Why You Should Practice Solving NP-Complete Problems
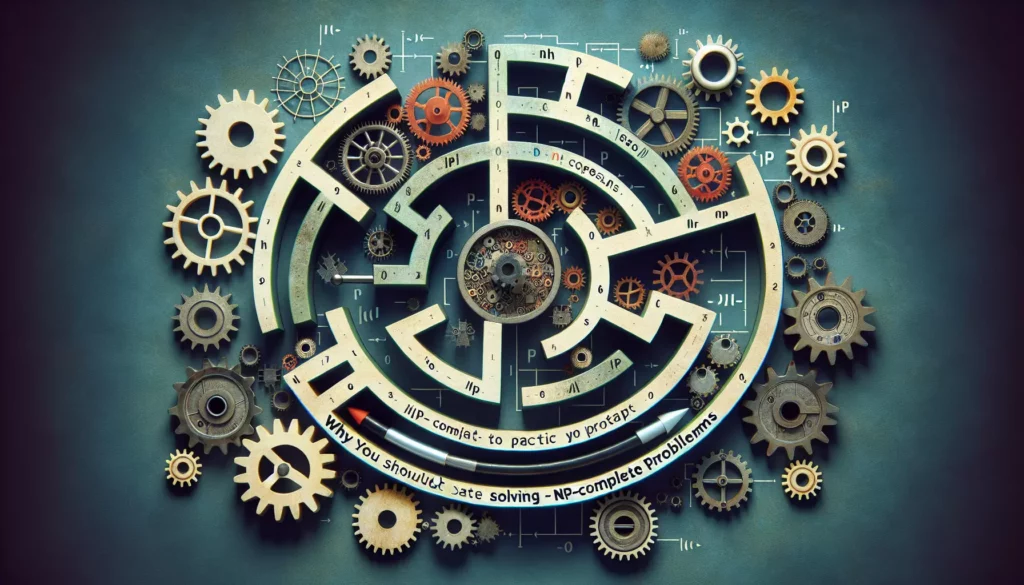
In the world of computer science and algorithm design, few topics are as challenging and intriguing as NP-complete problems. These computational puzzles have stumped mathematicians and computer scientists for decades, yet they remain at the forefront of theoretical computer science and practical problem-solving. If you’re a budding programmer, a computer science student, or even a seasoned developer looking to sharpen your skills, practicing NP-complete problems can be an invaluable exercise. In this comprehensive guide, we’ll explore why tackling these complex problems is not just an academic pursuit, but a practical skill that can significantly boost your programming prowess and problem-solving abilities.
What Are NP-Complete Problems?
Before diving into the benefits of practicing NP-complete problems, let’s briefly recap what they are. NP-complete problems are a class of computational problems that are considered to be among the hardest problems to solve efficiently. The term “NP” stands for “nondeterministic polynomial time,” which refers to the time complexity of solving these problems on a theoretical nondeterministic computer.
Some key characteristics of NP-complete problems include:
- They are decision problems (problems with yes/no answers).
- Solutions can be verified quickly (in polynomial time).
- No known polynomial-time algorithm exists to solve them.
- If a polynomial-time algorithm is found for one NP-complete problem, it could be used to solve all NP problems in polynomial time.
Examples of NP-complete problems include the Traveling Salesman Problem, the Boolean Satisfiability Problem (SAT), and the Knapsack Problem. These problems have applications in various fields, from logistics and scheduling to cryptography and artificial intelligence.
Why Practice Solving NP-Complete Problems?
1. Enhances Problem-Solving Skills
NP-complete problems are, by definition, some of the most challenging computational problems known. Tackling these problems head-on forces you to think creatively and approach problem-solving from multiple angles. As you work on these problems, you’ll develop and refine various problem-solving strategies, such as:
- Breaking down complex problems into smaller, manageable subproblems
- Identifying patterns and symmetries that can simplify the problem
- Applying heuristics and approximation algorithms when exact solutions are impractical
- Analyzing trade-offs between time complexity and solution quality
These skills are transferable to a wide range of programming and algorithmic challenges, making you a more versatile and effective problem solver.
2. Improves Algorithm Design and Analysis Skills
Working on NP-complete problems exposes you to a variety of advanced algorithmic techniques. You’ll become familiar with concepts such as:
- Dynamic programming
- Backtracking
- Branch and bound
- Approximation algorithms
- Randomized algorithms
As you implement and analyze these algorithms, you’ll gain a deeper understanding of algorithmic complexity, space-time trade-offs, and the limitations of computational approaches. This knowledge is crucial for designing efficient algorithms for real-world problems, even when they’re not NP-complete.
3. Prepares You for Technical Interviews
Many top tech companies, including FAANG (Facebook, Amazon, Apple, Netflix, Google) and others, often include algorithm-heavy questions in their technical interviews. While they may not explicitly ask you to solve NP-complete problems, the skills you develop by practicing these problems will give you a significant advantage.
You’ll be better equipped to:
- Quickly identify the complexity of a given problem
- Propose and analyze multiple approaches to solving a problem
- Communicate your thought process clearly and effectively
- Optimize solutions for better time or space complexity
These abilities are highly valued in technical interviews and can set you apart from other candidates.
4. Fosters Computational Thinking
Computational thinking is a problem-solving approach that involves breaking down complex problems, recognizing patterns, and developing step-by-step solutions. NP-complete problems are excellent exercises in computational thinking because they require you to:
- Analyze the problem structure and constraints
- Develop abstract models of the problem
- Design algorithms that exploit the problem’s properties
- Evaluate and refine your solutions
By honing your computational thinking skills, you’ll become better at tackling not just programming problems, but also complex challenges in other domains.
5. Builds Resilience and Persistence
NP-complete problems are notoriously difficult, and finding optimal solutions is often impossible for large instances. This challenge can be frustrating, but it also teaches valuable lessons in persistence and resilience. You’ll learn to:
- Persist in the face of difficult challenges
- Embrace failure as a learning opportunity
- Iterate on your solutions to make incremental improvements
- Recognize when to shift your approach or seek alternative solutions
These qualities are essential for success in any programming career, where complex problems and unexpected obstacles are common.
6. Exposes You to Important Theoretical Concepts
Working on NP-complete problems will inevitably lead you to explore fundamental concepts in theoretical computer science, such as:
- Complexity theory
- Computability
- Reduction techniques
- The P vs. NP problem
While these topics may seem abstract, they provide a deeper understanding of the limits and possibilities of computation. This knowledge can inform your approach to practical programming tasks and help you make more informed decisions about algorithm selection and problem-solving strategies.
7. Improves Code Optimization Skills
Because NP-complete problems are computationally intensive, working on them will push you to optimize your code for better performance. You’ll learn techniques for:
- Reducing time complexity through clever algorithm design
- Minimizing space usage with efficient data structures
- Implementing memoization and caching strategies
- Leveraging problem-specific optimizations
These optimization skills are valuable in any programming context, especially when dealing with large-scale systems or resource-constrained environments.
8. Encourages Interdisciplinary Thinking
NP-complete problems often have connections to various fields beyond computer science, such as:
- Mathematics (graph theory, combinatorics)
- Operations research
- Biology (protein folding)
- Physics (spin glass models)
- Economics (game theory)
Exploring these connections can broaden your perspective and help you apply computational thinking to diverse domains. This interdisciplinary approach can lead to innovative solutions and open up new career opportunities.
9. Develops Approximation and Heuristic Thinking
Given the intractability of NP-complete problems for large instances, working on them encourages you to think in terms of approximations and heuristics. You’ll learn to:
- Design and analyze approximation algorithms with provable performance guarantees
- Develop effective heuristics that work well in practice
- Evaluate the trade-offs between solution quality and computational resources
- Apply metaheuristic techniques like genetic algorithms or simulated annealing
These skills are invaluable when dealing with real-world optimization problems where finding the absolute best solution is often impractical or unnecessary.
10. Prepares You for Cutting-Edge Research and Development
Many cutting-edge areas of computer science and artificial intelligence involve tackling NP-complete or NP-hard problems. By familiarizing yourself with these problems, you’ll be better prepared to contribute to fields such as:
- Quantum computing
- Machine learning and optimization
- Cryptography
- Automated planning and scheduling
- Bioinformatics
This knowledge can open doors to exciting research opportunities or innovative projects in industry.
How to Practice Solving NP-Complete Problems
Now that we’ve explored the benefits of practicing NP-complete problems, let’s discuss some strategies for incorporating this practice into your learning routine:
1. Start with Classic Problems
Begin by familiarizing yourself with well-known NP-complete problems such as:
- Traveling Salesman Problem
- Boolean Satisfiability Problem (SAT)
- Knapsack Problem
- Graph Coloring
- Subset Sum Problem
Study their formulations, understand why they’re NP-complete, and explore different approaches to solving them.
2. Implement Multiple Algorithms
For each problem, try implementing different types of algorithms, such as:
- Exact algorithms (e.g., backtracking, branch and bound)
- Approximation algorithms
- Heuristic approaches
- Randomized algorithms
Compare their performance on various input sizes and analyze their trade-offs.
3. Use Online Platforms and Contests
Participate in coding contests and use online platforms that feature algorithmic problems. While not all problems will be NP-complete, many will require similar problem-solving skills. Some popular platforms include:
- LeetCode
- HackerRank
- Codeforces
- Project Euler
4. Study Research Papers and Advanced Texts
Delve into academic literature on NP-complete problems and approximation algorithms. This will expose you to cutting-edge techniques and deepen your theoretical understanding. Some recommended texts include:
- “Computers and Intractability: A Guide to the Theory of NP-Completeness” by Michael Garey and David Johnson
- “Approximation Algorithms” by Vijay V. Vazirani
- “The Nature of Computation” by Cristopher Moore and Stephan Mertens
5. Collaborate and Discuss
Join online communities or study groups focused on algorithms and computational complexity. Discussing problems and solutions with others can provide new insights and help you learn from different perspectives. Consider platforms like:
- Stack Overflow
- Computer Science Stack Exchange
- Reddit (r/compsci, r/algorithms)
- GitHub (for open-source algorithm implementations)
6. Apply to Real-World Scenarios
Look for opportunities to apply your knowledge of NP-complete problems to real-world scenarios. This could involve:
- Optimizing resource allocation in a project management tool
- Improving route planning for a delivery service
- Enhancing scheduling algorithms for a cloud computing platform
Practical applications will reinforce your understanding and demonstrate the relevance of these problems beyond academic exercises.
Conclusion
Practicing NP-complete problems is not just an academic exercise—it’s a powerful way to enhance your problem-solving skills, algorithmic thinking, and overall programming abilities. By tackling these challenging problems, you’ll develop a deeper understanding of computational complexity, improve your code optimization skills, and prepare yourself for advanced topics in computer science.
Whether you’re aiming to ace technical interviews at top tech companies, contribute to cutting-edge research, or simply become a more proficient programmer, the skills you gain from working on NP-complete problems will serve you well throughout your career. So embrace the challenge, persist through the difficulties, and enjoy the intellectual rewards that come from unraveling some of computer science’s most perplexing puzzles.
Remember, the journey of mastering NP-complete problems is ongoing. Each problem you tackle will teach you something new and push your boundaries as a problem solver and programmer. So start today, and watch as your computational thinking and algorithmic skills reach new heights!