Why You Struggle to Improve Your Code During Interviews
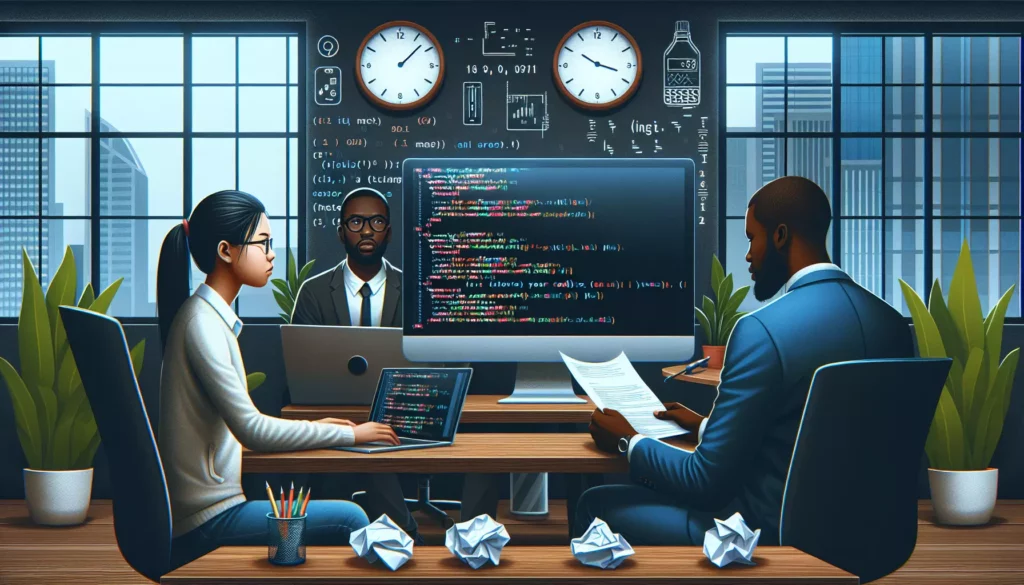
Technical interviews can be nerve-wracking experiences, especially when you’re asked to code on the spot. Many developers find themselves struggling to improve their code during interviews, even when they know they could write better solutions. This disconnect between what you know and what you can demonstrate under pressure is a common challenge that affects even experienced programmers.
In this comprehensive guide, we’ll explore why you might struggle to optimize your code during interviews and provide actionable strategies to overcome these challenges. Whether you’re preparing for your first technical interview or looking to improve your performance after previous attempts, understanding these obstacles is the first step toward conquering them.
The Psychology of Technical Interviews
Before diving into specific coding challenges, it’s important to understand the psychological factors at play during technical interviews.
Performance Anxiety and Its Impact
Technical interviews create a unique form of performance anxiety. Unlike regular coding sessions where you can take your time and refer to resources, interviews put you on the spot with someone actively evaluating your skills.
Research in cognitive psychology shows that anxiety can significantly reduce working memory capacity, which is crucial for problem-solving tasks. When you’re anxious, your brain allocates resources to managing that anxiety rather than solving the problem at hand. This explains why you might struggle to recall algorithms or optimization techniques that you know well in less stressful environments.
The Observer Effect
The presence of an interviewer watching your every keystroke creates what psychologists call the “observer effect.” Knowing you’re being evaluated can cause you to second-guess yourself and become overly cautious, leading to a more conservative approach to coding.
This often manifests as sticking with the first working solution you develop rather than exploring potentially better alternatives. The fear of making mistakes or appearing indecisive can prevent you from refactoring or optimizing your code as you would normally do in your day-to-day work.
Common Technical Challenges When Improving Code
Beyond psychological factors, there are specific technical challenges that make code improvement difficult during interviews.
Time Constraints and Prioritization
Most technical interviews have strict time limits, typically 45-60 minutes. This creates an immediate pressure to produce a working solution quickly, often at the expense of code quality or optimization.
Consider this common scenario: You’ve spent 30 minutes understanding the problem and implementing a basic solution that works. With only 15-20 minutes remaining, you face a critical decision:
- Refine and optimize your existing solution
- Ensure your current solution handles all edge cases
- Explain your thought process to the interviewer
Many candidates struggle with this prioritization, especially when they’re unsure how much the interviewer values optimization versus a working solution.
Difficulty Recognizing Optimization Opportunities
Even experienced developers can struggle to spot optimization opportunities under pressure. Common areas where candidates miss opportunities include:
Algorithmic Inefficiencies
Consider this example of a function to find duplicates in an array:
function findDuplicates(array) {
let duplicates = [];
for (let i = 0; i < array.length; i++) {
for (let j = i + 1; j < array.length; j++) {
if (array[i] === array[j] && !duplicates.includes(array[i])) {
duplicates.push(array[i]);
}
}
}
return duplicates;
}
This solution works but has O(n²) time complexity. Under interview pressure, many candidates miss the opportunity to optimize this to O(n) using a hash set:
function findDuplicatesOptimized(array) {
let seen = new Set();
let duplicates = new Set();
for (let item of array) {
if (seen.has(item)) {
duplicates.add(item);
} else {
seen.add(item);
}
}
return [...duplicates];
}
Unnecessary Operations
Interviews often reveal blind spots in recognizing redundant operations. For example, repeatedly calling expensive methods within loops or recalculating values that could be cached.
Data Structure Selection
Choosing the right data structure can make a dramatic difference in performance, but making this decision under pressure is challenging. Many candidates default to familiar structures (like arrays) even when alternatives (like hash maps or trees) would be more efficient.
Balancing Readability and Efficiency
Another common struggle is finding the right balance between code readability and efficiency. Some candidates focus so heavily on optimization that they create overly complex solutions that are difficult to understand and explain.
Consider these two implementations of a function to check if a string is a palindrome:
// Readable but not as efficient
function isPalindrome(str) {
const cleanStr = str.toLowerCase().replace(/[^a-z0-9]/g, '');
const reversed = cleanStr.split('').reverse().join('');
return cleanStr === reversed;
}
// More efficient but less readable
function isPalindromeOptimized(str) {
const cleaned = str.toLowerCase().replace(/[^a-z0-9]/g, '');
let left = 0;
let right = cleaned.length - 1;
while (left < right) {
if (cleaned[left] !== cleaned[right]) return false;
left++;
right--;
}
return true;
}
The first solution is more intuitive but creates additional memory overhead by generating new strings. The second solution is more efficient but requires more explanation. Choosing between these approaches during an interview requires balancing multiple considerations simultaneously.
Knowledge Gaps That Hinder Code Improvement
Beyond psychological pressures and technical challenges, specific knowledge gaps can prevent you from improving your code during interviews.
Algorithmic Fundamentals
A solid understanding of algorithm design and analysis is essential for code optimization. Common knowledge gaps include:
Time and Space Complexity Analysis
Many developers struggle to accurately analyze the time and space complexity of their solutions, making it difficult to identify inefficiencies. Without this skill, you might not recognize when your solution could be improved from O(n²) to O(n log n) or from O(n) to O(1) in certain operations.
Algorithm Selection
Knowing when to apply specific algorithms is crucial. For example, understanding when to use dynamic programming versus divide and conquer, or when a greedy algorithm is sufficient versus when you need a more complex approach.
Consider this problem: "Find the maximum sum of a contiguous subarray within an array."
A naive solution might use nested loops with O(n²) complexity:
function maxSubarraySum(arr) {
let maxSum = arr[0];
for (let i = 0; i < arr.length; i++) {
let currentSum = 0;
for (let j = i; j < arr.length; j++) {
currentSum += arr[j];
maxSum = Math.max(maxSum, currentSum);
}
}
return maxSum;
}
However, knowing Kadane's algorithm allows for an O(n) solution:
function maxSubarraySumOptimized(arr) {
let maxSoFar = arr[0];
let maxEndingHere = arr[0];
for (let i = 1; i < arr.length; i++) {
maxEndingHere = Math.max(arr[i], maxEndingHere + arr[i]);
maxSoFar = Math.max(maxSoFar, maxEndingHere);
}
return maxSoFar;
}
Without knowledge of such algorithms, you're limited in your ability to optimize solutions during interviews.
Language-Specific Optimizations
Each programming language has its own set of optimizations and best practices. Insufficient knowledge of language-specific features can lead to missed optimization opportunities.
Built-in Methods and Libraries
Modern programming languages provide numerous built-in methods and libraries that are often more efficient than custom implementations. For example, in JavaScript:
// Inefficient way to check if all elements meet a condition
function allPositive(numbers) {
for (let i = 0; i < numbers.length; i++) {
if (numbers[i] <= 0) return false;
}
return true;
}
// More efficient using built-in methods
function allPositiveOptimized(numbers) {
return numbers.every(num => num > 0);
}
Memory Management
Understanding how memory is managed in your language of choice can help you write more efficient code. For example, in languages with garbage collection like JavaScript or Python, creating numerous small objects can lead to performance issues due to increased garbage collection overhead.
System Design Considerations
For more senior roles, understanding how your code fits into larger systems is crucial. Knowledge gaps in areas like:
- Scalability concerns
- Concurrency and parallelism
- Caching strategies
- Database query optimization
These can prevent you from suggesting higher-level optimizations that might be more impactful than micro-optimizations.
Communication Barriers During Code Improvement
Even when you recognize optimization opportunities, communicating your thoughts effectively during an interview can be challenging.
Difficulty Articulating Trade-offs
Code optimization involves trade-offs, and explaining these trade-offs clearly is an essential skill. Common challenges include:
- Explaining why a more complex solution might be worthwhile for performance gains
- Articulating the space-time trade-offs of different approaches
- Discussing how different optimizations might affect maintainability or readability
For example, when explaining why you might choose a hash map over an array for lookups:
// Array-based lookup: O(n) time complexity
function containsValue(array, target) {
for (let item of array) {
if (item === target) return true;
}
return false;
}
// Hash-based lookup: O(1) average time complexity
function containsValueOptimized(array, target) {
const set = new Set(array);
return set.has(target);
}
Being able to clearly explain that the second approach trades additional space complexity for constant-time lookups demonstrates both technical knowledge and communication skills.
Thinking Aloud Effectively
Interviewers often encourage candidates to "think aloud" during problem-solving, but doing this while simultaneously trying to improve your code can be overwhelming. Many candidates either:
- Fall silent while thinking about optimizations, leaving the interviewer in the dark
- Verbalize thoughts in a disorganized way that makes it difficult for the interviewer to follow
Developing a structured approach to thinking aloud about code improvements is a skill that requires practice.
Practical Strategies to Improve Your Code During Interviews
Now that we've explored the challenges, let's focus on practical strategies to help you improve your code during technical interviews.
Preparation Techniques
Effective preparation can significantly reduce the cognitive load during interviews, freeing up mental resources for code optimization.
Build a Mental Library of Optimization Patterns
Rather than trying to memorize specific solutions, focus on recognizing common patterns and their optimizations. For example:
- When you see nested loops, think about using hash maps to reduce complexity
- When dealing with sorted arrays, consider binary search or two-pointer techniques
- For problems involving repeated calculations, think about memoization or dynamic programming
Here's an example of applying the hash map pattern to optimize a two-sum problem:
// Naive approach: O(n²)
function findTwoSum(nums, target) {
for (let i = 0; i < nums.length; i++) {
for (let j = i + 1; j < nums.length; j++) {
if (nums[i] + nums[j] === target) {
return [i, j];
}
}
}
return null;
}
// Optimized approach: O(n)
function findTwoSumOptimized(nums, target) {
const numMap = new Map();
for (let i = 0; i < nums.length; i++) {
const complement = target - nums[i];
if (numMap.has(complement)) {
return [numMap.get(complement), i];
}
numMap.set(nums[i], i);
}
return null;
}
Practice Incremental Improvement
When practicing coding problems, develop the habit of writing a working solution first, then systematically improving it. This simulates the interview process and builds the muscle memory for code optimization.
Follow these steps:
- Solve the problem with a basic approach
- Analyze the time and space complexity
- Identify bottlenecks or inefficiencies
- Refactor to improve performance
- Verify correctness after each change
This structured approach helps you develop a systematic process for code improvement that you can apply during interviews.
During the Interview
Once you're in the interview, these strategies can help you effectively improve your code:
Start with a Clear Plan
Before writing any code, outline your approach and discuss potential optimizations with the interviewer. This serves two purposes:
- It gives you a roadmap to follow, reducing cognitive load
- It shows the interviewer your thought process, even if you don't have time to implement all optimizations
For example: "I'll first implement a solution using a nested loop approach which will be O(n²). If time permits, I'll optimize it using a hash map to bring it down to O(n)."
Use the "Improvement Checklist" Technique
After implementing a working solution, mentally run through a checklist of common optimization opportunities:
- Can I reduce the time complexity? (e.g., eliminate nested loops)
- Can I reduce the space complexity? (e.g., use in-place operations)
- Are there any redundant operations? (e.g., repeated calculations)
- Can I use more appropriate data structures? (e.g., hash maps for lookups)
- Are there language-specific optimizations I can apply? (e.g., built-in methods)
This systematic approach ensures you don't miss obvious optimization opportunities under pressure.
Communicate Optimization Intentions
Even if you're running out of time, communicate the optimizations you would make if you had more time. This demonstrates your ability to recognize improvement opportunities even if you can't implement them all.
For example: "I notice this solution has O(n²) time complexity because of the nested loops. With more time, I would optimize it by using a hash map to store previously seen values, which would reduce the time complexity to O(n) at the cost of O(n) additional space."
Managing Psychological Pressure
Addressing the psychological aspects of interviews is just as important as technical preparation.
Practice Under Simulated Interview Conditions
Regular practice under interview-like conditions can help reduce anxiety and build confidence. Consider:
- Timed coding sessions
- Mock interviews with peers or using platforms that provide this service
- Coding while explaining your thought process aloud
The more familiar you become with the interview environment, the less cognitive resources you'll need to manage anxiety.
Develop a Stress Management Strategy
Identify techniques that help you manage stress in the moment:
- Deep breathing exercises before and during the interview
- Brief mental resets if you feel stuck (e.g., "I'm going to take 30 seconds to clear my head")
- Positive self-talk to counter negative thoughts
Having these tools available can help you maintain cognitive function under pressure.
Case Studies: Before and After Code Improvement
Let's examine some real-world examples of code improvements in interview settings.
Case Study 1: String Manipulation
Problem: Given a string, determine if it can be rearranged to form a palindrome.
Initial Solution:
function canFormPalindrome(str) {
// Count occurrences of each character
const charCount = {};
for (let char of str) {
if (charCount[char]) {
charCount[char]++;
} else {
charCount[char] = 1;
}
}
// Count characters with odd occurrences
let oddCount = 0;
for (let char in charCount) {
if (charCount[char] % 2 !== 0) {
oddCount++;
}
}
// A palindrome can have at most one character with odd count
return oddCount <= 1;
}
Improved Solution:
function canFormPalindromeOptimized(str) {
// Use a Set to track characters with odd counts
const oddChars = new Set();
for (let char of str) {
if (oddChars.has(char)) {
oddChars.delete(char); // Second occurrence makes it even
} else {
oddChars.add(char); // First occurrence is odd
}
}
// A palindrome can have at most one character with odd count
return oddChars.size <= 1;
}
Improvements:
- Reduced space complexity by using a Set to track only characters with odd counts
- Eliminated the need for a separate counting loop
- More elegant solution that's easier to explain and understand
Case Study 2: Array Processing
Problem: Find the first non-repeating element in an array.
Initial Solution:
function firstNonRepeating(arr) {
for (let i = 0; i < arr.length; i++) {
let isRepeated = false;
for (let j = 0; j < arr.length; j++) {
if (i !== j && arr[i] === arr[j]) {
isRepeated = true;
break;
}
}
if (!isRepeated) {
return arr[i];
}
}
return null; // No non-repeating element found
}
Improved Solution:
function firstNonRepeatingOptimized(arr) {
// Count occurrences of each element
const counts = new Map();
for (let num of arr) {
counts.set(num, (counts.get(num) || 0) + 1);
}
// Find the first element with count 1
for (let num of arr) {
if (counts.get(num) === 1) {
return num;
}
}
return null; // No non-repeating element found
}
Improvements:
- Reduced time complexity from O(n²) to O(n)
- Used a Map for efficient counting and lookup
- Separated the counting and searching phases for clarity
Advanced Optimization Techniques
For more experienced developers, these advanced techniques can help you stand out in technical interviews.
Algorithmic Optimizations
Beyond basic optimizations, consider these more advanced techniques:
Precomputation and Caching
Sometimes, calculating values upfront can lead to significant performance gains:
// Without precomputation
function fibonacci(n) {
if (n <= 1) return n;
return fibonacci(n - 1) + fibonacci(n - 2);
}
// With memoization
function fibonacciOptimized(n, memo = {}) {
if (n in memo) return memo[n];
if (n <= 1) return n;
memo[n] = fibonacciOptimized(n - 1, memo) + fibonacciOptimized(n - 2, memo);
return memo[n];
}
Early Termination and Short-Circuiting
Identifying opportunities to exit early can dramatically improve performance:
// Without early termination
function containsDuplicate(arr) {
const seen = new Set();
let hasDuplicate = false;
for (let num of arr) {
if (seen.has(num)) {
hasDuplicate = true;
}
seen.add(num);
}
return hasDuplicate;
}
// With early termination
function containsDuplicateOptimized(arr) {
const seen = new Set();
for (let num of arr) {
if (seen.has(num)) {
return true; // Exit as soon as a duplicate is found
}
seen.add(num);
}
return false;
}
Space-Time Trade-offs
Understanding when to trade memory for speed (or vice versa) is a crucial optimization skill.
Trading Space for Time
Consider this problem: "Determine if a given linked list has a cycle."
// Space-efficient solution using Floyd's Cycle Finding Algorithm
function hasCycle(head) {
if (!head || !head.next) return false;
let slow = head;
let fast = head;
while (fast && fast.next) {
slow = slow.next;
fast = fast.next.next;
if (slow === fast) return true; // Cycle detected
}
return false; // No cycle
}
// Time-efficient but space-intensive solution
function hasCycleWithSet(head) {
const visited = new Set();
let current = head;
while (current) {
if (visited.has(current)) {
return true; // Cycle detected
}
visited.add(current);
current = current.next;
}
return false; // No cycle
}
The first solution uses O(1) space but might take longer to detect a cycle. The second solution uses O(n) space but can detect cycles more quickly in some cases. Being able to explain this trade-off demonstrates advanced understanding.
Trading Time for Space
Sometimes, using more computation to reduce memory usage is the better approach, especially in memory-constrained environments:
// Space-intensive solution
function countUniqueValues(array) {
return new Set(array).size;
}
// Computation-intensive but space-efficient solution
function countUniqueValuesSpaceEfficient(array) {
if (array.length === 0) return 0;
array.sort((a, b) => a - b);
let uniqueCount = 1;
for (let i = 1; i < array.length; i++) {
if (array[i] !== array[i - 1]) {
uniqueCount++;
}
}
return uniqueCount;
}
System-Level Considerations
For senior-level interviews, demonstrating awareness of system-level optimizations can set you apart.
Parallelization Opportunities
Identifying when work can be parallelized shows advanced understanding:
// Sequential processing
function processData(data) {
const results = [];
for (let item of data) {
results.push(expensiveComputation(item));
}
return results;
}
// Parallel processing (conceptual JavaScript example)
async function processDataParallel(data) {
// Process items in parallel with a concurrency limit
const results = await Promise.all(
data.map(item => expensiveComputation(item))
);
return results;
}
Discussing Scalability
Explaining how your solution would scale with increasing data volume demonstrates systems thinking:
- How would your solution perform with 10x or 100x more data?
- What would be the bottlenecks?
- How might you adapt the solution for distributed processing?
Conclusion: Turning Struggles into Strengths
Improving code during interviews is challenging for most developers, but understanding why you struggle is the first step toward improvement. By addressing the psychological factors, filling knowledge gaps, and practicing structured optimization techniques, you can transform this weakness into a strength.
Remember that interviewers are often more interested in your problem-solving approach and communication skills than in perfect code. Demonstrating a methodical approach to code improvement, even if you don't implement all optimizations, can leave a strong impression.
Key takeaways from this guide:
- Prepare by building a mental library of optimization patterns
- Practice incremental improvement as part of your regular coding routine
- Use a systematic "improvement checklist" during interviews
- Communicate your optimization intentions, even when time is limited
- Manage psychological pressure through simulation and stress management techniques
With consistent practice and the right strategies, you can overcome the common struggles of code improvement during interviews and demonstrate your full potential as a developer.
Remember that mastering this skill not only helps in interview settings but also makes you a more effective developer in your day-to-day work. The ability to systematically improve code is a valuable asset throughout your career.