Why You Forget Programming Concepts You Don’t Use Regularly
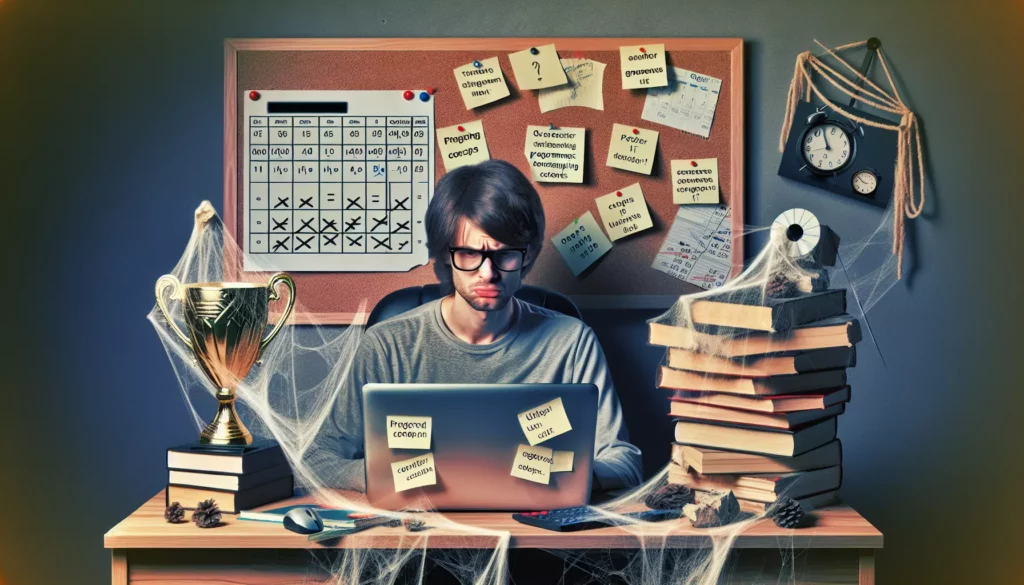
Every programmer has experienced that frustrating moment. You’re working on a project and need to implement a concept you learned months ago—maybe a specific algorithm or design pattern—but when you try to recall it, your mind goes blank. Despite having once understood it well, the details have somehow slipped away.
This phenomenon isn’t just common among novice programmers; even seasoned developers regularly experience the “use it or lose it” effect with programming knowledge. But why does this happen? And more importantly, what can we do about it?
In this article, we’ll explore the cognitive science behind why we forget programming concepts, identify which types of knowledge are most susceptible to forgetting, and provide practical strategies to maintain your programming knowledge over time.
The Science Behind Forgetting Programming Knowledge
To understand why we forget programming concepts, we first need to understand how memory works. Our brains don’t store information like computers do—memory is a complex, dynamic process influenced by numerous factors.
The Forgetting Curve
In the late 19th century, psychologist Hermann Ebbinghaus discovered what he called the “forgetting curve”—a mathematical formula that describes how information is lost over time when there is no attempt to retain it. His research showed that memory retention decreases at an exponential rate: we lose most information within days or weeks of learning it, with the sharpest decline occurring in the first few days.
For programmers, this means that unless you actively use or review a concept after learning it, you’ll likely forget most of the details within weeks—regardless of how well you initially understood it.
Types of Memory in Programming
When we code, we rely on different types of memory:
- Declarative memory: Facts and concepts, like syntax rules or how specific algorithms work
- Procedural memory: How to perform tasks, like debugging or implementing patterns
- Working memory: Temporarily holding and manipulating information while solving problems
Interestingly, these different types of memory decay at different rates. Procedural memory (the “how-to” knowledge) tends to be more resistant to forgetting than declarative memory (facts and concepts). This explains why you might forget the exact syntax of a rarely-used language feature, but still remember the general approach to implementing it.
The Role of Neural Pathways
Learning programming concepts creates neural pathways in your brain. When you regularly use these pathways, they strengthen—making recall easier and faster. When you don’t use them, these pathways weaken over time.
Think of it like a trail through a forest: a frequently traveled path remains clear and easy to follow, while an unused trail gradually becomes overgrown and difficult to navigate. Similarly, programming concepts you use daily remain accessible, while those you learned but never applied become increasingly difficult to recall.
Which Programming Knowledge Is Most Susceptible to Forgetting?
Not all programming knowledge is equally vulnerable to being forgotten. Understanding which types of knowledge fade fastest can help you focus your retention efforts more effectively.
Syntax and Language-Specific Details
The most volatile knowledge tends to be syntax and language-specific details. These are the elements that vary between programming languages and have little conceptual foundation to anchor them in memory:
- Method names and parameters
- Library-specific function calls
- Language-specific operators and symbols
- Configuration options and flags
For example, you might forget whether Python’s string split method returns a list or an iterator, or whether JavaScript’s Array.indexOf returns -1 or null when an element isn’t found.
Rarely Used Algorithms and Data Structures
Complex algorithms and data structures that you don’t regularly implement are also highly susceptible to forgetting:
- Red-black trees and AVL trees
- Dynamic programming techniques
- Advanced graph algorithms like Dijkstra’s or A*
- Specialized sorting algorithms (beyond the basics)
You might have thoroughly understood how to implement a balanced binary search tree during your computer science coursework, but if you haven’t used one since, you’ll likely struggle to implement it from memory years later.
Framework-Specific Knowledge
Knowledge tied to specific frameworks or libraries tends to fade quickly, especially given how rapidly they evolve:
- Configuration patterns
- Component lifecycles
- Framework-specific hooks or callbacks
- Middleware patterns
A React developer who switches to Vue.js for a year might struggle to remember React’s component lifecycle methods when returning to a React project.
What Tends to Persist Longer
On the other hand, some programming knowledge shows greater resistance to forgetting:
- Fundamental concepts: Basic principles like variables, loops, and functions
- Problem-solving approaches: General strategies for breaking down problems
- Design patterns: High-level architectural patterns that appear across languages
- Debugging techniques: General approaches to finding and fixing bugs
These elements persist because they’re conceptual rather than detail-oriented, they transfer between languages and environments, and they’re often reinforced through regular use even when specific implementations vary.
Why Programmers Forget: Beyond Basic Memory Science
While the forgetting curve explains the basic mechanism of memory decay, several factors specific to programming contribute to knowledge loss.
The Rapid Evolution of Technology
Programming knowledge becomes outdated faster than knowledge in many other fields. New languages, frameworks, and best practices emerge constantly, making it challenging to maintain expertise across multiple domains.
When you switch from one technology stack to another, you’re not just at risk of forgetting the old stack—you’re also dealing with the fact that it might have evolved significantly by the time you return to it. The Angular developer who worked with version 2 three years ago will find that Angular 12 has introduced many changes, making their previous knowledge partially obsolete.
Context-Dependent Memory
Research shows that memory recall is stronger when you’re in the same environment where you learned the information. This “context-dependent memory” affects programmers who switch between different:
- Programming languages
- IDEs or development environments
- Problem domains
- Team structures and codebases
When you move from one context to another, your brain has fewer environmental cues to help trigger relevant memories. This explains why you might struggle to recall a JavaScript pattern immediately after working extensively in Python, even if you once knew it well.
Shallow vs. Deep Learning
Programming concepts learned superficially are forgotten much more quickly than those learned deeply. Unfortunately, the pressure to learn quickly in the fast-paced tech industry often leads to shallow learning:
- Tutorial-hopping without building projects
- Copy-pasting solutions without understanding them
- Learning just enough to implement a feature without grasping underlying principles
This shallow learning creates fragile knowledge structures that quickly deteriorate when not reinforced through practice.
Cognitive Load and Information Overload
Programmers face an exceptionally high cognitive load. The sheer volume of information—from language syntax to framework peculiarities to business domain knowledge—can overwhelm working memory.
When cognitive resources are stretched thin, the brain becomes less efficient at encoding new information into long-term memory. This means that even as you’re learning new concepts, you may be losing others simply because your brain is prioritizing what seems most immediately relevant.
The Real-World Impact of Forgetting Programming Knowledge
Forgetting programming concepts isn’t just an academic concern—it has tangible effects on productivity, career development, and psychological well-being.
Productivity Costs
When you forget a programming concept you need for your current task, your workflow is disrupted. You must pause to:
- Search for documentation or tutorials
- Review old code examples
- Experiment with different approaches
- Consult with colleagues or online communities
This context-switching is costly. Research suggests that it can take up to 23 minutes to fully regain focus after an interruption. For complex programming tasks, the productivity loss can be substantial.
Career Implications
Knowledge retention affects career mobility and job performance:
- Technical interviews: Forgotten algorithms and data structures can make it difficult to pass interviews, even for positions you’re otherwise qualified for
- Project assignments: Teams may hesitate to assign you to projects using technologies you haven’t worked with recently
- Technology transitions: Returning to a previously known technology stack can involve a steep re-learning curve
In a field where versatility is valuable, the ability to maintain knowledge across multiple domains provides a significant competitive advantage.
Psychological Effects
Repeatedly forgetting concepts you once knew can trigger negative psychological responses:
- Impostor syndrome: “If I were a real programmer, I wouldn’t keep forgetting this”
- Anxiety about technical discussions or code reviews
- Reduced confidence in taking on new challenges
- Frustration with perceived limitations of memory
These psychological effects can create a negative feedback loop where anxiety about forgetting actually impairs learning and retention of new concepts.
Strategies to Combat Programming Knowledge Loss
Now that we understand why we forget programming knowledge, let’s explore effective strategies to combat this natural process.
Spaced Repetition: The Science of Optimal Review
Spaced repetition is a learning technique that incorporates increasing intervals of time between reviews of previously learned material. It’s based on the psychological spacing effect, which demonstrates that we learn more effectively when we spread out our learning over time.
For programmers, this might look like:
- Using spaced repetition software (like Anki) to review programming concepts
- Creating flashcards for syntax, algorithms, or design patterns
- Scheduling deliberate reviews of concepts at increasing intervals
The key insight of spaced repetition is that reviewing information just as you’re about to forget it strengthens memory most efficiently. Modern spaced repetition systems use algorithms to predict when you’re likely to forget information and schedule reviews accordingly.
Deliberate Practice and Project-Based Learning
Knowledge applied in practical contexts is much more resistant to forgetting than knowledge learned in isolation. To leverage this:
- Build side projects that incorporate concepts you want to retain
- Participate in coding challenges that use specific algorithms or data structures
- Contribute to open source projects in languages or frameworks you want to maintain proficiency in
- Create implementations of theoretical concepts (e.g., build a red-black tree from scratch)
The key is to move beyond passive review to active application. When you implement a concept in a real project, you encode the knowledge more deeply and create multiple pathways for recall.
Conceptual Understanding vs. Memorization
Focusing on deeper conceptual understanding rather than rote memorization creates more durable knowledge:
- Learn the “why” behind programming patterns and practices
- Study the underlying principles that connect different programming languages
- Look for analogies and mental models that make concepts more intuitive
- Explain concepts to others (through blogging, teaching, or mentoring)
When you understand the rationale behind a concept, you can often reconstruct the details even if you’ve forgotten them. This is why experienced programmers can quickly become productive in new languages—they understand the underlying patterns and principles.
Creating External Knowledge Systems
Instead of relying solely on memory, develop external systems to store and retrieve knowledge:
- Maintain a personal knowledge base or digital garden
- Document solutions to problems you solve
- Create code snippets libraries for frequently used patterns
- Develop cheat sheets for languages or frameworks you use occasionally
Tools like Notion, Obsidian, or even a well-organized GitHub repository can serve as extensions of your memory, allowing you to offload the burden of remembering every detail.
For example, instead of trying to memorize the exact syntax for a complex regular expression, document it with examples and explanations that help you quickly relearn it when needed.
Interleaved Practice
Rather than focusing on one programming concept at a time, interleaved practice involves mixing different topics during learning sessions:
- Alternate between different algorithms when practicing
- Switch between languages during review sessions
- Mix related but distinct concepts (e.g., different sorting algorithms)
Research shows that while interleaved practice feels more difficult in the moment, it leads to stronger long-term retention and better transfer of knowledge to new situations. The increased challenge forces your brain to form stronger connections between concepts.
Practical Examples: Remembering Different Types of Programming Knowledge
Different types of programming knowledge require different retention strategies. Let’s look at specific approaches for common categories.
Retaining Algorithm Knowledge
Algorithms are particularly susceptible to forgetting because they often involve complex sequences of steps that must be implemented precisely.
Effective retention strategies:
- Implement from scratch periodically: Set a calendar reminder to implement key algorithms from memory every few months
- Use visualizations: Associate algorithms with visual representations that make the steps more intuitive
- Create algorithm templates: Develop templates with the core structure that you can reference when needed
Example approach for remembering quicksort:
- Understand the high-level concept: partition around a pivot, recursively sort sub-arrays
- Visualize the process using a diagram or animation
- Implement it from scratch periodically (e.g., once every 3 months)
- Compare your implementation to a reference solution to identify misconceptions
Maintaining Language Syntax
Language syntax is often the first thing to fade when you switch between programming languages.
Effective retention strategies:
- Create syntax cheat sheets: Develop personalized reference guides for languages you use occasionally
- Practice with syntax-focused exercises: Use platforms like Exercism or CodeWars that emphasize language-specific features
- Highlight syntax differences: Create comparison tables between languages you frequently switch between
Example for maintaining Python and JavaScript syntax:
// JavaScript Array Methods vs Python List Methods
// JavaScript:
array.push(item); // Add to end
array.pop(); // Remove from end
array.unshift(item); // Add to beginning
array.shift(); // Remove from beginning
# Python:
my_list.append(item) # Add to end
my_list.pop() # Remove from end
my_list.insert(0, item) # Add to beginning
my_list.pop(0) # Remove from beginning
Preserving Framework Knowledge
Framework knowledge is particularly challenging to maintain given how quickly frameworks evolve.
Effective retention strategies:
- Build template projects: Create minimal implementations that demonstrate core framework patterns
- Follow release notes: Stay aware of changes even when not actively using a framework
- Maintain a “hello world plus”: A slightly more complex than minimal example that showcases key features
Example for React knowledge retention:
- Create a template React project that demonstrates hooks, context, and component patterns
- Add comments explaining key concepts and implementation details
- Review and update the template when major React versions are released
- When returning to React after a break, use the template as a refresher before diving into complex projects
The Role of AI Tools in Knowledge Retention
Modern AI tools like GitHub Copilot, ChatGPT, and language-specific assistants are changing how programmers approach knowledge retention.
AI as Memory Augmentation
AI tools can serve as extensions of your memory, providing just-in-time information when needed:
- Suggesting syntax and function signatures as you type
- Generating boilerplate code for patterns you vaguely remember
- Explaining unfamiliar code or concepts on demand
This raises an interesting question: If AI can reliably provide information when needed, how much programming knowledge do we actually need to memorize?
The New Balance: What to Remember vs. What to Outsource
With AI assistance, the balance is shifting toward:
- Remember: Conceptual understanding, problem-solving approaches, architectural patterns, and when/how to apply specific solutions
- Outsource: Exact syntax, boilerplate code, standard implementations of common algorithms, and configuration details
This doesn’t mean abandoning knowledge retention entirely—rather, it suggests focusing retention efforts on higher-level concepts while leveraging AI for implementation details.
Potential Pitfalls of Over-Reliance on AI
However, there are risks to becoming too dependent on AI tools:
- Reduced depth of understanding if you never struggle through implementations
- Difficulty identifying incorrect or suboptimal AI suggestions
- Loss of problem-solving skills that develop through working through challenges
- Decreased ability to work in environments where AI tools aren’t available
The most effective approach is likely a balanced one: use AI tools to augment your capabilities while still investing in understanding core concepts deeply enough to evaluate and adapt AI suggestions.
A Holistic Approach to Programming Knowledge Management
Beyond specific techniques, managing programming knowledge effectively requires a holistic approach that considers your career goals, learning style, and work environment.
Aligning Knowledge Retention with Career Goals
Not all programming knowledge is equally valuable to retain. Consider your career trajectory:
- Specialist path: Focus deep retention on your core technology stack, with shallower knowledge of adjacent technologies
- Generalist path: Maintain broader but less detailed knowledge across multiple domains, with systems to quickly refresh specific details
- Leadership path: Prioritize architectural patterns and principles over implementation details
By aligning retention efforts with career goals, you can use your limited cognitive resources more efficiently.
Creating Learning Loops in Your Development Workflow
Integrate knowledge reinforcement into your regular workflow:
- Schedule regular code reviews of unfamiliar parts of your codebase
- Rotate responsibilities within your team to maintain breadth of knowledge
- Add documentation as part of your definition of done for each feature
- Set aside time for deliberate learning and review during each sprint
These habits create natural spaced repetition without requiring separate study sessions.
Building a Personal Knowledge Management System
Develop a systematic approach to capturing and organizing what you learn:
- Choose tools that fit your workflow (e.g., note-taking apps, knowledge bases)
- Establish consistent patterns for documenting new knowledge
- Create retrieval paths that match how you think about problems
- Schedule regular maintenance of your knowledge system
The goal is to create a system that’s low-friction enough to use consistently but structured enough to be useful when you need to retrieve information.
Embracing the Forgetting Curve: A Realistic Perspective
While we’ve explored many strategies to combat forgetting, it’s important to acknowledge that some forgetting is inevitable and even beneficial.
The Adaptive Value of Forgetting
Forgetting isn’t just a failure of memory—it’s an adaptive feature that helps us manage cognitive resources:
- It reduces interference between similar concepts
- It allows us to update knowledge when things change
- It helps us focus on what’s currently relevant
- It creates space for new learning
In the rapidly evolving field of programming, some forgetting is necessary to adapt to new paradigms and approaches.
From Anxiety to Acceptance: Changing Your Relationship with Forgetting
Many programmers experience anxiety or shame about forgetting concepts they once knew. Shifting this perspective can be liberating:
- Recognize that forgetting is universal—even senior developers regularly look things up
- Focus on retrieval skills rather than perfect recall
- View relearning as an opportunity to deepen understanding
- Measure your capabilities by what you can accomplish, not what you can remember
This mindset shift reduces the psychological burden of forgetting and creates a healthier relationship with the ongoing learning process that defines a programming career.
Conclusion: The Sustainable Programmer
Programming knowledge naturally decays over time due to the forgetting curve, context switching, and the rapid evolution of technology. This isn’t a personal failing but a fundamental aspect of how human memory works, especially in a field as vast and dynamic as programming.
Rather than fighting against this reality, the sustainable approach is to develop systems and habits that work with your brain’s natural tendencies:
- Use spaced repetition to maintain high-priority knowledge
- Build projects that apply concepts you want to retain
- Focus on deep conceptual understanding over memorization
- Develop external knowledge systems to complement your memory
- Leverage AI tools strategically while maintaining core competencies
By accepting that forgetting is part of the process and implementing these strategies, you can develop a more sustainable relationship with programming knowledge—one that acknowledges limitations while maximizing your effectiveness as a developer.
Remember, the goal isn’t perfect recall of every programming concept you’ve ever encountered. It’s developing the ability to solve problems effectively, which comes from a combination of what you know, what you can quickly relearn, and how you leverage external resources—including both documentation and modern AI tools.
In the end, the most valuable skill isn’t memorization but knowing how to learn, relearn, and apply knowledge in a field that will continue to evolve throughout your entire career.