Why You Know Programming Concepts But Can’t Combine Them
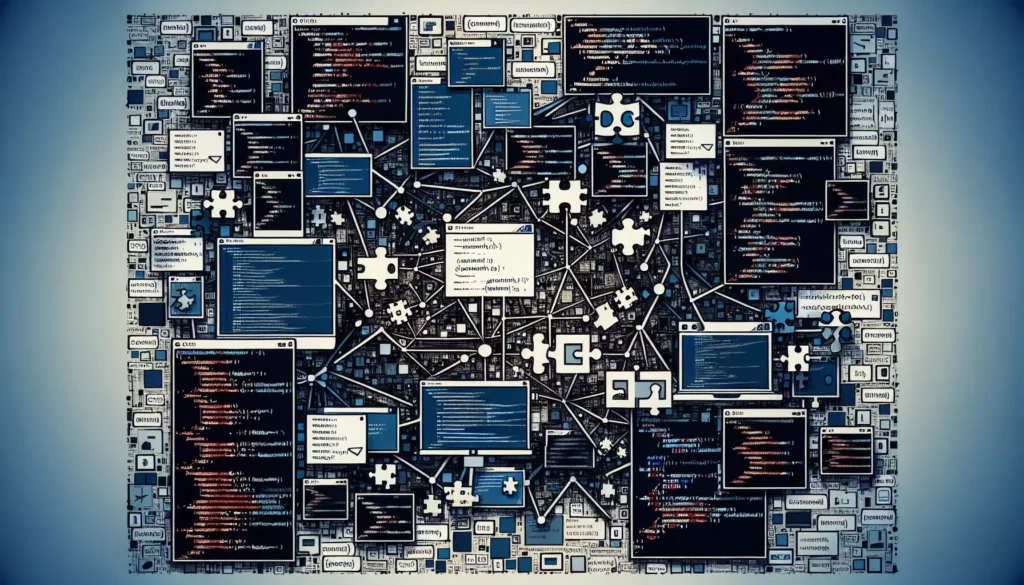
Have you ever found yourself in a situation where you understand individual programming concepts perfectly, but when it comes to building something from scratch, you freeze? You’re not alone. This phenomenon, which I call the “concept combination gap,” affects many programmers, from beginners to those with years of experience.
In this comprehensive guide, we’ll explore why understanding isolated programming concepts doesn’t automatically translate to the ability to combine them effectively. We’ll also provide actionable strategies to bridge this gap and help you become a more confident, creative programmer.
Table of Contents
- Understanding the Concept Combination Gap
- Why This Happens: The Cognitive Science Behind It
- Common Scenarios Where Programmers Get Stuck
- Bridging the Gap: Practical Strategies
- Developing Mental Models for Code Integration
- Effective Practice Techniques
- The Power of Project Based Learning
- Learning Design Patterns and Architectural Thinking
- The Underrated Skill of Reading Code
- Deliberate Practice for Programming Mastery
- Conclusion: From Knowledge to Application
Understanding the Concept Combination Gap
You’ve mastered variables, functions, loops, and conditionals. You understand object oriented programming principles and can explain recursion. Yet, when faced with a blank editor and a problem to solve, you struggle to piece these concepts together into a cohesive solution.
This disconnect between knowing programming concepts and being able to combine them effectively is what I call the “concept combination gap.” It’s the difference between theoretical knowledge and practical application, between understanding syntax and creating architecture.
Consider this analogy: knowing programming concepts without being able to combine them is like knowing all the ingredients for a cake without understanding how to mix them together in the right order and proportions. You might have flour, sugar, eggs, and butter, but without the recipe and baking skills, you won’t end up with a cake.
Why This Happens: The Cognitive Science Behind It
Understanding why this gap exists requires diving into how our brains learn and process information:
Chunking and Cognitive Load
Our working memory is limited. Cognitive scientists suggest we can only hold about 4-7 items in our working memory at once. When learning programming, each new concept occupies one of these slots. As a beginner, just understanding a for loop consumes significant cognitive resources.
Experienced programmers have “chunked” these basic concepts, meaning they’ve grouped related pieces of information together into single units. This frees up mental space to think about higher level problems and combinations.
Bottom Up vs. Top Down Learning
Most programming education follows a bottom up approach: learn variables, then functions, then objects, etc. This builds a foundation but doesn’t naturally lead to understanding how these elements combine in real world applications.
Top down learning, starting with complete applications and breaking them down, can provide context but might be overwhelming without foundational knowledge. The ideal learning path integrates both approaches, but most educational resources favor bottom up.
Transfer of Learning Challenges
Transfer of learning refers to applying knowledge from one context to another. Near transfer (applying knowledge in similar contexts) is easier than far transfer (applying in different contexts).
Programming education often involves learning concepts in isolation or in contrived examples, making it a far transfer challenge to apply them in real world problems with different constraints and requirements.
Common Scenarios Where Programmers Get Stuck
Let’s look at some common situations where programmers experience the concept combination gap:
The Blank Editor Syndrome
You understand how to write functions and use data structures, but when starting a new project, you freeze at the empty file. Where do you begin? How do you structure your code? These questions can be paralyzing.
Algorithm Implementation Struggles
You understand the theoretical steps of an algorithm (like binary search or quicksort), but translating that understanding into working code proves challenging.
For example, you might understand that binary search involves repeatedly dividing a sorted array in half, but implementing the proper indices and boundary conditions trips you up:
// You understand this conceptually
function binarySearch(array, target) {
// But where exactly do you start and end?
// How do you handle the middle calculation?
// What about edge cases?
}
Connecting Frontend to Backend
You might understand both frontend development (HTML, CSS, JavaScript) and backend concepts (databases, APIs), but connecting them together in a full stack application feels overwhelming.
Debug Paralysis
When your code doesn’t work as expected, you understand the individual components but struggle to trace the flow of data and execution to identify where things are going wrong.
Bridging the Gap: Practical Strategies
Now that we understand the problem, let’s explore strategies to overcome the concept combination gap:
Start with Scaffolding
Instead of facing the blank editor, use templates or starter code. Many frameworks provide command line tools that generate project structures:
// Using Create React App to scaffold a React project
npx create-react-app my-project
// Using Express Generator for Node.js applications
npx express-generator my-api
As you become more comfortable, you can gradually remove this scaffolding and build more from scratch.
Use the “Extend and Modify” Approach
Rather than building from nothing, start with working code and extend or modify it. This approach provides context and structure while still requiring you to combine concepts.
For example, if you’re learning React, start with a simple component:
function Counter() {
const [count, setCount] = React.useState(0);
return (
<div>
<p>Count: {count}</p>
<button onClick={() => setCount(count + 1)}>Increment</button>
</div>
);
}
Then extend it by adding features like a decrement button, reset functionality, or a maximum value. This incremental approach builds your combination skills gradually.
Follow the “Read, Run, Modify, Create” Progression
When learning a new concept or pattern:
- Read existing code that implements it
- Run the code to see how it works
- Modify parts of the code to understand how changes affect behavior
- Create your own implementation from scratch
This progression gradually increases the cognitive load and helps build your combination skills.
Developing Mental Models for Code Integration
Mental models are simplified representations of how something works. Developing strong mental models for how code components interact is crucial for bridging the concept combination gap.
The Building Blocks Model
Think of programming concepts as LEGO blocks. Individual blocks (functions, classes, loops) have specific shapes and purposes, but their value comes from how they connect.
For example, consider this function composition in JavaScript:
// Individual functions (blocks)
const double = x => x * 2;
const increment = x => x + 1;
// Combining them (stacking blocks)
const doubleAndIncrement = x => increment(double(x));
console.log(doubleAndIncrement(5)); // 11
The Data Flow Model
Visualize your program as a series of transformations that data flows through. Each function or method transforms the data in some way before passing it to the next stage.
This model is particularly helpful for understanding functional programming and pipeline patterns:
// Data flow through a pipeline of operations
const numbers = [1, 2, 3, 4, 5];
const result = numbers
.filter(n => n % 2 === 0) // Data flows through filter
.map(n => n * 10) // Then through map
.reduce((sum, n) => sum + n, 0); // Finally through reduce
console.log(result); // 60
The Conversation Model
For systems with multiple components (like client server applications), think of the interaction as a conversation with specific protocols and expectations.
// Client: "Can I have the user data for ID 123?"
fetch('/api/users/123')
.then(response => {
// Server: "Here's the data you requested"
return response.json();
})
.then(userData => {
// Client: "Thanks, I'll use this data now"
updateUserInterface(userData);
})
.catch(error => {
// Server: "Sorry, I couldn't fulfill that request"
handleError(error);
});
Effective Practice Techniques
Building your concept combination skills requires deliberate practice. Here are some effective techniques:
The Code Completion Exercise
Find partially complete code examples and challenge yourself to fill in the missing parts. This is less intimidating than starting from scratch and helps you understand how components should fit together.
// Complete this function to calculate the average of an array
function calculateAverage(numbers) {
// Your code here
// Hint: You'll need to sum the array and divide by its length
}
// Test cases
console.log(calculateAverage([1, 2, 3, 4, 5])); // Should return 3
console.log(calculateAverage([10, 20, 30])); // Should return 20
The Reverse Engineering Challenge
Take working code and break it down to understand how it works. Then try to rebuild it from memory. This helps you internalize patterns of how concepts combine.
For example, study this simple Express.js route handler:
app.get('/api/users/:id', async (req, res) => {
try {
const userId = req.params.id;
const user = await User.findById(userId);
if (!user) {
return res.status(404).json({ message: 'User not found' });
}
res.json(user);
} catch (error) {
res.status(500).json({ message: 'Server error', error: error.message });
}
});
Close the file, then try to recreate it, focusing on the pattern of request handling, async/await usage, error handling, and response sending.
The Incremental Complexity Exercise
Start with a simple implementation, then gradually add features and complexity. This builds your confidence and ability to combine concepts.
For instance, start with a basic to do list that just displays items:
// Stage 1: Basic display
function TodoList() {
const todos = ['Learn React', 'Build a project', 'Deploy to production'];
return (
<ul>
{todos.map(todo => <li key={todo}>{todo}</li>)}
</ul>
);
}
Then add the ability to add new items, then the ability to mark items as complete, then persistence with localStorage, and so on.
The Power of Project Based Learning
Project based learning is one of the most effective ways to bridge the concept combination gap. Working on complete projects forces you to integrate concepts in meaningful ways.
Start with Guided Projects
Begin with tutorials or courses that walk you through building complete applications. These provide the scaffolding needed to see how concepts fit together.
Good guided projects:
- Explain the “why” behind architectural decisions
- Show the evolution of code from simple to complex
- Discuss alternative approaches and their tradeoffs
- Include challenges that require you to apply what you’ve learned
Progress to Personal Projects
Once you’ve completed several guided projects, start building your own. Choose something that:
- You’re personally interested in (to maintain motivation)
- Has a clearly defined scope (to avoid overwhelm)
- Uses technologies you’re somewhat familiar with (to build confidence)
- Stretches your abilities in at least one area (to promote growth)
For example, if you’re comfortable with React basics, build a personal project that adds a new challenge like API integration or state management with Redux.
The Clone and Extend Method
Clone a simple existing application, then extend it with new features. This provides a working foundation while still requiring you to combine concepts.
For instance, clone a basic weather app, then add features like:
- Five day forecast
- Location detection
- Weather alerts
- Historical weather data visualization
Learning Design Patterns and Architectural Thinking
Design patterns are proven solutions to common programming problems. Learning these patterns helps you see how concepts should combine in different scenarios.
Start with Fundamental Patterns
Begin with basic patterns that appear frequently in code:
The Module Pattern
This pattern encapsulates functionality and exposes only what’s necessary:
// Basic module pattern in JavaScript
const counterModule = (function() {
let count = 0; // Private variable
return {
increment: function() {
count++;
return count;
},
decrement: function() {
count--;
return count;
},
getCount: function() {
return count;
}
};
})();
console.log(counterModule.getCount()); // 0
counterModule.increment();
console.log(counterModule.getCount()); // 1
The Observer Pattern
This pattern establishes a subscription mechanism between objects:
class EventEmitter {
constructor() {
this.events = {};
}
on(event, listener) {
if (!this.events[event]) {
this.events[event] = [];
}
this.events[event].push(listener);
}
emit(event, ...args) {
if (this.events[event]) {
this.events[event].forEach(listener => listener(...args));
}
}
}
// Usage
const emitter = new EventEmitter();
emitter.on('userCreated', user => console.log(`New user: ${user.name}`));
emitter.emit('userCreated', { name: 'John Doe' });
Study Architectural Patterns
As you advance, learn higher level architectural patterns that govern how applications are structured:
- MVC (Model View Controller): Separates data, user interface, and control logic
- MVVM (Model View ViewModel): Used in frameworks like Vue.js and Angular
- Flux/Redux: Unidirectional data flow patterns popular in React applications
- Microservices: Breaking applications into small, specialized services
Understanding these patterns helps you organize your thinking about how components should interact in larger applications.
The Underrated Skill of Reading Code
One of the most effective ways to learn how to combine programming concepts is to read high quality code written by experienced developers.
Study Open Source Projects
Open source projects provide real world examples of how concepts combine in production code:
- Start with smaller, well documented projects
- Focus on understanding the project structure before diving into specific files
- Pay attention to how different modules interact
- Study the commit history to see how the code evolved
Projects like Lodash (for utility functions), Express (for Node.js web applications), or Todo MVC implementations are good starting points.
Read Code with Intent
When reading code, ask specific questions:
- How does this code handle errors?
- How are concerns separated?
- How does data flow through the application?
- What design patterns are being used?
- How is the code tested?
This focused approach helps you extract patterns and principles that you can apply in your own projects.
Practice Code Reviews
Reviewing others’ code (or having your code reviewed) is an excellent way to develop your ability to see how concepts should combine:
- Participate in open source projects by reviewing pull requests
- Join coding communities where code reviews are encouraged
- Use platforms like GitHub to share your code and request feedback
Code reviews expose you to different approaches and help you develop a critical eye for code quality and organization.
Deliberate Practice for Programming Mastery
To truly bridge the concept combination gap, you need deliberate practice focused on integration skills:
The IOCE Framework
Use the Input Output Concept Extension (IOCE) framework for practice:
- Input: Define the starting point (requirements, existing code)
- Output: Define the desired result (functionality, performance)
- Concept: Identify the main programming concepts needed
- Extension: Add complexity or constraints to stretch your abilities
For example:
- Input: Create a function that takes an array of numbers
- Output: Return the sum of all even numbers
- Concept: Array methods, filtering, reduction
- Extension: Implement it using only reduce (no filter)
// Basic implementation
function sumEvenNumbers(numbers) {
return numbers
.filter(num => num % 2 === 0)
.reduce((sum, num) => sum + num, 0);
}
// Extension: Using only reduce
function sumEvenNumbersReduceOnly(numbers) {
return numbers.reduce((sum, num) => {
return num % 2 === 0 ? sum + num : sum;
}, 0);
}
Time Boxed Challenges
Set a timer and challenge yourself to build something within that timeframe. This forces you to make decisions about which concepts to prioritize and how to combine them efficiently.
For example, give yourself 30 minutes to build a basic API endpoint that:
- Accepts user data via POST
- Validates the input
- Stores it in memory
- Returns appropriate status codes
Spaced Repetition for Concept Integration
Use spaced repetition to reinforce your ability to combine concepts:
- Create a list of integration patterns you want to master (e.g., connecting a frontend form to an API)
- Practice implementing these patterns at increasing intervals
- Each time, try to implement from memory before checking references
- Vary the context to ensure flexible understanding
This approach helps build lasting neural pathways for concept combination.
Conclusion: From Knowledge to Application
The gap between understanding programming concepts and being able to combine them effectively is real, but it can be bridged with the right approach.
Remember these key points:
- The concept combination gap is a normal part of the learning process, not a sign of failure
- Building mental models helps you understand how concepts should fit together
- Scaffolded learning provides structure while you develop combination skills
- Project based learning forces you to integrate concepts in meaningful ways
- Reading code exposes you to proven patterns of concept combination
- Deliberate practice focused on integration accelerates your progress
As you apply these strategies, you’ll find yourself gradually closing the gap between knowing programming concepts and being able to combine them fluently. The blank editor will become less intimidating, and you’ll develop the confidence to tackle new programming challenges with creativity and skill.
Remember, every expert programmer once struggled with this same gap. What separates successful developers isn’t innate talent, but persistence in developing the crucial skill of concept combination. With deliberate practice and the right learning strategies, you can transform from someone who knows programming concepts to someone who can build with them.
Happy coding, and embrace the journey from knowledge to application!