Why You Struggle to Plan Your Solution Approach and How to Overcome It
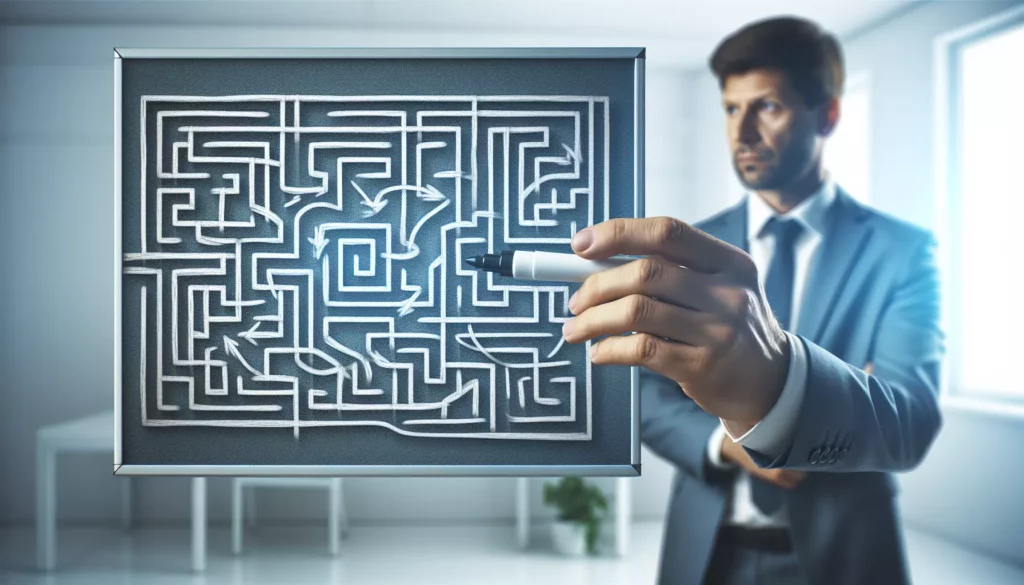
Have you ever sat in front of a coding problem, staring at the screen, unsure where to begin? You understand the problem statement, but translating that understanding into a coherent solution feels like trying to build a bridge while simultaneously designing it. This struggle to plan your solution approach is more common than you might think.
In this comprehensive guide, we’ll explore why many programmers struggle with solution planning and provide actionable strategies to overcome these challenges. Whether you’re preparing for technical interviews at major tech companies or simply trying to become a more effective programmer, mastering the art of solution planning is a critical skill.
Understanding the Solution Planning Struggle
Before we dive into solutions, let’s identify the common reasons why programmers struggle with planning their approach:
1. The Pressure to Code Immediately
In both educational settings and technical interviews, there’s often an implicit pressure to start coding right away. This pressure can lead to a “code first, think later” approach that results in disorganized solutions and wasted time.
Many programmers feel that if they’re not typing, they’re not making progress. This misconception can lead to premature coding before a clear plan is established.
2. Insufficient Problem Analysis
Rushing through the problem understanding phase is a common pitfall. When you don’t fully grasp what a problem is asking for, including edge cases and constraints, it becomes nearly impossible to formulate an effective solution plan.
3. Limited Pattern Recognition
Experienced programmers can quickly identify patterns in problems that relate to known algorithmic approaches. Beginners and intermediate programmers often lack this pattern recognition ability, making it difficult to determine which tools or techniques to apply.
4. Overcomplicating Solutions
The tendency to jump to complex solutions before considering simpler approaches can paralyze your planning process. This often stems from a desire to showcase advanced knowledge or from not fully understanding the problem’s core requirements.
5. Difficulty Breaking Down Problems
Large problems can be overwhelming when viewed as a whole. The inability to decompose problems into smaller, manageable components makes planning seem insurmountable.
The Cost of Poor Solution Planning
Failing to adequately plan your solution approach has several consequences:
- Wasted Time: Coding without a plan often leads to numerous rewrites and refactoring.
- Suboptimal Solutions: Hastily implemented code typically results in less efficient algorithms in terms of time and space complexity.
- Increased Bugs: Unplanned code tends to have more logical errors and edge case failures.
- Interview Failure: In technical interviews, poor planning signals to interviewers that you may struggle with complex problems in real work scenarios.
A Structured Approach to Solution Planning
Now that we understand the challenges, let’s explore a methodical approach to planning your solutions:
1. Master the Problem Understanding Phase
Before attempting to solve any problem, ensure you completely understand what’s being asked:
- Restate the problem in your own words to confirm understanding
- Identify inputs and outputs clearly
- Clarify constraints such as time/space complexity requirements or input limitations
- Work through examples to verify your understanding
- Consider edge cases like empty inputs, negative numbers, duplicates, etc.
For example, if given a problem about finding duplicates in an array, you might think:
Input: An array of integers
Output: All integers that appear more than once
Constraints: O(n) time complexity desired
Edge cases: Empty array, no duplicates, all duplicates
2. Visualize the Problem
Many programmers underestimate the power of visualization in solution planning. Try these approaches:
- Draw diagrams representing the problem state
- Trace through examples step by step
- Use tables or matrices to track state changes
For graph problems, actually drawing the graph can provide immense clarity. For array manipulations, tracing through the steps visually helps identify patterns.
3. Consider Multiple Approaches
Before committing to a solution, brainstorm several potential approaches:
- Brute force solution: What’s the simplest way to solve this, regardless of efficiency?
- Optimization strategies: How can I improve upon the brute force solution?
- Common patterns: Does this problem fit a known pattern (two pointers, sliding window, etc.)?
- Data structure selection: Which data structures might help solve this efficiently?
For instance, when finding duplicates in an array:
- Brute force: Check each element against all others (O(n²))
- Sorting approach: Sort first, then check adjacent elements (O(n log n))
- Hash set approach: Track seen elements in a set (O(n))
4. Break Down the Problem
Large problems become manageable when broken into smaller components:
- Identify subproblems that can be solved independently
- Determine dependencies between subproblems
- Create a step-by-step plan for solving each component
For example, if building a function to validate a binary search tree, you might break it down into:
- Define what makes a valid BST (each node’s value greater than all left children, less than all right children)
- Create a helper function to check if a subtree’s values fall within a valid range
- Recursively apply the helper function with updated ranges
5. Think Aloud
Whether in an interview or studying alone, verbalizing your thought process helps clarify your planning:
- Narrate your understanding of the problem
- Explain potential approaches and their trade-offs
- Walk through your selected approach step by step
This practice not only helps you organize your thoughts but also demonstrates your problem-solving process to interviewers.
Practical Techniques for Effective Solution Planning
Let’s explore some specific techniques that can enhance your solution planning abilities:
The UMPIRE Method
This structured approach provides a comprehensive framework for problem-solving:
- Understand: Make sure you grasp what the problem is asking
- Match: Identify if the problem matches known patterns or algorithms
- Plan: Outline your approach before coding
- Implement: Write your code following the plan
- Review: Check your solution for correctness and efficiency
- Evaluate: Consider alternative approaches and optimizations
The Time-Box Technique
Allocate specific time blocks for different phases of your solution process:
- 5 minutes for problem understanding
- 10 minutes for solution planning
- 20 minutes for implementation
- 5 minutes for testing and review
This prevents you from getting stuck in any one phase and ensures adequate planning time.
Reverse Engineering
Start with the desired output and work backward to determine the steps needed to get there. This can be particularly effective for complex problems where the forward path isn’t immediately clear.
Template-Based Planning
Develop mental templates for common problem types. For example, for dynamic programming problems:
- Identify if the problem has optimal substructure and overlapping subproblems
- Define the state (what information needs to be tracked)
- Determine the recurrence relation (how states relate to each other)
- Identify the base cases
- Decide between top-down (memoization) or bottom-up (tabulation) approach
Common Solution Patterns to Recognize
Developing pattern recognition skills is crucial for effective solution planning. Here are some common patterns to familiarize yourself with:
Two Pointers Technique
Useful for array and string problems where you need to find pairs or subarrays with certain properties.
Example problem: Find a pair of numbers in a sorted array that sum to a target value.
function findPairSum(arr, target) {
let left = 0;
let right = arr.length - 1;
while (left < right) {
const sum = arr[left] + arr[right];
if (sum === target) {
return [left, right];
} else if (sum < target) {
left++;
} else {
right--;
}
}
return null; // No pair found
}
Sliding Window
Effective for finding subarrays or substrings that satisfy certain conditions.
Example problem: Find the maximum sum subarray of size k.
function maxSubarraySum(arr, k) {
if (arr.length < k) return null;
let maxSum = 0;
let windowSum = 0;
// Calculate sum of first window
for (let i = 0; i < k; i++) {
windowSum += arr[i];
}
maxSum = windowSum;
// Slide the window
for (let i = k; i < arr.length; i++) {
windowSum = windowSum - arr[i - k] + arr[i];
maxSum = Math.max(maxSum, windowSum);
}
return maxSum;
}
Binary Search
Applicable not just for sorted arrays, but any problem where the search space can be continuously halved.
Example problem: Find the first occurrence of a number in a sorted array.
function firstOccurrence(arr, target) {
let left = 0;
let right = arr.length - 1;
let result = -1;
while (left <= right) {
const mid = Math.floor((left + right) / 2);
if (arr[mid] === target) {
result = mid;
right = mid - 1; // Continue searching on the left side
} else if (arr[mid] < target) {
left = mid + 1;
} else {
right = mid - 1;
}
}
return result;
}
Breadth-First Search (BFS)
Ideal for finding the shortest path in unweighted graphs and for level-order traversals.
Example problem: Find the shortest path from start to end in a maze.
function shortestPath(maze, start, end) {
const rows = maze.length;
const cols = maze[0].length;
const queue = [{ position: start, distance: 0 }];
const visited = new Set();
visited.add(`${start[0]},${start[1]}`);
// Directions: up, right, down, left
const directions = [[-1, 0], [0, 1], [1, 0], [0, -1]];
while (queue.length > 0) {
const { position, distance } = queue.shift();
const [row, col] = position;
if (row === end[0] && col === end[1]) {
return distance;
}
for (const [dr, dc] of directions) {
const newRow = row + dr;
const newCol = col + dc;
const newPos = `${newRow},${newCol}`;
if (
newRow >= 0 && newRow < rows &&
newCol >= 0 && newCol < cols &&
maze[newRow][newCol] === 0 &&
!visited.has(newPos)
) {
visited.add(newPos);
queue.push({ position: [newRow, newCol], distance: distance + 1 });
}
}
}
return -1; // No path found
}
Depth-First Search (DFS)
Useful for exploring all possible paths, detecting cycles, and solving problems involving backtracking.
Example problem: Find all valid paths in a graph from start to end.
function findAllPaths(graph, start, end) {
const result = [];
const path = [start];
function dfs(current) {
if (current === end) {
result.push([...path]);
return;
}
for (const neighbor of graph[current]) {
if (!path.includes(neighbor)) { // Avoid cycles
path.push(neighbor);
dfs(neighbor);
path.pop(); // Backtrack
}
}
}
dfs(start);
return result;
}
Dynamic Programming
Essential for optimization problems with overlapping subproblems and optimal substructure.
Example problem: Calculate the nth Fibonacci number.
// Top-down approach with memoization
function fibonacci(n, memo = {}) {
if (n in memo) return memo[n];
if (n <= 1) return n;
memo[n] = fibonacci(n - 1, memo) + fibonacci(n - 2, memo);
return memo[n];
}
// Bottom-up approach with tabulation
function fibonacciDP(n) {
if (n <= 1) return n;
const dp = new Array(n + 1);
dp[0] = 0;
dp[1] = 1;
for (let i = 2; i <= n; i++) {
dp[i] = dp[i - 1] + dp[i - 2];
}
return dp[n];
}
Overcoming Mental Blocks in Solution Planning
Even with structured approaches, you may still encounter mental blocks. Here are strategies to overcome them:
Start with What You Know
If you’re stuck, begin by implementing the parts of the solution you’re confident about. This can create momentum and provide clarity for the more challenging aspects.
Work with Examples
When general approaches seem elusive, create specific examples and solve them manually. The patterns you discover can guide your general solution.
Use Analogies
Relate the current problem to ones you’ve solved before. How are they similar? How are they different? Can you adapt a previous solution?
Change Your Perspective
If you’re stuck viewing a problem one way, try a completely different angle:
- Can you solve it backward?
- Would a different data structure make it easier?
- Can you reduce it to a known problem?
Take Strategic Breaks
Sometimes the best way to overcome a block is to step away briefly. Your subconscious mind often continues working on the problem, leading to “aha” moments when you return.
Practicing and Improving Your Solution Planning Skills
Like any skill, solution planning improves with deliberate practice:
Solve Problems Deliberately
Focus on the planning phase rather than rushing to code. After understanding a problem, spend at least 5-10 minutes planning before writing any code.
Review and Reflect
After solving a problem, reflect on your planning process:
- Was your initial plan effective?
- Did you have to make significant adjustments during implementation?
- Could you have identified a better approach earlier?
Study Solution Patterns
Actively study and categorize problems based on their solution patterns. Create a personal catalog of problem types and their corresponding approaches.
Pair Programming
Working with another programmer can provide insights into different planning approaches and help you identify blind spots in your own process.
Mock Interviews
Practice explaining your solution planning process out loud in mock interview settings to refine your ability to communicate your thought process clearly.
Common Planning Pitfalls to Avoid
As you develop your solution planning skills, watch out for these common pitfalls:
Analysis Paralysis
While planning is important, overthinking can lead to inaction. Set a reasonable time limit for planning before moving to implementation.
Ignoring Constraints
Always keep the problem constraints in mind during planning. A solution that exceeds time or space complexity requirements isn’t a valid solution.
Premature Optimization
Sometimes it’s better to start with a simpler solution and optimize later. Don’t let the pursuit of the perfect algorithm prevent you from making progress.
Not Testing Your Plan
Before coding, mentally trace through your planned approach with example inputs to verify it works as expected.
Rigid Thinking
Be willing to abandon your initial plan if you discover a better approach during implementation. Flexibility is key to effective problem-solving.
Solution Planning for Different Problem Types
Different types of problems require different planning approaches:
Array and String Problems
Focus on:
- Identifying patterns in the data
- Considering sliding window, two pointers, or hash table approaches
- Determining if sorting would simplify the solution
Tree and Graph Problems
Focus on:
- Choosing between DFS and BFS based on the problem requirements
- Identifying special tree properties (BST, balanced, etc.)
- Determining if the problem involves path finding or connectivity
Dynamic Programming Problems
Focus on:
- Defining the state and transition function
- Identifying base cases
- Deciding between top-down and bottom-up approaches
System Design Problems
Focus on:
- Clarifying requirements and constraints
- Identifying components and their interactions
- Considering scalability, reliability, and performance
Case Study: Evolution of a Solution Plan
Let’s walk through a complete example of planning a solution to the following problem:
Given an array of integers, find the contiguous subarray with the largest sum.
Step 1: Understand the Problem
- Input: An array of integers (can be positive or negative)
- Output: The sum of the contiguous subarray with the largest sum
- Examples:
- Input: [-2, 1, -3, 4, -1, 2, 1, -5, 4]
- Output: 6 (subarray [4, -1, 2, 1])
- Edge cases: Empty array, all negative numbers
Step 2: Consider Multiple Approaches
Brute Force Approach:
- Consider all possible subarrays
- Calculate the sum of each
- Return the maximum sum
- Time complexity: O(n³) if we use three nested loops, or O(n²) if we optimize the sum calculation
Divide and Conquer Approach:
- Divide the array into halves
- The maximum subarray must either be entirely in the left half, entirely in the right half, or cross the middle
- Recursively find the maximum in each half
- Calculate the maximum subarray that crosses the middle
- Return the maximum of these three values
- Time complexity: O(n log n)
Dynamic Programming Approach (Kadane’s Algorithm):
- Track the maximum sum ending at each position
- For each element, decide whether to start a new subarray or extend the existing one
- Time complexity: O(n)
Step 3: Select and Plan the Optimal Approach
Kadane’s Algorithm provides the most efficient solution with O(n) time complexity:
- Initialize two variables:
currentMax
(maximum sum ending at current position) andglobalMax
(overall maximum sum) - Iterate through the array:
- For each element, decide whether to start a new subarray (
currentMax = current element
) or extend the existing one (currentMax += current element
) - Update
globalMax
ifcurrentMax
is larger
- For each element, decide whether to start a new subarray (
- Return
globalMax
as the result
Step 4: Implementation Based on the Plan
function maxSubarraySum(nums) {
if (nums.length === 0) return 0;
let currentMax = nums[0];
let globalMax = nums[0];
for (let i = 1; i < nums.length; i++) {
// Either start a new subarray or extend the existing one
currentMax = Math.max(nums[i], currentMax + nums[i]);
// Update the global maximum if needed
globalMax = Math.max(globalMax, currentMax);
}
return globalMax;
}
Step 5: Test and Refine
Let’s trace through the example: [-2, 1, -3, 4, -1, 2, 1, -5, 4]
- Initialize: currentMax = -2, globalMax = -2
- i=1: currentMax = max(1, -2+1) = 1, globalMax = 1
- i=2: currentMax = max(-3, 1+(-3)) = -2, globalMax = 1
- i=3: currentMax = max(4, -2+4) = 4, globalMax = 4
- i=4: currentMax = max(-1, 4+(-1)) = 3, globalMax = 4
- i=5: currentMax = max(2, 3+2) = 5, globalMax = 5
- i=6: currentMax = max(1, 5+1) = 6, globalMax = 6
- i=7: currentMax = max(-5, 6+(-5)) = 1, globalMax = 6
- i=8: currentMax = max(4, 1+4) = 5, globalMax = 6
The result is 6, which matches our expected output.
Conclusion: Mastering the Art of Solution Planning
Effective solution planning is not just about getting to the answer; it’s about developing a systematic approach that leads to optimal solutions while demonstrating your problem-solving capabilities. By understanding why planning is challenging, adopting structured approaches, recognizing common patterns, and practicing deliberately, you can transform your ability to tackle complex programming problems.
Remember that planning is not a fixed phase but an iterative process. As you gain insights during implementation, you may need to refine your plan. The goal is not to create a perfect plan from the start but to establish a thoughtful framework that guides your solution development.
With practice, what once seemed like an insurmountable challenge will become a natural part of your problem-solving toolkit. Your ability to plan effective solutions will not only help you ace technical interviews but also make you a more efficient and capable programmer in your day-to-day work.
The next time you face a complex coding problem, resist the urge to dive straight into coding. Take a breath, analyze the problem thoroughly, consider multiple approaches, break it down into manageable pieces, and create a clear plan. Your future self will thank you for the time invested in this critical step.