Why You Rush to Code Instead of Planning in Interviews (And How to Stop)
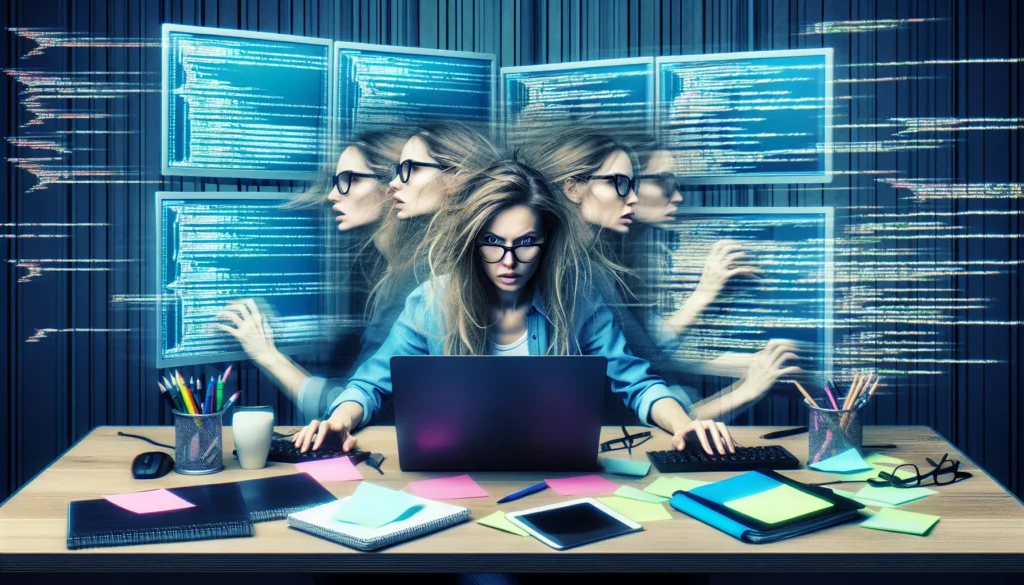
When faced with a coding interview, does this scenario sound familiar? The interviewer presents you with a problem, and before they’ve even finished explaining, your fingers are already itching to start typing. You dive headfirst into coding, convinced that the faster you produce something, the better impression you’ll make.
This rush to code is one of the most common mistakes candidates make during technical interviews. Despite being technically skilled, many developers fall into this trap, potentially sabotaging their chances at landing their dream job.
In this comprehensive guide, we’ll explore why we instinctively rush to code during interviews, the hidden costs of this approach, and most importantly, how to develop a more effective strategy that will impress interviewers at top tech companies.
Why We Rush to Code: Understanding the Psychology
Before we can fix this habit, we need to understand why it happens in the first place. Several psychological factors contribute to our tendency to rush into coding:
The Pressure Cooker Effect
Technical interviews are inherently stressful. With a ticking clock and an evaluator watching your every move, the urge to demonstrate immediate progress becomes overwhelming. This pressure activates our fight-or-flight response, pushing us to take action rather than pause for thoughtful consideration.
A study by the University of Chicago found that under pressure, people tend to focus on speed over accuracy—a phenomenon that perfectly explains the coding interview rush. When we feel we’re being evaluated, our brains prioritize visible activity over methodical reasoning.
The Impostor Syndrome Factor
Many developers, even experienced ones, suffer from impostor syndrome—the persistent feeling that they’re not as competent as others perceive them to be. This insecurity fuels the need to prove oneself quickly, leading to premature coding.
“I always felt like I needed to show I could code quickly to prove I belonged,” shares Michael, a senior developer at a major tech company. “It took bombing several interviews to realize that thoughtful planning impressed interviewers far more than rapid typing.”
Misunderstanding What Interviewers Value
There’s a common misconception that interviewers are primarily evaluating how quickly you can produce code. In reality, they’re much more interested in your problem-solving approach, communication skills, and ability to write maintainable, bug-free code—all of which require planning.
As one Google interviewer put it: “I can teach syntax, but I can’t teach systematic thinking. I’d much rather see a candidate spend 10 minutes planning and 20 minutes coding than 2 minutes planning and 28 minutes debugging.”
The Hidden Costs of Rushing to Code
Jumping straight into coding might feel productive, but it comes with significant drawbacks that can derail your interview performance:
Increased Bug Rate and Debugging Time
Without proper planning, you’re much more likely to introduce bugs and edge cases that you’ll later need to fix. Studies in software development consistently show that bugs caught in the design phase cost 10-100x less to fix than those caught during implementation.
In the context of an interview, this means you’ll spend precious minutes debugging issues that could have been avoided with upfront planning.
Suboptimal Solution Selection
When you rush to implement the first solution that comes to mind, you often miss more efficient alternatives. This is particularly problematic for algorithmic questions where the difference between an O(n²) and O(n log n) solution could be the difference between a pass and a fail.
Consider this common scenario: A candidate immediately implements a brute force solution for a string matching problem, only to realize 20 minutes later that a hash map approach would have been much more efficient. By then, there’s often not enough time to reimplement the solution.
Poor Communication with the Interviewer
Rushing into code means you’re not taking the time to communicate your thought process, which is a critical component of the evaluation. Interviewers want to understand how you approach problems, not just whether you can produce working code.
“Some of my best hires weren’t necessarily the ones who solved the problem perfectly,” says Sarah, a technical hiring manager. “They were the ones who clearly articulated their thinking, considered multiple approaches, and collaborated with me throughout the process.”
Higher Stress and Lower Confidence
The cycle of rushing, encountering bugs, and frantically debugging creates mounting stress during the interview. As your anxiety increases, your cognitive abilities decrease—exactly the opposite of what you need in that moment.
This stress spiral can severely impact your performance on subsequent questions, even if you eventually solve the first one.
The Planning Advantage: What Top Candidates Do Differently
Successful candidates approach technical interviews differently. They understand that planning isn’t just a preliminary step—it’s a competitive advantage that sets them apart.
They Demonstrate Systematic Thinking
Top performers show interviewers that they can break down complex problems into manageable components. This systematic approach signals that they’ll be able to handle ambiguous, large-scale challenges in real-world scenarios.
For example, when faced with a system design question, successful candidates don’t immediately start drawing database schemas. Instead, they first clarify requirements, identify key components, and discuss potential architectural approaches at a high level.
They Catch Edge Cases Early
Planning allows candidates to identify edge cases before writing a single line of code. This proactive approach demonstrates foresight and attention to detail—qualities that are highly valued in professional development environments.
Consider this common interview question: “Write a function to find the longest substring without repeating characters.” A candidate who plans might say: “I need to consider empty strings, strings with all repeating characters, and cases where the longest substring appears multiple times.”
They Build Interviewer Confidence
When you take time to plan and communicate your approach, you give the interviewer confidence that you’re methodical and thoughtful in your work. This impression matters just as much as your technical solution.
“I’m always more impressed by candidates who take a breath and think before coding,” explains David, a senior interviewer at a FAANG company. “It shows maturity and professional judgment—qualities that translate directly to on-the-job performance.”
The Effective Planning Framework for Technical Interviews
Now that we understand why planning matters, let’s explore a concrete framework you can use in your next technical interview:
Step 1: Understand and Clarify (2-3 minutes)
Before writing any code, make sure you fully understand the problem:
- Restate the problem in your own words to confirm understanding
- Ask clarifying questions about inputs, outputs, and constraints
- Discuss any assumptions you’re making
For example, if asked to implement a function that finds all pairs of numbers in an array that sum to a target value, you might clarify:
- “Can the array contain duplicates?”
- “Should I return the indices or the actual values?”
- “Can a number be paired with itself?”
- “What should I return if no pairs are found?”
Step 2: Explore Multiple Approaches (3-5 minutes)
Instead of implementing the first solution that comes to mind, consider multiple approaches:
- Brainstorm at least 2-3 different strategies
- Analyze the time and space complexity of each
- Discuss tradeoffs between different approaches
For our pair-sum example, you might consider:
- A brute force approach using nested loops (O(n²) time, O(1) space)
- A hash map approach (O(n) time, O(n) space)
- A two-pointer approach if the array is sorted (O(n log n) time for sorting, O(1) additional space)
Step 3: Select and Outline Your Approach (2-3 minutes)
Once you’ve explored options, select the most appropriate solution and outline it:
- Explain why you chose this particular approach
- Sketch the high-level algorithm steps
- Identify potential edge cases and how you’ll handle them
For the pair-sum problem, you might outline the hash map approach:
- Initialize an empty hash map and result array
- Iterate through the input array
- For each number, check if (target – number) exists in the hash map
- If it exists, add the pair to the result
- Add the current number to the hash map
- Return the result array
Step 4: Implement with Confidence (10-15 minutes)
Now that you have a clear plan, you can implement your solution with confidence:
- Write clean, well-structured code
- Add comments to explain key steps
- Verbalize your thinking as you code
Here’s how you might implement the pair-sum solution:
function findPairsWithSum(array, targetSum) {
const pairs = [];
const numMap = new Map();
for (let i = 0; i < array.length; i++) {
const current = array[i];
const complement = targetSum - current;
if (numMap.has(complement)) {
pairs.push([complement, current]);
}
numMap.set(current, i);
}
return pairs;
}
Step 5: Test Thoroughly (3-5 minutes)
After implementation, test your solution with various scenarios:
- Start with a simple, typical case
- Test edge cases you identified earlier
- Walk through the code execution step-by-step
For our pair-sum example:
// Test case 1: Typical case
console.log(findPairsWithSum([1, 2, 3, 4, 5], 7)); // Expected: [[2,5], [3,4]]
// Test case 2: No valid pairs
console.log(findPairsWithSum([1, 2, 3], 10)); // Expected: []
// Test case 3: Duplicates
console.log(findPairsWithSum([1, 2, 3, 2, 4], 5)); // Expected: [[1,4], [2,3], [3,2]]
Real-World Examples: Planning vs. Rushing
To illustrate the difference planning makes, let’s compare two approaches to a common interview problem:
Problem: Design a data structure that supports adding new words and finding if a string matches any previously added string or is a prefix of any previously added string.
Candidate A: The Rusher
Immediately jumps into coding a solution using arrays:
class WordDictionary {
constructor() {
this.words = [];
}
addWord(word) {
this.words.push(word);
}
search(prefix) {
for (let word of this.words) {
if (word.startsWith(prefix)) {
return true;
}
}
return false;
}
}
The implementation works for simple cases, but has O(n) search time where n is the number of words. When the interviewer asks about handling millions of words, the candidate struggles to optimize and runs out of time trying to refactor.
Candidate B: The Planner
Takes time to understand the problem and considers different data structures:
“This looks like a prefix matching problem. I could use an array and check each word, but that would be O(n) per search. A more efficient approach would be using a Trie data structure, which is optimized for prefix operations and would give us O(m) search time where m is the length of the prefix.”
Outlines the approach:
- Create a Trie node structure with children and an end-of-word marker
- Implement addWord by inserting characters into the Trie
- Implement search by traversing the Trie based on prefix characters
Then implements:
class TrieNode {
constructor() {
this.children = {};
this.isEndOfWord = false;
}
}
class WordDictionary {
constructor() {
this.root = new TrieNode();
}
addWord(word) {
let node = this.root;
for (let char of word) {
if (!node.children[char]) {
node.children[char] = new TrieNode();
}
node = node.children[char];
}
node.isEndOfWord = true;
}
search(prefix) {
let node = this.root;
for (let char of prefix) {
if (!node.children[char]) {
return false;
}
node = node.children[char];
}
return true;
}
// Additional method to check if a complete word exists
exactWordExists(word) {
let node = this.root;
for (let char of word) {
if (!node.children[char]) {
return false;
}
node = node.children[char];
}
return node.isEndOfWord;
}
}
The result? Candidate B not only implements a more efficient solution but also demonstrates knowledge of appropriate data structures and algorithmic thinking. The interviewer is impressed by both the technical solution and the methodical approach.
Overcoming the Urge to Rush: Practical Techniques
Even with a clear understanding of why planning matters, fighting the urge to rush can be difficult. Here are practical techniques to help you stay disciplined:
The Explicit Pause Technique
When presented with a problem, explicitly tell the interviewer that you’d like to take a moment to think. This sets expectations and gives you permission to pause:
“That’s an interesting problem. I’d like to take a minute to think about it before diving into code. Is that okay?”
Most interviewers will not only agree but will appreciate your thoughtful approach.
The Notepad Method
Having a physical or digital notepad ready for planning can create a tangible reminder to think before coding. Start by writing down:
- The problem statement
- Input/output examples
- Possible approaches
- Time/space complexity analysis
This visual aid helps organize your thoughts and demonstrates your systematic approach to the interviewer.
The Time Box Strategy
Allocate specific time limits for each phase of your solution:
- Understanding: 2-3 minutes
- Planning: 3-5 minutes
- Coding: 10-15 minutes
- Testing: 3-5 minutes
You can even share this plan with your interviewer: “I’m going to spend about 5 minutes planning my approach before I start coding.” This creates accountability and helps manage both your time and the interviewer’s expectations.
The Interviewer Partnership Approach
Treat the interviewer as a collaborator in your problem-solving process:
“I’m thinking about two approaches here: a hash map solution with O(n) time complexity or a two-pointer approach with O(n log n). Would you like me to explore the tradeoffs between these?”
This engagement demonstrates communication skills and gives the interviewer insight into your thought process.
Planning in Different Types of Technical Interviews
The planning approach needs slight modifications depending on the type of interview you’re facing:
Algorithmic Problem Solving
For classic algorithm questions (e.g., array manipulation, string parsing), focus your planning on:
- Identifying the underlying pattern (e.g., two pointers, sliding window, dynamic programming)
- Time and space complexity analysis
- Edge cases and corner conditions
System Design Interviews
For system design questions, planning is even more critical:
- Start by clarifying requirements and constraints
- Establish scale (users, data volume, traffic patterns)
- Sketch the high-level architecture before diving into components
- Discuss tradeoffs explicitly (consistency vs. availability, latency vs. throughput)
Object-Oriented Design
When designing classes and objects:
- Begin with use cases and requirements
- Identify the key entities and their relationships
- Consider patterns that might apply (factory, singleton, observer)
- Sketch class diagrams before implementing any code
Coding Assignments and Take-Home Challenges
Even without the time pressure of a live interview, planning remains valuable:
- Document your thought process in comments or a README
- Create a design doc before implementation
- Set up a testing strategy in advance
Common Planning Pitfalls and How to Avoid Them
Even with the best intentions, planning can go awry. Here are common pitfalls and how to avoid them:
Analysis Paralysis
The Pitfall: Getting stuck in endless planning without making progress toward a solution.
The Solution: Set a time limit for your planning phase. If you’re still uncertain after 5 minutes, pick the most promising approach and start implementing, communicating your thought process along the way.
Over-Engineering
The Pitfall: Designing an unnecessarily complex solution when a simpler one would suffice.
The Solution: Start with the simplest solution that correctly handles the problem, then optimize if needed. Explicitly discuss the tradeoff between simplicity and performance with your interviewer.
Silent Planning
The Pitfall: Planning in your head without communicating your thoughts to the interviewer.
The Solution: Verbalize your thinking process, even during planning stages. This gives the interviewer insight into your approach and allows them to provide guidance if needed.
Inflexible Planning
The Pitfall: Becoming so attached to your initial plan that you can’t adapt when problems arise.
The Solution: Be prepared to pivot if your approach isn’t working. When you encounter an issue, explicitly acknowledge it and consider alternatives rather than forcing your original solution.
Building the Planning Habit: Practice Strategies
Like any skill, effective planning requires practice. Here are strategies to build this habit:
The Mock Interview Routine
Regular mock interviews with explicit focus on your planning process can help make it second nature:
- Ask a friend to observe and provide feedback specifically on your planning approach
- Record your mock interviews to review your planning-to-coding ratio
- Use platforms like Pramp or interviewing.io for structured practice
The Planning Journal
Keep a journal of practice problems where you document:
- Your initial thoughts and approaches
- The plan you developed
- How the implementation matched or deviated from the plan
- What you would change about your planning process next time
The Five-Minute Rule
For every practice problem, force yourself to spend at least five minutes planning before writing any code. This artificial constraint helps build the planning muscle.
The Planning Buddy System
Partner with another job seeker and review each other’s planning approaches. External accountability can help reinforce good habits.
Conclusion: The Paradox of Slowing Down to Speed Up
The urge to rush into coding during interviews is natural but counterproductive. By investing time in planning, you actually save time overall, produce higher quality solutions, and demonstrate the kind of methodical thinking that employers value.
Remember the paradox: slowing down at the beginning allows you to speed up overall. The few minutes you spend planning will pay dividends in reduced debugging time, more optimal solutions, and clearer communication with your interviewer.
As you prepare for your next technical interview, commit to fighting the rush. Embrace planning as not just a preliminary step, but as a core component of your problem-solving approach. With practice, this methodical approach will become second nature, setting you apart from candidates who rush to code and setting you up for success in even the most challenging technical interviews.
The next time an interviewer presents you with a problem, take a deep breath, smile, and say: “Let me think about this for a moment before I start coding.” That single sentence might be the most powerful tool in your interview arsenal.
Additional Resources
- “Cracking the Coding Interview” by Gayle Laakmann McDowell
- “System Design Interview” by Alex Xu
- Interactive practice platforms like LeetCode, HackerRank, and AlgoCademy
- Mock interview services like interviewing.io and Pramp
Remember, the goal isn’t just to get the job—it’s to develop problem-solving habits that will serve you throughout your career. Happy interviewing!