.
function isPalindrome(str) {
str = str.toLowerCase().replace(/[^a-z0-9]/g, '');
let left = 0;
let right = str.length - 1;
while (left < right) {
if (str[left] !== str[right]) {
return false;
}
left++;
right--;
}
return true;
}
The abstract thinker recognizes the symmetry property of palindromes and applies a general technique for checking symmetry rather than focusing on string reversal.
Example 3: Finding Duplicates
Concrete Approach:
function findDuplicates(array) {
const duplicates = [];
for (let i = 0; i < array.length; i++) {
for (let j = i + 1; j < array.length; j++) {
if (array[i] === array[j] && !duplicates.includes(array[i])) {
duplicates.push(array[i]);
break;
}
}
}
return duplicates;
}
Abstract Approach:
Recognizing this as a frequency counting problem and using appropriate data structures.
function findDuplicates(array) {
const seen = new Set();
const duplicates = new Set();
for (const item of array) {
if (seen.has(item)) {
duplicates.add(item);
} else {
seen.add(item);
}
}
return Array.from(duplicates);
}
The abstract thinker identifies the core problem as tracking frequencies and selects an appropriate data structure (Set) to simplify the solution.
Abstract Thinking in System Design
As you progress in your programming career, abstract thinking becomes crucial for system design. Here’s how it applies at this level:
1. Architectural Patterns
Abstract thinkers recognize common architectural patterns like microservices, event-driven architecture, or layered architecture. They understand the principles behind these patterns and can apply them appropriately.
For example, recognizing when a system would benefit from an event-driven architecture:
// Event system abstraction
class EventBus {
private listeners = new Map<string, Function[]>();
subscribe(event: string, callback: Function) {
if (!this.listeners.has(event)) {
this.listeners.set(event, []);
}
this.listeners.get(event).push(callback);
}
publish(event: string, data: any) {
if (this.listeners.has(event)) {
for (const callback of this.listeners.get(event)) {
callback(data);
}
}
}
}
This abstraction allows components to communicate without direct dependencies, creating a more flexible system.
2. Separation of Concerns
Abstract thinking helps identify distinct responsibilities within a system and separate them appropriately. This leads to modular designs where components have clear boundaries and interfaces.
Consider how a web application might be divided:
- Presentation layer (UI components)
- Application layer (business logic)
- Data access layer (database interactions)
- Infrastructure layer (logging, authentication, etc.)
Abstract thinkers naturally identify these separations rather than creating monolithic systems.
3. API Design
Designing effective APIs requires abstract thinking about how different components will interact. It involves creating interfaces that expose the essential functionality while hiding implementation details.
For example, designing a clean API for a user service:
interface UserService {
findById(id: string): Promise<User>;
findByEmail(email: string): Promise<User>;
create(userData: UserCreateDTO): Promise<User>;
update(id: string, userData: UserUpdateDTO): Promise<User>;
delete(id: string): Promise<void>;
}
This interface abstracts away database interactions, validation logic, and other implementation details, providing a clean contract for other components.
The Role of AI Tools in Developing Abstract Thinking
Modern AI-powered coding tools can both help and hinder the development of abstract thinking skills. Here’s how to use them effectively:
Benefits of AI Tools
- Pattern Recognition: AI tools can identify patterns in your code and suggest abstractions you might have missed.
- Alternative Approaches: Tools like GitHub Copilot can show you different ways to solve the same problem, helping you recognize the underlying patterns.
- Code Explanations: AI can explain complex code in conceptual terms, helping bridge the gap between implementation and abstraction.
Potential Pitfalls
- Over-reliance: Using AI to generate solutions without understanding the underlying principles can hinder abstract thinking development.
- Implementation Focus: Many AI tools focus on generating implementation code rather than helping with abstract problem-solving.
- Missed Learning Opportunities: When AI solves problems for you, you might miss the valuable struggle that builds abstract thinking skills.
Effective Use of AI Tools
To use AI tools while still developing abstract thinking:
- First attempt to solve problems independently, focusing on the abstract approach
- Use AI to suggest alternative implementations after you’ve developed your own solution
- Ask AI to explain the patterns and principles behind its suggestions
- Use AI to refactor your code and identify potential abstractions
Platforms like AlgoCademy integrate AI assistance in ways that support abstract thinking development rather than replacing it.
Measuring Your Progress in Abstract Thinking
How do you know if your abstract thinking skills are improving? Look for these indicators:
1. Faster Problem Recognition
You can quickly identify the type of problem you’re facing and match it to appropriate solution patterns.
2. Cleaner Code Design
Your code naturally follows principles like DRY (Don’t Repeat Yourself) and SRP (Single Responsibility Principle) because you recognize patterns and abstractions.
3. Improved Technical Discussions
You can discuss solutions at a conceptual level without getting lost in implementation details.
4. Transfer of Knowledge
You can apply lessons from one problem domain to another, recognizing the common patterns despite different contexts.
5. Better System Design Skills
You can design software systems with appropriate abstractions, clear interfaces, and logical component boundaries.
Regularly assess these indicators to track your progress and identify areas for further improvement.
Conclusion: Embracing the Journey to Abstract Thinking
Developing abstract thinking skills is a gradual process that requires patience and deliberate practice. Don’t be discouraged if you find it challenging at first — even experienced programmers continuously work to improve these skills.
Remember that concrete thinking isn’t inherently bad. Effective programming requires both concrete implementation skills and abstract conceptual thinking. The goal is to develop the ability to move fluidly between these modes of thought as needed.
By understanding the barriers to abstract thinking and implementing the strategies outlined in this article, you’ll gradually transform how you approach programming problems. You’ll move from seeing each problem as a unique challenge to recognizing the patterns and principles that connect different problems.
This shift not only makes you a more effective programmer but also opens doors to advanced roles in software architecture and system design. Perhaps most importantly, it makes programming more enjoyable as you develop an appreciation for the elegant patterns that underlie seemingly complex problems.
Start small, be patient with yourself, and celebrate your progress along the way. The ability to think abstractly about programming problems is a skill worth developing, and the journey itself is rewarding.
Further Resources
To continue developing your abstract thinking skills, consider these resources:
- Books:
- “Design Patterns: Elements of Reusable Object-Oriented Software” by Gang of Four
- “Clean Code” by Robert C. Martin
- “Thinking in Systems” by Donella H. Meadows
- Online Courses:
- Algorithm specializations on platforms like Coursera and edX
- System design courses that focus on architectural patterns
- Practice Platforms:
- AlgoCademy’s pattern-based problem collections
- LeetCode’s explore sections organized by concept
- Communities:
- Join programming discussion forums where abstract concepts are regularly discussed
- Participate in code reviews to see how others approach problems
The path to mastering abstract thinking in programming is ongoing, but with consistent effort and the right resources, you’ll make steady progress that transforms your capabilities as a developer.