Why You Miss Important Details in Problem Statements and How to Fix It
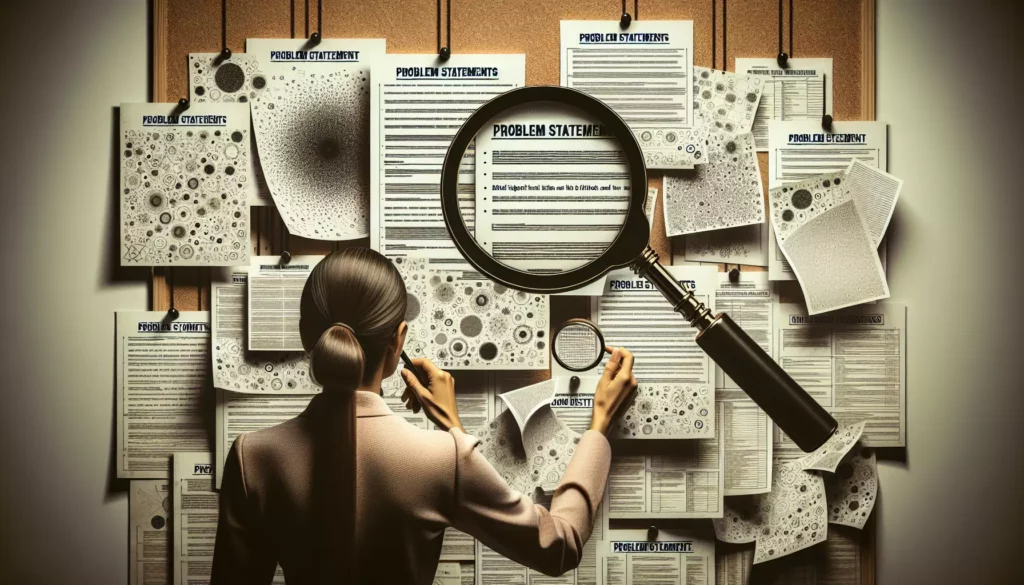
In the world of programming and technical interviews, one skill stands above all others: the ability to carefully read and understand problem statements. Whether you’re working through a coding challenge on AlgoCademy, preparing for a technical interview at a FAANG company, or simply trying to build a personal project, missing critical details in requirements can lead to hours of wasted effort and frustration.
Many programmers, both beginners and experienced, fall into the trap of rushing through problem statements, eager to start coding. This habit can be particularly costly during technical interviews where time is limited and precision is essential.
Table of Contents
- Why We Miss Important Details
- The Consequences of Missing Details
- Cognitive Biases That Affect Problem Understanding
- Techniques to Improve Problem Statement Analysis
- Special Considerations for Technical Interviews
- Real World Examples of Missed Details
- Methods to Practice Detail Orientation
- Tools and Frameworks to Help Catch Missing Details
- Conclusion
Why We Miss Important Details
Before we can solve this problem, we need to understand the root causes. Here are the most common reasons why programmers miss critical details:
1. Eagerness to Start Coding
Many programmers experience an adrenaline rush when presented with a new problem. There’s an inherent excitement in tackling challenges, which can lead to premature coding. This eagerness often manifests as skimming through problem statements rather than thoroughly analyzing them.
Consider this scenario: You’re given a problem about finding the maximum sum subarray. You quickly identify it as the classic Kadane’s algorithm problem and start implementing it. However, you miss the detail that the problem requires you to return the start and end indices of the subarray, not just the sum.
2. Pattern Recognition Overshadowing Unique Requirements
Experienced programmers develop a mental library of common problem patterns. While this is generally beneficial, it can lead to assumptions about a problem’s requirements based on similar problems encountered in the past.
For example, if you’ve solved numerous binary search tree problems, you might automatically assume a new tree problem involves a binary search tree when it actually involves a general binary tree or even an n-ary tree.
3. Cognitive Overload
Complex problem statements with multiple constraints, edge cases, and requirements can overwhelm our working memory. When our cognitive resources are stretched thin, we tend to focus on the most obvious aspects of a problem while overlooking subtle but critical details.
This is particularly common in algorithm problems with multiple constraints like “the array contains only positive integers” or “the graph is guaranteed to be acyclic.”
4. Time Pressure
In timed environments like coding interviews or competitive programming contests, the pressure to produce a solution quickly can lead to hasty reading and incomplete understanding of the problem statement.
Studies have shown that time pressure significantly reduces attention to detail and increases the likelihood of missing important information.
5. Language and Communication Barriers
Problem statements may sometimes be unclear, ambiguous, or written in technical jargon that is unfamiliar. Non-native English speakers may face additional challenges in fully comprehending nuanced requirements.
The Consequences of Missing Details
Missing important details in problem statements can have serious consequences:
1. Wasted Development Time
The most immediate consequence is wasted time. Implementing a solution based on a misunderstood problem often leads to code that doesn’t address the actual requirements. This necessitates significant rework, which could have been avoided with a more careful initial reading.
2. Failed Technical Interviews
In a technical interview setting, missing crucial details can be the difference between receiving a job offer and being rejected. Interviewers are not just evaluating your coding ability but also your attention to detail and problem-solving approach.
3. Production Bugs and Issues
In professional settings, misunderstanding requirements can lead to implementing features that don’t meet user needs or introducing bugs that affect system reliability. These issues often require more effort to fix than would have been needed to understand the requirements correctly in the first place.
4. Decreased Confidence
Repeatedly missing details can lead to decreased confidence in your abilities. This negative feedback loop can make you more anxious about future problems, potentially exacerbating the issue.
Cognitive Biases That Affect Problem Understanding
Several cognitive biases can affect how we process and understand problem statements:
1. Confirmation Bias
We tend to focus on information that confirms our preexisting beliefs or assumptions. If you believe a problem is similar to one you’ve solved before, you might unconsciously look for evidence that supports this belief while ignoring contradicting details.
2. Anchoring Bias
The first piece of information we encounter tends to have a disproportionate influence on our understanding. If the first sentence of a problem statement mentions sorting, you might anchor your entire understanding around sorting algorithms, even if the problem ultimately requires a different approach.
3. The Curse of Knowledge
Once we know something, it’s difficult to imagine not knowing it. Experienced programmers may fill in gaps in problem statements with their existing knowledge, sometimes incorrectly.
4. Functional Fixedness
This bias limits us to thinking about objects or concepts only in terms of their typical use. In programming, this might manifest as an inability to see alternative applications of a data structure or algorithm beyond its most common use case.
Techniques to Improve Problem Statement Analysis
Now that we understand why we miss details, let’s explore techniques to improve our problem statement analysis:
1. The REREAD Method
Develop a habit of reading problem statements at least three times:
- First read: Get a general overview of what the problem is asking.
- Second read: Identify specific requirements, constraints, and edge cases.
- Third read: Look for subtle details that might affect your solution approach.
2. Active Reading Techniques
Engage with the problem statement actively rather than passively:
- Highlight or underline key information as you read.
- Rewrite the problem in your own words to ensure comprehension.
- Create a checklist of requirements that your solution must satisfy.
- Draw diagrams or visualizations to represent the problem.
3. The Five Ws and How Framework
Apply the journalistic questions to problem statements:
- What: What exactly is the problem asking for?
- Why: Why is this problem important or relevant?
- Who: Who are the stakeholders or users of this solution?
- When: Are there any time constraints or performance requirements?
- Where: In what context or environment will this solution operate?
- How: How will the solution be evaluated or tested?
4. Explicit Constraint Identification
Create a separate list of all constraints mentioned in the problem:
- Input ranges and types
- Time and space complexity requirements
- Edge cases to handle
- Assumptions you’re allowed to make
5. Example Analysis
Most problem statements include examples. Don’t just glance at them; analyze them thoroughly:
- Trace through each example manually
- Understand why the expected output is correct
- Create additional examples, especially edge cases
- Use examples to verify your understanding of the problem
6. The “Explain It To Someone Else” Technique
One of the most effective ways to ensure you understand a problem is to explain it to someone else, even if that “someone else” is imaginary. This technique, often called the “rubber duck debugging” method in the context of troubleshooting, works equally well for problem comprehension.
Try to explain the problem out loud, covering:
- The goal of the problem
- The inputs and expected outputs
- All constraints and special conditions
- How the examples demonstrate the problem
If you struggle to explain any aspect clearly, that’s a signal that you need to revisit the problem statement.
Special Considerations for Technical Interviews
Technical interviews present unique challenges when it comes to understanding problem statements:
1. Clarifying Questions
Unlike self-study situations, interviews give you the opportunity to ask questions. Use this to your advantage:
- Ask about input ranges and types
- Clarify any ambiguous requirements
- Confirm your understanding of the problem
- Discuss potential edge cases
Many interviewers deliberately leave details vague to see if candidates will ask clarifying questions. Asking good questions demonstrates thoroughness and attention to detail.
2. Thinking Aloud
Verbalizing your understanding of the problem serves two purposes:
- It helps you organize your thoughts and catch misunderstandings
- It gives the interviewer a chance to correct any misconceptions early
A good pattern to follow is: “So, as I understand it, the problem is asking me to… Is that correct?”
3. Managing Interview Anxiety
Anxiety can significantly impair your ability to process information carefully. Some strategies to manage interview anxiety include:
- Deep breathing exercises before and during the interview
- Practicing with mock interviews to build familiarity
- Reminding yourself that it’s okay to take your time
- Having a consistent process for approaching problems
Real World Examples of Missed Details
Let’s examine some common examples of details that programmers often miss in problem statements:
Example 1: The “Return” Trap
Consider this problem statement:
Write a function that finds the maximum sum of a contiguous subarray within an array of integers. Return the sum.
Many programmers correctly implement Kadane’s algorithm but miss the subtle detail about what to return. Some might return the subarray itself rather than just the sum.
The correct implementation would be:
def max_subarray_sum(nums):
current_sum = max_sum = nums[0]
for num in nums[1:]:
current_sum = max(num, current_sum + num)
max_sum = max(max_sum, current_sum)
return max_sum # Return the sum, not the subarray
Example 2: The “Modification” Oversight
Consider this problem:
Given an array of integers, modify the array in-place such that all duplicates are removed and the remaining elements are shifted to the front of the array. Return the new length.
A common mistake is to create a new array for the result, missing the “in-place” requirement. The correct approach would modify the original array without using additional space (except for a few variables).
The correct implementation would be:
def remove_duplicates(nums):
if not nums:
return 0
# Position to place the next unique element
position = 1
for i in range(1, len(nums)):
if nums[i] != nums[i-1]:
nums[position] = nums[i]
position += 1
return position # Return the new length
Example 3: The “Constraint” Neglect
Consider this problem:
Given a sorted array of integers, find if a target value exists in the array. The array has been rotated at an unknown pivot point. The solution should have O(log n) time complexity.
Many programmers might miss the O(log n) time complexity constraint and implement a linear search. The correct approach would be to adapt the binary search algorithm to handle the rotated array.
The correct implementation would be:
def search_rotated(nums, target):
left, right = 0, len(nums) - 1
while left <= right:
mid = (left + right) // 2
if nums[mid] == target:
return mid
# Check if the left half is sorted
if nums[left] <= nums[mid]:
# Check if target is in the left half
if nums[left] <= target < nums[mid]:
right = mid - 1
else:
left = mid + 1
# Right half is sorted
else:
# Check if target is in the right half
if nums[mid] < target <= nums[right]:
left = mid + 1
else:
right = mid - 1
return -1 # Target not found
Methods to Practice Detail Orientation
Improving your attention to detail is a skill that can be developed with practice:
1. Deliberate Practice with Problem Dissection
Select a set of problems and focus solely on breaking down the requirements before attempting to solve them:
- List all explicit requirements
- Identify implicit requirements
- Note constraints and edge cases
- Create additional test cases
Only after this thorough analysis should you begin thinking about solutions.
2. Problem Rewriting Exercise
Take existing problem statements and rewrite them in your own words, making sure to capture all details. Then compare your version with the original to see if you missed anything.
3. Peer Review
Exchange problem analyses with a peer. Have them review your understanding of a problem statement and vice versa. This can reveal blind spots in your reading approach.
4. Post-Solution Analysis
After solving a problem, review the problem statement again and check if your solution addresses all requirements. If you missed something, analyze why and how you could have caught it earlier.
5. Detail-Oriented Games and Puzzles
Engage in activities that train attention to detail:
- Spot the difference puzzles
- Chess or Go (which require careful analysis)
- Mystery novels (which often hide crucial clues in plain sight)
- Logic puzzles that require tracking multiple conditions
Tools and Frameworks to Help Catch Missing Details
Several tools and frameworks can assist in ensuring you don’t miss important details:
1. Problem Analysis Templates
Create a template for analyzing problem statements that includes sections for:
- Problem objective
- Input specification
- Output specification
- Constraints
- Edge cases
- Example walkthrough
Fill this template for each problem before writing any code.
2. Checklists
Develop a personal checklist of common details to verify in problem statements:
- Input validation requirements
- Time and space complexity constraints
- Return type and format
- Edge cases to handle
- Modification permissions (in-place vs. returning new data)
3. Test-Driven Development (TDD)
While not directly related to reading problem statements, TDD forces you to think about requirements in detail before implementing a solution:
- Write tests based on your understanding of the problem
- Implement the solution to pass those tests
- Refine both tests and implementation as your understanding improves
4. Visual Mapping Tools
Use mind maps or concept maps to visualize the problem requirements and their relationships. Tools like XMind, MindMeister, or even simple drawing applications can help organize complex requirements visually.
5. Algorithmic Frameworks
Frameworks like UMPIRE (Understand, Match, Plan, Implement, Review, Evaluate) provide a structured approach to problem-solving that emphasizes thorough understanding before implementation.
The Understand phase specifically focuses on:
- Restating the problem
- Identifying inputs and outputs
- Working through examples
- Identifying edge cases
- Asking clarifying questions
Conclusion
Missing important details in problem statements is a common challenge that affects programmers at all skill levels. The consequences can range from minor frustrations during practice to significant setbacks in technical interviews or professional projects.
By understanding the psychological factors that contribute to this issue and implementing structured approaches to problem analysis, you can significantly improve your ability to catch critical details. Remember that this is a skill that improves with deliberate practice and conscious effort.
The next time you’re faced with a coding problem, resist the urge to dive immediately into implementation. Take the time to thoroughly understand the problem statement, identify all requirements and constraints, and analyze examples. This upfront investment will save you time and frustration in the long run and lead to more elegant and correct solutions.
Whether you’re using AlgoCademy to prepare for technical interviews, working on personal projects, or tackling professional challenges, improved attention to detail will serve you well throughout your programming career. It’s not just about coding skill; it’s about developing the mindset of a thoughtful, thorough, and detail-oriented software engineer.
Remember: The most elegant algorithm is worthless if it solves the wrong problem. Take the time to understand what you’re really being asked to do.