Why You Should Learn Git as a Junior Developer
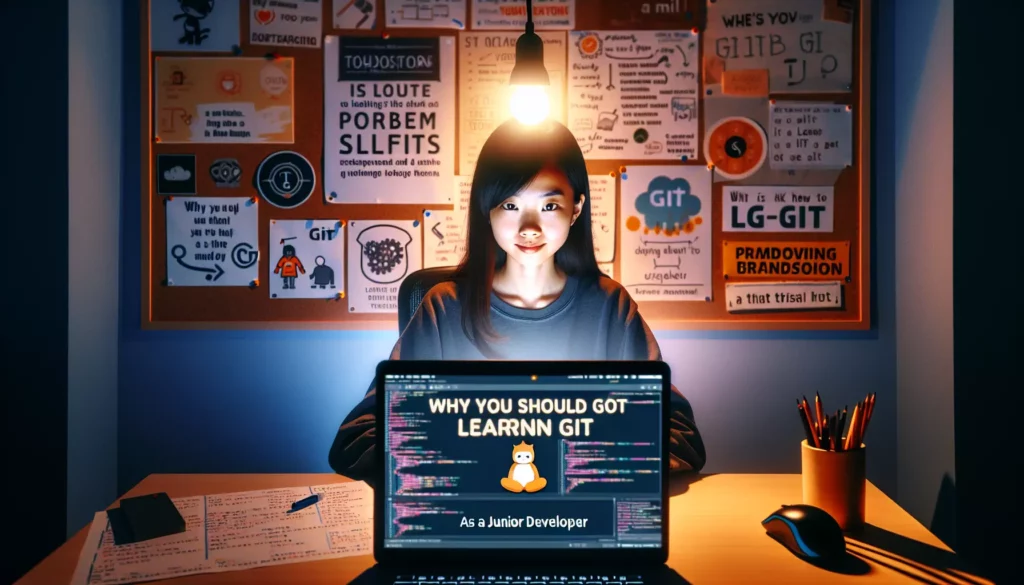
As a junior developer embarking on your coding journey, you’re likely focused on mastering programming languages, understanding data structures, and honing your problem-solving skills. While these are undoubtedly crucial aspects of your development, there’s another tool that’s equally important but often overlooked by beginners: Git. In this comprehensive guide, we’ll explore why learning Git should be a priority for every junior developer and how it can significantly boost your career prospects.
What is Git?
Before diving into the reasons why Git is essential, let’s briefly explain what Git is. Git is a distributed version control system that helps developers track changes in their code, collaborate with others, and manage different versions of their projects. It was created by Linus Torvalds in 2005 and has since become the de facto standard for version control in the software development industry.
1. Version Control is Crucial for Professional Development
As a junior developer, you might be working on small projects or assignments where keeping track of changes seems manageable without a specialized tool. However, as you progress in your career and start working on larger, more complex projects, version control becomes indispensable. Here’s why:
- Track Changes: Git allows you to track every change made to your codebase, providing a detailed history of your project’s evolution.
- Revert Mistakes: Made a mistake? Git enables you to easily roll back to a previous version of your code.
- Experiment Safely: With Git branches, you can experiment with new features or ideas without affecting the main codebase.
- Collaborate Effectively: Git facilitates seamless collaboration among team members, allowing multiple developers to work on the same project simultaneously.
2. Git is an Industry Standard
Learning Git isn’t just about adding another tool to your skillset; it’s about aligning yourself with industry standards. Here’s why this matters:
- Job Requirements: Most software development job listings mention Git as a required skill. By learning Git early, you’re making yourself more marketable to potential employers.
- Professional Workflow: Understanding Git helps you adapt quickly to professional development workflows used in most companies.
- Open Source Contributions: Git is the backbone of open-source development. If you want to contribute to open-source projects (which can greatly enhance your portfolio), Git knowledge is essential.
3. Git Enhances Your Problem-Solving Skills
While Git is primarily a tool for version control, learning and using it can significantly improve your problem-solving abilities:
- Logical Thinking: Understanding Git’s branching and merging concepts requires logical thinking, which is a crucial skill for any developer.
- Conflict Resolution: Resolving merge conflicts in Git teaches you to analyze and reconcile different versions of code, a valuable skill in collaborative environments.
- System Design: As you work with Git, you’ll start thinking about how to structure your commits and branches, which parallels the thought process in system design.
4. Git Improves Code Quality
Using Git can lead to improvements in your overall code quality:
- Code Reviews: Git platforms like GitHub and GitLab facilitate code reviews, allowing you to receive feedback from peers and mentors.
- Atomic Commits: Git encourages the practice of making small, focused commits, which leads to cleaner, more maintainable code.
- Documentation: Writing clear commit messages serves as a form of documentation, helping you and others understand the reasoning behind code changes.
5. Git Facilitates Continuous Integration and Deployment
In modern software development, continuous integration and continuous deployment (CI/CD) are crucial practices. Git plays a central role in these processes:
- Automated Testing: Git integrates seamlessly with CI tools, allowing automated tests to run on every commit or pull request.
- Deployment Pipelines: Many deployment pipelines are triggered by Git actions, such as merging to a specific branch.
- Feature Flags: Git branches can be used in conjunction with feature flags for controlled feature releases.
6. Git Provides a Safety Net
As a junior developer, making mistakes is part of the learning process. Git provides a safety net that allows you to experiment and learn without fear:
- Undo Changes: If you make a mistake, you can easily revert your changes to a previous working state.
- Backup: By pushing your code to remote repositories, you create backups of your work, protecting against data loss.
- Experimentation: Git branches allow you to experiment with new ideas without risking the stability of your main codebase.
7. Git Enhances Your Understanding of Software Development Lifecycle
Using Git exposes you to various aspects of the software development lifecycle:
- Project Management: Git issues and project boards are often used for task tracking and project management.
- Release Management: Git tags and releases help in managing software versions and releases.
- Code Review Process: Pull requests in Git platforms introduce you to the code review process, an essential part of professional development.
8. Git Builds Your Professional Network
Git platforms like GitHub serve as social networks for developers:
- Portfolio Building: Your Git repositories can serve as a portfolio of your work, showcasing your skills to potential employers.
- Collaboration Opportunities: By contributing to open-source projects, you can connect with other developers and build your professional network.
- Community Engagement: Participating in discussions on Git platforms can help you engage with the developer community and stay updated with industry trends.
Getting Started with Git
Now that we’ve explored the numerous reasons why learning Git is crucial for junior developers, let’s look at how you can get started:
1. Install Git
First, you need to install Git on your computer. You can download it from the official Git website: https://git-scm.com/downloads
2. Configure Git
After installation, configure your Git username and email. Open a terminal and run these commands:
git config --global user.name "Your Name"
git config --global user.email "youremail@example.com"
3. Learn Basic Git Commands
Start by learning these essential Git commands:
git init
: Initialize a new Git repositorygit clone
: Clone a repository from a remote sourcegit add
: Stage changes for commitgit commit
: Commit staged changesgit push
: Push commits to a remote repositorygit pull
: Fetch and merge changes from a remote repositorygit branch
: List, create, or delete branchesgit merge
: Merge changes from different branches
4. Practice with a Simple Project
Create a simple project and practice using Git. Here’s a basic workflow:
mkdir my-first-git-project
cd my-first-git-project
git init
echo "# My First Git Project" > README.md
git add README.md
git commit -m "Initial commit"
git branch -M main
git remote add origin https://github.com/yourusername/my-first-git-project.git
git push -u origin main
5. Explore Git Platforms
Sign up for GitHub, GitLab, or Bitbucket and start exploring. Create repositories, contribute to open-source projects, and engage with the community.
6. Learn Advanced Git Concepts
As you become comfortable with the basics, explore more advanced Git concepts:
- Rebasing
- Cherry-picking
- Interactive rebase
- Git hooks
- Git submodules
Common Git Challenges for Junior Developers
As you start using Git, you might encounter some challenges. Here are some common ones and how to address them:
1. Merge Conflicts
Merge conflicts occur when Git can’t automatically merge changes. To resolve:
- Open the conflicting file(s) in your editor.
- Look for conflict markers (<<<<<<<, =======, >>>>>>>).
- Manually edit the file to resolve the conflict.
- Stage the resolved files with
git add
. - Complete the merge with
git commit
.
2. Undoing Mistakes
If you make a mistake, Git offers several ways to undo changes:
- Use
git reset
to undo commits. - Use
git revert
to create a new commit that undoes previous changes. - Use
git checkout -- <file>
to discard changes in a file.
3. Understanding Git’s Staging Area
The staging area (or index) is a unique feature of Git that can be confusing at first. Remember:
git add
stages changes for commit.git commit
commits only staged changes.- Use
git status
to see which changes are staged and which aren’t.
4. Branching and Merging
Branching is powerful but can be complex. Some tips:
- Use descriptive branch names.
- Regularly merge or rebase your feature branches with the main branch to avoid large, complex merges.
- Delete branches after they’re merged to keep your repository clean.
Git Best Practices for Junior Developers
As you learn Git, it’s important to develop good habits. Here are some best practices to follow:
1. Commit Often
Make small, frequent commits rather than large, infrequent ones. This makes it easier to track changes and revert if necessary.
2. Write Clear Commit Messages
Your commit messages should clearly describe what changes were made and why. A good format is:
Short (50 chars or less) summary of changes
More detailed explanatory text, if necessary. Wrap it to about 72
characters or so. In some contexts, the first line is treated as the
subject of an email and the rest of the text as the body.
- Bullet points are okay, too
- Typically a hyphen or asterisk is used for the bullet, preceded
by a single space, with blank lines in between, but conventions
vary here
3. Use Branches
Create a new branch for each feature or bug fix. This keeps your main branch clean and makes it easier to manage different aspects of your project.
4. Pull Before You Push
Always pull the latest changes from the remote repository before pushing your own changes. This helps avoid conflicts.
5. Review Your Changes Before Committing
Use git diff
to review your changes before staging them, and git diff --staged
to review staged changes before committing.
6. Use .gitignore
Create a .gitignore file to specify which files or directories Git should ignore. This typically includes:
- Build artifacts
- Dependency directories (like node_modules)
- IDE-specific files
- Sensitive information (like API keys)
Git and Its Role in Modern Development Workflows
Understanding Git is crucial because it plays a central role in modern development workflows:
1. Continuous Integration/Continuous Deployment (CI/CD)
Git integrates seamlessly with CI/CD pipelines. When you push changes to a Git repository, it can automatically trigger:
- Building of the application
- Running of automated tests
- Deployment to staging or production environments
2. Code Review Processes
Git platforms facilitate code reviews through pull requests or merge requests. This process typically involves:
- Creating a feature branch
- Making changes and committing them
- Opening a pull request
- Reviewers commenting on the changes
- Making necessary revisions
- Merging the changes into the main branch
3. DevOps Practices
Git is a key tool in DevOps practices, enabling:
- Infrastructure as Code (IaC)
- Configuration management
- Automated testing and deployment
4. Agile Development
Git supports Agile development methodologies by enabling:
- Rapid iteration
- Feature branching
- Continuous delivery
Conclusion
As a junior developer, learning Git is not just about mastering another tool—it’s about adopting a mindset that aligns with professional software development practices. Git will not only help you manage your code more effectively but also prepare you for collaborative work, enhance your problem-solving skills, and make you more attractive to potential employers.
Remember, becoming proficient with Git is a journey. Start with the basics, practice regularly, and gradually explore more advanced features. Don’t be afraid to make mistakes—Git’s design allows you to experiment and learn safely. As you grow more comfortable with Git, you’ll find it an indispensable tool in your development toolkit.
Embrace Git early in your career, and you’ll be setting yourself up for success in the dynamic and collaborative world of software development. Happy coding, and may your commits always be clear and your merges conflict-free!