Why You Should Learn Object-Oriented Programming in Python
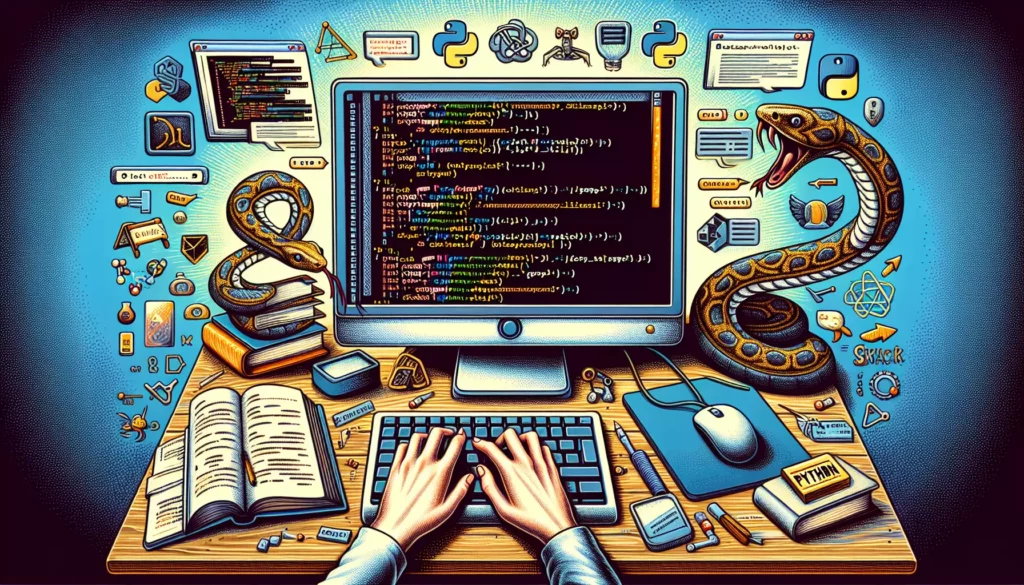
In the ever-evolving landscape of programming, Object-Oriented Programming (OOP) stands as a cornerstone of modern software development. When combined with the versatility and simplicity of Python, OOP becomes an even more powerful tool in a programmer’s arsenal. This article will explore the numerous reasons why you should learn Object-Oriented Programming in Python, and how it can significantly enhance your coding skills and career prospects.
1. Understanding the Basics of Object-Oriented Programming
Before diving into the benefits of learning OOP in Python, let’s briefly review what Object-Oriented Programming is and its core concepts.
1.1 What is Object-Oriented Programming?
Object-Oriented Programming is a programming paradigm that organizes code into objects, which are instances of classes. This approach allows for better organization, reusability, and maintenance of code. OOP is based on four main principles:
- Encapsulation: Bundling data and methods that operate on that data within a single unit (class).
- Inheritance: Creating new classes based on existing classes, inheriting their attributes and methods.
- Polymorphism: The ability of objects to take on multiple forms and behave differently based on their context.
- Abstraction: Hiding complex implementation details and exposing only the necessary features of an object.
1.2 OOP in Python
Python is an excellent language for learning and implementing OOP concepts. Its syntax is clear and concise, making it easier to understand and write object-oriented code. Here’s a simple example of a class in Python:
class Dog:
def __init__(self, name, age):
self.name = name
self.age = age
def bark(self):
print(f"{self.name} says: Woof!")
# Creating an instance of the Dog class
my_dog = Dog("Buddy", 3)
my_dog.bark() # Output: Buddy says: Woof!
This simple example demonstrates how easy it is to create classes and objects in Python, making it an ideal language for learning OOP concepts.
2. Enhancing Code Organization and Reusability
One of the primary reasons to learn OOP in Python is its ability to improve code organization and reusability. Let’s explore how OOP achieves this:
2.1 Modular Code Structure
OOP allows you to organize your code into logical, self-contained units (classes). This modular structure makes it easier to manage large codebases and collaborate with other developers. Each class can be developed, tested, and maintained independently, reducing the complexity of the overall system.
2.2 Code Reusability
With OOP, you can create reusable components that can be easily integrated into different projects. This not only saves time but also promotes consistency across your codebase. For example, you might create a generic “Database” class that can be used in multiple projects:
class Database:
def __init__(self, connection_string):
self.connection_string = connection_string
def connect(self):
# Code to establish database connection
pass
def execute_query(self, query):
# Code to execute SQL query
pass
# Using the Database class in different projects
project1_db = Database("connection_string_1")
project2_db = Database("connection_string_2")
This reusable Database class can be easily imported and used in various projects, saving time and ensuring consistency in database operations.
3. Improving Code Maintainability
Maintainability is crucial for long-term project success. OOP in Python offers several features that enhance code maintainability:
3.1 Encapsulation for Data Protection
Encapsulation allows you to hide the internal details of a class and expose only what’s necessary. This protects the data from unintended modifications and makes the code more robust. Python uses naming conventions to implement encapsulation:
class BankAccount:
def __init__(self, balance):
self.__balance = balance # Private attribute
def deposit(self, amount):
if amount > 0:
self.__balance += amount
def get_balance(self):
return self.__balance
account = BankAccount(1000)
account.deposit(500)
print(account.get_balance()) # Output: 1500
# print(account.__balance) # This would raise an AttributeError
In this example, the __balance attribute is private and can only be accessed or modified through the class methods, ensuring data integrity.
3.2 Inheritance for Code Extension
Inheritance allows you to create new classes based on existing ones, promoting code reuse and establishing a hierarchical relationship between classes. This makes it easier to extend and modify code without affecting the existing functionality:
class Animal:
def __init__(self, name):
self.name = name
def speak(self):
pass
class Dog(Animal):
def speak(self):
return f"{self.name} says Woof!"
class Cat(Animal):
def speak(self):
return f"{self.name} says Meow!"
dog = Dog("Buddy")
cat = Cat("Whiskers")
print(dog.speak()) # Output: Buddy says Woof!
print(cat.speak()) # Output: Whiskers says Meow!
This example demonstrates how inheritance allows you to create specialized classes (Dog and Cat) from a more general class (Animal), reusing common attributes and methods while adding or overriding specific behaviors.
4. Facilitating Problem-Solving and Design
Learning OOP in Python can significantly improve your problem-solving skills and ability to design efficient solutions:
4.1 Abstraction for Complexity Management
Abstraction allows you to manage complexity by hiding unnecessary details and exposing only the essential features of an object. This makes it easier to design and implement complex systems:
from abc import ABC, abstractmethod
class Shape(ABC):
@abstractmethod
def area(self):
pass
class Circle(Shape):
def __init__(self, radius):
self.radius = radius
def area(self):
return 3.14 * self.radius ** 2
class Rectangle(Shape):
def __init__(self, width, height):
self.width = width
self.height = height
def area(self):
return self.width * self.height
# Using the shapes
shapes = [Circle(5), Rectangle(4, 6)]
for shape in shapes:
print(f"Area: {shape.area()}")
In this example, the Shape class provides an abstraction for different geometric shapes. The specific implementation details are hidden within the Circle and Rectangle classes, allowing you to work with shapes at a higher level of abstraction.
4.2 Polymorphism for Flexible Design
Polymorphism allows objects of different classes to be treated as objects of a common base class. This promotes flexibility in design and makes it easier to extend your code:
class Employee:
def __init__(self, name, salary):
self.name = name
self.salary = salary
def get_bonus(self):
return self.salary * 0.1
class Manager(Employee):
def get_bonus(self):
return self.salary * 0.2
class Developer(Employee):
def get_bonus(self):
return self.salary * 0.15
# Calculate bonuses
employees = [
Employee("John", 50000),
Manager("Alice", 80000),
Developer("Bob", 60000)
]
for employee in employees:
print(f"{employee.name}'s bonus: ${employee.get_bonus()}")
This example demonstrates how polymorphism allows you to treat different types of employees uniformly while still maintaining their specific bonus calculation logic.
5. Preparing for Advanced Programming Concepts
Learning OOP in Python lays a strong foundation for understanding and implementing advanced programming concepts:
5.1 Design Patterns
OOP is fundamental to understanding and implementing design patterns, which are reusable solutions to common software design problems. For example, the Singleton pattern ensures that a class has only one instance:
class Singleton:
_instance = None
def __new__(cls):
if cls._instance is None:
cls._instance = super().__new__(cls)
return cls._instance
# Usage
s1 = Singleton()
s2 = Singleton()
print(s1 is s2) # Output: True
Understanding OOP principles makes it easier to grasp and implement such design patterns, which are crucial for writing efficient and maintainable code.
5.2 Framework and Library Usage
Many popular Python frameworks and libraries, such as Django, Flask, and PyQt, are built using OOP principles. Learning OOP will help you understand and effectively use these tools:
from flask import Flask
app = Flask(__name__)
@app.route('/')
def hello_world():
return 'Hello, World!'
if __name__ == '__main__':
app.run()
In this Flask example, the app object is an instance of the Flask class, demonstrating how OOP is used in real-world web development frameworks.
6. Enhancing Career Opportunities
Proficiency in OOP with Python can significantly boost your career prospects in the tech industry:
6.1 Industry Demand
Many companies, especially those working on large-scale projects, prefer developers with strong OOP skills. Python’s popularity in fields like web development, data science, and machine learning makes OOP skills in Python particularly valuable.
6.2 Interview Preparation
OOP concepts are often a focus in technical interviews, especially for roles at major tech companies. Being able to design and implement object-oriented solutions can give you an edge in these interviews:
class ParkingLot:
def __init__(self, capacity):
self.capacity = capacity
self.available_spots = capacity
self.parked_cars = {}
def park_car(self, car_id):
if self.available_spots > 0:
self.parked_cars[car_id] = True
self.available_spots -= 1
return True
return False
def remove_car(self, car_id):
if car_id in self.parked_cars:
del self.parked_cars[car_id]
self.available_spots += 1
return True
return False
# Usage in an interview scenario
parking_lot = ParkingLot(100)
print(parking_lot.park_car("ABC123")) # Output: True
print(parking_lot.park_car("XYZ789")) # Output: True
print(parking_lot.remove_car("ABC123")) # Output: True
print(parking_lot.remove_car("DEF456")) # Output: False
This example demonstrates how OOP can be used to model real-world scenarios, a common task in technical interviews.
7. Facilitating Collaboration and Team Work
OOP promotes better collaboration among developers working on the same project:
7.1 Clear Code Structure
The class-based structure of OOP makes it easier for team members to understand and work on different parts of a project independently. Each class represents a distinct component with clear responsibilities.
7.2 Interface-Based Development
OOP allows for interface-based development, where teams can agree on method signatures and behaviors before implementing the details. This is particularly useful in large projects:
from abc import ABC, abstractmethod
class PaymentGateway(ABC):
@abstractmethod
def process_payment(self, amount):
pass
class PayPalGateway(PaymentGateway):
def process_payment(self, amount):
# Implementation for PayPal
pass
class StripeGateway(PaymentGateway):
def process_payment(self, amount):
# Implementation for Stripe
pass
# Usage
def checkout(payment_gateway, amount):
payment_gateway.process_payment(amount)
# Different team members can work on different gateways
paypal = PayPalGateway()
stripe = StripeGateway()
checkout(paypal, 100)
checkout(stripe, 200)
This example shows how different team members can work on separate payment gateways while adhering to a common interface, facilitating parallel development and integration.
8. Improving Code Testing and Debugging
OOP principles facilitate better testing and debugging practices:
8.1 Unit Testing
The modular nature of OOP makes it easier to write and run unit tests for individual components of your system:
import unittest
class Calculator:
def add(self, a, b):
return a + b
def subtract(self, a, b):
return a - b
class TestCalculator(unittest.TestCase):
def setUp(self):
self.calc = Calculator()
def test_add(self):
self.assertEqual(self.calc.add(3, 5), 8)
def test_subtract(self):
self.assertEqual(self.calc.subtract(10, 5), 5)
if __name__ == '__main__':
unittest.main()
This example demonstrates how OOP facilitates the creation of testable code units, improving overall code quality and reliability.
8.2 Debugging
The encapsulation provided by OOP helps in isolating bugs to specific classes or methods, making debugging more straightforward.
9. Preparing for Advanced Python Features
Understanding OOP in Python prepares you for more advanced Python features:
9.1 Decorators
Decorators, a powerful feature in Python, are easier to understand and implement with a solid grasp of OOP concepts:
def log_function_call(func):
def wrapper(*args, **kwargs):
print(f"Calling function: {func.__name__}")
result = func(*args, **kwargs)
print(f"Function {func.__name__} returned: {result}")
return result
return wrapper
@log_function_call
def add(a, b):
return a + b
print(add(3, 5))
# Output:
# Calling function: add
# Function add returned: 8
# 8
This example shows how decorators can be used to modify or enhance the behavior of functions or methods, a concept rooted in OOP principles.
9.2 Metaclasses
Metaclasses, which are classes of classes in Python, become more approachable with a strong understanding of OOP:
class SingletonMeta(type):
_instances = {}
def __call__(cls, *args, **kwargs):
if cls not in cls._instances:
cls._instances[cls] = super().__call__(*args, **kwargs)
return cls._instances[cls]
class Singleton(metaclass=SingletonMeta):
pass
# Usage
s1 = Singleton()
s2 = Singleton()
print(s1 is s2) # Output: True
This example demonstrates how metaclasses can be used to implement advanced patterns like the Singleton pattern, showcasing the power and flexibility of OOP in Python.
10. Conclusion
Learning Object-Oriented Programming in Python offers numerous benefits that can significantly enhance your programming skills and career prospects. From improving code organization and reusability to facilitating problem-solving and design, OOP provides a powerful paradigm for developing robust and maintainable software.
By mastering OOP in Python, you’ll be better prepared to tackle complex programming challenges, collaborate effectively in team environments, and leverage advanced Python features. Whether you’re aiming to build large-scale applications, contribute to open-source projects, or excel in technical interviews, a strong foundation in OOP will prove invaluable.
As you continue your journey in programming, remember that OOP is not just a set of techniques, but a way of thinking about and structuring your code. Embrace these principles, practice regularly, and you’ll find yourself writing cleaner, more efficient, and more powerful Python code.
Start your OOP journey in Python today, and unlock a world of programming possibilities!