Why You Miss Obvious Solutions When Interviewing: Understanding Interview Blind Spots
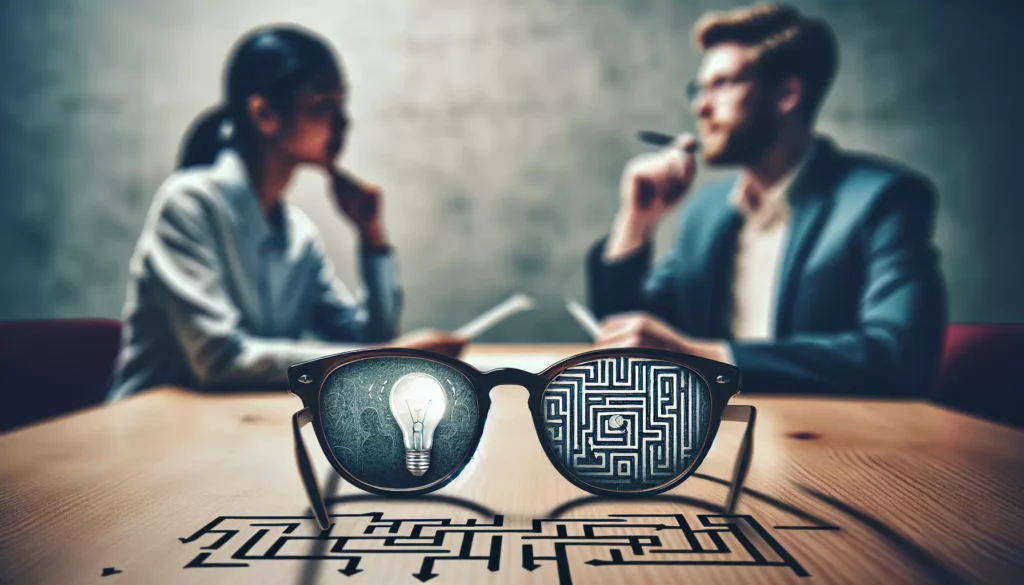
Have you ever walked out of a technical interview thinking, “How did I miss that solution? It was right in front of me!” You’re not alone. Even experienced developers frequently overlook seemingly obvious solutions during high-pressure interviews. This phenomenon is so common that it deserves a deeper look.
In this article, we’ll explore why our brains sometimes fail to see straightforward solutions during coding interviews, and more importantly, what you can do to overcome these blind spots.
The Paradox of Interview Performance
Technical interviews create a unique paradox: they test skills you use daily as a developer, yet they create an environment that makes accessing those skills unusually difficult. Let’s break down why this happens.
The Pressure Cooker Effect
When you’re solving a problem during your normal workday, you have time to think, research, and iterate. In an interview, you’re working under intense scrutiny with a ticking clock. This pressure activates your body’s stress response, which can significantly impair cognitive function.
Research in cognitive psychology shows that stress triggers the release of cortisol, which directly impacts the prefrontal cortex—the area responsible for complex problem-solving and logical thinking. Essentially, the very skills you need most in a coding interview can be compromised by the interview environment itself.
The Overthinking Trap
Many candidates miss simple solutions because they immediately assume interview problems must require complex algorithms or data structures. This is what psychologists call “functional fixedness”—getting stuck on a particular approach and failing to see simpler alternatives.
For example, a candidate might immediately try to implement a complex dynamic programming solution when a simple hash map would suffice. This tendency to overcomplicate is particularly common among candidates who have extensively practiced advanced algorithms.
Common Blind Spots in Technical Interviews
Understanding the specific ways our thinking can go astray helps us guard against these pitfalls. Here are the most common blind spots candidates experience:
1. Missing the Brute Force Approach
Sometimes, candidates jump straight to optimizing before they’ve even solved the problem. Remember that a working solution, even if inefficient, is better than no solution at all.
Consider this scenario: You’re asked to find pairs in an array that sum to a target value. You might immediately try to devise an O(n) solution using a hash map, but get stuck in the implementation details. Meanwhile, you could have at least started with the O(n²) nested loop approach:
function findPairs(arr, target) {
const result = [];
for (let i = 0; i < arr.length; i++) {
for (let j = i + 1; j < arr.length; j++) {
if (arr[i] + arr[j] === target) {
result.push([arr[i], arr[j]]);
}
}
}
return result;
}
This gives you a working solution that you can then optimize if time permits.
2. Overlooking Built-in Functions
Many candidates reinvent the wheel when language-provided functions would solve part of their problem. This is especially common with sorting, searching, and string manipulation functions.
For instance, instead of writing your own sorting algorithm, in most interview contexts it’s perfectly acceptable (and often preferred) to use the language’s built-in sort:
// Instead of implementing bubble sort, merge sort, etc.
const sortedArray = array.sort((a, b) => a - b);
3. Failing to Consider Edge Cases
In the rush to solve the core problem, many candidates miss obvious edge cases like empty inputs, negative numbers, or boundary conditions. These are the “obvious” checks that experienced developers routinely make, but interview pressure can cause us to overlook them.
For example, when writing a function to find the minimum value in an array:
function findMinimum(arr) {
// Edge case: empty array
if (arr.length === 0) {
throw new Error("Cannot find minimum of empty array");
}
let min = arr[0];
for (let i = 1; i < arr.length; i++) {
if (arr[i] < min) {
min = arr[i];
}
}
return min;
}
Missing the empty array check would be a simple oversight that could cost you points in an interview.
4. Tunnel Vision on the First Approach
Once we start implementing a particular solution, we tend to become committed to it, even if it’s not working well. This “sunk cost fallacy” can prevent us from stepping back and considering alternative approaches.
For instance, if you begin solving a string manipulation problem with an elaborate set of nested loops and conditional statements, you might persist with this approach even when it becomes unwieldy—instead of stepping back and considering whether a regular expression or different data structure might simplify things.
The Psychology Behind Missing Obvious Solutions
To effectively combat these blind spots, it helps to understand the psychological mechanisms that create them.
Cognitive Load Theory
According to cognitive load theory, our working memory has limited capacity. During an interview, this capacity is taxed by multiple demands:
- Parsing and understanding the problem
- Recalling relevant algorithms and data structures
- Translating your thoughts into code
- Keeping track of variables and logic
- Managing the social interaction with the interviewer
- Monitoring the time
When your working memory is overloaded, your brain takes shortcuts and narrows focus, often missing the forest for the trees.
The Einstellung Effect
The Einstellung effect describes our tendency to stick with familiar solutions rather than exploring potentially better alternatives. If you’ve practiced solving problems with specific techniques, you’re likely to apply those same techniques even when simpler solutions exist.
For example, if you’ve extensively practiced dynamic programming problems, you might try to apply that approach to a problem that could be solved more simply with a greedy algorithm or even just a hash map.
Performance Anxiety and Impostor Syndrome
Many candidates, especially those interviewing at prestigious companies like FAANG organizations, experience performance anxiety stemming from impostor syndrome. Thoughts like “I don’t belong here” or “They’re going to discover I’m not good enough” consume valuable cognitive resources that could otherwise be used for problem-solving.
This anxiety creates a self-fulfilling prophecy: you perform worse because you’re worried about performing poorly, which then confirms your fears.
Strategies to Overcome Interview Blind Spots
Now that we understand why we miss obvious solutions, let’s explore practical strategies to overcome these challenges.
1. Develop a Systematic Problem-Solving Approach
Having a consistent methodology for approaching problems reduces cognitive load and ensures you don’t skip important steps. Here’s a framework you can follow:
- Understand the problem completely: Restate it in your own words and verify with the interviewer.
- Clarify constraints and edge cases: Ask about input sizes, edge cases, and expected output format.
- Explore simple examples: Work through small, concrete examples before coding.
- Consider brute force first: Outline the simplest solution that would work, regardless of efficiency.
- Optimize incrementally: Improve your solution step by step, explaining your thinking.
- Test your solution: Trace through your code with an example before declaring you’re done.
This structured approach ensures you don’t miss obvious steps and gives your brain a familiar pattern to follow even under pressure.
2. Talk Through Your Thinking
Verbalizing your thought process serves multiple purposes:
- It forces you to clarify your own thinking
- It helps the interviewer follow your reasoning and potentially guide you
- It slows you down (in a good way) to prevent rushing into suboptimal solutions
For example, instead of silently coding a solution, say something like: “I’m thinking about using a hash map to store the elements I’ve seen so far, which would give me O(n) time complexity. Before I implement that, let me think if there’s an even simpler approach…”
3. Practice Under Interview Conditions
One of the most effective ways to overcome interview blind spots is to simulate interview conditions during practice:
- Set a timer for 30-45 minutes per problem
- Practice explaining your solutions out loud
- Have a friend or mentor act as an interviewer
- Use platforms like AlgoCademy that simulate real interview environments
The more familiar you become with the pressure of interviews, the less it will impact your cognitive function during the real thing.
4. Develop a Mental Checklist for Solution Validation
Before submitting your final answer, run through a mental checklist:
- Have I handled all edge cases (empty inputs, single elements, etc.)?
- Is there a simpler or more efficient solution I’m overlooking?
- Am I using the appropriate built-in functions?
- Does my solution actually solve the problem as stated?
- What’s the time and space complexity, and is it acceptable?
This final check can catch many of the obvious oversights that candidates typically make.
Specific Techniques for Common Problem Types
Different types of problems have their own common “obvious” solutions that candidates often miss. Let’s examine some of these by category:
Array and String Problems
For array and string manipulation problems, these approaches are often overlooked:
Two-Pointer Technique
Many array problems can be solved efficiently using two pointers. For example, to check if an array has a pair that sums to a target value (assuming the array is sorted):
function hasPairWithSum(arr, target) {
let left = 0;
let right = arr.length - 1;
while (left < right) {
const sum = arr[left] + arr[right];
if (sum === target) {
return true;
} else if (sum < target) {
left++;
} else {
right--;
}
}
return false;
}
This O(n) solution is much more efficient than the nested loops approach, yet many candidates default to the latter.
Hash Maps for Lookup
Using a hash map (or object in JavaScript) for O(1) lookups is another commonly overlooked technique:
function findPairWithSum(arr, target) {
const seen = {};
for (let i = 0; i < arr.length; i++) {
const complement = target - arr[i];
if (seen[complement] !== undefined) {
return [seen[complement], i];
}
seen[arr[i]] = i;
}
return null;
}
This transforms what would be an O(n²) problem into an O(n) solution.
Tree and Graph Problems
When working with trees and graphs, candidates often miss these straightforward approaches:
Standard Traversal Algorithms
Many tree problems can be solved with standard traversal algorithms (in-order, pre-order, post-order for trees; BFS or DFS for graphs). For example, to find the maximum depth of a binary tree:
function maxDepth(root) {
if (!root) return 0;
return 1 + Math.max(maxDepth(root.left), maxDepth(root.right));
}
This recursive DFS approach is elegant and efficient, but candidates sometimes try to implement more complex solutions involving queues or custom data structures.
Iterative Solutions with Stacks/Queues
For candidates who struggle with recursion, most tree/graph traversals can be implemented iteratively using a stack (for DFS) or queue (for BFS):
function bfsTraversal(root) {
if (!root) return [];
const result = [];
const queue = [root];
while (queue.length) {
const node = queue.shift();
result.push(node.val);
if (node.left) queue.push(node.left);
if (node.right) queue.push(node.right);
}
return result;
}
Many candidates fixate on recursive solutions when iterative approaches might be more comfortable for them.
Dynamic Programming Problems
Dynamic programming questions are notorious for causing candidates to miss simple solutions:
Memoization
Adding memoization to a recursive solution can transform an exponential-time algorithm into a linear or polynomial one:
function fibonacci(n, memo = {}) {
if (n in memo) return memo[n];
if (n <= 1) return n;
memo[n] = fibonacci(n - 1, memo) + fibonacci(n - 2, memo);
return memo[n];
}
This simple addition makes the function run in O(n) time instead of O(2ⁿ).
Bottom-Up Iteration
Many recursive DP solutions can be rewritten iteratively to avoid stack overflow and improve performance:
function fibonacciIterative(n) {
if (n <= 1) return n;
let prev = 0;
let curr = 1;
for (let i = 2; i <= n; i++) {
const next = prev + curr;
prev = curr;
curr = next;
}
return curr;
}
This approach is not only faster but also uses constant space, which is often overlooked by candidates focused on recursive solutions.
Real-World Examples: Before and After
Let’s look at some real-world examples of how candidates miss obvious solutions, and how they could approach these problems more effectively.
Example 1: Finding a Palindrome
Problem: Determine if a string is a palindrome (reads the same backward as forward).
Suboptimal Approach:
function isPalindrome(str) {
// Create a reversed string
let reversed = '';
for (let i = str.length - 1; i >= 0; i--) {
reversed += str[i];
}
// Compare with original
return str === reversed;
}
This solution works but creates an unnecessary copy of the string.
Obvious Solution Missed:
function isPalindrome(str) {
let left = 0;
let right = str.length - 1;
while (left < right) {
if (str[left] !== str[right]) {
return false;
}
left++;
right--;
}
return true;
}
This two-pointer approach is more efficient as it uses O(1) space instead of O(n).
Example 2: Finding the Maximum Subarray Sum
Problem: Find the contiguous subarray with the largest sum.
Suboptimal Approach:
function maxSubarraySum(nums) {
let maxSum = Number.NEGATIVE_INFINITY;
for (let i = 0; i < nums.length; i++) {
for (let j = i; j < nums.length; j++) {
let currentSum = 0;
for (let k = i; k <= j; k++) {
currentSum += nums[k];
}
maxSum = Math.max(maxSum, currentSum);
}
}
return maxSum;
}
This O(n³) solution is unnecessarily complex.
Obvious Solution Missed:
function maxSubarraySum(nums) {
if (nums.length === 0) return 0;
let maxSoFar = nums[0];
let maxEndingHere = nums[0];
for (let i = 1; i < nums.length; i++) {
// Either extend the previous subarray or start a new one
maxEndingHere = Math.max(nums[i], maxEndingHere + nums[i]);
maxSoFar = Math.max(maxSoFar, maxEndingHere);
}
return maxSoFar;
}
This is Kadane’s algorithm, which solves the problem in O(n) time and O(1) space.
Example 3: Finding Duplicate Elements
Problem: Find any duplicate in an array of integers where values are between 1 and n (array length).
Suboptimal Approach:
function findDuplicate(nums) {
nums.sort((a, b) => a - b);
for (let i = 1; i < nums.length; i++) {
if (nums[i] === nums[i - 1]) {
return nums[i];
}
}
return -1;
}
This solution modifies the input array and has O(n log n) time complexity due to sorting.
Obvious Solution Missed:
function findDuplicate(nums) {
const seen = new Set();
for (const num of nums) {
if (seen.has(num)) {
return num;
}
seen.add(num);
}
return -1;
}
This hash set approach is O(n) time and doesn’t modify the input array.
Applying These Insights to Your Interview Preparation
Now that we’ve identified common blind spots and strategies to overcome them, here’s how to incorporate these insights into your interview preparation:
1. Review Your Past Solutions
Go back to problems you’ve solved and ask yourself:
- Is there a simpler way to solve this?
- Did I handle all edge cases?
- Could I use built-in functions instead of custom implementations?
- What’s the most elegant solution to this problem?
This retrospective analysis helps train your brain to recognize patterns and simplifications.
2. Practice Explaining Your Solutions
Get in the habit of explaining your solutions out loud, even when practicing alone. This verbal component is crucial for:
- Clarifying your own thinking
- Identifying logical gaps
- Building communication skills that interviewers value
Record yourself if possible, and listen for areas where your explanation is unclear or where you might be overcomplicating things.
3. Build a Personal Checklist
Based on your personal tendencies and blind spots, create a checklist to review before submitting any solution:
- Have I considered all edge cases?
- Is my solution unnecessarily complex?
- Am I using appropriate data structures?
- Have I optimized for the right constraints (time vs. space)?
- Does my solution actually solve the problem as stated?
Tailor this checklist based on your own common mistakes.
4. Use Platforms with Solution Comparisons
Practice on platforms that show you multiple solutions after you submit yours. AlgoCademy and similar platforms often provide multiple approaches to the same problem, ranging from brute force to optimal solutions. This helps you recognize patterns and simpler approaches you might have missed.
5. Implement Stress Management Techniques
Since stress is a major factor in missing obvious solutions, incorporate stress management into your preparation:
- Deep breathing: Practice 4-7-8 breathing (inhale for 4 seconds, hold for 7, exhale for 8) before and during interviews.
- Positive visualization: Imagine yourself calmly solving problems in the interview.
- Physical preparation: Ensure you’re well-rested, hydrated, and comfortable before interviews.
These techniques help reduce the cognitive impact of stress, leaving more mental resources available for problem-solving.
The Bigger Picture: What Interviewers Actually Want
Remember that interviewers aren’t just looking for candidates who can produce optimal solutions immediately. They’re evaluating:
- Problem-solving process: How you approach and break down problems
- Communication skills: How clearly you explain your thinking
- Adaptability: How you respond to hints and feedback
- Coding proficiency: How cleanly and correctly you implement solutions
An interview where you initially miss the optimal solution but reason your way to it through good communication and systematic thinking can still be a successful interview.
What to Do When You Realize You’ve Missed Something Obvious
If you realize during an interview that you’ve overlooked a simpler solution, don’t panic. Instead:
- Acknowledge it openly: “I just realized there’s a simpler approach we could take here.”
- Explain your new insight: “Instead of using a nested loop, we could use a hash map to achieve O(n) time complexity.”
- Ask if you should implement the new approach: “Would you like me to implement this more efficient solution instead?”
This demonstrates self-awareness and the ability to iteratively improve solutions—both valuable traits in a developer.
Conclusion: Turning Blind Spots into Strengths
Missing obvious solutions during interviews is a common experience, even for talented developers. The combination of stress, time pressure, and the desire to impress creates a perfect storm that can cloud our thinking and lead us to overlook simpler approaches.
By understanding the psychological mechanisms behind these blind spots and implementing structured approaches to problem-solving, you can significantly reduce their impact. Remember:
- Follow a systematic problem-solving methodology
- Talk through your thinking process
- Practice under simulated interview conditions
- Develop a mental checklist for solution validation
- Learn the common patterns and techniques for different problem types
With deliberate practice and these strategies in mind, you can transform interview blind spots from weaknesses into opportunities to demonstrate your problem-solving process and adaptability.
The next time you’re in an interview and that little voice says, “Am I missing something obvious here?”—listen to it. Take a moment to step back, reassess the problem, and consider whether there’s a simpler path forward. That moment of reflection might be the difference between missing the obvious and nailing the interview.
Happy coding, and best of luck with your interviews!