Why You Should Spend More Time on Problem Decomposition than Code Writing
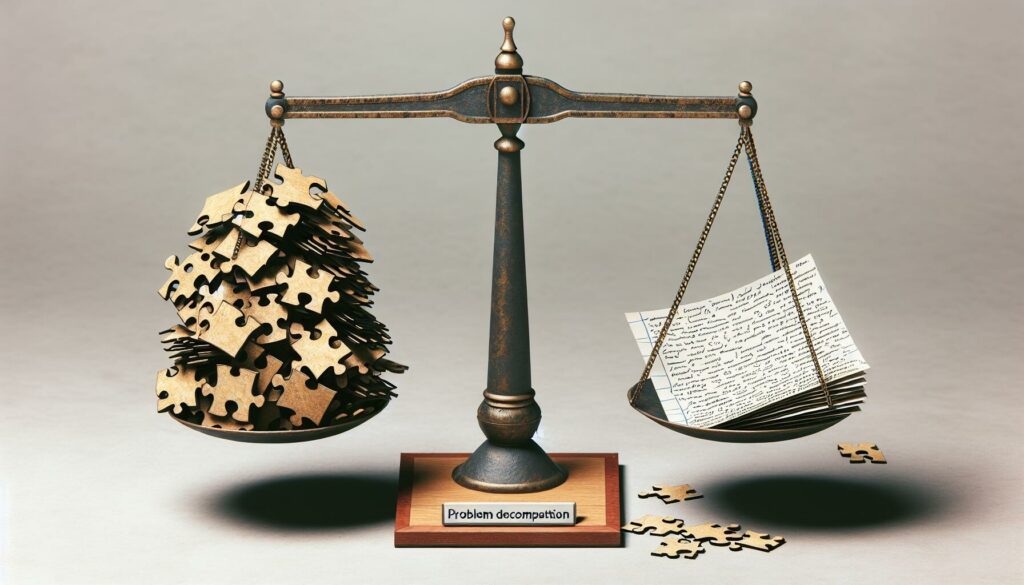
In the world of software development and coding, there’s often a rush to start typing away at the keyboard, eager to see lines of code materialize on the screen. However, experienced programmers and successful software engineers know a secret: the key to efficient and effective coding lies not in how fast you can type, but in how well you can break down a problem before you even touch the keyboard. This article will explore why spending more time on problem decomposition is crucial for your success as a programmer, and how it can dramatically improve your coding skills and outcomes.
Understanding Problem Decomposition
Before we dive into the benefits of problem decomposition, let’s clarify what it means. Problem decomposition is the process of breaking down a complex problem into smaller, more manageable parts. It’s a fundamental skill in computer science and programming that allows developers to tackle intricate challenges by addressing them piece by piece.
In the context of coding, problem decomposition involves:
- Analyzing the requirements of the problem
- Identifying the main components or steps needed to solve the problem
- Breaking these components down into smaller, solvable sub-problems
- Determining the relationships and dependencies between these sub-problems
- Outlining a high-level approach to solving each sub-problem
This process is akin to creating a roadmap for your coding journey. Instead of jumping straight into writing code, you’re taking the time to plan your route, identify potential obstacles, and decide on the best path forward.
The Benefits of Prioritizing Problem Decomposition
1. Clearer Understanding of the Problem
When you decompose a problem, you force yourself to thoroughly understand its requirements and nuances. This deeper understanding helps you avoid misinterpretations and ensures that you’re solving the right problem in the first place.
For example, consider a task to create a program that sorts a list of numbers. Through decomposition, you might realize that you need to consider:
- The type of numbers (integers, floating-point, etc.)
- The size of the list (will it fit in memory?)
- The desired order (ascending or descending)
- The efficiency requirements (time and space complexity)
By identifying these aspects upfront, you’re better equipped to choose the most appropriate sorting algorithm and implementation strategy.
2. More Efficient Problem-Solving
Breaking down a problem into smaller parts allows you to tackle each piece individually. This approach is often more efficient than trying to solve the entire problem at once. It’s easier to focus on and solve smaller, well-defined tasks than to grapple with a large, ambiguous problem.
For instance, if you’re building a web application, you might decompose it into these components:
- User authentication system
- Database design and implementation
- Frontend user interface
- Backend API
- Integration with third-party services
By addressing each component separately, you can make steady progress and avoid feeling overwhelmed by the project’s complexity.
3. Improved Code Structure and Organization
When you spend time decomposing a problem, you naturally start to identify logical separations and groupings within the solution. This understanding translates directly into better-structured code. You’re more likely to create modular, well-organized code with clear separations of concerns when you’ve thought through the problem’s components beforehand.
For example, let’s say you’re writing a program to analyze stock market data. Your decomposition might lead to a structure like this:
project/
├── data_fetcher/
│ ├── api_client.py
│ └── data_parser.py
├── analysis/
│ ├── trend_calculator.py
│ └── risk_assessor.py
├── visualization/
│ ├── chart_generator.py
│ └── report_builder.py
└── main.py
This clear structure emerges from a thorough decomposition of the problem, making the codebase more maintainable and easier to navigate.
4. Easier Collaboration and Communication
In a team setting, problem decomposition facilitates better communication and collaboration. When you can articulate the components of a problem and how they fit together, it’s easier to divide work among team members, discuss approaches, and integrate different parts of the solution.
For instance, in an e-commerce project, different team members might be assigned to work on:
- Product catalog management
- Shopping cart functionality
- Payment processing
- Order fulfillment system
With a clear decomposition, each team member understands their role and how their work fits into the larger project.
5. Reduced Debugging Time
When you decompose a problem effectively, you’re more likely to identify potential issues and edge cases before you start coding. This foresight can significantly reduce the time spent debugging later. Moreover, when bugs do occur, a well-decomposed solution makes it easier to isolate and fix the problem.
Consider a function that processes user input. Through decomposition, you might identify these steps:
- Validate input format
- Sanitize input
- Convert input to appropriate data type
- Process the data
- Handle any errors or exceptions
By breaking down the function this way, you can easily pinpoint where an issue might be occurring and focus your debugging efforts more efficiently.
6. Enhanced Problem-Solving Skills
Regularly practicing problem decomposition sharpens your analytical and problem-solving skills. These skills are transferable across different programming languages, frameworks, and even non-programming contexts. As you become better at breaking down complex problems, you’ll find that you can approach new challenges with greater confidence and clarity.
7. More Accurate Time and Resource Estimation
When you thoroughly decompose a problem, you gain a clearer picture of the work involved in solving it. This understanding allows for more accurate estimation of time and resources needed for a project. It’s much easier to estimate how long it will take to complete several small, well-defined tasks than to guess at the time required for one large, ambiguous project.
8. Improved Code Reusability
Problem decomposition often leads to the identification of common patterns or functionalities that can be abstracted into reusable components. This not only makes your current project more efficient but also creates building blocks for future projects.
For example, in developing a social media application, you might identify these reusable components:
- User authentication module
- Content posting and retrieval system
- Notification service
- Friend/follower management
These components could potentially be reused in other social or community-based applications.
Techniques for Effective Problem Decomposition
Now that we’ve explored the benefits of problem decomposition, let’s look at some techniques to improve your decomposition skills:
1. Ask the Right Questions
Start by asking yourself questions about the problem:
- What are the inputs and outputs?
- What are the constraints or limitations?
- What are the edge cases or special scenarios to consider?
- Can the problem be broken down into smaller, independent sub-problems?
- Are there any similar problems I’ve solved before?
2. Use Visual Aids
Visual representations can help in understanding and decomposing complex problems. Try using:
- Flowcharts
- Mind maps
- UML diagrams
- Pseudocode
3. Apply the Top-Down Approach
Start with the big picture and progressively break it down into smaller components. This approach helps maintain a clear overview of the problem while diving into the details.
4. Use the SOLID Principles
The SOLID principles of object-oriented design can guide your problem decomposition:
- Single Responsibility Principle
- Open-Closed Principle
- Liskov Substitution Principle
- Interface Segregation Principle
- Dependency Inversion Principle
These principles can help you identify logical separations and create more maintainable, flexible solutions.
5. Practice with Small Problems
Start by decomposing small, manageable problems and gradually work your way up to more complex ones. This progressive approach helps build your decomposition skills over time.
Implementing Problem Decomposition in Your Workflow
To make problem decomposition a natural part of your coding process, consider the following steps:
1. Allocate Time for Planning
Before you start coding, set aside dedicated time for problem analysis and decomposition. This might feel counterintuitive at first, especially if you’re used to diving straight into code, but it will save time in the long run.
2. Document Your Decomposition
Write down your problem breakdown, either in a document, as comments in your code, or using a project management tool. This documentation serves as a reference during development and can be invaluable for future maintenance or when onboarding new team members.
3. Review and Refine
As you work on the problem, be prepared to revisit and refine your initial decomposition. New insights often emerge during the coding process that can inform a better problem breakdown.
4. Seek Feedback
If possible, discuss your problem decomposition with colleagues or mentors. Fresh perspectives can help identify overlooked aspects or alternative approaches.
5. Reflect on Completed Projects
After completing a project, take time to reflect on how well your initial decomposition matched the final solution. Use these insights to improve your decomposition skills for future projects.
Case Study: Applying Problem Decomposition to a Real-World Scenario
Let’s walk through a practical example of how problem decomposition can be applied to a real-world coding challenge. Imagine you’ve been tasked with creating a simple task management application. Here’s how you might approach the decomposition:
Step 1: Define the High-Level Requirements
- Users should be able to create, read, update, and delete tasks
- Tasks should have a title, description, due date, and status
- Users should be able to categorize tasks
- The application should provide a way to view tasks by different criteria (e.g., due date, category)
Step 2: Break Down the Main Components
- User Interface (UI)
- Task list view
- Task detail view
- Task creation/edit form
- Category management interface
- Data Management
- Task data structure
- Category data structure
- Data storage mechanism (e.g., database, file system)
- Business Logic
- Task CRUD operations
- Category CRUD operations
- Task filtering and sorting
Step 3: Identify Potential Challenges and Considerations
- How to handle task dependencies?
- Should there be user authentication and multi-user support?
- How to implement reminders or notifications for upcoming due dates?
- Should the application support data export/import?
- How to ensure data persistence and handle potential data loss?
Step 4: Outline the Implementation Approach
- Design the data models for tasks and categories
- Implement the core CRUD operations for tasks and categories
- Create a basic command-line interface for testing the core functionality
- Design and implement the user interface
- Create reusable UI components (e.g., task list item, task form)
- Implement the main views (list view, detail view, edit view)
- Add navigation between views
- Implement additional features (filtering, sorting, reminders)
- Add data persistence (database integration)
- Implement error handling and input validation
- Optimize performance and user experience
- Write tests for critical functionality
Step 5: Identify Potential Reusable Components
- Data validation module
- Date and time handling utilities
- Generic CRUD operations interface
- UI components (e.g., date picker, dropdown menu)
By decomposing the problem this way, you’ve created a clear roadmap for implementing the task management application. This breakdown makes it easier to:
- Estimate the time and effort required for each component
- Divide work among team members if working collaboratively
- Identify potential challenges early in the development process
- Create a modular, well-structured application
- Test each component independently
As you progress through the implementation, you can refer back to this decomposition, adjusting it as needed based on new insights or changing requirements.
Conclusion
In the world of coding and software development, the temptation to dive straight into writing code can be strong. However, as we’ve explored in this article, taking the time to properly decompose a problem before coding can lead to numerous benefits:
- Better understanding of the problem at hand
- More efficient problem-solving
- Improved code structure and organization
- Easier collaboration and communication
- Reduced debugging time
- Enhanced overall problem-solving skills
- More accurate time and resource estimation
- Improved code reusability
By incorporating problem decomposition into your regular coding practice, you’ll not only improve the quality of your code but also become a more effective and efficient programmer. Remember, the time spent on thoughtful problem decomposition is an investment that pays off in smoother development processes, fewer bugs, and more maintainable code.
As you continue your journey in coding and software development, make it a habit to pause and decompose problems before you start typing. With practice, this skill will become second nature, and you’ll find yourself approaching even the most complex challenges with confidence and clarity. In the long run, the ability to effectively break down problems will set you apart as a programmer and contribute significantly to your success in the field.