Why You Struggle to Implement What You Visualize: Bridging the Gap Between Concept and Code
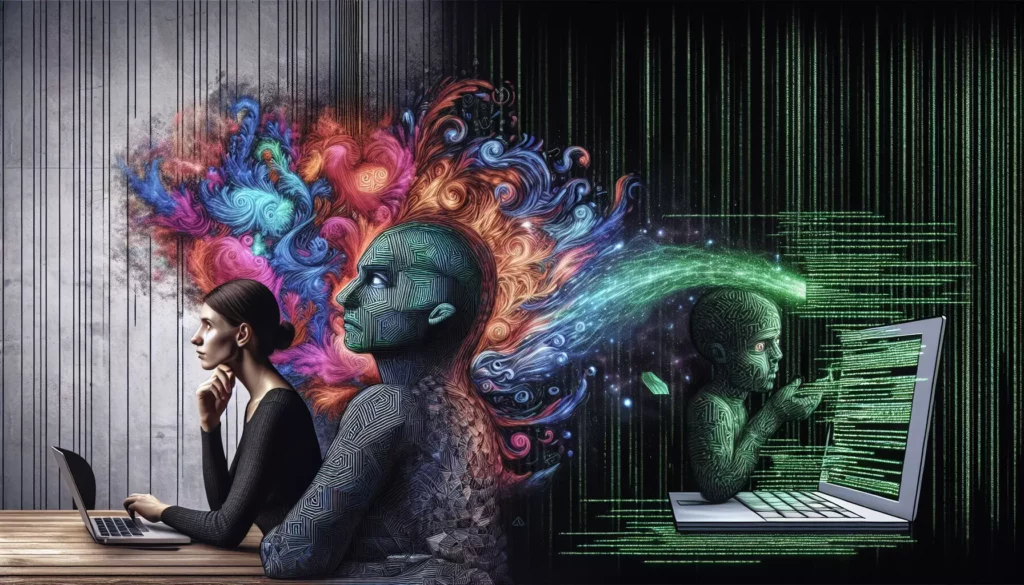
You’ve been there before: a brilliant idea flashes in your mind, a perfect solution to a coding problem materializes in your thoughts, but when you sit down to implement it, your fingers freeze over the keyboard. The elegant solution you visualized becomes tangled in syntax errors, logical flaws, and implementation hurdles. This disconnect between visualization and implementation is a common struggle for programmers at all levels, from beginners to seasoned developers.
In this comprehensive guide, we’ll explore why this gap exists and provide actionable strategies to bridge it. Whether you’re learning to code or preparing for technical interviews at top tech companies, understanding how to transform your mental models into functioning code is a critical skill for your programming journey.
Understanding the Visualization Implementation Gap
The ability to visualize a solution is fundamentally different from the ability to implement it. This gap exists for several key reasons:
1. Abstract vs. Concrete Thinking
When you visualize a solution, you’re operating in the realm of abstract thinking. You might see the big picture, understand the flow of data, or grasp the general approach. Implementation, however, requires concrete thinking: specific syntax, language constructs, and precise logical steps.
Consider a simple task like sorting a list. In your mind, you might visualize elements moving into their correct positions. But implementation requires choosing a specific algorithm, understanding its time and space complexity, handling edge cases, and writing syntactically correct code.
2. The Curse of Knowledge
Our brains often simplify complex processes when visualizing solutions. We might unconsciously skip details that seem obvious or trivial, only to discover their importance during implementation. This cognitive bias, known as the “curse of knowledge,” makes us overestimate how easily our mental models can translate to code.
3. Language and Framework Constraints
Your visualization exists in an ideal world without the limitations of programming languages or frameworks. Implementation, however, must conform to the rules, syntax, and capabilities of your chosen tools. What seems straightforward in concept might require workarounds or alternative approaches in practice.
4. Working Memory Limitations
The human brain has limited working memory capacity. While visualizing, you might hold a simplified model of the problem. During implementation, you must juggle numerous details simultaneously: variable names, function signatures, loop conditions, edge cases, and more. This cognitive load can overwhelm your working memory, causing the clear visualization to fragment during implementation.
Common Scenarios Where Visualization Fails to Translate
Let’s examine specific situations where programmers commonly experience this disconnect:
Algorithm Implementation
You might understand how a binary search works conceptually: repeatedly dividing the search space in half. However, implementing it requires handling details like calculating the middle index correctly, updating bounds properly, and managing edge cases like empty arrays or values not present in the array.
Consider this seemingly simple binary search implementation that contains a subtle bug:
function binarySearch(arr, target) {
let left = 0;
let right = arr.length - 1;
while (left <= right) {
let mid = (left + right) / 2; // Potential integer overflow in some languages
if (arr[mid] === target) {
return mid;
} else if (arr[mid] < target) {
left = mid + 1;
} else {
right = mid - 1;
}
}
return -1;
}
The bug here is subtle: calculating the middle index as (left + right) / 2
can cause integer overflow in some languages when dealing with large arrays. A better implementation would use left + Math.floor((right - left) / 2)
.
Data Structure Design
You might visualize a tree structure for organizing hierarchical data, but implementing tree traversal algorithms, maintaining balanced trees, or handling insertion and deletion operations requires attention to numerous details that may not be apparent in your mental model.
Asynchronous Programming
The concept of asynchronous operations seems straightforward: start a task and continue with other work until the task completes. However, implementing this with callbacks, promises, or async/await requires understanding complex execution flows, handling errors properly, and avoiding common pitfalls like race conditions.
User Interface Implementation
Visualizing a sleek, responsive user interface is one thing; implementing it with HTML, CSS, and JavaScript while ensuring cross-browser compatibility, accessibility, and performance is another challenge entirely.
Strategies to Bridge the Gap
Now that we understand the challenges, let’s explore effective strategies to bridge the gap between visualization and implementation:
1. Pseudocode as a Bridge
Pseudocode serves as an intermediate step between your mental model and actual code. It allows you to express your solution in a language-agnostic way, focusing on the algorithm rather than syntax details.
For example, before implementing a function to find the longest substring without repeating characters, you might write pseudocode like:
function findLongestSubstring(s):
if string is empty, return 0
create a map to track characters and their positions
set maxLength = 0
set startIndex = 0
for each character at index i in the string:
if character exists in map AND its position is >= startIndex:
update startIndex to the position after the repeated character
update maxLength if current substring length is greater
store current character position in map
return maxLength
This pseudocode captures the essence of the sliding window approach without getting bogged down in language-specific details.
2. Incremental Implementation
Rather than attempting to implement your entire visualization at once, break it down into smaller, manageable pieces. Implement one piece at a time, testing and verifying each component before moving on.
For a complex data processing pipeline, you might:
- First implement the data loading and parsing component
- Next, add the filtering logic
- Then implement the transformation steps
- Finally, add the output formatting
This incremental approach reduces cognitive load and allows you to focus on one aspect of the problem at a time.
3. Visual Aids and Diagrams
Externalize your mental model by creating diagrams, flowcharts, or other visual representations. Tools like draw.io, Miro, or even pen and paper can help you clarify your thinking and identify gaps in your understanding.
For example, when implementing a state management system, drawing a state diagram that shows all possible states and transitions can reveal edge cases you might otherwise miss during implementation.
4. Test-Driven Development (TDD)
Writing tests before implementation forces you to clarify your expectations and provides a concrete target for your code. Tests serve as specifications that guide your implementation and help you verify that your code matches your mental model.
For a function that calculates the median of an array, you might write tests like:
test('median of [1, 2, 3] should be 2', () => {
expect(findMedian([1, 2, 3])).toBe(2);
});
test('median of [1, 2, 3, 4] should be 2.5', () => {
expect(findMedian([1, 2, 3, 4])).toBe(2.5);
});
test('median of empty array should throw an error', () => {
expect(() => findMedian([])).toThrow();
});
These tests clarify the expected behavior, including edge cases, before you write a single line of implementation code.
5. Pattern Recognition and Templates
Many programming problems follow established patterns. Learning to recognize these patterns helps bridge the gap between visualization and implementation by providing proven templates you can adapt.
For example, once you recognize that a problem requires a binary search, you can apply the binary search template and focus on adapting it to your specific requirements rather than implementing it from scratch.
6. Deliberate Practice with Feedback
Regular practice with immediate feedback helps align your mental models with implementation reality. Platforms like AlgoCademy provide structured problems and feedback that help you build this alignment over time.
When practicing, focus on:
- Articulating your mental model before coding
- Implementing the solution
- Comparing your implementation to your visualization
- Identifying and learning from any discrepancies
Advanced Techniques for Complex Problems
For more complex programming challenges, additional techniques can help bridge the visualization-implementation gap:
1. Domain-Specific Languages (DSLs)
Sometimes, the gap exists because general-purpose programming languages don’t align well with how you visualize a particular problem. In such cases, creating or using a domain-specific language can provide a more natural expression of your solution.
For example, if you’re working with complex regular expressions, using a DSL or library that makes regex patterns more readable can help:
// Hard to visualize and implement directly
const emailRegex = /^[a-zA-Z0-9._%+-]+@[a-zA-Z0-9.-]+\.[a-zA-Z]{2,}$/;
// Using a hypothetical DSL or builder pattern
const emailRegex = RegexBuilder.startOfLine()
.oneOrMore(CharacterClass.alphanumeric().or('._%+-'))
.exactly('@')
.oneOrMore(CharacterClass.alphanumeric().or('.-'))
.exactly('.')
.range(CharacterClass.alpha(), 2, null)
.endOfLine()
.build();
2. Abstract Syntax Trees (ASTs)
For problems involving code manipulation, parsing, or language processing, working with ASTs can help bridge the gap between your visualization of the code structure and its implementation.
Instead of manipulating code as text, you can work with its structured representation, which often aligns better with how you visualize code transformations.
3. State Machines
For complex systems with many states and transitions, formal state machines provide a rigorous way to move from visualization to implementation. Libraries like XState allow you to define state machines declaratively and then execute them.
const toggleMachine = createMachine({
id: 'toggle',
initial: 'inactive',
states: {
inactive: {
on: { TOGGLE: 'active' }
},
active: {
on: { TOGGLE: 'inactive' }
}
}
});
This approach ensures that your implementation precisely matches your visualization of the system’s behavior.
Common Implementation Pitfalls and How to Avoid Them
Even with strategies to bridge the gap, certain implementation pitfalls commonly trip up programmers. Here’s how to recognize and avoid them:
1. Off-by-One Errors
These errors occur when loop boundaries or array indices are miscalculated by one position. They’re especially common when working with zero-indexed collections or when defining ranges.
Solution: Use explicit boundary checking and test with small examples. For ranges, consider using exclusive upper bounds (like Python’s range function) to make the code more intuitive.
2. Edge Cases
Mental visualizations often focus on the “happy path” and overlook edge cases like empty inputs, boundary values, or unexpected data types.
Solution: Systematically identify and test edge cases. Ask questions like: “What if the input is empty?” “What if values are negative?” “What if the input is already sorted?”
3. State Management Complexity
As applications grow, managing state becomes increasingly complex. What seems simple in visualization can become unwieldy in implementation.
Solution: Use established state management patterns and libraries. Break down complex state into smaller, manageable pieces. Consider immutable data structures to simplify reasoning about state changes.
4. Implicit Assumptions
Your visualization may include implicit assumptions that don’t translate to code. For example, you might assume inputs are always valid or that certain operations are atomic.
Solution: Make assumptions explicit through validation, error handling, and documentation. When working with concurrent systems, use appropriate synchronization mechanisms.
5. Premature Optimization
Attempting to optimize code before it’s working correctly can lead to unnecessary complexity and bugs.
Solution: Follow the principle “Make it work, make it right, make it fast”—in that order. Focus first on a correct implementation that matches your visualization, then refine and optimize.
The Role of Programming Languages and Abstractions
Different programming languages and abstractions can either widen or narrow the visualization-implementation gap:
High-Level vs. Low-Level Languages
High-level languages like Python or JavaScript often align more closely with how we visualize solutions, while low-level languages like C or Assembly require more detailed implementation knowledge.
Compare these implementations of finding the maximum value in an array:
Python (high-level):
def find_max(arr):
return max(arr) if arr else None
C (lower-level):
int find_max(int arr[], int size) {
if (size <= 0) return -1; // Error code
int max_val = arr[0];
for (int i = 1; i < size; i++) {
if (arr[i] > max_val) {
max_val = arr[i];
}
}
return max_val;
}
The Python implementation is much closer to how we might visualize “find the maximum value” as a single conceptual operation.
Declarative vs. Imperative Programming
Declarative approaches (describing what you want) often align better with visualization than imperative approaches (describing how to do it).
Compare these implementations for filtering even numbers:
Declarative (SQL):
SELECT * FROM numbers WHERE number % 2 = 0;
Imperative (Java):
List<Integer> filterEven(List<Integer> numbers) {
List<Integer> result = new ArrayList<>();
for (Integer num : numbers) {
if (num % 2 == 0) {
result.add(num);
}
}
return result;
}
The SQL query more directly expresses what we want (even numbers) without specifying the implementation details.
Domain-Specific Languages (DSLs)
DSLs designed for specific problem domains can significantly reduce the visualization-implementation gap by providing abstractions that match how domain experts think about problems.
For example, HTML is a DSL for describing document structure, CSS is a DSL for styling, and regular expressions form a DSL for pattern matching in text.
Case Study: Transforming a Visualization into Code
Let’s walk through a complete example of bridging the visualization-implementation gap for a common algorithmic problem: finding all valid permutations of parentheses.
Problem Statement:
Given n pairs of parentheses, write a function to generate all combinations of well-formed parentheses.
Step 1: Visualization
We might visualize this as a tree where each node represents a partial solution, and we have two choices at each step: add an opening parenthesis ‘(‘ or a closing parenthesis ‘)’.
We need to maintain two constraints:
- We can’t use more than n opening parentheses
- At any point, we can’t have more closing parentheses than opening ones (to keep the string valid)
Step 2: Pseudocode
function generateParentheses(n):
result = []
function backtrack(currentString, openCount, closeCount):
if currentString.length == 2*n:
add currentString to result
return
if openCount < n:
backtrack(currentString + '(', openCount + 1, closeCount)
if closeCount < openCount:
backtrack(currentString + ')', openCount, closeCount + 1)
backtrack('', 0, 0)
return result
Step 3: Implementation
Now we can translate our pseudocode into actual JavaScript code:
function generateParentheses(n) {
const result = [];
function backtrack(currentString, openCount, closeCount) {
// Base case: if we've used all parentheses
if (currentString.length === 2 * n) {
result.push(currentString);
return;
}
// We can add an opening parenthesis if we haven't used all n
if (openCount < n) {
backtrack(currentString + '(', openCount + 1, closeCount);
}
// We can add a closing parenthesis if it won't create an invalid string
if (closeCount < openCount) {
backtrack(currentString + ')', openCount, closeCount + 1);
}
}
backtrack('', 0, 0);
return result;
}
Step 4: Testing and Refinement
Let’s test our implementation with a small example:
console.log(generateParentheses(2));
// Expected output: ["(())", "()()"]
console.log(generateParentheses(3));
// Expected output: ["((()))", "(()())", "(())()", "()(())", "()()()"]
Step 5: Analysis and Optimization
Our implementation correctly bridges the gap between our visualization and working code. We could optimize it further by:
- Using string building techniques that are more efficient than concatenation
- Estimating the result size in advance to pre-allocate the result array
- Avoiding unnecessary function calls for invalid states
However, these optimizations would make the code more complex and potentially less aligned with our original visualization. This illustrates the tradeoff between code that closely matches our mental model and code optimized for performance.
Learning to Think Like a Computer
Ultimately, bridging the visualization-implementation gap requires developing the skill of “thinking like a computer”—understanding how your abstract ideas translate to concrete operations a computer can execute.
Mental Simulation
Practice mentally simulating how the computer will execute your code. Trace through your algorithm step by step, tracking variable values and execution flow. This helps align your visualization with the actual implementation behavior.
Understanding Abstraction Layers
Programming involves multiple layers of abstraction. Develop an awareness of when you’re thinking at one level (e.g., high-level algorithm) but implementing at another (e.g., language syntax). Explicitly switching between these levels can help manage the translation process.
Building a Library of Patterns
As you gain experience, build a mental library of implementation patterns that correspond to common visualizations. This reduces the translation effort for frequently encountered problems.
Conclusion: The Journey from Visualization to Implementation
The gap between visualizing a solution and implementing it is a fundamental challenge in programming. By understanding the cognitive processes involved and applying structured strategies to bridge this gap, you can become more effective at translating your ideas into working code.
Remember that this skill develops over time through deliberate practice. Each time you successfully implement a visualized solution, you strengthen the neural pathways that connect abstract thinking to concrete implementation details.
The strategies we’ve explored—pseudocode, incremental implementation, visual aids, test-driven development, pattern recognition, and deliberate practice—provide a framework for improving this crucial skill. As you continue your programming journey, whether you’re learning the basics or preparing for technical interviews at top tech companies, consciously applying these techniques will help you bridge the visualization-implementation gap more effectively.
The next time you find yourself stuck between a brilliant idea and its implementation, remember: the gap is normal, and with the right approach, you can systematically bridge it to bring your visualization to life in code.