Why You Struggle to Find Alternative Approaches in Coding Problems
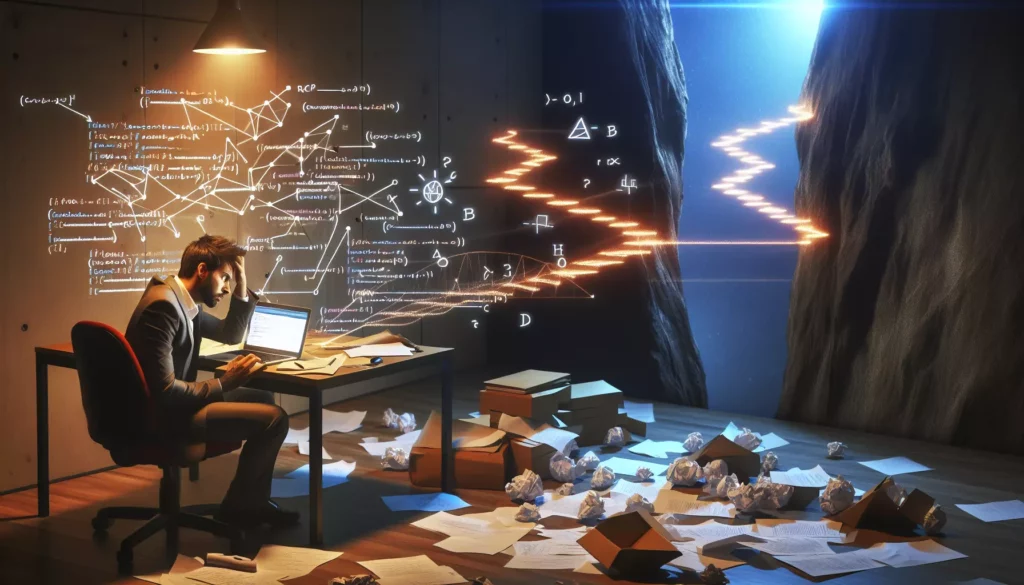
In the world of programming and coding interviews, one of the most valuable skills isn’t just finding a solution, but finding the optimal solution. Yet many programmers, from beginners to those with years of experience, often find themselves stuck in a pattern of implementing the first approach that comes to mind without exploring alternatives.
This tendency to latch onto a single solution path can be particularly problematic during technical interviews or when tackling complex algorithmic challenges. But why does this happen? And more importantly, how can we overcome this limitation to become more creative and effective problem solvers?
The Psychological Barriers to Alternative Thinking
Before we dive into strategies for improvement, it’s essential to understand the psychological mechanisms that limit our ability to consider multiple approaches to coding problems.
Cognitive Fixation: The First Solution Trap
Cognitive fixation, often called “functional fixedness” in psychology, is our tendency to perceive an object or problem in only one way. In programming, this manifests as becoming mentally locked into the first solution strategy that comes to mind.
For example, if you’ve recently learned about hash maps and encounter a new problem, you might immediately try to apply a hash map solution without considering whether a simpler array or a different data structure might be more appropriate.
Research in cognitive psychology shows that once we have a mental representation of a problem and a potential solution path, it becomes significantly harder to “unsee” that approach and consider alternatives. This is why even experienced programmers sometimes miss elegant solutions that might be obvious to others.
The Pressure of Time Constraints
Whether in a coding interview or working under a deadline, time pressure can significantly narrow our thinking. When we feel rushed, our brain tends to fall back on familiar patterns and solutions rather than exploring the problem space more broadly.
During technical interviews, this pressure is amplified by the presence of evaluators, leading many candidates to rush toward implementing the first viable solution they identify rather than taking time to consider multiple approaches.
Confirmation Bias in Problem Solving
Once we’ve identified a potential solution, confirmation bias kicks in. We naturally look for evidence that supports our chosen approach and may unconsciously dismiss information that suggests alternative routes might be better.
For instance, if you’ve decided to solve a problem using dynamic programming, you might focus on the overlapping subproblems that make this approach viable while ignoring signs that a greedy algorithm might be simpler and more efficient.
The Comfort of Familiarity
We all have our “go-to” techniques and patterns that we’ve used successfully in the past. While leveraging past experience is valuable, overreliance on familiar approaches can prevent us from adopting more efficient or elegant solutions.
This is especially true for self-taught programmers or those who have worked within limited domains, as they may have developed strong habits around particular problem-solving patterns without exposure to alternatives.
The Cost of Limited Thinking in Coding
The inability to consider multiple approaches when solving coding problems comes with several significant costs:
Suboptimal Solutions
The most obvious consequence is ending up with solutions that work but are far from optimal in terms of time complexity, space complexity, or code readability. This can result in performance issues, scalability problems, and higher maintenance costs.
Consider a sorting problem: if you automatically reach for bubble sort because it’s the first algorithm you learned, you might implement an O(n²) solution when an O(n log n) approach like merge sort or quicksort would be far more efficient.
Missed Learning Opportunities
Each problem you solve is an opportunity to expand your problem-solving toolkit. By sticking with familiar approaches, you miss chances to learn new techniques, algorithms, and data structures that could serve you well in future challenges.
Interview Performance
Technical interviews at top companies often explicitly evaluate a candidate’s ability to consider multiple approaches and discuss trade-offs. Interviewers may ask questions like “Can you think of another way to solve this?” or “What are the pros and cons of your approach versus alternatives?”
Candidates who can only present one solution path often appear less flexible and less thorough in their problem-solving process, even if their implemented solution works correctly.
Strategies to Discover Alternative Approaches
Now that we understand the barriers, let’s explore practical strategies to expand our thinking and discover alternative approaches to coding problems.
Embrace the Five-Minute Rule
Before writing any code, commit to spending at least five minutes thinking about different ways to approach the problem. This simple rule can break the habit of immediately implementing the first solution that comes to mind.
During these five minutes, sketch out multiple high-level approaches, considering different data structures, algorithms, and even brute force methods. This investment of time often leads to discovering more efficient solutions before committing to an implementation.
Systematically Consider Common Paradigms
Develop a mental checklist of common problem-solving paradigms and run through them for each new problem:
- Brute force: What’s the straightforward way to solve this?
- Divide and conquer: Can I break this into smaller subproblems?
- Dynamic programming: Are there overlapping subproblems?
- Greedy algorithms: Can I make locally optimal choices that lead to a global optimum?
- Backtracking: Is there a search space to explore?
- BFS/DFS: Can I represent this as a graph or tree traversal problem?
- Two pointers or sliding window: For array or string problems, can these techniques apply?
- Mathematical insights: Is there a mathematical pattern or property I can leverage?
By methodically considering each paradigm, you train your mind to explore the problem space more thoroughly.
Reverse the Problem
Sometimes, approaching a problem from the opposite direction can unlock new insights. If you’re struggling to find a path from input to output, try working backward from the desired output to the input.
For example, in a dynamic programming problem where you need to find the minimum cost path, you might start from the destination and work backward to the starting point, which can sometimes simplify the state transitions.
Visualize the Problem
Many programmers underestimate the power of visualization in problem-solving. Drawing diagrams, sketching data structures, or tracing through examples can often reveal patterns and approaches that aren’t obvious when thinking abstractly.
For graph problems, actually drawing the graph can help you see if BFS might be more appropriate than DFS. For array manipulations, visualizing the array and tracking changes can inspire sliding window or two-pointer techniques.
Practice Deliberate Constraints
After solving a problem, challenge yourself to solve it again with artificial constraints:
- “Solve this without using extra space.”
- “Solve this in O(n) time.”
- “Solve this using only recursion (or only iteration).”
- “Solve this without using a particular data structure you relied on.”
These self-imposed constraints force you to think outside your comfort zone and discover alternative approaches.
Study Multiple Solutions to Classic Problems
For common algorithmic problems, make it a habit to study multiple solution approaches. After solving a problem, look up alternative solutions in textbooks, online platforms, or discussion forums.
For instance, the classic “Two Sum” problem can be solved using:
- A brute force nested loop (O(n²) time, O(1) space)
- Sorting followed by two pointers (O(n log n) time, O(1) space if modifying input, O(n) otherwise)
- A hash map (O(n) time, O(n) space)
Understanding these trade-offs enriches your problem-solving toolkit.
Practical Examples: Finding Alternative Approaches
Let’s apply these strategies to some common coding problems to illustrate how different approaches can lead to dramatically different solutions.
Example 1: Finding the Maximum Subarray Sum
The problem: Given an array of integers, find the contiguous subarray with the largest sum.
Approach 1: Brute Force (O(n³) time)
function maxSubarraySum(nums) {
let maxSum = -Infinity;
for (let i = 0; i < nums.length; i++) {
for (let j = i; j < nums.length; j++) {
let currentSum = 0;
for (let k = i; k <= j; k++) {
currentSum += nums[k];
}
maxSum = Math.max(maxSum, currentSum);
}
}
return maxSum;
}
Approach 2: Optimized Brute Force (O(n²) time)
function maxSubarraySum(nums) {
let maxSum = -Infinity;
for (let i = 0; i < nums.length; i++) {
let currentSum = 0;
for (let j = i; j < nums.length; j++) {
currentSum += nums[j];
maxSum = Math.max(maxSum, currentSum);
}
}
return maxSum;
}
Approach 3: Kadane’s Algorithm (O(n) time)
function maxSubarraySum(nums) {
let maxSoFar = nums[0];
let maxEndingHere = nums[0];
for (let i = 1; i < nums.length; i++) {
maxEndingHere = Math.max(nums[i], maxEndingHere + nums[i]);
maxSoFar = Math.max(maxSoFar, maxEndingHere);
}
return maxSoFar;
}
Approach 4: Divide and Conquer (O(n log n) time)
function maxSubarraySum(nums) {
return maxSubarraySumHelper(nums, 0, nums.length - 1);
}
function maxSubarraySumHelper(nums, left, right) {
if (left === right) return nums[left];
const mid = Math.floor((left + right) / 2);
const leftSum = maxSubarraySumHelper(nums, left, mid);
const rightSum = maxSubarraySumHelper(nums, mid + 1, right);
const crossSum = maxCrossingSum(nums, left, mid, right);
return Math.max(leftSum, rightSum, crossSum);
}
function maxCrossingSum(nums, left, mid, right) {
let leftSum = -Infinity;
let sum = 0;
for (let i = mid; i >= left; i--) {
sum += nums[i];
leftSum = Math.max(leftSum, sum);
}
sum = 0;
let rightSum = -Infinity;
for (let i = mid + 1; i <= right; i++) {
sum += nums[i];
rightSum = Math.max(rightSum, sum);
}
return leftSum + rightSum;
}
As we can see, the same problem can be approached in multiple ways with dramatically different time complexities. Kadane's algorithm provides an elegant O(n) solution that might not be immediately obvious if you're fixated on the brute force approach.
Example 2: Detecting a Cycle in a Linked List
The problem: Given a linked list, determine if it contains a cycle.
Approach 1: Hash Set (O(n) time, O(n) space)
function hasCycle(head) {
const visited = new Set();
let current = head;
while (current !== null) {
if (visited.has(current)) {
return true;
}
visited.add(current);
current = current.next;
}
return false;
}
Approach 2: Floyd's Tortoise and Hare (O(n) time, O(1) space)
function hasCycle(head) {
if (head === null || head.next === null) {
return false;
}
let slow = head;
let fast = head;
while (fast !== null && fast.next !== null) {
slow = slow.next;
fast = fast.next.next;
if (slow === fast) {
return true;
}
}
return false;
}
Approach 3: Marking Nodes (O(n) time, O(1) space, but modifies the list)
function hasCycle(head) {
let current = head;
while (current !== null) {
if (current.visited) {
return true;
}
current.visited = true;
current = current.next;
}
return false;
}
Here, the Floyd's Tortoise and Hare algorithm provides an elegant solution that uses constant space, which is a significant improvement over the hash set approach if memory usage is a concern.
Building a Systematic Approach to Alternative Thinking
To truly excel at finding alternative approaches, you need to make this kind of thinking a regular part of your problem-solving process. Here's a framework you can follow:
1. Understand the Problem Deeply
Before considering solutions, make sure you fully understand:
- What are the inputs and their constraints?
- What is the expected output?
- Are there any edge cases or special conditions?
- What are the performance requirements or limitations?
A thorough understanding of the problem often reveals multiple paths forward.
2. Generate Multiple High-Level Approaches
Before diving into implementation details, brainstorm at least 2-3 high-level approaches. For each approach, consider:
- What data structures would be useful?
- What algorithms or techniques could apply?
- What's the expected time and space complexity?
3. Analyze Trade-offs
For each approach you've identified, analyze the trade-offs:
- Time complexity in best, average, and worst cases
- Space complexity
- Implementation complexity
- Readability and maintainability
- Scalability with input size
4. Choose the Most Appropriate Solution
Based on your analysis, select the approach that best meets the requirements of the problem and the context in which it will be used.
5. Implement with Care
As you implement your chosen solution, remain open to insights that might suggest further optimizations or alternative approaches.
6. Reflect and Learn
After solving the problem, take time to reflect:
- Were there approaches you didn't consider initially?
- How could you modify your solution to handle different constraints?
- What new techniques or patterns did you learn from this problem?
Common Data Structures and Their Alternative Uses
One way to expand your ability to find alternative approaches is to deepen your understanding of common data structures and the variety of problems they can solve.
Arrays and Strings
Beyond the obvious uses, arrays and strings can be used for:
- Implementing simple hash tables (when the key range is small)
- Representing graphs (adjacency matrix)
- Implementing queues and stacks
- Bit manipulation (using character arrays)
- Dynamic programming memoization
Hash Maps and Sets
Hash structures are versatile tools for:
- Counting frequencies (histogram creation)
- Finding duplicates or unique elements
- Implementing caches (e.g., for memoization)
- Grouping elements by some property
- Implementing graph adjacency lists
Stacks
Stacks can be creatively used for:
- Parsing expressions (e.g., calculator problems)
- Implementing recursive algorithms iteratively
- Tracking state in depth-first search
- Finding matching pairs (like parentheses)
- Implementing undo functionality
Queues
Queues are useful for:
- Breadth-first search
- Level-order traversal of trees
- Implementing caches (e.g., LRU cache)
- Task scheduling
- Simulating real-world queuing scenarios
Trees
Trees have applications beyond hierarchical data:
- Representing sets (BST, AVL, Red-Black)
- Priority queues (heaps)
- Implementing tries for prefix matching
- Union-find data structure (disjoint set)
- Spatial partitioning (quadtrees, k-d trees)
Graphs
Graphs can model a wide range of problems:
- Network flow and matching problems
- Dependency resolution
- Path finding and navigation
- Social networks analysis
- State machines and transitions
Learning from Others: The Power of Code Reviews and Discussions
One of the most effective ways to expand your ability to consider alternative approaches is to learn from other programmers. Here are some strategies:
Participate in Code Reviews
Whether at work or in open-source projects, code reviews expose you to different ways of thinking about problems. When reviewing code:
- Ask why the author chose a particular approach
- Suggest alternative implementations and discuss trade-offs
- Pay attention to techniques and patterns you haven't seen before
Join Coding Communities
Platforms like LeetCode, HackerRank, and Codeforces have active discussion forums where programmers share different approaches to the same problems. After solving a problem:
- Read through the top-rated solutions
- Compare approaches that use different algorithms or data structures
- Analyze why certain approaches are more efficient or elegant
Pair Programming
Coding with a partner forces you to articulate your thought process and exposes you to different problem-solving styles. During pair programming:
- Take turns suggesting approaches
- Challenge each other's assumptions
- Discuss the merits of different solutions before implementing
Study Classic Algorithms and Their Variations
Many classic algorithms have multiple variations or optimizations. For example:
- Quicksort has variations in pivot selection and partitioning
- Dijkstra's algorithm can be optimized with different priority queue implementations
- Binary search has iterative and recursive implementations, as well as variations for different scenarios
Understanding these variations broadens your problem-solving toolkit.
Overcoming Perfectionism and Analysis Paralysis
While considering multiple approaches is valuable, it's also possible to get stuck in analysis paralysis, endlessly evaluating options without making progress. Here's how to strike a balance:
Time-box Your Exploration
Set a specific time limit for exploring different approaches before committing to one. This might be 5-10 minutes for a practice problem or longer for complex production code.
Implement the Simplest Solution First
Sometimes it's best to implement a simple, working solution first, then refine it. This approach, sometimes called "make it work, make it right, make it fast," ensures you have a baseline solution before optimizing.
Use the Appropriate Level of Optimization
Not every piece of code needs to be perfectly optimized. Consider the context:
- For interview problems, showing that you can consider multiple approaches is often more important than implementing the most optimal one
- For production code, factors like readability, maintainability, and development time must be balanced against performance
- For performance-critical paths, thorough exploration of alternatives is justified
Learn from Implementation Experience
Sometimes the insights gained from implementing one approach will reveal better alternatives. Be open to pivoting if you discover a better solution during implementation.
Conclusion: Cultivating a Flexible Problem-Solving Mindset
The ability to discover and evaluate alternative approaches to coding problems is a skill that distinguishes exceptional programmers. Like any skill, it improves with deliberate practice and conscious effort.
By understanding the psychological barriers that limit our thinking, adopting strategies to explore the problem space more thoroughly, and learning from a diverse range of solutions, you can develop the mental flexibility needed to tackle complex coding challenges effectively.
Remember that the goal isn't just to find any solution or even the theoretically optimal solution, but rather to develop a process that allows you to consider the full range of possibilities and make informed decisions based on the specific requirements and constraints of each problem.
As you continue your coding journey, challenge yourself to look beyond your first instinct when approaching problems. Ask "What's another way I could solve this?" and "What are the trade-offs between these approaches?" These questions will lead you to deeper insights, more elegant solutions, and ultimately, greater success as a programmer.
Whether you're preparing for technical interviews, working on personal projects, or contributing to production code, the ability to consider alternative approaches will serve you well throughout your programming career.