Why You Should Embrace the ‘I Don’t Know’ Moment in Coding
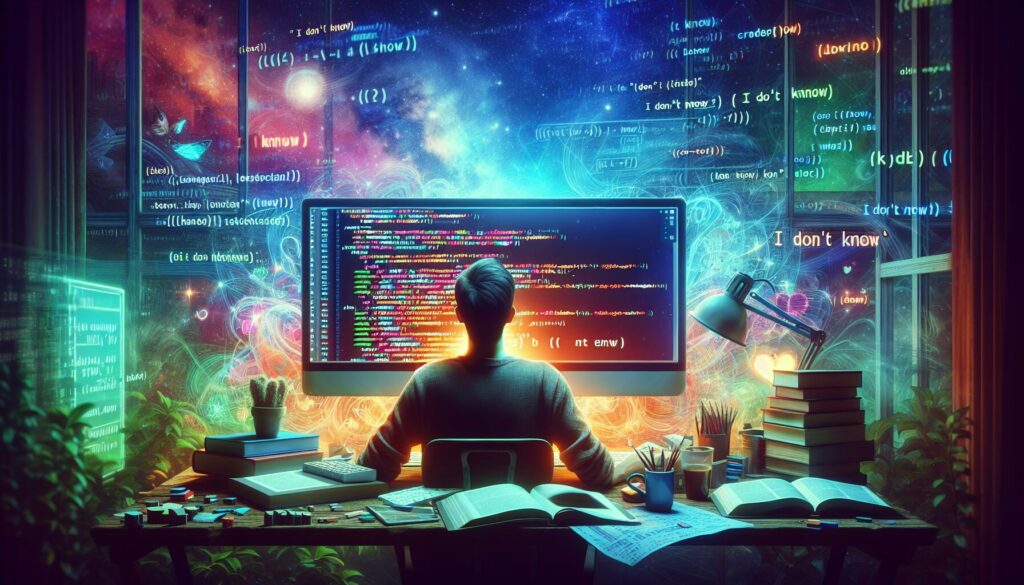
In the world of coding and software development, there’s a pervasive myth that programmers should know everything. This belief can lead to imposter syndrome, stress, and a reluctance to admit when we don’t understand something. However, embracing the “I don’t know” moment is not only natural but essential for growth and success in the field. In this article, we’ll explore why acknowledging your knowledge gaps is crucial and how it can make you a better programmer.
The Power of Admitting What You Don’t Know
Coding is a vast and ever-evolving field. With new languages, frameworks, and technologies emerging constantly, it’s impossible for anyone to know everything. Recognizing and admitting what you don’t know is the first step towards learning and improvement.
1. It Opens the Door to Learning
When you admit that you don’t know something, you create an opportunity to learn. This mindset shift from “I should know this” to “I get to learn this” can be incredibly empowering. It turns every knowledge gap into an exciting challenge rather than a source of stress or shame.
2. It Builds Trust and Credibility
Contrary to what you might think, admitting that you don’t know something can actually increase your credibility among peers and superiors. It shows honesty and integrity, which are highly valued traits in any professional setting. People are more likely to trust someone who is upfront about their limitations than someone who pretends to know everything.
3. It Encourages Collaboration
When you’re open about what you don’t know, it invites others to share their knowledge. This fosters a collaborative environment where team members can learn from each other. In coding, where complex problems often require diverse perspectives, this kind of collaboration is invaluable.
The ‘I Don’t Know’ Moment in Practice
Let’s look at some practical scenarios where embracing the “I don’t know” moment can be beneficial:
During Code Reviews
Imagine you’re reviewing a colleague’s code, and you come across a design pattern or algorithm you’re not familiar with. Instead of glossing over it or making assumptions, you could say something like:
“I’m not familiar with this pattern. Could you explain how it works and why you chose to use it here?”
This approach not only helps you learn but also gives your colleague a chance to explain their thought process, potentially leading to a valuable discussion about code design.
In Team Meetings
During a team meeting, a new project using a technology you’re not familiar with is proposed. Instead of staying silent or pretending to understand, you could say:
“I’m not familiar with that technology. Could someone give a brief overview of its key features and how it fits into our stack?”
This question can lead to a beneficial discussion for the whole team and ensure everyone is on the same page.
When Debugging
You’re working on a bug, and you’ve hit a wall. Instead of spinning your wheels or making random changes, you could reach out to a colleague:
“I’ve been stuck on this bug for a while, and I’m not sure what’s causing it. Could you take a look and see if you spot something I’m missing?”
This approach can lead to faster problem-solving and provides an opportunity for knowledge sharing.
Strategies for Embracing the ‘I Don’t Know’ Moment
While it’s important to embrace the “I don’t know” moment, it’s equally important to do so in a way that’s productive and professional. Here are some strategies to help you navigate these situations:
1. Be Specific About What You Don’t Know
Instead of saying “I don’t know how to do this,” try to pinpoint the specific aspect you’re unsure about. For example:
“I understand the overall structure of the function, but I’m not clear on how this particular method is being used in this context.”
This approach shows that you’ve made an effort to understand and helps others provide more targeted explanations.
2. Show Your Thought Process
Even if you don’t know the answer, sharing your thought process can be valuable. It shows that you’re actively engaging with the problem. For instance:
“I’m not sure how to optimize this query, but I’ve considered indexing these columns. I’m wondering if that would be sufficient or if we need a more complex solution.”
3. Follow Up with a Plan to Learn
After admitting you don’t know something, follow up with a plan to address that knowledge gap. This shows initiative and a commitment to growth. For example:
“I’m not familiar with Docker, but I’ll spend some time this week going through the official documentation and trying out some basic examples. Would it be okay if I come to you with any questions?”
4. Use It as an Opportunity to Learn from Others
When you admit you don’t know something, use it as an opportunity to learn from those around you. Ask questions, seek explanations, and be open to guidance. This not only helps you learn but also strengthens your relationships with colleagues.
The Role of ‘I Don’t Know’ in Problem-Solving
In coding, problem-solving is a crucial skill, and the “I don’t know” moment plays a significant role in this process. Here’s how:
1. It Helps Define the Problem
Often, admitting what you don’t know helps clarify the actual problem you’re facing. It forces you to articulate your confusion, which is the first step in problem-solving.
2. It Encourages Research
When you acknowledge a gap in your knowledge, it naturally leads to research. This could involve reading documentation, searching Stack Overflow, or diving into academic papers. This research often leads to a deeper understanding of the problem and potential solutions.
3. It Promotes Creative Thinking
When you’re honest about not knowing something, you’re more likely to approach the problem with fresh eyes. This can lead to creative solutions that might not have been apparent if you were trying to force a solution based on what you already know.
4. It Facilitates Breaking Down Complex Problems
Complex coding problems often require breaking them down into smaller, more manageable parts. Identifying what you don’t know helps in this process, allowing you to tackle each unknown element separately.
The ‘I Don’t Know’ Moment in Different Coding Scenarios
Let’s explore how the “I don’t know” moment manifests in different coding scenarios and how to handle it:
When Learning a New Programming Language
When diving into a new programming language, it’s natural to encounter many “I don’t know” moments. Here’s an example of how to approach this:
// Python code
def process_data(data):
# I don't know how to use list comprehensions in Python
result = []
for item in data:
if item > 0:
result.append(item * 2)
return result
# TODO: Research Python list comprehensions and refactor this function
In this scenario, you’ve acknowledged what you don’t know (list comprehensions) and left a comment to remind yourself to research and improve the code later.
When Dealing with a New Framework or Library
Frameworks and libraries often have their own conventions and best practices. Here’s how you might handle an “I don’t know” moment:
// React component
import React from 'react';
function MyComponent() {
// I'm not sure if this is the best way to handle state in React
const [count, setCount] = React.useState(0);
return (
<div>
<p>Count: {count}</p>
<button onClick={() => setCount(count + 1)}>Increment</button>
</div>
);
}
// TODO: Research React hooks and state management best practices
Here, you’ve implemented a basic solution while acknowledging that there might be better ways to handle state in React.
When Optimizing Performance
Performance optimization often involves specialized knowledge. Here’s an example of how to approach an “I don’t know” moment in this context:
// JavaScript function
function sortLargeArray(arr) {
// I don't know if this is the most efficient sorting algorithm for large datasets
return arr.sort((a, b) => a - b);
}
// TODO: Research efficient sorting algorithms for large datasets
// Consider implementing quicksort or mergesort
In this case, you’ve implemented a basic solution using the built-in sort method, but you’ve acknowledged that there might be more efficient algorithms for large datasets.
The Learning Journey: From ‘I Don’t Know’ to ‘I Understand’
The journey from “I don’t know” to “I understand” is at the heart of a programmer’s growth. Here’s a typical progression:
1. Acknowledgment
The first step is acknowledging what you don’t know. This could be a specific syntax, a programming concept, or how to use a particular tool.
2. Research
Once you’ve identified what you don’t know, the next step is research. This might involve reading documentation, watching tutorials, or asking questions on forums like Stack Overflow.
3. Experimentation
After gathering information, it’s time to experiment. Try implementing what you’ve learned in small, isolated examples.
4. Application
Once you’re comfortable with the concept in isolation, try applying it to your actual project or problem.
5. Refinement
As you use your new knowledge, you’ll likely encounter edge cases or nuances you didn’t initially understand. This leads to further research and experimentation, refining your understanding.
6. Teaching
One of the best ways to solidify your understanding is to teach others. This could be through writing blog posts, giving presentations, or simply explaining concepts to colleagues.
Let’s look at an example of this journey in action:
// Initial state: I don't know how to use async/await in JavaScript
// Research: Reading MDN documentation on async/await
// Experimentation:
async function fetchData() {
try {
const response = await fetch('https://api.example.com/data');
const data = await response.json();
console.log(data);
} catch (error) {
console.error('Error:', error);
}
}
// Application: Using async/await in a real project
async function getUserData(userId) {
try {
const response = await fetch(`https://api.example.com/users/${userId}`);
const userData = await response.json();
return userData;
} catch (error) {
console.error('Error fetching user data:', error);
return null;
}
}
// Refinement: Handling more complex scenarios
async function fetchMultipleUsers(userIds) {
try {
const userPromises = userIds.map(id => fetch(`https://api.example.com/users/${id}`).then(res => res.json()));
const users = await Promise.all(userPromises);
return users;
} catch (error) {
console.error('Error fetching multiple users:', error);
return [];
}
}
// Teaching: Writing a blog post or giving a presentation on async/await
This journey from “I don’t know” to “I understand” is continuous in a programmer’s career. Each new technology or concept you encounter is an opportunity to go through this process, constantly expanding your knowledge and skills.
The Role of ‘I Don’t Know’ in Technical Interviews
Technical interviews, especially for major tech companies, can be intimidating. It’s tempting to try to know everything, but embracing the “I don’t know” moment can actually be beneficial in these high-pressure situations.
1. It Shows Honesty and Integrity
Interviewers appreciate candidates who are honest about their knowledge gaps. It’s better to admit you don’t know something than to try to bluff your way through an answer.
2. It Demonstrates Problem-Solving Skills
When you encounter a question you can’t immediately answer, use it as an opportunity to showcase your problem-solving skills. Talk through your thought process, ask clarifying questions, and explain how you would go about finding the answer.
3. It Shows Willingness to Learn
Admitting you don’t know something and then explaining how you would learn it demonstrates a growth mindset, which is highly valued in the tech industry.
Example Scenario
Interviewer: “Can you explain how a B-tree differs from a binary search tree?”
Candidate: “I’m familiar with binary search trees, but I’m not entirely sure about the specifics of B-trees. I know they’re both used for efficient data storage and retrieval, but I’d need to refresh my knowledge on the exact differences. In a real-world scenario, I would research this topic, possibly starting with academic resources or reputable programming websites. Could you tell me more about the context in which you’re asking this question? That might help me understand the key aspects I should focus on when learning about B-trees.”
This response acknowledges the knowledge gap, shows willingness to learn, and asks for more context to guide the discussion.
Building a Culture of ‘I Don’t Know’ in Development Teams
Creating an environment where team members feel comfortable saying “I don’t know” can lead to more effective collaboration, faster problem-solving, and continuous learning. Here are some ways to foster this culture:
1. Lead by Example
As a team leader or senior developer, openly admit when you don’t know something. This sets the tone for the rest of the team.
2. Encourage Questions
Create an atmosphere where asking questions is encouraged. This could involve dedicating time in meetings for Q&A or setting up channels for team members to ask for help.
3. Celebrate Learning
Recognize and celebrate when team members learn new things. This could be as simple as sharing interesting findings in team meetings or as structured as regular knowledge-sharing sessions.
4. Pair Programming
Implement pair programming sessions where team members can work together on challenging tasks. This provides a safe environment for admitting knowledge gaps and learning from each other.
5. Code Review Culture
Foster a code review culture where it’s normal to ask questions and seek clarification. This not only improves code quality but also provides learning opportunities for both the reviewer and the author.
Conclusion: Embracing ‘I Don’t Know’ as a Path to Growth
In the dynamic world of coding and software development, embracing the “I don’t know” moment is not a sign of weakness, but a powerful tool for growth and improvement. It opens doors to learning, fosters collaboration, builds trust, and ultimately makes you a better programmer.
Remember, every expert was once a beginner, and every piece of knowledge you now possess was once unknown to you. By acknowledging what you don’t know, you’re taking the first step on a journey of discovery and improvement.
So the next time you encounter something unfamiliar in your coding journey, don’t shy away from it. Embrace the “I don’t know” moment, use it as a springboard for learning, and watch as it propels you towards greater understanding and expertise in your field.
After all, in the words of the ancient philosopher Socrates, “The only true wisdom is in knowing you know nothing.” In the ever-evolving world of technology, this wisdom is more relevant than ever. Happy coding, and happy learning!